mirror of
https://github.com/rust-lang/rust-analyzer
synced 2024-11-16 09:48:10 +00:00
Merge #9498
9498: feat: Yeet `replace_unwrap_with_match` in favor of `inline_call` r=Veykril a=Veykril `inline_call` can basically do this job now and more. 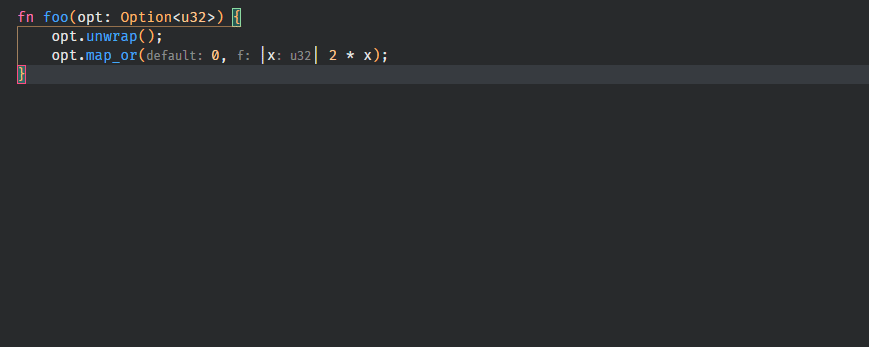 Co-authored-by: Lukas Wirth <lukastw97@gmail.com>
This commit is contained in:
commit
c7b1973a1d
3 changed files with 0 additions and 211 deletions
|
@ -1,186 +0,0 @@
|
|||
use std::iter;
|
||||
|
||||
use syntax::{
|
||||
ast::{
|
||||
self,
|
||||
edit::{AstNodeEdit, IndentLevel},
|
||||
make,
|
||||
},
|
||||
AstNode,
|
||||
};
|
||||
|
||||
use crate::{
|
||||
utils::{render_snippet, Cursor},
|
||||
AssistContext, AssistId, AssistKind, Assists,
|
||||
};
|
||||
use ide_db::ty_filter::TryEnum;
|
||||
|
||||
// Assist: replace_unwrap_with_match
|
||||
//
|
||||
// Replaces `unwrap` with a `match` expression. Works for Result and Option.
|
||||
//
|
||||
// ```
|
||||
// # //- minicore: result
|
||||
// fn main() {
|
||||
// let x: Result<i32, i32> = Ok(92);
|
||||
// let y = x.$0unwrap();
|
||||
// }
|
||||
// ```
|
||||
// ->
|
||||
// ```
|
||||
// fn main() {
|
||||
// let x: Result<i32, i32> = Ok(92);
|
||||
// let y = match x {
|
||||
// Ok(it) => it,
|
||||
// $0_ => unreachable!(),
|
||||
// };
|
||||
// }
|
||||
// ```
|
||||
pub(crate) fn replace_unwrap_with_match(acc: &mut Assists, ctx: &AssistContext) -> Option<()> {
|
||||
let method_call: ast::MethodCallExpr = ctx.find_node_at_offset()?;
|
||||
let name = method_call.name_ref()?;
|
||||
if name.text() != "unwrap" {
|
||||
return None;
|
||||
}
|
||||
let caller = method_call.receiver()?;
|
||||
let ty = ctx.sema.type_of_expr(&caller)?;
|
||||
let happy_variant = TryEnum::from_ty(&ctx.sema, &ty)?.happy_case();
|
||||
let target = method_call.syntax().text_range();
|
||||
acc.add(
|
||||
AssistId("replace_unwrap_with_match", AssistKind::RefactorRewrite),
|
||||
"Replace unwrap with match",
|
||||
target,
|
||||
|builder| {
|
||||
let ok_path = make::ext::ident_path(happy_variant);
|
||||
let it = make::ext::simple_ident_pat(make::name("it")).into();
|
||||
let ok_tuple = make::tuple_struct_pat(ok_path, iter::once(it)).into();
|
||||
|
||||
let bind_path = make::ext::ident_path("it");
|
||||
let ok_arm = make::match_arm(iter::once(ok_tuple), None, make::expr_path(bind_path));
|
||||
|
||||
let err_arm = make::match_arm(
|
||||
iter::once(make::wildcard_pat().into()),
|
||||
None,
|
||||
make::ext::expr_unreachable(),
|
||||
);
|
||||
|
||||
let match_arm_list = make::match_arm_list(vec![ok_arm, err_arm]);
|
||||
let match_expr = make::expr_match(caller.clone(), match_arm_list)
|
||||
.indent(IndentLevel::from_node(method_call.syntax()));
|
||||
|
||||
let range = method_call.syntax().text_range();
|
||||
match ctx.config.snippet_cap {
|
||||
Some(cap) => {
|
||||
let err_arm = match_expr
|
||||
.syntax()
|
||||
.descendants()
|
||||
.filter_map(ast::MatchArm::cast)
|
||||
.last()
|
||||
.unwrap();
|
||||
let snippet =
|
||||
render_snippet(cap, match_expr.syntax(), Cursor::Before(err_arm.syntax()));
|
||||
builder.replace_snippet(cap, range, snippet)
|
||||
}
|
||||
None => builder.replace(range, match_expr.to_string()),
|
||||
}
|
||||
},
|
||||
)
|
||||
}
|
||||
|
||||
#[cfg(test)]
|
||||
mod tests {
|
||||
use crate::tests::{check_assist, check_assist_target};
|
