fix: make make_body respect comments in extract_function
Possible fix for #13621
### Points to help in review:
- Earlier we were only considering statements in a block expr and hence comments were being ignored, now we handle tokens hence making it aware of comments and then preserving them using `hacky_block_expr_with_comments`
Seems like I am not able to attach output video, github is glitching for it :(
fix: breaking snippets on typed incomplete suggestions
Possible fix for #7929
Fix the case where if a user types `&&42.o`, snippet completion was still applying &&Ok(42). Note this was fixed previously on `&&42.` but this still remained a problem for this case
Previous relevant PR: #13517
### Points to help in review:
- The main problem why everything broke on adding an extra `o` was, earlier `dot_receiver` was `42.` which was a `LITERAL` but now `42.o` becomes a `FIELD_EXPR`
- Till now `include_references` was just checking for parent of `LITERAL` and if it was a `REF_EXPR`, but now we consider `FIELD_EXPR` and traverse all of them, finally to reach `REF_EXPR`. If `REF_EXPR` is not found we just return the original `initial_element`
- We are constructing a new node during `include_references` because if we rely on `dot_receiver` solely we would get `&&42.o` to be replaced with, but we want `&&42` to be replaced with
### Output Video:
https://user-images.githubusercontent.com/49019259/205420166-efbdef78-5b3a-4aef-ab4b-d892dac056a0.mov
Hope everything I wrote makes sense 😅
Also interestingly previous PR's number was `13517` and this PR's number is `13715`, nicee
feat: allow unwrap block in let initializers
Possible fix for #13679
### Points to help in review:
- I just added a parent case for let statements and it seems everything else was in place already, so turned out to be a small fix
fix: add fallback case in generated `PartialEq` impl
Partially fixes#13727.
When generating `PartialEq` implementations for enums, the original code can already generate the following fallback case:
```rs
_ => std::mem::discriminant(self) == std::mem::discriminant(other),
```
However, it has been suppressed in the following example for no good reason:
```rs
enum Either<T, U> {
Left(T),
Right(U),
}
impl<T, U> PartialEq for Either<T, U> {
fn eq(&self, other: &Self) -> bool {
match (self, other) {
(Self::Left(l0), Self::Left(r0)) => l0 == r0,
(Self::Right(l0), Self::Right(r0)) => l0 == r0,
// _ => std::mem::discriminant(self) == std::mem::discriminant(other),
// ^ this completes the match arms!
}
}
}
```
This PR has removed that suppression logic.
~~Of course, the PR could have suppressed the fallback case generation for single-variant enums instead, but I believe that this case is quite rare and should be caught by `#[warn(unreachable_patterns)]` anyway.~~
After this fix, when the enum has >1 variants, the following fallback arm will be generated :
* `_ => false,` if we've already gone through every case where the variants of `self` and `other` match;
* The original one (as stated above) in other cases.
---
Note: The code example is still wrong after the fix due to incorrect trait bounds.
fix: normalize projection after discarding free `BoundVar`s in RPIT
Fixes#13307
When we lower the return type of a function, it may contain free `BoundVar`s in `OpaqueType`'s substitution, which would cause panic during canonicalization as part of projection normalization. Those `BoundVar`s are irrelevant in this context and will be discarded, and we should defer projection normalization until then.
fix: only shift `BoundVar`s that come from outside lowering context
Fixes#13734
There are some free functions `TyLoweringContext` methods call, which do not know anything about current binders in scope. We need to shift in the `BoundVar`s in substitutions that we get from them (#4952), but not those we get from `TyLoweringContext` methods.
Compute data layout of types
cc #4091
Things that aren't working:
* Closures
* Generators (so no support for `Future` I think)
* Opaque types
* Type alias and associated types which may need normalization
Things that show wrong result:
* ~Enums with explicit discriminant~
* SIMD types
* ~`NonZero*` and similar standard library items which control layout with special attributes~
At the user level, I didn't put much work, since I wasn't confident about what is the best way to present this information. Currently it shows size and align for ADTs, and size, align, offset for struct fields, in the hover, similar to clangd. I used it some days and I feel I liked it, but we may consider it too noisy and move it to an assist or command.
The old value was for the old chalk-engine solver, nowadays the newer chalk-recursive solver is used.
The new solver currently uses fuel a bit more quickly, so a higher value is needed.
