mirror of
https://github.com/rust-lang/rust-analyzer
synced 2024-12-27 21:43:37 +00:00
Merge #3564
3564: Better handling of a few kinds of cargo/clippy diagnostics r=matklad a=kiljacken This was initially supposed to just be a fix for #3433, but I caught a few things that ended up being useful as well. This PR primarily makes us handle multi-edit fix suggestions properly. Instead of just applying the first fix we apply all the parts of the fix in a single action. Second up, this PR handles diagnostics with multiple primary spans, f.x. the unused import diagnostic from rustc:  The LSP doesn't handle this too well, as it only support a single complete range for each diagnostic, so we get duplicate messages in the problem panel of VSCode: 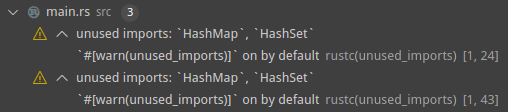 However, I feel like the improved visual aspect in-editor outweighs the duplication in the problem panel. I'm open to not including the second commit if anybody really doesn't like the idea of duplicate diagnostics in the problem pane. Fixes #3433 Fixes #3257 Co-authored-by: Emil Lauridsen <mine809@gmail.com>
This commit is contained in:
commit
7bbdca6182
11 changed files with 729 additions and 465 deletions
|
@ -8,6 +8,7 @@ use lsp_types::{
|
|||
Location, NumberOrString, Position, Range, TextEdit, Url, WorkspaceEdit,
|
||||
};
|
||||
use std::{
|
||||
collections::HashMap,
|
||||
fmt::Write,
|
||||
path::{Component, Path, PathBuf, Prefix},
|
||||
str::FromStr,
|
||||
|
@ -126,44 +127,34 @@ fn map_rust_child_diagnostic(
|
|||
rd: &RustDiagnostic,
|
||||
workspace_root: &PathBuf,
|
||||
) -> MappedRustChildDiagnostic {
|
||||
let span: &DiagnosticSpan = match rd.spans.iter().find(|s| s.is_primary) {
|
||||
Some(span) => span,
|
||||
None => {
|
||||
// `rustc` uses these spanless children as a way to print multi-line
|
||||
// messages
|
||||
return MappedRustChildDiagnostic::MessageLine(rd.message.clone());
|
||||
let spans: Vec<&DiagnosticSpan> = rd.spans.iter().filter(|s| s.is_primary).collect();
|
||||
if spans.is_empty() {
|
||||
// `rustc` uses these spanless children as a way to print multi-line
|
||||
// messages
|
||||
return MappedRustChildDiagnostic::MessageLine(rd.message.clone());
|
||||
}
|
||||
|
||||
let mut edit_map: HashMap<Url, Vec<TextEdit>> = HashMap::new();
|
||||
for &span in &spans {
|
||||
if let Some(suggested_replacement) = &span.suggested_replacement {
|
||||
let location = map_span_to_location(span, workspace_root);
|
||||
let edit = TextEdit::new(location.range, suggested_replacement.clone());
|
||||
edit_map.entry(location.uri).or_default().push(edit);
|
||||
}
|
||||
};
|
||||
|
||||
// If we have a primary span use its location, otherwise use the parent
|
||||
let location = map_span_to_location(&span, workspace_root);
|
||||
|
||||
if let Some(suggested_replacement) = &span.suggested_replacement {
|
||||
// Include our replacement in the title unless it's empty
|
||||
let title = if !suggested_replacement.is_empty() {
|
||||
format!("{}: '{}'", rd.message, suggested_replacement)
|
||||
} else {
|
||||
rd.message.clone()
|
||||
};
|
||||
|
||||
let edit = {
|
||||
let edits = vec![TextEdit::new(location.range, suggested_replacement.clone())];
|
||||
let mut edit_map = std::collections::HashMap::new();
|
||||
edit_map.insert(location.uri, edits);
|
||||
WorkspaceEdit::new(edit_map)
|
||||
};
|
||||
}
|
||||
|
||||
if !edit_map.is_empty() {
|
||||
MappedRustChildDiagnostic::SuggestedFix(CodeAction {
