2017-07-04 12:27:32 +00:00
|
|
|
use std::collections::HashMap;
|
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
use libs::tera::Tera;
|
2017-07-04 12:27:32 +00:00
|
|
|
|
2018-05-06 20:58:39 +00:00
|
|
|
use config::Config;
|
2022-04-26 18:51:04 +00:00
|
|
|
use markdown::{render_content, RenderContext};
|
2018-10-18 20:50:06 +00:00
|
|
|
use templates::ZOLA_TERA;
|
2020-02-05 08:13:14 +00:00
|
|
|
use utils::slugs::SlugifyStrategy;
|
2022-04-26 18:51:04 +00:00
|
|
|
use utils::types::InsertAnchor;
|
2017-07-04 12:27:32 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
mod common;
|
2017-10-23 12:18:05 +00:00
|
|
|
|
|
|
|
#[test]
|
2022-02-25 16:13:29 +00:00
|
|
|
fn can_render_basic_markdown() {
|
|
|
|
let cases = vec",
|
|
|
|
"",
|
|
|
|
"<h1>some html</h1>",
|
2017-10-23 12:18:05 +00:00
|
|
|
];
|
2017-07-04 12:27:32 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
let body = common::render(&cases.join("\n")).unwrap().body;
|
|
|
|
insta::assert_snapshot!(body);
|
2017-07-04 12:27:32 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
2022-02-25 16:13:29 +00:00
|
|
|
fn can_make_zola_internal_links() {
|
|
|
|
let body = common::render(
|
2018-10-31 07:18:57 +00:00
|
|
|
r#"
|
2022-02-25 16:13:29 +00:00
|
|
|
[rel link](@/pages/about.md)
|
|
|
|
[rel link with anchor](@/pages/about.md#cv)
|
|
|
|
[abs link](https://getzola.org/about/)
|
|
|
|
"#,
|
2018-10-31 07:18:57 +00:00
|
|
|
)
|
2022-02-25 16:13:29 +00:00
|
|
|
.unwrap()
|
|
|
|
.body;
|
|
|
|
insta::assert_snapshot!(body);
|
2018-08-24 21:46:28 +00:00
|
|
|
}
|
2018-11-16 17:19:38 +00:00
|
|
|
|
|
|
|
#[test]
|
2022-02-25 16:13:29 +00:00
|
|
|
fn can_handle_heading_ids() {
|
|
|
|
let mut config = Config::default_for_test();
|
2018-11-16 17:19:38 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
let cases = vec",
|
|
|
|
// https://github.com/Keats/gutenberg/issues/297
|
|
|
|
"# [Rust](https://rust-lang.org \"Rust homepage\")",
|
|
|
|
// and then some markdown in them
|
|
|
|
"# `hi`",
|
|
|
|
"# *hi*",
|
|
|
|
"# **hi**",
|
|
|
|
// See https://github.com/getzola/zola/issues/569
|
|
|
|
"# text [^1] there\n[^1]: footnote",
|
2022-03-03 23:16:48 +00:00
|
|
|
// Chosen slug that already exists with space
|
|
|
|
"# Classes {#classes .bold .another}",
|
2022-02-25 16:13:29 +00:00
|
|
|
];
|
|
|
|
let body = common::render_with_config(&cases.join("\n"), config.clone()).unwrap().body;
|
|
|
|
insta::assert_snapshot!(body);
|
2018-11-16 17:19:38 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
// And now test without slugifying everything
|
|
|
|
config.slugify.anchors = SlugifyStrategy::Safe;
|
|
|
|
let body = common::render_with_config(&cases.join("\n"), config).unwrap().body;
|
|
|
|
insta::assert_snapshot!(body);
|
|
|
|
}
|
2018-11-16 17:19:38 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
#[test]
|
|
|
|
fn can_insert_anchors() {
|
|
|
|
let cases = vec",
|
|
|
|
"# Hello*_()",
|
|
|
|
];
|
|
|
|
let body =
|
|
|
|
common::render_with_insert_anchor(&cases.join("\n"), InsertAnchor::Left).unwrap().body;
|
|
|
|
insta::assert_snapshot!(body);
|
|
|
|
let body =
|
|
|
|
common::render_with_insert_anchor(&cases.join("\n"), InsertAnchor::Right).unwrap().body;
|
|
|
|
insta::assert_snapshot!(body);
|
2022-07-10 13:02:07 +00:00
|
|
|
let body =
|
|
|
|
common::render_with_insert_anchor(&cases.join("\n"), InsertAnchor::Heading).unwrap().body;
