# Objective
- I hit an issue using the `file_watcher` feature to hot reload assets
for my game. The change in this PR allows me to now hot reload assets.
- The issue stemmed from my project being a multi crate workspace
project structured like so:
```
└── my_game
├── my_game_core
│ ├── src
│ └── assets
├── my_game_editor
│ └── src/main.rs
└── my_game
└── src/main.rs
```
- `my_game_core` is a crate that holds all my game logic and assets
- `my_game` is the crate that creates the binary for my game (depends on
the game logic and assets in `my_game_core`)
- `my_game_editor` is an editor tool for my game (it also depends on the
game logic and assets in `my_game_core`)
Whilst running `my_game` and `my_game_editor` from cargo during
development I would use `AssetPlugin` like so:
```rust
default_plugins.set(AssetPlugin {
watch_for_changes_override: Some(true),
file_path: "../my_game_core/assets".to_string(),
..Default::default()
})
```
This works fine; bevy picks up the assets. However on saving an asset I
would get the following panic from `file_watcher`. It wouldn't kill the
app, but I wouldn't see the asset hot reload:
```
thread 'notify-rs debouncer loop' panicked at /Users/ian/.cargo/registry/src/index.crates.io-6f17d22bba15001f/bevy_asset-0.12.1/src/io/file/file_watcher.rs:48:58:
called `Result::unwrap()` on an `Err` value: StripPrefixError(())
note: run with `RUST_BACKTRACE=1` environment variable to display a backtrace
```
## Solution
- The solution is to collapse dot segments in the root asset path
`FileWatcher` is using
- There was already bevy code to do this in `AssetPath`, so I extracted
that code so it could be reused in `FileWatcher`
# Objective
- Simplify `Srgba` hex string parsing using std hex parsing functions
and removing loops in favor of bitwise ops.
This is a follow-up of the `bevy_color` upstream PR review:
https://github.com/bevyengine/bevy/pull/12013#discussion_r1497408114
## Solution
- Reworked `Srgba::hex` to use `from_str_radix` and some bitwise ops;
---------
Co-authored-by: Rob Parrett <robparrett@gmail.com>
# Objective
- Sometimes, it is useful to get a `Handle<T>` from an `AssetId<T>`. For
example, when iterating `Assets` to find a specific asset. So much so
that it's possible to do so with `AssetServer::get_id_handle`
- However, `AssetServer::get_id_handle` doesn't work with assets
directly added to `Assets` using `Assets::add`.
- Fixes#12087
## Solution
- Add `Assets::get_strong_handle` to convert an `AssetId` into an
`Handle`
- Document `AssetServer::get_id_handle` to explain its limitation and
point to `get_strong_handle`.
- Add a test for `get_strong_handle`
- Add a `duplicate_handles` field to `Assets` to avoid dropping assets
with a live handle generated by `get_strong_handle` (more reference
counting yay…)
- Fix typos in `Assets` docs
---
## Changelog
- Add `Assets::get_strong_handle` to convert an `AssetId` into an
`Handle`
# Objective
- Add the new `-Zcheck-cfg` checks to catch more warnings
- Fixes#12091
## Solution
- Create a new `cfg-check` to the CI that runs `cargo check -Zcheck-cfg
--workspace` using cargo nightly (and fails if there are warnings)
- Fix all warnings generated by the new check
---
## Changelog
- Remove all redundant imports
- Fix cfg wasm32 targets
- Add 3 dead code exceptions (should StandardColor be unused?)
- Convert ios_simulator to a feature (I'm not sure if this is the right
way to do it, but the check complained before)
## Migration Guide
No breaking changes
---------
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
# Objective
- Make `GizmoConfigStore` play well with reflection-based workflows like
editors.
## Solution
- `#[derive(Reflect)]` and call `.register_type()`.
# Objective
The migration process for `bevy_color` (#12013) will be fairly involved:
there will be hundreds of affected files, and a large number of APIs.
## Solution
To allow us to proceed granularly, we're going to keep both
`bevy_color::Color` (new) and `bevy_render::Color` (old) around until
the migration is complete.
However, simply doing this directly is confusing! They're both called
`Color`, making it very hard to tell when a portion of the code has been
ported.
As discussed in #12056, by renaming the old `Color` type, we can make it
easier to gradually migrate over, one API at a time.
## Migration Guide
THIS MIGRATION GUIDE INTENTIONALLY LEFT BLANK.
This change should not be shipped to end users: delete this section in
the final migration guide!