Running analysis-stats showed that a value of 100 increases the amount of unknown types,
while for a value of 1000 it's staying mostly the same.
Add `move_const_to_impl` assist
Closes#13277
For the initial implementation, this assist:
- only applies to inherent impl. Much as we can *technically* provide this assist for default impl in trait definitions, it'd be complicated to get it right.
- may break code when the const's name collides with an item of a trait the self type implements.
Comments in the code explain those caveats in a bit more detail.
Fix the case where if a user types `&&42.o`, snippet completion
was still applying &&Ok(42). Note this was fixed previously
on `&&42.` but this still remained a problem for this case
Don't show runnable code lenses in libraries outside of the workspace
Addresses #13664. For now I'm just disabling runnable code lenses since the ones that display the number of references and implementations do work correctly with external code.
Also made a tiny TypeScript change to use the typed `sendNotification` overload.
fix: check tail expressions more precisely in `extract_function`
Fixes#13620
When extracting expressions with control flows into a function, we can avoid wrapping tail expressions in `Option` or `Result` when they are also tail expressions of the container we're extracting from (see #7840, #9773). This is controlled by `ContainerInfo::is_in_tail`, but we've been computing it by checking if the tail expression of the range to extract is contained in the container's syntactically last expression, which may be a block that contains both tail and non-tail expressions (e.g. in #13620, the range to be extracted is not a tail expression but we set the flag to true).
This PR tries to compute the flag as precise as possible by utilizing `for_each_tail_expr()` (and also moves the flag to `Function` struct as it's more of a property of the function to be extracted than of the container).
Mega-sync from `rust-lang/rust`
This essentially implements `@oli-obk's` suggestion here https://github.com/rust-lang/rust-analyzer/pull/13459#issuecomment-1297285607, with `@eddyb's` help.
This PR is equivalent to 14 syncs (back and forth) between `rust-lang/rust` and `rust-lang/rust-analyzer`.
Working from this list (from bottom to top):
```
(x) a2a1d9954⬆️ rust-analyzer
(x) 79923c382⬆️ rust-analyzer
(x) c60b1f641⬆️ rust-analyzer
(x) 8807fc4cc⬆️ rust-analyzer
(x) a99a48e78⬆️ rust-analyzer
(x) 4f55ebbd4⬆️ rust-analyzer
(x) f5fde4df4⬆️ rust-analyzer
(x) 459bbb422⬆️ rust-analyzer
(x) 65e1dc4d9⬆️ rust-analyzer
(x) 3e358a682⬆️ rust-analyzer
(x) 31519bb39⬆️ rust-analyzer
(x) 8231fee46⬆️ rust-analyzer
(x) 22c8c9c40⬆️ rust-analyzer
(x) 9d2cb42a4⬆️ rust-analyzer
```
(This listed was assembled by doing a `git subtree push`, which made a branch, and looking at the new commits in that branch, picking only those that were `⬆️ rust-analyzer` commits)
We used the following commands to simulate merges in both directions:
```shell
TO_MERGE=22c8c9c40 # taken from the list above, bottom to top
git merge --no-edit --no-ff $TO_MERGE
git merge --no-edit --no-ff $(git -C ../rust log --pretty=format:'%cN | %s | %ad => %P' | rg -m1 -F "$(git show --no-patch --pretty=format:%ad $TO_MERGE)" | tee /dev/stderr | rg '.* => \S+ (\S+)$' --replace '$1')
```
We encountered no merge conflicts that Git wasn't able to solve by doing it this way.
Here's what the commit graph looks like (as shown in the Git Lens VSCode extension):
<img width="1345" alt="image" src="https://user-images.githubusercontent.com/7998310/203984523-7c1a690a-8224-416c-8015-ed6e49667066.png">
This PR closes#13459
## Does this unbreak `rust->ra` syncs?
Yes, here's how we tried:
In `rust-analyzer`:
* check out `subtree-fix` (this PR's branch)
* make a new branch off of it: `git checkout -b subtree-fix-merge-test`
* simulate this PR getting merged with `git merge master`
In `rust`:
* pull latest master
* make a new branch: `git checkout -b test-change`
* mess with rust-analyzer (I added a comment to `src/tools/rust-analyzer/Cargo.toml`)
* commit
* run `git subtree push -P src/tools/rust-analyzer ra-local final-sync` (this follows the [Clippy sync guide](https://doc.rust-lang.org/nightly/clippy/development/infrastructure/sync.html))
This created a `final-sync` branch in `rust-analyzer`.
