2023-04-15 21:48:31 +00:00
|
|
|
//! This example shows how to create a custom render pass that runs after the main pass
|
|
|
|
//! and reads the texture generated by the main pass.
|
|
|
|
//!
|
|
|
|
//! The example shader is a very simple implementation of chromatic aberration.
|
|
|
|
//!
|
|
|
|
//! This is a fairly low level example and assumes some familiarity with rendering concepts and wgpu.
|
2022-06-06 00:06:49 +00:00
|
|
|
|
|
|
|
use bevy::{
|
2023-11-26 20:48:03 +00:00
|
|
|
core_pipeline::{core_3d, fullscreen_vertex_shader::fullscreen_shader_vertex_state},
|
2023-08-10 02:02:30 +00:00
|
|
|
ecs::query::QueryItem,
|
2022-06-06 00:06:49 +00:00
|
|
|
prelude::*,
|
|
|
|
render::{
|
2023-04-15 21:48:31 +00:00
|
|
|
extract_component::{
|
|
|
|
ComponentUniforms, ExtractComponent, ExtractComponentPlugin, UniformComponentPlugin,
|
|
|
|
},
|
2023-08-10 02:02:30 +00:00
|
|
|
render_graph::{
|
|
|
|
NodeRunError, RenderGraphApp, RenderGraphContext, ViewNode, ViewNodeRunner,
|
|
|
|
},
|
2022-06-06 00:06:49 +00:00
|
|
|
render_resource::{
|
Bind group layout entries (#10224)
# Objective
- Follow up to #9694
## Solution
- Same api as #9694 but adapted for `BindGroupLayoutEntry`
- Use the same `ShaderStages` visibilty for all entries by default
- Add `BindingType` helper function that mirror the wgsl equivalent and
that make writing layouts much simpler.
Before:
```rust
let layout = render_device.create_bind_group_layout(&BindGroupLayoutDescriptor {
label: Some("post_process_bind_group_layout"),
entries: &[
BindGroupLayoutEntry {
binding: 0,
visibility: ShaderStages::FRAGMENT,
ty: BindingType::Texture {
sample_type: TextureSampleType::Float { filterable: true },
view_dimension: TextureViewDimension::D2,
multisampled: false,
},
count: None,
},
BindGroupLayoutEntry {
binding: 1,
visibility: ShaderStages::FRAGMENT,
ty: BindingType::Sampler(SamplerBindingType::Filtering),
count: None,
},
BindGroupLayoutEntry {
binding: 2,
visibility: ShaderStages::FRAGMENT,
ty: BindingType::Buffer {
ty: bevy::render::render_resource::BufferBindingType::Uniform,
has_dynamic_offset: false,
min_binding_size: Some(PostProcessSettings::min_size()),
},
count: None,
},
],
});
```
After:
```rust
let layout = render_device.create_bind_group_layout(
"post_process_bind_group_layout"),
&BindGroupLayoutEntries::sequential(
ShaderStages::FRAGMENT,
(
texture_2d_f32(),
sampler(SamplerBindingType::Filtering),
uniform_buffer(false, Some(PostProcessSettings::min_size())),
),
),
);
```
Here's a more extreme example in bevy_solari:
https://github.com/JMS55/bevy/pull/15/commits/86dab7f5da23da45d4ab668ae30553dadb816d8f
---
## Changelog
- Added `BindGroupLayoutEntries` and all `BindingType` helper functions.
## Migration Guide
`RenderDevice::create_bind_group_layout()` doesn't take a
`BindGroupLayoutDescriptor` anymore. You need to provide the parameters
separately
```rust
// 0.12
let layout = render_device.create_bind_group_layout(&BindGroupLayoutDescriptor {
label: Some("post_process_bind_group_layout"),
entries: &[
BindGroupLayoutEntry {
// ...
},
],
});
// 0.13
let layout = render_device.create_bind_group_layout(
"post_process_bind_group_layout",
&[
BindGroupLayoutEntry {
// ...
},
],
);
```
## TODO
- [x] implement a `Dynamic` variant
- [x] update the `RenderDevice::create_bind_group_layout()` api to match
the one from `RenderDevice::creat_bind_group()`
- [x] docs
2023-11-28 04:00:49 +00:00
|
|
|
binding_types::{sampler, texture_2d, uniform_buffer},
|
|
|
|
*,
|
2022-06-06 00:06:49 +00:00
|
|
|
},
|
2023-04-15 21:48:31 +00:00
|
|
|
renderer::{RenderContext, RenderDevice},
|
2022-08-07 20:26:13 +00:00
|
|
|
texture::BevyDefault,
|
2023-08-07 23:02:32 +00:00
|
|
|
view::ViewTarget,
|
2023-04-15 21:48:31 +00:00
|
|
|
RenderApp,
|
2022-06-06 00:06:49 +00:00
|
|
|
},
|
|
|
|
};
|
|
|
|
|
|
|
|
fn main() {
|
2022-09-19 16:36:38 +00:00
|
|
|
App::new()
|
Multiple Asset Sources (#9885)
This adds support for **Multiple Asset Sources**. You can now register a
named `AssetSource`, which you can load assets from like you normally
would:
```rust
let shader: Handle<Shader> = asset_server.load("custom_source://path/to/shader.wgsl");
```
Notice that `AssetPath` now supports `some_source://` syntax. This can
now be accessed through the `asset_path.source()` accessor.
Asset source names _are not required_. If one is not specified, the
default asset source will be used:
```rust
let shader: Handle<Shader> = asset_server.load("path/to/shader.wgsl");
```
The behavior of the default asset source has not changed. Ex: the
`assets` folder is still the default.
As referenced in #9714
## Why?
**Multiple Asset Sources** enables a number of often-asked-for
scenarios:
* **Loading some assets from other locations on disk**: you could create
a `config` asset source that reads from the OS-default config folder
(not implemented in this PR)
* **Loading some assets from a remote server**: you could register a new
`remote` asset source that reads some assets from a remote http server
(not implemented in this PR)
* **Improved "Binary Embedded" Assets**: we can use this system for
"embedded-in-binary assets", which allows us to replace the old
`load_internal_asset!` approach, which couldn't support asset
processing, didn't support hot-reloading _well_, and didn't make
embedded assets accessible to the `AssetServer` (implemented in this pr)
## Adding New Asset Sources
An `AssetSource` is "just" a collection of `AssetReader`, `AssetWriter`,
and `AssetWatcher` entries. You can configure new asset sources like
this:
```rust
app.register_asset_source(
"other",
AssetSource::build()
.with_reader(|| Box::new(FileAssetReader::new("other")))
)
)
```
Note that `AssetSource` construction _must_ be repeatable, which is why
a closure is accepted.
`AssetSourceBuilder` supports `with_reader`, `with_writer`,
`with_watcher`, `with_processed_reader`, `with_processed_writer`, and
`with_processed_watcher`.
Note that the "asset source" system replaces the old "asset providers"
system.
## Processing Multiple Sources
The `AssetProcessor` now supports multiple asset sources! Processed
assets can refer to assets in other sources and everything "just works".
Each `AssetSource` defines an unprocessed and processed `AssetReader` /
`AssetWriter`.
Currently this is all or nothing for a given `AssetSource`. A given
source is either processed or it is not. Later we might want to add
support for "lazy asset processing", where an `AssetSource` (such as a
remote server) can be configured to only process assets that are
directly referenced by local assets (in order to save local disk space
and avoid doing extra work).
## A new `AssetSource`: `embedded`
One of the big features motivating **Multiple Asset Sources** was
improving our "embedded-in-binary" asset loading. To prove out the
**Multiple Asset Sources** implementation, I chose to build a new
`embedded` `AssetSource`, which replaces the old `load_interal_asset!`
system.
The old `load_internal_asset!` approach had a number of issues:
* The `AssetServer` was not aware of (or capable of loading) internal
assets.
* Because internal assets weren't visible to the `AssetServer`, they
could not be processed (or used by assets that are processed). This
would prevent things "preprocessing shaders that depend on built in Bevy
shaders", which is something we desperately need to start doing.
* Each "internal asset" needed a UUID to be defined in-code to reference
it. This was very manual and toilsome.
The new `embedded` `AssetSource` enables the following pattern:
```rust
// Called in `crates/bevy_pbr/src/render/mesh.rs`
embedded_asset!(app, "mesh.wgsl");
// later in the app
let shader: Handle<Shader> = asset_server.load("embedded://bevy_pbr/render/mesh.wgsl");
```
Notice that this always treats the crate name as the "root path", and it
trims out the `src` path for brevity. This is generally predictable, but
if you need to debug you can use the new `embedded_path!` macro to get a
`PathBuf` that matches the one used by `embedded_asset`.
You can also reference embedded assets in arbitrary assets, such as WGSL
shaders:
```rust
#import "embedded://bevy_pbr/render/mesh.wgsl"
```
This also makes `embedded` assets go through the "normal" asset
lifecycle. They are only loaded when they are actually used!
We are also discussing implicitly converting asset paths to/from shader
modules, so in the future (not in this PR) you might be able to load it
like this:
```rust
#import bevy_pbr::render::mesh::Vertex
```
Compare that to the old system!
```rust
pub const MESH_SHADER_HANDLE: Handle<Shader> = Handle::weak_from_u128(3252377289100772450);
load_internal_asset!(app, MESH_SHADER_HANDLE, "mesh.wgsl", Shader::from_wgsl);
// The mesh asset is the _only_ accessible via MESH_SHADER_HANDLE and _cannot_ be loaded via the AssetServer.
