# Objective
- Fixes#12068
## Solution
- Split `bevy_render::color::colorspace` across the various space
implementations in `bevy_color` as appropriate.
- Moved `From` implementations involving
`bevy_render::color::LegacyColor` into `bevy_render::color`
## Migration Guide
###
`bevy_render::color::colorspace::SrgbColorSpace::<f32>::linear_to_nonlinear_srgb`
Use `bevy_color::color::gamma_function_inverse`
###
`bevy_render::color::colorspace::SrgbColorSpace::<f32>::nonlinear_to_linear_srgb`
Use `bevy_color::color::gamma_function`
###
`bevy_render::color::colorspace::SrgbColorSpace::<u8>::linear_to_nonlinear_srgb`
Modify the `u8` value to instead be an `f32` (`|x| x as f32 / 255.`),
use `bevy_color::color::gamma_function_inverse`, and back again.
###
`bevy_render::color::colorspace::SrgbColorSpace::<u8>::nonlinear_to_linear_srgb`
Modify the `u8` value to instead be an `f32` (`|x| x as f32 / 255.`),
use `bevy_color::color::gamma_function`, and back again.
###
`bevy_render::color::colorspace::HslRepresentation::hsl_to_nonlinear_srgb`
Use `Hsla`'s implementation of `Into<Srgba>`
###
`bevy_render::color::colorspace::HslRepresentation::nonlinear_srgb_to_hsl`
Use `Srgba`'s implementation of `Into<Hsla>`
###
`bevy_render::color::colorspace::LchRepresentation::lch_to_nonlinear_srgb`
Use `Lcha`'s implementation of `Into<Srgba>`
###
`bevy_render::color::colorspace::LchRepresentation::nonlinear_srgb_to_lch`
Use `Srgba`'s implementation of `Into<Lcha>`
# Objective
The physical width and height (pixels) of an image is always integers,
but for `GpuImage` bevy currently stores them as `Vec2` (`f32`).
Switching to `UVec2` makes this more consistent with the [underlying
texture data](https://docs.rs/wgpu/latest/wgpu/struct.Extent3d.html).
I'm not sure if this is worth the change in the surface level API. If
not, feel free to close this PR.
## Solution
- Replace uses of `Vec2` with `UVec2` when referring to texture
dimensions.
- Use integer types for the texture atlas dimensions and sections.
[`Sprite::rect`](a81a2d1da3/crates/bevy_sprite/src/sprite.rs (L29))
remains unchanged, so manually specifying a sub-pixel region of an image
is still possible.
---
## Changelog
- `GpuImage` now stores its size as `UVec2` instead of `Vec2`.
- Texture atlases store their size and sections as `UVec2` and `URect`
respectively.
- `UiImageSize` stores its size as `UVec2`.
## Migration Guide
- Change floating point types (`Vec2`, `Rect`) to their respective
unsigned integer versions (`UVec2`, `URect`) when using `GpuImage`,
`TextureAtlasLayout`, `TextureAtlasBuilder`,
`DynamicAtlasTextureBuilder` or `FontAtlas`.
# Objective
- Simplify `Srgba` hex string parsing using std hex parsing functions
and removing loops in favor of bitwise ops.
This is a follow-up of the `bevy_color` upstream PR review:
https://github.com/bevyengine/bevy/pull/12013#discussion_r1497408114
## Solution
- Reworked `Srgba::hex` to use `from_str_radix` and some bitwise ops;
---------
Co-authored-by: Rob Parrett <robparrett@gmail.com>
# Objective
- Add the new `-Zcheck-cfg` checks to catch more warnings
- Fixes#12091
## Solution
- Create a new `cfg-check` to the CI that runs `cargo check -Zcheck-cfg
--workspace` using cargo nightly (and fails if there are warnings)
- Fix all warnings generated by the new check
---
## Changelog
- Remove all redundant imports
- Fix cfg wasm32 targets
- Add 3 dead code exceptions (should StandardColor be unused?)
- Convert ios_simulator to a feature (I'm not sure if this is the right
way to do it, but the check complained before)
## Migration Guide
No breaking changes
---------
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
# Objective
The migration process for `bevy_color` (#12013) will be fairly involved:
there will be hundreds of affected files, and a large number of APIs.
## Solution
To allow us to proceed granularly, we're going to keep both
`bevy_color::Color` (new) and `bevy_render::Color` (old) around until
the migration is complete.
However, simply doing this directly is confusing! They're both called
`Color`, making it very hard to tell when a portion of the code has been
ported.
As discussed in #12056, by renaming the old `Color` type, we can make it
easier to gradually migrate over, one API at a time.
## Migration Guide
THIS MIGRATION GUIDE INTENTIONALLY LEFT BLANK.
This change should not be shipped to end users: delete this section in
the final migration guide!