|
||||
title,
|
||||
title: rd.message.clone(),
|
||||
kind: Some("quickfix".to_string()),
|
||||
diagnostics: None,
|
||||
edit: Some(edit),
|
||||
edit: Some(WorkspaceEdit::new(edit_map)),
|
||||
command: None,
|
||||
is_preferred: None,
|
||||
})
|
||||
} else {
|
||||
MappedRustChildDiagnostic::Related(DiagnosticRelatedInformation {
|
||||
location,
|
||||
location: map_span_to_location(spans[0], workspace_root),
|
||||
message: rd.message.clone(),
|
||||
})
|
||||
}
|
||||
|
@ -189,13 +180,13 @@ pub(crate) struct MappedRustDiagnostic {
|
|||
pub(crate) fn map_rust_diagnostic_to_lsp(
|
||||
rd: &RustDiagnostic,
|
||||
workspace_root: &PathBuf,
|
||||
) -> Option<MappedRustDiagnostic> {
|
||||
let primary_span = rd.spans.iter().find(|s| s.is_primary)?;
|
||||
|
||||
let location = map_span_to_location(&primary_span, workspace_root);
|
||||
) -> Vec<MappedRustDiagnostic> {
|
||||
let primary_spans: Vec<&DiagnosticSpan> = rd.spans.iter().filter(|s| s.is_primary).collect();
|
||||
if primary_spans.is_empty() {
|
||||
return vec![];
|
||||
}
|
||||
|
||||
let severity = map_level_to_severity(rd.level);
|
||||
let mut primary_span_label = primary_span.label.as_ref();
|
||||
|
||||
let mut source = String::from("rustc");
|
||||
let mut code = rd.code.as_ref().map(|c| c.code.clone());
|
||||
|
@ -208,19 +199,10 @@ pub(crate) fn map_rust_diagnostic_to_lsp(
|
|||
}
|
||||
}
|
||||
|
||||
let mut needs_primary_span_label = true;
|
||||
let mut related_information = vec![];
|
||||
let mut tags = vec![];
|
||||
|
||||
// If error occurs from macro expansion, add related info pointing to
|
||||
// where the error originated
|
||||
if !is_from_macro(&primary_span.file_name) && primary_span.expansion.is_some() {
|
||||
let def_loc = map_span_to_location_naive(&primary_span, workspace_root);
|
||||
related_information.push(DiagnosticRelatedInformation {
|
||||
location: def_loc,
|
||||
message: "Error originated from macro here".to_string(),
|
||||
});
|
||||
}
|
||||
|
||||
for secondary_span in rd.spans.iter().filter(|s| !s.is_primary) {
|
||||
let related = map_secondary_span_to_related(secondary_span, workspace_root);
|
||||
if let Some(related) = related {
|
||||
|
@ -240,15 +222,11 @@ pub(crate) fn map_rust_diagnostic_to_lsp(
|
|||
|
||||
// These secondary messages usually duplicate the content of the
|
||||
// primary span label.
|
||||
primary_span_label = None;
|
||||
needs_primary_span_label = false;
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
if let Some(primary_span_label) = primary_span_label {
|
||||
write!(&mut message, "\n{}", primary_span_label).unwrap();
|
||||
}
|
||||
|
||||
if is_unused_or_unnecessary(rd) {
|
||||
tags.push(DiagnosticTag::Unnecessary);
|
||||
}
|
||||
|
@ -257,21 +235,45 @@ pub(crate) fn map_rust_diagnostic_to_lsp(
|
|||
tags.push(DiagnosticTag::Deprecated);
|
||||
}
|
||||
|
||||
let diagnostic = Diagnostic {
|
||||
range: location.range,
|
||||
severity,
|
||||
code: code.map(NumberOrString::String),
|
||||
source: Some(source),
|
||||
message,
|
||||
related_information: if !related_information.is_empty() {
|
||||
Some(related_information)
|
||||
} else {
|
||||
None
|
||||
},
|
||||
tags: if !tags.is_empty() { Some(tags) } else { None },
|
||||
};
|
||||
primary_spans
|
||||
.iter()
|
||||
.map(|primary_span| {
|
||||
let location = map_span_to_location(&primary_span, workspace_root);
|
||||
|
||||