```
## Hot Reloading `embedded`
You can enable `embedded` hot reloading by enabling the
`embedded_watcher` cargo feature:
```
cargo run --features=embedded_watcher
```
## Improved Hot Reloading Workflow
First: the `filesystem_watcher` cargo feature has been renamed to
`file_watcher` for brevity (and to match the `FileAssetReader` naming
convention).
More importantly, hot asset reloading is no longer configured in-code by
default. If you enable any asset watcher feature (such as `file_watcher`
or `rust_source_watcher`), asset watching will be automatically enabled.
This removes the need to _also_ enable hot reloading in your app code.
That means you can replace this:
```rust
app.add_plugins(DefaultPlugins.set(AssetPlugin::default().watch_for_changes()))
```
with this:
```rust
app.add_plugins(DefaultPlugins)
```
If you want to hot reload assets in your app during development, just
run your app like this:
```
cargo run --features=file_watcher
```
This means you can use the same code for development and deployment! To
deploy an app, just don't include the watcher feature
```
cargo build --release
```
My intent is to move to this approach for pretty much all dev workflows.
In a future PR I would like to replace `AssetMode::ProcessedDev` with a
`runtime-processor` cargo feature. We could then group all common "dev"
cargo features under a single `dev` feature:
```sh
# this would enable file_watcher, embedded_watcher, runtime-processor, and more
cargo run --features=dev
```
## AssetMode
`AssetPlugin::Unprocessed`, `AssetPlugin::Processed`, and
`AssetPlugin::ProcessedDev` have been replaced with an `AssetMode` field
on `AssetPlugin`.
```rust
// before
app.add_plugins(DefaultPlugins.set(AssetPlugin::Processed { /* fields here */ })
// after
app.add_plugins(DefaultPlugins.set(AssetPlugin { mode: AssetMode::Processed, ..default() })
```
This aligns `AssetPlugin` with our other struct-like plugins. The old
"source" and "destination" `AssetProvider` fields in the enum variants
have been replaced by the "asset source" system. You no longer need to
configure the AssetPlugin to "point" to custom asset providers.
## AssetServerMode
To improve the implementation of **Multiple Asset Sources**,
`AssetServer` was made aware of whether or not it is using "processed"
or "unprocessed" assets. You can check that like this:
```rust
if asset_server.mode() == AssetServerMode::Processed {
/* do something */
}
```
Note that this refactor should also prepare the way for building "one to
many processed output files", as it makes the server aware of whether it
is loading from processed or unprocessed sources. Meaning we can store
and read processed and unprocessed assets differently!
## AssetPath can now refer to folders
The "file only" restriction has been removed from `AssetPath`. The
`AssetServer::load_folder` API now accepts an `AssetPath` instead of a
`Path`, meaning you can load folders from other asset sources!
## Improved AssetPath Parsing
AssetPath parsing was reworked to support sources, improve error
messages, and to enable parsing with a single pass over the string.
`AssetPath::new` was replaced by `AssetPath::parse` and
`AssetPath::try_parse`.
## AssetWatcher broken out from AssetReader
`AssetReader` is no longer responsible for constructing `AssetWatcher`.
This has been moved to `AssetSourceBuilder`.
## Duplicate Event Debouncing
Asset V2 already debounced duplicate filesystem events, but this was
_input_ events. Multiple input event types can produce the same _output_
`AssetSourceEvent`. Now that we have `embedded_watcher`, which does
expensive file io on events, it made sense to debounce output events
too, so I added that! This will also benefit the AssetProcessor by
preventing integrity checks for duplicate events (and helps keep the
noise down in trace logs).
## Next Steps
* **Port Built-in Shaders**: Currently the primary (and essentially
only) user of `load_interal_asset` in Bevy's source code is "built-in
shaders". I chose not to do that in this PR for a few reasons:
1. We need to add the ability to pass shader defs in to shaders via meta
files. Some shaders (such as MESH_VIEW_TYPES) need to pass shader def
values in that are defined in code.
2. We need to revisit the current shader module naming system. I think
we _probably_ want to imply modules from source structure (at least by
default). Ideally in a way that can losslessly convert asset paths
to/from shader modules (to enable the asset system to resolve modules
using the asset server).
3. I want to keep this change set minimal / get this merged first.
* **Deprecate `load_internal_asset`**: we can't do that until we do (1)
and (2)
* **Relative Asset Paths**: This PR significantly increases the need for
relative asset paths (which was already pretty high). Currently when
loading dependencies, it is assumed to be an absolute path, which means
if in an `AssetLoader` you call `context.load("some/path/image.png")` it
will assume that is the "default" asset source, _even if the current
asset is in a different asset source_. This will cause breakage for
AssetLoaders that are not designed to add the current source to whatever
paths are being used. AssetLoaders should generally not need to be aware
of the name of their current asset source, or need to think about the
"current asset source" generally. We should build apis that support
relative asset paths and then encourage using relative paths as much as
possible (both via api design and docs). Relative paths are also
important because they will allow developers to move folders around
(even across providers) without reprocessing, provided there is no path
breakage.
2023-10-13 23:17:32 +00:00
|
|
|
.add_plugins((DefaultPlugins, PostProcessPlugin))
|
2023-03-18 01:45:34 +00:00
|
|
|
.add_systems(Startup, setup)
|
2023-04-15 21:48:31 +00:00
|
|
|
.add_systems(Update, (rotate, update_settings))
|
2022-09-19 16:36:38 +00:00
|
|
|
.run();
|
2022-06-06 00:06:49 +00:00
|
|
|
}
|
|
|
|
|
2023-04-15 21:48:31 +00:00
|
|
|
/// It is generally encouraged to set up post processing effects as a plugin
|
|
|
|
struct PostProcessPlugin;
|
2022-06-06 00:06:49 +00:00
|
|
|
|
2023-04-15 21:48:31 +00:00
|
|
|
impl Plugin for PostProcessPlugin {
|
|
|
|
fn build(&self, app: &mut App) {
|
2023-06-21 20:51:03 +00:00
|
|
|
app.add_plugins((
|
2023-04-15 21:48:31 +00:00
|
|
|
// The settings will be a component that lives in the main world but will
|
|
|
|
// be extracted to the render world every frame.
|
|
|
|
// This makes it possible to control the effect from the main world.
|
|
|
|
// This plugin will take care of extracting it automatically.
|
|
|
|
// It's important to derive [`ExtractComponent`] on [`PostProcessingSettings`]
|
|
|
|
// for this plugin to work correctly.
|
2023-06-21 20:51:03 +00:00
|
|
|
ExtractComponentPlugin::<PostProcessSettings>::default(),
|
2023-04-15 21:48:31 +00:00
|
|
|
// The settings will also be the data used in the shader.
|
|
|
|
// This plugin will prepare the component for the GPU by creating a uniform buffer
|
|
|
|
// and writing the data to that buffer every frame.
|
2023-06-21 20:51:03 +00:00
|
|
|
UniformComponentPlugin::<PostProcessSettings>::default(),
|
|
|
|
));
|
2023-01-19 00:38:28 +00:00
|
|
|
|
2023-04-15 21:48:31 +00:00
|
|
|
// We need to get the render app from the main app
|
|
|
|
let Ok(render_app) = app.get_sub_app_mut(RenderApp) else {
|
|
|
|
return;
|
|
|
|
};
|
2022-06-06 00:06:49 +00:00
|
|
|
|
2023-04-15 21:48:31 +00:00
|
|
|
render_app
|
|
|
|
// Bevy's renderer uses a render graph which is a collection of nodes in a directed acyclic graph.
|
|
|
|
// It currently runs on each view/camera and executes each node in the specified order.
|
|
|
|
// It will make sure that any node that needs a dependency from another node
|
|
|
|
// only runs when that dependency is done.
|
|
|
|
//
|
|
|
|
// Each node can execute arbitrary work, but it generally runs at least one render pass.
|
|
|
|
// A node only has access to the render world, so if you need data from the main world
|
|
|
|
// you need to extract it manually or with the plugin like above.
|
|
|
|
// Add a [`Node`] to the [`RenderGraph`]
|
|
|
|
// The Node needs to impl FromWorld
|
2023-08-07 23:02:32 +00:00
|
|
|
//
|
|
|
|
// The [`ViewNodeRunner`] is a special [`Node`] that will automatically run the node for each view
|
|
|
|
// matching the [`ViewQuery`]
|
|
|
|
.add_render_graph_node::<ViewNodeRunner<PostProcessNode>>(
|
2023-07-10 00:11:51 +00:00
|
|
|
// Specify the name of the graph, in this case we want the graph for 3d
|
2023-04-15 21:48:31 +00:00
|
|
|
core_3d::graph::NAME,
|
|
|
|
// It also needs the name of the node
|
|
|
|
PostProcessNode::NAME,
|
|
|
|
)
|
|
|
|
.add_render_graph_edges(
|
|
|
|
core_3d::graph::NAME,
|
|
|
|
// Specify the node ordering.
|
|
|
|
// This will automatically create all required node edges to enforce the given ordering.