---------
Co-authored-by: Alice Cecile <alice.i.cecil@gmail.com>
# Objective
- Fixes#11977 - user defined shaders don't work in wasm
- After investigation, it won't work if the shader is not yet available
when compiling the pipeline on all platforms, for example if you load
many assets
## Solution
- Set the pipeline state to queued when it errs waiting for the shader
so that it's retried
This PR closes#11978
# Objective
Fix rendering on iOS Simulators.
iOS Simulator doesn't support the capability CUBE_ARRAY_TEXTURES, since
0.13 this started to make iOS Simulator not render anything with the
following message being outputted:
```
2024-02-19T14:59:34.896266Z ERROR bevy_render::render_resource::pipeline_cache: failed to create shader module: Validation Error
Caused by:
In Device::create_shader_module
Shader validation error:
Type [40] '' is invalid
Capability Capabilities(CUBE_ARRAY_TEXTURES) is required
```
## Solution
- Split up NO_ARRAY_TEXTURES_SUPPORT into both NO_ARRAY_TEXTURES_SUPPORT
and NO_CUBE_ARRAY_TEXTURES_SUPPORT and correctly apply
NO_ARRAY_TEXTURES_SUPPORT for iOS Simulator using the cfg flag
introduced in #10178.
---
## Changelog
### Fixed
- Rendering on iOS Simulator due to missing CUBE_ARRAY_TEXTURES support.
---------
Co-authored-by: Sam Pettersson <sam.pettersson@geoguessr.com>
# Objective
This PR adds some missing mime types to the
`ImageFormat::from_mime_type` method. As discussed [in this comment on
the Discord Bevy
community](https://discord.com/channels/691052431525675048/691052431974465548/1209904290227949729):
> It's strange that Bevy supports parsing `ImageFormat::WebP` from a
.webp str extension in the method below, but not from the mime type.
>
> In comparison, the image crate does parse it:
https://github.com/image-rs/image/blob/master/src/image.rs#L170
# Solution
For each of the missing mime types, I added them based on the
`ImageFormat::from_mime_type` of the image crate:
https://github.com/image-rs/image/blob/master/src/image.rs#L209, except
for `ImageFormat::Basis` and `ImageFormat::Ktx2` which are not present
in the image crate, and I ignore if they have a mime type or not*
\* apparently nowadays there is an official mime type: `image/ktx2`
https://www.iana.org/assignments/media-types/image/ktx2
Any feedback is welcome! I thought of refactoring a bit more and
delegating the mime type parsing to the image crate (and possibly the
same for extensions), let me know if that's desired 🙂
Fixes#12016.
Bump version after release
This PR has been auto-generated
Co-authored-by: Bevy Auto Releaser <41898282+github-actions[bot]@users.noreply.github.com>
Co-authored-by: François <mockersf@gmail.com>
# Objective
- Closes#11985
## Solution
- alpha.rs has been moved from bevy_pbr into bevy_render; bevy_pbr and
bevy_gltf now access `AlphaMode` through bevy_render.
---
## Migration Guide
In the present implementation, external consumers of `AlphaMode` will
have to access it through bevy_render rather than through bevy_pbr,
changing their import from `bevy_pbr::AlphaMode` to
`bevy_render::alpha::AlphaMode` (or the corresponding glob import from
`bevy_pbr::prelude::*` to `bevy_render::prelude::*`).
## Uncertainties
Some remaining things from this that I am uncertain about:
- Here, the `app.register_type<AlphaMode>()` call has been moved from
`PbrPlugin` to `RenderPlugin`; I'm not sure if this is quite right, and
I was unable to find any direct relationship between `PbrPlugin` and
`RenderPlugin`.
- `AlphaMode` was placed in the prelude of bevy_render. I'm not certain
that this is actually appropriate.
- bevy_pbr does not re-export `AlphaMode`, which makes this a breaking
change for external consumers.
Any of these things could be easily changed; I'm just not confident that
I necessarily adopted the right approach in these (known) ways since
this codebase and ecosystem is quite new to me.
Adopted #8266, so copy-pasting the description from there:
# Objective
Support the KHR_texture_transform extension for the glTF loader.
- Fixes#6335
- Fixes#11869
- Implements part of #11350
- Implements the GLTF part of #399
## Solution
As is, this only supports a single transform. Looking at Godot's source,
they support one transform with an optional second one for detail, AO,
and emission. glTF specifies one per texture. The public domain
materials I looked at seem to share the same transform. So maybe having
just one is acceptable for now. I tried to include a warning if multiple
different transforms exist for the same material.
Note the gltf crate doesn't expose the texture transform for the normal
and occlusion textures, which it should, so I just ignored those for
now. (note by @janhohenheim: this is still the case)
Via `cargo run --release --example scene_viewer
~/src/clone/glTF-Sample-Models/2.0/TextureTransformTest/glTF/TextureTransformTest.gltf`:
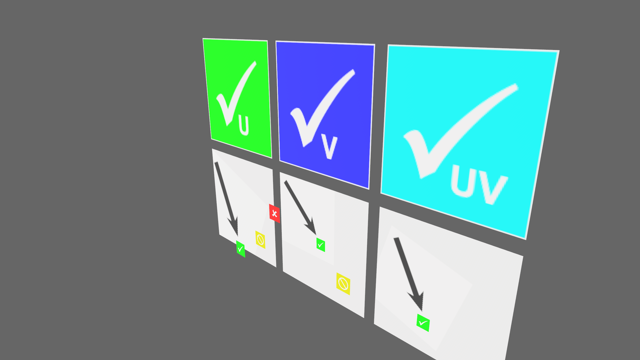
## Changelog
Support for the
[KHR_texture_transform](https://github.com/KhronosGroup/glTF/tree/main/extensions/2.0/Khronos/KHR_texture_transform)
extension added. Texture UVs that were scaled, rotated, or offset in a
GLTF are now properly handled.
