# Objective
- Implementing reflection for Cow<'static, [T]>
- Hopefully fixes#7429
## Solution
- Implementing Reflect, Typed, GetTypeRegistration, and FromReflect for
Cow<'static, [T]>
---
## Notes
I have not used bevy_reflection much yet, so I may not fully understand
all the use cases. This is also my first attempt at contributing, so I
would appreciate any feedback or recommendations for changes. I tried to
add cases for using Cow<'static, str> and Cow<'static, [u8]> to some of
the bevy_reflect tests, but I can't guarantee those tests are
comprehensive enough.
---------
Co-authored-by: MinerSebas <66798382+MinerSebas@users.noreply.github.com>
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
Repetitively fetching ReflectResource and ReflectComponent from the
TypeRegistry is costly.
We want to access the underlying `fn`s. to do so, we expose the
`ReflectResourceFns` and `ReflectComponentFns` stored in ReflectResource
and ReflectComponent.
---
## Changelog
- Add the `fn_pointers` methods to `ReflectResource` and
`ReflectComponent` returning the underlying `ReflectResourceFns` and
`ReflectComponentFns`
# Objective
Make the UI code more concise.
## Solution
Add two utility methods to make manipulating `UiRect` from code more
concise:
- `UiRect::px()` create a new `UiRect` like the `new()` function, but
with values in logical pixels directly.
- `UiRect::percent()` is similar, with values as percentages.
This saves a lot of typing and makes UI code more compact while
retaining readability.
---
## Changelog
### Added
Added two new constructors `UiRect::px()` and `UiRect::percent()` to
create a new `UiRect` from values directly specified in logical pixels
and percentages, respectively. The argument order is the same as
`UiRect::new()`, but avoids having to repeat `Val::Px` and
`Val::Percent`, respectively.
# Objective
- Fixes#7811
## Solution
- I added `Has<T>` (and `HasFetch<T>` ) and implemented `WorldQuery`,
`ReadonlyWorldQuery`, and `ArchetypeFilter` it
- I also added documentation with an example and a unit test
I believe I've done everything right but this is my first contribution
and I'm not an ECS expert so someone who is should probably check my
implementation. I based it on what `Or<With<T>,>`, would do. The only
difference is that `Has` does not update component access - adding `Has`
to a query should never affect whether or not it is disjoint with
another query *I think*.
---
## Changelog
## Added
- Added `Has<T>` WorldQuery to find out whether or not an entity has a
particular component.
---------
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
Co-authored-by: JoJoJet <21144246+JoJoJet@users.noreply.github.com>
# Objective
We can currently set `camera.target` to either an `Image` or `Window`.
For OpenXR & WebXR we need to be able to render to a `TextureView`.
This partially addresses #115 as with the addition we can create
internal and external xr crates.
## Solution
A `TextureView` item is added to the `RenderTarget` enum. It holds an id
which is looked up by a `ManualTextureViews` resource, much like how
`Assets<Image>` works.
I believe this approach was first used by @kcking in their [xr
fork](eb39afd51b/crates/bevy_render/src/camera/camera.rs (L322)).
The only change is that a `u32` is used to index the textures as
`FromReflect` does not support `uuid` and I don't know how to implement
that.
---
## Changelog
### Added
Render: Added `RenderTarget::TextureView` as a `camera.target` option,
enabling rendering directly to a `TextureView`.
## Migration Guide
References to the `RenderTarget` enum will need to handle the additional
field, ie in `match` statements.
---
## Comments
- The [wgpu
work](c039a74884)
done by @expenses allows us to create framebuffer texture views from
`wgpu v0.15, bevy 0.10`.
- I got the WebXR techniques from the [xr
fork](https://github.com/dekuraan/xr-bevy) by @dekuraan.
- I have tested this with a wip [external webxr
crate](018e22bb06/crates/bevy_webxr/src/bevy_utils/xr_render.rs (L50))
on an Oculus Quest 2.