---------
Co-authored-by: Alice Cecile <alice.i.cecil@gmail.com>
# Objective
Add XYZ colour space. This will be most useful as a conversion step when
working with other (more common) colour spaces. See
[Wikipedia](https://en.wikipedia.org/wiki/CIE_1931_color_space) for
details on this space.
## Solution
- Added `Xyza` to `Color` and as its own type.
---
## Changelog
- Added `Xyza` type.
- Added `Color::Xyza` variant.
## Migration Guide
- `Color` enum now has an additional member, `Xyza`. Convert it to any
other type to handle this case in match statements.
# Objective
- Fix a wrongly named type
## Solution
- Rename it properly
---------
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
Co-authored-by: Nicola Papale <nicopap@users.noreply.github.com>
# Objective
Fixes#11821.
## Solution
* Run `System::apply_deferred` in `System::run` after executing the
system.
* Switch to using `System::run_unsafe` in `SingleThreadedExecutor` to
preserve the current behavior.
* Remove the `System::apply_deferred` in `SimpleExecutor` as it's now
redundant.
* Remove the `System::apply_deferred` when running one-shot systems, as
it's now redundant.
---
## Changelog
Changed: `System::run` will now immediately apply deferred system params
after running the system.
## Migration Guide
`System::run` will now always run `System::apply_deferred` immediately
after running the system now. If you were running systems and then
applying their deferred buffers at a later point in time, you can
eliminate the latter.
```rust
// in 0.13
system.run(world);
// .. sometime later ...
system.apply_deferred(world);
// in 0.14
system.run(world);
```
---------
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
# Objective
Since #9822, `SimpleExecutor` panics when an automatic sync point is
inserted:
```rust
let mut sched = Schedule::default();
sched.set_executor_kind(ExecutorKind::Simple);
sched.add_systems((|_: Commands| (), || ()).chain());
sched.run(&mut World::new());
```
```
System's param_state was not found. Did you forget to initialize this system before running it?
note: run with `RUST_BACKTRACE=1` environment variable to display a backtrace
Encountered a panic in system `bevy_ecs::schedule::executor::apply_deferred`!
```
## Solution
Don't try to run the `apply_deferred` system.
# Objective
Fixes Skyboxes on WebGL, which broke in Bevy 0.13 due to the addition of
the `brightness` uniform, when previously the skybox pipeline only had
view and global uniforms.
```ignore
panicked at ~/.cargo/registry/src/index.crates.io-6f17d22bba15001f/wgpu-0.19.1/src/backend/wgpu_core.rs:3009:5:
wgpu error: Validation Error
Caused by:
In Device::create_render_pipeline
note: label = `skybox_pipeline`
In the provided shader, the type given for group 0 binding 3 has a size of 4. As the device does not support `DownlevelFlags::BUFFER_BINDINGS_NOT_16_BYTE_ALIGNED`, the type must have a size that is a multiple of 16 bytes.
```
It would be nice if this could be backported to a 0.13.1 patch as well
if possible. I'm needing to rely on my own fork for now.
## Solution
Similar to the Globals uniform solution here:
d31de3f139/crates/bevy_render/src/globals.rs (L59-L60)
I've added 3 conditional fields to `SkyboxUniforms`.
# Objective
- Example reflection_probe is not frame rate independent
## Solution
- Use time delta to rotate the camera, use the same rotation speed as
the load_gltf example
31d7fcd9cb/examples/3d/load_gltf.rs (L63)
---
# Objective
`Scope::spawn`, `Scope::spawn_on_external`, and `Scope::spawn_on_scope`
have different signatures depending on whether the `multi-threaded`
feature is enabled. The single-threaded version has a stricter signature
that prevents sending the `Scope` itself to spawned tasks.
## Solution
Changed the lifetime constraints in the single-threaded signatures from
`'env` to `'scope` to match the multi-threaded version.
This was split off from #11906.
# Objective
- Assist Bevy contributors in the creation of `bevy_color` spaces by
ensuring consistent API implementation.
## Solution
Created a `pub(crate)` trait `StandardColor` which has all the
requirements for a typical color space (e.g, `Srgba`, `Color`, etc.).
---
## Changelog
- Implemented traits missing from certain color spaces.
## Migration Guide
_No migration required_
# Objective
Improve code quality and prevent bugs.
## Solution
I removed the unnecessary wildcards from `<LogPlugin as Plugin>::build`.
I also changed the warnings that would occur if the subscriber/logger
was already set into errors.