---------
Co-authored-by: Al McElrath <hello@yrns.org>
Co-authored-by: Kanabenki <lucien.menassol@gmail.com>
# Objective
If multiple cameras render to the same target with MSAA enabled, only
the first and the last camera output will appear in the final output*.
This is because each camera maintains a separate flag to track the
active main texture. The first camera renders to texture A and all
subsequent cameras first write-back from A and then render into texture
B. Hence, camera 3 onwards will overwrite the work of the previous
camera.
\* This would manifest slightly differently if there were other calls to
post_process_write() in a more complex setup.
The is a functional regression from Bevy 0.12.
## Solution
The flag which tracks the active main texture should be shared between
cameras with the same `NormalizedRenderTarget`. Add the
`Arc<AtomicUsize>` to the existing per-target cache.
# Objective
We deprecated quite a few APIs in 0.13. 0.13 has shipped already. It
should be OK to remove them in 0.14's release. Fixes#4059. Fixes#9011.
## Solution
Remove them.
# Objective
There's a repeating pattern of `ThreadLocal<Cell<Vec<T>>>` which is very
useful for low overhead, low contention multithreaded queues that have
cropped up in a few places in the engine. This pattern is surprisingly
useful when building deferred mutation across multiple threads, as noted
by it's use in `ParallelCommands`.
However, `ThreadLocal<Cell<Vec<T>>>` is not only a mouthful, it's also
hard to ensure the thread-local queue is replaced after it's been
temporarily removed from the `Cell`.
## Solution
Wrap the pattern into `bevy_utils::Parallel<T>` which codifies the
entire pattern and ensures the user follows the contract. Instead of
fetching indivdual cells, removing the value, mutating it, and replacing
it, `Parallel::get` returns a `ParRef<'a, T>` which contains the
temporarily removed value and a reference back to the cell, and will
write the mutated value back to the cell upon being dropped.
I would like to use this to simplify the remaining part of #4899 that
has not been adopted/merged.
---
## Changelog
TODO
---------
Co-authored-by: Joseph <21144246+JoJoJet@users.noreply.github.com>
# Objective
Fixes#11964.
## Solution
Adds the `serde` feature to `bitflags` for `bevy_render`. This makes
`bevy_render` compile correctly when used alone.
---
## Changelog
- Fixed an issue where depending on `bevy_render` alone would fail to
compile.
# Objective
- I hated having to do `Cuboid::new(1.0, 1.0, 1.0)` or
`Cuboid::from_size(Vec3::splat(1.0))` when there should be a much easier
way to do this.
## Solution
- Implemented a `from_length()` method that only takes in a single
float, and constructs a primitive of equal size in all directions.
- Ex:
```rs
// These:
Cuboid::new(1.0, 1.0, 1.0);
Cuboid::from_size(Vec3::splat(1.0));
// Are equivalent to this:
Cuboid::from_length(1.0);
```
- For the rest of the changed primitives:
```rs
Rectangle::from_length(1.0);
Plane3d::default().mesh().from_length(1.0);
```
# Objective
Fixes#11908
## Solution
- Remove the `naga_oil` dependency from `bevy_pbr`.
- We were doing a little dance to disable `glsl` support on not-wasm, so
incorporate that dance into `bevy_render`'s `Cargo.toml`.
# Objective
- Being able to build for WebGPU
```
error[E0061]: this function takes 1 argument but 3 arguments were supplied
--> .cargo/registry/src/index.crates.io-6f17d22bba15001f/wgpu-0.19.1/src/backend/webgpu.rs:375:22
|
375 | let mut mapped = web_sys::GpuDepthStencilState::new(
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
376 | map_compare_function(desc.depth_compare),
| ---------------------------------------- unexpected argument of type `GpuCompareFunction`
377 | desc.depth_write_enabled,
| ------------------------ unexpected argument of type `bool`
|
note: associated function defined here
--> .cargo/registry/src/index.crates.io-6f17d22bba15001f/web-sys-0.3.68/src/features/gen_GpuDepthStencilState.rs:27:12
|
27 | pub fn new(format: GpuTextureFormat) -> Self {
| ^^^
help: remove the extra arguments
|
376 - map_compare_function(desc.depth_compare),
376 + map_texture_format(desc.format),
|
error[E0061]: this function takes 1 argument but 2 arguments were supplied
--> .cargo/registry/src/index.crates.io-6f17d22bba15001f/wgpu-0.19.1/src/backend/webgpu.rs:1693:13
|
1693 | web_sys::GpuVertexState::new(desc.vertex.entry_point, &module.0);
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^ -------------------------
| |
| unexpected argument of type `&str`
| help: remove the extra argument
|
note: associated function defined here
--> .cargo/registry/src/index.crates.io-6f17d22bba15001f/web-sys-0.3.68/src/features/gen_GpuVertexState.rs:27:12
|
27 | pub fn new(module: &GpuShaderModule) -> Self {
| ^^^
error[E0061]: this function takes 2 arguments but 3 arguments were supplied
--> .cargo/registry/src/index.crates.io-6f17d22bba15001f/wgpu-0.19.1/src/backend/webgpu.rs:1768:17
|