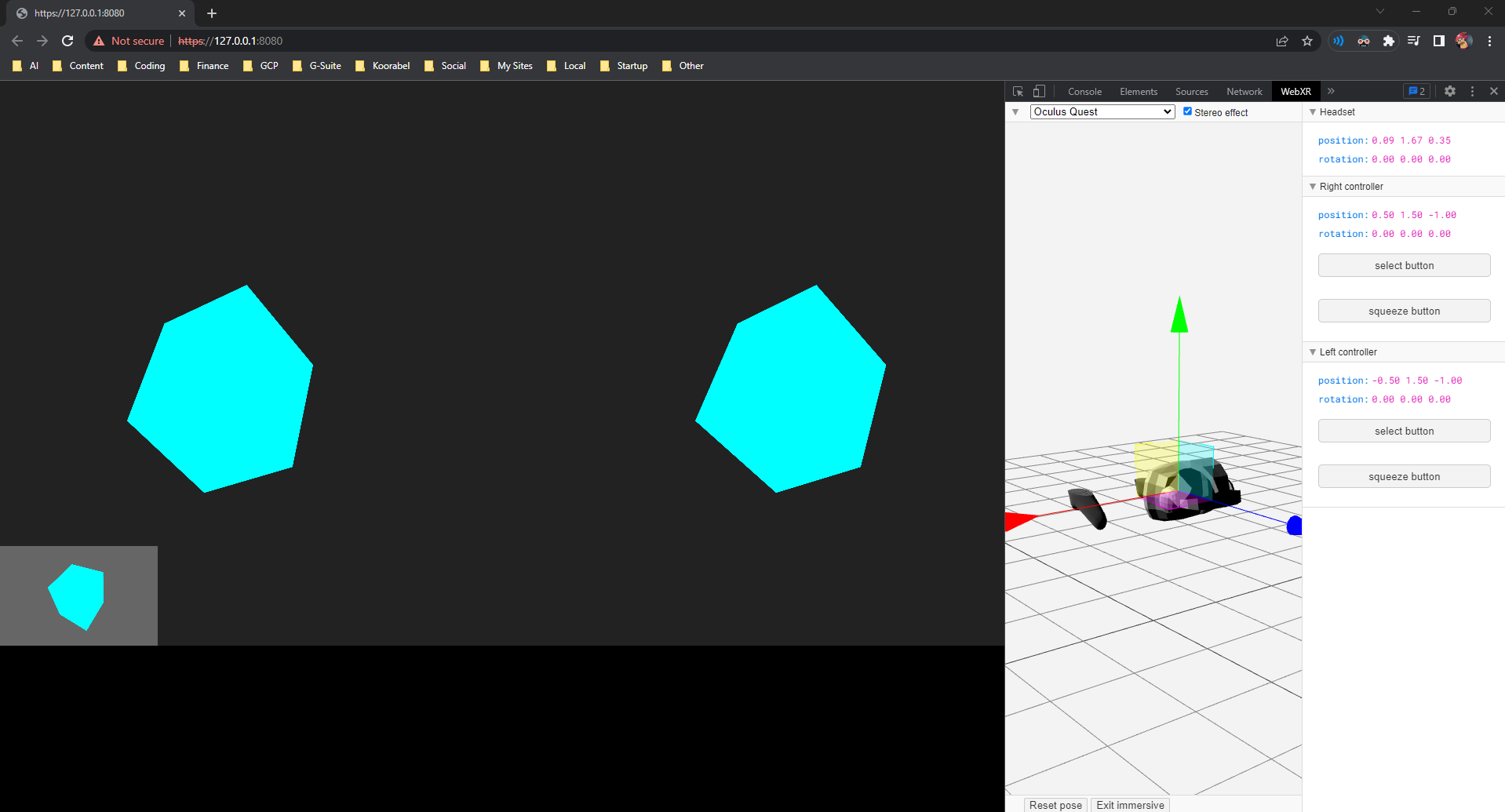
---------
Co-authored-by: Carter Anderson <mcanders1@gmail.com>
Co-authored-by: Paul Hansen <mail@paul.rs>
# Objective
- Cleanup the `reflect.rs` file in `bevy_ecs`, it's very large and can
get difficult to navigate
## Solution
- Split the file into 3 modules, re-export the types in the
`reflect/mod.rs` to keep a perfectly identical API.
- Add **internal** architecture doc explaining how `ReflectComponent`
works. Note that this doc is internal only, since `component.rs` is not
exposed publicly.
### Tips to reviewers
To review this change properly, you need to compare it to the previous
version of `reflect.rs`. The diff from this PR does not help at all!
What you will need to do is compare `reflect.rs` individually with each
newly created file.
Here is how I did it:
- Adding my fork as remote `git remote add nicopap
https://github.com/nicopap/bevy.git`
- Checkout out the branch `git checkout nicopap/split_ecs_reflect`
- Checkout the old `reflect.rs` by running `git checkout HEAD~1 --
crates/bevy_ecs/src/reflect.rs`
- Compare the old with the new with `git diff --no-index
crates/bevy_ecs/src/reflect.rs crates/bevy_ecs/src/reflect/component.rs`
You could also concatenate everything into a single file and compare
against it:
- `cat
crates/bevy_ecs/src/reflect/{component,resource,map_entities,mod}.rs >
new_reflect.rs`
- `git diff --no-index crates/bevy_ecs/src/reflect.rs new_reflect.rs`
# Objective
Fix https://github.com/bevyengine/bevy/issues/1018 (Textures on the
`Plane` shape appear flipped).
This bug have been around for a very long time apparently, I tested it
was still there (see test code bellow) and sure enough, this image:

... is flipped vertically when used as a texture on a plane (in main,
0.10.1 and 0.9):

I'm pretty confused because this bug is so easy to fix, it has been
around for so long, it is easy to encounter, and PRs touching this code
still didn't fix it: https://github.com/bevyengine/bevy/pull/7546 To the
point where I'm wondering if it's actually intended. If it is, please
explain why and this PR can be changed to "mention that in the doc".
