feat: Add an assist for inlining all type alias uses
## Description
`inline_type_alias_uses` assist tries to inline all selected type alias occurrences.
### Currently
Type alias used in `PathType` position are inlined.
### Not supported
- Removing type alias declaration if all uses are inlined.
- Removing redundant imports after inlining all uses in the file.
- Type alias not in `PathType` position, such as:
- `A::new()`
- `let x = A {}`
- `let bits = A::BITS`
- etc.
## Demonstration
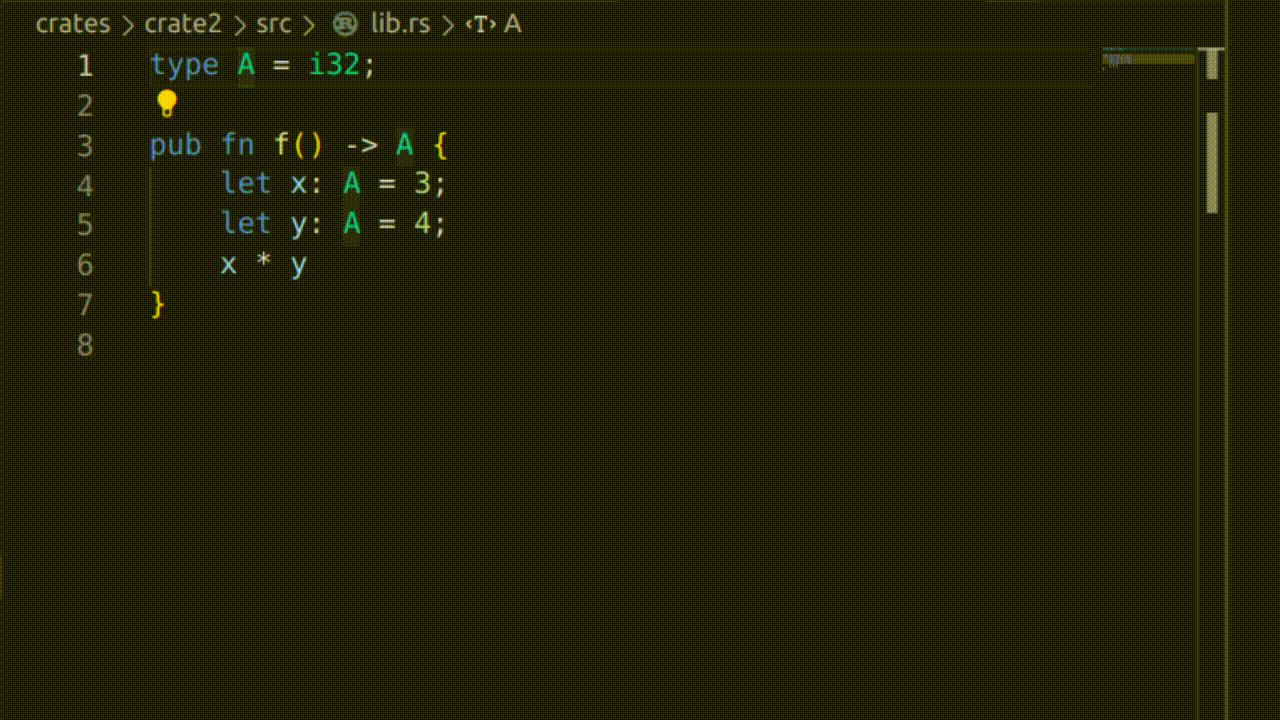
## Related Issues
Partially fixes#10881
This PR will fix some typos detected by [typos].
There are also some other typos in the function names, variable names, and file
names, which I leave as they are. I'm more certain that typos in comments
should be fixed.
[typos]: https://github.com/crate-ci/typos
fix: don't replace default members' body
cc #12779, #12821
addresses https://github.com/rust-lang/rust-analyzer/pull/12821#issuecomment-1190157506
`gen_trait_fn_body()` only attempts to implement required trait member functions, so we shouldn't call it for `Implement default members` assist.