1768 | web_sys::GpuFragmentState::new(frag.entry_point, &module.0, &targets);
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ ---------------- -------- unexpected argument of type `&js_sys::Array`
| |
| expected `&GpuShaderModule`, found `&str`
|
= note: expected reference `&GpuShaderModule`
found reference `&str`
note: associated function defined here
--> .cargo/registry/src/index.crates.io-6f17d22bba15001f/web-sys-0.3.68/src/features/gen_GpuFragmentState.rs:27:12
|
27 | pub fn new(module: &GpuShaderModule, targets: &::wasm_bindgen::JsValue) -> Self {
| ^^^
help: remove the extra argument
|
1768 - web_sys::GpuFragmentState::new(frag.entry_point, &module.0, &targets);
1768 + web_sys::GpuFragmentState::new(/* &GpuShaderModule */, &module.0);
|
error[E0061]: this function takes 1 argument but 2 arguments were supplied
--> .cargo/registry/src/index.crates.io-6f17d22bba15001f/wgpu-0.19.1/src/backend/webgpu.rs:1793:13
|
1793 | web_sys::GpuProgrammableStage::new(desc.entry_point, &shader_module.0);
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ ------------------
| |
| unexpected argument of type `&str`
| help: remove the extra argument
|
note: associated function defined here
--> .cargo/registry/src/index.crates.io-6f17d22bba15001f/web-sys-0.3.68/src/features/gen_GpuProgrammableStage.rs:27:12
|
27 | pub fn new(module: &GpuShaderModule) -> Self {
| ^^^
error[E0599]: no method named `write_timestamp` found for struct `GpuCommandEncoder` in the current scope
--> .cargo/registry/src/index.crates.io-6f17d22bba15001f/wgpu-0.19.1/src/backend/webgpu.rs:2505:14
|
2503 | / encoder_data
2504 | | .0
2505 | | .write_timestamp(&query_set_data.0, query_index);
| | -^^^^^^^^^^^^^^^ method not found in `GpuCommandEncoder`
| |_____________|
|
Some errors have detailed explanations: E0061, E0599.
For more information about an error, try `rustc --explain E0061`.
```
## Solution
- `web-sys` doesn't follow semver for the WebGPU APIs as they are
unstable. Force using a compatible version
---------
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
# Objective
Fixes#11846
## Solution
Add a `synchronous_pipeline_compilation ` field to `RenderPlugin`,
defaulting to `false`.
Most of the diff is whitespace.
## Changelog
Added `synchronous_pipeline_compilation ` to `RenderPlugin` for
disabling async pipeline creation.
## Migration Guide
TODO: consider combining this with the guide for #11846
`RenderPlugin` has a new `synchronous_pipeline_compilation ` property.
The default value is `false`. Set this to `true` if you want to retain
the previous synchronous behavior.
---------
Co-authored-by: JMS55 <47158642+JMS55@users.noreply.github.com>
Co-authored-by: François <mockersf@gmail.com>
This represents when the user has configured `ClearColorConfig::None` in
their application. If the clear color is `None`, we will always `Load`
instead of attempting to clear the attachment on the first call.
Fixes#11883.
# Objective
The new render graph labels do not (and cannot) implement normal
Reflect, which breaks spawning scenes with cameras (including GLTF
scenes). Likewise, the new `CameraMainTextureUsages` also does not (and
cannot) implement normal Reflect because it uses `wgpu::TextureUsages`
under the hood.
Fixes#11852
## Solution
This implements minimal "reflect value" for `CameraRenderGraph` and
`CameraMainTextureUsages` and registers the types, which satisfies our
spawn logic.
Note that this _does not_ fix scene serialization for these types, which
will require more significant changes. We will especially need to think
about how (and if) "interned labels" will fit into the scene system. For
the purposes of 0.13, I think this is the best we can do. Given that
this serialization issue is prevalent throughout Bevy atm, I'm ok with
adding a couple more to the pile. When we roll out the new scene system,
we will be forced to solve these on a case-by-case basis.
---
## Changelog
- Implement Reflect (value) for `CameraMainTextureUsages` and
`CameraRenderGraph`, and register those types.
# Objective
#10644 introduced nice "statically typed" labels that replace the old
strings. I would like to propose some changes to the names introduced:
* `SubGraph2d` -> `Core2d` and `SubGraph3d` -> `Core3d`. The names of
these graphs have been / should continue to be the "core 2d" graph not
the "sub graph 2d" graph. The crate is called `bevy_core_pipeline`, the
modules are still `core_2d` and `core_3d`, etc.
* `Labels2d` and `Labels3d`, at the very least, should not be plural to
follow naming conventions. A Label enum is not a "collection of labels",
it is a _specific_ Label. However I think `Label2d` and `Label3d` is
significantly less clear than `Node2d` and `Node3d`, so I propose those
changes here. I've done the same for `LabelsPbr` -> `NodePbr` and
`LabelsUi` -> `NodeUi`
Additionally, #10644 accidentally made one of the Camera2dBundle
constructors use the 3D graph instead of the 2D graph. I've fixed that
here.