## Solution
Fix the UV mapping on the Plane shape
Here is how it looks after the PR

## Test code
```rust
use bevy::{
prelude::*,
};
fn main () {
App::new()
.add_plugins(DefaultPlugins)
.add_startup_system(setup)
.run();
}
fn setup(
mut commands: Commands,
assets: ResMut<AssetServer>,
mut meshes: ResMut<Assets<Mesh>>,
mut materials: ResMut<Assets<StandardMaterial>>,
) {
commands.spawn(Camera3dBundle {
transform: Transform::from_xyz(0., 3., 0.).looking_at(Vec3::ZERO, Vec3::NEG_Z),
..default()
});
let mesh = meshes.add(Mesh::from(shape::Plane::default()));
let texture_image = assets.load("test.png");
let material = materials.add(StandardMaterial {
base_color_texture: Some(texture_image),
..default()
});
commands.spawn(PbrBundle {
mesh,
material,
..default()
});
}
```
## Changelog
Fix textures on `Plane` shapes being flipped vertically.
## Migration Guide
Flip the textures you use on `Plane` shapes.
# Objective
Resolves#7558.
Systems that are known to never modify the world implement the trait
`ReadOnlySystem`. This is a perfect place to add a safe API for running
a system with a shared reference to a World.
---
## Changelog
- Added the trait method `ReadOnlySystem::run_readonly`, which allows a
system to be run using `&World`.
# Objective
- The function `QueryParIter::for_each_unchecked` is a footgun: the only
ways to use it soundly can be done in safe code using `for_each` or
`for_each_mut`. See [this discussion on
discord](https://discord.com/channels/691052431525675048/749335865876021248/1118642977275924583).
## Solution
- Make `for_each_unchecked` private.
---
## Changelog
- Removed `QueryParIter::for_each_unchecked`. All use-cases of this
method were either unsound or doable in safe code using `for_each` or
`for_each_mut`.
## Migration Guide
The method `QueryParIter::for_each_unchecked` has been removed -- use
`for_each` or `for_each_mut` instead. If your use case can not be
achieved using either of these, then your code was likely unsound.
If you have a use-case for `for_each_unchecked` that you believe is
sound, please [open an
issue](https://github.com/bevyengine/bevy/issues/new/choose).
# Objective
`ComponentIdFor` is a type that gives you access to a component's
`ComponentId` in a system. It is currently awkward to use, since it must
be wrapped in a `Local<>` to be used.
## Solution
Make `ComponentIdFor` a proper SystemParam.
---
## Changelog
- Refactored the type `ComponentIdFor` in order to simplify how it is
used.
## Migration Guide
The type `ComponentIdFor<T>` now implements `SystemParam` instead of
`FromWorld` -- this means it should be used as the parameter for a
system directly instead of being used in a `Local`.
```rust
// Before:
fn my_system(
component_id: Local<ComponentIdFor<MyComponent>>,
) {
let component_id = **component_id;
}
// After:
fn my_system(
component_id: ComponentIdFor<MyComponent>,
) {
let component_id = component_id.get();
}
```
# Objective
Follow-up to #6404 and #8292.
Mutating the world through a shared reference is surprising, and it
makes the meaning of `&World` unclear: sometimes it gives read-only
access to the entire world, and sometimes it gives interior mutable
access to only part of it.
This is an up-to-date version of #6972.
## Solution
Use `UnsafeWorldCell` for all interior mutability. Now, `&World`
*always* gives you read-only access to the entire world.
---
## Changelog
TODO - do we still care about changelogs?
## Migration Guide
Mutating any world data using `&World` is now considered unsound -- the
type `UnsafeWorldCell` must be used to achieve interior mutability. The
following methods now accept `UnsafeWorldCell` instead of `&World`:
- `QueryState`: `get_unchecked`, `iter_unchecked`,
`iter_combinations_unchecked`, `for_each_unchecked`,
`get_single_unchecked`, `get_single_unchecked_manual`.
- `SystemState`: `get_unchecked_manual`
```rust
let mut world = World::new();
let mut query = world.query::<&mut T>();
// Before:
let t1 = query.get_unchecked(&world, entity_1);
let t2 = query.get_unchecked(&world, entity_2);
// After:
let world_cell = world.as_unsafe_world_cell();
let t1 = query.get_unchecked(world_cell, entity_1);
let t2 = query.get_unchecked(world_cell, entity_2);
```
The methods `QueryState::validate_world` and
`SystemState::matches_world` now take a `WorldId` instead of `&World`:
```rust
// Before:
query_state.validate_world(&world);
// After:
query_state.validate_world(world.id());
```
The methods `QueryState::update_archetypes` and
`SystemState::update_archetypes` now take `UnsafeWorldCell` instead of
`&World`:
```rust
// Before:
query_state.update_archetypes(&world);
// After:
query_state.update_archetypes(world.as_unsafe_world_cell_readonly());
```
# Objective
Implement borders for UI nodes.
