The current system with AstIds has two primaraly drawbacks:
* It is possible to manufacture IDs out of thin air.
For example, it's possible to create IDs for items which are not
considered in CrateDefMap due to cfg. Or it is possible to mixup
structs and unions, because they share ID space.
* Getting the ID of a parent requires a secondary index.
Instead, the plan is to pursue the more traditional approach, where
each items stores the id of the parent declaration. This makes
`FromSource` more awkward, but also more correct: now, to get from an
AST to HIR, we first do this recursively for the parent item, and the
just search the children of the parent for the matching def
This commit implements a general truncation framework for HirFormatter
that keeps track of how much has been output so far. This information
can then be used to perform truncation inside the language server,
instead of relying on the client.
Initial support is implemented for truncating types hints using the
maxInlayHintLength server config option. The existing solution in the
VSCode extension has been removed in favor of letting the server
truncate type hints.
2307: Support hover through macro r=matklad a=kjeremy
Allows hover to work through macros like `match_ast!`.
Co-authored-by: kjeremy <kjeremy@gmail.com>
We need to be more careful now when substituting bound variables (previously, we
didn't have anything that used bound variables except Chalk, so it was not a
problem).
This is obviously quite ad-hoc; Chalk has more infrastructure for handling this
in a principled way, which we maybe should adopt.
2252: Fix parsing of "postfix" range expressions. r=matklad a=goffrie
Right now they are handled in `postfix_dot_expr`, but that doesn't allow it to
correctly handle precedence. Integrate it more tightly with the Pratt parser
instead.
Also includes a drive-by fix for parsing `match .. {}`.
Fixes#2242.
Co-authored-by: Geoffry Song <goffrie@gmail.com>
Right now they are handled in `postfix_dot_expr`, but that doesn't allow it to
correctly handle precedence. Integrate it more tightly with the Pratt parser
instead.
Also includes a drive-by fix for parsing `match .. {}`.
Fixes#2242.
2165: ra_assists: Add add_new assist r=matklad a=rep-nop
Adds a new assist to autogenerate a new fn based on the selected struct, excluding tuple structs and unions. The fn will inherit the same visibility as the struct and the assist will attempt to reuse any existing impl blocks that exist at the same level of struct.
Not marking this as closing #1644 since there's a part 2 of adding autocompletion for when someone starts typing `[pub ]fn new(...`
Co-authored-by: Wesley Norris <repnop@outlook.com>
2249: Cleanup hover r=matklad a=kjeremy
Take advantage of classify_name to consolidate multiple hover paths. This isn't quite as clean as I want it to be (`no_fallback` bool is wonky). There's a relationship between `HoverResult` being empty and the range that is a little warty.
Also I noticed that HoverResults are always marked as exact and have been for quite a while... maybe that should be removed in another PR.
Co-authored-by: Jeremy Kolb <kjeremy@gmail.com>
2217: Implement FromStr for enum Edition r=matklad a=clemarescx
Just did this as I came across the comment in the code asking for implementing `std::str::FromStr` for `input::Edition`.
Not sure what was meant by "proper error handling" though, `panic!` with a descriptive message might not be it 😅
Co-authored-by: Metabaron <metabaron@tuta.io>
2200: Add variables to HIR r=matklad a=matklad
Introduce a `hir::Variable`, which should cover locals, parameters and `self`. Unlike `PatId`, variable knows it's owner so it is self-contained, and should be more convenient to use from `ra_ide_api`.
The goal here is to hide more details about `Body` from hir, which should make it easier to move `Body` into `hir_def`. I don't think that `ra_ide_api` intrracts with bodies directly at the moment anyway, but the glue layer is based basically on `ast::BindPat`, which seems pretty brittle.
Co-authored-by: Aleksey Kladov <aleksey.kladov@gmail.com>
2205: Implement bulitin line! macro r=matklad a=edwin0cheng
This PR implements bulitin macro `line!` and add basic infra-structure for other bulitin macros:
1. Extend `MacroDefId` to support builtin macros
2. Add a `quote!` macro for simple quasi quoting.
Note that for support others builtin macros, eager macro expansion have to be supported first, this PR not try to handle it. :)
Co-authored-by: Edwin Cheng <edwin0cheng@gmail.com>
This removes the special casing for the "core" prelude.
