# Objective
- Bevy has several `compile_fail` test
- #2254 added `#[derive(Component)]`
- Those tests now fail for a different reason.
- This was not caught as these test still "successfully" failed to compile.
## Solution
- Add `#[derive(Component)]` to the doctest
- Also changed their cfg attribute from `doc` to `doctest`, so that these tests don't appear when running `cargo doc` with `--document-private-items`
# Objective
- Fixes#2501
- Builds up on #2639 taking https://github.com/bevyengine/bevy/pull/2639#issuecomment-898701047 into account
## Solution
- keep the physical cursor position in `Window`, and expose it.
- still convert to logical position in event, and when getting `cursor_position`
Co-authored-by: Ahmed Charles <acharles@outlook.com>
# Objective
The update to wgpu 0.11 broke CI for android. This was due to a confusion between `bevy::render::ShaderStage` and `wgpu::ShaderStage`.
## Solution
Revert the incorrect change
#2605 changed the lifetime annotations on `get_component` introducing unsoundness as you could keep the returned borrow even after using the query.
Example unsoundness:
```rust
use bevy::prelude::*;
fn main() {
App::new()
.add_startup_system(startup)
.add_system(unsound)
.run();
}
#[derive(Debug, Component, PartialEq, Eq)]
struct Foo(Vec<u32>);
fn startup(mut c: Commands) {
let e = c.spawn().insert(Foo(vec![10])).id();
c.insert_resource(e);
}
fn unsound(mut q: Query<&mut Foo>, res: Res<Entity>) {
let foo = q.get_component::<Foo>(*res).unwrap();
let mut foo2 = q.iter_mut().next().unwrap();
let first_elem = &foo.0[0];
for _ in 0..16 {
foo2.0.push(12);
}
dbg!(*first_elem);
}
```
output:
`[src/main.rs:26] *first_elem = 0`
Add the entity ID and generation to the expect() message of two
world accessors, to make it easier to debug use-after-free issues.
Coupled with e.g. bevy-inspector-egui which also displays the entity ID,
this makes it much easier to identify what entity is being misused.
# Objective
Make it easier to identity an entity being accessed after being deleted.
## Solution
Augment the error message of some `expect()` call with the entity ID and
generation. Combined with some external tool like `bevy-inspector-egui`, which
also displays the entity ID, this increases the chances to be able to identify
the entity, and therefore find the error that led to a use-after-despawn.
Upgrades both the old and new renderer to wgpu 0.11 (and naga 0.7). This builds on @zicklag's work here #2556.
Co-authored-by: Carter Anderson <mcanders1@gmail.com>
# Objective
- Remove duplicate `Events::update` call in `gilrs_event_system` (fixes#2893)
- See #2893 for context
- While there, make the systems no longer exclusive, as that is not required of them
## Solution
- Do the change
r? @alice-i-cecile
Using RenderQueue in BufferVec allows removal of the staging buffer entirely, as well as removal of the SpriteNode.
Co-authored-by: Carter Anderson <mcanders1@gmail.com>
This PR adds a ControlNode which marks an entity as "transparent" to the UI layout system, meaning the children of this entity will be treated as the children of this entity s parent by the layout system(s).
# Objective
- Fixes#2904 (see for context)
## Solution
- Simply hoist span creation out of the threaded task
- Confirmed to solve the issue locally
Now all events have the full span parent tree up through `bevy_ecs::schedule::stage` all the way to `bevy_app::app::bevy_app` (and its parents in bevy-consumer code, if any).
# Objective
- Avoid usages of `format!` that ~immediately get passed to another `format!`. This avoids a temporary allocation and is just generally cleaner.
## Solution
- `bevy_derive::shader_defs` does a `format!("{}", val.to_string())`, which is better written as just `format!("{}", val)`
- `bevy_diagnostic::log_diagnostics_plugin` does a `format!("{:>}", format!(...))`, which is better written as `format!("{:>}", format_args!(...))`
- `bevy_ecs::schedule` does `tracing::info!(..., name = &*format!("{:?}", val))`, which is better written with the tracing shorthand `tracing::info!(..., name = ?val)`
- `bevy_reflect::reflect` does `f.write_str(&format!(...))`, which is better written as `write!(f, ...)` (this could also be written using `f.debug_tuple`, but I opted to maintain alt debug behavior)
- `bevy_reflect::serde::{ser, de}` do `serde::Error::custom(format!(...))`, which is better written as `Error::custom(format_args!(...))`, as `Error::custom` takes `impl Display` and just immediately calls `format!` again
# Objective
- removed unused RenderResourceId and SwapChainFrame (already unified with TextureView)
- added deref to BindGroup, this makes conversion to wgpu::BindGroup easier
## Solution
- cleans up the API
This implements the most minimal variant of #1843 - a derive for marker trait. This is a prerequisite to more complicated features like statically defined storage type or opt-out component reflection.