---
## Changelog
* Renamed `SubGraph2d` -> `Core2d`, `SubGraph3d` -> `Core3d`, `Labels2d`
-> `Node2d`, `Labels3d` -> `Node3d`, `LabelsUi` -> `NodeUi`, `LabelsPbr`
-> `NodePbr`
# Objective
After adding configurable exposure, we set the default ev100 value to
`7` (indoor). This brought us out of sync with Blender's configuration
and defaults. This PR changes the default to `9.7` (bright indoor or
very overcast outdoors), as I calibrated in #11577. This feels like a
very reasonable default.
The other changes generally center around tweaking Bevy's lighting
defaults and examples to play nicely with this number, alongside a few
other tweaks and improvements.
Note that for artistic reasons I have reverted some examples, which
changed to directional lights in #11581, back to point lights.
Fixes#11577
---
## Changelog
- Changed `Exposure::ev100` from `7` to `9.7` to better match Blender
- Renamed `ExposureSettings` to `Exposure`
- `Camera3dBundle` now includes `Exposure` for discoverability
- Bumped `FULL_DAYLIGHT ` and `DIRECT_SUNLIGHT` to represent the
middle-to-top of those ranges instead of near the bottom
- Added new `AMBIENT_DAYLIGHT` constant and set that as the new
`DirectionalLight` default illuminance.
- `PointLight` and `SpotLight` now have a default `intensity` of
1,000,000 lumens. This makes them actually useful in the context of the
new "semi-outdoor" exposure and puts them in the "cinema lighting"
category instead of the "common household light" category. They are also
reasonably close to the Blender default.
- `AmbientLight` default has been bumped from `20` to `80`.
## Migration Guide
- The increased `Exposure::ev100` means that all existing 3D lighting
will need to be adjusted to match (DirectionalLights, PointLights,
SpotLights, EnvironmentMapLights, etc). Or alternatively, you can adjust
the `Exposure::ev100` on your cameras to work nicely with your current
lighting values. If you are currently relying on default intensity
values, you might need to change the intensity to achieve the same
effect. Note that in Bevy 0.12, point/spot lights had a different hard
coded ev100 value than directional lights. In Bevy 0.13, they use the
same ev100, so if you have both in your scene, the _scale_ between these
light types has changed and you will likely need to adjust one or both
of them.
# Objective
Fix https://github.com/bevyengine/bevy/issues/11577.
## Solution
Fix the examples, add a few constants to make setting light values
easier, and change the default lighting settings to be more realistic.
(Now designed for an overcast day instead of an indoor environment)
---
I did not include any example-related changes in here.
## Changelogs (not including breaking changes)
### bevy_pbr
- Added `light_consts` module (included in prelude), which contains
common lux and lumen values for lights.
- Added `AmbientLight::NONE` constant, which is an ambient light with a
brightness of 0.
- Added non-EV100 variants for `ExposureSettings`'s EV100 constants,
which allow easier construction of an `ExposureSettings` from a EV100
constant.
## Breaking changes
### bevy_pbr
The several default lighting values were changed:
- `PointLight`'s default `intensity` is now `2000.0`
- `SpotLight`'s default `intensity` is now `2000.0`
- `DirectionalLight`'s default `illuminance` is now
`light_consts::lux::OVERCAST_DAY` (`1000.`)
- `AmbientLight`'s default `brightness` is now `20.0`
# Objective
- The current implementations for `&Visibility == Visibility` and
`Visibility == &Visibility` are ambiguous, so they raise a warning for
being unconditionally recursive.
- `TaskPool`'s `LOCAL_EXECUTOR` thread local calls a `const` constructor
in a non-`const` context.
## Solution
- Make `&Visibility == Visibility` and `Visibility == &Visibility`
implementations use `Visibility == Visibility`.
- Wrap `LocalExecutor::new` in a special `const` block supported by
[`thread_local`](https://doc.rust-lang.org/stable/std/macro.thread_local.html).
---
This lints were found by running:
```shell
$ cargo clippy --workspace
```
There are a few other warnings that were more complicated, so I chose
not to include them in this PR.
<details>
<summary>Here they are...</summary>
```shell
warning: function cannot return without recursing
--> crates/bevy_utils/src/cow_arc.rs:92:5
|
92 | / fn eq(&self, other: &Self) -> bool {
93 | | self.deref().eq(other.deref())
94 | | }
| |_____^
|
note: recursive call site
--> crates/bevy_utils/src/cow_arc.rs:93:9
|
93 | self.deref().eq(other.deref())
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
warning: method `get_path` is never used
--> crates/bevy_reflect/src/serde/de.rs:26:8
|
25 | trait StructLikeInfo {
| -------------- method in this trait
26 | fn get_path(&self) -> &str;
| ^^^^^^^^
|
= note: `#[warn(dead_code)]` on by default
warning: methods `get_path` and `get_field` are never used
--> crates/bevy_reflect/src/serde/de.rs:34:8
|
33 | trait TupleLikeInfo {
| ------------- methods in this trait
34 | fn get_path(&self) -> &str;
| ^^^^^^^^
35 | fn get_field(&self, index: usize) -> Option<&UnnamedField>;
| ^^^^^^^^^
```
The other warnings are fixed by #11865.
</details>
# Objective
It can sometimes be useful to combine several meshes into one. This
allows constructing more complex meshes out of simple primitives without
needing to use a 3D modeling program or entity hierarchies.
This could also be used internally to increase code reuse by using
existing mesh generation logic for e.g. circles and using that in
cylinder mesh generation logic to add the top and bottom of the
cylinder.
