2022-05-16 13:53:20 +00:00
|
|
|
//! Skinned mesh example with mesh and joints data loaded from a glTF file.
|
|
|
|
//! Example taken from <https://github.com/KhronosGroup/glTF-Tutorials/blob/master/gltfTutorial/gltfTutorial_019_SimpleSkin.md>
|
|
|
|
|
2022-10-22 18:52:29 +00:00
|
|
|
use std::f32::consts::*;
|
2022-03-29 18:31:13 +00:00
|
|
|
|
2024-04-01 19:59:08 +00:00
|
|
|
use bevy::{prelude::*, render::mesh::skinning::SkinnedMesh};
|
2022-03-29 18:31:13 +00:00
|
|
|
|
|
|
|
fn main() {
|
|
|
|
App::new()
|
|
|
|
.add_plugins(DefaultPlugins)
|
|
|
|
.insert_resource(AmbientLight {
|
Improve lighting in more examples (#12021)
# Objective
- #11868 changed the lighting system, forcing lights to increase their
intensity. The PR fixed most examples, but missed a few. These I later
caught in https://github.com/bevyengine/bevy-website/pull/1023.
- Related: #11982, #11981.
- While there, I noticed that the spotlight example could use a few easy
improvements.
## Solution
- Increase lighting in `skybox`, `spotlight`, `animated_transform`, and
`gltf_skinned_mesh`.
- Improve spotlight example.
- Make ground plane move with cubes, so they don't phase into each
other.
- Batch spawn cubes.
- Add controls text.
- Change controls to allow rotating around spotlights.
## Showcase
### Skybox
Before:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/8ba00d74-6d68-4414-97a8-28afb8305570">
After:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/ad15c471-6979-4dda-9889-9189136d8404">
### Spotlight
Before:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/53f966de-acf3-46b8-8299-0005c4cb8da0">
After:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/05c73c1e-0739-4226-83d6-e4249a9105e0">
### Animated Transform
Before:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/6d7d4ea0-e22e-42a5-9905-ea1731d474cf">
After:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/f1ee08d6-d17a-4391-91a6-d903b9fbdc3c">
### gLTF Skinned Mesh
Before:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/547569a6-d13b-4fe0-a8c1-e11f02c4f9a2">
After:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/34517aba-09e4-4e9b-982a-a4a8b893c48a">
---
## Changelog
- Increased lighting in `skybox`, `spotlight`, `animated_transform`, and
`gltf_skinned_mesh` examples.
- Improved usability of `spotlight` example.
2024-02-26 17:32:23 +00:00
|
|
|
brightness: 750.0,
|
2022-06-07 02:16:47 +00:00
|
|
|
..default()
|
2022-03-29 18:31:13 +00:00
|
|
|
})
|
2023-03-18 01:45:34 +00:00
|
|
|
.add_systems(Startup, setup)
|
|
|
|
.add_systems(Update, joint_animation)
|
2022-03-29 18:31:13 +00:00
|
|
|
.run();
|
|
|
|
}
|
|
|
|
|
|
|
|
fn setup(mut commands: Commands, asset_server: Res<AssetServer>) {
|
|
|
|
// Create a camera
|
Spawn now takes a Bundle (#6054)
# Objective
Now that we can consolidate Bundles and Components under a single insert (thanks to #2975 and #6039), almost 100% of world spawns now look like `world.spawn().insert((Some, Tuple, Here))`. Spawning an entity without any components is an extremely uncommon pattern, so it makes sense to give spawn the "first class" ergonomic api. This consolidated api should be made consistent across all spawn apis (such as World and Commands).
## Solution
All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input:
```rust
// before:
commands
.spawn()
.insert((A, B, C));
world
.spawn()
.insert((A, B, C);
// after
commands.spawn((A, B, C));
world.spawn((A, B, C));
```
All existing instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api. A new `spawn_empty` has been added, replacing the old `spawn` api.
By allowing `world.spawn(some_bundle)` to replace `world.spawn().insert(some_bundle)`, this opened the door to removing the initial entity allocation in the "empty" archetype / table done in `spawn()` (and subsequent move to the actual archetype in `.insert(some_bundle)`).
