2022-04-25 19:20:38 +00:00
|
|
|
use crate::{Size, UiRect};
|
2021-12-14 03:58:23 +00:00
|
|
|
use bevy_asset::Handle;
|
|
|
|
use bevy_ecs::{prelude::Component, reflect::ReflectComponent};
|
2022-09-02 12:35:23 +00:00
|
|
|
use bevy_math::{Rect, Vec2};
|
2022-05-03 19:20:13 +00:00
|
|
|
use bevy_reflect::prelude::*;
|
2021-12-14 03:58:23 +00:00
|
|
|
use bevy_render::{
|
|
|
|
color::Color,
|
|
|
|
texture::{Image, DEFAULT_IMAGE_HANDLE},
|
|
|
|
};
|
2020-11-28 00:39:59 +00:00
|
|
|
use serde::{Deserialize, Serialize};
|
2022-10-24 14:33:46 +00:00
|
|
|
use std::ops::{Div, DivAssign, Mul, MulAssign};
|
|
|
|
use thiserror::Error;
|
2020-01-13 00:51:21 +00:00
|
|
|
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Describes the size of a UI node
|
2023-01-04 19:58:09 +00:00
|
|
|
#[derive(Component, Debug, Clone, Reflect)]
|
2022-05-03 19:20:13 +00:00
|
|
|
#[reflect(Component, Default)]
|
2020-01-13 00:51:21 +00:00
|
|
|
pub struct Node {
|
2022-01-07 22:20:34 +00:00
|
|
|
/// The size of the node as width and height in pixels
|
2022-10-17 13:27:24 +00:00
|
|
|
/// automatically calculated by [`super::flex::flex_node_system`]
|
|
|
|
pub(crate) calculated_size: Vec2,
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Node {
|
|
|
|
/// The calculated node size as width and height in pixels
|
|
|
|
/// automatically calculated by [`super::flex::flex_node_system`]
|
|
|
|
pub fn size(&self) -> Vec2 {
|
|
|
|
self.calculated_size
|
|
|
|
}
|
2020-01-13 00:51:21 +00:00
|
|
|
}
|
2020-07-26 19:27:09 +00:00
|
|
|
|
2023-01-04 19:58:09 +00:00
|
|
|
impl Node {
|
|
|
|
pub const DEFAULT: Self = Self {
|
|
|
|
calculated_size: Vec2::ZERO,
|
|
|
|
};
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Default for Node {
|
|
|
|
fn default() -> Self {
|
|
|
|
Self::DEFAULT
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-01-07 22:20:34 +00:00
|
|
|
/// An enum that describes possible types of value in flexbox layout options
|
2023-01-04 19:58:09 +00:00
|
|
|
#[derive(Copy, Clone, PartialEq, Debug, Serialize, Deserialize, Reflect)]
|
bevy_reflect: Update enum derives (#5473)
> In draft until #4761 is merged. See the relevant commits [here](https://github.com/bevyengine/bevy/pull/5473/commits/a85fe94a183fdd62d512b2613376ce6eace8ab63).
---
# Objective
Update enums across Bevy to use the new enum reflection and get rid of `#[reflect_value(...)]` usages.
## Solution
Find and replace all[^1] instances of `#[reflect_value(...)]` on enum types.
---
## Changelog
- Updated all[^1] reflected enums to implement `Enum` (i.e. they are no longer `ReflectRef::Value`)
## Migration Guide
Bevy-defined enums have been updated to implement `Enum` and are not considered value types (`ReflectRef::Value`) anymore. This means that their serialized representations will need to be updated. For example, given the Bevy enum:
```rust
pub enum ScalingMode {
None,
WindowSize,
Auto { min_width: f32, min_height: f32 },
FixedVertical(f32),
FixedHorizontal(f32),
}
```
You will need to update the serialized versions accordingly.
```js
// OLD FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"value": FixedHorizontal(720),
},
// NEW FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"enum": {
"variant": "FixedHorizontal",
"tuple": [
{
"type": "f32",
"value": 720,
},
],
},
},
```
This may also have other smaller implications (such as `Debug` representation), but serialization is probably the most prominent.
[^1]: All enums except `HandleId` as neither `Uuid` nor `AssetPathId` implement the reflection traits
2022-08-02 22:40:29 +00:00
|
|
|
#[reflect(PartialEq, Serialize, Deserialize)]
|
2020-07-26 19:27:09 +00:00
|
|
|
pub enum Val {
|
2022-01-07 22:20:34 +00:00
|
|
|
/// No value defined
|
2020-07-26 19:27:09 +00:00
|
|
|
Undefined,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Automatically determine this value
|
2020-07-26 19:27:09 +00:00
|
|
|
Auto,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Set this value in pixels
|
2020-07-26 19:27:09 +00:00
|
|
|
Px(f32),
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Set this value in percent
|
2020-07-26 19:27:09 +00:00
|
|
|
Percent(f32),
|
|
|
|
}
|
|
|
|
|
2023-01-04 19:58:09 +00:00
|
|
|
impl Val {
|
|
|
|
pub const DEFAULT: Self = Self::Undefined;
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Default for Val {
|
|
|
|
fn default() -> Self {
|
|
|
|
Self::DEFAULT
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-10-24 14:33:46 +00:00
|
|
|
impl Mul<f32> for Val {
|
2020-07-26 19:27:09 +00:00
|
|
|
type Output = Val;
|
2020-07-28 21:24:03 +00:00
|
|
|
|
2022-10-24 14:33:46 +00:00
|
|
|
fn mul(self, rhs: f32) -> Self::Output {
|
2020-07-26 19:27:09 +00:00
|
|
|
match self {
|
|
|
|
Val::Undefined => Val::Undefined,
|
|
|
|
Val::Auto => Val::Auto,
|
2022-10-24 14:33:46 +00:00
|
|
|
Val::Px(value) => Val::Px(value * rhs),
|
|
|
|
Val::Percent(value) => Val::Percent(value * rhs),
|
2020-07-26 19:27:09 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-10-24 14:33:46 +00:00
|
|
|
impl MulAssign<f32> for Val {
|
|
|
|
fn mul_assign(&mut self, rhs: f32) {
|
2020-07-26 19:27:09 +00:00
|
|
|
match self {
|
2020-07-28 21:24:03 +00:00
|
|
|
Val::Undefined | Val::Auto => {}
|
2022-10-24 14:33:46 +00:00
|
|
|
Val::Px(value) | Val::Percent(value) => *value *= rhs,
|
2020-07-26 19:27:09 +00:00
|
|
|
}
|
|
|
|
}
|
2020-07-28 04:04:04 +00:00
|
|
|
}
|
|
|
|
|
2022-10-24 14:33:46 +00:00
|
|
|
impl Div<f32> for Val {
|
2022-08-01 16:27:16 +00:00
|
|
|
type Output = Val;
|
|
|
|
|
2022-10-24 14:33:46 +00:00
|
|
|
fn div(self, rhs: f32) -> Self::Output {
|
2022-08-01 16:27:16 +00:00
|
|
|
match self {
|
|
|
|
Val::Undefined => Val::Undefined,
|
|
|
|
Val::Auto => Val::Auto,
|
2022-10-24 14:33:46 +00:00
|
|
|
Val::Px(value) => Val::Px(value / rhs),
|
|
|
|
Val::Percent(value) => Val::Percent(value / rhs),
|
2022-08-01 16:27:16 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-10-24 14:33:46 +00:00
|
|
|
impl DivAssign<f32> for Val {
|
|
|
|
fn div_assign(&mut self, rhs: f32) {
|
2022-08-01 16:27:16 +00:00
|
|
|
match self {
|
|
|
|
Val::Undefined | Val::Auto => {}
|
2022-10-24 14:33:46 +00:00
|
|
|
Val::Px(value) | Val::Percent(value) => *value /= rhs,
|
2022-08-01 16:27:16 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-10-24 14:33:46 +00:00
|
|
|
#[derive(Debug, Eq, PartialEq, Clone, Copy, Error)]
|
|
|
|
pub enum ValArithmeticError {
|
|
|
|
#[error("the variants of the Vals don't match")]
|
|
|
|
NonIdenticalVariants,
|
|
|
|
#[error("the given variant of Val is not evaluateable (non-numeric)")]
|
|
|
|
NonEvaluateable,
|
|
|
|
}
|
2022-08-01 16:27:16 +00:00
|
|
|
|
2022-10-24 14:33:46 +00:00
|
|
|
impl Val {
|
|
|
|
/// Tries to add the values of two [`Val`]s.
|
|
|
|
/// Returns [`ValArithmeticError::NonIdenticalVariants`] if two [`Val`]s are of different variants.
|
|
|
|
/// When adding non-numeric [`Val`]s, it returns the value unchanged.
