internal: Add run-tests command
This command is similar to `cargo test` except that it uses r-a to run tests instead of compiling and running them with rustc. This is slower than `cargo test` and it is only useful for me to see a bird view of what needs to be fixed. The current output is:
```
48 passed, 5028 failed, 2 ignored
All tests 174.74s, 648ginstr
```
48 is very low, but higher than what I originally thought.
Now that there is some passing tests, I can show the plan:
https://github.com/rust-lang/rust-analyzer/assets/45197576/76d7d777-1843-4ca4-b7fe-e463bdade6cb
That is, at the end, I want to be able to immediately re run every test after every change. (0.5s is not really immediate, but it's not finished yet, and it is way better than 8s that running a typical test in r-a will take on my system)
Change comparsion for checking if number is negative to include 128
The last byte in Little-Endian representation of negative integers start at 128 (Ox80) till 255 (OxFF). The comparison before the fix didn't check for 128 which made is_negative variable as false.
Potentially fixes#15096
Added a test near positive extermes and two test near negative
extermes as well one for 0.
Added a test using the `as` cast and one with comparison with 0.
feature : assist delegate impl
This PR ( fixes#14386 ) introduces a new IDE assist that generates a trait impl for a struct that delegates a field. This is a draft because the current `ide_db::path_transform::PathTransform` produces some unwanted results when it deals with extern crates, an example of which I attach as a GIF.
GIFs :
1. A general case
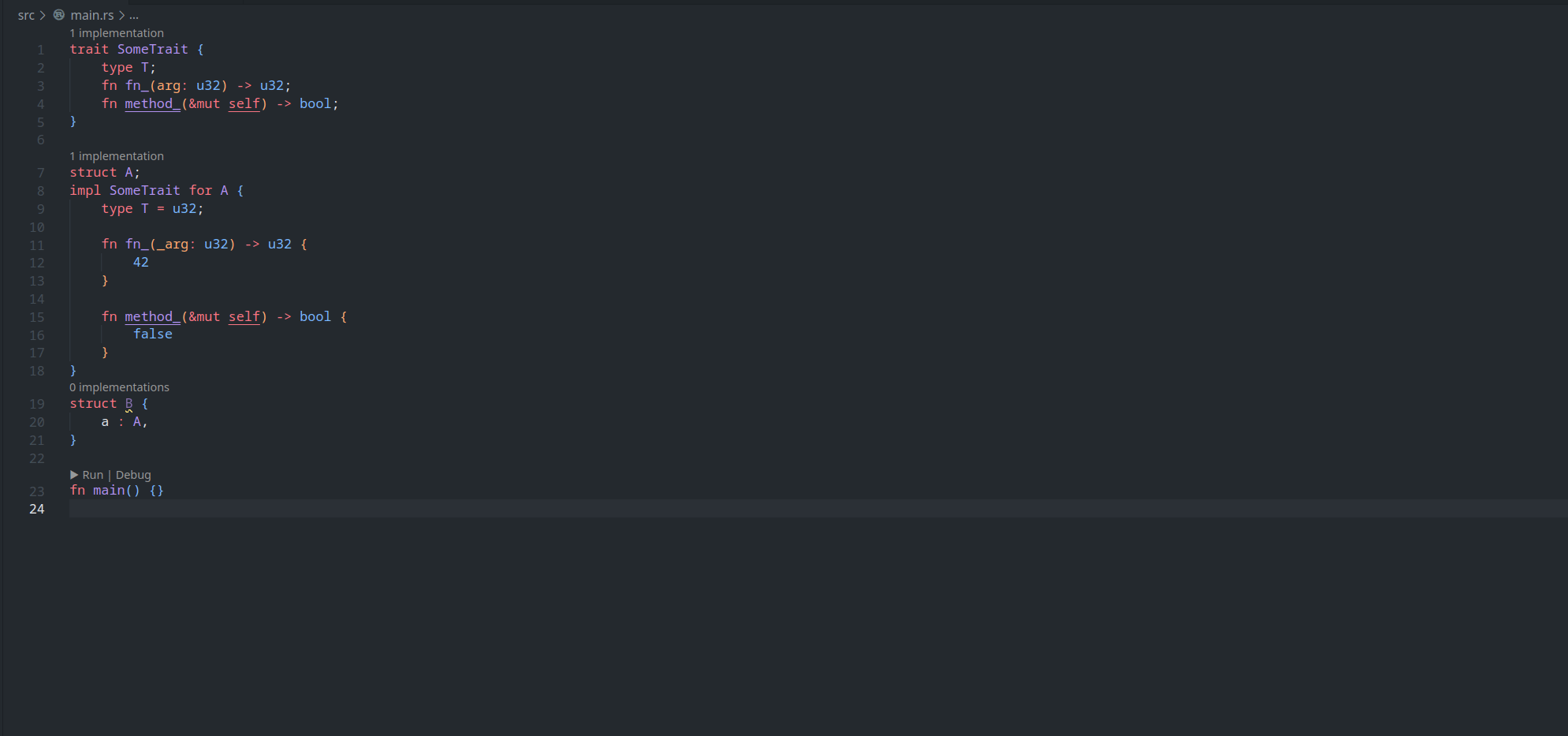
2. A case where `ide_db::path_transform::PathTransform` fails to correctly resolve a property ( take `Allocator` as an example ) to its full path, thus causing an error to occur. ( Not to even mention that resolving this causes another error `use of unstable library feature 'allocator_api'` to occur
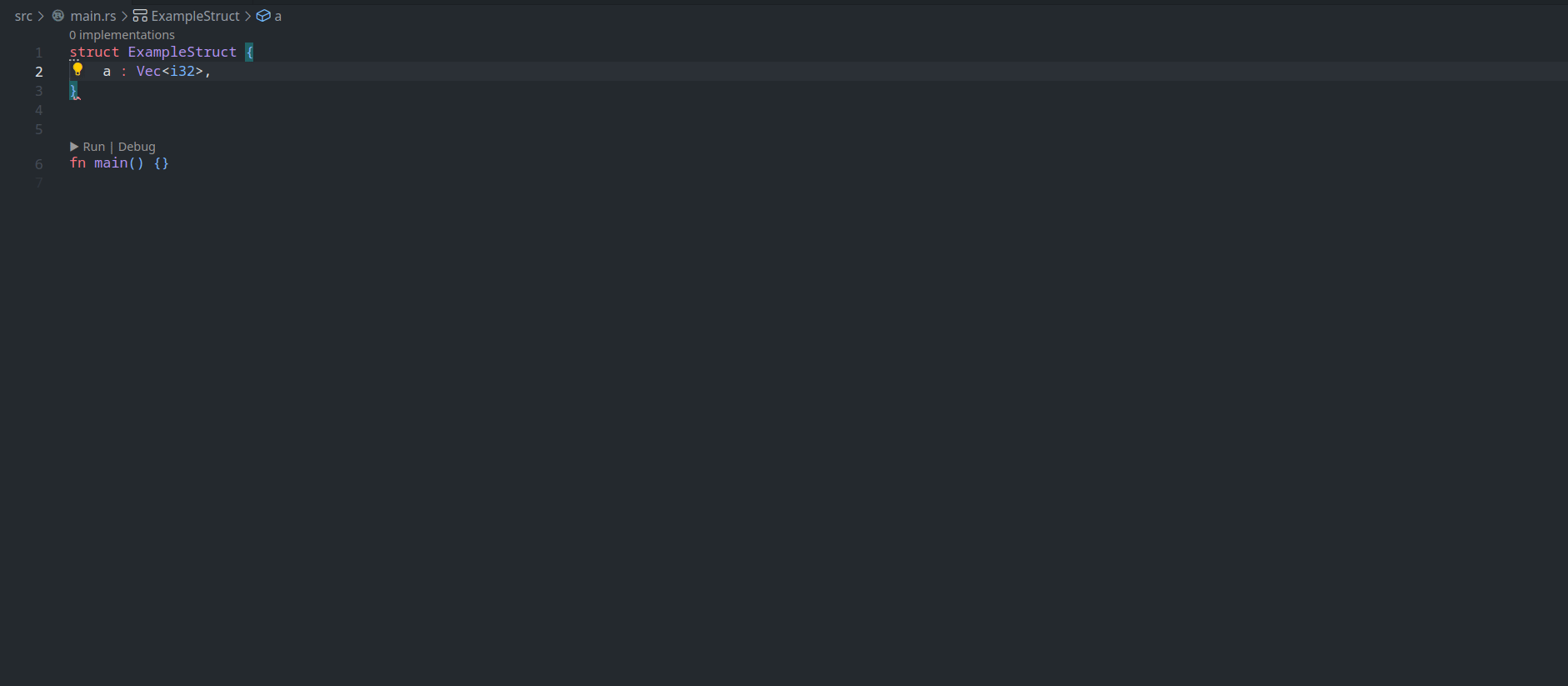
Reason: The last byte in Little Endian representation of negative
integers start at 128 (Ox80) till 255 (OxFF). The comparison before
the fix didn't check for 128 which made is_negative variable as false.
internal: remove spurious regex dependency
- replace tokio's env-filter with a smaller&simpler targets filter
- reshuffle logging infra a bit to make sure there's only a single place where we read environmental variables
- use anyhow::Result in rust-analyzer binary
- replace tokio's env-filter with a smaller&simpler targets filter
- reshuffle logging infra a bit to make sure there's only a single place
where we read environmental variables
- use anyhow::Result in rust-analyzer binary
Remove scope_for_def calls as the definition have been removed entirely.