This improves spawn performance by over 10%:
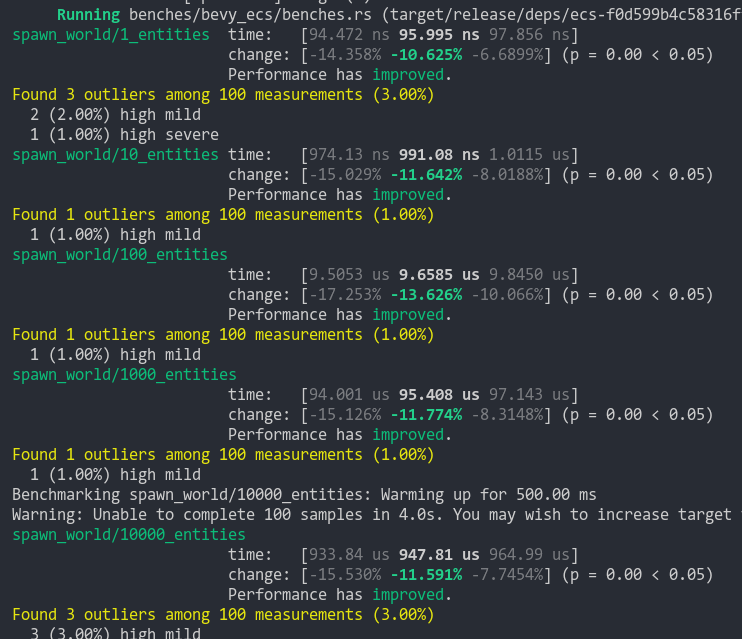
To take this measurement, I added a new `world_spawn` benchmark.
Unfortunately, optimizing `Commands::spawn` is slightly less trivial, as Commands expose the Entity id of spawned entities prior to actually spawning. Doing the optimization would (naively) require assurances that the `spawn(some_bundle)` command is applied before all other commands involving the entity (which would not necessarily be true, if memory serves). Optimizing `Commands::spawn` this way does feel possible, but it will require careful thought (and maybe some additional checks), which deserves its own PR. For now, it has the same performance characteristics of the current `Commands::spawn_bundle` on main.
**Note that 99% of this PR is simple renames and refactors. The only code that needs careful scrutiny is the new `World::spawn()` impl, which is relatively straightforward, but it has some new unsafe code (which re-uses battle tested BundlerSpawner code path).**
---
## Changelog
- All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input
- All instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api
- World and Commands now have `spawn_empty()`, which is equivalent to the old `spawn()` behavior.
## Migration Guide
```rust
// Old (0.8):
commands
.spawn()
.insert_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
commands.spawn_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
let entity = commands.spawn().id();
// New (0.9)
let entity = commands.spawn_empty().id();
// Old (0.8)
let entity = world.spawn().id();
// New (0.9)
let entity = world.spawn_empty();
```
2022-09-23 19:55:54 +00:00
|
|
|
commands.spawn(Camera3dBundle {
|
Improve lighting in more examples (#12021)
# Objective
- #11868 changed the lighting system, forcing lights to increase their
intensity. The PR fixed most examples, but missed a few. These I later
caught in https://github.com/bevyengine/bevy-website/pull/1023.
- Related: #11982, #11981.
- While there, I noticed that the spotlight example could use a few easy
improvements.
## Solution
- Increase lighting in `skybox`, `spotlight`, `animated_transform`, and
`gltf_skinned_mesh`.
- Improve spotlight example.
- Make ground plane move with cubes, so they don't phase into each
other.
- Batch spawn cubes.
- Add controls text.
- Change controls to allow rotating around spotlights.