|
|
|
|
insta::assert_snapshot!(body);
|
2022-02-25 16:13:29 +00:00
|
|
|
}
|
2018-11-16 17:19:38 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
#[test]
|
|
|
|
fn can_customise_anchor_template() {
|
|
|
|
let mut tera = Tera::default();
|
|
|
|
tera.extend(&ZOLA_TERA).unwrap();
|
|
|
|
tera.add_raw_template("anchor-link.html", " (in {{ lang }})").unwrap();
|
|
|
|
let permalinks_ctx = HashMap::new();
|
2021-06-02 19:46:19 +00:00
|
|
|
let config = Config::default_for_test();
|
2022-02-25 16:13:29 +00:00
|
|
|
let context = RenderContext::new(
|
2021-03-13 20:04:01 +00:00
|
|
|
&tera,
|
|
|
|
&config,
|
|
|
|
&config.default_language,
|
|
|
|
"",
|
|
|
|
&permalinks_ctx,
|
2022-02-25 16:13:29 +00:00
|
|
|
InsertAnchor::Right,
|
2021-03-13 20:04:01 +00:00
|
|
|
);
|
2022-02-25 16:13:29 +00:00
|
|
|
let body = render_content("# Hello", &context).unwrap().body;
|
|
|
|
insta::assert_snapshot!(body);
|
|
|
|
}
|
2018-11-16 17:19:38 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
#[test]
|
|
|
|
fn can_use_smart_punctuation() {
|
|
|
|
let mut config = Config::default_for_test();
|
|
|
|
config.markdown.smart_punctuation = true;
|
|
|
|
let body = common::render_with_config(r#"This -- is "it"..."#, config).unwrap().body;
|
|
|
|
insta::assert_snapshot!(body);
|
2018-11-16 17:19:38 +00:00
|
|
|
}
|
2019-01-10 16:17:07 +00:00
|
|
|
|
2020-07-29 18:20:43 +00:00
|
|
|
#[test]
|
2022-02-25 16:13:29 +00:00
|
|
|
fn can_use_external_links_options() {
|
|
|
|
let mut config = Config::default_for_test();
|
2020-07-29 18:20:43 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
// no options
|
|
|
|
let body = common::render("<https://google.com>").unwrap().body;
|
|
|
|
insta::assert_snapshot!(body);
|
2020-07-29 18:20:43 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
// target blank
|
|
|
|
config.markdown.external_links_target_blank = true;
|
|
|
|
let body = common::render_with_config("<https://google.com>", config.clone()).unwrap().body;
|
|
|
|
insta::assert_snapshot!(body);
|
2020-07-29 18:20:43 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
// no follow
|
|
|
|
config.markdown.external_links_target_blank = false;
|
|
|
|
config.markdown.external_links_no_follow = true;
|
|
|
|
let body = common::render_with_config("<https://google.com>", config.clone()).unwrap().body;
|
|
|
|
insta::assert_snapshot!(body);
|
2020-07-29 18:20:43 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
// no referrer
|
|
|
|
config.markdown.external_links_no_follow = false;
|
|
|
|
config.markdown.external_links_no_referrer = true;
|
|
|
|
let body = common::render_with_config("<https://google.com>", config.clone()).unwrap().body;
|
|
|
|
insta::assert_snapshot!(body);
|
2020-07-29 18:20:43 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
// all of them
|
|
|
|
config.markdown.external_links_no_follow = true;
|
|
|
|
config.markdown.external_links_target_blank = true;
|
|
|
|
config.markdown.external_links_no_referrer = true;
|
|
|
|
let body = common::render_with_config("<https://google.com>", config).unwrap().body;
|
|
|
|
insta::assert_snapshot!(body);
|
2020-07-29 18:20:43 +00:00
|
|
|
}
|
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
#[test]
|
|
|
|
fn can_render_emojis() {
|
|
|
|
let mut config = Config::default_for_test();
|
|
|
|
config.markdown.render_emoji = true;
|
|
|
|