**Note**: This is *not* implementing CSGs (Constructive Solid Geometry)
or any boolean operations, as that is much more complex. This is simply
adding the mesh data of another mesh to a mesh.
## Solution
Add a `merge` method to `Mesh`. It appends the vertex attributes and
indices of `other` to `self`, resulting in a `Mesh` that is the
combination of the two.
For example, you could do this:
```rust
let mut cuboid = Mesh::from(shape::Box::default());
let mut cylinder = Mesh::from(shape::Cylinder::default());
let mut torus = Mesh::from(shape::Torus::default());
cuboid.merge(cylinder);
cuboid.merge(torus);
```
This would result in `cuboid` being a `Mesh` that also has the cylinder
mesh and torus mesh. In this case, they would just be placed on top of
each other, but by utilizing #11454 we can transform the cylinder and
torus to get a result like this:
https://github.com/bevyengine/bevy/assets/57632562/557402c6-b896-4aba-bd95-312e7d1b5238
This is just a single entity and a single mesh.
# Objective
Reduce the size of `bevy_utils`
(https://github.com/bevyengine/bevy/issues/11478)
## Solution
Move `EntityHash` related types into `bevy_ecs`. This also allows us
access to `Entity`, which means we no longer need `EntityHashMap`'s
first generic argument.
---
## Changelog
- Moved `bevy::utils::{EntityHash, EntityHasher, EntityHashMap,
EntityHashSet}` into `bevy::ecs::entity::hash` .
- Removed `EntityHashMap`'s first generic argument. It is now hardcoded
to always be `Entity`.
## Migration Guide
- Uses of `bevy::utils::{EntityHash, EntityHasher, EntityHashMap,
EntityHashSet}` now have to be imported from `bevy::ecs::entity::hash`.
- Uses of `EntityHashMap` no longer have to specify the first generic
parameter. It is now hardcoded to always be `Entity`.
# Objective
Loading some textures from the days of yonder give me errors cause the
mipmap level is 0
## Solution
Set a minimum of 1
## Changelog
Make mipmap level at least 1
This fixes a `FIXME` in `extract_meshes` and results in a performance
improvement.
As a result of this change, meshes in the render world might not be
attached to entities anymore. Therefore, the `entity` parameter to
`RenderCommand::render()` is now wrapped in an `Option`. Most
applications that use the render app's ECS can simply unwrap the
`Option`.
Note that for now sprites, gizmos, and UI elements still use the render
world as usual.
## Migration guide
* For efficiency reasons, some meshes in the render world may not have
corresponding `Entity` IDs anymore. As a result, the `entity` parameter
to `RenderCommand::render()` is now wrapped in an `Option`. Custom
rendering code may need to be updated to handle the case in which no
`Entity` exists for an object that is to be rendered.
# Objective
The deprecation message of `bevy::render::mesh::shape::Quad` says that
you should use `bevy_math`'s `Quad` instead. But it doesn't exist.
## Solution
Mention the correct primitive: `Rectangle`
# Objective
- Encoding many GPU commands (such as in a renderpass with many draws,
such as the main opaque pass) onto a `wgpu::CommandEncoder` is very
expensive, and takes a long time.
- To improve performance, we want to perform the command encoding for
these heavy passes in parallel.
## Solution
- `RenderContext` can now queue up "command buffer generation tasks"
which are closures that will generate a command buffer when called.
- When finalizing the render context to produce the final list of
command buffers, these tasks are run in parallel on the
`ComputeTaskPool` to produce their corresponding command buffers.
- The general idea is that the node graph will run in serial, but in a
node, instead of doing rendering work, you can add tasks to do render
work in parallel with other node's tasks that get ran at the end of the
graph execution.
## Nodes Parallelized
- `MainOpaquePass3dNode`
- `PrepassNode`
- `DeferredGBufferPrepassNode`
- `ShadowPassNode` (One task per view)
## Future Work
- For large number of draws calls, might be worth further subdividing
passes into 2+ tasks.
- Extend this to UI, 2d, transparent, and transmissive nodes?
- Needs testing - small command buffers are inefficient - it may be
worth reverting to the serial command encoder usage for render phases
with few items.
- All "serial" (traditional) rendering work must finish before parallel
rendering tasks (the new stuff) can start to run.
- There is still only one submission to the graphics queue at the end of
the graph execution. There is still no ability to submit work earlier.
## Performance Improvement
Thanks to @Elabajaba for testing on Bistro.

TLDR: Without shadow mapping, this PR has no impact. _With_ shadow
mapping, this PR gives **~40 more fps** than main.
---
## Changelog
- `MainOpaquePass3dNode`, `PrepassNode`, `DeferredGBufferPrepassNode`,
and each shadow map within `ShadowPassNode` are now encoded in parallel,
giving _greatly_ increased CPU performance, mainly when shadow mapping
is enabled.
- Does not work on WASM or AMD+Windows+Vulkan.
- Added `RenderContext::add_command_buffer_generation_task()`.
- `RenderContext::new()` now takes adapter info
- Some render graph and Node related types and methods now have
additional lifetime constraints.
## Migration Guide
`RenderContext::new()` now takes adapter info
- Some render graph and Node related types and methods now have
additional lifetime constraints.