## Showcase
### Skybox
Before:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/8ba00d74-6d68-4414-97a8-28afb8305570">
After:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/ad15c471-6979-4dda-9889-9189136d8404">
### Spotlight
Before:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/53f966de-acf3-46b8-8299-0005c4cb8da0">
After:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/05c73c1e-0739-4226-83d6-e4249a9105e0">
### Animated Transform
Before:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/6d7d4ea0-e22e-42a5-9905-ea1731d474cf">
After:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/f1ee08d6-d17a-4391-91a6-d903b9fbdc3c">
### gLTF Skinned Mesh
Before:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/547569a6-d13b-4fe0-a8c1-e11f02c4f9a2">
After:
<img width="1392" alt="image"
src="https://github.com/bevyengine/bevy/assets/59022059/34517aba-09e4-4e9b-982a-a4a8b893c48a">
---
## Changelog
- Increased lighting in `skybox`, `spotlight`, `animated_transform`, and
`gltf_skinned_mesh` examples.
- Improved usability of `spotlight` example.
2024-02-26 17:32:23 +00:00
|
|
|
transform: Transform::from_xyz(-2.0, 2.5, 5.0)
|
|
|
|
.looking_at(Vec3::new(0.0, 1.0, 0.0), Vec3::Y),
|
2022-03-29 18:31:13 +00:00
|
|
|
..default()
|
|
|
|
});
|
|
|
|
|
|
|
|
// Spawn the first scene in `models/SimpleSkin/SimpleSkin.gltf`
|
Spawn now takes a Bundle (#6054)
# Objective
Now that we can consolidate Bundles and Components under a single insert (thanks to #2975 and #6039), almost 100% of world spawns now look like `world.spawn().insert((Some, Tuple, Here))`. Spawning an entity without any components is an extremely uncommon pattern, so it makes sense to give spawn the "first class" ergonomic api. This consolidated api should be made consistent across all spawn apis (such as World and Commands).
## Solution
All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input:
```rust
// before:
commands
.spawn()
.insert((A, B, C));
world
.spawn()
.insert((A, B, C);
// after
commands.spawn((A, B, C));
world.spawn((A, B, C));
```
All existing instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api. A new `spawn_empty` has been added, replacing the old `spawn` api.
By allowing `world.spawn(some_bundle)` to replace `world.spawn().insert(some_bundle)`, this opened the door to removing the initial entity allocation in the "empty" archetype / table done in `spawn()` (and subsequent move to the actual archetype in `.insert(some_bundle)`).
This improves spawn performance by over 10%:
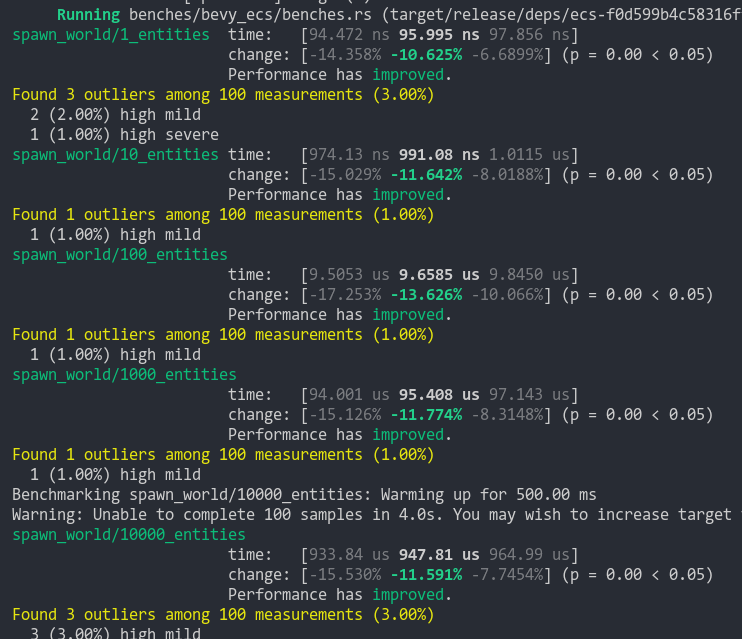
To take this measurement, I added a new `world_spawn` benchmark.
Unfortunately, optimizing `Commands::spawn` is slightly less trivial, as Commands expose the Entity id of spawned entities prior to actually spawning. Doing the optimization would (naively) require assurances that the `spawn(some_bundle)` command is applied before all other commands involving the entity (which would not necessarily be true, if memory serves). Optimizing `Commands::spawn` this way does feel possible, but it will require careful thought (and maybe some additional checks), which deserves its own PR. For now, it has the same performance characteristics of the current `Commands::spawn_bundle` on main.