In order to make component struct's purpose explicit and avoid misuse, it must be annotated with `#[derive(Component)]` (manual impl is discouraged for compatibility). Right now this is just a marker trait, but in the future it might be expanded. Making this change early allows us to make further changes later without breaking backward compatibility for derive macro users.
This already prevents a lot of issues, like using bundles in `insert` calls. Primitive types are no longer valid components as well. This can be easily worked around by adding newtype wrappers and deriving `Component` for them.
One funny example of prevented bad code (from our own tests) is when an newtype struct or enum variant is used. Previously, it was possible to write `insert(Newtype)` instead of `insert(Newtype(value))`. That code compiled, because function pointers (in this case newtype struct constructor) implement `Send + Sync + 'static`, so we allowed them to be used as components. This is no longer the case and such invalid code will trigger a compile error.
Co-authored-by: = <=>
Co-authored-by: TheRawMeatball <therawmeatball@gmail.com>
Co-authored-by: Carter Anderson <mcanders1@gmail.com>
# Objective
Fixes these issues:
- `WorldId`s currently aren't necessarily unique
- I want to guarantee that they're unique to safeguard my librarified version of https://github.com/bevyengine/bevy/discussions/2805
- There probably hasn't been a collision yet, but they could technically collide
- `SystemId` isn't used for anything
- It's no longer used now that `Locals` are stored within the `System`.
- `bevy_ecs` depends on rand
## Solution
- Instead of randomly generating `WorldId`s, just use an incrementing atomic counter, panicing on overflow.
- Remove `SystemId`
- We do need to allow Locals for exclusive systems at some point, but exclusive systems couldn't access their own `SystemId` anyway.
- Now that these don't depend on rand, move it to a dev-dependency
## Todo
Determine if `WorldId` should be `u32` based instead
If you need to build a texture atlas from an already created texture that is not match a grid, you need to use new_empty and add_texture to create it. However it is not straight forward to get the index to be used with TextureAtlasSprite. add_texture should be changed to return the index to the texture.
Currently you can do something like this:
```rs
let texture = asset_server.load::<Texture>::("texture.png");
let texture_atlas = TextureAtlas::new_empty(texture, Vec2::new(40.0, 40.0));
texture_atlas.add_texture(Rect {
min: Vec2::new(20.0, 20.0),
max: Vec2::new(40.0, 40.0),
});
let index = (texture_atlas.len() - 1) as u32;
let texture_atlas_sprite = TextureAtlasSprite {
index,
Default::default()
};
```
But this is more clear
```rs
let index = texture_atlas.add_texture(Rect {
min: Vec2::new(20.0, 20.0),
max: Vec2::new(40.0, 40.0),
});
```
Updates the requirements on [hexasphere](https://github.com/OptimisticPeach/hexasphere) to permit the latest version.
<details>
<summary>Commits</summary>
<ul>
<li>See full diff in <a href="https://github.com/OptimisticPeach/hexasphere/commits">compare view</a></li>
</ul>
</details>
<br />
Dependabot will resolve any conflicts with this PR as long as you don't alter it yourself. You can also trigger a rebase manually by commenting `@dependabot rebase`.