In `rust-analyzer`:
* `git merge --no-ff final-sync` (this follows the [Clippy sync guide](https://doc.rust-lang.org/nightly/clippy/development/infrastructure/sync.html))
Now `git log` in `rust-analyzer` shows this:
```
commit 460128387e46ddfc2b95921b2d7f6e913a3d2b9f (HEAD -> subtree-fix-merge-test)
Merge: 0513fc02a 9ce6a734f
Author: Amos Wenger <amoswenger@gmail.com>
Date: Fri Nov 25 13:28:24 2022 +0100
Merge branch 'final-sync' into subtree-fix-merge-test
commit 0513fc02a08ea9de952983624bd0a00e98044b36
Merge: 38c98d1ff6918009fe
Author: Amos Wenger <amoswenger@gmail.com>
Date: Fri Nov 25 13:28:02 2022 +0100
Merge branch 'master' into subtree-fix-merge-test
commit 9ce6a734f37ef8e53689f1c6f427a9efafe846bd (final-sync)
Author: Amos Wenger <amoswenger@gmail.com>
Date: Fri Nov 25 13:26:26 2022 +0100
Mess with rust-analyzer just for fun
```
And `git diff 0513fc02a08ea9de952983624bd0a00e98044b36` shows this:
```patch
diff --git a/Cargo.toml b/Cargo.toml
index 286ef1e7d..c9e24cd19 100644
--- a/Cargo.toml
+++ b/Cargo.toml
`@@` -32,3 +32,5 `@@` debug = 0
# ungrammar = { path = "../ungrammar" }
# salsa = { path = "../salsa" }
+
+# lol, hi
```
## Does this unbreak `ra->rust` syncs?
Yes, here's how we tried.
From `rust`:
* `git checkout -b sync-from-ra`
* `git subtree pull -P src/tools/rust-analyzer ra-local subtree-fix-merge-test` (this is adapted from the [Clippy sync guide](https://doc.rust-lang.org/nightly/clippy/development/infrastructure/sync.html#performing-the-sync-from-clippy-to-rust-langrust), you would normally use `ra-upstream master` but we're simulating things here)
A commit editor pops up, there was no merge conflicts.
## How do we prevent this from happening again?
Like `@bjorn3` said in https://github.com/rust-lang/rust-analyzer/pull/13459#issuecomment-1293587848
> Whenever syncing from rust-analyzer -> rust you have to immediately sync the merge commit from rust -> rust-analyzer to prevent merge conflicts in the future.
But if we get it wrong again, at least now we have a not-so-painful way to fix it.
Improve goto declaration
Closes https://github.com/rust-lang/rust-analyzer/issues/13599
- goto decl now goes to assoc items of trait declarations over the items of trait implementations
- goto decl now goes to the field declaration (opposed to goto def which shows both the field decl and binding created/local being used)
- also adds back the goto definition fallback that seems to have been dropped at some point.
feat: adds hover hint to ".." in record pattern
Hovering on the "rest" pattern in struct destructuring,
```rust
struct Baz {
a: u32,
b: u32,
c: u32,
d: u32
}
let Baz { a, b, ..$0} = Baz { a: 1, b: 2, c: 3, d: 4 };
```
shows:
```
.., c: u32, d: u32
```
Currently only works with struct patterns.
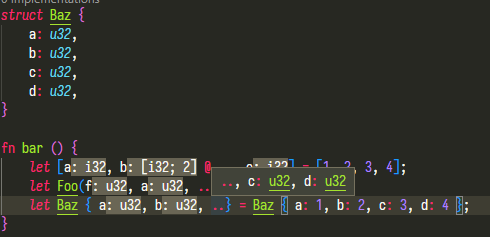
Add assist to generate trait impl's
resolves#13553
This pull request adds a `generate_trait_impl` assist, which generates trait impl's for a type. It is almost the same as the one to generate impl's and I also reduced the trigger range to only outside the `RecordFieldList`. Also moved all the tests into separate test functions. A few of the old tests seemed redundant, so I didn't port them.
Fix: Handle empty `checkOnSave/target` values
This fixes a regression introduced by #13290, in which failing to set `checkOnSave/target` (or `checkOnSave/targets`) would lead to an invalid config.