Some(MappedRustDiagnostic { location, diagnostic, fixes })
|
||||
let mut message = message.clone();
|
||||
if needs_primary_span_label {
|
||||
if let Some(primary_span_label) = &primary_span.label {
|
||||
write!(&mut message, "\n{}", primary_span_label).unwrap();
|
||||
}
|
||||
}
|
||||
|
||||
// If error occurs from macro expansion, add related info pointing to
|
||||
// where the error originated
|
||||
if !is_from_macro(&primary_span.file_name) && primary_span.expansion.is_some() {
|
||||
let def_loc = map_span_to_location_naive(&primary_span, workspace_root);
|
||||
related_information.push(DiagnosticRelatedInformation {
|
||||
location: def_loc,
|
||||
message: "Error originated from macro here".to_string(),
|
||||
});
|
||||
}
|
||||
|
||||
let diagnostic = Diagnostic {
|
||||
range: location.range,
|
||||
severity,
|
||||
code: code.clone().map(NumberOrString::String),
|
||||
source: Some(source.clone()),
|
||||
message,
|
||||
related_information: if !related_information.is_empty() {
|
||||
Some(related_information.clone())
|
||||
} else {
|
||||
None
|
||||
},
|
||||
tags: if !tags.is_empty() { Some(tags.clone()) } else { None },
|
||||
};
|
||||
|
||||
MappedRustDiagnostic { location, diagnostic, fixes: fixes.clone() }
|
||||
})
|
||||
.collect()
|
||||
}
|
||||
|
||||
/// Returns a `Url` object from a given path, will lowercase drive letters if present.
|
||||
|
|
|
@ -2,98 +2,100 @@
|
|||
source: crates/ra_cargo_watch/src/conv/test.rs
|
||||
expression: diag
|
||||
---
|
||||
MappedRustDiagnostic {
|
||||
location: Location {
|
||||
uri: "file:///test/compiler/mir/tagset.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 41,
|
||||
character: 23,
|
||||
},
|
||||
end: Position {
|
||||
line: 41,
|
||||
character: 28,
|
||||
},
|
||||
},
|
||||
},
|
||||
diagnostic: Diagnostic {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 41,
|
||||
character: 23,
|
||||
},
|
||||
end: Position {
|
||||
line: 41,
|
||||
character: 28,
|
||||
},
|
||||
},
|
||||
severity: Some(
|
||||
Warning,
|
||||
),
|
||||
code: Some(
|
||||
String(
|
||||
"trivially_copy_pass_by_ref",
|
||||
),
|
||||
),
|
||||
source: Some(
|
||||
"clippy",
|
||||
),
|
||||
message: "this argument is passed by reference, but would be more efficient if passed by value\n#[warn(clippy::trivially_copy_pass_by_ref)] implied by #[warn(clippy::all)]\nfor further information visit https://rust-lang.github.io/rust-clippy/master/index.html#trivially_copy_pass_by_ref",
|
||||
related_information: Some(
|
||||
[
|
||||
DiagnosticRelatedInformation {
|
||||
location: Location {
|
||||
uri: "file:///test/compiler/lib.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 0,
|
||||
character: 8,
|
||||
},
|
||||
end: Position {
|
||||
line: 0,
|
||||
character: 19,
|
||||
},
|
||||
},
|
||||
},
|
||||
message: "lint level defined here",
|
||||
[
|
||||
MappedRustDiagnostic {
|
||||
location: Location {
|
||||
uri: "file:///test/compiler/mir/tagset.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 41,
|
||||
character: 23,
|
||||
},
|
||||
],
|
||||
),
|
||||
tags: None,
|
||||
},
|
||||
fixes: [
|
||||
CodeAction {
|
||||
title: "consider passing by value instead: \'self\'",
|
||||
kind: Some(
|
||||
"quickfix",
|
||||
end: Position {
|
||||
line: 41,
|
||||
character: 28,
|
||||
},
|
||||
},
|
||||
},
|
||||
diagnostic: Diagnostic {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 41,
|
||||
character: 23,
|
||||
},
|
||||