|
|
|
|
&[
|
|
|
|
core_3d::graph::node::TONEMAPPING,
|
|
|
|
PostProcessNode::NAME,
|
|
|
|
core_3d::graph::node::END_MAIN_PASS_POST_PROCESSING,
|
|
|
|
],
|
|
|
|
);
|
|
|
|
}
|
2023-05-16 20:31:30 +00:00
|
|
|
|
|
|
|
fn finish(&self, app: &mut App) {
|
|
|
|
// We need to get the render app from the main app
|
|
|
|
let Ok(render_app) = app.get_sub_app_mut(RenderApp) else {
|
|
|
|
return;
|
|
|
|
};
|
|
|
|
|
|
|
|
render_app
|
|
|
|
// Initialize the pipeline
|
|
|
|
.init_resource::<PostProcessPipeline>();
|
|
|
|
}
|
2023-04-15 21:48:31 +00:00
|
|
|
}
|
2022-06-06 00:06:49 +00:00
|
|
|
|
2023-08-07 23:02:32 +00:00
|
|
|
// The post process node used for the render graph
|
|
|
|
#[derive(Default)]
|
|
|
|
struct PostProcessNode;
|
2023-04-15 21:48:31 +00:00
|
|
|
impl PostProcessNode {
|
2023-10-05 05:41:09 +00:00
|
|
|
pub const NAME: &'static str = "post_process";
|
2023-04-15 21:48:31 +00:00
|
|
|
}
|
2022-06-06 00:06:49 +00:00
|
|
|
|
2023-08-07 23:02:32 +00:00
|
|
|
// The ViewNode trait is required by the ViewNodeRunner
|
|
|
|
impl ViewNode for PostProcessNode {
|
|
|
|
// The node needs a query to gather data from the ECS in order to do its rendering,
|
|
|
|
// but it's not a normal system so we need to define it manually.
|
|
|
|
//
|
|
|
|
// This query will only run on the view entity
|
Rename `WorldQueryData` & `WorldQueryFilter` to `QueryData` & `QueryFilter` (#10779)
# Rename `WorldQueryData` & `WorldQueryFilter` to `QueryData` &
`QueryFilter`
Fixes #10776
## Solution
Traits `WorldQueryData` & `WorldQueryFilter` were renamed to `QueryData`
and `QueryFilter`, respectively. Related Trait types were also renamed.
---
## Changelog
- Trait `WorldQueryData` has been renamed to `QueryData`. Derive macro's
`QueryData` attribute `world_query_data` has been renamed to
`query_data`.
- Trait `WorldQueryFilter` has been renamed to `QueryFilter`. Derive
macro's `QueryFilter` attribute `world_query_filter` has been renamed to
`query_filter`.
- Trait's `ExtractComponent` type `Query` has been renamed to `Data`.
- Trait's `GetBatchData` types `Query` & `QueryFilter` has been renamed
to `Data` & `Filter`, respectively.
- Trait's `ExtractInstance` type `Query` has been renamed to `Data`.
- Trait's `ViewNode` type `ViewQuery` has been renamed to `ViewData`.
- Trait's `RenderCommand` types `ViewWorldQuery` & `ItemWorldQuery` has
been renamed to `ViewData` & `ItemData`, respectively.
## Migration Guide
Note: if merged before 0.13 is released, this should instead modify the
migration guide of #10776 with the updated names.
- Rename `WorldQueryData` & `WorldQueryFilter` trait usages to
`QueryData` & `QueryFilter` and their respective derive macro attributes
`world_query_data` & `world_query_filter` to `query_data` &
`query_filter`.
- Rename the following trait type usages:
- Trait's `ExtractComponent` type `Query` to `Data`.
- Trait's `GetBatchData` type `Query` to `Data`.
- Trait's `ExtractInstance` type `Query` to `Data`.
- Trait's `ViewNode` type `ViewQuery` to `ViewData`'
- Trait's `RenderCommand` types `ViewWolrdQuery` & `ItemWorldQuery` to
`ViewData` & `ItemData`, respectively.
```rust
// Before
#[derive(WorldQueryData)]
#[world_query_data(derive(Debug))]
struct EmptyQuery {
empty: (),
}
// After
#[derive(QueryData)]
#[query_data(derive(Debug))]
struct EmptyQuery {
empty: (),
}
// Before
#[derive(WorldQueryFilter)]
struct CustomQueryFilter<T: Component, P: Component> {
_c: With<ComponentC>,
_d: With<ComponentD>,
_or: Or<(Added<ComponentC>, Changed<ComponentD>, Without<ComponentZ>)>,
_generic_tuple: (With<T>, With<P>),
}
// After
#[derive(QueryFilter)]
struct CustomQueryFilter<T: Component, P: Component> {
_c: With<ComponentC>,
_d: With<ComponentD>,
_or: Or<(Added<ComponentC>, Changed<ComponentD>, Without<ComponentZ>)>,
_generic_tuple: (With<T>, With<P>),
}
// Before
impl ExtractComponent for ContrastAdaptiveSharpeningSettings {
type Query = &'static Self;
type Filter = With<Camera>;
type Out = (DenoiseCAS, CASUniform);
fn extract_component(item: QueryItem<Self::Query>) -> Option<Self::Out> {
//...
}
}
// After
impl ExtractComponent for ContrastAdaptiveSharpeningSettings {
type Data = &'static Self;
type Filter = With<Camera>;
type Out = (DenoiseCAS, CASUniform);
fn extract_component(item: QueryItem<Self::Data>) -> Option<Self::Out> {
//...
}
}
// Before
impl GetBatchData for MeshPipeline {
type Param = SRes<RenderMeshInstances>;
type Query = Entity;
type QueryFilter = With<Mesh3d>;
type CompareData = (MaterialBindGroupId, AssetId<Mesh>);
type BufferData = MeshUniform;
fn get_batch_data(
mesh_instances: &SystemParamItem<Self::Param>,
entity: &QueryItem<Self::Query>,
) -> (Self::BufferData, Option<Self::CompareData>) {
// ....
}
}
// After
impl GetBatchData for MeshPipeline {
type Param = SRes<RenderMeshInstances>;
type Data = Entity;
type Filter = With<Mesh3d>;
type CompareData = (MaterialBindGroupId, AssetId<Mesh>);
type BufferData = MeshUniform;
fn get_batch_data(
mesh_instances: &SystemParamItem<Self::Param>,
entity: &QueryItem<Self::Data>,
) -> (Self::BufferData, Option<Self::CompareData>) {
// ....
}
}
// Before
impl<A> ExtractInstance for AssetId<A>
where
A: Asset,
{
type Query = Read<Handle<A>>;
type Filter = ();
fn extract(item: QueryItem<'_, Self::Query>) -> Option<Self> {
Some(item.id())
}
}
// After
impl<A> ExtractInstance for AssetId<A>
where
A: Asset,
{
type Data = Read<Handle<A>>;
type Filter = ();
fn extract(item: QueryItem<'_, Self::Data>) -> Option<Self> {
Some(item.id())
}
}
// Before
impl ViewNode for PostProcessNode {
type ViewQuery = (
&'static ViewTarget,
&'static PostProcessSettings,
);
fn run(
&self,
_graph: &mut RenderGraphContext,
render_context: &mut RenderContext,
(view_target, _post_process_settings): QueryItem<Self::ViewQuery>,
world: &World,
) -> Result<(), NodeRunError> {
// ...
}
}
// After
impl ViewNode for PostProcessNode {
type ViewData = (
&'static ViewTarget,
&'static PostProcessSettings,
);
fn run(
&self,
_graph: &mut RenderGraphContext,
render_context: &mut RenderContext,
(view_target, _post_process_settings): QueryItem<Self::ViewData>,
world: &World,
) -> Result<(), NodeRunError> {
// ...
}
}
// Before
impl<P: CachedRenderPipelinePhaseItem> RenderCommand<P> for SetItemPipeline {
type Param = SRes<PipelineCache>;
type ViewWorldQuery = ();
type ItemWorldQuery = ();
#[inline]
fn render<'w>(
item: &P,
_view: (),
_entity: (),
pipeline_cache: SystemParamItem<'w, '_, Self::Param>,
pass: &mut TrackedRenderPass<'w>,
) -> RenderCommandResult {
// ...
}
}
// After
impl<P: CachedRenderPipelinePhaseItem> RenderCommand<P> for SetItemPipeline {
type Param = SRes<PipelineCache>;
type ViewData = ();
type ItemData = ();
#[inline]
fn render<'w>(
item: &P,
_view: (),
_entity: (),
pipeline_cache: SystemParamItem<'w, '_, Self::Param>,
pass: &mut TrackedRenderPass<'w>,
) -> RenderCommandResult {
// ...
}
}
```
2023-12-12 19:45:50 +00:00
|
|
|
type ViewData = (
|
2023-11-14 22:35:40 +00:00
|
|
|
&'static ViewTarget,
|
|
|
|
// This makes sure the node only runs on cameras with the PostProcessSettings component
|
|
|
|
&'static PostProcessSettings,
|
|
|
|
);
|
2022-06-06 00:06:49 +00:00
|
|
|
|
2023-04-15 21:48:31 +00:00
|
|
|
// Runs the node logic
|
|
|
|
// This is where you encode draw commands.
|
|
|
|
//
|
2023-08-07 23:02:32 +00:00
|
|
|
// This will run on every view on which the graph is running.
|
|
|
|
// If you don't want your effect to run on every camera,
|
|
|
|
// you'll need to make sure you have a marker component as part of [`ViewQuery`]
|
|
|
|
// to identify which camera(s) should run the effect.
|
2023-04-15 21:48:31 +00:00
|
|
|
fn run(
|
|
|
|
&self,
|
2023-08-07 23:02:32 +00:00
|
|
|
_graph: &mut RenderGraphContext,
|
2023-04-15 21:48:31 +00:00
|
|
|
render_context: &mut RenderContext,
|
Rename `WorldQueryData` & `WorldQueryFilter` to `QueryData` & `QueryFilter` (#10779)
# Rename `WorldQueryData` & `WorldQueryFilter` to `QueryData` &
`QueryFilter`
Fixes #10776
## Solution
Traits `WorldQueryData` & `WorldQueryFilter` were renamed to `QueryData`
and `QueryFilter`, respectively. Related Trait types were also renamed.
