New assist: move_format_string_arg
The name might need some improving.
```rust
fn main() {
print!("{x + 1}");
}
```
to
```rust
fn main() {
print!("{}"$0, x + 1);
}
```
fixes#13180
ref to #5988 for similar work
* extracted `format_like`'s parser to it's own module in `ide-db`
* reworked the parser's API to be more direct
* added assist to extract expressions in format args
fix: handle lifetime variables in projection normalization
Fixes#12674
The problem is that we've been skipping the binders of normalized projections assuming they should be empty, but the assumption is unfortunately wrong. We may get back lifetime variables and should handle them before returning them as normalized projections. For those who are curious why we get those even though we treat all lifetimes as 'static, [this comment in chalk](d875af0ff1/chalk-solve/src/infer/unify.rs (L888-L908)) may be interesting.
I thought using `InferenceTable` would be cleaner than the other ways as it already has the methods for canonicalization, normalizing projection, and resolving variables, so moved goal building and trait solving logic to a new `HirDatabase` query. I made it transparent query as the query itself doesn't do much work but the eventual call to `HirDatabase::trait_solve_query()` does.
fix: handle trait methods as inherent methods for trait-related types
Fixes#10677
When resolving methods for trait object types and placeholder types that are bounded by traits, we need to count the methods of the trait and its super traits as inherent methods. This matters because these trait methods have higher priority than the other traits' methods.
Relevant code in rustc: [`assemble_inherent_candidates_from_object()`](0631ea5d73/compiler/rustc_typeck/src/check/method/probe.rs (L783-L792)) for trait object types, [`assemble_inherent_candidates_from_param()`](0631ea5d73/compiler/rustc_typeck/src/check/method/probe.rs (L838-L847)) for placeholder types. Notice the second arg of `push_candidate()` is `is_inherent`.
Previously, annotations would only appear above the name of an item (function signature, struct declaration, etc).
Now, rust-analyzer can be configured to show annotations either above the name or above the whole item (including doc comments and attributes).
The name might need some improving.
extract format_like's parser to it's own module in ide-db
reworked the parser's API to be more direct
added assist to extract expressions in format args
fix: sort all bounds on trait object types
Fixes#13181#12793 allowed different ordering of trait bounds in trait object types but failed to account for the ordering of projection bounds. I opted for sorting all the bounds at once rather than splitting them into `SmallVec`s so it's easier to do the same thing for other bounds when we have them.
fix: Insert whitespaces into static & const bodies if they are expanded from macro on hover
Partially fixes#13143.
To resolve the other part we need to expand macros in unevaluated static & const bodies, and I'm not sure we want to. If for example it includes a call to `assert!()`, expanding it will lead to worse hover.
It seems that we've accidentally deleted the tests here couple of years
ago, and then fairly recently made a typo during refactor as well.
Reinstall tests, with coverage marks this time :-)
A Resolver *always* has a module scope at the end of its scope stack,
instead of encoding this as an invariant we can just lift this scope
out into a field, allowing us to skip going through the scope vec
indirection entirely.
feat: Implement `feature(exhaustive_patterns)` from unstable Rust
Closes#12753
Recognize Rust's unstable `#![feature(exhaustive_patterns)]` (RFC 1872). Allow omitting visibly uninhabited variants from `match` expressions when the feature is on.
This adjusts match checking to the current implementation of the postponed RFC 1872 in rustc.
fix: Parse TypePathFn with preceding `::`
e.g. `impl Fn::() -> ()`.
Fixes#13157. This was the problem, not that the path was not at the end.
I could unify the parsing of `::` of TypePathFn with that of generic arg list, but some code relies on the `::` of generic arg list to be inside it.
fix: Lower float literals with underscores
Fixes#13155 (the problem was the `PI` is defined with `_f64` suffix). `PI` is still truncated, though, because `f64` cannot represent the value with full precision.
