11461: Extract struct from enum variant filters generics r=jo-goro a=jo-goro
Fixes#11452.
This PR updates extract_struct_from_enum_variant. Extracting a struct `A` form an enum like
```rust
enum X<'a, 'b> {
A { a: &'a () },
B { b: &'b () },
}
```
will now be correctly generated as
```rust
struct A<'a> { a: &'a () }
enum X<'a, 'b> {
A(A<'a>),
B { b: &'b () },
}
```
instead of the previous
```rust
struct A<'a, 'b>{ a: &'a () } // <- should not have 'b
enum X<'a, 'b> {
A(A<'a, 'b>),
B { b: &'b () },
}
```
This also works for generic type parameters and const generics.
Bounds are also copied, however I have not yet implemented a filter for unneeded bounds. Extracting `B` from the following enum
```rust
enum X<'a, 'b: 'a> {
A { a: &'a () },
B { b: &'b () },
}
```
will be generated as
```rust
struct B<'b: 'a> { b: &'b () } // <- should be `struct B<'b> { b: &'b () }`
enum X<'a, 'b: 'a> {
A { a: &'a () },
B(B<'b>),
}
```
Extracting bounds with where clauses is also still not implemented.
Co-authored-by: Jonas Goronczy <goronczy.jonas@gmail.com>
11472: fix: visibility in impl items and pub(crate) to pub in extract_module r=feniljain a=feniljain
Should fix#11007 and #11443
Makes following changes:
- Removes visiblity modifiers from trait items
- Respect user given visibility
- Updated tests for the same
Co-authored-by: vi_mi <fkjainco@gmail.com>
Co-authored-by: vi_mi <49019259+feniljain@users.noreply.github.com>
11490: Correctly fix formatting doc tests with generics r=Veykril a=KarlWithK
Before the doc_test would be outputted like this:
```zsh
"Foo<T, U>::t"
```
However, this would cause problems with shell redirection. I've changed it
so when generics are involved we simply wrap the expression under quotes as so:
```zsh
"\"Foo<T, U>::t\""
```
Note:
At the cost of adding this, I had to allocate a new string via
`format!{}`. However, I argue this is alright as this for just for
outputting the name of the doc test.
The following tests have been changed:
```
runnables::tests::doc_test_type_params
runnables::tests::test_doc_runnables_impl_mod
runnables::tests::test_runnables_doc_test_in_impl
```
Closes https://github.com/rust-analyzer/rust-analyzer/issues/11489
Co-authored-by: KarlWithK <jocelinc60@outlook.com>
Co-authored-by: SeniorMars <jocelinc60@outlook.com>
11481: Display parameter names when hovering over a function pointer r=Veykril a=Vannevelj
Implements #11474
The idea is pretty straightforward: previously we constructed the hover based on just the parameter types, now we pass in the parameter names as well. I went for a quick-hit approach here but I expect someone will be able to point me to a better way of resolving the identifier.
I haven't figured out yet how to actually run my rust-analyzer locally so I can see it in action but the unit test indicates it should work.
Co-authored-by: Jeroen Vannevel <jer_vannevel@outlook.com>
11424: Pass required features to cargo when using run action r=Veykril a=WaffleLapkin
When using `F1`->`Rust Analyzer: Run` action on an `example`, pass its `required-features` to `cargo run`. This allows to run examples that were otherwise impossible to run with RA.
Co-authored-by: Maybe Waffle <waffle.lapkin@gmail.com>
Before the doc_test would be outputted like this:
"Foo<T, U>::t"
However, this would cause shells with shell redirection. I've changed it
so when generics are involved we simply wrap the expression under escape
chanters as so:
"\"Foo<T, U>::t\""
Note:
At the cost of adding this, I had to allocate a new string via
format!{}. However, I argue this is alright as this for just for
outputting the name of the doc test.
The following tests have been changed:
runnables::tests::doc_test_type_params
runnables::tests::test_doc_runnables_impl_mod
runnables::tests::test_runnables_doc_test_in_impl
11369: feat: Add type hint for keyword expression hovers r=Veykril a=danii
Adds the return type of keywords to tool-tips where it makes sense. This applies to: `if`, `else`, `match`, `loop`, `unsafe` and `await`. Thanks to `@Veykril` for sharing the idea of putting return type highlighting on other keywords!
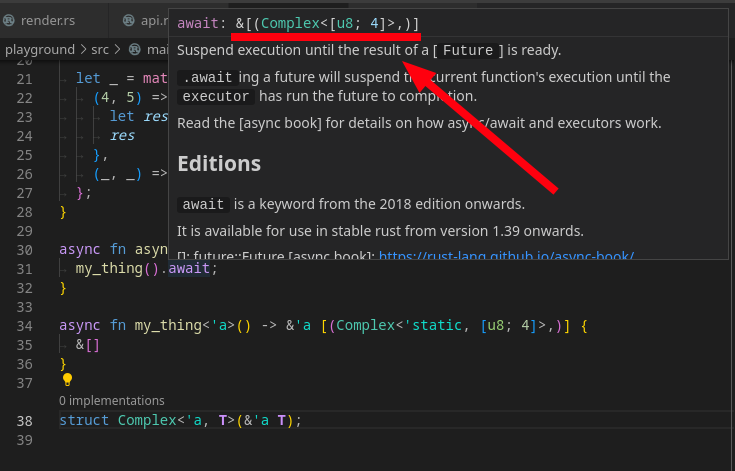
Closes#11359
Co-authored-by: Daniel Conley <himself@danii.dev>
11442: fix(rename): Use text range of a mod name after macro expansion r=Veykril a=tysg
Fixes#11417.