Relevant discussion: #7785
Related: #5924, #3991
<img width="283" alt="borders"
src="https://user-images.githubusercontent.com/27962798/220968899-7661d5ec-6f5b-4b0f-af29-bf9af02259b5.PNG">
## Solution
Add an extraction function to draw the borders.
---
Can only do one colour rectangular borders due to the limitations of the
Bevy UI renderer.
Maybe it can be combined with #3991 eventually to add curved border
support.
## Changelog
* Added a component `BorderColor`.
* Added the `extract_uinode_borders` system to the UI Render App.
* Added the UI example `borders`
---------
Co-authored-by: Nico Burns <nico@nicoburns.com>
# Objective
The method `UnsafeWorldCell::read_change_tick` was renamed in #8588, but
I forgot to update a usage of this method in a doctest.
## Solution
Update the method call.
# Objective
To mirror the `Ref` added as `WorldQuery`, and the `Mut` in
`EntityMut::get_mut`, we add `EntityRef::get_ref`, which retrieves `T`
with tick information, but *immutably*.
## Solution
- Add the method in question, also add it to`UnsafeEntityCell` since
this seems to be the best way of getting that information.
Also update/add safety comments to neighboring code.
---
## Changelog
- Add `EntityRef::get_ref` to get an `Option<Ref<T>>` from `EntityRef`
---------
Co-authored-by: James Liu <contact@jamessliu.com>
# Objective
The method `QueryState::par_iter` does not currently force the query to
be read-only. This means you can unsoundly mutate a world through an
immutable reference in safe code.
```rust
fn bad_system(world: &World, mut query: Local<QueryState<&mut T>>) {
query.par_iter(world).for_each_mut(|mut x| *x = unsoundness);
}
```
## Solution
Use read-only versions of the `WorldQuery` types.
---
## Migration Guide
The function `QueryState::par_iter` now forces any world accesses to be
read-only, similar to how `QueryState::iter` works. Any code that
previously mutated the world using this method was *unsound*. If you
need to mutate the world, use `par_iter_mut` instead.
# Objective
Make a combined system cloneable if both systems are cloneable on their
own. This is necessary for using chained conditions (e.g
`cond1.and_then(cond2)`) with `distributive_run_if()`.
## Solution
Implement `Clone` for `CombinatorSystem<Func, A, B>` where `A, B:
Clone`.
# Objective
- Fixes#8782
## Solution
- Call `init_resource` on `register_diagnostic` so that a
`DiagnosticStore` gets initialized if it does not exist.
---------
Co-authored-by: François <mockersf@gmail.com>
# Objective
The `extract` function is given the main app world, and the subapp, not
vice versa as the comment would lead us to believe.
## Solution
Fix the doc.
# Objective
- Rename the `render::primitives::Plane` struct as to not confuse it
with `bevy_render::mesh::shape::Plane`
- Fixes https://github.com/bevyengine/bevy/issues/8730
## Solution
- Refactor the `render::primitives::Plane` struct to
`render::primitives::HalfSpace`
- Modify documentation to reflect this change
## Changelog
- Renamed `Plane` to `HalfSpace` to more accurately represent it's use
- Renamed `planes` member in `Frustum` to `half_spaces` to reflect
changes
## Migration Guide
- `Plane` has been renamed to `HalfSpace`
- `planes` member in `Frustum` has been renamed to `half_spaces`
---------
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
Co-authored-by: Nicola Papale <nicopap@users.noreply.github.com>
# Objective
When browsing the bevy source code to try and learn about
`bevy_core_pipeline`, I noticed that the `DrawFunctions` resources,
`sort_phase_system`s and texture preparation for the `Opaque3d` and
`AlphaMask3d` phase items are all set up in `bevy_core_pipeline`, while
the `Opaque3dPrepass` and `AlphaMask3dPrepass` phase items are only
*declared* in `bevy_core_pipeline`, and actually registered properly
with the renderer in `bevy_pbr`.
This means that, if I am trying to make crate that replaces `bevy_pbr`,
I need to make sure I manually fix this unfinished setup the same way
that `bevy_pbr` does. Worse, it means that if I try to use the
`PrepassNode` `bevy_core_pipeline` adds *without* fixing this, the
engine will simply crash because the `DrawFunctions<T>` resources cannot
be accessed.
The only advantage I can think of for bevy doing it this way is an
ambiguous performance save due to the prepass render phases not being
present unless you are using prepass materials with PBR.
## Solution
I have moved the registration of `DrawFunctions<T>`,
`sort_phase_system::<T>`, camera `RenderPhase` extraction, and texture
preparation for prepass's phase items into `bevy_core_pipeline`
alongside the equivalent code that sets up the `Opaque3d`, `AlphaMask3d`
and `Transparent3d` phase items.