Whenever a later dependency also exports a prelude, it will replace
the formerly imported prelude. The utilized prelude then depends
purely on import order.
This adds support for completion and goto definition of
types defined within the "core" crate. The core crate is
added as a dependency to each crate in the project.
The core crate exported it's own prelude. This caused
now all crates to inherit the core crates prelude instead
of the std crates. In order to avoid the problem the
prelude resolution has been changed to overwrite
an already resolved prelude if this was set to a crate
named core - in order to pick a better prelude like std.
Fixes#2199
Adds a new assist to autogenerate a new fn based on the selected struct,
excluding tuple structs and unions. The fn will inherit the same
visibility as the struct and the assist will attempt to reuse any
existing impl blocks that exist at the same level of struct.
2169: MBE: Mapping spans for goto definition r=matklad a=edwin0cheng
Currently, go to definition gives the wrong span in MBE. This PR implement a mapping mechanism to fix it and it could be used for future MBE hygiene implementation.
The basic idea of the mapping is:
1. When expanding the macro, generated 2 `TokenMap` which maps the macro args and macro defs between tokens and input text-ranges.
2. Before converting generated `TokenTree` to `SyntaxNode`, generated a `ExpandedRangeMap` which is a mapping between token and output text-ranges.
3. Using these 3 mappings to construct an `ExpansionInfo` which can map between input text ranges and output text ranges.
Co-authored-by: Edwin Cheng <edwin0cheng@gmail.com>
Removes nodrop and extra arrayvec
We have an extra crossbeam-queue and crossbeam-utils left but those should
drop once rayon accepts https://github.com/rayon-rs/rayon/pull/704
2179: Use HirDatabase to compute `is_deprecated` r=matklad a=martskins
This PR fixes#2167 by introducing `attributes_query` and adding `fn attrs(&self, def: crate::AttrDef) -> Option<Arc<[Attr]>>;` to the `DefDatabase` trait.
I'm a little concerned about the two spots in `attributes_query` where code is repeated, but I couldn't figure out a way to avoid that, so.. I welcome suggestions 😄
Co-authored-by: Martin Asquino <martin.asquino@gmail.com>
2173: MBE: Add TokenId shift in macro_rules r=matklad a=edwin0cheng
As discussed in #2169 , for fixing duplication TokenId during expansion :
> What we can do here is to re-number the tokens during expansion. Specifically:
> * when we create macro_rules, we note the highest id of the token we have as shift>
> * when we expand macro rules, if we need to output a token from definition, we just re-use its id
> * if we need to output a token from the argument, we increase its id by shift (so it's guaranteed to not to collide with anything from the definition)
> * finally, when we have a HirFileId of the expansion, we can look up the original value of shift and classify node to the arg/def by comparing it's id with shift.
>
This PR implement first 3 points of above solution.
Co-authored-by: Edwin Cheng <edwin0cheng@gmail.com>
2149: Handle IfLet in convert_to_guarded_return. r=matklad a=krk
Fixes https://github.com/rust-analyzer/rust-analyzer/issues/2124
I could not move the cursor position out of `let`:
`le<|>t` vs `let<|>`.
Also, please suggest extra test cases.
Co-authored-by: krk <keremkat@gmail.com>
2160: Set `deprecated` field on `CompletionItem`s r=matklad a=martskins
This PR aims to address #2042 by setting the deprecated field for completion items.
The setting the tags field for LSP 3.15 part still needs fixing, but that one is blocked due to lsp-types not being up to date with 3.15 yet.
Co-authored-by: Martin Asquino <martin.asquino@gmail.com>
2103: Expand signature help r=matklad a=kjeremy
Signature help using call syntax with tuple structs and enum variants
Fixes#2102.
Co-authored-by: Jeremy Kolb <kjeremy@gmail.com>
Co-authored-by: kjeremy <kjeremy@gmail.com>
2111: Fix autoimport not choosing the deepest use tree in some situations r=matklad a=flodiebold
Co-authored-by: Florian Diebold <flodiebold@gmail.com>
2097: Be more precise with function signatures r=matklad a=kjeremy
Finds the closest call expr.
Fixes#2093
Co-authored-by: Jeremy Kolb <kjeremy@gmail.com>
Whitespace can have special meaning in markdown. For instance
ending a line with three spaces will render a new line.