|
|
|
|
pub fn try_add(&self, rhs: Val) -> Result<Val, ValArithmeticError> {
|
|
|
|
match (self, rhs) {
|
|
|
|
(Val::Undefined, Val::Undefined) | (Val::Auto, Val::Auto) => Ok(*self),
|
|
|
|
(Val::Px(value), Val::Px(rhs_value)) => Ok(Val::Px(value + rhs_value)),
|
|
|
|
(Val::Percent(value), Val::Percent(rhs_value)) => Ok(Val::Percent(value + rhs_value)),
|
|
|
|
_ => Err(ValArithmeticError::NonIdenticalVariants),
|
2022-08-01 16:27:16 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-10-24 14:33:46 +00:00
|
|
|
/// Adds `rhs` to `self` and assigns the result to `self` (see [`Val::try_add`])
|
|
|
|
pub fn try_add_assign(&mut self, rhs: Val) -> Result<(), ValArithmeticError> {
|
|
|
|
*self = self.try_add(rhs)?;
|
|
|
|
Ok(())
|
|
|
|
}
|
|
|
|
|
|
|
|
/// Tries to subtract the values of two [`Val`]s.
|
|
|
|
/// Returns [`ValArithmeticError::NonIdenticalVariants`] if two [`Val`]s are of different variants.
|
|
|
|
/// When adding non-numeric [`Val`]s, it returns the value unchanged.
|
|
|
|
pub fn try_sub(&self, rhs: Val) -> Result<Val, ValArithmeticError> {
|
|
|
|
match (self, rhs) {
|
|
|
|
(Val::Undefined, Val::Undefined) | (Val::Auto, Val::Auto) => Ok(*self),
|
|
|
|
(Val::Px(value), Val::Px(rhs_value)) => Ok(Val::Px(value - rhs_value)),
|
|
|
|
(Val::Percent(value), Val::Percent(rhs_value)) => Ok(Val::Percent(value - rhs_value)),
|
|
|
|
_ => Err(ValArithmeticError::NonIdenticalVariants),
|
2022-08-01 16:27:16 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-10-24 14:33:46 +00:00
|
|
|
/// Subtracts `rhs` from `self` and assigns the result to `self` (see [`Val::try_sub`])
|
|
|
|
pub fn try_sub_assign(&mut self, rhs: Val) -> Result<(), ValArithmeticError> {
|
|
|
|
*self = self.try_sub(rhs)?;
|
|
|
|
Ok(())
|
|
|
|
}
|
2022-08-01 16:27:16 +00:00
|
|
|
|
2022-10-24 14:33:46 +00:00
|
|
|
/// A convenience function for simple evaluation of [`Val::Percent`] variant into a concrete [`Val::Px`] value.
|
|
|
|
/// Returns a [`ValArithmeticError::NonEvaluateable`] if the [`Val`] is impossible to evaluate into [`Val::Px`].
|
|
|
|
/// Otherwise it returns an [`f32`] containing the evaluated value in pixels.
|
|
|
|
///
|
|
|
|
/// **Note:** If a [`Val::Px`] is evaluated, it's innver value returned unchanged.
|
|
|
|
pub fn evaluate(&self, size: f32) -> Result<f32, ValArithmeticError> {
|
2022-08-01 16:27:16 +00:00
|
|
|
match self {
|
2022-10-24 14:33:46 +00:00
|
|
|
Val::Percent(value) => Ok(size * value / 100.0),
|
|
|
|
Val::Px(value) => Ok(*value),
|
|
|
|
_ => Err(ValArithmeticError::NonEvaluateable),
|
2022-08-01 16:27:16 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-10-24 14:33:46 +00:00
|
|
|
/// Similar to [`Val::try_add`], but performs [`Val::evaluate`] on both values before adding.
|
|
|
|
/// Returns an [`f32`] value in pixels.
|
|
|
|
pub fn try_add_with_size(&self, rhs: Val, size: f32) -> Result<f32, ValArithmeticError> {
|
|
|
|
let lhs = self.evaluate(size)?;
|
|
|
|
let rhs = rhs.evaluate(size)?;
|
|
|
|
|
|
|
|
Ok(lhs + rhs)
|
|
|
|
}
|
|
|
|
|
|
|
|
/// Similar to [`Val::try_add_assign`], but performs [`Val::evaluate`] on both values before adding.
|
|
|
|
/// The value gets converted to [`Val::Px`].
|
|
|
|
pub fn try_add_assign_with_size(
|
|
|
|
&mut self,
|
|
|
|
rhs: Val,
|
|
|
|
size: f32,
|
|
|
|
) -> Result<(), ValArithmeticError> {
|
|
|
|
*self = Val::Px(self.evaluate(size)? + rhs.evaluate(size)?);
|
|
|
|
Ok(())
|
|
|
|
}
|
|
|
|
|
|
|
|
/// Similar to [`Val::try_sub`], but performs [`Val::evaluate`] on both values before subtracting.
|
|
|
|
/// Returns an [`f32`] value in pixels.
|
|
|
|
pub fn try_sub_with_size(&self, rhs: Val, size: f32) -> Result<f32, ValArithmeticError> {
|
|
|
|
let lhs = self.evaluate(size)?;
|
|
|
|
let rhs = rhs.evaluate(size)?;
|
|
|
|
|
|
|
|
Ok(lhs - rhs)
|
|
|
|
}
|
|
|
|
|
|
|
|
/// Similar to [`Val::try_sub_assign`], but performs [`Val::evaluate`] on both values before adding.
|
|
|
|
/// The value gets converted to [`Val::Px`].
|
|
|
|
pub fn try_sub_assign_with_size(
|
|
|
|
&mut self,
|
|
|
|
rhs: Val,
|
|
|
|
size: f32,
|
|
|
|
) -> Result<(), ValArithmeticError> {
|
|
|
|
*self = Val::Px(self.try_add_with_size(rhs, size)?);
|
|
|
|
Ok(())
|
2022-08-01 16:27:16 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Describes the style of a UI node
|
|
|
|
///
|
|
|
|
/// It uses the [Flexbox](https://cssreference.io/flexbox/) system.
|
2021-10-03 19:23:44 +00:00
|
|
|
#[derive(Component, Clone, PartialEq, Debug, Reflect)]
|
2022-05-03 19:20:13 +00:00
|
|
|
#[reflect(Component, Default, PartialEq)]
|
2020-07-26 19:27:09 +00:00
|
|
|
pub struct Style {
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Whether to arrange this node and its children with flexbox layout
|
Disable UI node `Interaction` when `ComputedVisibility` is false (#5361)
# Objective
UI nodes can be hidden by setting their `Visibility` property. Since #5310 was merged, this is now ergonomic to use, as visibility is now inherited.
However, UI nodes still receive (and store) interactions when hidden, resulting in surprising hidden state (and an inability to otherwise disable UI nodes.
## Solution
Fixes #5360.
I've updated the `ui_focus_system` to accomplish this in a minimally intrusive way, and updated the docs to match.
**NOTE:** I have not added automated tests to verify this behavior, as we do not currently have a good testing paradigm for `bevy_ui`. I'm not thrilled with that by any means, but I'm not sure fixing it is within scope.
## Paths not taken
### Separate `Disabled` component
This is a much larger and more controversial change, and not well-scoped to UI.
Furthermore, it is extremely rare that you want hidden UI elements to function: the most common cases are for things like changing tabs, collapsing elements or so on.
Splitting this behavior would be more complex, and substantially violate user expectations.
### A separate limbo world
Mentioned in the linked issue. Super cool, but all of the problems of the `Disabled` component solution with a whole new RFC-worth of complexity.
### Using change detection to reduce the amount of redundant work
Adds a lot of complexity for questionable performance gains. Likely involves a complete refactor of the entire system.
We simply don't have the tests or benchmarks here to justify this.
## Changelog
- UI nodes are now always in an `Interaction::None` state while they are hidden (via the `ComputedVisibility` component).