Highlight namerefs by syntax until proc-macros have been loaded
Usually when loading up a project, once loading is done we start answering highlight requests while proc-macros haven't always been loaded yet, so we start out with showing a lot of unresolved name-refs. After this PR, we'll use syntax based highlighting for those unresolved namerefs until the proc-macros have been loaded.
Remove hir::Expr::MacroStmts
This hir expression isn't needed and only existed as it was simpler to
deal with at first as it gave us a direct mapping for the ast version of
the same construct. This PR removes it, properly handling the statements
that are introduced by macro call expressions.
This hir expression isn't needed and only existed as it was simpler to
deal with at first as it gave us a direct mapping for the ast version of
the same construct. This PR removes it, properly handling the statements
that are introduced by macro call expressions.
feature: Assist to turn match into matches! invocation
Resolves#12510
This PR adds an assist, which convert 2-arm match that evaluates to a boolean into the equivalent matches! invocation.
fix: Only move comments when extracting a struct from an enum variant
Motivating example:
```rs
#[derive(Debug, thiserror::Error)]
enum Error {
/// Some explanation for this error
#[error("message")]
$0Woops {
code: u32
}
}
```
now becomes
```rs
/// Some explanation for this error
#[derive(Debug, thiserror::Error)]
struct Woops{
code: u32
}
#[derive(Debug, thiserror::Error)]
enum Error {
#[error("message")]
Woops(Woops)
}
```
(the `thiserror::Error` derive being copied and the struct formatting aren't ideal, though those are issues for another day)
fix: sort and deduplicate auto traits in trait object types
Fixes#12739
Chalk solver doesn't sort and deduplicate auto traits in trait object types, so we need to handle them ourselves in the lowering phase, just like [`rustc`](880416180b/compiler/rustc_typeck/src/astconv/mod.rs (L1487-L1488)) and [`chalk-integration`](https://github.com/rust-lang/chalk/blob/master/chalk-integration/src/lowering.rs#L575) do.
Quoting from [the Chalk book](https://rust-lang.github.io/chalk/book/types/rust_types.html#dyn-types):
> Note that -- for this purpose -- ordering of bounds is significant. That means that if you create a `dyn Foo + Send` and a `dyn Send + Foo`, chalk would consider them distinct types. The assumption is that bounds are ordered in some canonical fashion somewhere else.
Also, trait object types with more than one non-auto traits were previously allowed, but are now disallowed with this patch.
Use correct type in "Replace turbofish with type"
And support `?` and `.await` expressions.
Fixes#13148.
The assist can still show up even if the turbofish's type is not used at all, e.g.:
```rust
fn foo<T>() {}
let v = foo::<i32>();
```
I implemented that by checking the expressions' type.
This could probably be implemented better by taking the function's return type and substituting the generic parameter with the provided turbofish, but this is more complicated.
feat: Add a "Unmerge match arm" assist to split or-patterns inside match expressions
Fixes#13072.
The way I implemented it it leaves the `OrPat` in place even if there is only one pattern now but I don't think something will break because of that, and when more code will be typed we'll parse it again anyway. Removing it (but keeping the child pattern) is hard, I don't know how to do that.
Move empty diagnostics workaround back into the server
This only touches on the diagnostics in one place instead of multiple as was previously done, since all published diagnostics will go through this code path anyways.
Closes https://github.com/rust-lang/rust-analyzer/issues/13130
Make use of NoHash hashing for FileId and CrateId
Both of these are mere integers so there is nothing to hash here.
Ideally we would use this for `la_arena::Idx` too, but that doesn't work due to the orphan rule, and `la_arena` is unfortunately a public library so we can't really do much here... Unless we remove the trait restriction but I'd like not to
internal: Re-export standard semantic token types and mods
Should help in preventing future occurences of #13099 by having all token types and mods come through the same place
Add some more highlighting configurations
The following can be enabled/disabled now in terms of highlighting:
- doc comment injection (enabled by default)
- punctuation highlighting (disabled by default)
- operator highlighting (enabled by default)
- punctuation specialized highlighting (disabled by default)
- operator specialized highlighting (disabled by default)
- macro call bang highlighting (disabled by default)
This PR also changes our `attribute` semantic token type to the `decorator` type which landed upstream (but not yet in lsp-types).
