`For` example should be used instead `while` in WHILE_LET_ON_ITERATOR
For example should be used instead while in WHILE_LET_ON_ITERATOR
Revert some changes
Fix cargo dev fmt
feat(lint): add default_iter_empty
close#8915
This PR adds `default_iter_empty` lint.
This lint checks `std::iter::Empty::default()` and replace with `std::iter::empty()`.
Thank you in advance.
---
changelog: add `default_instead_of_iter_empty` lint.
Update description in clippy_lints/src/default_iter_empty.rs
Co-authored-by: Fridtjof Stoldt <xFrednet@gmail.com>
Update clippy_lints/src/default_iter_empty.rs
Co-authored-by: Alex Macleod <alex@macleod.io>
Update clippy_lints/src/default_iter_empty.rs
Co-authored-by: Alex Macleod <alex@macleod.io>
renamed default_iter_empty to default_instead_of_iter_empty
Avoid duplicate messages
add tests for regression
rewrite 'Why is this bad?'
cargo dev fmt
delete default_iter_empty lint in renamed_lint.rs
rewrite a message in the suggestion
cargo dev update_lints --check
Make `ExprKind::Closure` a struct variant.
Simple refactor since we both need it to introduce additional fields in `ExprKind::Closure`.
r? ``@Aaron1011``
Rework `branches_sharing_code`
fixes#7378
This changes the lint from checking pairs of blocks, to checking all the blocks at the same time. As such there's almost none of the original code left.
changelog: Don't lint `branches_sharing_code` when using different binding names
And likewise for the `Const::val` method.
Because its type is called `ConstKind`. Also `val` is a confusing name
because `ConstKind` is an enum with seven variants, one of which is
called `Value`. Also, this gives consistency with `TyS` and `PredicateS`
which have `kind` fields.
The commit also renames a few `Const` variables from `val` to `c`, to
avoid confusion with the `ConstKind::Value` variant.
Fix some `#[expect]` lint interaction
Fixing the first few lints that aren't caught by `#[expect]`. The root cause of these examples was, that the lint was emitted at the wrong location.
---
changelog: none
r? `@Jarcho`
cc: rust-lang/rust#97660
Improve lint doc consistency
changelog: none
This is a continuation of #8908.
Notable changes:
- Removed empty `Known Problems` sections
- Removed "Good"/"Bad" language (replaced with "Use instead")
- Removed (and added some 😄) duplication
- Ignored the [`create_dir`] example so it doesn't create `clippy_lints/foo` 😄
fix(lint): check const context
close: https://github.com/rust-lang/rust-clippy/issues/8898
This PR fixes a bug in checked_conversions.
Thank you in advance.
changelog: check const context in checked_conversions.
This commit makes type folding more like the way chalk does it.
Currently, `TypeFoldable` has `fold_with` and `super_fold_with` methods.
- `fold_with` is the standard entry point, and defaults to calling
`super_fold_with`.
- `super_fold_with` does the actual work of traversing a type.
- For a few types of interest (`Ty`, `Region`, etc.) `fold_with` instead
calls into a `TypeFolder`, which can then call back into
`super_fold_with`.
With the new approach, `TypeFoldable` has `fold_with` and
`TypeSuperFoldable` has `super_fold_with`.
- `fold_with` is still the standard entry point, *and* it does the
actual work of traversing a type, for all types except types of
interest.
- `super_fold_with` is only implemented for the types of interest.
Benefits of the new model.
- I find it easier to understand. The distinction between types of
interest and other types is clearer, and `super_fold_with` doesn't
exist for most types.
- With the current model is easy to get confused and implement a
`super_fold_with` method that should be left defaulted. (Some of the
precursor commits fixed such cases.)
- With the current model it's easy to call `super_fold_with` within
`TypeFolder` impls where `fold_with` should be called. The new
approach makes this mistake impossible, and this commit fixes a number
of such cases.