---
## Changelog
- Trait `WorldQueryData` has been renamed to `QueryData`. Derive macro's
`QueryData` attribute `world_query_data` has been renamed to
`query_data`.
- Trait `WorldQueryFilter` has been renamed to `QueryFilter`. Derive
macro's `QueryFilter` attribute `world_query_filter` has been renamed to
`query_filter`.
- Trait's `ExtractComponent` type `Query` has been renamed to `Data`.
- Trait's `GetBatchData` types `Query` & `QueryFilter` has been renamed
to `Data` & `Filter`, respectively.
- Trait's `ExtractInstance` type `Query` has been renamed to `Data`.
- Trait's `ViewNode` type `ViewQuery` has been renamed to `ViewData`.
- Trait's `RenderCommand` types `ViewWorldQuery` & `ItemWorldQuery` has
been renamed to `ViewData` & `ItemData`, respectively.
## Migration Guide
Note: if merged before 0.13 is released, this should instead modify the
migration guide of #10776 with the updated names.
- Rename `WorldQueryData` & `WorldQueryFilter` trait usages to
`QueryData` & `QueryFilter` and their respective derive macro attributes
`world_query_data` & `world_query_filter` to `query_data` &
`query_filter`.
- Rename the following trait type usages:
- Trait's `ExtractComponent` type `Query` to `Data`.
- Trait's `GetBatchData` type `Query` to `Data`.
- Trait's `ExtractInstance` type `Query` to `Data`.
- Trait's `ViewNode` type `ViewQuery` to `ViewData`'
- Trait's `RenderCommand` types `ViewWolrdQuery` & `ItemWorldQuery` to
`ViewData` & `ItemData`, respectively.
```rust
// Before
#[derive(WorldQueryData)]
#[world_query_data(derive(Debug))]
struct EmptyQuery {
empty: (),
}
// After
#[derive(QueryData)]
#[query_data(derive(Debug))]
struct EmptyQuery {
empty: (),
}
// Before
#[derive(WorldQueryFilter)]
struct CustomQueryFilter<T: Component, P: Component> {
_c: With<ComponentC>,
_d: With<ComponentD>,
_or: Or<(Added<ComponentC>, Changed<ComponentD>, Without<ComponentZ>)>,
_generic_tuple: (With<T>, With<P>),
}
// After
#[derive(QueryFilter)]
struct CustomQueryFilter<T: Component, P: Component> {
_c: With<ComponentC>,
_d: With<ComponentD>,
_or: Or<(Added<ComponentC>, Changed<ComponentD>, Without<ComponentZ>)>,
_generic_tuple: (With<T>, With<P>),
}
// Before
impl ExtractComponent for ContrastAdaptiveSharpeningSettings {
type Query = &'static Self;
type Filter = With<Camera>;
type Out = (DenoiseCAS, CASUniform);
fn extract_component(item: QueryItem<Self::Query>) -> Option<Self::Out> {
//...
}
}
// After
impl ExtractComponent for ContrastAdaptiveSharpeningSettings {
type Data = &'static Self;
type Filter = With<Camera>;
type Out = (DenoiseCAS, CASUniform);
fn extract_component(item: QueryItem<Self::Data>) -> Option<Self::Out> {
//...
}
}
// Before
impl GetBatchData for MeshPipeline {
type Param = SRes<RenderMeshInstances>;
type Query = Entity;
type QueryFilter = With<Mesh3d>;
type CompareData = (MaterialBindGroupId, AssetId<Mesh>);
type BufferData = MeshUniform;
fn get_batch_data(
mesh_instances: &SystemParamItem<Self::Param>,
entity: &QueryItem<Self::Query>,
) -> (Self::BufferData, Option<Self::CompareData>) {
// ....
}
}
// After
impl GetBatchData for MeshPipeline {
type Param = SRes<RenderMeshInstances>;
type Data = Entity;
type Filter = With<Mesh3d>;
type CompareData = (MaterialBindGroupId, AssetId<Mesh>);
type BufferData = MeshUniform;
fn get_batch_data(
mesh_instances: &SystemParamItem<Self::Param>,
entity: &QueryItem<Self::Data>,
) -> (Self::BufferData, Option<Self::CompareData>) {
// ....
}
}
// Before
impl<A> ExtractInstance for AssetId<A>
where
A: Asset,
{
type Query = Read<Handle<A>>;
type Filter = ();
fn extract(item: QueryItem<'_, Self::Query>) -> Option<Self> {
Some(item.id())
}
}
// After
impl<A> ExtractInstance for AssetId<A>
where
A: Asset,
{
type Data = Read<Handle<A>>;
type Filter = ();
fn extract(item: QueryItem<'_, Self::Data>) -> Option<Self> {
Some(item.id())
}
}
// Before
impl ViewNode for PostProcessNode {
type ViewQuery = (
&'static ViewTarget,
&'static PostProcessSettings,
);
fn run(
&self,
_graph: &mut RenderGraphContext,
render_context: &mut RenderContext,
(view_target, _post_process_settings): QueryItem<Self::ViewQuery>,
world: &World,
) -> Result<(), NodeRunError> {
// ...
}
}
// After
impl ViewNode for PostProcessNode {
type ViewData = (
&'static ViewTarget,
&'static PostProcessSettings,
);
fn run(
&self,
_graph: &mut RenderGraphContext,
render_context: &mut RenderContext,
(view_target, _post_process_settings): QueryItem<Self::ViewData>,
world: &World,
) -> Result<(), NodeRunError> {
// ...
}
}
// Before
impl<P: CachedRenderPipelinePhaseItem> RenderCommand<P> for SetItemPipeline {
type Param = SRes<PipelineCache>;
type ViewWorldQuery = ();
type ItemWorldQuery = ();
#[inline]
fn render<'w>(
item: &P,
_view: (),
_entity: (),
pipeline_cache: SystemParamItem<'w, '_, Self::Param>,
pass: &mut TrackedRenderPass<'w>,
) -> RenderCommandResult {
// ...
}
}
// After
impl<P: CachedRenderPipelinePhaseItem> RenderCommand<P> for SetItemPipeline {
type Param = SRes<PipelineCache>;
type ViewData = ();
type ItemData = ();
#[inline]
fn render<'w>(
item: &P,
_view: (),
_entity: (),
pipeline_cache: SystemParamItem<'w, '_, Self::Param>,
pass: &mut TrackedRenderPass<'w>,
) -> RenderCommandResult {
// ...
}
}
```
2023-12-12 19:45:50 +00:00
|
|
|
(view_target, _post_process_settings): QueryItem<Self::ViewData>,
|
2023-04-15 21:48:31 +00:00
|
|
|
world: &World,
|
|
|
|
) -> Result<(), NodeRunError> {
|
2023-08-07 23:02:32 +00:00
|
|
|
// Get the pipeline resource that contains the global data we need
|
|
|
|
// to create the render pipeline
|
2023-04-15 21:48:31 +00:00
|
|
|
let post_process_pipeline = world.resource::<PostProcessPipeline>();
|
2022-06-06 00:06:49 +00:00
|
|
|
|
2023-04-15 21:48:31 +00:00
|
|
|
// The pipeline cache is a cache of all previously created pipelines.
|
2023-08-07 23:02:32 +00:00
|
|
|
// It is required to avoid creating a new pipeline each frame,
|
|
|
|
// which is expensive due to shader compilation.
|
2023-04-15 21:48:31 +00:00
|
|
|
let pipeline_cache = world.resource::<PipelineCache>();
|
2022-06-06 00:06:49 +00:00
|
|
|
|
2023-04-15 21:48:31 +00:00
|
|
|
// Get the pipeline from the cache
|
2023-08-25 12:34:24 +00:00
|
|
|
let Some(pipeline) = pipeline_cache.get_render_pipeline(post_process_pipeline.pipeline_id)
|
|
|
|
else {
|
2023-04-15 21:48:31 +00:00
|
|
|
return Ok(());
|
|
|
|
};
|
|
|
|
|
|
|
|
// Get the settings uniform binding
|
|
|
|
let settings_uniforms = world.resource::<ComponentUniforms<PostProcessSettings>>();
|
|
|
|
let Some(settings_binding) = settings_uniforms.uniforms().binding() else {
|
|
|
|
return Ok(());
|
|
|
|
};
|
|
|
|
|
|
|
|
// This will start a new "post process write", obtaining two texture
|
|
|
|
// views from the view target - a `source` and a `destination`.
|
|
|
|
// `source` is the "current" main texture and you _must_ write into
|
|
|
|
// `destination` because calling `post_process_write()` on the
|
|
|
|
// [`ViewTarget`] will internally flip the [`ViewTarget`]'s main
|
|
|
|
// texture to the `destination` texture. Failing to do so will cause
|
|
|
|
// the current main texture information to be lost.
|
|
|
|
let post_process = view_target.post_process_write();
|
|
|
|
|
|
|
|
// The bind_group gets created each frame.
|
|
|
|
//
|
2023-08-07 23:02:32 +00:00
|
|
|
// Normally, you would create a bind_group in the Queue set,
|
|
|
|
// but this doesn't work with the post_process_write().
|
2023-04-15 21:48:31 +00:00
|
|
|
// The reason it doesn't work is because each post_process_write will alternate the source/destination.
|
2023-08-07 23:02:32 +00:00
|
|
|
// The only way to have the correct source/destination for the bind_group
|
|
|
|
// is to make sure you get it during the node execution.
|
Bind group entries (#9694)
# Objective
Simplify bind group creation code. alternative to (and based on) #9476
## Solution
- Add a `BindGroupEntries` struct that can transparently be used where
`&[BindGroupEntry<'b>]` is required in BindGroupDescriptors.
