2022-05-30 15:57:25 +00:00
|
|
|
//! A compute shader that simulates Conway's Game of Life.
|
2022-05-16 13:53:20 +00:00
|
|
|
//!
|
|
|
|
//! Compute shaders use the GPU for computing arbitrary information, that may be independent of what
|
|
|
|
//! is rendered to the screen.
|
|
|
|
|
2022-01-05 19:43:11 +00:00
|
|
|
use bevy::{
|
|
|
|
prelude::*,
|
|
|
|
render::{
|
2022-05-30 18:36:03 +00:00
|
|
|
extract_resource::{ExtractResource, ExtractResourcePlugin},
|
2022-01-05 19:43:11 +00:00
|
|
|
render_asset::RenderAssets,
|
|
|
|
render_graph::{self, RenderGraph},
|
|
|
|
render_resource::*,
|
|
|
|
renderer::{RenderContext, RenderDevice},
|
2023-03-18 01:45:34 +00:00
|
|
|
Render, RenderApp, RenderSet,
|
2022-01-05 19:43:11 +00:00
|
|
|
},
|
2023-01-19 00:38:28 +00:00
|
|
|
window::WindowPlugin,
|
2022-01-05 19:43:11 +00:00
|
|
|
};
|
2022-03-23 00:27:26 +00:00
|
|
|
use std::borrow::Cow;
|
2022-01-05 19:43:11 +00:00
|
|
|
|
|
|
|
const SIZE: (u32, u32) = (1280, 720);
|
|
|
|
const WORKGROUP_SIZE: u32 = 8;
|
|
|
|
|
|
|
|
fn main() {
|
|
|
|
App::new()
|
|
|
|
.insert_resource(ClearColor(Color::BLACK))
|
2023-06-21 20:51:03 +00:00
|
|
|
.add_plugins((
|
|
|
|
DefaultPlugins.set(WindowPlugin {
|
|
|
|
primary_window: Some(Window {
|
|
|
|
// uncomment for unthrottled FPS
|
|
|
|
// present_mode: bevy::window::PresentMode::AutoNoVsync,
|
|
|
|
..default()
|
|
|
|
}),
|
Plugins own their settings. Rework PluginGroup trait. (#6336)
# Objective
Fixes #5884 #2879
Alternative to #2988 #5885 #2886
"Immutable" Plugin settings are currently represented as normal ECS resources, which are read as part of plugin init. This presents a number of problems:
1. If a user inserts the plugin settings resource after the plugin is initialized, it will be silently ignored (and use the defaults instead)
2. Users can modify the plugin settings resource after the plugin has been initialized. This creates a false sense of control over settings that can no longer be changed.
(1) and (2) are especially problematic and confusing for the `WindowDescriptor` resource, but this is a general problem.
## Solution
Immutable Plugin settings now live on each Plugin struct (ex: `WindowPlugin`). PluginGroups have been reworked to support overriding plugin values. This also removes the need for the `add_plugins_with` api, as the `add_plugins` api can use the builder pattern directly. Settings that can be used at runtime continue to be represented as ECS resources.
Plugins are now configured like this:
```rust
app.add_plugin(AssetPlugin {
watch_for_changes: true,
..default()
})
```
PluginGroups are now configured like this:
```rust
app.add_plugins(DefaultPlugins
.set(AssetPlugin {
watch_for_changes: true,
..default()
})
)
```
This is an alternative to #2988, which is similar. But I personally prefer this solution for a couple of reasons:
* ~~#2988 doesn't solve (1)~~ #2988 does solve (1) and will panic in that case. I was wrong!
* This PR directly ties plugin settings to Plugin types in a 1:1 relationship, rather than a loose "setup resource" <-> plugin coupling (where the setup resource is consumed by the first plugin that uses it).
* I'm not a huge fan of overloading the ECS resource concept and implementation for something that has very different use cases and constraints.
## Changelog
- PluginGroups can now be configured directly using the builder pattern. Individual plugin values can be overridden by using `plugin_group.set(SomePlugin {})`, which enables overriding default plugin values.
- `WindowDescriptor` plugin settings have been moved to `WindowPlugin` and `AssetServerSettings` have been moved to `AssetPlugin`
- `app.add_plugins_with` has been replaced by using `add_plugins` with the builder pattern.