[Fixes#13660]
Support multiple targets for checkOnSave (in conjunction with cargo 1.64.0+)
This PR adds support for the ability to pass multiple `--target` flags when using
`cargo` 1.64.0+.
## Questions
I needed to change the type of two configurations options, but I did not plurialize the names to
avoid too much churn, should I ?
## Zulip thread
https://rust-lang.zulipchat.com/#narrow/stream/185405-t-compiler.2Frust-analyzer/topic/Issue.2013282.20.28supporting.20multiple.20targets.20with.201.2E64.2B.29
## Example
To see it working, on a macOS machine:
```sh
$ cd /tmp
$ cargo new cargo-multiple-targets-support-ra-test
$ cd !$
$ mkdir .cargo
$ echo '
[build]
target = [
"aarch64-apple-darwin",
"x86_64-apple-darwin",
]
' > .cargo/config.toml
$ echo '
fn main() {
#[cfg(all(target_arch = "aarch64", target_os = "macos"))]
{
let a = std::fs::read_to_string("/tmp/test-read");
}
#[cfg(all(target_arch = "x86_64", target_os = "macos"))]
{
let a = std::fs::read_to_string("/tmp/test-read");
}
#[cfg(all(target_arch = "x86_64", target_os = "windows"))]
{
let a = std::fs::read_to_string("/tmp/test-read");
}
}
' > src/main.rs
# launch your favorite editor with the version of RA from this PR
#
# You should see warnings under the first two `let a = ...` but not the third
```
## Screen
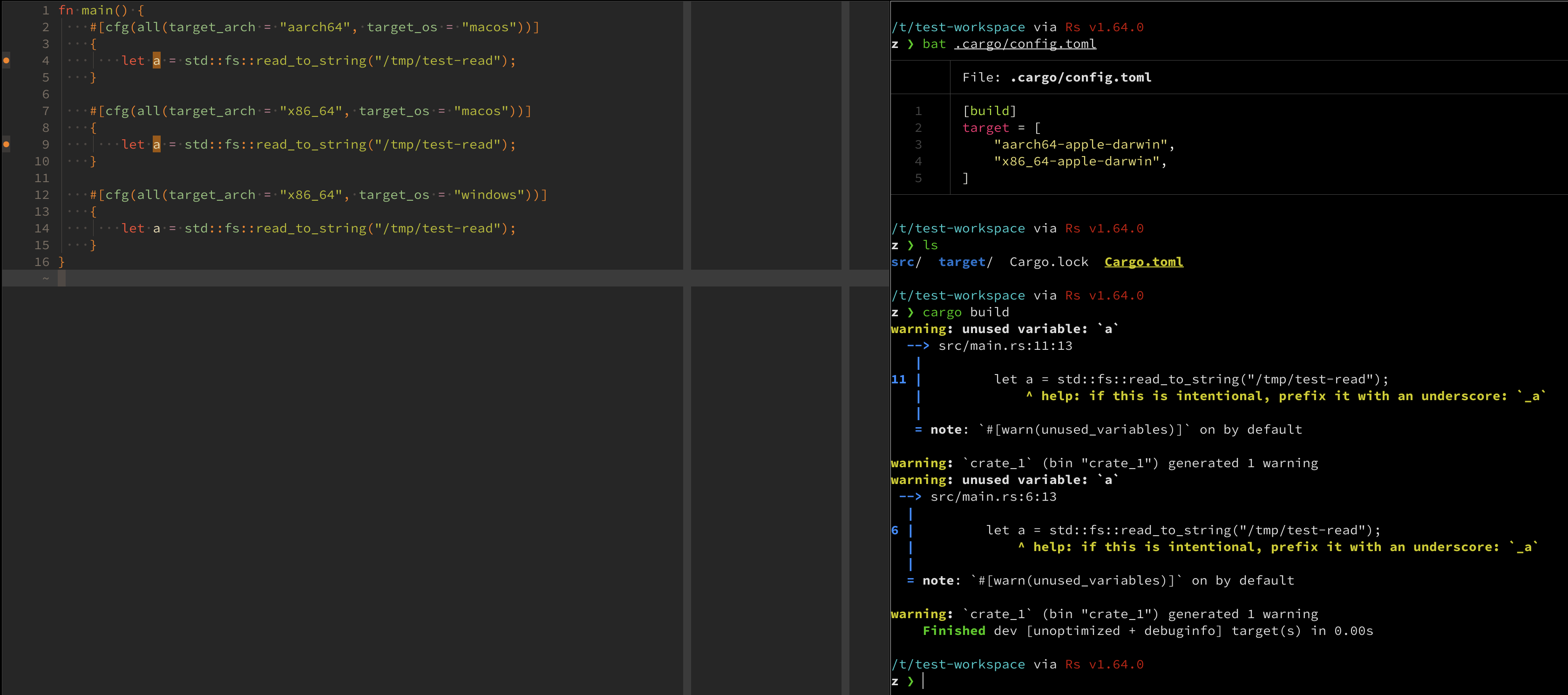
Helps with #13282
fix: format expression parsing edge-cases
- Handle positional arguments with formatting options (i.e. `{:b}`). Previously copied `:b` as an argument, producing broken code.