**Note that 99% of this PR is simple renames and refactors. The only code that needs careful scrutiny is the new `World::spawn()` impl, which is relatively straightforward, but it has some new unsafe code (which re-uses battle tested BundlerSpawner code path).**
---
## Changelog
- All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input
- All instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api
- World and Commands now have `spawn_empty()`, which is equivalent to the old `spawn()` behavior.
## Migration Guide
```rust
// Old (0.8):
commands
.spawn()
.insert_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
commands.spawn_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
let entity = commands.spawn().id();
// New (0.9)
let entity = commands.spawn_empty().id();
// Old (0.8)
let entity = world.spawn().id();
// New (0.9)
let entity = world.spawn_empty();
```
2022-09-23 19:55:54 +00:00
|
|
|
commands.spawn(SceneBundle {
|
2024-05-31 23:25:57 +00:00
|
|
|
scene: asset_server
|
|
|
|
.load(GltfAssetLabel::Scene(0).from_asset("models/SimpleSkin/SimpleSkin.gltf")),
|
2022-06-09 20:34:09 +00:00
|
|
|
..default()
|
|
|
|
});
|
2022-03-29 18:31:13 +00:00
|
|
|
}
|
|
|
|
|
2022-05-16 13:53:20 +00:00
|
|
|
/// The scene hierarchy currently looks somewhat like this:
|
2022-03-29 18:31:13 +00:00
|
|
|
///
|
2024-01-01 16:50:56 +00:00
|
|
|
/// ```text
|
2022-03-29 18:31:13 +00:00
|
|
|
/// <Parent entity>
|
|
|
|
/// + Mesh node (without `PbrBundle` or `SkinnedMesh` component)
|
|
|
|
/// + Skinned mesh entity (with `PbrBundle` and `SkinnedMesh` component, created by glTF loader)
|
|
|
|
/// + First joint
|
|
|
|
/// + Second joint
|
|
|
|
/// ```
|
|
|
|
///
|
|
|
|
/// In this example, we want to get and animate the second joint.
|
|
|
|
/// It is similar to the animation defined in `models/SimpleSkin/SimpleSkin.gltf`.
|
|
|
|
fn joint_animation(
|
|
|
|
time: Res<Time>,
|
|
|
|
parent_query: Query<&Parent, With<SkinnedMesh>>,
|
|
|
|
children_query: Query<&Children>,
|
|
|
|
mut transform_query: Query<&mut Transform>,
|
|
|
|
) {
|
|
|
|
// Iter skinned mesh entity
|
2022-07-11 15:28:50 +00:00
|
|
|
for skinned_mesh_parent in &parent_query {
|
2022-03-29 18:31:13 +00:00
|
|
|
// Mesh node is the parent of the skinned mesh entity.
|
2022-07-10 20:29:06 +00:00
|
|
|
let mesh_node_entity = skinned_mesh_parent.get();
|
2022-03-29 18:31:13 +00:00
|
|
|
// Get `Children` in the mesh node.
|
|
|
|
let mesh_node_children = children_query.get(mesh_node_entity).unwrap();
|
|
|
|
|
|
|
|
// First joint is the second child of the mesh node.
|
|
|
|
let first_joint_entity = mesh_node_children[1];
|
|
|
|
// Get `Children` in the first joint.
|
|
|
|
let first_joint_children = children_query.get(first_joint_entity).unwrap();
|
|
|
|
|
|
|
|
// Second joint is the first child of the first joint.
|
|
|
|
let second_joint_entity = first_joint_children[0];
|
|
|
|
// Get `Transform` in the second joint.
|
|
|
|
let mut second_joint_transform = transform_query.get_mut(second_joint_entity).unwrap();
|
|
|
|
|
2022-08-30 19:52:11 +00:00
|
|
|
second_joint_transform.rotation =
|
2022-10-22 18:52:29 +00:00
|
|
|
Quat::from_rotation_z(FRAC_PI_2 * time.elapsed_seconds().sin());
|
2022-03-29 18:31:13 +00:00
|
|
|
}
|
|
|
|
}
|