## Migration Guide
The `WindowDescriptor` settings have been moved from a resource to `WindowPlugin::window`:
```rust
// Old (Bevy 0.8)
app
.insert_resource(WindowDescriptor {
width: 400.0,
..default()
})
.add_plugins(DefaultPlugins)
// New (Bevy 0.9)
app.add_plugins(DefaultPlugins.set(WindowPlugin {
window: WindowDescriptor {
width: 400.0,
..default()
},
..default()
}))
```
The `AssetServerSettings` resource has been removed in favor of direct `AssetPlugin` configuration:
```rust
// Old (Bevy 0.8)
app
.insert_resource(AssetServerSettings {
watch_for_changes: true,
..default()
})
.add_plugins(DefaultPlugins)
// New (Bevy 0.9)
app.add_plugins(DefaultPlugins.set(AssetPlugin {
watch_for_changes: true,
..default()
}))
```
`add_plugins_with` has been replaced by `add_plugins` in combination with the builder pattern:
```rust
// Old (Bevy 0.8)
app.add_plugins_with(DefaultPlugins, |group| group.disable::<AssetPlugin>());
// New (Bevy 0.9)
app.add_plugins(DefaultPlugins.build().disable::<AssetPlugin>());
```
2022-10-24 21:20:33 +00:00
|
|
|
..default()
|
2023-01-19 00:38:28 +00:00
|
|
|
}),
|
2023-06-21 20:51:03 +00:00
|
|
|
GameOfLifeComputePlugin,
|
|
|
|
))
|
2023-03-18 01:45:34 +00:00
|
|
|
.add_systems(Startup, setup)
|
2022-01-05 19:43:11 +00:00
|
|
|
.run();
|
|
|
|
}
|
|
|
|
|
|
|
|
fn setup(mut commands: Commands, mut images: ResMut<Assets<Image>>) {
|
|
|
|
let mut image = Image::new_fill(
|
|
|
|
Extent3d {
|
|
|
|
width: SIZE.0,
|
|
|
|
height: SIZE.1,
|
|
|
|
depth_or_array_layers: 1,
|
|
|
|
},
|
|
|
|
TextureDimension::D2,
|
|
|
|
&[0, 0, 0, 255],
|
|
|
|
TextureFormat::Rgba8Unorm,
|
|
|
|
);
|
|
|
|
image.texture_descriptor.usage =
|
|
|
|
TextureUsages::COPY_DST | TextureUsages::STORAGE_BINDING | TextureUsages::TEXTURE_BINDING;
|
|
|
|
let image = images.add(image);
|
|
|
|
|
Spawn now takes a Bundle (#6054)
# Objective
Now that we can consolidate Bundles and Components under a single insert (thanks to #2975 and #6039), almost 100% of world spawns now look like `world.spawn().insert((Some, Tuple, Here))`. Spawning an entity without any components is an extremely uncommon pattern, so it makes sense to give spawn the "first class" ergonomic api. This consolidated api should be made consistent across all spawn apis (such as World and Commands).
## Solution
All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input:
```rust
// before:
commands
.spawn()
.insert((A, B, C));
world
.spawn()
.insert((A, B, C);
// after
commands.spawn((A, B, C));
world.spawn((A, B, C));
```
All existing instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api. A new `spawn_empty` has been added, replacing the old `spawn` api.
By allowing `world.spawn(some_bundle)` to replace `world.spawn().insert(some_bundle)`, this opened the door to removing the initial entity allocation in the "empty" archetype / table done in `spawn()` (and subsequent move to the actual archetype in `.insert(some_bundle)`).
This improves spawn performance by over 10%:
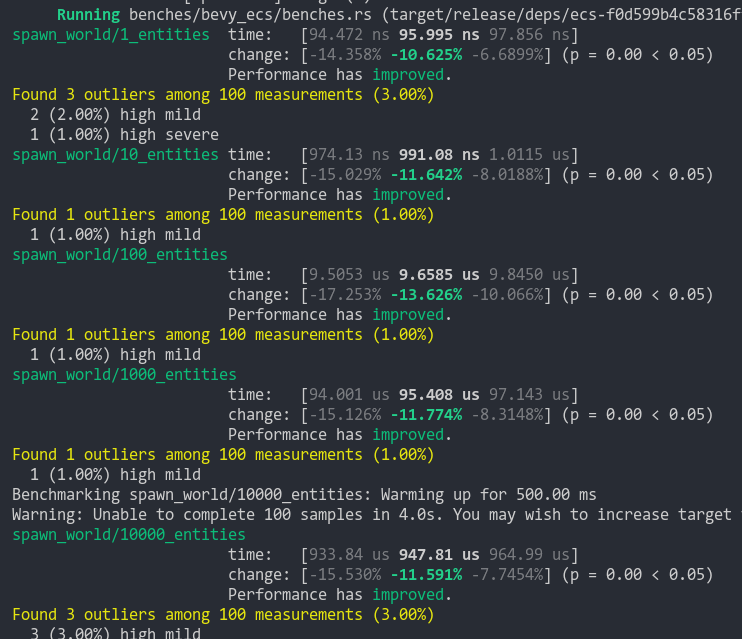
To take this measurement, I added a new `world_spawn` benchmark.
Unfortunately, optimizing `Commands::spawn` is slightly less trivial, as Commands expose the Entity id of spawned entities prior to actually spawning. Doing the optimization would (naively) require assurances that the `spawn(some_bundle)` command is applied before all other commands involving the entity (which would not necessarily be true, if memory serves). Optimizing `Commands::spawn` this way does feel possible, but it will require careful thought (and maybe some additional checks), which deserves its own PR. For now, it has the same performance characteristics of the current `Commands::spawn_bundle` on main.
**Note that 99% of this PR is simple renames and refactors. The only code that needs careful scrutiny is the new `World::spawn()` impl, which is relatively straightforward, but it has some new unsafe code (which re-uses battle tested BundlerSpawner code path).**
---
## Changelog
- All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input
- All instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api
- World and Commands now have `spawn_empty()`, which is equivalent to the old `spawn()` behavior.