Allows constructing the descriptor's entries as:
```rust
render_device.create_bind_group(
"my_bind_group",
&my_layout,
&BindGroupEntries::with_indexes((
(2, &my_sampler),
(3, my_uniform),
)),
);
```
instead of
```rust
render_device.create_bind_group(
"my_bind_group",
&my_layout,
&[
BindGroupEntry {
binding: 2,
resource: BindingResource::Sampler(&my_sampler),
},
BindGroupEntry {
binding: 3,
resource: my_uniform,
},
],
);
```
or
```rust
render_device.create_bind_group(
"my_bind_group",
&my_layout,
&BindGroupEntries::sequential((&my_sampler, my_uniform)),
);
```
instead of
```rust
render_device.create_bind_group(
"my_bind_group",
&my_layout,
&[
BindGroupEntry {
binding: 0,
resource: BindingResource::Sampler(&my_sampler),
},
BindGroupEntry {
binding: 1,
resource: my_uniform,
},
],
);
```
the structs has no user facing macros, is tuple-type-based so stack
allocated, and has no noticeable impact on compile time.
- Also adds a `DynamicBindGroupEntries` struct with a similar api that
uses a `Vec` under the hood and allows extending the entries.
- Modifies `RenderDevice::create_bind_group` to take separate arguments
`label`, `layout` and `entries` instead of a `BindGroupDescriptor`
struct. The struct can't be stored due to the internal references, and
with only 3 members arguably does not add enough context to justify
itself.
- Modify the codebase to use the new api and the `BindGroupEntries` /
`DynamicBindGroupEntries` structs where appropriate (whenever the
entries slice contains more than 1 member).
## Migration Guide
- Calls to `RenderDevice::create_bind_group({BindGroupDescriptor {
label, layout, entries })` must be amended to
`RenderDevice::create_bind_group(label, layout, entries)`.
- If `label`s have been specified as `"bind_group_name".into()`, they
need to change to just `"bind_group_name"`. `Some("bind_group_name")`
and `None` will still work, but `Some("bind_group_name")` can optionally
be simplified to just `"bind_group_name"`.
---------
Co-authored-by: IceSentry <IceSentry@users.noreply.github.com>
2023-10-21 15:39:22 +00:00
|
|
|
let bind_group = render_context.render_device().create_bind_group(
|
|
|
|
"post_process_bind_group",
|
|
|
|
&post_process_pipeline.layout,
|
|
|
|
// It's important for this to match the BindGroupLayout defined in the PostProcessPipeline
|
|
|
|
&BindGroupEntries::sequential((
|
|
|
|
// Make sure to use the source view
|
|
|
|
post_process.source,
|
|
|
|
// Use the sampler created for the pipeline
|
|
|
|
&post_process_pipeline.sampler,
|
|
|
|
// Set the settings binding
|
|
|
|
settings_binding.clone(),
|
|
|
|
)),
|
|
|
|
);
|
2023-04-15 21:48:31 +00:00
|
|
|
|
|
|
|
// Begin the render pass
|
|
|
|
let mut render_pass = render_context.begin_tracked_render_pass(RenderPassDescriptor {
|
|
|
|
label: Some("post_process_pass"),
|
|
|
|
color_attachments: &[Some(RenderPassColorAttachment {
|
|
|
|
// We need to specify the post process destination view here
|
|
|
|
// to make sure we write to the appropriate texture.
|
|
|
|
view: post_process.destination,
|
|
|
|
resolve_target: None,
|
|
|
|
ops: Operations::default(),
|
|
|
|
})],
|
|
|
|
depth_stencil_attachment: None,
|
2023-12-14 02:45:47 +00:00
|
|
|
timestamp_writes: None,
|
|
|
|
occlusion_query_set: None,
|
2023-04-15 21:48:31 +00:00
|
|
|
});
|
|
|
|
|
|
|
|
// This is mostly just wgpu boilerplate for drawing a fullscreen triangle,
|
|
|
|
// using the pipeline/bind_group created above
|
|
|
|
render_pass.set_render_pipeline(pipeline);
|
|
|
|
render_pass.set_bind_group(0, &bind_group, &[]);
|
|
|
|
render_pass.draw(0..3, 0..1);
|
|
|
|
|
|
|
|
Ok(())
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
// This contains global data used by the render pipeline. This will be created once on startup.
|
|
|
|
#[derive(Resource)]
|
|
|
|
struct PostProcessPipeline {
|
|
|
|
layout: BindGroupLayout,
|
|
|
|
sampler: Sampler,
|
|
|
|
pipeline_id: CachedRenderPipelineId,
|
|
|
|
}
|
|
|
|
|
|
|
|
impl FromWorld for PostProcessPipeline {
|
|
|
|
fn from_world(world: &mut World) -> Self {
|
|
|
|
let render_device = world.resource::<RenderDevice>();
|
|
|
|
|
|
|
|
// We need to define the bind group layout used for our pipeline
|
Bind group layout entries (#10224)
# Objective
- Follow up to #9694
## Solution
- Same api as #9694 but adapted for `BindGroupLayoutEntry`
- Use the same `ShaderStages` visibilty for all entries by default
- Add `BindingType` helper function that mirror the wgsl equivalent and
that make writing layouts much simpler.
Before:
```rust
let layout = render_device.create_bind_group_layout(&BindGroupLayoutDescriptor {
label: Some("post_process_bind_group_layout"),
entries: &[
BindGroupLayoutEntry {
binding: 0,
visibility: ShaderStages::FRAGMENT,
ty: BindingType::Texture {
sample_type: TextureSampleType::Float { filterable: true },
view_dimension: TextureViewDimension::D2,
multisampled: false,
},
count: None,
},
BindGroupLayoutEntry {
binding: 1,
visibility: ShaderStages::FRAGMENT,
ty: BindingType::Sampler(SamplerBindingType::Filtering),
count: None,
},
BindGroupLayoutEntry {
binding: 2,
visibility: ShaderStages::FRAGMENT,
ty: BindingType::Buffer {
ty: bevy::render::render_resource::BufferBindingType::Uniform,
has_dynamic_offset: false,
min_binding_size: Some(PostProcessSettings::min_size()),
},
count: None,
},
],
});
```
After:
```rust
let layout = render_device.create_bind_group_layout(
"post_process_bind_group_layout"),
&BindGroupLayoutEntries::sequential(
ShaderStages::FRAGMENT,
(
texture_2d_f32(),
sampler(SamplerBindingType::Filtering),
uniform_buffer(false, Some(PostProcessSettings::min_size())),
),
),
);
```
Here's a more extreme example in bevy_solari:
https://github.com/JMS55/bevy/pull/15/commits/86dab7f5da23da45d4ab668ae30553dadb816d8f
---
## Changelog
- Added `BindGroupLayoutEntries` and all `BindingType` helper functions.
## Migration Guide
`RenderDevice::create_bind_group_layout()` doesn't take a
`BindGroupLayoutDescriptor` anymore. You need to provide the parameters
separately
```rust
// 0.12
let layout = render_device.create_bind_group_layout(&BindGroupLayoutDescriptor {
label: Some("post_process_bind_group_layout"),
entries: &[
BindGroupLayoutEntry {
// ...
},
],
});
// 0.13
let layout = render_device.create_bind_group_layout(
"post_process_bind_group_layout",
&[
BindGroupLayoutEntry {
// ...
},
],
);
```
## TODO
- [x] implement a `Dynamic` variant
- [x] update the `RenderDevice::create_bind_group_layout()` api to match
the one from `RenderDevice::creat_bind_group()`
- [x] docs
2023-11-28 04:00:49 +00:00
|
|
|
let layout = render_device.create_bind_group_layout(
|
|
|
|
"post_process_bind_group_layout",
|
|
|
|
&BindGroupLayoutEntries::sequential(
|
|
|
|
// The layout entries will only be visible in the fragment stage
|
|
|
|
ShaderStages::FRAGMENT,
|
|
|
|
(
|
|
|
|
// The screen texture
|
|
|
|
texture_2d(TextureSampleType::Float { filterable: true }),
|
|
|
|
// The sampler that will be used to sample the screen texture
|
|
|
|
sampler(SamplerBindingType::Filtering),
|
|
|
|
// The settings uniform that will control the effect
|
|
|
|
uniform_buffer::<PostProcessSettings>(false),
|
|
|
|
),
|
|
|
|
),
|
|
|
|
);
|
2023-04-15 21:48:31 +00:00
|
|
|
|
|
|
|
// We can create the sampler here since it won't change at runtime and doesn't depend on the view
|
|
|
|
let sampler = render_device.create_sampler(&SamplerDescriptor::default());
|
|
|
|
|
|
|
|
// Get the shader handle
|
|
|
|
let shader = world
|
|
|
|
.resource::<AssetServer>()
|
|
|
|
.load("shaders/post_processing.wgsl");
|
|
|
|
|
|
|
|
let pipeline_id = world
|
|
|
|
.resource_mut::<PipelineCache>()
|
|
|
|
// This will add the pipeline to the cache and queue it's creation
|
|
|
|
.queue_render_pipeline(RenderPipelineDescriptor {
|
|
|
|
label: Some("post_process_pipeline".into()),
|
|
|
|
layout: vec![layout.clone()],
|
|
|
|
// This will setup a fullscreen triangle for the vertex state
|
|
|
|
vertex: fullscreen_shader_vertex_state(),
|
|
|
|
fragment: Some(FragmentState {
|
|
|
|
shader,
|
|
|
|
shader_defs: vec![],
|
|
|
|
// Make sure this matches the entry point of your shader.
|
|
|
|
// It can be anything as long as it matches here and in the shader.