Specialized highlighting is disabled by default, as all clients will have to ship something to map these back to the standard punctuation/operator token (we do this in VSCode via the inheritance mapping for example). This is a lot of maintenance work, and not something every client wants to do, pushing that need to use the user. As this is a rather niche use in the first place this will just be disabled by default.
Punctuation highlighting is disabled by default, punctuation is usually something that can be done by the native syntactic highlighting of the client, so there is no loss in quality. The main reason for this though is that punctuation adds a lot of extra token data that we sent over, a lot of clients struggle with applying this, so disabling this improves the UX for a lot of people. Note that we still highlight punctuations with special meaning as that special entity, (the never type `!` will still be tagged as a builtin type if it occurs as such)
Separate highlighting of the macro call bang `!` is disabled by default, as I think people actually didn't like that change that much, though at the same time I feel like not many people even noticed that change (I prefer it be separate, but that's not enough reason for it to be enabled by default I believe :^) )
cc https://github.com/rust-lang/rust-analyzer/issues/12783https://github.com/rust-lang/rust-analyzer/issues/13066
fix: Fix panics on GATs involving const generics
This workaround avoids constant crashing of rust analyzer when using GATs with const generics,
even when the const generics are only on the `impl` block.
The workaround treats GATs as non-existing if either itself or the parent has const generics and
removes relevant panicking code-paths.
Fixes#11989, fixes#12193
feat: Generate static method using Self::assoc() syntax
This change improves the `generate_function` assist to support generating static methods/associated functions using the `Self::assoc()` syntax. Previously, one could generate a static method, but only when specifying the type name directly (like `Foo::assoc()`). After this change, `Self` is supported as well as the type name.
Fixes#13012
feat: Add an assist for inlining all type alias uses
## Description
`inline_type_alias_uses` assist tries to inline all selected type alias occurrences.
### Currently
Type alias used in `PathType` position are inlined.
### Not supported
- Removing type alias declaration if all uses are inlined.
- Removing redundant imports after inlining all uses in the file.
- Type alias not in `PathType` position, such as:
- `A::new()`
- `let x = A {}`
- `let bits = A::BITS`
- etc.
## Demonstration
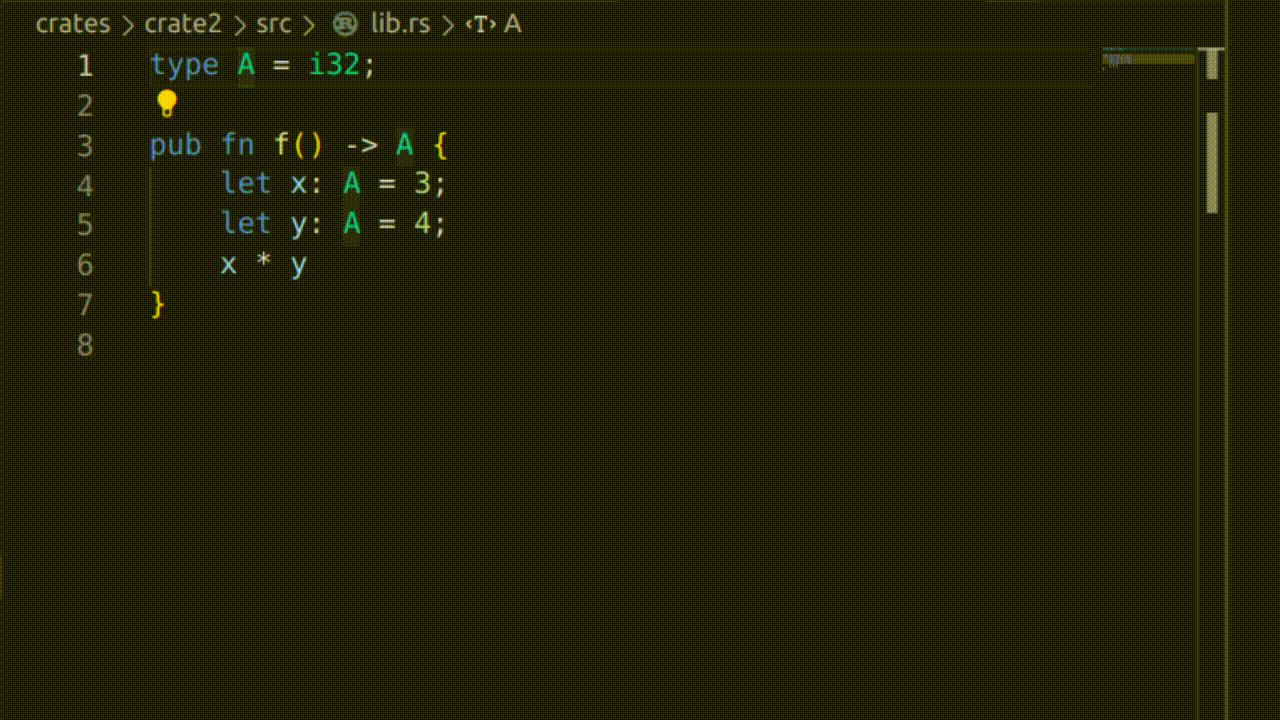
## Related Issues
Partially fixes#10881
feat: Run test mod from anywhere in parent file
