mirror of
https://github.com/bevyengine/bevy
synced 2024-11-26 22:50:19 +00:00
# Objective Lets say we need to rotate stretched object for this purpose we can created stretched `Child` and add as child to `Parent`, later we can rotate `Parent`, `Child` in this situation should rotate keeping it form, it is not the case with `SpriteBundle` currently. If you try to do it with `SpriteBundle` it will deform. ## Solution My pull request fixes order of transformations to scale -> rotate -> translate, with this fix `SpriteBundle` behaves as expected in described rotation, without deformation. Here is quote from "Essential Mathematics for Games": > Generally, the desired order we wish to use for these transforms is to scale first, then rotate, then translate. Scaling first gives us the scaling along the axes we expect. We can then rotate around the origin of the frame, and then translate it into place. I'm must say when I was using `MaterialMesh2dBundle` it behaves correctly in both cases with `bevy main` and with my fix, don't know why, was not able to figure it out why there is difference. here is code I was using for testing: ```rust use bevy::{ prelude::*, render::render_resource::{Extent3d, TextureDimension, TextureFormat}, sprite::{MaterialMesh2dBundle, Mesh2dHandle}, }; fn main() { let mut app = App::new(); app.insert_resource(ClearColor(Color::rgb(0.1, 0.2, 0.3))) .add_plugins(DefaultPlugins) .add_startup_system(setup); app.run(); } fn setup( mut commands: Commands, mut images: ResMut<Assets<Image>>, mut meshes: ResMut<Assets<Mesh>>, mut materials: ResMut<Assets<ColorMaterial>>, ) { let mut c = OrthographicCameraBundle::new_2d(); c.orthographic_projection.scale = 1.0 / 10.0; commands.spawn_bundle(c); // note: mesh somehow works for both variants // let quad: Mesh2dHandle = meshes.add(Mesh::from(shape::Quad::default())).into(); // let child = commands // .spawn_bundle(MaterialMesh2dBundle { // mesh: quad.clone(), // transform: Transform::from_translation(Vec3::new(0.0, 0.0, -1.0)) // .with_scale(Vec3::new(10.0, 1.0, 1.0)), // material: materials.add(ColorMaterial::from(Color::BLACK)), // ..Default::default() // }) // .id(); // commands // .spawn_bundle(MaterialMesh2dBundle { // mesh: quad, // transform: Transform::from_rotation(Quat::from_rotation_z(0.78)) // .with_translation(Vec3::new(0.0, 0.0, 10.0)), // material: materials.add(ColorMaterial::from(Color::WHITE)), // ..Default::default() // }) // .push_children(&[child]); let white = images.add(get_image(Color::rgb(1.0, 1.0, 1.0))); let black = images.add(get_image(Color::rgb(0.0, 0.0, 0.0))); let child = commands .spawn_bundle(SpriteBundle { texture: black, transform: Transform::from_translation(Vec3::new(0.0, 0.0, -1.0)) .with_scale(Vec3::new(10.0, 1.0, 1.0)), ..Default::default() }) .id(); commands .spawn_bundle(SpriteBundle { texture: white, transform: Transform::from_rotation(Quat::from_rotation_z(0.78)) .with_translation(Vec3::new(0.0, 0.0, 10.0)), ..Default::default() }) .push_children(&[child]); } fn get_image(color: Color) -> Image { let mut bytes = Vec::with_capacity((1 * 1 * 4 * 4) as usize); let color = color.as_rgba_f32(); bytes.extend(color[0].to_le_bytes()); bytes.extend(color[1].to_le_bytes()); bytes.extend(color[2].to_le_bytes()); bytes.extend(1.0_f32.to_le_bytes()); Image::new( Extent3d { width: 1, height: 1, depth_or_array_layers: 1, }, TextureDimension::D2, bytes, TextureFormat::Rgba32Float, ) } ``` here is screenshot with `bevy main` and my fix: 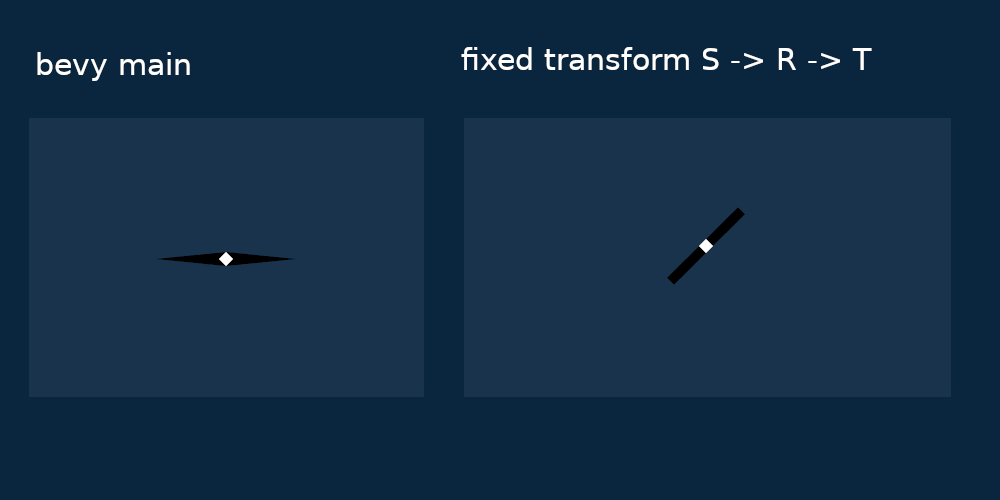 |
||
---|---|---|
.. | ||
bevy_app | ||
bevy_asset | ||
bevy_audio | ||
bevy_core | ||
bevy_core_pipeline | ||
bevy_crevice | ||
bevy_derive | ||
bevy_diagnostic | ||
bevy_dylib | ||
bevy_dynamic_plugin | ||
bevy_ecs | ||
bevy_ecs_compile_fail_tests | ||
bevy_gilrs | ||
bevy_gltf | ||
bevy_input | ||
bevy_internal | ||
bevy_log | ||
bevy_macro_utils | ||
bevy_math | ||
bevy_pbr | ||
bevy_reflect | ||
bevy_render | ||
bevy_scene | ||
bevy_sprite | ||
bevy_tasks | ||
bevy_text | ||
bevy_transform | ||
bevy_ui | ||
bevy_utils | ||
bevy_window | ||
bevy_winit |