2022-07-20 21:26:47 +00:00
|
|
|
///
|
|
|
|
/// If this is set to [`Display::None`], this node will be collapsed.
|
2020-07-26 19:27:09 +00:00
|
|
|
pub display: Display,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Whether to arrange this node relative to other nodes, or positioned absolutely
|
2020-07-26 19:27:09 +00:00
|
|
|
pub position_type: PositionType,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Which direction the content of this node should go
|
2020-07-26 19:27:09 +00:00
|
|
|
pub direction: Direction,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Whether to use column or row layout
|
2020-07-26 19:27:09 +00:00
|
|
|
pub flex_direction: FlexDirection,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// How to wrap nodes
|
2020-07-26 19:27:09 +00:00
|
|
|
pub flex_wrap: FlexWrap,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// How items are aligned according to the cross axis
|
2020-07-26 19:27:09 +00:00
|
|
|
pub align_items: AlignItems,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Like align_items but for only this item
|
2020-07-26 19:27:09 +00:00
|
|
|
pub align_self: AlignSelf,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// How to align each line, only applies if flex_wrap is set to
|
|
|
|
/// [`FlexWrap::Wrap`] and there are multiple lines of items
|
2020-07-26 19:27:09 +00:00
|
|
|
pub align_content: AlignContent,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// How items align according to the main axis
|
2020-07-26 19:27:09 +00:00
|
|
|
pub justify_content: JustifyContent,
|
2022-07-21 20:46:54 +00:00
|
|
|
/// The position of the node as described by its Rect
|
2022-08-01 16:27:16 +00:00
|
|
|
pub position: UiRect,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// The margin of the node
|
2022-08-01 16:27:16 +00:00
|
|
|
pub margin: UiRect,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// The padding of the node
|
2022-08-01 16:27:16 +00:00
|
|
|
pub padding: UiRect,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// The border of the node
|
2022-08-01 16:27:16 +00:00
|
|
|
pub border: UiRect,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Defines how much a flexbox item should grow if there's space available
|
2020-07-26 19:27:09 +00:00
|
|
|
pub flex_grow: f32,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// How to shrink if there's not enough space available
|
2020-07-26 19:27:09 +00:00
|
|
|
pub flex_shrink: f32,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// The initial size of the item
|
2020-07-26 19:27:09 +00:00
|
|
|
pub flex_basis: Val,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// The size of the flexbox
|
2022-08-01 16:27:16 +00:00
|
|
|
pub size: Size,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// The minimum size of the flexbox
|
2022-08-01 16:27:16 +00:00
|
|
|
pub min_size: Size,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// The maximum size of the flexbox
|
2022-08-01 16:27:16 +00:00
|
|
|
pub max_size: Size,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// The aspect ratio of the flexbox
|
2020-07-26 19:27:09 +00:00
|
|
|
pub aspect_ratio: Option<f32>,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// How to handle overflow
|
2021-12-19 05:44:28 +00:00
|
|
|
pub overflow: Overflow,
|
2022-12-21 02:15:53 +00:00
|
|
|
/// The size of the gutters between the rows and columns of the flexbox layout
|
|
|
|
///
|
|
|
|
/// Values of `Size::UNDEFINED` and `Size::AUTO` are treated as zero.
|
|
|
|
pub gap: Size,
|
2020-07-26 19:27:09 +00:00
|
|
|
}
|
|
|
|
|
2023-01-04 19:58:09 +00:00
|
|
|
impl Style {
|
|
|
|
pub const DEFAULT: Self = Self {
|
|
|
|
display: Display::DEFAULT,
|
|
|
|
position_type: PositionType::DEFAULT,
|
|
|
|
direction: Direction::DEFAULT,
|
|
|
|
flex_direction: FlexDirection::DEFAULT,
|
|
|
|
flex_wrap: FlexWrap::DEFAULT,
|
|
|
|
align_items: AlignItems::DEFAULT,
|
|
|
|
align_self: AlignSelf::DEFAULT,
|
|
|
|
align_content: AlignContent::DEFAULT,
|
|
|
|
justify_content: JustifyContent::DEFAULT,
|
|
|
|
position: UiRect::DEFAULT,
|
|
|
|
margin: UiRect::DEFAULT,
|
|
|
|
padding: UiRect::DEFAULT,
|
|
|
|
border: UiRect::DEFAULT,
|
|
|
|
flex_grow: 0.0,
|
|
|
|
flex_shrink: 1.0,
|
|
|
|
flex_basis: Val::Auto,
|
|
|
|
size: Size::AUTO,
|
|
|
|
min_size: Size::AUTO,
|
|
|
|
max_size: Size::AUTO,
|
|
|
|
aspect_ratio: None,
|
|
|
|
overflow: Overflow::DEFAULT,
|
|
|
|
gap: Size::UNDEFINED,
|
|
|
|
};
|
|
|
|
}
|
|
|
|
|
2020-07-26 19:27:09 +00:00
|
|
|
impl Default for Style {
|
|
|
|
fn default() -> Self {
|
2023-01-04 19:58:09 +00:00
|
|
|
Self::DEFAULT
|
2020-07-26 19:27:09 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-01-07 22:20:34 +00:00
|
|
|
/// How items are aligned according to the cross axis
|
2023-01-04 19:58:09 +00:00
|
|
|
#[derive(Copy, Clone, PartialEq, Eq, Debug, Serialize, Deserialize, Reflect)]
|
bevy_reflect: Update enum derives (#5473)
> In draft until #4761 is merged. See the relevant commits [here](https://github.com/bevyengine/bevy/pull/5473/commits/a85fe94a183fdd62d512b2613376ce6eace8ab63).
---
# Objective
Update enums across Bevy to use the new enum reflection and get rid of `#[reflect_value(...)]` usages.
## Solution
Find and replace all[^1] instances of `#[reflect_value(...)]` on enum types.
---
## Changelog
- Updated all[^1] reflected enums to implement `Enum` (i.e. they are no longer `ReflectRef::Value`)
## Migration Guide
Bevy-defined enums have been updated to implement `Enum` and are not considered value types (`ReflectRef::Value`) anymore. This means that their serialized representations will need to be updated. For example, given the Bevy enum:
```rust
pub enum ScalingMode {
None,
WindowSize,
Auto { min_width: f32, min_height: f32 },
FixedVertical(f32),
FixedHorizontal(f32),
}
```
You will need to update the serialized versions accordingly.
```js
// OLD FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"value": FixedHorizontal(720),
},
// NEW FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"enum": {
"variant": "FixedHorizontal",
"tuple": [
{
"type": "f32",
"value": 720,
},
],
},
},
```
This may also have other smaller implications (such as `Debug` representation), but serialization is probably the most prominent.
[^1]: All enums except `HandleId` as neither `Uuid` nor `AssetPathId` implement the reflection traits
2022-08-02 22:40:29 +00:00
|
|
|
#[reflect(PartialEq, Serialize, Deserialize)]
|
2020-07-26 19:27:09 +00:00
|
|
|
pub enum AlignItems {
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Items are aligned at the start
|
2020-07-26 19:27:09 +00:00
|
|
|
FlexStart,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Items are aligned at the end
|
2020-07-26 19:27:09 +00:00
|
|
|
FlexEnd,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Items are aligned at the center
|
2020-07-26 19:27:09 +00:00
|
|
|
Center,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Items are aligned at the baseline
|
2020-07-26 19:27:09 +00:00
|
|
|
Baseline,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Items are stretched across the whole cross axis
|
2020-07-26 19:27:09 +00:00
|
|
|
Stretch,
|
|
|
|
}
|
|
|
|
|
2023-01-04 19:58:09 +00:00
|
|
|
impl AlignItems {
|
|
|
|
pub const DEFAULT: Self = Self::Stretch;
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Default for AlignItems {
|
|
|
|
fn default() -> Self {
|
|
|
|
Self::DEFAULT
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Works like [`AlignItems`] but applies only to a single item
|
2023-01-04 19:58:09 +00:00
|
|
|
#[derive(Copy, Clone, PartialEq, Eq, Debug, Serialize, Deserialize, Reflect)]
|
bevy_reflect: Update enum derives (#5473)
> In draft until #4761 is merged. See the relevant commits [here](https://github.com/bevyengine/bevy/pull/5473/commits/a85fe94a183fdd62d512b2613376ce6eace8ab63).
---
# Objective
Update enums across Bevy to use the new enum reflection and get rid of `#[reflect_value(...)]` usages.
## Solution
Find and replace all[^1] instances of `#[reflect_value(...)]` on enum types.
---
## Changelog
- Updated all[^1] reflected enums to implement `Enum` (i.e. they are no longer `ReflectRef::Value`)
## Migration Guide
Bevy-defined enums have been updated to implement `Enum` and are not considered value types (`ReflectRef::Value`) anymore. This means that their serialized representations will need to be updated. For example, given the Bevy enum:
```rust
pub enum ScalingMode {
None,
WindowSize,
Auto { min_width: f32, min_height: f32 },
FixedVertical(f32),
FixedHorizontal(f32),
}
```
You will need to update the serialized versions accordingly.
```js
// OLD FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"value": FixedHorizontal(720),
},
// NEW FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"enum": {
"variant": "FixedHorizontal",
"tuple": [
{
"type": "f32",
"value": 720,
},
],
},
},
```
This may also have other smaller implications (such as `Debug` representation), but serialization is probably the most prominent.