|
|
|
|
entry_point: "fragment".into(),
|
|
|
|
targets: vec![Some(ColorTargetState {
|
|
|
|
format: TextureFormat::bevy_default(),
|
|
|
|
blend: None,
|
|
|
|
write_mask: ColorWrites::ALL,
|
|
|
|
})],
|
|
|
|
}),
|
2023-07-26 06:04:01 +00:00
|
|
|
// All of the following properties are not important for this effect so just use the default values.
|
|
|
|
// This struct doesn't have the Default trait implemented because not all field can have a default value.
|
2023-04-15 21:48:31 +00:00
|
|
|
primitive: PrimitiveState::default(),
|
|
|
|
depth_stencil: None,
|
|
|
|
multisample: MultisampleState::default(),
|
|
|
|
push_constant_ranges: vec![],
|
|
|
|
});
|
|
|
|
|
|
|
|
Self {
|
|
|
|
layout,
|
|
|
|
sampler,
|
|
|
|
pipeline_id,
|
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
// This is the component that will get passed to the shader
|
|
|
|
#[derive(Component, Default, Clone, Copy, ExtractComponent, ShaderType)]
|
|
|
|
struct PostProcessSettings {
|
|
|
|
intensity: f32,
|
2023-08-04 17:44:29 +00:00
|
|
|
// WebGL2 structs must be 16 byte aligned.
|
|
|
|
#[cfg(feature = "webgl2")]
|
|
|
|
_webgl2_padding: Vec3,
|
2023-04-15 21:48:31 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
/// Set up a simple 3D scene
|
|
|
|
fn setup(
|
|
|
|
mut commands: Commands,
|
|
|
|
mut meshes: ResMut<Assets<Mesh>>,
|
|
|
|
mut materials: ResMut<Assets<StandardMaterial>>,
|
|
|
|
) {
|
|
|
|
// camera
|
Spawn now takes a Bundle (#6054)
# Objective
Now that we can consolidate Bundles and Components under a single insert (thanks to #2975 and #6039), almost 100% of world spawns now look like `world.spawn().insert((Some, Tuple, Here))`. Spawning an entity without any components is an extremely uncommon pattern, so it makes sense to give spawn the "first class" ergonomic api. This consolidated api should be made consistent across all spawn apis (such as World and Commands).
## Solution
All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input:
```rust
// before:
commands
.spawn()
.insert((A, B, C));
world
.spawn()
.insert((A, B, C);
// after
commands.spawn((A, B, C));
world.spawn((A, B, C));
```
All existing instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api. A new `spawn_empty` has been added, replacing the old `spawn` api.
By allowing `world.spawn(some_bundle)` to replace `world.spawn().insert(some_bundle)`, this opened the door to removing the initial entity allocation in the "empty" archetype / table done in `spawn()` (and subsequent move to the actual archetype in `.insert(some_bundle)`).
This improves spawn performance by over 10%:
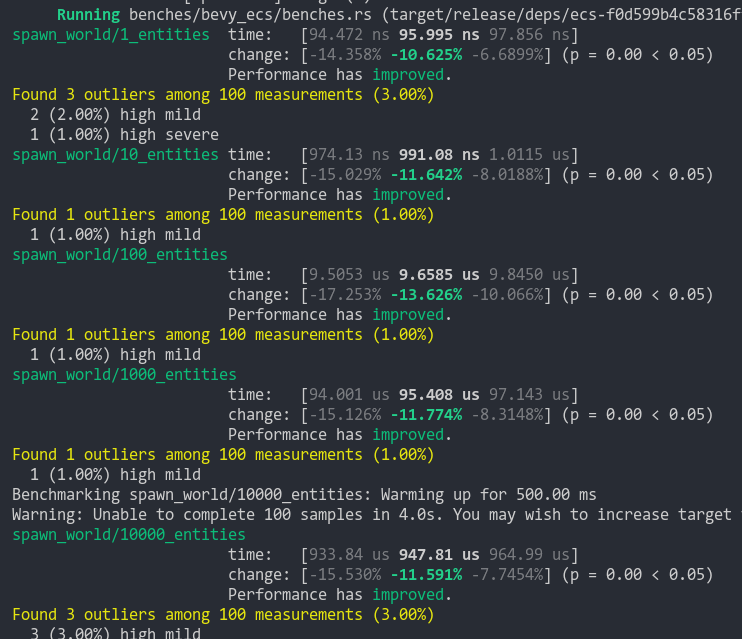
To take this measurement, I added a new `world_spawn` benchmark.
Unfortunately, optimizing `Commands::spawn` is slightly less trivial, as Commands expose the Entity id of spawned entities prior to actually spawning. Doing the optimization would (naively) require assurances that the `spawn(some_bundle)` command is applied before all other commands involving the entity (which would not necessarily be true, if memory serves). Optimizing `Commands::spawn` this way does feel possible, but it will require careful thought (and maybe some additional checks), which deserves its own PR. For now, it has the same performance characteristics of the current `Commands::spawn_bundle` on main.
**Note that 99% of this PR is simple renames and refactors. The only code that needs careful scrutiny is the new `World::spawn()` impl, which is relatively straightforward, but it has some new unsafe code (which re-uses battle tested BundlerSpawner code path).**
---
## Changelog
- All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input
- All instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api
- World and Commands now have `spawn_empty()`, which is equivalent to the old `spawn()` behavior.
## Migration Guide
```rust
// Old (0.8):
commands
.spawn()
.insert_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
commands.spawn_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
let entity = commands.spawn().id();
// New (0.9)
let entity = commands.spawn_empty().id();
// Old (0.8)
let entity = world.spawn().id();
// New (0.9)
let entity = world.spawn_empty();
```
2022-09-23 19:55:54 +00:00
|
|
|
commands.spawn((
|
2023-04-15 21:48:31 +00:00
|
|
|
Camera3dBundle {
|
|
|
|
transform: Transform::from_translation(Vec3::new(0.0, 0.0, 5.0))
|
|
|
|
.looking_at(Vec3::default(), Vec3::Y),
|
|
|
|
camera_3d: Camera3d {
|
2023-11-26 20:48:03 +00:00
|
|
|
clear_color: Color::WHITE.into(),
|
2022-06-06 00:06:49 +00:00
|
|
|
..default()
|
|
|
|
},
|
|
|
|
..default()
|
Spawn now takes a Bundle (#6054)
# Objective
Now that we can consolidate Bundles and Components under a single insert (thanks to #2975 and #6039), almost 100% of world spawns now look like `world.spawn().insert((Some, Tuple, Here))`. Spawning an entity without any components is an extremely uncommon pattern, so it makes sense to give spawn the "first class" ergonomic api. This consolidated api should be made consistent across all spawn apis (such as World and Commands).
## Solution
All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input:
```rust
// before:
commands
.spawn()
.insert((A, B, C));
world
.spawn()
.insert((A, B, C);
// after
commands.spawn((A, B, C));
world.spawn((A, B, C));
```
All existing instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api. A new `spawn_empty` has been added, replacing the old `spawn` api.
By allowing `world.spawn(some_bundle)` to replace `world.spawn().insert(some_bundle)`, this opened the door to removing the initial entity allocation in the "empty" archetype / table done in `spawn()` (and subsequent move to the actual archetype in `.insert(some_bundle)`).
This improves spawn performance by over 10%:
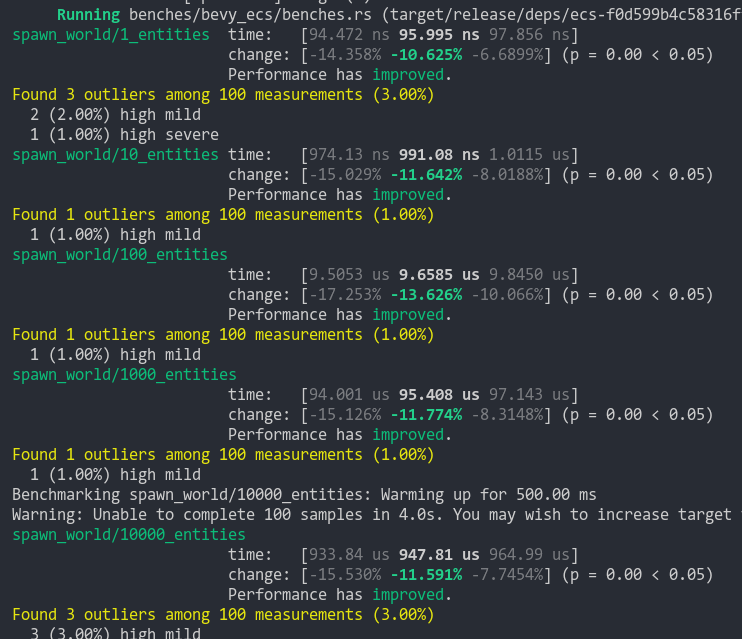
To take this measurement, I added a new `world_spawn` benchmark.
Unfortunately, optimizing `Commands::spawn` is slightly less trivial, as Commands expose the Entity id of spawned entities prior to actually spawning. Doing the optimization would (naively) require assurances that the `spawn(some_bundle)` command is applied before all other commands involving the entity (which would not necessarily be true, if memory serves). Optimizing `Commands::spawn` this way does feel possible, but it will require careful thought (and maybe some additional checks), which deserves its own PR. For now, it has the same performance characteristics of the current `Commands::spawn_bundle` on main.
**Note that 99% of this PR is simple renames and refactors. The only code that needs careful scrutiny is the new `World::spawn()` impl, which is relatively straightforward, but it has some new unsafe code (which re-uses battle tested BundlerSpawner code path).**
---
## Changelog
- All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input
- All instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api
- World and Commands now have `spawn_empty()`, which is equivalent to the old `spawn()` behavior.
