10603: fix: Don't resolve attributes to non attribute macros r=Veykril a=Veykril
Also changes `const`s to `static`s for `Limit`s as we have interior mutability in those(though only used with a certain feature flag enabled).
Co-authored-by: Lukas Wirth <lukastw97@gmail.com>
10563: feat: Make "Generate getter" assist use semantic info r=agluszak a=agluszak
This PR makes "Generate getter" assist use semantic info instead of dealing with types encoded as strings.
Getters for types which are:
- `Copy` no longer return references
- `AsRef<str>` (i.e. `String`) return `&str` (instead of `&String`)
- `AsRef<[T]>` (i.e. `Vec<T>`) return `&[T]` (instead of `&Vec<T>`)
- `AsRef<T>` (i.e. `Box<T>`) return `&T` (instead of `&Box<T>`)
- `Option<T>` return `Option<&T>` (instead of `&Option<T>`)
- `Result<T, E>` return `Result<&T, &E>` (instead of `&Result<T, E>`)
String, Vec, Box and Option were previously handled as special cases.
Closes#10295
Co-authored-by: Andrzej Głuszak <gluszak.andrzej@gmail.com>
10387: Move `IdxRange` into la-arena r=Veykril a=arzg
Currently, `IdxRange` (named `IdRange`) is located in `hir_def::item_tree`, when really it isn’t specific to `hir_def` and could become part of la-arena. The rename from `IdRange` to `IdxRange` is to maintain consistency with the naming convention used throughout la-arena (`Idx` instead of `Id`, `RawIdx` instead of `RawId`). This PR also adds a few new APIs to la-arena on top of `IdxRange` for convenience, namely:
- indexing into an `Arena` by an `IdxRange` and getting a slice of values back
- creating an `IdxRange` from an inclusive range
Currently this PR also exposes a new `Arena::next_idx` method to make constructing inclusive`IdxRange`s using `IdxRange::new` easier; however, it would in my opinion be better to remove this as it allows for easy creation of out-of-bounds `Idx`s, when `IdxRange::new_inclusive` mostly covers the same use-case while being less error-prone.
I decided to bump the la-arena version to 0.3.0 from 0.2.0 because adding a new `Index` impl for `Arena` turned out to be a breaking change: I had to add a type hint in `crates/hir_def/src/body/scope.rs` when one wasn’t necessary before, since rustc couldn’t work out the type of a closure parameter now that there are multiple `Index` impls. I’m not sure whether this is the right decision, though.
Co-authored-by: Aramis Razzaghipour <aramisnoah@gmail.com>
10440: Fix Clippy warnings and replace some `if let`s with `match` r=Veykril a=arzg
I decided to try fixing a bunch of Clippy warnings. I am aware of this project’s opinion of Clippy (I have read both [rust-lang/clippy#5537](https://github.com/rust-lang/rust-clippy/issues/5537) and [rust-analyzer/rowan#57 (comment)](https://github.com/rust-analyzer/rowan/pull/57#discussion_r415676159)), so I totally understand if part of or the entirety of this PR is rejected. In particular, I can see how the semicolons and `if let` vs `match` commits provide comparatively little benefit when compared to the ensuing churn.
I tried to separate each kind of change into its own commit to make it easier to discard certain changes. I also only applied Clippy suggestions where I thought they provided a definite improvement to the code (apart from semicolons, which is IMO more of a formatting/consistency question than a linting question). In the end I accumulated a list of 28 Clippy lints I ignored entirely.
Sidenote: I should really have asked about this on Zulip before going through all 1,555 `if let`s in the codebase to decide which ones definitely look better as `match` :P
Co-authored-by: Aramis Razzaghipour <aramisnoah@gmail.com>
Consider these expples
{ 92 }
async { 92 }
'a: { 92 }
#[a] { 92 }
Previously the tree for them were
BLOCK_EXPR
{ ... }
EFFECT_EXPR
async
BLOCK_EXPR
{ ... }
EFFECT_EXPR
'a:
BLOCK_EXPR
{ ... }
BLOCK_EXPR
#[a]
{ ... }
As you see, it gets progressively worse :) The last two items are
especially odd. The last one even violates the balanced curleys
invariant we have (#10357) The new approach is to say that the stuff in
`{}` is stmt_list, and the block is stmt_list + optional modifiers
BLOCK_EXPR
STMT_LIST
{ ... }
BLOCK_EXPR
async
STMT_LIST
{ ... }
BLOCK_EXPR
'a:
STMT_LIST
{ ... }
BLOCK_EXPR
#[a]
STMT_LIST
{ ... }
10213: minor: Improve resilience of match checking r=flodiebold a=iDawer
In bug condition the match checking strives to recover giving false no-error diagnostic.
Suggested in https://github.com/rust-analyzer/rust-analyzer/pull/9105#discussion_r644656085
Co-authored-by: Dawer <7803845+iDawer@users.noreply.github.com>
Should fix#10090, #10046, #10179.
This is only a workaround, but the proper fix requires some bigger
refactoring (also related to fixing #10058), and this at least prevents
the crash.
9954: feat: Show try operator propogated types on ranged hover r=matklad a=Veykril
Basically this just shows the type of the inner expression of the `?` expression as well as the type of the expression that the `?` returns from:
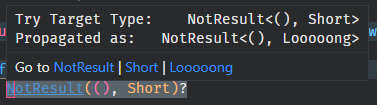
Unless both of these types are `core::result::Result` in which case we show the error types only.
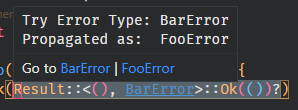
If both types are `core::option::Option` with different type params we do not show this special hover either as it would be pointless(instead fallback to default type hover)
Very much open to changes to the hover text here(I suppose we also want to show the actual type of the `?` expression, that is its output type?).
Fixes#9931
Co-authored-by: Lukas Wirth <lukastw97@gmail.com>
9975: minor: Fix panic caused by #9966 r=flodiebold a=flodiebold
Chalk can introduce new type variables when doing lazy normalization, so we have to do the proper 'fudging' after all.
Co-authored-by: Florian Diebold <flodiebold@gmail.com>
9453: Add first-class limits. r=matklad,lnicola a=rbartlensky
Partially fixes#9286.
This introduces a new `Limits` structure which is passed as an input
to `SourceDatabase`. This makes limits accessible almost everywhere in
the code, since most places have a database in scope.
One downside of this approach is that whenever you query limits, you
essentially do an `Arc::clone` which is less than ideal.
Let me know if I missed anything, or would like me to take a different approach!
Co-authored-by: Robert Bartlensky <bartlensky.robert@gmail.com>
... instead of using `AliasTy`. Chalk turns the alias type into the
placeholder during unification anyway, which confuses our method
resolution logic.
Fixes#9530.
9260: tree-wide: make rustdoc links spiky so they are clickable r=matklad a=lf-
Rustdoc was complaining about these while I was running with --document-private-items and I figure they should be fixed.
Co-authored-by: Jade <software@lfcode.ca>