[//]: # (dependabot-automerge-start)
[//]: # (dependabot-automerge-end)
---
<details>
<summary>Dependabot commands and options</summary>
<br />
You can trigger Dependabot actions by commenting on this PR:
- `@dependabot rebase` will rebase this PR
- `@dependabot recreate` will recreate this PR, overwriting any edits that have been made to it
- `@dependabot merge` will merge this PR after your CI passes on it
- `@dependabot squash and merge` will squash and merge this PR after your CI passes on it
- `@dependabot cancel merge` will cancel a previously requested merge and block automerging
- `@dependabot reopen` will reopen this PR if it is closed
- `@dependabot close` will close this PR and stop Dependabot recreating it. You can achieve the same result by closing it manually
- `@dependabot ignore this major version` will close this PR and stop Dependabot creating any more for this major version (unless you reopen the PR or upgrade to it yourself)
- `@dependabot ignore this minor version` will close this PR and stop Dependabot creating any more for this minor version (unless you reopen the PR or upgrade to it yourself)
- `@dependabot ignore this dependency` will close this PR and stop Dependabot creating any more for this dependency (unless you reopen the PR or upgrade to it yourself)
</details>
# Objective
Noticed a comment saying changed detection should be enabled for hierarchy maintenance once stable
Fixes#891
## Solution
Added `Changed<Parent>` filter on the query
Changed out unwraps to use if let syntax instead. Returning false when None.
Also modified an existing test to encompass these methods
This PR fixes#2828
# Objective
Using fullscreen or trying to resize a window caused a panic. Fix that.
## Solution
- Don't wholesale overwrite the ExtractedWindows resource when extracting windows
- This could cause an accumulation of unused windows that are holding onto swap chain frames?
- Check the if width and/or height changed since the last frame
- If the size changed, recreate the swap chain
- Ensure dimensions are >= 1 to avoid panics due to any dimension being 0
This changes how render logic is composed to make it much more modular. Previously, all extraction logic was centralized for a given "type" of rendered thing. For example, we extracted meshes into a vector of ExtractedMesh, which contained the mesh and material asset handles, the transform, etc. We looked up bindings for "drawn things" using their index in the `Vec<ExtractedMesh>`. This worked fine for built in rendering, but made it hard to reuse logic for "custom" rendering. It also prevented us from reusing things like "extracted transforms" across contexts.
To make rendering more modular, I made a number of changes:
* Entities now drive rendering:
* We extract "render components" from "app components" and store them _on_ entities. No more centralized uber lists! We now have true "ECS-driven rendering"
* To make this perform well, I implemented #2673 in upstream Bevy for fast batch insertions into specific entities. This was merged into the `pipelined-rendering` branch here: #2815
* Reworked the `Draw` abstraction:
* Generic `PhaseItems`: each draw phase can define its own type of "rendered thing", which can define its own "sort key"
* Ported the 2d, 3d, and shadow phases to the new PhaseItem impl (currently Transparent2d, Transparent3d, and Shadow PhaseItems)
* `Draw` trait and and `DrawFunctions` are now generic on PhaseItem
* Modular / Ergonomic `DrawFunctions` via `RenderCommands`
* RenderCommand is a trait that runs an ECS query and produces one or more RenderPass calls. Types implementing this trait can be composed to create a final DrawFunction. For example the DrawPbr DrawFunction is created from the following DrawCommand tuple. Const generics are used to set specific bind group locations:
```rust
pub type DrawPbr = (
SetPbrPipeline,
SetMeshViewBindGroup<0>,
SetStandardMaterialBindGroup<1>,
SetTransformBindGroup<2>,
DrawMesh,
);
```
* The new `custom_shader_pipelined` example illustrates how the commands above can be reused to create a custom draw function:
```rust
type DrawCustom = (
SetCustomMaterialPipeline,
SetMeshViewBindGroup<0>,
SetTransformBindGroup<2>,
DrawMesh,
);
```
* ExtractComponentPlugin and UniformComponentPlugin:
* Simple, standardized ways to easily extract individual components and write them to GPU buffers
* Ported PBR and Sprite rendering to the new primitives above.
* Removed staging buffer from UniformVec in favor of direct Queue usage
* Makes UniformVec much easier to use and more ergonomic. Completely removes the need for custom render graph nodes in these contexts (see the PbrNode and view Node removals and the much simpler call patterns in the relevant Prepare systems).
* Added a many_cubes_pipelined example to benchmark baseline 3d rendering performance and ensure there were no major regressions during this port. Avoiding regressions was challenging given that the old approach of extracting into centralized vectors is basically the "optimal" approach. However thanks to a various ECS optimizations and render logic rephrasing, we pretty much break even on this benchmark!