Note that this behavior diverges from RLS.
Fixes#1997
2099: Fix panic on raw string assist r=matklad a=aee11
Strings that do not contain two quotation marks would cause a slice indexing panic because `find_usual_string_range` would return a range that only contained a single quotation mark.
Panic example:
```
fn main() {
let s = "<|>
}
```
I noticed a lot of panics from the `make_raw_string` assist while working on another issue today.
Co-authored-by: Alexander Elís Ebenesersson <alex2789@gmail.com>
Strings that do not contain two quotation marks
would cause a slice indexing panic because code
was assuming `find_usual_string_range` would return
a string with two quotes, but it would incorrectly
also return text ranges containing only a single quote.
When multiple traits bounds are present, expanded selection
from a single trait bound will include the nearest plus sign
(and whitespace after) before including the whole trait bound.
2006: Improvements around `Arc<[T]>` r=matklad a=sinkuu
First commit tries to avoid cloning `Arc<[T]>` to a temporary `Vec` for mutating it, if there are no other strong references. Second commit utilizes [`FromIterator for Arc<[T]>`](https://doc.rust-lang.org/std/sync/struct.Arc.html#impl-FromIterator%3CT%3E) instead of `.collect::<Vec<_>>().into()` to avoid allocation in `From<Vec<T>> for Arc<[T]>`.
Co-authored-by: Shotaro Yamada <sinkuu@sinkuu.xyz>
1924: Support inferring&completing `Self` type in enum/struct/union definitions r=ice1000 a=ice1000
Signed-off-by: ice1000 <ice1000kotlin@foxmail.com>
An attempt to fix#1908.
This code works, but I believe the implementation is ugly. Please give me suggestions!
Co-authored-by: ice1000 <ice1000kotlin@foxmail.com>
1951: Lower the precedence of the `as` operator. r=matklad a=goffrie
Previously, the `as` operator was being parsed like a postfix expression, and
therefore being given the highest possible precedence. That caused it to bind
more tightly than prefix operators, which it should not. Instead, parse it
somewhat like a normal binary expression with some special-casing.
Fixes#1851.
Co-authored-by: Geoffry Song <goffrie@gmail.com>
1928: Support `#[cfg(..)]` r=matklad a=oxalica
This PR implement `#[cfg(..)]` conditional compilation. It read default cfg options from `rustc --print cfg` with also hard-coded `test` and `debug_assertion` enabled.
Front-end settings are **not** included in this PR.
There is also a known issue that inner control attributes are totally ignored. I think it is **not** a part of `cfg` and create a separated issue for it. #1949Fixes#1920
Related: #1073
Co-authored-by: uHOOCCOOHu <hooccooh1896@gmail.com>
Co-authored-by: oxalica <oxalicc@pm.me>
Fixes#1807
This assist can transform expressions of the form `!x || !y` into
`!(x && y)`. This also works with `&&`.
This assist will only trigger if the cursor is on the central logical
operator.
The main limitation of this current implementation is that both operands
need to be an explicit negation, either of the form `!x`, or `x != y`.
More operands could be accepted, but this would complicate the implementation
quite a bit.
Forbidding block expressions entirely is too strict; instead, we should only
forbid them in contexts where we are parsing an optional RHS (i.e. the RHS of a
range expression).
Previously, the `as` operator was being parsed like a postfix expression, and
therefore being given the highest possible precedence. That caused it to bind
more tightly than prefix operators, which it should not. Instead, parse it
somewhat like a normal binary expression with some special-casing.
1945: Handle divergence in type inference for blocks r=flodiebold a=lnicola
Fixes#1944.
The `infer_basics` test is failing, not sure what to do about it.
Co-authored-by: Laurențiu Nicola <lnicola@dend.ro>
1815: Support correct `$crate` expansion in macros r=uHOOCCOOHu a=uHOOCCOOHu
This PR makes normal use cases of `$crate` from macros work as expected.
It makes more macros from `std` work. Type inference works well with `panic`, `unimplemented`, `format`, and maybe more.
Sadly that `vec![1, 2, 3]` still not works, but it is not longer an issue about macro.
