OK, here's my attempt at sprite flipping. There are a couple of points that I need review/help on, but I think the UX is about ideal:
```rust
.spawn(SpriteBundle {
material: materials.add(texture_handle.into()),
sprite: Sprite {
// Flip the sprite along the x axis
flip: SpriteFlip { x: true, y: false },
..Default::default()
},
..Default::default()
});
```
Now for the issues. The big issue is that for some reason, when flipping the UVs on the sprite, there is a light "bleeding" or whatever you call it where the UV tries to sample past the texture boundry and ends up clipping. This is only noticed when resizing the window, though. You can see a screenshot below.
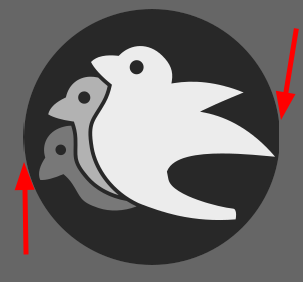
I am quite baffled why the texture sampling is overrunning like it is and could use some guidance if anybody knows what might be wrong.
The other issue, which I just worked around, is that I had to remove the `#[render_resources(from_self)]` annotation from the Spritesheet because the `SpriteFlip` render resource wasn't being picked up properly in the shader when using it. I'm not sure what the cause of that was, but by removing the annotation and re-organizing the shader inputs accordingly the problem was fixed.
I'm not sure if this is the most efficient way to do this or if there is a better way, but I wanted to try it out if only for the learning experience. Let me know what you think!
improve quality of text2d rendering
* remove coordinate tweaking in sprite-sheet shader
* fixes glyph shimmering of animated text
* reposition glyph before passing it to ab_glyph to normalize its rendering
The result of layout of sequence of glyphs causes individuals to have fractional positions, but since glyph renderings are reused for future instances of that glyph, this produces errors. This change accepts the errors but repositions the glyph to "0, 0" in an effort to get the cleanest possible rendering.
* only update global transforms when they (or their ancestors) have changed
* only update render resource nodes when they have changed (quality check plz)
* only update entity mesh specialization when mesh (or mesh component) has changed
* only update sprite size when changed
* remove stale bind groups
* fix setting size of loading sprites
* store unmatched render resource binding results
* reduce state changes
* cargo fmt + clippy
* remove cached "NoMatch" results when new bindings are added to RenderResourceBindings
* inline current_entity in world_builder
* try creating bind groups even when they havent changed
* render_resources_node: update all entities when resized
* fmt
Extend the Texture asset type to support 3D data
Textures are still loaded from images as 2D, but they can be reshaped
according to how the render pipeline would like to use them.
Also add an example of how this can be used with the texture2DArray uniform type.
* Remove cfg!(feature = "metal-auto-capture")
This cfg! has existed since the initial commit, but the corresponding
feature has never been part of Cargo.toml
* Remove unnecessary handle_create_window_events call
* Remove EventLoopProxyPtr wrapper
* Remove unnecessary statics
* Fix unrelated deprecation warning to fix CI