- It's potentially faster, because it avoids the `fold_with` ->
`super_fold_with` call in all cases except types of interest. A lot of
the time the compile would inline those away, but not necessarily
always.
* Don't lint on `.cloned().flatten()` when `T::Item` doesn't implement `IntoIterator`
* Reduce verbosity of lint message
* Narrow down the scope of the replacement range
List configuration values can now be extended instead of replaced
I've seen some `clippy.toml` files, that have a few additions to the default list of a configuration and then a copy of our default. The list will therefore not be updated, when we add new names. This change should make it simple for new users to append values instead of replacing them.
I'm uncertain if the documentation of the `".."` is apparent. Any suggestions are welcome. I've also check that the lint list displays the examples correctly.
<details>
<summary>Lint list screenshots</summary>
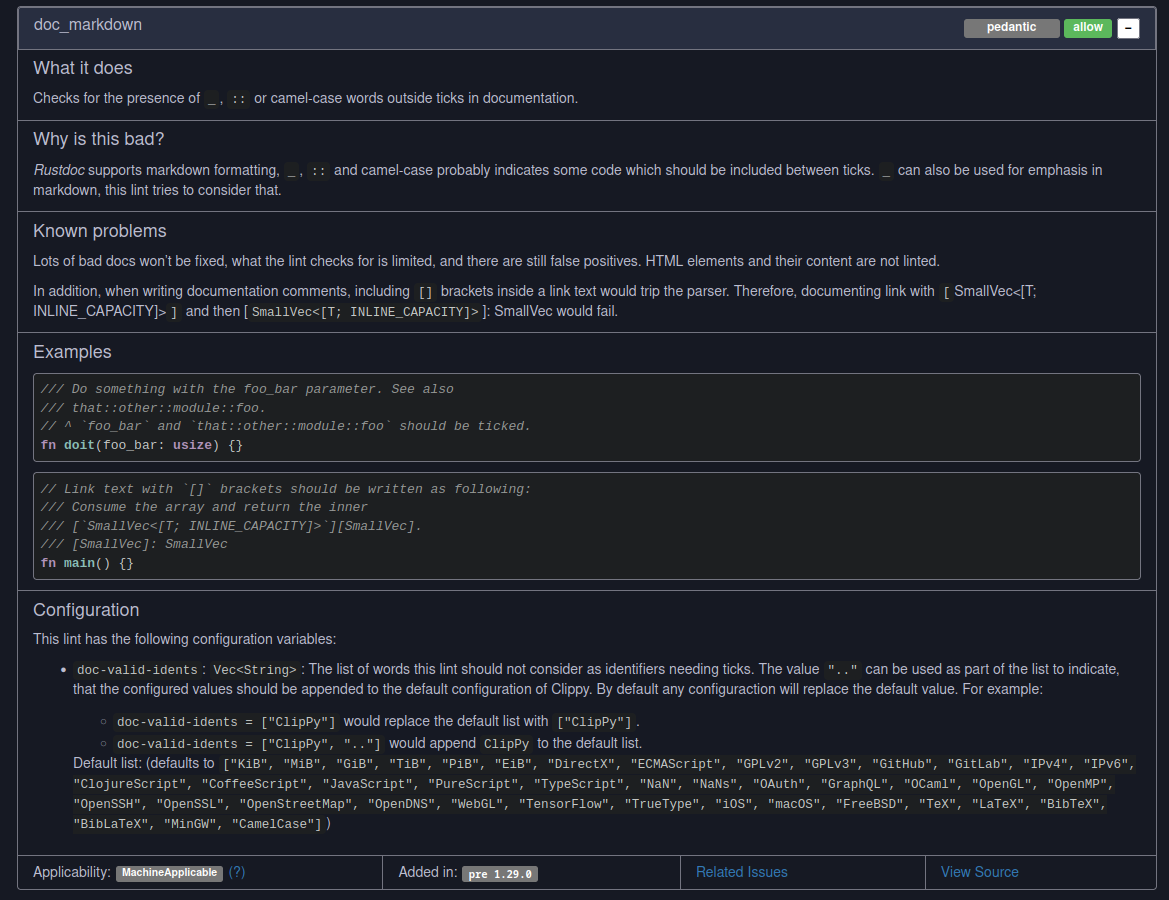
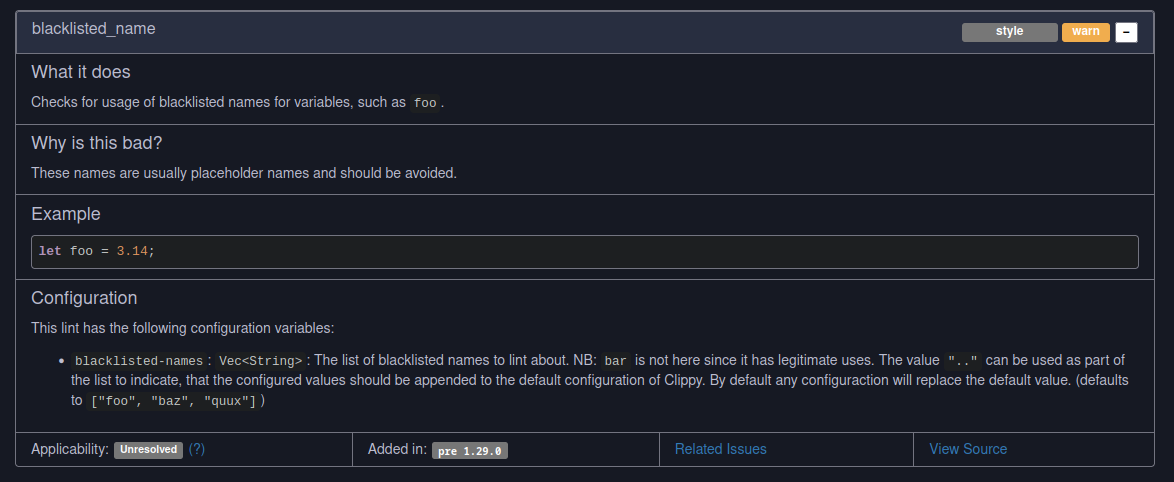
</details>
---
changelog: enhancement: [`doc_markdown`]: Users can now indicate, that the `doc-valid-idents` should extend the default and not replace it
changelog: enhancement: [`blacklisted-name`]: Users can now indicate, that the `blacklisted-names` should extend the default and not replace it