As a result of this change the problem with false path resolutions has been solved.
internal: Record file dependencies in crate graph construction
Should fix the bug mentioned in https://github.com/rust-lang/rust-analyzer/issues/8623 where removing a crate root file will panic. I'm not too happy with the way this is done here but I can't think of a better way right now.
Deduplicate tuple indices for completion
Follow-up to #15026
A tuple struct may dereference to a primitive tuple (though unusual, which is why I previously overlooked this case). We should not show the same tuple index in completion in such cases.
Deduplication of indices among multiple tuple structs is already handled in the previous PR.
fix: deduplicate fields and types in completion
Fixes#15024
- `hir_ty::autoderef()` (which is only meant to be used outside `hir-ty`) now deduplicates types and completely resolves inference variables within.
- field completion now deduplicates fields of the same name and only picks such field of the first type in the deref chain.
Lower const params with a bad id
cc #7434
This PR adds an `InTypeConstId` which is a `DefWithBodyId` and lower const generic parameters into bodies using it, and evaluate them with the mir interpreter. I think this is the last unimplemented const generic feature relative to rustc stable.
But there is a problem: The id used in the `InTypeConstId` is the raw `FileAstId`, which changes frequently. So these ids and their bodies will be invalidated very frequently, which is bad for incremental analysis.
Due this problem, I disabled lowering for local crates (in library crate the id is stable since files won't be changed). This might be overreacting (const generic expressions are usually small, maybe it would be better enabled with bad performance than disabled) but it makes motivation for doing it in the correct way, and it splits the potential panic and breakages that usually comes with const generic PRs in two steps.
Other than the id, I think (at least I hope) other parts are in the right direction.
Properly format documentation for `SignatureHelpRequest`s
Properly formats function documentation instead of returning it raw when responding to `SignatureHelpRequest`s.
I added a test in `crates/rust-analyzer/tests/slow-tests/main.rs` -- not sure if this is the best location given the relevant code is in `crates/rust-analyzer` or if it's possible to test in a less heavyweight manner.
Closes#14958
fix: implemeted lifetime transformation fot assits
A part of https://github.com/rust-lang/rust-analyzer/issues/13363
I expect to implement transformation of const params in a separate PR
Other assists and a completion affected:
- `generate_function` currently just ignores lifetimes and, consequently, is not affected
- `inline_call` and `replace_derive_with...` don't seem to need lifetime transformation
- `trait_impl` (a completion) is fixed and tested
Add span to group.
This appears to fix#14959, but I've never contributed to rust-analyzer before and there were some things that confused me:
- I had to add the `fn byte_range` method to get it to build. This was added to rust in [April](https://github.com/rust-lang/rust/pull/109002), so I don't understand why it wasn't needed until now
- When testing, I ran into the fact that rust recently updated its `METADATA_VERSION`, so I had to test this with nightly-2023-05-20. But then I noticed that rust has its own copy of `rust-analyzer`, and the metadata version bump has already been [handled there](60e95e76d0). So I guess I don't really understand the relationship between the code there and the code here.
internal: Migrate some assists to use the structured snippet API
Migrates the following assists:
- `add_missing_impl_members`
- `extract_type_alias`
As an additional requirement, these assists are also migrated to use the mutable AST API, since otherwise there would be overlapping `Indel` spans
Infer return type for async function in `generate_function`
Part of #10122
In `generate_function` assist, when we infer the return type of async function we're generating, we should retrieve the type of parent await expression rather than the call expression itself.
Emit `'_` for lifetime generics in `HirDisplay`
This makes the generated code not linted by `rust_2018_idioms` lint. But that is an allow by default lint, so should we do this? Maybe we should only do this for `DisplayTarget::SourceCode`?
fix: consider outer binders when folding captured items' type
Fixes#14966
Basically, the crash is caused by us producing a broken type and passing it to chalk: `&dyn for<type> [for<> Implemented(^1.0: A<^0.0>)]` (notice the innermost bound var `^0.0` has no corresponding binder). It's created in `CapturedItemWithoutTy::with_ty()`, which didn't consider outer binders when folding types to replace placeholders with bound variables.