---------
Co-authored-by: Elabajaba <Elabajaba@users.noreply.github.com>
Co-authored-by: François <mockersf@gmail.com>
# Objective
While profiling around to validate the results of #9172, I noticed that
`present_frames` can take a significant amount of time. Digging into the
cause, it seems like we're creating a new `QueryState` from scratch
every frame. This involves scanning the entire World's metadata instead
of just updating its view of the world.
## Solution
Use a `SystemState` argument to cache the `QueryState` to avoid this
construction cost.
## Performance
Against `many_foxes`, this seems to cut the time spent in
`present_frames` by nearly almost 2x. Yellow is this PR, red is main.

# Objective
#11431 and #11688 implemented meshing support for Bevy's new geometric
primitives. The next step is to deprecate the shapes in
`bevy_render::mesh::shape` and to later remove them completely for 0.14.
## Solution
Deprecate the shapes and reduce code duplication by utilizing the
primitive meshing API for the old shapes where possible.
Note that some shapes have behavior that can't be exactly reproduced
with the new primitives yet:
- `Box` is more of an AABB with min/max extents
- `Plane` supports a subdivision count
- `Quad` has a `flipped` property
These types have not been changed to utilize the new primitives yet.
---
## Changelog
- Deprecated all shapes in `bevy_render::mesh::shape`
- Changed all examples to use new primitives for meshing
## Migration Guide
Bevy has previously used rendering-specific types like `UVSphere` and
`Quad` for primitive mesh shapes. These have now been deprecated to use
the geometric primitives newly introduced in version 0.13.
Some examples:
```rust
let before = meshes.add(shape::Box::new(5.0, 0.15, 5.0));
let after = meshes.add(Cuboid::new(5.0, 0.15, 5.0));
let before = meshes.add(shape::Quad::default());
let after = meshes.add(Rectangle::default());
let before = meshes.add(shape::Plane::from_size(5.0));
// The surface normal can now also be specified when using `new`
let after = meshes.add(Plane3d::default().mesh().size(5.0, 5.0));
let before = meshes.add(
Mesh::try_from(shape::Icosphere {
radius: 0.5,
subdivisions: 5,
})
.unwrap(),
);
let after = meshes.add(Sphere::new(0.5).mesh().ico(5).unwrap());
```
# Objective
- Try not to drop the render world on the render thread, and drop the
main world after the render world.
- The render world has a drop check that will panic if it is dropped off
the main thread.
## Solution
- Keep track of where the render world is and wait for it to come back
when the channel resource is dropped.
---
## Changelog
- Wait for the render world when the main world is dropped.
## Migration Guide
- If you were using the pipelined rendering channels,
`MainToRenderAppSender` and `RenderToMainAppReceiver`, they have been
combined into the single resource `RenderAppChannels`.
---------
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
Co-authored-by: Friz64 <friz64@protonmail.com>
# Objective
Fix https://github.com/bevyengine/bevy/issues/11657
## Solution
Add a `ReflectKind` enum, add `Reflect::reflect_kind` which returns a
`ReflectKind`, and add `kind` method implementions to `ReflectRef`,
`ReflectMut`, and `ReflectOwned`, which returns a `ReflectKind`.
I also changed `AccessError` to use this new struct instead of it's own
`TypeKind` struct.
---
## Changelog
- Added `ReflectKind`, an enumeration over the kinds of a reflected type
without its data.
- Added `Reflect::reflect_kind` (with default implementation)
- Added implementation for the `kind` method on `ReflectRef`,
`ReflectMut`, and `ReflectOwned` which gives their kind without any
information, as a `ReflectKind`
# Objective
- Fixes#11740
## Solution
- Turned `Mesh::set_indices` into `Mesh::insert_indices` and added
related methods for completeness.
---
## Changelog
- Replaced `Mesh::set_indices(indices: Option<Indices>)` with
`Mesh::insert_indices(indices: Indices)`
- Replaced `Mesh::with_indices(indices: Option<Indices>)` with
`Mesh::with_inserted_indices(indices: Indices)` and
`Mesh::with_removed_indices()` mirroring the API for inserting /
removing attributes.
- Updated the examples and internal uses of the APIs described above.
## Migration Guide
- Use `Mesh::insert_indices` or `Mesh::with_inserted_indices` instead of
`Mesh::set_indices` / `Mesh::with_indices`.
- If you have passed `None` to `Mesh::set_indices` or
`Mesh::with_indices` you should use `Mesh::remove_indices` or
`Mesh::with_removed_indices` instead.
---------
Co-authored-by: François <mockersf@gmail.com>
# Objective
- System `create_surfaces` needs to happen before `prepare_windows` or
we lose one frame at startup
## Solution
- Specify the ordering, remove the set as it doesn't mean anything there
# Objective
Split up from #11007, fixing most of the remaining work for #10569.
Implement `Meshable` for `Cuboid`, `Sphere`, `Cylinder`, `Capsule`,
`Torus`, and `Plane3d`. This covers all shapes that Bevy has mesh
structs for in `bevy_render::mesh::shapes`.
`Cone` and `ConicalFrustum` are new shapes, so I can add them in a
follow-up, or I could just add them here directly if that's preferrable.
## Solution
Implement `Meshable` for `Cuboid`, `Sphere`, `Cylinder`, `Capsule`,
`Torus`, and `Plane3d`.