[^1]: All enums except `HandleId` as neither `Uuid` nor `AssetPathId` implement the reflection traits
2022-08-02 22:40:29 +00:00
|
|
|
#[reflect(PartialEq, Serialize, Deserialize)]
|
2020-07-26 19:27:09 +00:00
|
|
|
pub enum AlignSelf {
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Use the value of [`AlignItems`]
|
2020-07-26 19:27:09 +00:00
|
|
|
Auto,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// If the parent has [`AlignItems::Center`] only this item will be at the start
|
2020-07-26 19:27:09 +00:00
|
|
|
FlexStart,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// If the parent has [`AlignItems::Center`] only this item will be at the end
|
2020-07-26 19:27:09 +00:00
|
|
|
FlexEnd,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// If the parent has [`AlignItems::FlexStart`] only this item will be at the center
|
2020-07-26 19:27:09 +00:00
|
|
|
Center,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// If the parent has [`AlignItems::Center`] only this item will be at the baseline
|
2020-07-26 19:27:09 +00:00
|
|
|
Baseline,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// If the parent has [`AlignItems::Center`] only this item will stretch along the whole cross axis
|
2020-07-26 19:27:09 +00:00
|
|
|
Stretch,
|
|
|
|
}
|
|
|
|
|
2023-01-04 19:58:09 +00:00
|
|
|
impl AlignSelf {
|
|
|
|
pub const DEFAULT: Self = Self::Auto;
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Default for AlignSelf {
|
|
|
|
fn default() -> Self {
|
|
|
|
Self::DEFAULT
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Defines how each line is aligned within the flexbox.
|
|
|
|
///
|
|
|
|
/// It only applies if [`FlexWrap::Wrap`] is present and if there are multiple lines of items.
|
2023-01-04 19:58:09 +00:00
|
|
|
#[derive(Copy, Clone, PartialEq, Eq, Debug, Serialize, Deserialize, Reflect)]
|
bevy_reflect: Update enum derives (#5473)
> In draft until #4761 is merged. See the relevant commits [here](https://github.com/bevyengine/bevy/pull/5473/commits/a85fe94a183fdd62d512b2613376ce6eace8ab63).
---
# Objective
Update enums across Bevy to use the new enum reflection and get rid of `#[reflect_value(...)]` usages.
## Solution
Find and replace all[^1] instances of `#[reflect_value(...)]` on enum types.
---
## Changelog
- Updated all[^1] reflected enums to implement `Enum` (i.e. they are no longer `ReflectRef::Value`)
## Migration Guide
Bevy-defined enums have been updated to implement `Enum` and are not considered value types (`ReflectRef::Value`) anymore. This means that their serialized representations will need to be updated. For example, given the Bevy enum:
```rust
pub enum ScalingMode {
None,
WindowSize,
Auto { min_width: f32, min_height: f32 },
FixedVertical(f32),
FixedHorizontal(f32),
}
```
You will need to update the serialized versions accordingly.
```js
// OLD FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"value": FixedHorizontal(720),
},
// NEW FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"enum": {
"variant": "FixedHorizontal",
"tuple": [
{
"type": "f32",
"value": 720,
},
],
},
},
```
This may also have other smaller implications (such as `Debug` representation), but serialization is probably the most prominent.
[^1]: All enums except `HandleId` as neither `Uuid` nor `AssetPathId` implement the reflection traits
2022-08-02 22:40:29 +00:00
|
|
|
#[reflect(PartialEq, Serialize, Deserialize)]
|
2020-07-26 19:27:09 +00:00
|
|
|
pub enum AlignContent {
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Each line moves towards the start of the cross axis
|
2020-07-26 19:27:09 +00:00
|
|
|
FlexStart,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Each line moves towards the end of the cross axis
|
2020-07-26 19:27:09 +00:00
|
|
|
FlexEnd,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Each line moves towards the center of the cross axis
|
2020-07-26 19:27:09 +00:00
|
|
|
Center,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Each line will stretch to fill the remaining space
|
2020-07-26 19:27:09 +00:00
|
|
|
Stretch,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Each line fills the space it needs, putting the remaining space, if any
|
|
|
|
/// inbetween the lines
|
2020-07-26 19:27:09 +00:00
|
|
|
SpaceBetween,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Each line fills the space it needs, putting the remaining space, if any
|
|
|
|
/// around the lines
|
2020-07-26 19:27:09 +00:00
|
|
|
SpaceAround,
|
|
|
|
}
|
|
|
|
|
2023-01-04 19:58:09 +00:00
|
|
|
impl AlignContent {
|
|
|
|
pub const DEFAULT: Self = Self::Stretch;
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Default for AlignContent {
|
|
|
|
fn default() -> Self {
|
|
|
|
Self::DEFAULT
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Defines the text direction
|
|
|
|
///
|
|
|
|
/// For example English is written LTR (left-to-right) while Arabic is written RTL (right-to-left).
|
2023-01-04 19:58:09 +00:00
|
|
|
#[derive(Copy, Clone, PartialEq, Eq, Debug, Serialize, Deserialize, Reflect)]
|
bevy_reflect: Update enum derives (#5473)
> In draft until #4761 is merged. See the relevant commits [here](https://github.com/bevyengine/bevy/pull/5473/commits/a85fe94a183fdd62d512b2613376ce6eace8ab63).
---
# Objective
Update enums across Bevy to use the new enum reflection and get rid of `#[reflect_value(...)]` usages.
## Solution
Find and replace all[^1] instances of `#[reflect_value(...)]` on enum types.
---
## Changelog
- Updated all[^1] reflected enums to implement `Enum` (i.e. they are no longer `ReflectRef::Value`)
## Migration Guide
Bevy-defined enums have been updated to implement `Enum` and are not considered value types (`ReflectRef::Value`) anymore. This means that their serialized representations will need to be updated. For example, given the Bevy enum:
```rust
pub enum ScalingMode {
None,
WindowSize,
Auto { min_width: f32, min_height: f32 },
FixedVertical(f32),
FixedHorizontal(f32),
}
```
You will need to update the serialized versions accordingly.
```js
// OLD FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"value": FixedHorizontal(720),
},
// NEW FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"enum": {
"variant": "FixedHorizontal",
"tuple": [
{
"type": "f32",
"value": 720,
},
],
},
},
```
This may also have other smaller implications (such as `Debug` representation), but serialization is probably the most prominent.
[^1]: All enums except `HandleId` as neither `Uuid` nor `AssetPathId` implement the reflection traits
2022-08-02 22:40:29 +00:00
|
|
|
#[reflect(PartialEq, Serialize, Deserialize)]
|
2020-07-26 19:27:09 +00:00
|
|
|
pub enum Direction {
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Inherit from parent node
|
2020-07-26 19:27:09 +00:00
|
|
|
Inherit,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Text is written left to right
|
2021-08-24 01:50:21 +00:00
|
|
|
LeftToRight,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Text is written right to left
|
2021-08-24 01:50:21 +00:00
|
|
|
RightToLeft,
|
2020-07-26 19:27:09 +00:00
|
|
|
}
|
|
|
|
|
2023-01-04 19:58:09 +00:00
|
|
|
impl Direction {
|
|
|
|
pub const DEFAULT: Self = Self::Inherit;
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Default for Direction {
|
|
|
|
fn default() -> Self {
|
|
|
|
Self::DEFAULT
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
Disable UI node `Interaction` when `ComputedVisibility` is false (#5361)
# Objective
UI nodes can be hidden by setting their `Visibility` property. Since #5310 was merged, this is now ergonomic to use, as visibility is now inherited.
However, UI nodes still receive (and store) interactions when hidden, resulting in surprising hidden state (and an inability to otherwise disable UI nodes.
## Solution
Fixes #5360.
I've updated the `ui_focus_system` to accomplish this in a minimally intrusive way, and updated the docs to match.
**NOTE:** I have not added automated tests to verify this behavior, as we do not currently have a good testing paradigm for `bevy_ui`. I'm not thrilled with that by any means, but I'm not sure fixing it is within scope.
## Paths not taken
### Separate `Disabled` component
This is a much larger and more controversial change, and not well-scoped to UI.
Furthermore, it is extremely rare that you want hidden UI elements to function: the most common cases are for things like changing tabs, collapsing elements or so on.
Splitting this behavior would be more complex, and substantially violate user expectations.
### A separate limbo world
Mentioned in the linked issue. Super cool, but all of the problems of the `Disabled` component solution with a whole new RFC-worth of complexity.
### Using change detection to reduce the amount of redundant work
Adds a lot of complexity for questionable performance gains. Likely involves a complete refactor of the entire system.
We simply don't have the tests or benchmarks here to justify this.
## Changelog
- UI nodes are now always in an `Interaction::None` state while they are hidden (via the `ComputedVisibility` component).
2022-07-20 21:26:47 +00:00
|
|
|
/// Whether to use a Flexbox layout model.
|
|
|
|
///
|
|
|
|
/// Part of the [`Style`] component.
|
2023-01-04 19:58:09 +00:00
|
|
|
#[derive(Copy, Clone, PartialEq, Eq, Debug, Serialize, Deserialize, Reflect)]
|
bevy_reflect: Update enum derives (#5473)
> In draft until #4761 is merged. See the relevant commits [here](https://github.com/bevyengine/bevy/pull/5473/commits/a85fe94a183fdd62d512b2613376ce6eace8ab63).
---
# Objective
Update enums across Bevy to use the new enum reflection and get rid of `#[reflect_value(...)]` usages.
## Solution
Find and replace all[^1] instances of `#[reflect_value(...)]` on enum types.