* Lifetimeless SystemParams: this will be a bit divisive, but as we continue to embrace "trait driven systems" (ex: ExtractComponentPlugin, UniformComponentPlugin, DrawCommand), the ergonomics of `(Query<'static, 'static, (&'static A, &'static B, &'static)>, Res<'static, C>)` were getting very hard to bear. As a compromise, I added "static type aliases" for the relevant SystemParams. The previous example can now be expressed like this: `(SQuery<(Read<A>, Read<B>)>, SRes<C>)`. If anyone has better ideas / conflicting opinions, please let me know!
* RunSystem trait: a way to define Systems via a trait with a SystemParam associated type. This is used to implement the various plugins mentioned above. I also added SystemParamItem and QueryItem type aliases to make "trait stye" ecs interactions nicer on the eyes (and fingers).
* RenderAsset retrying: ensures that render assets are only created when they are "ready" and allows us to create bind groups directly inside render assets (which significantly simplified the StandardMaterial code). I think ultimately we should swap this out on "asset dependency" events to wait for dependencies to load, but this will require significant asset system changes.
* Updated some built in shaders to account for missing MeshUniform fields
## Objective
The upcoming Bevy Book makes many references to the API documentation of bevy.
Most references belong to the first two chapters of the Bevy Book:
- bevyengine/bevy-website#176
- bevyengine/bevy-website#182
This PR attempts to improve the documentation of `bevy_ecs` and `bevy_app` in order to help readers of the Book who want to delve deeper into technical details.
## Solution
- Add crate and level module documentation
- Document the most important items (basically those included in the preludes), with the following style, where applicable:
- **Summary.** Short description of the item.
- **Second paragraph.** Detailed description of the item, without going too much in the implementation.
- **Code example(s).**
- **Safety or panic notes.**
## Collaboration
Any kind of collaboration is welcome, especially corrections, wording, new ideas and guidelines on where the focus should be put in.
---
### Related issues
- Fixes#2246
# Objective
[Tracy](https://github.com/wolfpld/tracy) is:
> A real time, nanosecond resolution, remote telemetry, hybrid frame and sampling profiler for games and other applications.
With the `trace_tracy` feature enabled, you run your bevy app and either a headless server (`capture`) or a live, interactive profiler UI (`Tracy`), and connect that to your bevy application to then stream the metric data and events, and save it or inspect it live/offline.