Am open to tweaking this to improve the performance impact of prepass
things being around if the app doesn't use them if needed.
I've tested that the `shader_prepass` example still works with this
change.
# Objective
EntityRef::get_change_ticks mentions that ComponentTicks is useful to
create change detection for your own runtime.
However, ComponentTicks doesn't even expose enough data to create
something that implements DetectChanges. Specifically, we need to be
able to extract the last change tick.
## Solution
We add a method to get the last change tick. We also add a method to get
the added tick.
## Changelog
- Add `last_changed_tick` and `added_tick` to `ComponentTicks`
# Objective
- Fixes#8811 .
## Solution
- Rename "write" method to "apply" in Command trait definition.
- Rename other implementations of command trait throughout bevy's code
base.
---
## Changelog
- Changed: `Command::write` has been changed to `Command::apply`
- Changed: `EntityCommand::write` has been changed to
`EntityCommand::apply`
## Migration Guide
- `Command::write` implementations need to be changed to implement
`Command::apply` instead. This is a mere name change, with no further
actions needed.
- `EntityCommand::write` implementations need to be changed to implement
`EntityCommand::apply` instead. This is a mere name change, with no
further actions needed.
---------
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
# Objective
- I can't map unsized type using `Ref::map` (for example `dyn Reflect`)
## Solution
- Allow unsized types (this is possible because `Ref` stores a reference
to `T`)
# Objective
`QueryState` exposes a `get_manual` and `iter_manual` method. However,
there is now `iter_many_manual`.
`iter_many_manual` is useful when you have a `&World` (eg: the `world`
in a `Scene`) and want to run a query several times on it (eg:
iteratively navigate a hierarchy by calling `iter_many` on `Children`
component).
`iter_many`'s need for a `&mut World` makes the API much less flexible.
The exclusive access pattern requires doing some very funky dance and
excludes a category of algorithms for hierarchy traversal.
## Solution
- Add a `iter_many_manual` method to `QueryState`
### Alternative
My current workaround is to use `get_manual`. However, this doesn't
benefit from the optimizations on `QueryManyIter`.
---
## Changelog
- Add a `iter_many_manual` method to `QueryState`
# Objective
Title.
---------
Co-authored-by: François <mockersf@gmail.com>
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
Co-authored-by: James Liu <contact@jamessliu.com>
# Objective
`Ref` is a useful way of accessing change detection data.
However, unlike `Mut`, it doesn't expose a constructor or even a way to
go from `Ref<A>` to `Ref<B>`.
Such methods could be useful, for example, to 3rd party crates that want
to expose change detection information in a clean way.
My use case is to map a `Ref<T>` into a `Ref<dyn Reflect>`, and keep
change detection info to avoid running expansive routines.
## Solution
We add the `new` and `map` methods. Since similar methods exist on `Mut`
where they are much more footgunny to use, I judged that it was
acceptable to create such methods.
## Workaround
Currently, it's not possible to create/project `Ref`s. One can define
their own `Ref` and implement `ChangeDetection` on it. One would then
use `ChangeTrackers` to populate the custom `Ref` with tick data.
---
## Changelog
- Added the `Ref::map` and `Ref::new` methods for more ergonomic `Ref`s
---------
Co-authored-by: Gino Valente <49806985+MrGVSV@users.noreply.github.com>
# Objective
- Some reflect components weren't properly registered.
## Solution
- We register them
- I also sorted the register lines in `Plugin::build` in `bevy_ui`
### Note
How I did I find them:
- I picked up the list of `Component`s from the `Component` trait page
in rustdoc.
- Then I tried to register all of them. Removing the registration when
it doesn't implement `Reflect` to pass compilation.
- Then I added `app.register_type_data::<T, Foo>()`, for all Reflect
components. It panics if `T` is not registered.
- I repeated the last line N times until bevy stopped panicking at
startup
---
## Changelog
- Register the following components: `PrimaryWindow` `Fxaa`
`FogSettings` `NotShadowCaster` `NotShadowReceiver` `CalculatedClip`
`RelativeCursorPosition`
`AlphaMode` is not used as a component anywhere in the engine. It
shouldn't implement `Component`. It might mislead users into thinking it
has any effect as a component.
---
## Changelog
- Remove `Component` implementation for `AlphaMode`. It wasn't used by
anything.
## Migration Guide
`AlphaMode` is not a component anymore.
It wasn't used anywhere in the engine. If you were using it as a
component for your own purposes, you should use a newtype instead, as
follow:
```rust
#[derive(Component, Deref)]
struct MyAlphaMode(AlphaMode);
```
Then replace uses of `AlphaMode` with `MyAlphaMode`
```diff
- Query<&AlphaMode, …>,
+ Query<&MyAlphaMode, …>,
```
# Objective
- When a window is closed, the associated camera keeps rendering even if
the RenderTarget isn't valid anymore.