---
## Changelog
- Updated all[^1] reflected enums to implement `Enum` (i.e. they are no longer `ReflectRef::Value`)
## Migration Guide
Bevy-defined enums have been updated to implement `Enum` and are not considered value types (`ReflectRef::Value`) anymore. This means that their serialized representations will need to be updated. For example, given the Bevy enum:
```rust
pub enum ScalingMode {
None,
WindowSize,
Auto { min_width: f32, min_height: f32 },
FixedVertical(f32),
FixedHorizontal(f32),
}
```
You will need to update the serialized versions accordingly.
```js
// OLD FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"value": FixedHorizontal(720),
},
// NEW FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"enum": {
"variant": "FixedHorizontal",
"tuple": [
{
"type": "f32",
"value": 720,
},
],
},
},
```
This may also have other smaller implications (such as `Debug` representation), but serialization is probably the most prominent.
[^1]: All enums except `HandleId` as neither `Uuid` nor `AssetPathId` implement the reflection traits
2022-08-02 22:40:29 +00:00
|
|
|
#[reflect(PartialEq, Serialize, Deserialize)]
|
2020-07-26 19:27:09 +00:00
|
|
|
pub enum Display {
|
Disable UI node `Interaction` when `ComputedVisibility` is false (#5361)
# Objective
UI nodes can be hidden by setting their `Visibility` property. Since #5310 was merged, this is now ergonomic to use, as visibility is now inherited.
However, UI nodes still receive (and store) interactions when hidden, resulting in surprising hidden state (and an inability to otherwise disable UI nodes.
## Solution
Fixes #5360.
I've updated the `ui_focus_system` to accomplish this in a minimally intrusive way, and updated the docs to match.
**NOTE:** I have not added automated tests to verify this behavior, as we do not currently have a good testing paradigm for `bevy_ui`. I'm not thrilled with that by any means, but I'm not sure fixing it is within scope.
## Paths not taken
### Separate `Disabled` component
This is a much larger and more controversial change, and not well-scoped to UI.
Furthermore, it is extremely rare that you want hidden UI elements to function: the most common cases are for things like changing tabs, collapsing elements or so on.
Splitting this behavior would be more complex, and substantially violate user expectations.
### A separate limbo world
Mentioned in the linked issue. Super cool, but all of the problems of the `Disabled` component solution with a whole new RFC-worth of complexity.
### Using change detection to reduce the amount of redundant work
Adds a lot of complexity for questionable performance gains. Likely involves a complete refactor of the entire system.
We simply don't have the tests or benchmarks here to justify this.
## Changelog
- UI nodes are now always in an `Interaction::None` state while they are hidden (via the `ComputedVisibility` component).
2022-07-20 21:26:47 +00:00
|
|
|
/// Use Flexbox layout model to determine the position of this [`Node`].
|
2020-07-26 19:27:09 +00:00
|
|
|
Flex,
|
Disable UI node `Interaction` when `ComputedVisibility` is false (#5361)
# Objective
UI nodes can be hidden by setting their `Visibility` property. Since #5310 was merged, this is now ergonomic to use, as visibility is now inherited.
However, UI nodes still receive (and store) interactions when hidden, resulting in surprising hidden state (and an inability to otherwise disable UI nodes.
## Solution
Fixes #5360.
I've updated the `ui_focus_system` to accomplish this in a minimally intrusive way, and updated the docs to match.
**NOTE:** I have not added automated tests to verify this behavior, as we do not currently have a good testing paradigm for `bevy_ui`. I'm not thrilled with that by any means, but I'm not sure fixing it is within scope.
## Paths not taken
### Separate `Disabled` component
This is a much larger and more controversial change, and not well-scoped to UI.
Furthermore, it is extremely rare that you want hidden UI elements to function: the most common cases are for things like changing tabs, collapsing elements or so on.
Splitting this behavior would be more complex, and substantially violate user expectations.
### A separate limbo world
Mentioned in the linked issue. Super cool, but all of the problems of the `Disabled` component solution with a whole new RFC-worth of complexity.
### Using change detection to reduce the amount of redundant work
Adds a lot of complexity for questionable performance gains. Likely involves a complete refactor of the entire system.
We simply don't have the tests or benchmarks here to justify this.
## Changelog
- UI nodes are now always in an `Interaction::None` state while they are hidden (via the `ComputedVisibility` component).
2022-07-20 21:26:47 +00:00
|
|
|
/// Use no layout, don't render this node and its children.
|
|
|
|
///
|
|
|
|
/// If you want to hide a node and its children,
|
|
|
|
/// but keep its layout in place, set its [`Visibility`](bevy_render::view::Visibility) component instead.
|
2020-07-26 19:27:09 +00:00
|
|
|
None,
|
|
|
|
}
|
|
|
|
|
2023-01-04 19:58:09 +00:00
|
|
|
impl Display {
|
|
|
|
pub const DEFAULT: Self = Self::Flex;
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Default for Display {
|
|
|
|
fn default() -> Self {
|
|
|
|
Self::DEFAULT
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Defines how flexbox items are ordered within a flexbox
|
2023-01-04 19:58:09 +00:00
|
|
|
#[derive(Copy, Clone, PartialEq, Eq, Debug, Serialize, Deserialize, Reflect)]
|
bevy_reflect: Update enum derives (#5473)
> In draft until #4761 is merged. See the relevant commits [here](https://github.com/bevyengine/bevy/pull/5473/commits/a85fe94a183fdd62d512b2613376ce6eace8ab63).
---
# Objective
Update enums across Bevy to use the new enum reflection and get rid of `#[reflect_value(...)]` usages.
## Solution
Find and replace all[^1] instances of `#[reflect_value(...)]` on enum types.
---
## Changelog
- Updated all[^1] reflected enums to implement `Enum` (i.e. they are no longer `ReflectRef::Value`)
## Migration Guide
Bevy-defined enums have been updated to implement `Enum` and are not considered value types (`ReflectRef::Value`) anymore. This means that their serialized representations will need to be updated. For example, given the Bevy enum:
```rust
pub enum ScalingMode {
None,
WindowSize,
Auto { min_width: f32, min_height: f32 },
FixedVertical(f32),
FixedHorizontal(f32),
}
```
You will need to update the serialized versions accordingly.
```js
// OLD FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"value": FixedHorizontal(720),
},
// NEW FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"enum": {
"variant": "FixedHorizontal",
"tuple": [
{
"type": "f32",
"value": 720,
},
],
},
},
```
This may also have other smaller implications (such as `Debug` representation), but serialization is probably the most prominent.
[^1]: All enums except `HandleId` as neither `Uuid` nor `AssetPathId` implement the reflection traits
2022-08-02 22:40:29 +00:00
|
|
|
#[reflect(PartialEq, Serialize, Deserialize)]
|
2020-07-26 19:27:09 +00:00
|
|
|
pub enum FlexDirection {
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Same way as text direction along the main axis
|
2020-07-26 19:27:09 +00:00
|
|
|
Row,
|
2022-10-11 12:51:44 +00:00
|
|
|
/// Flex from top to bottom
|
2020-07-26 19:27:09 +00:00
|
|
|
Column,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Opposite way as text direction along the main axis
|
2020-07-26 19:27:09 +00:00
|
|
|
RowReverse,
|
2022-10-11 12:51:44 +00:00
|
|
|
/// Flex from bottom to top
|
2020-07-26 19:27:09 +00:00
|
|
|
ColumnReverse,
|
|
|
|
}
|
|
|
|
|
2023-01-04 19:58:09 +00:00
|
|
|
impl FlexDirection {
|
|
|
|
pub const DEFAULT: Self = Self::Row;
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Default for FlexDirection {
|
|
|
|
fn default() -> Self {
|
|
|
|
Self::DEFAULT
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Defines how items are aligned according to the main axis
|
2023-01-04 19:58:09 +00:00
|
|
|
#[derive(Copy, Clone, PartialEq, Eq, Debug, Serialize, Deserialize, Reflect)]
|
bevy_reflect: Update enum derives (#5473)
> In draft until #4761 is merged. See the relevant commits [here](https://github.com/bevyengine/bevy/pull/5473/commits/a85fe94a183fdd62d512b2613376ce6eace8ab63).
---
# Objective
Update enums across Bevy to use the new enum reflection and get rid of `#[reflect_value(...)]` usages.
## Solution
Find and replace all[^1] instances of `#[reflect_value(...)]` on enum types.
---
## Changelog
- Updated all[^1] reflected enums to implement `Enum` (i.e. they are no longer `ReflectRef::Value`)
## Migration Guide
Bevy-defined enums have been updated to implement `Enum` and are not considered value types (`ReflectRef::Value`) anymore. This means that their serialized representations will need to be updated. For example, given the Bevy enum:
```rust
pub enum ScalingMode {
None,
WindowSize,
Auto { min_width: f32, min_height: f32 },
FixedVertical(f32),
FixedHorizontal(f32),
}
```
You will need to update the serialized versions accordingly.