Previously when I implemented the spans across systems and stages and I was trying out different profiling tools, Tracy was too unstable on macOS to use. But now, quite some months later, it is working stably with Tracy 0.7.8. You can see timelines, aggregate statistics of mean system/stage execution times, and much more. It's very useful!
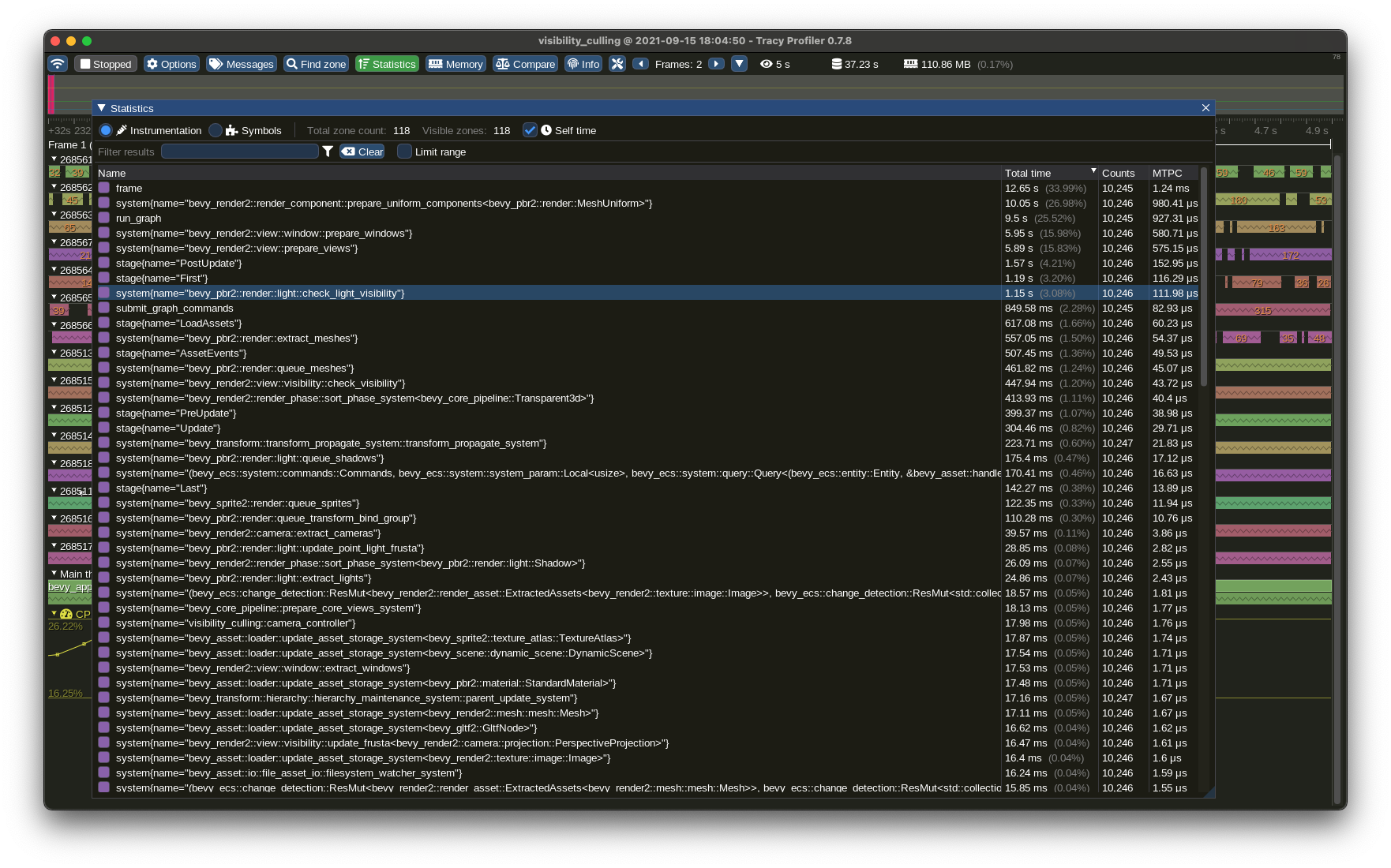
## Solution
- Use the `tracing-tracy` crate which supports our tracing spans
- Expose via the non-default feature `trace_tracy` for consistency with other `trace*` features
Before using this image resulted in an `Error in Queue::write_texture: copy of 0..4 would end up overrunning the bounds of the Source buffer of size 0`
# Objective
Bevy should expose all wgpu types needed for building rendering pipelines.
Closes#2818
## Solution
Add wgpu's StencilOperation to bevy_render2::render_resource's export.
This updates the `pipelined-rendering` branch to use the latest `bevy_ecs` from `main`. This accomplishes a couple of goals:
1. prepares for upcoming `custom-shaders` branch changes, which were what drove many of the recent bevy_ecs changes on `main`
2. prepares for the soon-to-happen merge of `pipelined-rendering` into `main`. By including bevy_ecs changes now, we make that merge simpler / easier to review.
I split this up into 3 commits:
1. **add upstream bevy_ecs**: please don't bother reviewing this content. it has already received thorough review on `main` and is a literal copy/paste of the relevant folders (the old folders were deleted so the directories are literally exactly the same as `main`).
2. **support manual buffer application in stages**: this is used to enable the Extract step. we've already reviewed this once on the `pipelined-rendering` branch, but its worth looking at one more time in the new context of (1).
3. **support manual archetype updates in QueryState**: same situation as (2).
# Objective
- CI is failing again
- These failures result from https://github.com/rust-lang/rust/pull/85200
## Solution
- Fix the errors which result from this by using the given fields
- I also removed the now unused `Debug` impl.
I suspect that we shouldn't use -D warnings for nightly CI - ideally we'd get a discord webhook message into some (non-#github) dedicated channel on warnings.
But this does not implement that.
# Objective
The vast majority of `.single()` usage I've seen is immediately followed by a `.unwrap()`. Since it seems most people use it without handling the error, I think making it easier to just get what you want fast while also having a more verbose alternative when you want to handle the error could help.
## Solution
Instead of having a lot of `.unwrap()` everywhere, this PR introduces a `try_single()` variant that behaves like the current `.single()` and make the new `.single()` panic on error.
# Objective
My attempt at fixing #2075 .
This is my very first contribution to this repo. Also, I'm very new to both Rust and bevy, so any feedback is *deeply* appreciated.