The "Run" feature of rust-analyzer is super useful, especially for running
individual tests or test-modules during development.
One common pattern in rust development is to develop tests in the same file as
production code, inside a module (usually called `test` or `tests`) marked with
`#[cfg(test)]`. Unforunately, this pattern is not well supported by r-a today,
as a test module won't show up as a runnable unless the cursor is inside it.
In my experience, it is quite common to want to run the tests associated with
some production code immediately after editing it, not only after editing the
tests themselves. As such it would be better if test modules were available
from the "Run" menu even when the cursor is outside the test module.
This change updates the filtration logic for runnables in
`handlers::handle_runnables` to special case `RunnableKind::TestMod`, making
test modules available regardless of the cursor location. Other `RunnableKind`s
are unnaffected.
Fixes#9589
fix: resolve associated types of bare dyn types
Fixes#13031
We've been dropping the associated type bindings of trait object types that were written without the `dyn` keyword. This patch reuses the lowering logic for `TypeRef::DynTrait` so the associated type bindings are properly lowered.
The "Run" feature of rust-analyzer is super useful, especially for running
individual tests or test-modules during development.
One common pattern in rust development is to develop tests in the same file as
production code, inside a module (usually called `test` or `tests`) marked with
`#[cfg(test)]`. Unforunately, this pattern is not well supported by r-a today,
as a test module won't show up as a runnable unless the cursor is inside it.
In my experience, it is quite common to want to run the tests associated with
some production code immediately after editing it, not only after editing the
tests themselves. As such it would be better if test modules were available
from the "Run" menu even when the cursor is outside the test module.
This change updates the filtration logic for runnables in
`handlers::handle_runnables` to special case `RunnableKind::TestMod`, making
test modules available regardless of the cursor location. Other `RunnableKind`s
are unnaffected.
Fixes#9589
fix: a bunch of typos
This PR will fix some typos detected by [typos].
There are also some other typos in the function names, variable names, and file
names, which I leave as they are. I'm more certain that typos in comments
should be fixed.
[typos]: https://github.com/crate-ci/typos
This PR will fix some typos detected by [typos].
There are also some other typos in the function names, variable names, and file
names, which I leave as they are. I'm more certain that typos in comments
should be fixed.
