2020-08-29 00:08:51 +00:00
|
|
|
[package]
|
|
|
|
name = "bevy_utils"
|
2024-07-08 12:54:08 +00:00
|
|
|
version = "0.15.0-dev"
|
2021-10-27 00:12:14 +00:00
|
|
|
edition = "2021"
|
2020-08-29 00:08:51 +00:00
|
|
|
description = "A collection of utils for Bevy Engine"
|
|
|
|
homepage = "https://bevyengine.org"
|
|
|
|
repository = "https://github.com/bevyengine/bevy"
|
2021-07-23 21:11:51 +00:00
|
|
|
license = "MIT OR Apache-2.0"
|
2020-08-29 00:08:51 +00:00
|
|
|
keywords = ["bevy"]
|
|
|
|
|
Introduce detailed_trace macro, use in TrackedRenderPass (#7639)
Profiles show that in extremely hot loops, like the draw loops in the renderer, invoking the trace! macro has noticeable overhead, even if the trace log level is not enabled.
Solve this by introduce a 'wrapper' detailed_trace macro around trace, that wraps the trace! log statement in a trivially false if statement unless a cargo feature is enabled
# Objective
- Eliminate significant overhead observed with trace-level logging in render hot loops, even when trace log level is not enabled.
- This is an alternative solution to the one proposed in #7223
## Solution
- Introduce a wrapper around the `trace!` macro called `detailed_trace!`. This macro wraps the `trace!` macro with an if statement that is conditional on a new cargo feature, `detailed_trace`. When the feature is not enabled (the default), then the if statement is trivially false and should be optimized away at compile time.
- Convert the observed hot occurrences of trace logging in `TrackedRenderPass` with this new macro.
Testing the results of
```
cargo run --profile stress-test --features bevy/trace_tracy --example many_cubes -- spheres
```
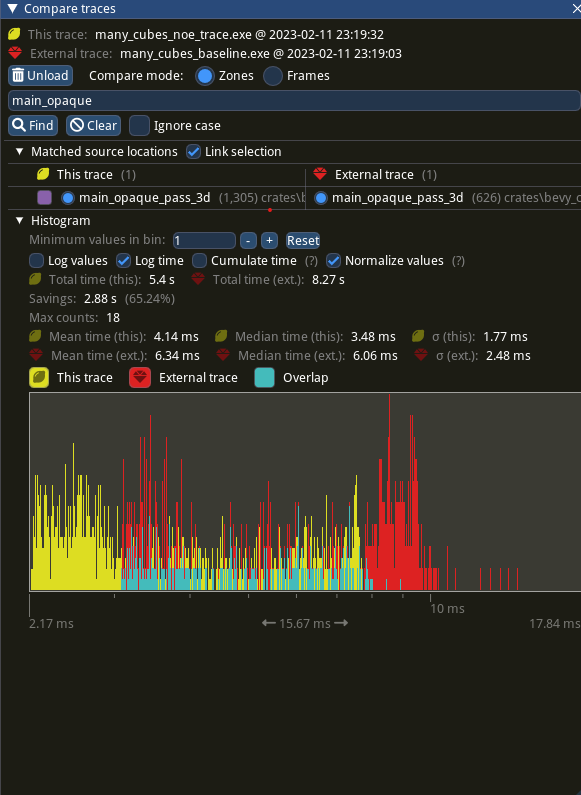
shows significant improvement of the `main_opaque_pass_3d` of the renderer, a median time decrease from 6.0ms to 3.5ms.
---
## Changelog
- For performance reasons, some detailed renderer trace logs now require the use of cargo feature `detailed_trace` in addition to setting the log level to `TRACE` in order to be shown.
## Migration Guide
- Some detailed bevy trace events now require the use of the cargo feature `detailed_trace` in addition to enabling `TRACE` level logging to view. Should you wish to see these logs, please compile your code with the bevy feature `detailed_trace`. Currently, the only logs that are affected are the renderer logs pertaining to `TrackedRenderPass` functions
2023-02-13 18:20:27 +00:00
|
|
|
[features]
|
|
|
|
detailed_trace = []
|
|
|
|
|
2020-08-29 00:08:51 +00:00
|
|
|
[dependencies]
|
2024-02-10 08:38:34 +00:00
|
|
|
ahash = "0.8.7"
|
2022-04-27 19:08:11 +00:00
|
|
|
tracing = { version = "0.1", default-features = false, features = ["std"] }
|
fix: upgrade to winit v0.30 (#13366)
# Objective
- Upgrade winit to v0.30
- Fixes https://github.com/bevyengine/bevy/issues/13331
## Solution
This is a rewrite/adaptation of the new trait system described and
implemented in `winit` v0.30.