## Migration Guide
```rust
// Old (0.8):
commands
.spawn()
.insert_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
commands.spawn_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
let entity = commands.spawn().id();
// New (0.9)
let entity = commands.spawn_empty().id();
// Old (0.8)
let entity = world.spawn().id();
// New (0.9)
let entity = world.spawn_empty();
```
2022-09-23 19:55:54 +00:00
|
|
|
},
|
2023-04-15 21:48:31 +00:00
|
|
|
// Add the setting to the camera.
|
|
|
|
// This component is also used to determine on which camera to run the post processing effect.
|
2023-08-04 17:44:29 +00:00
|
|
|
PostProcessSettings {
|
|
|
|
intensity: 0.02,
|
|
|
|
..default()
|
|
|
|
},
|
Spawn now takes a Bundle (#6054)
# Objective
Now that we can consolidate Bundles and Components under a single insert (thanks to #2975 and #6039), almost 100% of world spawns now look like `world.spawn().insert((Some, Tuple, Here))`. Spawning an entity without any components is an extremely uncommon pattern, so it makes sense to give spawn the "first class" ergonomic api. This consolidated api should be made consistent across all spawn apis (such as World and Commands).
## Solution
All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input:
```rust
// before:
commands
.spawn()
.insert((A, B, C));
world
.spawn()
.insert((A, B, C);
// after
commands.spawn((A, B, C));
world.spawn((A, B, C));
```
All existing instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api. A new `spawn_empty` has been added, replacing the old `spawn` api.
By allowing `world.spawn(some_bundle)` to replace `world.spawn().insert(some_bundle)`, this opened the door to removing the initial entity allocation in the "empty" archetype / table done in `spawn()` (and subsequent move to the actual archetype in `.insert(some_bundle)`).
This improves spawn performance by over 10%:
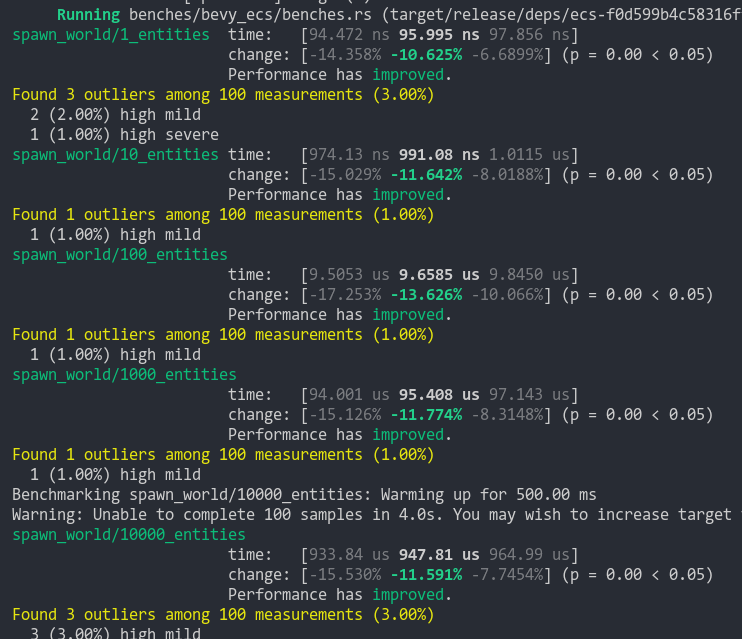
To take this measurement, I added a new `world_spawn` benchmark.
Unfortunately, optimizing `Commands::spawn` is slightly less trivial, as Commands expose the Entity id of spawned entities prior to actually spawning. Doing the optimization would (naively) require assurances that the `spawn(some_bundle)` command is applied before all other commands involving the entity (which would not necessarily be true, if memory serves). Optimizing `Commands::spawn` this way does feel possible, but it will require careful thought (and maybe some additional checks), which deserves its own PR. For now, it has the same performance characteristics of the current `Commands::spawn_bundle` on main.
**Note that 99% of this PR is simple renames and refactors. The only code that needs careful scrutiny is the new `World::spawn()` impl, which is relatively straightforward, but it has some new unsafe code (which re-uses battle tested BundlerSpawner code path).**
---
## Changelog
- All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input
- All instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api
- World and Commands now have `spawn_empty()`, which is equivalent to the old `spawn()` behavior.
## Migration Guide
```rust
// Old (0.8):
commands
.spawn()
.insert_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
commands.spawn_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
let entity = commands.spawn().id();
// New (0.9)
let entity = commands.spawn_empty().id();
// Old (0.8)
let entity = world.spawn().id();
// New (0.9)
let entity = world.spawn_empty();
```
2022-09-23 19:55:54 +00:00
|
|
|
));
|
2022-06-06 00:06:49 +00:00
|
|
|
|
2023-04-15 21:48:31 +00:00
|
|
|
// cube
|
Spawn now takes a Bundle (#6054)
# Objective
Now that we can consolidate Bundles and Components under a single insert (thanks to #2975 and #6039), almost 100% of world spawns now look like `world.spawn().insert((Some, Tuple, Here))`. Spawning an entity without any components is an extremely uncommon pattern, so it makes sense to give spawn the "first class" ergonomic api. This consolidated api should be made consistent across all spawn apis (such as World and Commands).
## Solution
All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input:
```rust
// before:
commands
.spawn()
.insert((A, B, C));
world
.spawn()
.insert((A, B, C);
// after
commands.spawn((A, B, C));
world.spawn((A, B, C));
```
All existing instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api. A new `spawn_empty` has been added, replacing the old `spawn` api.
By allowing `world.spawn(some_bundle)` to replace `world.spawn().insert(some_bundle)`, this opened the door to removing the initial entity allocation in the "empty" archetype / table done in `spawn()` (and subsequent move to the actual archetype in `.insert(some_bundle)`).
This improves spawn performance by over 10%:
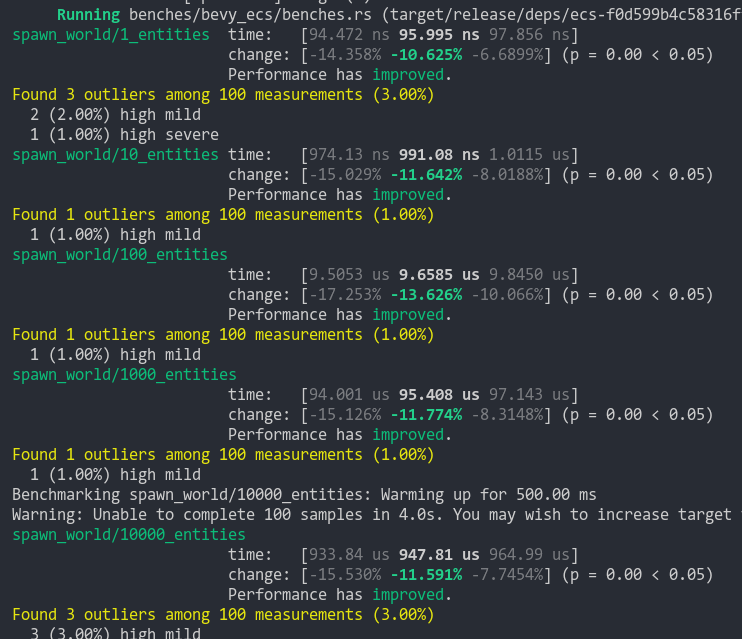
To take this measurement, I added a new `world_spawn` benchmark.
Unfortunately, optimizing `Commands::spawn` is slightly less trivial, as Commands expose the Entity id of spawned entities prior to actually spawning. Doing the optimization would (naively) require assurances that the `spawn(some_bundle)` command is applied before all other commands involving the entity (which would not necessarily be true, if memory serves). Optimizing `Commands::spawn` this way does feel possible, but it will require careful thought (and maybe some additional checks), which deserves its own PR. For now, it has the same performance characteristics of the current `Commands::spawn_bundle` on main.
**Note that 99% of this PR is simple renames and refactors. The only code that needs careful scrutiny is the new `World::spawn()` impl, which is relatively straightforward, but it has some new unsafe code (which re-uses battle tested BundlerSpawner code path).**
---
## Changelog
- All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input
- All instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api
- World and Commands now have `spawn_empty()`, which is equivalent to the old `spawn()` behavior.
## Migration Guide
```rust
// Old (0.8):
commands
.spawn()
.insert_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
commands.spawn_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
let entity = commands.spawn().id();
// New (0.9)
let entity = commands.spawn_empty().id();
// Old (0.8)
let entity = world.spawn().id();
// New (0.9)
let entity = world.spawn_empty();
```
2022-09-23 19:55:54 +00:00
|
|
|
commands.spawn((
|
2023-04-15 21:48:31 +00:00
|
|
|
PbrBundle {
|
|
|
|
mesh: meshes.add(Mesh::from(shape::Cube { size: 1.0 })),
|
|
|
|
material: materials.add(Color::rgb(0.8, 0.7, 0.6).into()),
|
|
|
|
transform: Transform::from_xyz(0.0, 0.5, 0.0),
|
|
|
|
..default()
|
Spawn now takes a Bundle (#6054)
# Objective
Now that we can consolidate Bundles and Components under a single insert (thanks to #2975 and #6039), almost 100% of world spawns now look like `world.spawn().insert((Some, Tuple, Here))`. Spawning an entity without any components is an extremely uncommon pattern, so it makes sense to give spawn the "first class" ergonomic api. This consolidated api should be made consistent across all spawn apis (such as World and Commands).