This patch also documents the precondition of `gen_trait_fn_body()` and inserts `debug_assert!`, but I'm not entirely sure if the assertions are appropriate.
fix: Insert `pub(crate)` after doc comments and attribute macros
Fixes#12790
Original behavior was to insert `pub(crate)` at the `first_child_or_token`, which for an item with a comment or attribute macro, would put the visibility marker before the comment or macro, instead of after.
This merge request alters the call to find the node with appropriate `SyntaxKind` in the `children_or_tokens`. It also adds a test case to the module to verify the behavior. Test case verifies function, module, records, enum, impl, trait, and type cases.
fix: Prevent panic in Remove Unused Parameter assist
Instead of calling `builder.delete` for every text range we find with
`process_usage`, we now ensure that the ranges do not overlap before removing
them. If a range is fully contained by a prior one, it is dropped.
fixes#12784
Instead of calling `builder.delete` for every text range we find with
`process_usage`, we now ensure that the ranges do not overlap before removing
them. If a range is fully contained by a prior one, it is dropped.
fixes#12784
Fix extract variable assist for subexpression in mutable borrow
This checks if the expression is in a mutable borrow and if so makes the extracted variable `mut`.
Closes#12786
Automatically instaciate trivially instaciable structs in "Generate new" and "Fill struct fields"
As proposed in #12535 this PR changes the "Generate new" and "Fill struct fields" assist/diagnostic to instanciate structs with no fields and enums with a single empty variant.
For example:
```rust
pub enum Bar {
Bar {},
}
struct Foo<T> {
a: usize,
bar: Bar,
_phantom: std::marker::PhantomData<T>,
}
impl<T> Foo<T> {
/* generate new */
fn random() -> Self {
Self { /* Fill struct fields */ }
}
}
```
was previously:
```rust
impl<T> Foo<T> {
fn new(a: usize, bar: Bar, _phantom: std::marker::PhantomData<T>) -> Self {
Self { a, bar, _phantom }
}
fn random() -> Self {
Self {
a: todo!(),
bar: todo!(),
_phantom: todo!(),
}
}
}
```
and is now:
```rust
impl<T> Foo<T> {
fn new(a: usize) -> Self {
Self {
a,
bar: Bar::Bar {},
_phantom: std::marker::PhantomData
}
}
fn random() -> Self {
Self {
a: todo!(),
bar: Bar::Bar {},
_phantom: std::marker::PhantomData,
}
}
}
```
I'd be happy about any suggestions.
## TODO
- [x] deduplicate `use_trivial_constructor` (unclear how to do as it's used in two separate crates)
- [x] write tests
Closes#12535
fix: Support generics in extract_function assist
This change attempts to resolve issue #7636: Extract into Function does not
create a generic function with constraints when extracting generic code.
In `FunctionBody::analyze_container`, we now traverse the `ancestors` in search
of `AnyHasGenericParams`, and attach any `GenericParamList`s and `WhereClause`s
we find to the `ContainerInfo`.
Later, in `format_function`, we collect all the `GenericParam`s and
`WherePred`s from the container, and filter them to keep only types matching
`TypeParam`s used within the newly extracted function body or param list. We
can then include the new `GenericParamList` and `WhereClause` in the new
function definition.
This change only impacts `TypeParam`s. `LifetimeParam`s and `ConstParam`s are
out of scope for this change.
I've never contributed to this project before, but I did try to follow the style guide. I believe that this change represents an improvement over the status quo, but I think it's also fair to argue that it doesn't fully "fix" the linked issue. I'm totally open to merging this as is, or going further to try to make a more complete solution. Also: if there are other unit or integration tests I should add, please let me know where to look!
This change attempts to resolve issue #7636: Extract into Function does not
create a generic function with constraints when extracting generic code.
In `FunctionBody::analyze_container`, we now traverse the `ancestors` in search
of `AnyHasGenericParams`, and attach any `GenericParamList`s and `WhereClause`s
we find to the `ContainerInfo`.
Later, in `format_function`, we collect all the `GenericParam`s and
`WherePred`s from the container, and filter them to keep only types matching
`TypeParam`s used within the newly extracted function body or param list. We
can then include the new `GenericParamList` and `WhereClause` in the new
function definition.
This change only impacts `TypeParam`s. `LifetimeParam`s and `ConstParam`s are
out of scope for this change.