Added remove unused imports assist
This resolves the most important part of #5131. I needed to make a couple of cosmetic changes to the search infrastructure to do this.
A few open questions:
* Should imports that don't resolve to anything be considered unused? I figured probably not, but it would be a trivial change to make if we want it.
* Is there a cleaner way to make the edits to the use list?
* Is there a cleaner way to get the list of uses that intersect the current selection?
* Is the performance acceptable? When testing this on itself, it takes a good couple seconds to perform the assist.
* Is there a way to hide the rustc diagnostics that overlap with this functionality?
assist : generate trait from impl
fixes#14987 . As the name suggests this assist is used to generate traits from inherent impls while adapting the original impl to fit to the newly generated trait. I made some decisions regarding when the assist should be applicable. These are surely open to discussion. I looking forward to any feedback.
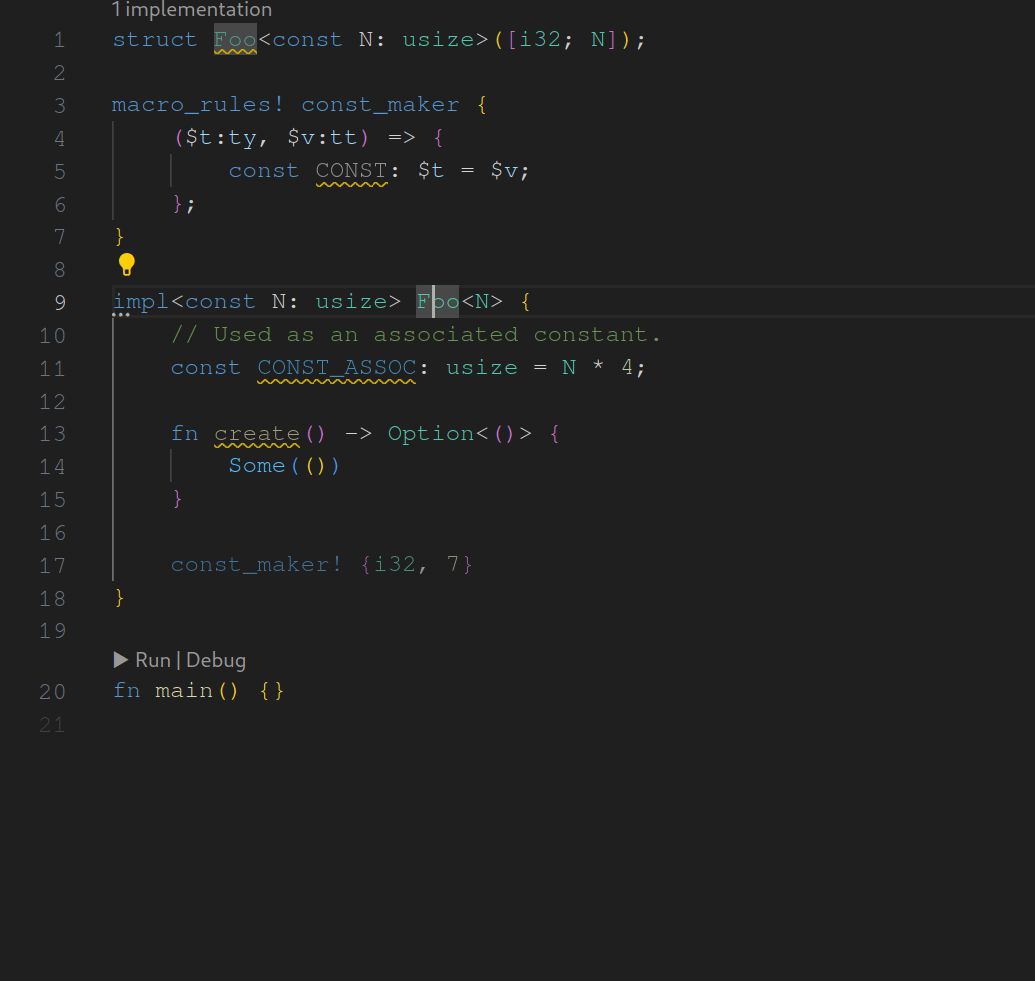
This removes an existing generic param from the `GenericParamList`. It
also considers to remove the extra colon & whitespace to the previous
sibling.
* change order to get all param types first and mark them as mutable
before the first edit happens
* add helper function to remove a generic parameter
* fix test output
This adds a new assist named "replace named generic with impl" to move
the generic param type from the generic param list into the function
signature.
```rust
fn new<T: ToString>(input: T) -> Self {}
```
becomes
```rust
fn new(input: impl ToString) -> Self {}
```
The first step is to determine if the assist can be applied, there has
to be a match between generic trait param & function paramter types.
* replace function parameter type(s) with impl
* add new `impl_trait_type` function to generate the new trait bounds with `impl` keyword for use in the
function signature
fix: assists no longer break indentation
Fixes https://github.com/rust-lang/rust-analyzer/issues/14674
These are _ad hoc_ patches for a number of assists that can produce incorrectly indented code, namely:
- generate_derive
- add_missing_impl_members
- add_missing_default_members
Some general solution is required in future, as the same problem arises in many other assists, e.g.
- replace_derive_with...
- generate_default_from_enum...
- generate_default_from_new
- generate_delegate_methods
(the list is incomplete)
Fix: a TODO and some clippy fixes
- fix(todo): implement IntoIterator for ArenaMap<IDX, V>
- chore: remove unused method
- fix: remove useless `return`s
- fix: various clippy lints
- fix: simplify boolean test to a single negation
Add syntax::make::ty_alias
There was until now no function that returns TypeAlias. This commit introduces a func that is fully compliant with the Rust Reference. I had problems working with Ident so for now the function uses simple string manipulation until ast_from_text function is called. I am however open to any ideas that could replace ident param in such a way that it accepts syntax::ast::Ident
Simple fix for make::impl_trait
This is my first PR in this project. I made this PR because I needed this function to work properly for the main PR I am working on (#14386). This is a small amendment to what it was before. We still need to improve this in order for it to fully comply with its syntactic definition as stated [here](https://doc.rust-lang.org/reference/items/implementations.html).