||||
|
||||
use super::*;
|
||||
|
||||
#[test]
|
||||
fn test_replace_result_unwrap_with_match() {
|
||||
check_assist(
|
||||
replace_unwrap_with_match,
|
||||
r#"
|
||||
//- minicore: result
|
||||
fn i<T>(a: T) -> T { a }
|
||||
fn main() {
|
||||
let x: Result<i32, i32> = Ok(92);
|
||||
let y = i(x).$0unwrap();
|
||||
}
|
||||
"#,
|
||||
r#"
|
||||
fn i<T>(a: T) -> T { a }
|
||||
fn main() {
|
||||
let x: Result<i32, i32> = Ok(92);
|
||||
let y = match i(x) {
|
||||
Ok(it) => it,
|
||||
$0_ => unreachable!(),
|
||||
};
|
||||
}
|
||||
"#,
|
||||
)
|
||||
}
|
||||
|
||||
#[test]
|
||||
fn test_replace_option_unwrap_with_match() {
|
||||
check_assist(
|
||||
replace_unwrap_with_match,
|
||||
r#"
|
||||
//- minicore: option
|
||||
fn i<T>(a: T) -> T { a }
|
||||
fn main() {
|
||||
let x = Some(92);
|
||||
let y = i(x).$0unwrap();
|
||||
}
|
||||
"#,
|
||||
r#"
|
||||
fn i<T>(a: T) -> T { a }
|
||||
fn main() {
|
||||
let x = Some(92);
|
||||
let y = match i(x) {
|
||||
Some(it) => it,
|
||||
$0_ => unreachable!(),
|
||||
};
|
||||
}
|
||||
"#,
|
||||
);
|
||||
}
|
||||
|
||||
#[test]
|
||||
fn test_replace_result_unwrap_with_match_chaining() {
|
||||
check_assist(
|
||||
replace_unwrap_with_match,
|
||||
r#"
|
||||
//- minicore: result
|
||||
fn i<T>(a: T) -> T { a }
|
||||
fn main() {
|
||||
let x: Result<i32, i32> = Ok(92);
|
||||
let y = i(x).$0unwrap().count_zeroes();
|
||||
}
|
||||
"#,
|
||||
r#"
|
||||
fn i<T>(a: T) -> T { a }
|
||||
fn main() {
|
||||
let x: Result<i32, i32> = Ok(92);
|
||||
let y = match i(x) {
|
||||
Ok(it) => it,
|
||||
$0_ => unreachable!(),
|
||||
}.count_zeroes();
|
||||
}
|
||||
"#,
|
||||
)
|
||||
}
|
||||
|
||||
#[test]
|
||||
fn replace_unwrap_with_match_target() {
|
||||
check_assist_target(
|
||||
replace_unwrap_with_match,
|
||||
r#"
|
||||
//- minicore: option
|
||||
fn i<T>(a: T) -> T { a }
|
||||
fn main() {
|
||||
let x = Some(92);
|
||||
let y = i(x).$0unwrap();
|
||||
}
|
||||
"#,
|
||||
r"i(x).unwrap()",
|
||||
);
|
||||
}
|
||||
}
|
|
@ -109,7 +109,6 @@ mod handlers {
|
|||
mod replace_let_with_if_let;
|
||||
mod replace_qualified_name_with_use;
|
||||
mod replace_string_with_char;
|
||||
mod replace_unwrap_with_match;
|
||||
mod split_import;
|
||||
mod toggle_ignore;
|
||||
mod unmerge_use;
|
||||
|
@ -182,7 +181,6 @@ mod handlers {
|
|||
replace_impl_trait_with_generic::replace_impl_trait_with_generic,
|
||||
replace_let_with_if_let::replace_let_with_if_let,
|
||||
replace_qualified_name_with_use::replace_qualified_name_with_use,
|
||||
replace_unwrap_with_match::replace_unwrap_with_match,
|
||||
split_import::split_import,
|
||||
toggle_ignore::toggle_ignore,
|
||||
unmerge_use::unmerge_use,
|
||||
|
|
|
@ -1506,29 +1506,6 @@ fn main() {
|
|||
)
|
||||
}
|
||||
|
||||
#[test]
|
||||
fn doctest_replace_unwrap_with_match() {
|
||||
check_doc_test(
|
||||
"replace_unwrap_with_match",
|
||||
r#####"
|
||||
//- minicore: result
|
||||
fn main() {
|
||||
let x: Result<i32, i32> = Ok(92);
|
||||
let y = x.$0unwrap();
|
||||
}
|
||||
"#####,
|
||||
r#####"
|
||||
fn main() {
|
||||
let x: Result<i32, i32> = Ok(92);
|
||||
let y = match x {
|
||||
Ok(it) => it,
|
||||
$0_ => unreachable!(),
|
||||
};
|
||||
}
|
||||
"#####,
|
||||
)
|
||||
}
|
||||
|
||||
#[test]
|
||||
fn doctest_split_import() {
|
||||
check_doc_test(
|
||||
|
|
Loading…
Reference in a new issue