let body = common::render_with_config("Hello, World! :smile:", config).unwrap().body;
|
|
|
|
assert_eq!(body, "<p>Hello, World! 😄</p>\n");
|
|
|
|
}
|
2019-07-15 18:18:24 +00:00
|
|
|
|
|
|
|
// https://github.com/getzola/zola/issues/747
|
2019-10-10 18:23:16 +00:00
|
|
|
// https://github.com/getzola/zola/issues/816
|
2019-07-15 18:18:24 +00:00
|
|
|
#[test]
|
2022-02-25 16:13:29 +00:00
|
|
|
fn custom_url_schemes_are_untouched() {
|
|
|
|
let body = common::render(
|
|
|
|
r#"
|
|
|
|
[foo@bar.tld](xmpp:foo@bar.tld)
|
2019-10-10 18:23:16 +00:00
|
|
|
|
|
|
|
[(123) 456-7890](tel:+11234567890)
|
|
|
|
|
|
|
|
[blank page](about:blank)
|
2022-02-25 16:13:29 +00:00
|
|
|
"#,
|
|
|
|
)
|
|
|
|
.unwrap()
|
|
|
|
.body;
|
|
|
|
insta::assert_snapshot!(body);
|
|
|
|
}
|
2019-10-10 18:23:16 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
#[test]
|
|
|
|
fn all_markdown_features_integration() {
|
|
|
|
let body = common::render(
|
|
|
|
r#"
|
|
|
|
<!-- Adapted from https://markdown-it.github.io/ -->
|
2019-10-10 18:23:16 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
# h1 Heading
|
2019-10-10 18:23:16 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
## h2 Heading
|
2019-10-10 18:23:16 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
### h3 Heading
|
2019-10-10 18:23:16 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
#### h4 Heading
|
2020-01-11 09:34:03 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
##### h5 Heading
|
2020-01-11 09:34:03 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
###### h6 Heading
|
2020-01-11 09:34:03 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
## Horizontal Rules
|
2020-01-11 09:34:03 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
___
|
2020-01-11 09:34:03 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
---
|
2020-01-11 09:34:03 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
***
|
2020-07-29 18:20:43 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
## Emphasis
|
2020-07-29 18:20:43 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
**This is bold text**
|
2020-07-29 18:20:43 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
__This is bold text__
|
2020-07-29 18:20:43 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
*This is italic text*
|
2020-07-29 18:20:43 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
_This is italic text_
|
2020-07-29 18:20:43 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
~~Strikethrough~~
|
2020-07-29 18:20:43 +00:00
|
|
|
|
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
## Blockquotes
|
2020-07-29 18:20:43 +00:00
|
|
|
|
2020-09-22 20:11:42 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
> Blockquotes can also be nested...
|
|
|
|
>> ...by using additional greater-than signs right next to each other...
|
|
|
|
> > > ...or with spaces between arrows.
|
2020-09-22 20:11:42 +00:00
|
|
|
|
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
## Lists
|
2020-09-22 20:11:42 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
Unordered
|
2020-09-22 20:11:42 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
+ Create a list by starting a line with `+`, `-`, or `*`
|
|
|
|
+ Sub-lists are made by indenting 2 spaces:
|
|
|
|
- Marker character change forces new list start:
|
|
|
|
* Ac tristique libero volutpat at
|
|
|
|
+ Facilisis in pretium nisl aliquet
|
|
|
|
- Nulla volutpat aliquam velit
|
|
|
|
+ Very easy!