Screenshot:
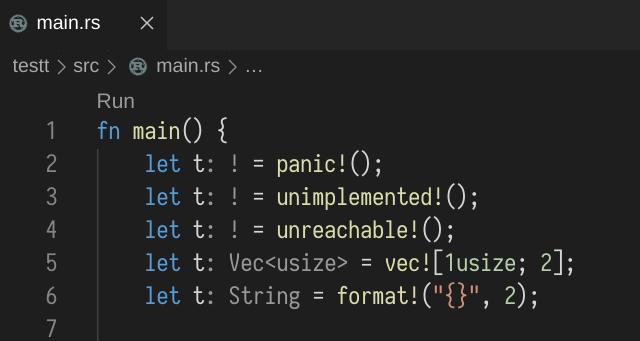
Co-authored-by: uHOOCCOOHu <hooccooh1896@gmail.com>
1912: add new editing API, suitable for modifying several nodes at once r=viorina a=matklad
r? @viorina
Co-authored-by: Aleksey Kladov <aleksey.kladov@gmail.com>
1895: Handle associated type shorthand (`T::Item`) (Second attempt) r=flodiebold a=flodiebold
This is only allowed for generic parameters (including `Self` in traits), and
special care needs to be taken to not run into cycles while resolving it,
because we use the where clauses of the generic parameter to find candidates for
the trait containing the associated type, but the where clauses may themselves
contain instances of short-hand associated types.
In some cases this is even fine, e.g. we might have `T: Trait<U::Item>, U:
Iterator`. If there is a cycle, we'll currently panic, which isn't great, but
better than overflowing the stack...
Co-authored-by: Florian Diebold <flodiebold@gmail.com>
This is only allowed for generic parameters (including `Self` in traits), and
special care needs to be taken to not run into cycles while resolving it,
because we use the where clauses of the generic parameter to find candidates for
the trait containing the associated type, but the where clauses may themselves
contain instances of short-hand associated types.
In some cases this is even fine, e.g. we might have `T: Trait<U::Item>, U:
Iterator`. If there is a cycle, we'll currently panic, which isn't great, but
better than overflowing the stack...
1853: Introduce FromSource trait r=matklad a=viorina
The idea is to provide an ability to get HIR from AST in a more general way than it's possible using `source_binder`.
It also could help with #1622 fixing.
Co-authored-by: Ekaterina Babshukova <ekaterina.babshukova@yandex.ru>
1862: Assoc item resolution refactoring (again) r=flodiebold a=flodiebold
This is #1849, with the associated type selection code removed for now. Handling cycles there will need some more thought.
Co-authored-by: Florian Diebold <flodiebold@gmail.com>
I must confess I don't really understand what this code is trying to
do, but it definitely misreports changes during fixedpoint iteration,
and no tests fail if I remove it, so...
Type-relative paths (`<T>::foo`) also need to work in type context, for example
`<T>::Item` is legal. So rather than returning the type ref from the resolver
function, just check it before.
E.g. `fn foo<T: Iterator>() -> T::Item`. It seems that rustc does this only for
type parameters and only based on their bounds, so we also only consider traits
from bounds.
1848: Parse `..` as a full pattern r=matklad a=ecstatic-morse
Resolves#1479.
This PR implements [RFC 2707](https://github.com/rust-lang/rfcs/pull/2707) in the parser. It introduces a new `DotDotPat` AST node modeled on `PlaceholderPat` and changes the parsing of tuple and slice patterns to conform to the RFC.
Notably, this PR does *not* change the resulting AST when `..` appears in a struct pattern (e.g. `Struct { a, b: c, .. }`). I *think* this is the behavior mandated by RFC 2707, but someone should confirm this.
Co-authored-by: Dylan MacKenzie <ecstaticmorse@gmail.com>
Nameres related types, like `PerNs<Resolution>`, can represent
unreasonable situations, like a local in a type namespace. We should
clean this up, by requiring that call-site specifies the kind of
resolution it expects.
1821: Macro completion tweaks r=matklad a=SomeoneToIgnore
Thanks @uHOOCCOOHu for making the macro completion happen :)
I've added a few tweaks to the current completion to make it a bit more convenient:
* Automatically add braces and put the editor cursor inside of them:
<img width="159" alt="image" src="https://user-images.githubusercontent.com/2690773/64737220-022b9f00-d4f5-11e9-8088-76d4678921ab.png">
Currently I have to add the braces manually which is a bit cumbersome.
One further improvement can be to detect if macro accepts no parameters and place the cursor differently for this case.