## Solution
- Changed `move_camera_system` so it only targets the camera entity. My approach here differs from the one used in the [cheatbook](https://bevy-cheatbook.github.io/cookbook/cursor2world.html?highlight=maincamera#2d-games) (in which a marker component is used to track the camera), so please, let me know which of them is more idiomatic.
- `move_camera_system` does not require both `Position` and `Transform` anymore (I used `rotate` for rotating the `Transform` in place, but couldn't find an equivalent `translate` method).
- Changed `tick_system` so it only targets the timer entity.
- Sprites are now spawned via a single `spawn_batch` instead of multiple `spawn`s.
# Objective
- Fixes#2751
## Solution
- Avoid changing the window size if there is a scale factor override
- Can be tested with the `scale_factor_override` example - use <kbd>⏎</kbd> to active overriding the scale factor
A few minor changes to fix warnings emitted from clippy on the nightly toolchain, including redundant_allocation, unwrap_or_else_default, and collapsible_match, fixes#2698
# Objective
Make it easier to construct transforms. E.g.
```rs
Transform::from_xyz(0.0, 0.0, 10.0).with_scale(Vec3::splat(2.0))
```
I found myself writing an extension method to do this so I don't have to write:
```rs
Transform {
translation: Vec3::new(0.0, 0.0, 10.0),
scale: Vec3::splat(2.0),
..Default::default()
}
```
## Solution
Add *builder style* methods to `Transform`.
Methods:
- `with_translation`
- `with_rotation`
- `with_scale`
I also added these methods to `GlobalTransform`. But they are probably less useful there.
# Objective
Expand the documentation for NixOS setups (as discussed in Discord)
## Solution
Added more info to `linux_dependencies.md` about NixOS. This is based off my own experience (as documented in [this blog post](https://blog.thomasheartman.com/posts/bevy-getting-started-on-nixos)), so I can't confirm that it'll work for everyone. However, if there are further tweaks necessary, then I think that this should nevertheless work as a good starting point and should give future users an idea of what they may need to change or update.
Feedback and tweaks are very welcome 😄
Updates the requirements on [glam](https://github.com/bitshifter/glam-rs) to permit the latest version.
<details>
<summary>Changelog</summary>
<p><em>Sourced from <a href="https://github.com/bitshifter/glam-rs/blob/main/CHANGELOG.md">glam's changelog</a>.</em></p>
<blockquote>
<h2>[0.18.0] - 2021-08-26</h2>
<h3>Breaking changes</h3>
<ul>
<li>Minimum Supported Version of Rust bumped to 1.51.0 for <code>wasm-bindgen-test</code>
and <code>rustdoc</code> <code>alias</code> support.</li>
</ul>
<h3>Added</h3>
<ul>
<li>Added <code>wasm32</code> SIMD intrinsics support.</li>
<li>Added optional support for the <code>rkyv</code> serialization crate.</li>
<li>Added <code>Rem</code> and <code>RemAssign</code> implementations for all vector types.</li>
<li>Added quaternion <code>xyz()</code> method for returning the vector part of the
quaternion.</li>
<li>Added <code>From((Scalar, Vector3))</code> for 4D vector types.</li>
</ul>
<h3>Changed</h3>
<ul>
<li>Deprecated <code>as_f32()</code>, <code>as_f64()</code>, <code>as_i32()</code> and <code>as_u32()</code> methods in favor
of more specific methods such as <code>as_vec2()</code>, <code>as_dvec2()</code>, <code>as_ivec2()</code> and
<code>as_uvec2()</code> and so on.</li>
</ul>
<h2>[0.17.3] - 2021-07-18</h2>
<h3>Fixed</h3>
<ul>
<li>Fix alignment unit tests on non x86 platforms.</li>
</ul>
<h2>[0.17.2] - 2021-07-15</h2>
<h3>Fixed</h3>
<ul>
<li>Fix alignment unit tests on i686 and S390x.</li>
</ul>
<h2>[0.17.1] - 2021-06-29</h2>
<h3>Added</h3>
<ul>
<li>Added <code>serde</code> support for <code>Affine2</code>, <code>DAffine2</code>, <code>Affine3A</code> and <code>DAffine3</code>.</li>
</ul>
<h2>[0.17.0] - 2021-06-26</h2>
<h3>Breaking changes</h3>
<ul>
<li>The addition of <code>Add</code> and <code>Sub</code> implementations of scalar values for vector
types may create ambiguities with existing calls to <code>add</code> and <code>sub</code>.</li>
<li>Removed <code>From<Mat3></code> implementation for <code>Mat2</code> and <code>From<DMat3></code> for <code>DMat2</code>.