## Migration Guide
```rust
// Old (0.8):
commands
.spawn()
.insert_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
commands.spawn_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
let entity = commands.spawn().id();
// New (0.9)
let entity = commands.spawn_empty().id();
// Old (0.8)
let entity = world.spawn().id();
// New (0.9)
let entity = world.spawn_empty();
```
2022-09-23 19:55:54 +00:00
|
|
|
commands.spawn(SpriteBundle {
|
2022-01-05 19:43:11 +00:00
|
|
|
sprite: Sprite {
|
|
|
|
custom_size: Some(Vec2::new(SIZE.0 as f32, SIZE.1 as f32)),
|
2022-03-01 20:52:09 +00:00
|
|
|
..default()
|
2022-01-05 19:43:11 +00:00
|
|
|
},
|
|
|
|
texture: image.clone(),
|
2022-03-01 20:52:09 +00:00
|
|
|
..default()
|
2022-01-05 19:43:11 +00:00
|
|
|
});
|
Spawn now takes a Bundle (#6054)
# Objective
Now that we can consolidate Bundles and Components under a single insert (thanks to #2975 and #6039), almost 100% of world spawns now look like `world.spawn().insert((Some, Tuple, Here))`. Spawning an entity without any components is an extremely uncommon pattern, so it makes sense to give spawn the "first class" ergonomic api. This consolidated api should be made consistent across all spawn apis (such as World and Commands).
## Solution
All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input:
```rust
// before:
commands
.spawn()
.insert((A, B, C));
world
.spawn()
.insert((A, B, C);
// after
commands.spawn((A, B, C));
world.spawn((A, B, C));
```
All existing instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api. A new `spawn_empty` has been added, replacing the old `spawn` api.
By allowing `world.spawn(some_bundle)` to replace `world.spawn().insert(some_bundle)`, this opened the door to removing the initial entity allocation in the "empty" archetype / table done in `spawn()` (and subsequent move to the actual archetype in `.insert(some_bundle)`).
This improves spawn performance by over 10%:
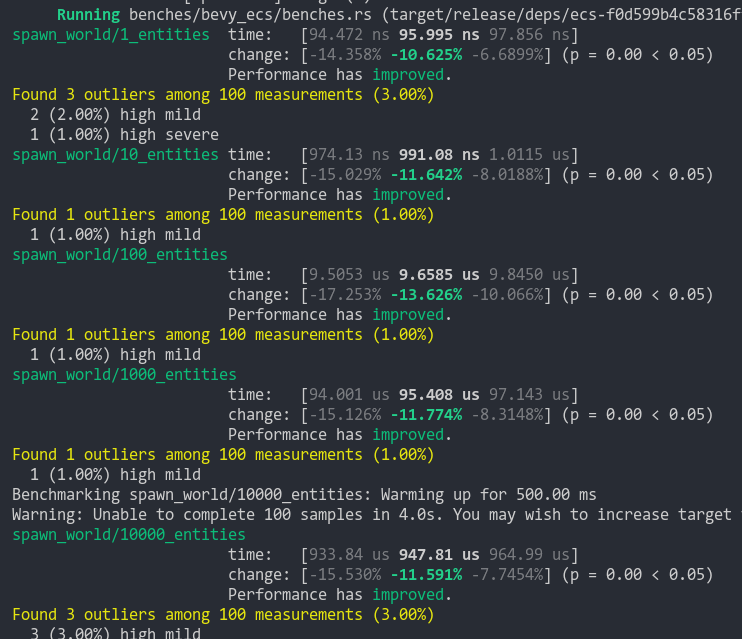
To take this measurement, I added a new `world_spawn` benchmark.
Unfortunately, optimizing `Commands::spawn` is slightly less trivial, as Commands expose the Entity id of spawned entities prior to actually spawning. Doing the optimization would (naively) require assurances that the `spawn(some_bundle)` command is applied before all other commands involving the entity (which would not necessarily be true, if memory serves). Optimizing `Commands::spawn` this way does feel possible, but it will require careful thought (and maybe some additional checks), which deserves its own PR. For now, it has the same performance characteristics of the current `Commands::spawn_bundle` on main.
**Note that 99% of this PR is simple renames and refactors. The only code that needs careful scrutiny is the new `World::spawn()` impl, which is relatively straightforward, but it has some new unsafe code (which re-uses battle tested BundlerSpawner code path).**
---
## Changelog
- All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input
- All instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api
- World and Commands now have `spawn_empty()`, which is equivalent to the old `spawn()` behavior.