# Objective
This provides a new set of color types and operations for Bevy.
Fixes: #10986#1402
## Solution
The new crate provides a set of distinct types for various useful color
spaces, along with utilities for manipulating and converting colors.
This is not a breaking change, as no Bevy APIs are modified (yet).
---------
Co-authored-by: François <mockersf@gmail.com>
# Objective
When dealing with custom asset sources it can be a bit tricky to get
asset roots combined with relative paths correct.
It's even harder when it isn't mentioned which path was problematic.
## Solution
Mention which path failed for the file watcher.
Signed-off-by: Torstein Grindvik <torstein.grindvik@muybridge.com>
Co-authored-by: Torstein Grindvik <torstein.grindvik@muybridge.com>
# Objective
- Fixes#11977 - user defined shaders don't work in wasm
- After investigation, it won't work if the shader is not yet available
when compiling the pipeline on all platforms, for example if you load
many assets
## Solution
- Set the pipeline state to queued when it errs waiting for the shader
so that it's retried
# Objective
- Some properties of public types are private but sometimes it's useful
to be able to set those
## Solution
- Make more stuff pub
---
## Changelog
- `MaterialBindGroupId` internal id is now pub and added a new()
constructor
- `ExtractedPointLight` and `ExtractedDirectionalLight` properties are
now all pub
---------
Co-authored-by: James Liu <contact@jamessliu.com>
This PR closes#11978
# Objective
Fix rendering on iOS Simulators.
iOS Simulator doesn't support the capability CUBE_ARRAY_TEXTURES, since
0.13 this started to make iOS Simulator not render anything with the
following message being outputted:
```
2024-02-19T14:59:34.896266Z ERROR bevy_render::render_resource::pipeline_cache: failed to create shader module: Validation Error
Caused by:
In Device::create_shader_module
Shader validation error:
Type [40] '' is invalid
Capability Capabilities(CUBE_ARRAY_TEXTURES) is required
```
## Solution
- Split up NO_ARRAY_TEXTURES_SUPPORT into both NO_ARRAY_TEXTURES_SUPPORT
and NO_CUBE_ARRAY_TEXTURES_SUPPORT and correctly apply
NO_ARRAY_TEXTURES_SUPPORT for iOS Simulator using the cfg flag
introduced in #10178.
---
## Changelog
### Fixed
- Rendering on iOS Simulator due to missing CUBE_ARRAY_TEXTURES support.
---------
Co-authored-by: Sam Pettersson <sam.pettersson@geoguessr.com>
# Objective
- Taplo in CI is not running. The link used to download taplo doesn't
work anymore.
## Solution
- Compile taplo directly with cargo
- Improve docs a little
- Run taplo
---------
Co-authored-by: François <mockersf@gmail.com>
# Objective
- The `touch_screen_input_system` system runs on every tick.
- It unconditionally calls `update(&mut self)`, on the `Touches`
resource.
- This blocks the usage of a `resource_changed::<Touches>` run
condition.
## Solution
- Remove `update(&mut self)` as it's only used in this one system, and
in-lining the method implementation removes an indirection to an
ambiguously named method.
- Add conditional checks around the calls to clearing the internal maps.
---------
Signed-off-by: Jean Mertz <git@jeanmertz.com>
# Objective
- Some members of `bevy_dynamic_plugin` are not documented.
- Part of #3492.
## Solution
- Add documentation to members missing it in `bevy_dynamic_plugin`.
- Update existing documentation for clarity and formatting.
---
## Changelog
- Completely document `bevy_dynamic_plugin`.
---------
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
Co-authored-by: James Liu <contact@jamessliu.com>
# Objective
- Part of #11590
- Fix `unsafe_op_in_unsafe_fn` for trivial cases in bevy_ecs
## Solution
Fix `unsafe_op_in_unsafe_fn` in bevy_ecs for trivial cases, i.e., add an
`unsafe` block when the safety comment already exists or add a comment
like "The invariants are uphold by the caller".
---------
Co-authored-by: James Liu <contact@jamessliu.com>
## Objective
Always have `some_system.into_system().type_id() ==
some_system.into_system_set().system_type().unwrap()`.
System sets have a `fn system_type() -> Option<TypeId>` that is
implemented by `SystemTypeSet` to returning the TypeId of the system's
function type. This was implemented in
https://github.com/bevyengine/bevy/pull/7715 and is used in
`bevy_mod_debugdump` to handle `.after(function)` constraints.