These have been replaced by <code>Mat2::from_mat3()</code> and <code>DMat2::from_mat3()</code>.</li>
<li>Removed <code>From<Mat4></code> implementation for <code>Mat3</code> and <code>From<DMat4></code> for <code>DMat3</code>.
These have been replaced by <code>Mat3::from_mat4()</code> and <code>DMat3::from_mat4()</code>.</li>
</ul>
<!-- raw HTML omitted -->
</blockquote>
<p>... (truncated)</p>
</details>
<details>
<summary>Commits</summary>
<ul>
<li><a href="1b703518e7"><code>1b70351</code></a> Merge pull request <a href="https://github-redirect.dependabot.com/bitshifter/glam-rs/issues/231">#231</a> from bitshifter/prepare-0.18.0</li>
<li><a href="935ad5cf64"><code>935ad5c</code></a> Prepare 0.18.0 release.</li>
<li><a href="8d79d8e907"><code>8d79d8e</code></a> Still managed to mess up the tarpaulin config...</li>
<li><a href="78c30fc72c"><code>78c30fc</code></a> Fix syntax error in tarpaulin config.</li>
<li><a href="0258ce710d"><code>0258ce7</code></a> Can use rustdoc alias after msrv bump to 1.51.0.</li>
<li><a href="f9f7f2407c"><code>f9f7f24</code></a> Tidy up tarpaulin exlcudes.</li>
<li><a href="95dab216e1"><code>95dab21</code></a> Make some dev deps wasm only on not wasm.</li>
<li><a href="342176dde9"><code>342176d</code></a> Merge pull request <a href="https://github-redirect.dependabot.com/bitshifter/glam-rs/issues/230">#230</a> from DJMcNab/bytemuck-spirv</li>
<li><a href="837e5ebf7f"><code>837e5eb</code></a> Bytemuck now compiles on spirv</li>
<li><a href="bb35b1a691"><code>bb35b1a</code></a> Merge pull request <a href="https://github-redirect.dependabot.com/bitshifter/glam-rs/issues/228">#228</a> from bitshifter/wasm32-simd</li>
<li>Additional commits viewable in <a href="https://github.com/bitshifter/glam-rs/compare/0.17.3...0.18.0">compare view</a></li>
</ul>
</details>
<br />
Dependabot will resolve any conflicts with this PR as long as you don't alter it yourself. You can also trigger a rebase manually by commenting `@dependabot rebase`.
[//]: # (dependabot-automerge-start)
[//]: # (dependabot-automerge-end)
---
<details>
<summary>Dependabot commands and options</summary>
<br />
You can trigger Dependabot actions by commenting on this PR:
- `@dependabot rebase` will rebase this PR
- `@dependabot recreate` will recreate this PR, overwriting any edits that have been made to it
- `@dependabot merge` will merge this PR after your CI passes on it
- `@dependabot squash and merge` will squash and merge this PR after your CI passes on it
- `@dependabot cancel merge` will cancel a previously requested merge and block automerging
- `@dependabot reopen` will reopen this PR if it is closed
- `@dependabot close` will close this PR and stop Dependabot recreating it. You can achieve the same result by closing it manually
- `@dependabot ignore this major version` will close this PR and stop Dependabot creating any more for this major version (unless you reopen the PR or upgrade to it yourself)
- `@dependabot ignore this minor version` will close this PR and stop Dependabot creating any more for this minor version (unless you reopen the PR or upgrade to it yourself)
- `@dependabot ignore this dependency` will close this PR and stop Dependabot creating any more for this dependency (unless you reopen the PR or upgrade to it yourself)
</details>
# Objective
- All new PRs should get the "S-Needs-Triage" label. But at the moment we for example are getting quite a few PRs to the new renderer branch that do not get the label.
## Solution
- Remove the required target "main" from the workflow
- Also removed configuration for not needed functionality of the labeler action (see [docs](https://github.com/actions/labeler#inputs))
# Objective
- The breakout scoreboard was not using the correct text section to display the score integer.
## Solution
- This updates the code to use the correct text section.