```js
// OLD FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"value": FixedHorizontal(720),
},
// NEW FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"enum": {
"variant": "FixedHorizontal",
"tuple": [
{
"type": "f32",
"value": 720,
},
],
},
},
```
This may also have other smaller implications (such as `Debug` representation), but serialization is probably the most prominent.
[^1]: All enums except `HandleId` as neither `Uuid` nor `AssetPathId` implement the reflection traits
2022-08-02 22:40:29 +00:00
|
|
|
#[reflect(PartialEq, Serialize, Deserialize)]
|
2020-07-26 19:27:09 +00:00
|
|
|
pub enum JustifyContent {
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Pushed towards the start
|
2020-07-26 19:27:09 +00:00
|
|
|
FlexStart,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Pushed towards the end
|
2020-07-26 19:27:09 +00:00
|
|
|
FlexEnd,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Centered along the main axis
|
2020-07-26 19:27:09 +00:00
|
|
|
Center,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Remaining space is distributed between the items
|
2020-07-26 19:27:09 +00:00
|
|
|
SpaceBetween,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Remaining space is distributed around the items
|
2020-07-26 19:27:09 +00:00
|
|
|
SpaceAround,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Like [`JustifyContent::SpaceAround`] but with even spacing between items
|
2020-07-26 19:27:09 +00:00
|
|
|
SpaceEvenly,
|
|
|
|
}
|
|
|
|
|
2023-01-04 19:58:09 +00:00
|
|
|
impl JustifyContent {
|
|
|
|
pub const DEFAULT: Self = Self::FlexStart;
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Default for JustifyContent {
|
|
|
|
fn default() -> Self {
|
|
|
|
Self::DEFAULT
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Whether to show or hide overflowing items
|
2023-01-04 19:58:09 +00:00
|
|
|
#[derive(Copy, Clone, PartialEq, Eq, Debug, Reflect, Serialize, Deserialize)]
|
bevy_reflect: Update enum derives (#5473)
> In draft until #4761 is merged. See the relevant commits [here](https://github.com/bevyengine/bevy/pull/5473/commits/a85fe94a183fdd62d512b2613376ce6eace8ab63).
---
# Objective
Update enums across Bevy to use the new enum reflection and get rid of `#[reflect_value(...)]` usages.
## Solution
Find and replace all[^1] instances of `#[reflect_value(...)]` on enum types.
---
## Changelog
- Updated all[^1] reflected enums to implement `Enum` (i.e. they are no longer `ReflectRef::Value`)
## Migration Guide
Bevy-defined enums have been updated to implement `Enum` and are not considered value types (`ReflectRef::Value`) anymore. This means that their serialized representations will need to be updated. For example, given the Bevy enum:
```rust
pub enum ScalingMode {
None,
WindowSize,
Auto { min_width: f32, min_height: f32 },
FixedVertical(f32),
FixedHorizontal(f32),
}
```
You will need to update the serialized versions accordingly.
```js
// OLD FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"value": FixedHorizontal(720),
},
// NEW FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"enum": {
"variant": "FixedHorizontal",
"tuple": [
{
"type": "f32",
"value": 720,
},
],
},
},
```
This may also have other smaller implications (such as `Debug` representation), but serialization is probably the most prominent.
[^1]: All enums except `HandleId` as neither `Uuid` nor `AssetPathId` implement the reflection traits
2022-08-02 22:40:29 +00:00
|
|
|
#[reflect(PartialEq, Serialize, Deserialize)]
|
2021-12-19 05:44:28 +00:00
|
|
|
pub enum Overflow {
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Show overflowing items
|
2021-12-19 05:44:28 +00:00
|
|
|
Visible,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Hide overflowing items
|
2021-12-19 05:44:28 +00:00
|
|
|
Hidden,
|
|
|
|
}
|
2020-07-26 19:27:09 +00:00
|
|
|
|
2023-01-04 19:58:09 +00:00
|
|
|
impl Overflow {
|
|
|
|
pub const DEFAULT: Self = Self::Visible;
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Default for Overflow {
|
|
|
|
fn default() -> Self {
|
|
|
|
Self::DEFAULT
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-01-07 22:20:34 +00:00
|
|
|
/// The strategy used to position this node
|
2023-01-04 19:58:09 +00:00
|
|
|
#[derive(Copy, Clone, PartialEq, Eq, Debug, Serialize, Deserialize, Reflect)]
|
bevy_reflect: Update enum derives (#5473)
> In draft until #4761 is merged. See the relevant commits [here](https://github.com/bevyengine/bevy/pull/5473/commits/a85fe94a183fdd62d512b2613376ce6eace8ab63).
---
# Objective
Update enums across Bevy to use the new enum reflection and get rid of `#[reflect_value(...)]` usages.
## Solution
Find and replace all[^1] instances of `#[reflect_value(...)]` on enum types.
---
## Changelog
- Updated all[^1] reflected enums to implement `Enum` (i.e. they are no longer `ReflectRef::Value`)
## Migration Guide
Bevy-defined enums have been updated to implement `Enum` and are not considered value types (`ReflectRef::Value`) anymore. This means that their serialized representations will need to be updated. For example, given the Bevy enum:
```rust
pub enum ScalingMode {
None,
WindowSize,
Auto { min_width: f32, min_height: f32 },
FixedVertical(f32),
FixedHorizontal(f32),
}
```
You will need to update the serialized versions accordingly.
```js
// OLD FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"value": FixedHorizontal(720),
},
// NEW FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"enum": {
"variant": "FixedHorizontal",
"tuple": [
{
"type": "f32",
"value": 720,
},
],
},
},
```
This may also have other smaller implications (such as `Debug` representation), but serialization is probably the most prominent.
[^1]: All enums except `HandleId` as neither `Uuid` nor `AssetPathId` implement the reflection traits
2022-08-02 22:40:29 +00:00
|
|
|
#[reflect(PartialEq, Serialize, Deserialize)]
|
2020-07-26 19:27:09 +00:00
|
|
|
pub enum PositionType {
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Relative to all other nodes with the [`PositionType::Relative`] value
|
2020-07-26 19:27:09 +00:00
|
|
|
Relative,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Independent of all other nodes
|
|
|
|
///
|
|
|
|
/// As usual, the `Style.position` field of this node is specified relative to its parent node
|
2020-07-26 19:27:09 +00:00
|
|
|
Absolute,
|
|
|
|
}
|
|
|
|
|
2023-01-04 19:58:09 +00:00
|
|
|
impl PositionType {
|
|
|
|
const DEFAULT: Self = Self::Relative;
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Default for PositionType {
|
|
|
|
fn default() -> Self {
|
|
|
|
Self::DEFAULT
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Defines if flexbox items appear on a single line or on multiple lines
|
2023-01-04 19:58:09 +00:00
|
|
|
#[derive(Copy, Clone, PartialEq, Eq, Debug, Serialize, Deserialize, Reflect)]
|
bevy_reflect: Update enum derives (#5473)
> In draft until #4761 is merged. See the relevant commits [here](https://github.com/bevyengine/bevy/pull/5473/commits/a85fe94a183fdd62d512b2613376ce6eace8ab63).
---
# Objective
Update enums across Bevy to use the new enum reflection and get rid of `#[reflect_value(...)]` usages.
## Solution
Find and replace all[^1] instances of `#[reflect_value(...)]` on enum types.
---
## Changelog
- Updated all[^1] reflected enums to implement `Enum` (i.e. they are no longer `ReflectRef::Value`)
## Migration Guide
Bevy-defined enums have been updated to implement `Enum` and are not considered value types (`ReflectRef::Value`) anymore. This means that their serialized representations will need to be updated. For example, given the Bevy enum:
```rust
pub enum ScalingMode {
None,
WindowSize,
Auto { min_width: f32, min_height: f32 },
FixedVertical(f32),
FixedHorizontal(f32),
}
```
You will need to update the serialized versions accordingly.
```js
// OLD FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"value": FixedHorizontal(720),
},
// NEW FORMAT
{
"type": "bevy_render::camera::projection::ScalingMode",
"enum": {
"variant": "FixedHorizontal",
"tuple": [
{
"type": "f32",
"value": 720,
},
],
},
},
```
This may also have other smaller implications (such as `Debug` representation), but serialization is probably the most prominent.