## Migration Guide
```rust
// Old (0.8):
commands
.spawn()
.insert_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
commands.spawn_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
let entity = commands.spawn().id();
// New (0.9)
let entity = commands.spawn_empty().id();
// Old (0.8)
let entity = world.spawn().id();
// New (0.9)
let entity = world.spawn_empty();
```
2022-09-23 19:55:54 +00:00
|
|
|
commands.spawn(Camera2dBundle::default());
|
2022-01-05 19:43:11 +00:00
|
|
|
|
2022-02-13 22:33:55 +00:00
|
|
|
commands.insert_resource(GameOfLifeImage(image));
|
2022-01-05 19:43:11 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
pub struct GameOfLifeComputePlugin;
|
|
|
|
|
|
|
|
impl Plugin for GameOfLifeComputePlugin {
|
|
|
|
fn build(&self, app: &mut App) {
|
2022-05-30 18:36:03 +00:00
|
|
|
// Extract the game of life image resource from the main world into the render world
|
|
|
|
// for operation on by the compute shader and display on the sprite.
|
2023-06-21 20:51:03 +00:00
|
|
|
app.add_plugins(ExtractResourcePlugin::<GameOfLifeImage>::default());
|
2022-01-05 19:43:11 +00:00
|
|
|
let render_app = app.sub_app_mut(RenderApp);
|
Webgpu support (#8336)
# Objective
- Support WebGPU
- alternative to #5027 that doesn't need any async / await
- fixes #8315
- Surprise fix #7318
## Solution
### For async renderer initialisation
- Update the plugin lifecycle:
- app builds the plugin
- calls `plugin.build`
- registers the plugin
- app starts the event loop
- event loop waits for `ready` of all registered plugins in the same
order
- returns `true` by default
- then call all `finish` then all `cleanup` in the same order as
registered
- then execute the schedule
In the case of the renderer, to avoid anything async:
- building the renderer plugin creates a detached task that will send
back the initialised renderer through a mutex in a resource
- `ready` will wait for the renderer to be present in the resource
- `finish` will take that renderer and place it in the expected
resources by other plugins
- other plugins (that expect the renderer to be available) `finish` are
called and they are able to set up their pipelines
- `cleanup` is called, only custom one is still for pipeline rendering
### For WebGPU support
- update the `build-wasm-example` script to support passing `--api
webgpu` that will build the example with WebGPU support
- feature for webgl2 was always enabled when building for wasm. it's now
in the default feature list and enabled on all platforms, so check for
this feature must also check that the target_arch is `wasm32`
---
## Migration Guide
- `Plugin::setup` has been renamed `Plugin::cleanup`
- `Plugin::finish` has been added, and plugins adding pipelines should
do it in this function instead of `Plugin::build`
```rust
// Before
impl Plugin for MyPlugin {
fn build(&self, app: &mut App) {
app.insert_resource::<MyResource>
.add_systems(Update, my_system);
let render_app = match app.get_sub_app_mut(RenderApp) {
Ok(render_app) => render_app,
Err(_) => return,
};
render_app
.init_resource::<RenderResourceNeedingDevice>()
.init_resource::<OtherRenderResource>();
}
}
// After
impl Plugin for MyPlugin {
fn build(&self, app: &mut App) {
app.insert_resource::<MyResource>
.add_systems(Update, my_system);
let render_app = match app.get_sub_app_mut(RenderApp) {
Ok(render_app) => render_app,
Err(_) => return,
};
render_app
.init_resource::<OtherRenderResource>();
}
fn finish(&self, app: &mut App) {
let render_app = match app.get_sub_app_mut(RenderApp) {
Ok(render_app) => render_app,
Err(_) => return,
};
render_app
.init_resource::<RenderResourceNeedingDevice>();
}
}
```
2023-05-04 22:07:57 +00:00
|
|
|
render_app.add_systems(Render, queue_bind_group.in_set(RenderSet::Queue));
|
2022-01-05 19:43:11 +00:00
|
|
|
|
2022-02-27 22:37:18 +00:00
|
|
|
let mut render_graph = render_app.world.resource_mut::<RenderGraph>();
|
2022-03-23 00:27:26 +00:00
|
|
|
render_graph.add_node("game_of_life", GameOfLifeNode::default());
|
2022-11-21 21:58:39 +00:00
|
|
|
render_graph.add_node_edge(
|
|
|
|
"game_of_life",
|
|
|
|
bevy::render::main_graph::node::CAMERA_DRIVER,
|
|
|
|
);
|
2022-01-05 19:43:11 +00:00
|
|
|
}
|
Webgpu support (#8336)
# Objective
- Support WebGPU
- alternative to #5027 that doesn't need any async / await
- fixes #8315
- Surprise fix #7318
## Solution
### For async renderer initialisation
- Update the plugin lifecycle:
- app builds the plugin
- calls `plugin.build`
- registers the plugin
- app starts the event loop
- event loop waits for `ready` of all registered plugins in the same
order
- returns `true` by default
- then call all `finish` then all `cleanup` in the same order as
registered
- then execute the schedule
In the case of the renderer, to avoid anything async:
- building the renderer plugin creates a detached task that will send
back the initialised renderer through a mutex in a resource
- `ready` will wait for the renderer to be present in the resource
- `finish` will take that renderer and place it in the expected
resources by other plugins
- other plugins (that expect the renderer to be available) `finish` are
called and they are able to set up their pipelines