* Add an exclamation mark to the macro completion label
This helps to distinguish macros from other completion items and also allows to show only macros in completion if you type `!`:
<img width="722" alt="image" src="https://user-images.githubusercontent.com/2690773/64736987-8b8ea180-d4f4-11e9-8355-2ce4f83b7aa8.png">
<img width="732" alt="image" src="https://user-images.githubusercontent.com/2690773/64737214-ffc94500-d4f4-11e9-946e-1ba2db1c7fb1.png">
Additionally, automatic formatting hooks had adjusted two `help.rs` files, I've added them as a last commit to the PR even though they are not really related.
Co-authored-by: Kirill Bulatov <mail4score@gmail.com>
Parser has the invariant that `{}` are balanced.
Previous code tried (unsucesfuly) maintain the same invariant for
`$()` as well, but it was done in a rather ad-hoc manner: it's not at
all obvious that it is possible to maintain both invariants!
1795: Make macro scope a real name scope and fix some details r=matklad a=uHOOCCOOHu
This PR make macro's module scope a real name scope in `PerNs`, instead of handling `Either<PerNs, MacroDef>` everywhere.
In `rustc`, the macro scope behave exactly the same as type and value scope.
It is valid that macros, types and values having exact the same name, and a `use` statement will import all of them. This happened to module `alloc::vec` and macro `alloc::vec!`.
So `Either` is not suitable here.
There is a trap that not only does `#[macro_use]` import all `#[macro_export] macro_rules`, but also imports all macros `use`d in the crate root.
In other words, it just _imports all macros in the module scope of crate root_. (Visibility of `use` doesn't matter.)
And it also happened to `libstd` which has `use alloc_crate::vec;` in crate root to re-export `alloc::vec`, which it both a module and a macro.
The current implementation of `#[macro_use] extern crate` doesn't work here, so that is why only macros directly from `libstd` like `dbg!` work, while `vec!` from `liballoc` doesn't.
This PR fixes this.
Another point is that, after some tests, I figure out that _`macro_rules` does NOT define macro in current module scope at all_.
It defines itself in legacy textual scope. And if `#[macro_export]` is given, it also is defined ONLY in module scope of crate root. (Then being `macro_use`d, as mentioned above)
(Well, the nightly [Declarative Macro 2.0](https://github.com/rust-lang/rust/issues/39412) simply always define in current module scope only, just like normal items do. But it is not yet supported by us)
After this PR, in my test, all non-builtin macros are resolved now. (Hover text for documentation is available) So it fixes#1688 . Since compiler builtin macros are marked as `#[rustc_doc_only_macro]` instead of `#[macro_export]`, we can simply tweak the condition to let it resolved, but it may cause expansion error.
Some critical notes are also given in doc-comments.
<img width="447" alt="Screenshot_20190909_223859" src="https://user-images.githubusercontent.com/14816024/64540366-ac1ef600-d352-11e9-804f-566ba7559206.png">
Co-authored-by: uHOOCCOOHu <hooccooh1896@gmail.com>
Some method resolution tests now yield `{unknown}` where they did not
before.
Other tests now succeed, likely because this is helping the solver
steer its efforts.
This is to make debugging rust-analyzer easier.
The idea is that `dbg!(krate.debug(db))` will print the actual, fuzzy
crate name, instead of precise ID. Debug printing infra is a separate
thing, to make sure that the actual hir doesn't have access to global
information.
Do not use `.debug` for `log::` logging: debugging executes queries,
and might introduce unneded dependencies to the crate graph
1793: Fix outer doc-comments of `macro_rules` r=matklad a=uHOOCCOOHu
Document comments of `macro_rules!` is currently parsed outside the `MACRO_CALL` node,
which makes `DocCommentsOwner::doc_comments()` always empty.
For the input:
```rust
/// Some docs
macro_rules! foo {
() => {};
}
```
Current parsing tree is:
```
SOURCE_FILE
COMMENT // <- This should be children of MACRO_CALL
WHITESPACE
MACRO_CALL
PATH
<...omitted...>
```
It should be:
```
SOURCE_FILE
MACRO_CALL
COMMENT
WHITESPACE
PATH
<...omitted...>
```
Co-authored-by: uHOOCCOOHu <hooccooh1896@gmail.com>