Back then, `System::type_id` always also returned the type id of the
function item, not of `FunctionSystem<M, F>`.
https://github.com/bevyengine/bevy/pull/11728 changes the behaviour of
`System::type_id` so that it returns the id of the
`FunctionSystem`/`ExclusiveFunctionSystem` wrapper, but it did not
change `SystemTypeSet::system_type`, so doing the lookup breaks in
`bevy_mod_debugdump`.
## Solution
Change `IntoSystemSet` for functions to return a
`SystemTypeSet<FunctionSystem>` /
`SystemTypeSet<ExclusiveFunctionSystem>` instead of `SystemTypeSet<F>`.
# Objective
- Globals are supposed to be available in vertex shader but that was
mistakenly removed in 0.13
## Solution
- Configure the visibility of the globals correctly
Fixes https://github.com/bevyengine/bevy/issues/12015
# Objective
- The file asset source currently creates the `imported_assets/Default`
directory with relative path, which leads to wrongly created directories
when the executable is run with a working directory different from the
project root.
## Solution
- Use the full path instead.
# Objective
This PR adds some missing mime types to the
`ImageFormat::from_mime_type` method. As discussed [in this comment on
the Discord Bevy
community](https://discord.com/channels/691052431525675048/691052431974465548/1209904290227949729):
> It's strange that Bevy supports parsing `ImageFormat::WebP` from a
.webp str extension in the method below, but not from the mime type.
>
> In comparison, the image crate does parse it:
https://github.com/image-rs/image/blob/master/src/image.rs#L170
# Solution
For each of the missing mime types, I added them based on the
`ImageFormat::from_mime_type` of the image crate:
https://github.com/image-rs/image/blob/master/src/image.rs#L209, except
for `ImageFormat::Basis` and `ImageFormat::Ktx2` which are not present
in the image crate, and I ignore if they have a mime type or not*
\* apparently nowadays there is an official mime type: `image/ktx2`
https://www.iana.org/assignments/media-types/image/ktx2
Any feedback is welcome! I thought of refactoring a bit more and
delegating the mime type parsing to the image crate (and possibly the
same for extensions), let me know if that's desired 🙂
Fixes#12016.
Bump version after release
This PR has been auto-generated
Co-authored-by: Bevy Auto Releaser <41898282+github-actions[bot]@users.noreply.github.com>
Co-authored-by: François <mockersf@gmail.com>
# Objective
Move Gizmo examples into the separate directory
Fixes#11899
---------
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
Co-authored-by: Joona Aalto <jondolf.dev@gmail.com>
# Objective
- Closes#11985
## Solution
- alpha.rs has been moved from bevy_pbr into bevy_render; bevy_pbr and
bevy_gltf now access `AlphaMode` through bevy_render.
---
## Migration Guide
In the present implementation, external consumers of `AlphaMode` will
have to access it through bevy_render rather than through bevy_pbr,
changing their import from `bevy_pbr::AlphaMode` to
`bevy_render::alpha::AlphaMode` (or the corresponding glob import from
`bevy_pbr::prelude::*` to `bevy_render::prelude::*`).
## Uncertainties
Some remaining things from this that I am uncertain about:
- Here, the `app.register_type<AlphaMode>()` call has been moved from
`PbrPlugin` to `RenderPlugin`; I'm not sure if this is quite right, and
I was unable to find any direct relationship between `PbrPlugin` and
`RenderPlugin`.
- `AlphaMode` was placed in the prelude of bevy_render. I'm not certain
that this is actually appropriate.
- bevy_pbr does not re-export `AlphaMode`, which makes this a breaking
change for external consumers.
Any of these things could be easily changed; I'm just not confident that
I necessarily adopted the right approach in these (known) ways since
this codebase and ecosystem is quite new to me.
Adopted #8266, so copy-pasting the description from there:
# Objective
Support the KHR_texture_transform extension for the glTF loader.
- Fixes#6335
- Fixes#11869
- Implements part of #11350
- Implements the GLTF part of #399
## Solution
As is, this only supports a single transform. Looking at Godot's source,
they support one transform with an optional second one for detail, AO,
and emission. glTF specifies one per texture. The public domain
materials I looked at seem to share the same transform. So maybe having
just one is acceptable for now. I tried to include a warning if multiple
different transforms exist for the same material.