- `cleanup` is called, only custom one is still for pipeline rendering
### For WebGPU support
- update the `build-wasm-example` script to support passing `--api
webgpu` that will build the example with WebGPU support
- feature for webgl2 was always enabled when building for wasm. it's now
in the default feature list and enabled on all platforms, so check for
this feature must also check that the target_arch is `wasm32`
---
## Migration Guide
- `Plugin::setup` has been renamed `Plugin::cleanup`
- `Plugin::finish` has been added, and plugins adding pipelines should
do it in this function instead of `Plugin::build`
```rust
// Before
impl Plugin for MyPlugin {
fn build(&self, app: &mut App) {
app.insert_resource::<MyResource>
.add_systems(Update, my_system);
let render_app = match app.get_sub_app_mut(RenderApp) {
Ok(render_app) => render_app,
Err(_) => return,
};
render_app
.init_resource::<RenderResourceNeedingDevice>()
.init_resource::<OtherRenderResource>();
}
}
// After
impl Plugin for MyPlugin {
fn build(&self, app: &mut App) {
app.insert_resource::<MyResource>
.add_systems(Update, my_system);
let render_app = match app.get_sub_app_mut(RenderApp) {
Ok(render_app) => render_app,
Err(_) => return,
};
render_app
.init_resource::<OtherRenderResource>();
}
fn finish(&self, app: &mut App) {
let render_app = match app.get_sub_app_mut(RenderApp) {
Ok(render_app) => render_app,
Err(_) => return,
};
render_app
.init_resource::<RenderResourceNeedingDevice>();
}
}
```
2023-05-04 22:07:57 +00:00
|
|
|
|
|
|
|
fn finish(&self, app: &mut App) {
|
|
|
|
let render_app = app.sub_app_mut(RenderApp);
|
|
|
|
render_app.init_resource::<GameOfLifePipeline>();
|
|
|
|
}
|
2022-01-05 19:43:11 +00:00
|
|
|
}
|
|
|
|
|
Make `Resource` trait opt-in, requiring `#[derive(Resource)]` V2 (#5577)
*This PR description is an edited copy of #5007, written by @alice-i-cecile.*
# Objective
Follow-up to https://github.com/bevyengine/bevy/pull/2254. The `Resource` trait currently has a blanket implementation for all types that meet its bounds.
While ergonomic, this results in several drawbacks:
* it is possible to make confusing, silent mistakes such as inserting a function pointer (Foo) rather than a value (Foo::Bar) as a resource
* it is challenging to discover if a type is intended to be used as a resource
* we cannot later add customization options (see the [RFC](https://github.com/bevyengine/rfcs/blob/main/rfcs/27-derive-component.md) for the equivalent choice for Component).
* dependencies can use the same Rust type as a resource in invisibly conflicting ways
* raw Rust types used as resources cannot preserve privacy appropriately, as anyone able to access that type can read and write to internal values
* we cannot capture a definitive list of possible resources to display to users in an editor
## Notes to reviewers
* Review this commit-by-commit; there's effectively no back-tracking and there's a lot of churn in some of these commits.
*ira: My commits are not as well organized :')*
* I've relaxed the bound on Local to Send + Sync + 'static: I don't think these concerns apply there, so this can keep things simple. Storing e.g. a u32 in a Local is fine, because there's a variable name attached explaining what it does.
* I think this is a bad place for the Resource trait to live, but I've left it in place to make reviewing easier. IMO that's best tackled with https://github.com/bevyengine/bevy/issues/4981.
## Changelog
`Resource` is no longer automatically implemented for all matching types. Instead, use the new `#[derive(Resource)]` macro.
## Migration Guide
Add `#[derive(Resource)]` to all types you are using as a resource.
If you are using a third party type as a resource, wrap it in a tuple struct to bypass orphan rules. Consider deriving `Deref` and `DerefMut` to improve ergonomics.
`ClearColor` no longer implements `Component`. Using `ClearColor` as a component in 0.8 did nothing.
Use the `ClearColorConfig` in the `Camera3d` and `Camera2d` components instead.
Co-authored-by: Alice <alice.i.cecile@gmail.com>
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
Co-authored-by: devil-ira <justthecooldude@gmail.com>
Co-authored-by: Carter Anderson <mcanders1@gmail.com>
2022-08-08 21:36:35 +00:00
|
|
|
#[derive(Resource, Clone, Deref, ExtractResource)]
|
2022-01-05 19:43:11 +00:00
|
|
|
struct GameOfLifeImage(Handle<Image>);
|
2022-05-16 13:53:20 +00:00
|
|
|
|
Make `Resource` trait opt-in, requiring `#[derive(Resource)]` V2 (#5577)
*This PR description is an edited copy of #5007, written by @alice-i-cecile.*
# Objective
Follow-up to https://github.com/bevyengine/bevy/pull/2254. The `Resource` trait currently has a blanket implementation for all types that meet its bounds.
While ergonomic, this results in several drawbacks:
* it is possible to make confusing, silent mistakes such as inserting a function pointer (Foo) rather than a value (Foo::Bar) as a resource
* it is challenging to discover if a type is intended to be used as a resource
* we cannot later add customization options (see the [RFC](https://github.com/bevyengine/rfcs/blob/main/rfcs/27-derive-component.md) for the equivalent choice for Component).