- This is essentially just wasting a lot of performance.
## Solution
- Detect the window close event and disable any camera that used the
window has a RenderTarget.
## Notes
It's possible a similar thing could be done for camera that use an image
handle, but I would fix that in a separate PR.
# Objective
`NoFrustumCulling` doesn't implement `Reflect`, while nothing prevents
it from implementing it.
## Solution
Implement `Reflect` for it.
---
## Changelog
- Add `Reflect` derive to `NoFrustrumCulling`.
- Add `FromReflect` derive to `Visibility`.
# Objective
This calculation is performed componentwise but all the values are
vectors so it should be using vector operations.
Works correctly with the `relative_cursor_position` example.
# Objective
To upgrade winit's dependency, it's useful to reuse SmolStr, which
replaces/improves the too restrictive Key letter enums.
As Input<Key> is a resource it should implement Reflect through all its
fields.
## Solution
Add smol_str to bevy_reflect supported types, behind a feature flag.
This PR blocks winit's upgrade PR:
https://github.com/bevyengine/bevy/pull/8745.
# Current state
- I'm discovering bevy_reflect, I appreciate all feedbacks, and send me
your nitpicks!
- Lacking more tests
---------
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
Co-authored-by: Gino Valente <49806985+MrGVSV@users.noreply.github.com>
# Objective
The goal of this PR is to receive touchpad magnification and rotation
events.
## Solution
Implement pendants for winit's `TouchpadMagnify` and `TouchpadRotate`
events.
Adjust the `mouse_input_events.rs` example to debug magnify and rotate
events.
Since winit only reports these events on macOS, the Bevy events for
touchpad magnification and rotation are currently only fired on macOS.
Updates the requirements on
[notify](https://github.com/notify-rs/notify) to permit the latest
version.
<details>
<summary>Changelog</summary>
<p><em>Sourced from <a
href="https://github.com/notify-rs/notify/blob/main/CHANGELOG.md">notify's
changelog</a>.</em></p>
<blockquote>
<h2>notify 6.0.0 (2023-05-17)</h2>
<ul>
<li>CHANGE: files and directories moved into a watch folder on Linux
will now be reported as <code>rename to</code> events instead of
<code>create</code> events <a
href="https://redirect.github.com/notify-rs/notify/issues/480">#480</a></li>
<li>CHANGE: on Linux <code>rename from</code> events will be emitted
immediately without starting a new thread <a
href="https://redirect.github.com/notify-rs/notify/issues/480">#480</a></li>
<li>CHANGE: raise MSRV to 1.60 <a
href="https://redirect.github.com/notify-rs/notify/issues/480">#480</a></li>
</ul>
<h2>debouncer-mini 0.3.0 (2023-05-17)</h2>
<ul>
<li>CHANGE: upgrade to notify 6.0.0, pushing MSRV to 1.60 <a
href="https://redirect.github.com/notify-rs/notify/issues/480">#480</a></li>
</ul>
<h2>debouncer-full 0.1.0 (2023-05-17)</h2>
<p>Newly introduced alternative debouncer with more features. <a
href="https://redirect.github.com/notify-rs/notify/issues/480">#480</a></p>
<ul>
<li>FEATURE: only emit a single <code>rename</code> event if the rename
<code>From</code> and <code>To</code> events can be matched</li>
<li>FEATURE: merge multiple <code>rename</code> events</li>
<li>FEATURE: keep track of the file system IDs all files and stiches
rename events together (FSevents, Windows)</li>
<li>FEATURE: emit only one <code>remove</code> event when deleting a
directory (inotify)</li>
<li>FEATURE: don't emit duplicate create events</li>
<li>FEATURE: don't emit <code>Modify</code> events after a
<code>Create</code> event</li>
</ul>
<p><a
href="https://redirect.github.com/notify-rs/notify/issues/480">#480</a>:
<a
href="https://redirect.github.com/notify-rs/notify/pull/480">notify-rs/notify#480</a></p>
<h2>notify 5.2.0 (2023-05-17)</h2>
<ul>
<li>CHANGE: implement <code>Copy</code> for <code>EventKind</code> and
<code>ModifyKind</code> <a
href="https://redirect.github.com/notify-rs/notify/issues/481">#481</a></li>
</ul>
<p><a
href="https://redirect.github.com/notify-rs/notify/issues/481">#481</a>:
<a
href="https://redirect.github.com/notify-rs/notify/pull/481">notify-rs/notify#481</a></p>
<h2>notify 5.1.0 (2023-01-15)</h2>
<ul>
<li>CHANGE: switch from winapi to windows-sys <a
href="https://redirect.github.com/notify-rs/notify/issues/457">#457</a></li>
<li>FIX: kqueue-backend: batch file-watching together to improve
performance <a
href="https://redirect.github.com/notify-rs/notify/issues/454">#454</a></li>
<li>DOCS: include license file in crate again <a
href="https://redirect.github.com/notify-rs/notify/issues/461">#461</a></li>
<li>DOCS: typo and examples fixups</li>
</ul>
<p><a
href="https://redirect.github.com/notify-rs/notify/issues/454">#454</a>:
<a