- Handle indexed positional arguments (`{0}`) ([reference](https://doc.rust-lang.org/std/fmt/#positional-parameters)). Previously copied over `0` as an argument.
Note: the assist also breaks when named arguments are used (`"{name}$0", name = 2 + 2` is converted to `"{}"$0, name`. I'm working on fix for that as well.
Update several crates to bring support for the new Tier 3 Windows tar…
`cargo t` has passed on Windows 11 with both `x86_64-pc-windows-gnu` and `x86_64-pc-windows-gnullvm` targets.
fix: check visibility of each path segment
Upon path resolution, we have not been checking if every def pointed to by each segment of the path is visible from the original module. This leads to incorrect import resolutions, in particular when one uses glob imports and names collide.
There is decent amount of changes in this PR because:
- some of our tests were not correct in terms of visibility
- I left several basic nameres tests as-is (with expect test updated) since I thought it would be nice to ensure we don't resolve defs that are not visible.
- `fix_visibility` assist relied on `Semantics::resolve_path()`, which uses the name resolution procedure I'm fixing and wouldn't be able to "see through" the items with strict visibility with this patch
The first commit is the gist of the fix itself.
Fixes#10991Fixes#11473Fixes#13252
Fix `tt::Punct`'s spacing calculation
Fixes#13499
We currently set a `tt::Punct`'s spacing to `Spacing::Joint` unless its next token is a trivia (i.e. whitespaces or comment). As I understand it, rustc only [sets `Spacing::Joint` if the next token is an operator](5b3e909075/compiler/rustc_parse/src/lexer/tokentrees.rs (L77-L78)) and we should follow it to guarantee the consistent behavior of proc macros.
Fix the length displayed for byte string literals with escaped newlines
This is a fix for the problem I reported earlier: "the length of byte strings containing escaped newlines is displayed two bytes longer when the first escaped character is a newline".
I would appreciate it if you could review the fix.
Many thanks.
Closes#13567
The length of byte strings containing escaped newlines is displayed two
bytes longer when the first escaped character is a newline.
This is due to a small bug in handling the first escaped newline in
string literals.
Closes#13567
Feat: extracted method from trait impl is placed in existing impl
**Before**
https://user-images.githubusercontent.com/1759192/183872883-3b0eafd2-d1dc-440e-9e66-38e3372f8b64.mp4
**After**
https://user-images.githubusercontent.com/1759192/183875769-87f34c7d-52f0-4dfc-9766-f591ee738ebb.mp4
Previously, when triggering a method extraction from within an impl trait block, then this would always create a new impl block for
the struct, even if there already is one. Now, if there is already an existing trait-less impl block, then it'll put the extracted method in there.
**Caveats**:
- It currently requires the target impl block to be non-empty. This limitation is because the current architecture takes a `node_to_insert_after` as reference for where to insert the extracted function. An empty impl block doesn't have such a reference node, since it's empty. It seems that supporting this requires a much larger and more complex change.
- This is my first contribution in rust, so apologies for any beginner mistakes.
internal: Migrate `ide_assists::utils` and `ide_assists::handlers` to use format arg captures (part 1)
This not only serves as making future migration to mutable syntax trees easier, it also finds out what needs to be migrated in the first place.