* dependencies can use the same Rust type as a resource in invisibly conflicting ways
* raw Rust types used as resources cannot preserve privacy appropriately, as anyone able to access that type can read and write to internal values
* we cannot capture a definitive list of possible resources to display to users in an editor
## Notes to reviewers
* Review this commit-by-commit; there's effectively no back-tracking and there's a lot of churn in some of these commits.
*ira: My commits are not as well organized :')*
* I've relaxed the bound on Local to Send + Sync + 'static: I don't think these concerns apply there, so this can keep things simple. Storing e.g. a u32 in a Local is fine, because there's a variable name attached explaining what it does.
* I think this is a bad place for the Resource trait to live, but I've left it in place to make reviewing easier. IMO that's best tackled with https://github.com/bevyengine/bevy/issues/4981.
## Changelog
`Resource` is no longer automatically implemented for all matching types. Instead, use the new `#[derive(Resource)]` macro.
## Migration Guide
Add `#[derive(Resource)]` to all types you are using as a resource.
If you are using a third party type as a resource, wrap it in a tuple struct to bypass orphan rules. Consider deriving `Deref` and `DerefMut` to improve ergonomics.
`ClearColor` no longer implements `Component`. Using `ClearColor` as a component in 0.8 did nothing.
Use the `ClearColorConfig` in the `Camera3d` and `Camera2d` components instead.
Co-authored-by: Alice <alice.i.cecile@gmail.com>
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
Co-authored-by: devil-ira <justthecooldude@gmail.com>
Co-authored-by: Carter Anderson <mcanders1@gmail.com>
2022-08-08 21:36:35 +00:00
|
|
|
#[derive(Resource)]
|
2022-01-05 19:43:11 +00:00
|
|
|
struct GameOfLifeImageBindGroup(BindGroup);
|
|
|
|
|
|
|
|
fn queue_bind_group(
|
|
|
|
mut commands: Commands,
|
|
|
|
pipeline: Res<GameOfLifePipeline>,
|
|
|
|
gpu_images: Res<RenderAssets<Image>>,
|
|
|
|
game_of_life_image: Res<GameOfLifeImage>,
|
|
|
|
render_device: Res<RenderDevice>,
|
|
|
|
) {
|
|
|
|
let view = &gpu_images[&game_of_life_image.0];
|
|
|
|
let bind_group = render_device.create_bind_group(&BindGroupDescriptor {
|
|
|
|
label: None,
|
|
|
|
layout: &pipeline.texture_bind_group_layout,
|
|
|
|
entries: &[BindGroupEntry {
|
|
|
|
binding: 0,
|
|
|
|
resource: BindingResource::TextureView(&view.texture_view),
|
|
|
|
}],
|
|
|
|
});
|
|
|
|
commands.insert_resource(GameOfLifeImageBindGroup(bind_group));
|
|
|
|
}
|
|
|
|
|
Make `Resource` trait opt-in, requiring `#[derive(Resource)]` V2 (#5577)
*This PR description is an edited copy of #5007, written by @alice-i-cecile.*
# Objective
Follow-up to https://github.com/bevyengine/bevy/pull/2254. The `Resource` trait currently has a blanket implementation for all types that meet its bounds.
While ergonomic, this results in several drawbacks:
* it is possible to make confusing, silent mistakes such as inserting a function pointer (Foo) rather than a value (Foo::Bar) as a resource
* it is challenging to discover if a type is intended to be used as a resource
* we cannot later add customization options (see the [RFC](https://github.com/bevyengine/rfcs/blob/main/rfcs/27-derive-component.md) for the equivalent choice for Component).
* dependencies can use the same Rust type as a resource in invisibly conflicting ways
* raw Rust types used as resources cannot preserve privacy appropriately, as anyone able to access that type can read and write to internal values
* we cannot capture a definitive list of possible resources to display to users in an editor
## Notes to reviewers
* Review this commit-by-commit; there's effectively no back-tracking and there's a lot of churn in some of these commits.
*ira: My commits are not as well organized :')*
* I've relaxed the bound on Local to Send + Sync + 'static: I don't think these concerns apply there, so this can keep things simple. Storing e.g. a u32 in a Local is fine, because there's a variable name attached explaining what it does.
* I think this is a bad place for the Resource trait to live, but I've left it in place to make reviewing easier. IMO that's best tackled with https://github.com/bevyengine/bevy/issues/4981.
## Changelog
`Resource` is no longer automatically implemented for all matching types. Instead, use the new `#[derive(Resource)]` macro.
## Migration Guide
Add `#[derive(Resource)]` to all types you are using as a resource.
If you are using a third party type as a resource, wrap it in a tuple struct to bypass orphan rules. Consider deriving `Deref` and `DerefMut` to improve ergonomics.
`ClearColor` no longer implements `Component`. Using `ClearColor` as a component in 0.8 did nothing.
Use the `ClearColorConfig` in the `Camera3d` and `Camera2d` components instead.