## Solution
All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input:
```rust
// before:
commands
.spawn()
.insert((A, B, C));
world
.spawn()
.insert((A, B, C);
// after
commands.spawn((A, B, C));
world.spawn((A, B, C));
```
All existing instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api. A new `spawn_empty` has been added, replacing the old `spawn` api.
By allowing `world.spawn(some_bundle)` to replace `world.spawn().insert(some_bundle)`, this opened the door to removing the initial entity allocation in the "empty" archetype / table done in `spawn()` (and subsequent move to the actual archetype in `.insert(some_bundle)`).
This improves spawn performance by over 10%:
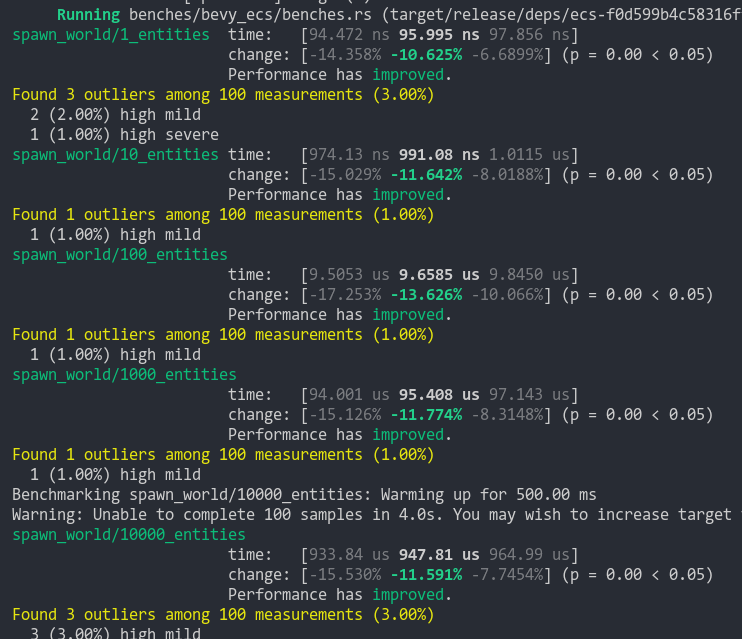
To take this measurement, I added a new `world_spawn` benchmark.
Unfortunately, optimizing `Commands::spawn` is slightly less trivial, as Commands expose the Entity id of spawned entities prior to actually spawning. Doing the optimization would (naively) require assurances that the `spawn(some_bundle)` command is applied before all other commands involving the entity (which would not necessarily be true, if memory serves). Optimizing `Commands::spawn` this way does feel possible, but it will require careful thought (and maybe some additional checks), which deserves its own PR. For now, it has the same performance characteristics of the current `Commands::spawn_bundle` on main.
**Note that 99% of this PR is simple renames and refactors. The only code that needs careful scrutiny is the new `World::spawn()` impl, which is relatively straightforward, but it has some new unsafe code (which re-uses battle tested BundlerSpawner code path).**
---
## Changelog
- All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input
- All instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api
- World and Commands now have `spawn_empty()`, which is equivalent to the old `spawn()` behavior.
## Migration Guide
```rust
// Old (0.8):
commands
.spawn()
.insert_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
commands.spawn_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
let entity = commands.spawn().id();
// New (0.9)
let entity = commands.spawn_empty().id();
// Old (0.8)
let entity = world.spawn().id();
// New (0.9)
let entity = world.spawn_empty();
```
2022-09-23 19:55:54 +00:00
|
|
|
},
|
2023-04-15 21:48:31 +00:00
|
|
|
Rotates,
|
Spawn now takes a Bundle (#6054)
# Objective
Now that we can consolidate Bundles and Components under a single insert (thanks to #2975 and #6039), almost 100% of world spawns now look like `world.spawn().insert((Some, Tuple, Here))`. Spawning an entity without any components is an extremely uncommon pattern, so it makes sense to give spawn the "first class" ergonomic api. This consolidated api should be made consistent across all spawn apis (such as World and Commands).
## Solution
All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input:
```rust
// before:
commands
.spawn()
.insert((A, B, C));
world
.spawn()
.insert((A, B, C);
// after
commands.spawn((A, B, C));
world.spawn((A, B, C));
```
All existing instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api. A new `spawn_empty` has been added, replacing the old `spawn` api.
By allowing `world.spawn(some_bundle)` to replace `world.spawn().insert(some_bundle)`, this opened the door to removing the initial entity allocation in the "empty" archetype / table done in `spawn()` (and subsequent move to the actual archetype in `.insert(some_bundle)`).
This improves spawn performance by over 10%:
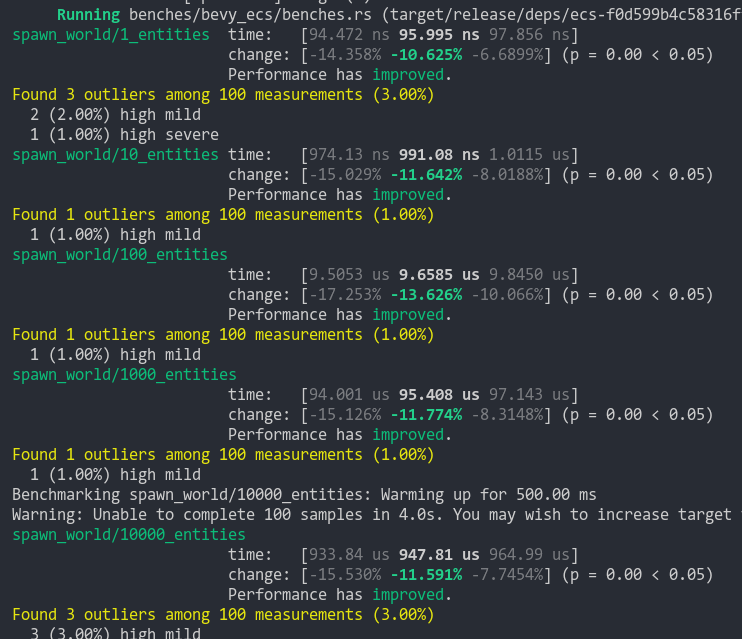
To take this measurement, I added a new `world_spawn` benchmark.
Unfortunately, optimizing `Commands::spawn` is slightly less trivial, as Commands expose the Entity id of spawned entities prior to actually spawning. Doing the optimization would (naively) require assurances that the `spawn(some_bundle)` command is applied before all other commands involving the entity (which would not necessarily be true, if memory serves). Optimizing `Commands::spawn` this way does feel possible, but it will require careful thought (and maybe some additional checks), which deserves its own PR. For now, it has the same performance characteristics of the current `Commands::spawn_bundle` on main.
**Note that 99% of this PR is simple renames and refactors. The only code that needs careful scrutiny is the new `World::spawn()` impl, which is relatively straightforward, but it has some new unsafe code (which re-uses battle tested BundlerSpawner code path).**
---
## Changelog
- All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input
- All instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api
- World and Commands now have `spawn_empty()`, which is equivalent to the old `spawn()` behavior.
## Migration Guide
```rust
// Old (0.8):
commands
.spawn()
.insert_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
commands.spawn_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
let entity = commands.spawn().id();
// New (0.9)
let entity = commands.spawn_empty().id();
// Old (0.8)
let entity = world.spawn().id();
// New (0.9)
let entity = world.spawn_empty();
```
2022-09-23 19:55:54 +00:00
|
|
|
));
|
2023-04-15 21:48:31 +00:00
|
|
|
// light
|
|
|
|
commands.spawn(PointLightBundle {
|
|
|
|
transform: Transform::from_translation(Vec3::new(0.0, 0.0, 10.0)),
|
|
|
|
..default()
|
|
|
|
});
|
2022-06-06 00:06:49 +00:00
|
|
|
}
|
|
|
|
|
2023-04-15 21:48:31 +00:00
|
|
|
#[derive(Component)]
|
|
|
|
struct Rotates;
|
|
|
|
|
|
|
|
/// Rotates any entity around the x and y axis
|
|
|
|
fn rotate(time: Res<Time>, mut query: Query<&mut Transform, With<Rotates>>) {
|
2022-07-11 15:28:50 +00:00
|
|
|
for mut transform in &mut query {
|
2022-07-01 03:58:54 +00:00
|
|
|
transform.rotate_x(0.55 * time.delta_seconds());
|
|
|
|
transform.rotate_z(0.15 * time.delta_seconds());
|
2022-06-06 00:06:49 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2023-04-15 21:48:31 +00:00
|
|
|
// Change the intensity over time to show that the effect is controlled from the main world
|
|
|
|
fn update_settings(mut settings: Query<&mut PostProcessSettings>, time: Res<Time>) {
|
|
|
|
for mut setting in &mut settings {
|
|
|
|
let mut intensity = time.elapsed_seconds().sin();
|
|
|
|
// Make it loop periodically
|
|
|
|
intensity = intensity.sin();
|
|
|
|
// Remap it to 0..1 because the intensity can't be negative
|
|
|
|
intensity = intensity * 0.5 + 0.5;
|
|
|
|
// Scale it to a more reasonable level
|
|
|
|
intensity *= 0.015;
|
2022-06-06 00:06:49 +00:00
|
|
|
|
2023-08-07 23:02:32 +00:00
|
|
|
// Set the intensity.
|
|
|
|
// This will then be extracted to the render world and uploaded to the gpu automatically by the [`UniformComponentPlugin`]
|
2023-04-15 21:48:31 +00:00
|
|
|
setting.intensity = intensity;
|
2022-06-06 00:06:49 +00:00
|
|
|
}
|
|
|
|
}
|