11460: fix: documentation of SsrParams r=Veykril a=nemethf
Fix#11429 by extending the documentation of SsrParms with the
mandatory field 'selections'. Copy its description from lsp_ext.rs.
Co-authored-by: Tianyi Song <42670338+tysg@users.noreply.github.com>
Co-authored-by: Felicián Németh <felician.nemeth@gmail.com>
Extracting a struct from an enum variant now filters out only the
generic parameters necessary for the new struct.
Bounds will be copied to the new struct, but unneeded ones are not
filtered out.
Extracting bounds in a where clause are still not implemented.
11444: feat: Fix up syntax errors in attribute macro inputs to make completion work more often r=flodiebold a=flodiebold
This implements the "fix up syntax nodes" workaround mentioned in #11014. It isn't much more than a proof of concept; I have only implemented a few cases, but it already helps quite a bit.
Some notes:
- I'm not super happy about how much the fixup procedure needs to interact with the syntax node -> token tree conversion code (e.g. needing to share the token map). This could maybe be simplified with some refactoring of that code.
- It would maybe be nice to have the fixup procedure reuse or share information with the parser, though I'm not really sure how much that would actually help.
Co-authored-by: Florian Diebold <flodiebold@gmail.com>
11437: [ide_completion] render if a function is async/const/unsafe in completion details r=Veykril a=jhgg
this change renders in the autocomplete detail, whether a function is async/const/unsafe.
i found myself wanting to know this information at a glance, so now it renders here:

Co-authored-by: Jake Heinz <jh@discordapp.com>
When using `F1`->`Rust Analyzer: Run` action on an `example`, pass its
`required-features` to `cargo run`. This allows to run examples that
were otherwise impossible to run with RA.
In code like this:
```rust
impl<T> Option<T> {
fn as_deref(&self) -> T::Target where T: Deref {}
}
```
when trying to resolve the associated type `T::Target`, we were only
looking at the bounds on the impl (where the type parameter is defined),
but the method can add additional bounds that can also be used to refer
to associated types. Hence, when resolving such an associated type, it's
not enough to just know the type parameter T, we also need to know
exactly where we are currently.
This fixes#11364 (beta apparently switched some bounds around).
11322: Extract function also extracts comments r=Vannevelj a=Vannevelj
Fixes#9011
The difficulty I came across is that the original assist works from the concept of a `ast::StmtList`, a node, but that does not allow me to (easily) represent comments, which are tokens. To combat this, I do a whole bunch of roundtrips: from the `ast::StmtList` I retrieve the `NodeOrToken`s it encompasses.
I then cast all `Node` ones back to a `Stmt` so I can apply indentation to it, after which it is again parsed as a `NodeOrToken`.
Lastly, I add a new `make::` api that accepts `NodeOrToken` rather than `StmtList` so we can write the comment tokens.
Co-authored-by: Jeroen Vannevel <jer_vannevel@outlook.com>
11182: fix: don't panic on seeing an unexpected offset r=Veykril a=dimbleby
Intended as a fix, or at least a sticking plaster, for #11081.
I have arranged that [offset()](1ba9a924d7/crates/ide_db/src/line_index.rs (L105-L107)) returns `Option<TextSize>` instead of going out of bounds; other changes are the result of following the compiler after doing this.
Perhaps there's still an issue here - I suppose the server and client have gotten out of sync and that probably shouldn't happen in the first place? I see that https://github.com/rust-analyzer/rust-analyzer/issues/10138#issuecomment-913727554 suggests what sounds like a more substantial fix which I think might be aimed in this direction. So perhaps that one should be left open to cover such things?
Meanwhile, I hope that not-crashing is a good improvement: and I can confirm that it works out just fine in the repro I have at #11081.
Co-authored-by: David Hotham <david.hotham@metaswitch.com>
This patch makes RA understand `#![recursion_limit = "N"]` annotations.
- `crate_limits` query is moved to `DefDatabase`
- `DefMap` now has `recursion_limit: Option<u32>` field
This allows fetching crate limits like `recursion_limit`. The
implementation is currently dummy and just returns the defaults.
Future work: Use this query instead of the hardcoded constant.
Future work: Actually implement this query by parsing
`#![recursion_limit = N]` attribute.
11281: ide: parallel prime caches r=jonas-schievink a=jhgg
cache priming goes brrrr... the successor to #10149
---
this PR implements a parallel cache priming strategy that uses a topological work queue to feed a pool of worker threads the crates to index in parallel.
## todo
- [x] should we keep the old prime caches?
- [x] we should use num_cpus to detect how many cpus to use to prime caches. should we also expose a config for # of worker CPU threads to use?
- [x] something is wonky with cancellation, need to figure it out before this can merge.
Co-authored-by: Jake Heinz <jh@discordapp.com>
Fixes#8607.
This commit changes the auto-import functionality and causes it to add
imports after any leading comments, which are commonly license headers.
This does not affect comments on items as they're considered part of the
item itself and not separate.