end: Position {
|
||||
line: 41,
|
||||
character: 28,
|
||||
},
|
||||
},
|
||||
severity: Some(
|
||||
Warning,
|
||||
),
|
||||
diagnostics: None,
|
||||
edit: Some(
|
||||
WorkspaceEdit {
|
||||
changes: Some(
|
||||
{
|
||||
"file:///test/compiler/mir/tagset.rs": [
|
||||
TextEdit {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 41,
|
||||
character: 23,
|
||||
},
|
||||
end: Position {
|
||||
line: 41,
|
||||
character: 28,
|
||||
},
|
||||
},
|
||||
new_text: "self",
|
||||
code: Some(
|
||||
String(
|
||||
"trivially_copy_pass_by_ref",
|
||||
),
|
||||
),
|
||||
source: Some(
|
||||
"clippy",
|
||||
),
|
||||
message: "this argument is passed by reference, but would be more efficient if passed by value\n#[warn(clippy::trivially_copy_pass_by_ref)] implied by #[warn(clippy::all)]\nfor further information visit https://rust-lang.github.io/rust-clippy/master/index.html#trivially_copy_pass_by_ref",
|
||||
related_information: Some(
|
||||
[
|
||||
DiagnosticRelatedInformation {
|
||||
location: Location {
|
||||
uri: "file:///test/compiler/lib.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 0,
|
||||
character: 8,
|
||||
},
|
||||
],
|
||||
end: Position {
|
||||
line: 0,
|
||||
character: 19,
|
||||
},
|
||||
},
|
||||
},
|
||||
),
|
||||
document_changes: None,
|
||||
},
|
||||
message: "lint level defined here",
|
||||
},
|
||||
],
|
||||
),
|
||||
command: None,
|
||||
is_preferred: None,
|
||||
tags: None,
|
||||
},
|
||||
],
|
||||
}
|
||||
fixes: [
|
||||
CodeAction {
|
||||
title: "consider passing by value instead",
|
||||
kind: Some(
|
||||
"quickfix",
|
||||
),
|
||||
diagnostics: None,
|
||||
edit: Some(
|
||||
WorkspaceEdit {
|
||||
changes: Some(
|
||||
{
|
||||
"file:///test/compiler/mir/tagset.rs": [
|
||||
TextEdit {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 41,
|
||||
character: 23,
|
||||
},
|
||||
end: Position {
|
||||
line: 41,
|
||||
character: 28,
|
||||
},
|
||||
},
|
||||
new_text: "self",
|
||||
},
|
||||
],
|
||||
},
|
||||
),
|
||||
document_changes: None,
|
||||
},
|
||||
),
|
||||
command: None,
|
||||
is_preferred: None,
|
||||
},
|
||||
],
|
||||
},
|
||||
]
|
||||
|
|
|
@ -2,45 +2,47 @@
|
|||
source: crates/ra_cargo_watch/src/conv/test.rs
|
||||
expression: diag
|
||||
---
|
||||
MappedRustDiagnostic {
|
||||
location: Location {
|
||||
uri: "file:///test/src/main.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 1,
|
||||
character: 4,
|
||||
},
|
||||
end: Position {
|
||||
line: 1,
|
||||
character: 26,
|
||||
[
|
||||
MappedRustDiagnostic {
|
||||
location: Location {
|
||||
uri: "file:///test/src/main.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 1,
|
||||
character: 4,
|
||||
},
|
||||
end: Position {
|
||||
line: 1,
|
||||
character: 26,
|
||||
},
|
||||
},
|
||||
},
|
||||
},
|
||||
diagnostic: Diagnostic {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 1,
|
||||
character: 4,
|
||||
diagnostic: Diagnostic {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 1,
|
||||
character: 4,
|
||||
},
|
||||
end: Position {
|
||||
line: 1,
|
||||
character: 26,
|
||||
},
|
||||
},
|
||||
end: Position {
|
||||
line: 1,
|
||||
character: 26,
|
||||
},
|
||||
},
|
||||
severity: Some(
|
||||
Error,
|
||||
),
|
||||
code: Some(
|
||||
String(
|
||||
"E0277",
|
||||
severity: Some(
|
||||
Error,
|
||||
),
|
||||
),
|
||||
source: Some(
|
||||
"rustc",
|
||||
),
|
||||