[^1]: All enums except `HandleId` as neither `Uuid` nor `AssetPathId` implement the reflection traits
2022-08-02 22:40:29 +00:00
|
|
|
#[reflect(PartialEq, Serialize, Deserialize)]
|
2020-07-26 19:27:09 +00:00
|
|
|
pub enum FlexWrap {
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Single line, will overflow if needed
|
2020-07-26 19:27:09 +00:00
|
|
|
NoWrap,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Multiple lines, if needed
|
2020-07-26 19:27:09 +00:00
|
|
|
Wrap,
|
2022-01-07 22:20:34 +00:00
|
|
|
/// Same as [`FlexWrap::Wrap`] but new lines will appear before the previous one
|
2020-07-26 19:27:09 +00:00
|
|
|
WrapReverse,
|
|
|
|
}
|
|
|
|
|
2023-01-04 19:58:09 +00:00
|
|
|
impl FlexWrap {
|
|
|
|
const DEFAULT: Self = Self::NoWrap;
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Default for FlexWrap {
|
|
|
|
fn default() -> Self {
|
|
|
|
Self::DEFAULT
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-01-07 22:20:34 +00:00
|
|
|
/// The calculated size of the node
|
2023-01-04 19:58:09 +00:00
|
|
|
#[derive(Component, Copy, Clone, Debug, Reflect)]
|
2021-12-14 03:58:23 +00:00
|
|
|
#[reflect(Component)]
|
2021-03-10 22:37:02 +00:00
|
|
|
pub struct CalculatedSize {
|
2022-01-07 22:20:34 +00:00
|
|
|
/// The size of the node
|
2021-03-10 22:37:02 +00:00
|
|
|
pub size: Size,
|
2022-12-20 16:44:12 +00:00
|
|
|
/// Whether to attempt to preserve the aspect ratio when determing the layout for this item
|
|
|
|
pub preserve_aspect_ratio: bool,
|
2021-03-10 22:37:02 +00:00
|
|
|
}
|
2021-12-14 03:58:23 +00:00
|
|
|
|
2023-01-04 19:58:09 +00:00
|
|
|
impl CalculatedSize {
|
|
|
|
const DEFAULT: Self = Self {
|
|
|
|
size: Size::DEFAULT,
|
|
|
|
preserve_aspect_ratio: false,
|
|
|
|
};
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Default for CalculatedSize {
|
|
|
|
fn default() -> Self {
|
|
|
|
Self::DEFAULT
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-09-25 00:39:17 +00:00
|
|
|
/// The background color of the node
|
|
|
|
///
|
|
|
|
/// This serves as the "fill" color.
|
|
|
|
/// When combined with [`UiImage`], tints the provided texture.
|
2023-01-04 19:58:09 +00:00
|
|
|
#[derive(Component, Copy, Clone, Debug, Reflect)]
|
2022-05-03 19:20:13 +00:00
|
|
|
#[reflect(Component, Default)]
|
2022-09-25 00:39:17 +00:00
|
|
|
pub struct BackgroundColor(pub Color);
|
2021-12-14 03:58:23 +00:00
|
|
|
|
2023-01-04 19:58:09 +00:00
|
|
|
impl BackgroundColor {
|
|
|
|
pub const DEFAULT: Self = Self(Color::WHITE);
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Default for BackgroundColor {
|
|
|
|
fn default() -> Self {
|
|
|
|
Self::DEFAULT
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-09-25 00:39:17 +00:00
|
|
|
impl From<Color> for BackgroundColor {
|
2021-12-14 03:58:23 +00:00
|
|
|
fn from(color: Color) -> Self {
|
|
|
|
Self(color)
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-09-25 00:39:17 +00:00
|
|
|
/// The 2D texture displayed for this UI node
|
2022-11-14 21:59:17 +00:00
|
|
|
#[derive(Component, Clone, Debug, Reflect)]
|
2022-05-03 19:20:13 +00:00
|
|
|
#[reflect(Component, Default)]
|
2022-11-14 21:59:17 +00:00
|
|
|
pub struct UiImage {
|
|
|
|
/// Handle to the texture
|
|
|
|
pub texture: Handle<Image>,
|
|
|
|
/// Whether the image should be flipped along its x-axis
|
|
|
|
pub flip_x: bool,
|
|
|
|
/// Whether the image should be flipped along its y-axis
|
|
|
|
pub flip_y: bool,
|
|
|
|
}
|
2021-12-14 03:58:23 +00:00
|
|
|
|
|
|
|
impl Default for UiImage {
|
2022-11-14 21:59:17 +00:00
|
|
|
fn default() -> UiImage {
|
|
|
|
UiImage {
|
|
|
|
texture: DEFAULT_IMAGE_HANDLE.typed(),
|
|
|
|
flip_x: false,
|
|
|
|
flip_y: false,
|
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
impl UiImage {
|
|
|
|
pub fn new(texture: Handle<Image>) -> Self {
|
|
|
|
Self {
|
|
|
|
texture,
|
|
|
|
..Default::default()
|
|
|
|
}
|
2021-12-14 03:58:23 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
impl From<Handle<Image>> for UiImage {
|
2022-11-14 21:59:17 +00:00
|
|
|
fn from(texture: Handle<Image>) -> Self {
|
|
|
|
Self::new(texture)
|
2021-12-14 03:58:23 +00:00
|
|
|
}
|
|
|
|
}
|
2021-12-19 05:44:28 +00:00
|
|
|
|
2022-01-07 22:20:34 +00:00
|
|
|
/// The calculated clip of the node
|
2021-12-19 05:44:28 +00:00
|
|
|
#[derive(Component, Default, Copy, Clone, Debug, Reflect)]
|
|
|
|
#[reflect(Component)]
|
|
|
|
pub struct CalculatedClip {
|
2022-01-07 22:20:34 +00:00
|
|
|
/// The rect of the clip
|
2022-09-02 12:35:23 +00:00
|
|
|
pub clip: Rect,
|
2021-12-19 05:44:28 +00:00
|
|
|
}
|
2022-10-24 14:33:46 +00:00
|
|
|
|
Add z-index support with a predictable UI stack (#5877)
# Objective
Add consistent UI rendering and interaction where deep nodes inside two different hierarchies will never render on top of one-another by default and offer an escape hatch (z-index) for nodes to change their depth.
## The problem with current implementation
The current implementation of UI rendering is broken in that regard, mainly because [it sets the Z value of the `Transform` component based on a "global Z" space](https://github.com/bevyengine/bevy/blob/main/crates/bevy_ui/src/update.rs#L43) shared by all nodes in the UI. This doesn't account for the fact that each node's final `GlobalTransform` value will be relative to its parent. This effectively makes the depth unpredictable when two deep trees are rendered on top of one-another.
At the moment, it's also up to each part of the UI code to sort all of the UI nodes. The solution that's offered here does the full sorting of UI node entities once and offers the result through a resource so that all systems can use it.
## Solution
### New ZIndex component
This adds a new optional `ZIndex` enum component for nodes which offers two mechanism:
- `ZIndex::Local(i32)`: Overrides the depth of the node relative to its siblings.
- `ZIndex::Global(i32)`: Overrides the depth of the node relative to the UI root. This basically allows any node in the tree to "escape" the parent and be ordered relative to the entire UI.
Note that in the current implementation, omitting `ZIndex` on a node has the same result as adding `ZIndex::Local(0)`. Additionally, the "global" stacking context is essentially a way to add your node to the root stacking context, so using `ZIndex::Local(n)` on a root node (one without parent) will share that space with all nodes using `Index::Global(n)`.
### New UiStack resource
This adds a new `UiStack` resource which is calculated from both hierarchy and `ZIndex` during UI update and contains a vector of all node entities in the UI, ordered by depth (from farthest from camera to closest). This is exposed publicly by the bevy_ui crate with the hope that it can be used for consistent ordering and to reduce the amount of sorting that needs to be done by UI systems (i.e. instead of sorting everything by `global_transform.z` in every system, this array can be iterated over).
### New z_index example
This also adds a new z_index example that showcases the new `ZIndex` component. It's also a good general demo of the new UI stack system, because making this kind of UI was very broken with the old system (e.g. nodes would render on top of each other, not respecting hierarchy or insert order at all).
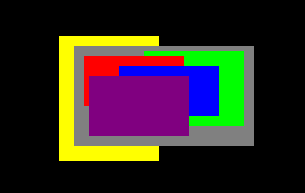
---
## Changelog
- Added the `ZIndex` component to bevy_ui.
- Added the `UiStack` resource to bevy_ui, and added implementation in a new `stack.rs` module.
- Removed the previous Z updating system from bevy_ui, because it was replaced with the above.
- Changed bevy_ui rendering to use UiStack instead of z ordering.
- Changed bevy_ui focus/interaction system to use UiStack instead of z ordering.
- Added a new z_index example.