The fix is one-liner, but I've also refactored the surrounding code a little.
- use `DefWithBodyId::as_generic_def_id()`
- add comments on `InferenceResult` invariant
- move local helper function to bottom to comply with style guide
Fix drop scopes problems in mir
Fix false positives of `need-mut` emerged from #14955
There are still 5 `need-mut` false positives on self, all related to `izip!` macro hygenic issue. I will try to do something about that before monday release.
Fix Assist "replace named generic type with impl trait"
This is a follow-up PR to fix the assist "replace named generic type with impl trait" described in #14626 to filter invalid param types. It integrates the feedback given in PR #14816 .
The change updates the logic to determine when a function parameter is safe to replace a type param with its trait implementation. Some parameter definitions are invalid & should not be replaced by their traits, therefore skipping the assist completely.
First, all usages of the generic type under the cursor are determined. These usage references are checked to see if they occur outside the function parameter list. If an outside reference is found, e.g. in body, return type or where clause, the assist is skipped. All remaining usages need to appear only in the function param list. For each usage the param type is further inspected to see if it's valid. The logic to determine if a function parameter is valid, follows a heuristic and may not cover all possible parameter definitions.
With this change the following param types (as given in [this comment](https://github.com/rust-lang/rust-analyzer/pull/14816#discussion_r1206834603)) are not replaced & therefore skip the assist.
```rust
fn foo<P: Trait>(
_: <P as Trait>::Assoc, // within path type qualifier
_: <() as OtherTrait<P>>::Assoc, // same as above
_: P::Assoc, // associated type shorthand
_: impl OtherTrait<P> // generic arg in impl trait (note that associated type bindings are fine)
_: &dyn Fn(P) // param type and/or return type for Fn* traits
) {}
```
The change updates the logic to determine if a function parameter is
valid for replacing the type param with the trait implementation.
First all usages are determined, to check if they are used outside the function
parameter list. If an outside reference is found, e.g. in body, return type or
where clause, the assist is skipped. All remaining usages only appear in the
function param list. For each usage the param type is checked to see if
it's valid.
**Please note** the logic currently follows a heuristic and may not cover
all existing parameter declarations.
* determine valid usage references by checking ancestors (on AST level)
* split test into separate ones
fix: Fix nav target calculation discarding file ids from differing macro upmapping
Fixes https://github.com/rust-lang/rust-analyzer/issues/14792
Turns out there was the assumption that upmapping from a macro will always end in the same root file, which is no longer the case thanks to `include!`
Add signature help for tuple patterns and expressions
~~These are somewhat wonky since their signature changes as you type depending on context but they help out nevertheless.~~ should be less wonky now with added parser and lowering recoveries
fix: Don't duplicate sysroot crates in rustc workspace
Since we handle `library` as the sysroot source directly in the rustc workspace, we now duplicate the crates there, once as sysroot and once as just plain workspace crate. This causes a variety of issues for `vec!` macros and similar that emit `$crate` tokens across crates.
Prioritize threads affected by user typing
To this end I’ve introduced a new custom thread pool type which can spawn threads using each QoS class. This way we can run latency-sensitive requests under one QoS class and everything else under another QoS class. The implementation is very similar to that of the `threadpool` crate (which is currently used by rust-analyzer) but with unused functionality stripped out.
I’ll have to rebase on master once #14859 is merged but I think everything else is alright :D
Fix edits for `convert_named_struct_to_tuple_struct`
Two fixes:
- When replacing syntax nodes, macro files weren't taken into account. Edits were simply made for `node.syntax().text_range()`, which would be wrong range when `node` is inside a macro file.
- We do ancestor node traversal for every struct name reference to find record expressions/patterns to edit, but we didn't verify that expressions/patterns do actually refer to the struct we're operating on.
Best reviewed one commit at a time.
Fixes#13780Fixes#14927
Previously we didn't verify that record expressions/patterns that were
found did actually point to the struct we're operating on. Moreover,
when that record expressions/patterns had missing child nodes, we would
continue traversing their ancestor nodes.
This code replaces the thread pool implementation we were using
previously (from the `threadpool` crate). By making the thread pool
aware of QoS, each job spawned on the thread pool can have a different
QoS class.
This commit also replaces every QoS class used previously with Default
as a temporary measure so that each usage can be chosen deliberately.