href="https://redirect.github.com/notify-rs/notify/pull/454">notify-rs/notify#454</a>
<a
href="https://redirect.github.com/notify-rs/notify/issues/461">#461</a>:
<a
href="https://redirect.github.com/notify-rs/notify/pull/461">notify-rs/notify#461</a>
<a
href="https://redirect.github.com/notify-rs/notify/issues/457">#457</a>:
<a
href="https://redirect.github.com/notify-rs/notify/pull/457">notify-rs/notify#457</a></p>
<h2>debouncer-mini 0.2.1 (2022-09-05)</h2>
<ul>
<li>DOCS: correctly document the <code>crossbeam</code> feature <a
href="https://redirect.github.com/notify-rs/notify/issues/440">#440</a></li>
</ul>
<p><a
href="https://redirect.github.com/notify-rs/notify/issues/440">#440</a>:
<a
href="https://redirect.github.com/notify-rs/notify/pull/440">notify-rs/notify#440</a></p>
<h2>debouncer-mini 0.2.0 (2022-08-30)</h2>
<p>Upgrade notify dependency to 5.0.0</p>
<!-- raw HTML omitted -->
</blockquote>
<p>... (truncated)</p>
</details>
<details>
<summary>Commits</summary>
<ul>
<li>See full diff in <a
href="https://github.com/notify-rs/notify/commits">compare view</a></li>
</ul>
</details>
<br />
Dependabot will resolve any conflicts with this PR as long as you don't
alter it yourself. You can also trigger a rebase manually by commenting
`@dependabot rebase`.
[//]: # (dependabot-automerge-start)
[//]: # (dependabot-automerge-end)
---
<details>
<summary>Dependabot commands and options</summary>
<br />
You can trigger Dependabot actions by commenting on this PR:
- `@dependabot rebase` will rebase this PR
- `@dependabot recreate` will recreate this PR, overwriting any edits
that have been made to it
- `@dependabot merge` will merge this PR after your CI passes on it
- `@dependabot squash and merge` will squash and merge this PR after
your CI passes on it
- `@dependabot cancel merge` will cancel a previously requested merge
and block automerging
- `@dependabot reopen` will reopen this PR if it is closed
- `@dependabot close` will close this PR and stop Dependabot recreating
it. You can achieve the same result by closing it manually
- `@dependabot ignore this major version` will close this PR and stop
Dependabot creating any more for this major version (unless you reopen
the PR or upgrade to it yourself)
- `@dependabot ignore this minor version` will close this PR and stop
Dependabot creating any more for this minor version (unless you reopen
the PR or upgrade to it yourself)
- `@dependabot ignore this dependency` will close this PR and stop
Dependabot creating any more for this dependency (unless you reopen the
PR or upgrade to it yourself)
</details>
Signed-off-by: dependabot[bot] <support@github.com>
Co-authored-by: dependabot[bot] <49699333+dependabot[bot]@users.noreply.github.com>
Updates the requirements on
[ruzstd](https://github.com/KillingSpark/zstd-rs) to permit the latest
version.
<details>
<summary>Release notes</summary>
<p><em>Sourced from <a
href="https://github.com/KillingSpark/zstd-rs/releases">ruzstd's
releases</a>.</em></p>
<blockquote>
<h2>No-std support and better dict API</h2>
<p>This release features no-std support with big thanks to <a
href="https://github.com/antangelo"><code>@antangelo</code></a>!</p>
<p>Also the API for dictionaries has been revised, which required some
breaking changes in that department</p>
</blockquote>
</details>
<details>
<summary>Commits</summary>
<ul>
<li><a
href="fa7bd9c7b3"><code>fa7bd9c</code></a>
allow streaming decoder to also be used with a &mut FrameDecoder for
easier r...</li>
<li><a
href="3b6403b8e7"><code>3b6403b</code></a>
reenable forcing a different dict</li>
<li><a
href="2be7fbb01b"><code>2be7fbb</code></a>
Merge pull request <a
href="https://redirect.github.com/KillingSpark/zstd-rs/issues/40">#40</a>
from KillingSpark/overhaul_dicts</li>
<li><a
href="343d69b339"><code>343d69b</code></a>
no need to check that the dict still matches at the start of each decode
call</li>
<li><a
href="d73f5e689a"><code>d73f5e6</code></a>
cargo fmt</li>
<li><a
href="f3f09c76f0"><code>f3f09c7</code></a>
improve initing the decoder from a dict</li>
<li><a
href="0b9331dd19"><code>0b9331d</code></a>
make clippy happy</li>
<li><a
href="06433dec34"><code>06433de</code></a>
start overhauling dict API</li>
<li><a
href="1256944604"><code>1256944</code></a>
Update ci.yml</li>
<li><a
href="3449d0a2bf"><code>3449d0a</code></a>
Merge pull request <a
href="https://redirect.github.com/KillingSpark/zstd-rs/issues/39">#39</a>
from antangelo/no_std</li>
<li>Additional commits viewable in <a
href="https://github.com/KillingSpark/zstd-rs/compare/v0.3.1...v0.4.0">compare
view</a></li>
</ul>
</details>
<br />
Dependabot will resolve any conflicts with this PR as long as you don't
alter it yourself. You can also trigger a rebase manually by commenting
`@dependabot rebase`.