## Migration Guide
The custom UserEvent is now renamed as WakeUp, used to wake up the loop
if anything happens outside the app (a new
[custom_user_event](https://github.com/bevyengine/bevy/pull/13366/files#diff-2de8c0a8d3028d0059a3d80ae31b2bbc1cde2595ce2d317ea378fe3e0cf6ef2d)
shows this behavior.
The internal `UpdateState` has been removed and replaced internally by
the AppLifecycle. When changed, the AppLifecycle is sent as an event.
The `UpdateMode` now accepts only two values: `Continuous` and
`Reactive`, but the latter exposes 3 new properties to enable reactive
to device, user or window events. The previous `UpdateMode::Reactive` is
now equivalent to `UpdateMode::reactive()`, while
`UpdateMode::ReactiveLowPower` to `UpdateMode::reactive_low_power()`.
The `ApplicationLifecycle` has been renamed as `AppLifecycle`, and now
contains the possible values of the application state inside the event
loop:
* `Idle`: the loop has not started yet
* `Running` (previously called `Started`): the loop is running
* `WillSuspend`: the loop is going to be suspended
* `Suspended`: the loop is suspended
* `WillResume`: the loop is going to be resumed
Note: the `Resumed` state has been removed since the resumed app is just
running.
Finally, now that `winit` enables this, it extends the `WinitPlugin` to
support custom events.
## Test platforms
- [x] Windows
- [x] MacOs
- [x] Linux (x11)
- [x] Linux (Wayland)
- [x] Android
- [x] iOS
- [x] WASM/WebGPU
- [x] WASM/WebGL2
## Outstanding issues / regressions
- [ ] iOS: build failed in CI
- blocking, but may just be flakiness
- [x] Cross-platform: when the window is maximised, changes in the scale
factor don't apply, to make them apply one has to make the window
smaller again. (Re-maximising keeps the updated scale factor)
- non-blocking, but good to fix
- [ ] Android: it's pretty easy to quickly open and close the app and
then the music keeps playing when suspended.
- non-blocking but worrying
- [ ] Web: the application will hang when switching tabs
- Not new, duplicate of https://github.com/bevyengine/bevy/issues/13486
- [ ] Cross-platform?: Screenshot failure, `ERROR present_frames:
wgpu_core::present: No work has been submitted for this frame before`
taking the first screenshot, but after pressing space
- non-blocking, but good to fix
---------
Co-authored-by: François <francois.mockers@vleue.com>
2024-06-03 13:06:48 +00:00
|
|
|
web-time = { version = "1.1" }
|
2024-08-04 13:23:28 +00:00
|
|
|
hashbrown = { version = "0.14.2", features = ["serde"] }
|
2024-07-08 12:54:08 +00:00
|
|
|
bevy_utils_proc_macros = { version = "0.15.0-dev", path = "macros" }
|
refactor: Extract parallel queue abstraction (#7348)
# Objective
There's a repeating pattern of `ThreadLocal<Cell<Vec<T>>>` which is very
useful for low overhead, low contention multithreaded queues that have
cropped up in a few places in the engine. This pattern is surprisingly
useful when building deferred mutation across multiple threads, as noted
by it's use in `ParallelCommands`.
However, `ThreadLocal<Cell<Vec<T>>>` is not only a mouthful, it's also
hard to ensure the thread-local queue is replaced after it's been
temporarily removed from the `Cell`.
## Solution
Wrap the pattern into `bevy_utils::Parallel<T>` which codifies the
entire pattern and ensures the user follows the contract. Instead of
fetching indivdual cells, removing the value, mutating it, and replacing
it, `Parallel::get` returns a `ParRef<'a, T>` which contains the
temporarily removed value and a reference back to the cell, and will
write the mutated value back to the cell upon being dropped.
I would like to use this to simplify the remaining part of #4899 that
has not been adopted/merged.
---
## Changelog
TODO
---------
Co-authored-by: Joseph <21144246+JoJoJet@users.noreply.github.com>
2024-02-19 16:31:15 +00:00
|
|
|
thread_local = "1.0"
|
2020-10-21 22:55:35 +00:00
|
|
|
|
2024-01-02 22:08:30 +00:00
|
|
|
[dev-dependencies]
|
|
|
|
static_assertions = "1.1.0"
|
|
|
|
|
2020-10-21 22:55:35 +00:00
|
|
|
[target.'cfg(target_arch = "wasm32")'.dependencies]
|
2023-10-25 19:20:17 +00:00
|
|
|
getrandom = { version = "0.2.0", features = ["js"] }
|
2023-11-18 20:58:48 +00:00
|
|
|
|
|
|
|
[lints]
|
|
|
|
workspace = true
|
2024-03-23 02:22:52 +00:00
|
|
|
|
|
|
|
[package.metadata.docs.rs]
|
2024-07-29 23:10:16 +00:00
|
|
|
rustdoc-args = ["-Zunstable-options", "--generate-link-to-definition"]
|
2024-03-23 02:22:52 +00:00
|
|
|
all-features = true
|