message: "can\'t compare `{integer}` with `&str`\nthe trait `std::cmp::PartialEq<&str>` is not implemented for `{integer}`",
|
||||
related_information: None,
|
||||
tags: None,
|
||||
code: Some(
|
||||
String(
|
||||
"E0277",
|
||||
),
|
||||
),
|
||||
source: Some(
|
||||
"rustc",
|
||||
),
|
||||
message: "can\'t compare `{integer}` with `&str`\nthe trait `std::cmp::PartialEq<&str>` is not implemented for `{integer}`",
|
||||
related_information: None,
|
||||
tags: None,
|
||||
},
|
||||
fixes: [],
|
||||
},
|
||||
fixes: [],
|
||||
}
|
||||
]
|
||||
|
|
|
@ -2,60 +2,62 @@
|
|||
source: crates/ra_cargo_watch/src/conv/test.rs
|
||||
expression: diag
|
||||
---
|
||||
MappedRustDiagnostic {
|
||||
location: Location {
|
||||
uri: "file:///test/crates/ra_hir_def/src/data.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 79,
|
||||
character: 15,
|
||||
},
|
||||
end: Position {
|
||||
line: 79,
|
||||
character: 41,
|
||||
[
|
||||
MappedRustDiagnostic {
|
||||
location: Location {
|
||||
uri: "file:///test/crates/ra_hir_def/src/data.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 79,
|
||||
character: 15,
|
||||
},
|
||||
end: Position {
|
||||
line: 79,
|
||||
character: 41,
|
||||
},
|
||||
},
|
||||
},
|
||||
},
|
||||
diagnostic: Diagnostic {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 79,
|
||||
character: 15,
|
||||
diagnostic: Diagnostic {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 79,
|
||||
character: 15,
|
||||
},
|
||||
end: Position {
|
||||
line: 79,
|
||||
character: 41,
|
||||
},
|
||||
},
|
||||
end: Position {
|
||||
line: 79,
|
||||
character: 41,
|
||||
},
|
||||
},
|
||||
severity: Some(
|
||||
Error,
|
||||
),
|
||||
code: None,
|
||||
source: Some(
|
||||
"rustc",
|
||||
),
|
||||
message: "Please register your known path in the path module",
|
||||
related_information: Some(
|
||||
[
|
||||
DiagnosticRelatedInformation {
|
||||
location: Location {
|
||||
uri: "file:///test/crates/ra_hir_def/src/path.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 264,
|
||||
character: 8,
|
||||
},
|
||||
end: Position {
|
||||
line: 264,
|
||||
character: 76,
|
||||
severity: Some(
|
||||
Error,
|
||||
),
|
||||
code: None,
|
||||
source: Some(
|
||||
"rustc",
|
||||
),
|
||||
message: "Please register your known path in the path module",
|
||||
related_information: Some(
|
||||
[
|
||||
DiagnosticRelatedInformation {
|
||||
location: Location {
|
||||
uri: "file:///test/crates/ra_hir_def/src/path.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 264,
|
||||
character: 8,
|
||||
},
|
||||
end: Position {
|
||||
line: 264,
|
||||
character: 76,
|
||||
},
|
||||
},
|
||||
},
|
||||
message: "Error originated from macro here",
|
||||
},
|
||||
message: "Error originated from macro here",
|
||||
},
|
||||
],
|
||||
),
|
||||
tags: None,
|
||||
],
|
||||
),
|
||||
tags: None,
|
||||
},
|
||||
fixes: [],
|
||||
},
|
||||
fixes: [],
|
||||
}
|
||||
]
|
||||
|
|
|
@ -0,0 +1,114 @@
|
|||
---
|
||||
source: crates/ra_cargo_watch/src/conv/test.rs
|
||||
expression: diag
|
||||
---
|
||||
[
|
||||
MappedRustDiagnostic {
|
||||
location: Location {
|
||||
uri: "file:///test/src/main.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 3,
|
||||
character: 4,
|
||||
},
|
||||
end: Position {
|
||||
line: 3,
|
||||
character: 5,
|
||||
},
|
||||
},
|
||||
},
|
||||
diagnostic: Diagnostic {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 3,
|
||||
character: 4,
|
||||
},
|
||||
end: Position {
