mirror of
https://github.com/bevyengine/bevy
synced 2024-12-21 02:23:08 +00:00
Adopted #8266, so copy-pasting the description from there: # Objective Support the KHR_texture_transform extension for the glTF loader. - Fixes #6335 - Fixes #11869 - Implements part of #11350 - Implements the GLTF part of #399 ## Solution As is, this only supports a single transform. Looking at Godot's source, they support one transform with an optional second one for detail, AO, and emission. glTF specifies one per texture. The public domain materials I looked at seem to share the same transform. So maybe having just one is acceptable for now. I tried to include a warning if multiple different transforms exist for the same material. Note the gltf crate doesn't expose the texture transform for the normal and occlusion textures, which it should, so I just ignored those for now. (note by @janhohenheim: this is still the case) Via `cargo run --release --example scene_viewer ~/src/clone/glTF-Sample-Models/2.0/TextureTransformTest/glTF/TextureTransformTest.gltf`: 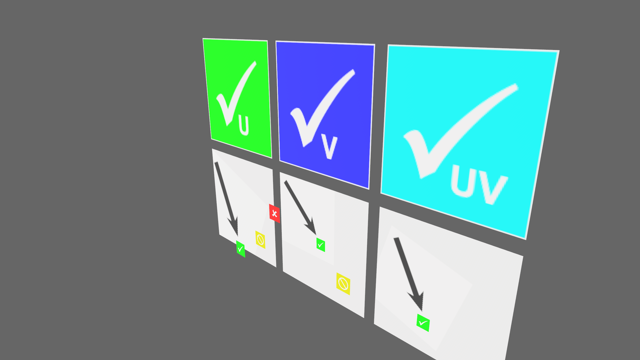 ## Changelog Support for the [KHR_texture_transform](https://github.com/KhronosGroup/glTF/tree/main/extensions/2.0/Khronos/KHR_texture_transform) extension added. Texture UVs that were scaled, rotated, or offset in a GLTF are now properly handled. --------- Co-authored-by: Al McElrath <hello@yrns.org> Co-authored-by: Kanabenki <lucien.menassol@gmail.com>
45 lines
1.5 KiB
WebGPU Shading Language
45 lines
1.5 KiB
WebGPU Shading Language
#define_import_path bevy_sprite::mesh2d_functions
|
|
|
|
#import bevy_sprite::{
|
|
mesh2d_view_bindings::view,
|
|
mesh2d_bindings::mesh,
|
|
}
|
|
#import bevy_render::maths::{affine3_to_square, mat2x4_f32_to_mat3x3_unpack}
|
|
|
|
fn get_model_matrix(instance_index: u32) -> mat4x4<f32> {
|
|
return affine3_to_square(mesh[instance_index].model);
|
|
}
|
|
|
|
fn mesh2d_position_local_to_world(model: mat4x4<f32>, vertex_position: vec4<f32>) -> vec4<f32> {
|
|
return model * vertex_position;
|
|
}
|
|
|
|
fn mesh2d_position_world_to_clip(world_position: vec4<f32>) -> vec4<f32> {
|
|
return view.view_proj * world_position;
|
|
}
|
|
|
|
// NOTE: The intermediate world_position assignment is important
|
|
// for precision purposes when using the 'equals' depth comparison
|
|
// function.
|
|
fn mesh2d_position_local_to_clip(model: mat4x4<f32>, vertex_position: vec4<f32>) -> vec4<f32> {
|
|
let world_position = mesh2d_position_local_to_world(model, vertex_position);
|
|
return mesh2d_position_world_to_clip(world_position);
|
|
}
|
|
|
|
fn mesh2d_normal_local_to_world(vertex_normal: vec3<f32>, instance_index: u32) -> vec3<f32> {
|
|
return mat2x4_f32_to_mat3x3_unpack(
|
|
mesh[instance_index].inverse_transpose_model_a,
|
|
mesh[instance_index].inverse_transpose_model_b,
|
|
) * vertex_normal;
|
|
}
|
|
|
|
fn mesh2d_tangent_local_to_world(model: mat4x4<f32>, vertex_tangent: vec4<f32>) -> vec4<f32> {
|
|
return vec4<f32>(
|
|
mat3x3<f32>(
|
|
model[0].xyz,
|
|
model[1].xyz,
|
|
model[2].xyz
|
|
) * vertex_tangent.xyz,
|
|
vertex_tangent.w
|
|
);
|
|
}
|