Co-authored-by: Alice <alice.i.cecile@gmail.com>
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
Co-authored-by: devil-ira <justthecooldude@gmail.com>
Co-authored-by: Carter Anderson <mcanders1@gmail.com>
2022-08-08 21:36:35 +00:00
|
|
|
#[derive(Resource)]
|
2022-01-05 19:43:11 +00:00
|
|
|
pub struct GameOfLifePipeline {
|
|
|
|
texture_bind_group_layout: BindGroupLayout,
|
2022-03-23 00:27:26 +00:00
|
|
|
init_pipeline: CachedComputePipelineId,
|
|
|
|
update_pipeline: CachedComputePipelineId,
|
2022-01-05 19:43:11 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
impl FromWorld for GameOfLifePipeline {
|
|
|
|
fn from_world(world: &mut World) -> Self {
|
|
|
|
let texture_bind_group_layout =
|
2022-03-23 00:27:26 +00:00
|
|
|
world
|
|
|
|
.resource::<RenderDevice>()
|
|
|
|
.create_bind_group_layout(&BindGroupLayoutDescriptor {
|
|
|
|
label: None,
|
|
|
|
entries: &[BindGroupLayoutEntry {
|
|
|
|
binding: 0,
|
|
|
|
visibility: ShaderStages::COMPUTE,
|
|
|
|
ty: BindingType::StorageTexture {
|
|
|
|
access: StorageTextureAccess::ReadWrite,
|
|
|
|
format: TextureFormat::Rgba8Unorm,
|
|
|
|
view_dimension: TextureViewDimension::D2,
|
|
|
|
},
|
|
|
|
count: None,
|
|
|
|
}],
|
|
|
|
});
|
|
|
|
let shader = world
|
|
|
|
.resource::<AssetServer>()
|
|
|
|
.load("shaders/game_of_life.wgsl");
|
2023-01-16 15:41:14 +00:00
|
|
|
let pipeline_cache = world.resource::<PipelineCache>();
|
2022-03-23 00:27:26 +00:00
|
|
|
let init_pipeline = pipeline_cache.queue_compute_pipeline(ComputePipelineDescriptor {
|
2022-01-05 19:43:11 +00:00
|
|
|
label: None,
|
2023-02-17 06:20:16 +00:00
|
|
|
layout: vec![texture_bind_group_layout.clone()],
|
|
|
|
push_constant_ranges: Vec::new(),
|
2022-03-23 00:27:26 +00:00
|
|
|
shader: shader.clone(),
|
|
|
|
shader_defs: vec![],
|
|
|
|
entry_point: Cow::from("init"),
|
2022-01-05 19:43:11 +00:00
|
|
|
});
|
2022-03-23 00:27:26 +00:00
|
|
|
let update_pipeline = pipeline_cache.queue_compute_pipeline(ComputePipelineDescriptor {
|
2022-01-05 19:43:11 +00:00
|
|
|
label: None,
|
2023-02-17 06:20:16 +00:00
|
|
|
layout: vec![texture_bind_group_layout.clone()],
|
|
|
|
push_constant_ranges: Vec::new(),
|
2022-03-23 00:27:26 +00:00
|
|
|
shader,
|
|
|
|
shader_defs: vec![],
|
|
|
|
entry_point: Cow::from("update"),
|
2022-01-05 19:43:11 +00:00
|
|
|
});
|
|
|
|
|
|
|
|
GameOfLifePipeline {
|
|
|
|
texture_bind_group_layout,
|
2022-03-23 00:27:26 +00:00
|
|
|
init_pipeline,
|
|
|
|
update_pipeline,
|
2022-01-05 19:43:11 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-03-23 00:27:26 +00:00
|
|
|
enum GameOfLifeState {
|
|
|
|
Loading,
|
|
|
|
Init,
|
|
|
|
Update,
|
2022-01-05 19:43:11 +00:00
|
|
|
}
|
|
|
|
|
2022-03-23 00:27:26 +00:00
|
|
|
struct GameOfLifeNode {
|
|
|
|
state: GameOfLifeState,
|
2022-01-05 19:43:11 +00:00
|
|
|
}
|
2022-03-23 00:27:26 +00:00
|
|
|
|
|
|
|
impl Default for GameOfLifeNode {
|
2022-01-05 19:43:11 +00:00
|
|
|
fn default() -> Self {
|
|
|
|
Self {
|
2022-03-23 00:27:26 +00:00
|
|
|
state: GameOfLifeState::Loading,
|
2022-01-05 19:43:11 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|
2022-03-23 00:27:26 +00:00
|
|
|
|
|
|
|
impl render_graph::Node for GameOfLifeNode {
|
|
|
|
fn update(&mut self, world: &mut World) {
|
|
|
|
let pipeline = world.resource::<GameOfLifePipeline>();
|
|
|
|
let pipeline_cache = world.resource::<PipelineCache>();
|
|
|
|
|
|
|
|
// if the corresponding pipeline has loaded, transition to the next stage
|
|
|
|
match self.state {
|
|
|
|
GameOfLifeState::Loading => {
|
|
|
|
if let CachedPipelineState::Ok(_) =
|
|
|
|
pipeline_cache.get_compute_pipeline_state(pipeline.init_pipeline)
|
|
|
|
{
|
2022-05-31 01:38:07 +00:00
|
|
|
self.state = GameOfLifeState::Init;
|
2022-03-23 00:27:26 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
GameOfLifeState::Init => {
|
|
|
|
if let CachedPipelineState::Ok(_) =
|
|
|
|
pipeline_cache.get_compute_pipeline_state(pipeline.update_pipeline)
|
|
|
|
{
|
2022-05-31 01:38:07 +00:00
|
|
|
self.state = GameOfLifeState::Update;
|