~~Aside from the first commit, subsequent commits are structured to only deal with one file/handler at a time.~~
This is the first of 3 PRs, migrating:
Utils:
- `gen_trait_fn_body`
- `render_snippet`
- `ReferenceConversion`
- `convert_type`
- `getter`
Handlers:
- `add_explicit_type`
- `add_return_type`
- `add_turbo_fish`
- `apply_demorgan`
- `auto_import`
- `convert_comment_block`
- `convert_integer_literal`
- `convert_into_to_from`
- `convert_iter_for_each_to_for`
- `convert_let_else_to_match`
- `convert_tuple_struct_to_named_struct`
- `convert_two_arm_bool_match_to_matches_macro`
- `destructure_tuple_binding`
- `extract_function`
- `extract_module`
- `extract_struct_from_enum_variant`
- `extract_type_alias`
- `extract_variable`
- `fix_visibility`
scip: Generate symbols for local crates.
Consider something like:
```
// a.rs
pub struct Foo { .. } // Foo is "local 1"
fn something() {
crate:🅱️:Bar::new() // Bar is "local 1", but of "b.rs"
}
// b.rs
pub struct Bar { .. } // "local 1"
```
Without this there's no way to disambiguate whether "local 1" references "Bar" or "Foo".
feat: add config for inserting must_use in `generate_enum_as_method`
Should fix#13312
Didn't add a test because I was not sure on how to add test for a specific configuration option, tried to look for the usages for other `AssistConfig` variants but couldn't find any in `tests`. If there is a way to test this, do point me towards it.
I tried to extract the formatting string as a common `template_string` and only have if-else for that, but it didn't compile :(
Also it seems these tests are failing:
```
test config::tests::generate_config_documentation ... FAILED
test config::tests::generate_package_json_config ... FAILED
```
Can you also point me to how to correct these 😅 ( I guess there is some command to automatically generate these? )
fix: make custom expr prefix completions to understand refs
Possible fix of #7929
While reviewing the postfix completion code I saw that while calling `add_custom_postfix_completions` we were doing it under the part where reference was not taken into consideration, but as we are only adding postfix completions with `Expr` scope ( [source](ba28e19b78/crates/ide-completion/src/completions/postfix.rs (L272)) )
I shifted the `add_custom_postfix_completions` call to part where references are considered
I am not sure if this is the correct fix or I am understanding the problem exactly but this small move seemed to have fixed the issue :)
feat: show signature help when calling generic types implementing `FnOnce`
This queries chalk for the `FnOnce` impl of callees and takes argument and return types from there, making generic `Callable`s available to the IDE. This makes signature help work for them, and potentially allows other features to take generic callables into account in the future.
fix: disregard type variable expectation for if expressions
Fixes#13522
As [the comment](8142d1f606/crates/hir-ty/src/infer.rs (L1087-L1090)) on `Expectation::adjust_for_branches` explains:
> If the expected type is just a type variable, then don't use an expected type. Otherwise, we might write parts of the type when checking the 'then' block which are incompatible with the 'else' branch.
Note that we already use it in match expressions. I've added tests for them too nevertheless.
Switch to upstream `positionEncoding`
Closes#13481
This drops support for the custom extension, but that's probably fine.
Draft because it's not tested yet.
fix: Test all generic args for trait when finding matching impl
Addresses https://github.com/rust-lang/rust-analyzer/pull/13463#issuecomment-1287816680
When finding matching impl for a trait method, we've been testing the unifiability of self type. However, there can be multiple impl of a trait for the same type with different generic arguments for the trait. This patch takes it into account and tests the unifiability of all type arguments for the trait (the first being the self type) thus enables rust-analyzer to find the correct impl even in such cases.
Support const generics for builtin derive macro
Fixes#13121
We have been treating every generic parameter as type parameter during builtin derive macro expansion. This patch adds support for const generics in such expansions.
feat: add multiple getters mode in `generate_getter`
This commit adds two modes to generate_getter action.
First, the plain old working on single fields.
Second, working on a selected range of fields.
Should partially solve #13246
If this gets approved will create a separate PR for setters version of the same
### Points to help in review:
- `generate_getter_from_record_info` contains code which is mostly taken from assist before refactor
- Same goes for `parse_record_fields`
- There are changes in other assists, as one of the methods in utils named `find_struct_impl` is changed, before it used to accept a single `fn_name`, now it takes a list of function names to check against. All old impls are updated to create a small list to pass their single element.
### Assumptions:
- If any of the fields have an implementation, the action will quit.