Note the gltf crate doesn't expose the texture transform for the normal
and occlusion textures, which it should, so I just ignored those for
now. (note by @janhohenheim: this is still the case)
Via `cargo run --release --example scene_viewer
~/src/clone/glTF-Sample-Models/2.0/TextureTransformTest/glTF/TextureTransformTest.gltf`:
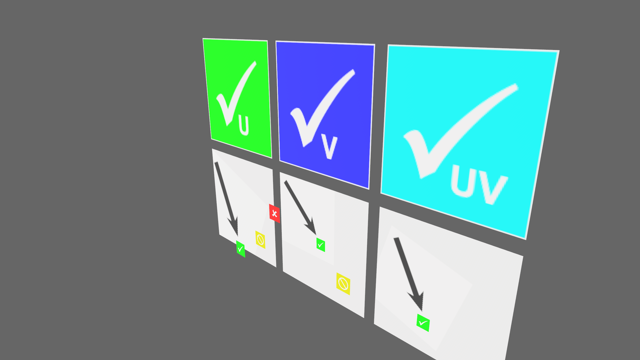
## Changelog
Support for the
[KHR_texture_transform](https://github.com/KhronosGroup/glTF/tree/main/extensions/2.0/Khronos/KHR_texture_transform)
extension added. Texture UVs that were scaled, rotated, or offset in a
GLTF are now properly handled.
---------
Co-authored-by: Al McElrath <hello@yrns.org>
Co-authored-by: Kanabenki <lucien.menassol@gmail.com>
# Objective
If multiple cameras render to the same target with MSAA enabled, only
the first and the last camera output will appear in the final output*.
This is because each camera maintains a separate flag to track the
active main texture. The first camera renders to texture A and all
subsequent cameras first write-back from A and then render into texture
B. Hence, camera 3 onwards will overwrite the work of the previous
camera.
\* This would manifest slightly differently if there were other calls to
post_process_write() in a more complex setup.
The is a functional regression from Bevy 0.12.
## Solution
The flag which tracks the active main texture should be shared between
cameras with the same `NormalizedRenderTarget`. Add the
`Arc<AtomicUsize>` to the existing per-target cache.
# Objective
- Save 16 bytes per MeshUniform in uniform/storage buffers.
## Solution
- Reorder members of MeshUniform to capitalise on alignment and size
rules for tighter data packing. Before the size of a MeshUniform was 160
bytes, and after it is 144 bytes, saving 16 bytes of unused padding for
alignment.
---
## Changelog
- Reduced the size of MeshUniform by 16 bytes.
# Objective
The derive macro wasn't doc-commented, so it showed up in the generated
doc as follow:
```rust
#[derive(Asset, TypePath)]
let shader = asset_server.load::<Shader>("embedded://bevy_rock/render/rock.wgsl");
```
Which is very confusing
## Solution
Comment the `derive` attribute as well
# Objective
Since https://github.com/bevyengine/bevy-website/pull/880, the old book
is now the Quick Start Guide, but the references to the old book have
not been updated.
## Solution
Update references to the old book to point to the Quick Start Guide.
# Objective
While doing a bunch of testing on examples, I noticed that this
particular example had lots of room for improvement. This example is
shown in example showcase, so it might as well look nice.
- The "overflow containers" have no vertical separation from each other
- The "overflow containers" are aligned awkwardly
- The instructions say you can "toggle" overflow, but it's actually
cycling through various overflow modes, with no indication of which mode
is currently active.
- The UI structure could be simplified
## Solution
- Simplify the UI structure by
- Moving the instructions into an absolutely positioned node, which we
do in most other examples with instructions
- Using a grid layout and removing some unnecessary containers
- Show the current overflow mode
- Add a gap between the overflow containers
- Other misc cleanup
## Before / After
<img width="1280" alt="Screenshot 2024-02-15 at 9 48 02 AM"
src="https://github.com/bevyengine/bevy/assets/200550/046e53b3-f744-48a2-b5ac-24b3f35b64ca">
<img width="1280" alt="Screenshot 2024-02-15 at 9 48 26 AM"
src="https://github.com/bevyengine/bevy/assets/200550/7e5e4ecf-dd1d-440a-b4a8-dd43bee3ef0a">
# Objective
We deprecated quite a few APIs in 0.13. 0.13 has shipped already. It
should be OK to remove them in 0.14's release. Fixes#4059. Fixes#9011.
## Solution
Remove them.
# Objective
- Some workspace members do not inherit the global lints.
## Solution
- Add a `[lints]` entry for all files returned by `rg
--files-without-match -F "[lints]" **/Cargo.toml`, except the compile
failure tests since these aren't part of the workspace.
- Add some docstrings where needed.
---------
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>