## ZIndex demo
Here's a demo I wrote to test these features
https://user-images.githubusercontent.com/1060971/188329295-d7beebd6-9aee-43ab-821e-d437df5dbe8a.mp4
Co-authored-by: Carter Anderson <mcanders1@gmail.com>
2022-11-02 22:06:04 +00:00
|
|
|
/// Indicates that this [`Node`] entity's front-to-back ordering is not controlled solely
|
|
|
|
/// by its location in the UI hierarchy. A node with a higher z-index will appear on top
|
|
|
|
/// of other nodes with a lower z-index.
|
|
|
|
///
|
|
|
|
/// UI nodes that have the same z-index will appear according to the order in which they
|
|
|
|
/// appear in the UI hierarchy. In such a case, the last node to be added to its parent
|
|
|
|
/// will appear in front of this parent's other children.
|
|
|
|
///
|
|
|
|
/// Internally, nodes with a global z-index share the stacking context of root UI nodes
|
|
|
|
/// (nodes that have no parent). Because of this, there is no difference between using
|
|
|
|
/// [`ZIndex::Local(n)`] and [`ZIndex::Global(n)`] for root nodes.
|
|
|
|
///
|
|
|
|
/// Nodes without this component will be treated as if they had a value of [`ZIndex::Local(0)`].
|
|
|
|
#[derive(Component, Copy, Clone, Debug, Reflect)]
|
|
|
|
pub enum ZIndex {
|
|
|
|
/// Indicates the order in which this node should be rendered relative to its siblings.
|
|
|
|
Local(i32),
|
|
|
|
/// Indicates the order in which this node should be rendered relative to root nodes and
|
|
|
|
/// all other nodes that have a global z-index.
|
|
|
|
Global(i32),
|
|
|
|
}
|
|
|
|
|
|
|
|
impl Default for ZIndex {
|
|
|
|
fn default() -> Self {
|
|
|
|
Self::Local(0)
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-10-24 14:33:46 +00:00
|
|
|
#[cfg(test)]
|
|
|
|
mod tests {
|
|
|
|
use crate::ValArithmeticError;
|
|
|
|
|
|
|
|
use super::Val;
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn val_try_add() {
|
|
|
|
let undefined_sum = Val::Undefined.try_add(Val::Undefined).unwrap();
|
|
|
|
let auto_sum = Val::Auto.try_add(Val::Auto).unwrap();
|
|
|
|
let px_sum = Val::Px(20.).try_add(Val::Px(22.)).unwrap();
|
|
|
|
let percent_sum = Val::Percent(50.).try_add(Val::Percent(50.)).unwrap();
|
|
|
|
|
|
|
|
assert_eq!(undefined_sum, Val::Undefined);
|
|
|
|
assert_eq!(auto_sum, Val::Auto);
|
|
|
|
assert_eq!(px_sum, Val::Px(42.));
|
|
|
|
assert_eq!(percent_sum, Val::Percent(100.));
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn val_try_add_to_self() {
|
|
|
|
let mut val = Val::Px(5.);
|
|
|
|
|
|
|
|
val.try_add_assign(Val::Px(3.)).unwrap();
|
|
|
|
|
|
|
|
assert_eq!(val, Val::Px(8.));
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn val_try_sub() {
|
|
|
|
let undefined_sum = Val::Undefined.try_sub(Val::Undefined).unwrap();
|
|
|
|
let auto_sum = Val::Auto.try_sub(Val::Auto).unwrap();
|
|
|
|
let px_sum = Val::Px(72.).try_sub(Val::Px(30.)).unwrap();
|
|
|
|
let percent_sum = Val::Percent(100.).try_sub(Val::Percent(50.)).unwrap();
|
|
|
|
|
|
|
|
assert_eq!(undefined_sum, Val::Undefined);
|
|
|
|
assert_eq!(auto_sum, Val::Auto);
|
|
|
|
assert_eq!(px_sum, Val::Px(42.));
|
|
|
|
assert_eq!(percent_sum, Val::Percent(50.));
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn different_variant_val_try_add() {
|
|
|
|
let different_variant_sum_1 = Val::Undefined.try_add(Val::Auto);
|
|
|
|
let different_variant_sum_2 = Val::Px(50.).try_add(Val::Percent(50.));
|
|
|
|
let different_variant_sum_3 = Val::Percent(50.).try_add(Val::Undefined);
|
|
|
|
|
|
|
|
assert_eq!(
|
|
|
|
different_variant_sum_1,
|
|
|
|
Err(ValArithmeticError::NonIdenticalVariants)
|
|
|
|
);
|
|
|
|
assert_eq!(
|
|
|
|
different_variant_sum_2,
|
|
|
|
Err(ValArithmeticError::NonIdenticalVariants)
|
|
|
|
);
|
|
|
|
assert_eq!(
|
|
|
|
different_variant_sum_3,
|
|
|
|
Err(ValArithmeticError::NonIdenticalVariants)
|
|
|
|
);
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn different_variant_val_try_sub() {
|
|
|
|
let different_variant_diff_1 = Val::Undefined.try_sub(Val::Auto);
|
|
|
|
let different_variant_diff_2 = Val::Px(50.).try_sub(Val::Percent(50.));
|
|
|
|
let different_variant_diff_3 = Val::Percent(50.).try_sub(Val::Undefined);
|
|
|
|
|
|
|
|
assert_eq!(
|
|
|
|
different_variant_diff_1,
|
|
|
|
Err(ValArithmeticError::NonIdenticalVariants)
|
|
|
|
);
|
|
|
|
assert_eq!(
|
|
|
|
different_variant_diff_2,
|
|
|
|
Err(ValArithmeticError::NonIdenticalVariants)
|
|
|
|
);
|
|
|
|
assert_eq!(
|
|
|
|
different_variant_diff_3,
|
|
|
|
Err(ValArithmeticError::NonIdenticalVariants)
|
|
|
|
);
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn val_evaluate() {
|
|
|
|
let size = 250.;
|
|
|
|
let result = Val::Percent(80.).evaluate(size).unwrap();
|
|
|
|
|
|
|
|
assert_eq!(result, size * 0.8);
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn val_evaluate_px() {
|
|
|
|
let size = 250.;
|
|
|
|
let result = Val::Px(10.).evaluate(size).unwrap();
|
|
|
|
|
|
|
|
assert_eq!(result, 10.);
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn val_invalid_evaluation() {
|
|
|
|
let size = 250.;
|
|
|
|
let evaluate_undefined = Val::Undefined.evaluate(size);
|
|
|
|
let evaluate_auto = Val::Auto.evaluate(size);
|
|
|
|
|
|
|
|
assert_eq!(evaluate_undefined, Err(ValArithmeticError::NonEvaluateable));
|
|
|
|
assert_eq!(evaluate_auto, Err(ValArithmeticError::NonEvaluateable));
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn val_try_add_with_size() {
|
|
|
|
let size = 250.;
|
|
|
|
|
|
|
|
let px_sum = Val::Px(21.).try_add_with_size(Val::Px(21.), size).unwrap();
|
|
|
|
let percent_sum = Val::Percent(20.)
|
|
|
|
.try_add_with_size(Val::Percent(30.), size)
|
|
|
|
.unwrap();
|
|
|
|
let mixed_sum = Val::Px(20.)
|
|
|
|
.try_add_with_size(Val::Percent(30.), size)
|
|
|
|
.unwrap();
|
|
|
|
|
|
|
|
assert_eq!(px_sum, 42.);
|
|
|
|
assert_eq!(percent_sum, 0.5 * size);
|
|
|
|
assert_eq!(mixed_sum, 20. + 0.3 * size);
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn val_try_sub_with_size() {
|
|
|
|
let size = 250.;
|
|
|
|
|
|
|
|
let px_sum = Val::Px(60.).try_sub_with_size(Val::Px(18.), size).unwrap();
|
|
|
|
let percent_sum = Val::Percent(80.)
|
|
|
|
.try_sub_with_size(Val::Percent(30.), size)
|
|
|
|
.unwrap();
|
|
|
|
let mixed_sum = Val::Percent(50.)
|
|
|
|
.try_sub_with_size(Val::Px(30.), size)
|
|
|
|
.unwrap();
|
|
|
|
|
|
|
|
assert_eq!(px_sum, 42.);
|
|
|
|
assert_eq!(percent_sum, 0.5 * size);
|
|
|
|
assert_eq!(mixed_sum, 0.5 * size - 30.);
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn val_try_add_non_numeric_with_size() {
|
|
|
|
let size = 250.;
|
|
|
|
|
|
|
|
let undefined_sum = Val::Undefined.try_add_with_size(Val::Undefined, size);
|
|
|
|
let percent_sum = Val::Auto.try_add_with_size(Val::Auto, size);
|
|
|
|
|
|
|
|
assert_eq!(undefined_sum, Err(ValArithmeticError::NonEvaluateable));
|
|
|
|
assert_eq!(percent_sum, Err(ValArithmeticError::NonEvaluateable));
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn val_arithmetic_error_messages() {
|
|
|
|
assert_eq!(
|
|
|
|
format!("{}", ValArithmeticError::NonIdenticalVariants),
|
|
|
|
"the variants of the Vals don't match"
|
|
|
|
);
|
|
|
|
assert_eq!(
|
|
|
|
format!("{}", ValArithmeticError::NonEvaluateable),
|
|
|
|
"the given variant of Val is not evaluateable (non-numeric)"
|
|
|
|
);
|
|
|
|
}
|
|
|
|
}
|