2022-03-23 00:27:26 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
GameOfLifeState::Update => {}
|
2022-01-05 19:43:11 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
fn run(
|
|
|
|
&self,
|
|
|
|
_graph: &mut render_graph::RenderGraphContext,
|
|
|
|
render_context: &mut RenderContext,
|
|
|
|
world: &World,
|
|
|
|
) -> Result<(), render_graph::NodeRunError> {
|
2022-02-27 22:37:18 +00:00
|
|
|
let texture_bind_group = &world.resource::<GameOfLifeImageBindGroup>().0;
|
2022-03-23 00:27:26 +00:00
|
|
|
let pipeline_cache = world.resource::<PipelineCache>();
|
|
|
|
let pipeline = world.resource::<GameOfLifePipeline>();
|
2022-01-05 19:43:11 +00:00
|
|
|
|
|
|
|
let mut pass = render_context
|
Support recording multiple CommandBuffers in RenderContext (#7248)
# Objective
`RenderContext`, the core abstraction for running the render graph, currently only supports recording one `CommandBuffer` across the entire render graph. This means the entire buffer must be recorded sequentially, usually via the render graph itself. This prevents parallelization and forces users to only encode their commands in the render graph.
## Solution
Allow `RenderContext` to store a `Vec<CommandBuffer>` that it progressively appends to. By default, the context will not have a command encoder, but will create one as soon as either `begin_tracked_render_pass` or the `command_encoder` accesor is first called. `RenderContext::add_command_buffer` allows users to interrupt the current command encoder, flush it to the vec, append a user-provided `CommandBuffer` and reset the command encoder to start a new buffer. Users or the render graph will call `RenderContext::finish` to retrieve the series of buffers for submitting to the queue.
This allows users to encode their own `CommandBuffer`s outside of the render graph, potentially in different threads, and store them in components or resources.
Ideally, in the future, the core pipeline passes can run in `RenderStage::Render` systems and end up saving the completed command buffers to either `Commands` or a field in `RenderPhase`.
## Alternatives
The alternative is to use to use wgpu's `RenderBundle`s, which can achieve similar results; however it's not universally available (no OpenGL, WebGL, and DX11).
---
## Changelog
Added: `RenderContext::new`
Added: `RenderContext::add_command_buffer`
Added: `RenderContext::finish`
Changed: `RenderContext::render_device` is now private. Use the accessor `RenderContext::render_device()` instead.
Changed: `RenderContext::command_encoder` is now private. Use the accessor `RenderContext::command_encoder()` instead.
Changed: `RenderContext` now supports adding external `CommandBuffer`s for inclusion into the render graphs. These buffers can be encoded outside of the render graph (i.e. in a system).
## Migration Guide
`RenderContext`'s fields are now private. Use the accessors on `RenderContext` instead, and construct it with `RenderContext::new`.
2023-01-22 00:21:55 +00:00
|
|
|
.command_encoder()
|
2022-01-05 19:43:11 +00:00
|
|
|
.begin_compute_pass(&ComputePassDescriptor::default());
|
|
|
|
|
|
|
|
pass.set_bind_group(0, texture_bind_group, &[]);
|
2022-03-23 00:27:26 +00:00
|
|
|
|
|
|
|
// select the pipeline based on the current state
|
|
|
|
match self.state {
|
|
|
|
GameOfLifeState::Loading => {}
|
|
|
|
GameOfLifeState::Init => {
|
|
|
|
let init_pipeline = pipeline_cache
|
|
|
|
.get_compute_pipeline(pipeline.init_pipeline)
|
|
|
|
.unwrap();
|
|
|
|
pass.set_pipeline(init_pipeline);
|
2022-07-14 21:17:16 +00:00
|
|
|
pass.dispatch_workgroups(SIZE.0 / WORKGROUP_SIZE, SIZE.1 / WORKGROUP_SIZE, 1);
|
2022-03-23 00:27:26 +00:00
|
|
|
}
|
|
|
|
GameOfLifeState::Update => {
|
|
|
|
let update_pipeline = pipeline_cache
|
|
|
|
.get_compute_pipeline(pipeline.update_pipeline)
|
|
|
|
.unwrap();
|
|
|
|
pass.set_pipeline(update_pipeline);
|
2022-07-14 21:17:16 +00:00
|
|
|
pass.dispatch_workgroups(SIZE.0 / WORKGROUP_SIZE, SIZE.1 / WORKGROUP_SIZE, 1);
|
2022-03-23 00:27:26 +00:00
|
|
|
}
|
|
|
|
}
|
2022-01-05 19:43:11 +00:00
|
|
|
|
|
|
|
Ok(())
|
|
|
|
}
|
|
|
|
}
|