Closes: #8877
That's it. Have a fantastic weekend to everyone reading this. Here is a cookie 🍪
Add new lint [`needless_parens_on_range_literals`]
changelog: Adds a new lint [`needless_parens_on_range_literals`] to warn on needless braces on literals in a range statement
For example, the lint would catch
```log
error: needless parenthesis on range literals can be removed
--> $DIR/needless_parens_on_range_literals.rs:8:13
|
LL | let _ = ('a')..=('z');
| ^^^^^ help: try: `'a'`
|
= note: `-D clippy::needless-parens-on-range-literals` implied by `-D warnings`
```
improve [`for_loops_over_fallibles`] to detect the usage of iter, iter_mut and into_iterator
fix#6762
detects code like
```rust
for _ in option.iter() {
//..
}
```
changelog: Improve [`for_loops_over_fallibles`] to detect `for _ in option.iter() {}` or using `iter_mut()` or `into_iterator()`.
Remove the unneeded wrapping and unwrapping in suggestion creation.
Collecting to Option<Vec<_>> only returns None if one of the elements is
None and that is never the case here.
fix(manual_find_map and manual_filter_map): check clone method
close#8920
Added conditional branching when the clone method is used.
Thank you in advance.
---
changelog: check `clone()` and other variant preserving methods in [`manual_find_map`] and [`manual_filter_map`]