[typos]: https://github.com/crate-ci/typos
fix: escape keywords used as names in earlier editions
Fixes#13030
There are keywords in Rust 2018+ that you can use as names without escaping when your crate is in Rust 2015 e.g. "try". We need to be consistent on how to keep track of the names regardless of how they are actually written in each crate. This patch attempts at it by taking such names into account and storing them uniformly in their escaped form.
This change improves the `generate_function` assist to support generating static methods/associated functions using the `Self::assoc()` syntax. Previously, one could generate a static method, but only when specifying the type name directly (like `Foo::assoc()`). After this change, `Self` is supported as well as the type name.
Fixes#13012
This workaround avoids constant crashing of rust analyzer when using GATs with const generics,
even when the const generics are only on the `impl` block.
The workaround treats GATs as non-existing if either itself or the parent has const generics and
removes relevant panicking code-paths.
fix: Fix incorrect type mismatch with `cfg_if!` and other macros in expression position
Fixes https://github.com/rust-lang/rust-analyzer/issues/12940
This is a bit of a hack, ideally `MacroStmts` would not exist at all after HIR lowering, but that requires changing how the lowering code works.
rust-analyzer's RUSTC_WRAPPER unconditionally succeeds `cargo check`
invocations tripping up build scripts using `cargo check` to probe for
successful compilations. To prevent this from happening the RUSTC_WRAPPER
now checks if it's run from a build script by looking for the
`CARGO_CFG_TARGET_ARCH` env var that cargo sets only when running build
scripts.
Make `Name` hold escaped name
Resolves#12787Resolvesrust-lang/rust#99361
This PR effectively swaps `Name` and `EscapedName` in hir. In other words, it makes `Name` hold and print escaped raw identifiers and introduces another struct `UnescapedName` for cases where you need to print names without "r#" prefix.
My rationale is that it makes it easier for us to format an escaped name into string, which is what we want when we serialize names in general. This is because we format a name into string usually when we are presenting it to the users and arguably they expect its escaped form as that's what they see and write in the source code.
I split the change for `Name` into 3 commits to make it easier to follow but it also made some tests fail in the intermediate commits. I can squash them into a commit after the review if desired. I've also made similar changes for `ModPath` and `EscapedModPath` as it makes them consistent with `Name`.
For reference, there was a brief discussion on this in [a zulip thread](https://rust-lang.zulipchat.com/#narrow/stream/185405-t-compiler.2Frust-analyzer/topic/escaping.20.60Name.60s).
internal: Remove incomplete 1.64 ABI
This no longer works for current nightlies and should not be needed any more, since we can use the toolchain's proc macro server instead.
Parse range patterns in struct and slice without trailing comma
Resolves#12935
This patch includes the support for range patterns in slices, which is unstable (tracked in https://github.com/rust-lang/rust/issues/67264). If it's not desired I can remove it.
Add fixups for incomplete in proc-macros
Partially implements https://github.com/rust-lang/rust-analyzer/issues/12777.
Added support for for loops and match statements.
I couldn't do paths like `crate::foo::` as I wasn't able to add `SyntheticTokens` to the end of `foo::`, they always ended up after `crate::`
This is my first contribution so please don't be shy about letting me know if I've done anything wrong!
fix: make `concat!` work with char
Fixes#12921
- I avoided making `unquote_str()` take char literals as well because it's depended on by another function `parse_string()` that's only supposed to take strings.
- Even with this patch, we don't output `\0` as `\u{0}` which #12921 pointed out ~~, but we're not actually responsible for serializing it but rowan is~~. They are functionally equivalent and I don't think it'd cause any confusion, but we *could* try escaping them before serialization (for reference, `rustc -Zunpretty=expanded`, which `cargo expand` uses under the hood, [makes use of `str::escape_default()`](3830ecaa8d/compiler/rustc_ast/src/util/literal.rs (L161)).