Previously, we only inverted comparison operators (< and the like) if
the type implemented Ord. This doesn't make sense: if `<` works, then
`>=` will work as well!
Extra semantic checks greatly reduce robustness and predictability of
the assist, it's better to keep things simple.
9871: Jump to generated func r=mahdi-frms a=rylev
Worked on this with `@yoshuawuyts.`
We thought we ran into an issue with the `generate_function` assist where the code was not being generated in a certain situations. However, it wasn't actually a bug just a very confusing implementation where the cursor is not moved to the newly generated function. This happened when the return type was successfully inferred (and not unit). The function would be generated, but selection would not be changed.
This can be very confusing: If the function is generated somewhat far from where the assist is being invoked, the user never sees that the code was generated (nor are they given the chance to actually implement the function body).
This PR makes it so that the cursor is _always_ moved to somewhere in the newly generated function. In addition, if we can infer unit as the type, then we do not still generate `-> ()` as the return type. Instead, we simply omit the return type.
This means the selection will move to either one of two places:
* A generated `-> ()` return type when we cannot successfully infer the return type.
* The `todo!()` body when we can successfully infer the return type.
Co-authored-by: Ryan Levick <me@ryanlevick.com>
9863: feat: Generate default trait fn impl when generating `PartialEq` r=yoshuawuyts a=yoshuawuyts
Implements a default trait function body when generating the `PartialEq` trait for a type. Thanks!
r? `@veykril`
Co-authored-by: Yoshua Wuyts <yoshuawuyts@gmail.com>
9846: feat: Generate default trait fn impl when generating `Clone` r=Veykril a=yoshuawuyts
Implements a default trait function body when generating the `Clone` trait for a type. Thanks!
r? `@\veykril`
Co-authored-by: Yoshua Wuyts <yoshuawuyts@gmail.com>
9804: Generate method from call r=matklad a=mahdi-frms
Needs a bit of refactoring. Tests also should be added.
Co-authored-by: mahdi-frms <mahdif1380@outlook.com>
9825: Generate default impl when converting #[derive(Default)] to manual impl r=Veykril a=yoshuawuyts
Similar to https://github.com/rust-analyzer/rust-analyzer/pull/9814, but for `#[derive(Default)]`. Thanks!
## Follow-up steps
I've added the tests inside `handlers/replace_derive_with_manual_impl.rs` again, but I'm planning a follow-up PR to extract these to `utils/` so we can share them between assists - and maybe even add another assist just for the purpose of testing these impls (e.g. `generate_default_trait_body`).
The step after _that_ is likely to fill out the remaining traits, so we can make it so whenever RA auto-completes a trait which also can be derived, we provide a default function body.
Co-authored-by: Yoshua Wuyts <yoshuawuyts@gmail.com>
9814: Generate default impl when converting `#[derive(Debug)]` to manual impl r=yoshuawuyts a=yoshuawuyts
This patch makes it so when you convert `#[derive(Debug)]` to a manual impl, a default body is provided that's equivalent to the original output of `#[derive(Debug)]`. This should make it drastically easier to write custom `Debug` impls, especially when all you want to do is quickly omit a single field which is `!Debug`.
This is implemented for enums, record structs, tuple structs, empty structs - and it sets us up to implement variations on this in the future for other traits (like `PartialEq` and `Hash`).
Thanks!
## Codegen diff
This is the difference in codegen for record structs with this patch:
```diff
struct Foo {
bar: String,
}
impl fmt::Debug for Foo {
fn fmt(&self, f: &mut fmt::Formatter<'_>) -> fmt::Result {
- todo!();
+ f.debug_struct("Foo").field("bar", &self.bar).finish()
}
}
```
Co-authored-by: Irina Shestak <shestak.irina@gmail.com>
Co-authored-by: Yoshua Wuyts <yoshuawuyts@gmail.com>
Co-authored-by: Yoshua Wuyts <yoshuawuyts+github@gmail.com>
9739: generate function assist favors deref cmpt types r=matklad a=mahdi-frms
Fixes#9713
Although that's still not relying on sematic info.
Co-authored-by: mahdi-frms <mahdif1380@outlook.com>
9731: feat: Add `replace_char_with_string` assist r=Veykril a=Veykril
Adds the counterpart for the `replace_string_with_char` assist and fixes the assist not escaping the `'` in the string `"'"` when transforming that to a char.
bors r+
Co-authored-by: Lukas Wirth <lukastw97@gmail.com>
9593: fix: Adding remove_unused_param for method and fixing same for assoc func r=matklad a=feniljain
Solves #9571
Co-authored-by: vi_mi <fenil.jain2018@vitstudent.ac.in>
9453: Add first-class limits. r=matklad,lnicola a=rbartlensky
Partially fixes#9286.
This introduces a new `Limits` structure which is passed as an input
to `SourceDatabase`. This makes limits accessible almost everywhere in
the code, since most places have a database in scope.
One downside of this approach is that whenever you query limits, you
essentially do an `Arc::clone` which is less than ideal.
Let me know if I missed anything, or would like me to take a different approach!
Co-authored-by: Robert Bartlensky <bartlensky.robert@gmail.com>
9652: Don't concat path in replace_qualified assist when they start with a keyword r=Veykril a=Veykril
Also keep the path if we can't find a path to the item instead of becoming non applicable.
bors r+
Co-authored-by: Lukas Wirth <lukastw97@gmail.com>
9614: Parse input expressions for dbg! invocations in remove_dbg r=Veykril a=Veykril
Instead of inspecting the input tokentree manually, parse the input as `,` delimited expressions instead and act on that. This simplifies the assist quite a bit.
Fixes#8455
Co-authored-by: Lukas Wirth <lukastw97@gmail.com>
9567: remove unneded special case r=matklad a=matklad
bors r+
🤖
9568: feat: add 'for' postfix completion r=lnicola a=mahdi-frms
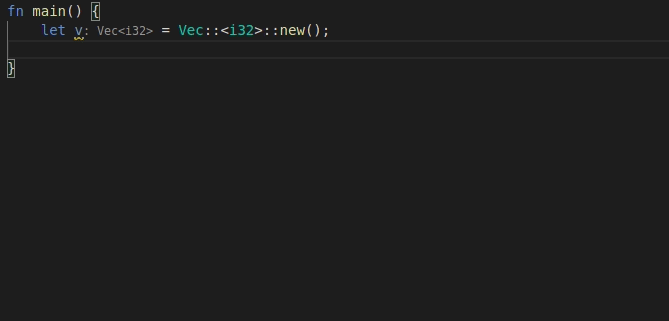
adds #9561
used ```ele``` as identifier for each element in the iteration
Co-authored-by: Aleksey Kladov <aleksey.kladov@gmail.com>
Co-authored-by: mahdi-frms <mahdif1380@outlook.com>
9553: minor: Disambiguate replace with if let assist labels r=Veykril a=Veykril
Turns out we have two assists for replacing something with `if let` constructs, so having the cursor on a `let` keyword inside a match gave you two identical assist labels which is rather confusing.
bors r+
Co-authored-by: Lukas Wirth <lukastw97@gmail.com>