|
2020-09-22 20:11:42 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
Ordered
|
2020-09-22 20:11:42 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
1. Lorem ipsum dolor sit amet
|
|
|
|
2. Consectetur adipiscing elit
|
|
|
|
3. Integer molestie lorem at massa
|
2020-09-22 20:11:42 +00:00
|
|
|
|
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
1. You can use sequential numbers...
|
|
|
|
1. ...or keep all the numbers as `1.`
|
2020-09-22 20:11:42 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
Start numbering with offset:
|
2020-09-22 20:11:42 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
57. foo
|
|
|
|
1. bar
|
2020-09-22 20:11:42 +00:00
|
|
|
|
2020-09-23 17:21:21 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
## Code
|
2020-09-23 17:21:21 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
Inline `code`
|
2020-09-23 17:21:21 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
Indented code
|
2020-09-23 17:21:21 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
// Some comments
|
|
|
|
line 1 of code
|
|
|
|
line 2 of code
|
|
|
|
line 3 of code
|
2020-09-23 17:21:21 +00:00
|
|
|
|
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
Block code "fences"
|
2020-09-23 17:21:21 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
```
|
|
|
|
Sample text here...
|
|
|
|
```
|
2020-10-30 16:02:07 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
Syntax highlighting
|
2020-10-30 16:02:07 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
``` js
|
|
|
|
var foo = function (bar) {
|
|
|
|
return bar++;
|
|
|
|
};
|
2020-11-21 21:20:54 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
console.log(foo(5));
|
|
|
|
```
|
2020-12-14 15:29:10 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
## Shortcodes
|
2020-12-14 15:29:10 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
## Tables
|
2020-12-14 15:29:10 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
| Option | Description |
|
|
|
|
| ------ | ----------- |
|
|
|
|
| data | path to data files to supply the data that will be passed into templates. |
|
|
|
|
| engine | engine to be used for processing templates. Handlebars is the default. |
|
|
|
|
| ext | extension to be used for dest files. |
|
2020-12-14 15:29:10 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
Right aligned columns
|
2020-12-14 15:29:10 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
| Option | Description |
|
|
|
|
| ------:| -----------:|
|
|
|
|
| data | path to data files to supply the data that will be passed into templates. |
|
|
|
|
| engine | engine to be used for processing templates. Handlebars is the default. |
|
|
|
|
| ext | extension to be used for dest files. |
|
2020-12-14 15:29:10 +00:00