The logic is mostly just a copy of the the existing `bevy_render`
shapes, but `Plane3d` has a configurable surface normal that affects the
orientation. Some property names have also been changed to be more
consistent.
The default values differ from the old shapes to make them a bit more
logical:
- Spheres now have a radius of 0.5 instead of 1.0. The default capsule
is equivalent to the default cylinder with the sphere's halves glued on.
- The inner and outer radius of the torus are now 0.5 and 1.0 instead of
0.5 and 1.5 (i.e. the new minor and major radii are 0.25 and 0.75). It's
double the width of the default cuboid, half of its height, and the
default sphere matches the size of the hole.
- `Cuboid` is 1x1x1 by default unlike the dreaded `Box` which is 2x1x1.
Before, with "old" shapes:

Now, with primitive meshing:

I only changed the `3d_shapes` example to use primitives for now. I can
change them all in this PR or a follow-up though, whichever way is
preferrable.
### Sphere API
Spheres have had separate `Icosphere` and `UVSphere` structs, but with
primitives we only have one `Sphere`.
We need to handle this with builders:
```rust
// Existing structs
let ico = Mesh::try_from(Icophere::default()).unwrap();
let uv = Mesh::from(UVSphere::default());
// Primitives
let ico = Sphere::default().mesh().ico(5).unwrap();
let uv = Sphere::default().mesh().uv(32, 18);
```
We could add methods on `Sphere` directly to skip calling `.mesh()`.
I also added a `SphereKind` enum that can be used with the `kind`
method:
```rust
let ico = Sphere::default()
.mesh()
.kind(SphereKind::Ico { subdivisions: 8 })
.build();
```
The default mesh for a `Sphere` is an icosphere with 5 subdivisions
(like the default `Icosphere`).
---
## Changelog
- Implement `Meshable` and `Default` for `Cuboid`, `Sphere`, `Cylinder`,
`Capsule`, `Torus`, and `Plane3d`
- Use primitives in `3d_shapes` example
---------
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
# Objective
During my exploratory work on the remote editor, I found a couple of
types that were either not registered, or that were missing
`ReflectDefault`.
## Solution
- Added registration and `ReflectDefault` where applicable
- (Drive by fix) Moved `Option<f32>` registration to `bevy_core` instead
of `bevy_ui`, along with similar types.
---
## Changelog
- Fixed: Registered `FogSettings`, `FogFalloff`,
`ParallaxMappingMethod`, `OpaqueRendererMethod` structs for reflection
- Fixed: Registered `ReflectDefault` trait for `ColorGrading` and
`CascadeShadowConfig` structs
# Objective
- There are too many `NonSendMarker`
https://docs.rs/bevy/0.12.1/bevy/index.html?search=nonsendmarker
- There should be only one
## Solution
- Use the marker type from bevy_core in bevy_render
---
## Migration Guide
- If you were using `bevy::render::view::NonSendMarker` or
`bevy::render::view:🪟:NonSendMarker`, use
`bevy::core::NonSendMarker` instead
# Objective
- Change set of systems as I made a mistake in #11672
- Don't block main when not needed
- Fixes#11235
## Solution
- add a run condition so that the system won't run and block main if not
needed
# Objective
- Some places manually use a `bool` /`AtomicBool` to warn once.
## Solution
- Use the `warn_once` macro which internally creates an `AtomicBool`.
Downside: in some case the warning state would have been reset after
recreating the struct carrying the warn state, whereas now it will
always warn only once per program run (For example, if all
`MeshPipeline`s are dropped or the `World` is recreated for
`Local<bool>`/ a `bool` resource, which shouldn't happen over the course
of a standard `App` run).
---
## Changelog
### Removed
- `FontAtlasWarning` has been removed, but the corresponding warning is
still emitted.
# Objective
- Pipeline compilation is slow and blocks the frame
- Closes https://github.com/bevyengine/bevy/issues/8224
## Solution
- Compile pipelines in a Task on the AsyncComputeTaskPool
---
## Changelog
- Render/compute pipeline compilation is now done asynchronously over
multiple frames when the multi-threaded feature is enabled and on
non-wasm and non-macOS platforms
- Added `CachedPipelineState::Creating`
- Added `PipelineCache::block_on_render_pipeline()`
- Added `bevy_utils::futures::check_ready`
- Added `bevy_render/multi-threaded` cargo feature
## Migration Guide
- Match on the new `Creating` variant for exhaustive matches of
`CachedPipelineState`
# Objective
Fixes#11653
## Solution
- Just added the formats to the docstring, I played around with having
the format appear in the type somehow so that it didn't need to be
written manually in the docstring but it ended up being more trouble
than it was worth.
Co-authored-by: James Liu <contact@jamessliu.com>
Frustum computation is nontrivial amount of code private in
`update_frusta` system.
Make it public.
This is needed to decide which entities to spawn/despawn in `Update`
based on camera changes. But if `Update` also changed camera, frustum is
not yet recomputed.
Technically it is probably possible to run an iteration of
`update_frusta` system by a user in `Update` schedule after propagating
`GlobalTransform` to the cameras, but it is easier to just compute
frustum manually using API added in this PR.
Also replace two places where this code is used.
---------
Co-authored-by: vero <email@atlasdostal.com>