[//]: # (dependabot-automerge-start)
[//]: # (dependabot-automerge-end)
---
<details>
<summary>Dependabot commands and options</summary>
<br />
You can trigger Dependabot actions by commenting on this PR:
- `@dependabot rebase` will rebase this PR
- `@dependabot recreate` will recreate this PR, overwriting any edits
that have been made to it
- `@dependabot merge` will merge this PR after your CI passes on it
- `@dependabot squash and merge` will squash and merge this PR after
your CI passes on it
- `@dependabot cancel merge` will cancel a previously requested merge
and block automerging
- `@dependabot reopen` will reopen this PR if it is closed
- `@dependabot close` will close this PR and stop Dependabot recreating
it. You can achieve the same result by closing it manually
- `@dependabot ignore this major version` will close this PR and stop
Dependabot creating any more for this major version (unless you reopen
the PR or upgrade to it yourself)
- `@dependabot ignore this minor version` will close this PR and stop
Dependabot creating any more for this minor version (unless you reopen
the PR or upgrade to it yourself)
- `@dependabot ignore this dependency` will close this PR and stop
Dependabot creating any more for this dependency (unless you reopen the
PR or upgrade to it yourself)
</details>
Signed-off-by: dependabot[bot] <support@github.com>
Co-authored-by: dependabot[bot] <49699333+dependabot[bot]@users.noreply.github.com>
Followup to #7184
This makes `Reflect: DynamicTypePath` which allows us to remove
`Reflect::get_type_path`, reducing unnecessary codegen and simplifying
`Reflect` implementations.
# Objective
Be consistent with `Resource`s and `Components` and have `Event` types
be more self-documenting.
Although not susceptible to accidentally using a function instead of a
value due to `Event`s only being initialized by their type, much of the
same reasoning for removing the blanket impl on `Resource` also applies
here.
* Not immediately obvious if a type is intended to be an event
* Prevent invisible conflicts if the same third-party or primitive types
are used as events
* Allows for further extensions (e.g. opt-in warning for missed events)
## Solution
Remove the blanket impl for the `Event` trait. Add a derive macro for
it.
---
## Changelog
- `Event` is no longer implemented for all applicable types. Add the
`#[derive(Event)]` macro for events.
## Migration Guide
* Add the `#[derive(Event)]` macro for events. Third-party types used as
events should be wrapped in a newtype.
# Objective
It was accidentally found that rustc is unable to parse certain
constructs in `where` clauses properly. `bevy_reflect::Reflect`'s habit
of copying and pasting the field types in a type's definition to its
`where` clauses made it very easy to accidentally run into this
behaviour - particularly with the construct
```rust
where
for<'a> fn(&'a T) -> &'a T: Trait1 + Trait2
```
which was incorrectly parsed as
```rust
where
for<'a> (fn(&'a T) -> &'a T: Trait1 + Trait2)
^ ^ incorrect syntax grouping
```
instead of
```rust
where
(for<'a> fn(&'a T) -> &'a T): Trait1 + Trait2
^ ^ correct syntax grouping
```
Fixes#8759
## Solution
This commit fixes the issue by inserting explicit parentheses to
disambiguate types from their bound lists.
# Objective
- Fixes https://github.com/bevyengine/bevy/issues/8586.
## Solution
- Add `preferred_theme` field to `Window` and set it when window
creation
- Add `window_theme` field to `InternalWindowState` to store current
window theme
- Expose winit `WindowThemeChanged` event
---------
Co-authored-by: hate <15314665+hate@users.noreply.github.com>
Co-authored-by: Nicola Papale <nicopap@users.noreply.github.com>
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
Co-authored-by: François <mockersf@gmail.com>
# Objective
Fixes#8751
## Solution
The doc string for the `primary_window` field on `Window` now mentions
that the default spawned primary window is spawned with the
`PrimaryWindow` marker component.
---------
Co-authored-by: François <mockersf@gmail.com>
# Objective
- Fix#8658
- `without_winit` example panics `thread 'Compute Task Pool (2)'
panicked at 'called `Option::unwrap()` on a `None` value',
crates/bevy_render/src/pipelined_rendering.rs:134:84`
## Solution
- In the default runner method `run_once`, correctly finish the
initialisation of the plugins. `run_once` can't be called twice so it's
ok to do it there