|
|
|
|
2020-11-21 21:20:54 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
## Links
|
2020-11-21 21:20:54 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
[link text](http://duckduckgo.com)
|
2020-11-21 21:20:54 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
[link with title](http://duckduckgo.com/ "Duck duck go")
|
2020-11-21 21:20:54 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
## Images
|
2020-11-27 10:11:19 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
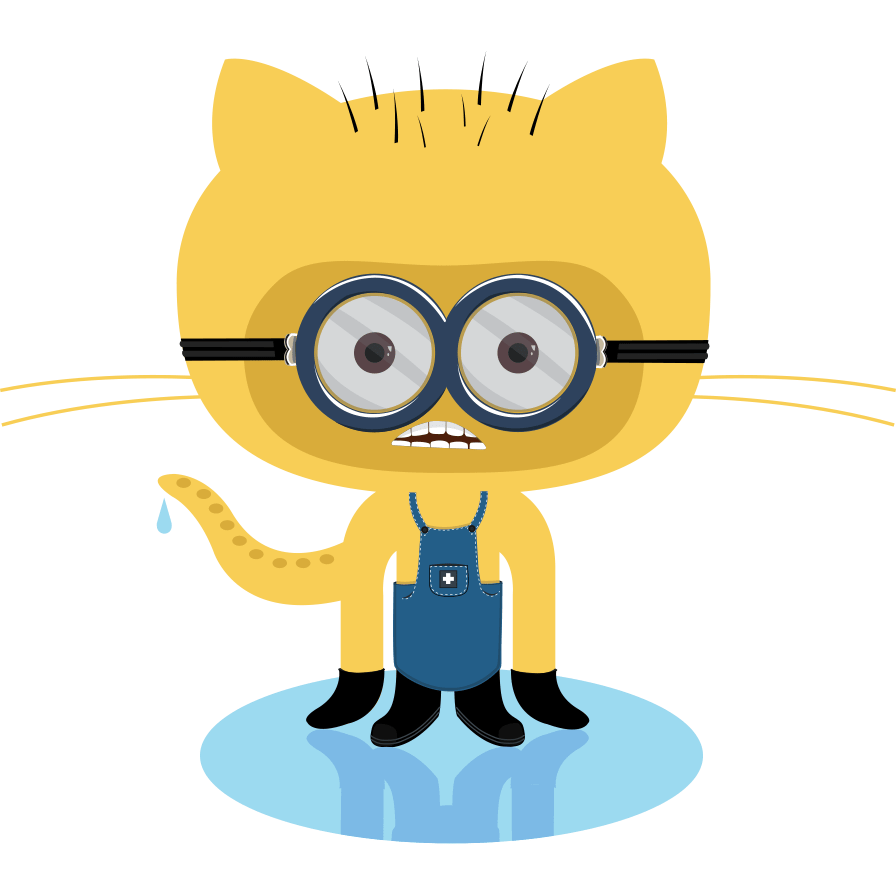
|
|
|
|

|
2021-12-07 19:22:34 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
Like links, Images also have a footnote style syntax
|
2021-12-07 19:22:34 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
![Alt text][id]
|
2021-12-07 19:22:34 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
With a reference later in the document defining the URL location:
|
2021-12-07 19:22:34 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
[id]: https://octodex.github.com/images/dojocat.jpg "The Dojocat"
|
2021-12-07 19:22:34 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
## Smileys
|
2021-12-09 20:00:48 +00:00
|
|
|
|
2022-02-25 16:13:29 +00:00
|
|
|
Like :smile:, :cry:
|
|
|
|
|
|
|
|
### Footnotes
|
|
|
|
|
|
|
|
Footnote 1 link[^first].
|
|
|
|
|
|
|
|
Footnote 2 link[^second].
|
|
|
|
|
|
|
|
Duplicated footnote reference[^second].
|
|
|
|
|
|
|
|
[^first]: Footnote **can have markup**
|
|
|
|
and multiple paragraphs.
|
|
|
|
|
|
|
|
[^second]: Footnote text.
|
|
|
|
"#,
|
|
|
|
)
|
|
|
|
.unwrap()
|
|
|
|
.body;
|
|
|
|
insta::assert_snapshot!(body);
|
2021-12-09 20:00:48 +00:00
|
|
|
}
|
2024-05-09 13:45:47 +00:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn github_style_footnotes() {
|
|
|
|
let mut config = Config::default_for_test();
|
|
|
|
config.markdown.bottom_footnotes = true;
|
|
|
|
|
|
|
|
let markdown = r#"This text has a footnote[^1]
|
|
|
|
|
|
|
|
[^1]:But it is meaningless.
|
|
|
|
|
|
|
|
This text has two[^3] footnotes[^2].
|
|
|
|
|
|
|
|
[^2]: not sorted.
|
|
|
|
[^3]: But they are
|
|
|
|
|
|
|
|
[^4]:It's before the reference.
|
|
|
|
|
|
|
|
There is footnote definition?[^4]
|
|
|
|
|
|
|
|
This text has two[^5] identical footnotes[^5]
|
|
|
|
[^5]: So one is present.
|
|
|
|
[^6]: But another in not.
|
|
|
|
|
|
|
|
This text has a footnote[^7]
|
|
|
|
|
|
|
|
[^7]: But the footnote has another footnote[^8].
|
|
|
|
|
|
|
|
[^8]: That's it.
|
|
|
|
|
|
|
|
Footnotes can also be referenced with identifiers[^first].
|
|
|
|
|
|
|
|
[^first]: Like this: `[^first]`.
|
|
|
|
"#;
|
|
|
|
|
|
|
|
let body = common::render_with_config(&markdown, config).unwrap().body;
|
|
|
|
insta::assert_snapshot!(body);
|
|
|
|
}
|