|
||||
line: 3,
|
||||
character: 5,
|
||||
},
|
||||
},
|
||||
severity: Some(
|
||||
Warning,
|
||||
),
|
||||
code: Some(
|
||||
String(
|
||||
"let_and_return",
|
||||
),
|
||||
),
|
||||
source: Some(
|
||||
"clippy",
|
||||
),
|
||||
message: "returning the result of a let binding from a block\n`#[warn(clippy::let_and_return)]` on by default\nfor further information visit https://rust-lang.github.io/rust-clippy/master/index.html#let_and_return",
|
||||
related_information: Some(
|
||||
[
|
||||
DiagnosticRelatedInformation {
|
||||
location: Location {
|
||||
uri: "file:///test/src/main.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 2,
|
||||
character: 4,
|
||||
},
|
||||
end: Position {
|
||||
line: 2,
|
||||
character: 30,
|
||||
},
|
||||
},
|
||||
},
|
||||
message: "unnecessary let binding",
|
||||
},
|
||||
],
|
||||
),
|
||||
tags: None,
|
||||
},
|
||||
fixes: [
|
||||
CodeAction {
|
||||
title: "return the expression directly",
|
||||
kind: Some(
|
||||
"quickfix",
|
||||
),
|
||||
diagnostics: None,
|
||||
edit: Some(
|
||||
WorkspaceEdit {
|
||||
changes: Some(
|
||||
{
|
||||
"file:///test/src/main.rs": [
|
||||
TextEdit {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 2,
|
||||
character: 4,
|
||||
},
|
||||
end: Position {
|
||||
line: 2,
|
||||
character: 30,
|
||||
},
|
||||
},
|
||||
new_text: "",
|
||||
},
|
||||
TextEdit {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 3,
|
||||
character: 4,
|
||||
},
|
||||
end: Position {
|
||||
line: 3,
|
||||
character: 5,
|
||||
},
|
||||
},
|
||||
new_text: "(0..10).collect()",
|
||||
},
|
||||
],
|
||||
},
|
||||
),
|
||||
document_changes: None,
|
||||
},
|
||||
),
|
||||
command: None,
|
||||
is_preferred: None,
|
||||
},
|
||||
],
|
||||
},
|
||||
]
|
|
@ -2,45 +2,47 @@
|
|||
source: crates/ra_cargo_watch/src/conv/test.rs
|
||||
expression: diag
|
||||
---
|
||||
MappedRustDiagnostic {
|
||||
location: Location {
|
||||
uri: "file:///test/compiler/ty/list_iter.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 51,
|
||||
character: 4,
|
||||
},
|
||||
end: Position {
|
||||
line: 51,
|
||||
character: 47,
|
||||
[
|
||||
MappedRustDiagnostic {
|
||||
location: Location {
|
||||
uri: "file:///test/compiler/ty/list_iter.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 51,
|
||||
character: 4,
|
||||
},
|
||||
end: Position {
|
||||
line: 51,
|
||||
character: 47,
|
||||
},
|
||||
},
|
||||
},
|
||||
},
|
||||
diagnostic: Diagnostic {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 51,
|
||||
character: 4,
|
||||
diagnostic: Diagnostic {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 51,
|
||||
character: 4,
|
||||
},
|
||||
end: Position {
|
||||
line: 51,
|
||||
character: 47,
|
||||
},
|
||||
},
|
||||
end: Position {
|
||||
line: 51,
|
||||
character: 47,
|
||||
},
|
||||
},
|
||||
severity: Some(
|
||||
Error,
|
||||
),
|
||||
code: Some(
|
||||
String(
|
||||
"E0053",
|
||||
severity: Some(
|
||||
Error,
|
||||
),
|
||||
),
|
||||
source: Some(
|
||||
"rustc",
|
||||
),
|
||||
message: "method `next` has an incompatible type for trait\nexpected type `fn(&mut ty::list_iter::ListIterator<\'list, M>) -> std::option::Option<&ty::Ref<M>>`\n found type `fn(&ty::list_iter::ListIterator<\'list, M>) -> std::option::Option<&\'list ty::Ref<M>>`",
|
||||
related_information: None,
|
||||
tags: None,
|
||||
code: Some(
|
||||
String(
|
||||
"E0053",
|
||||
),
|
||||
),
|
||||
source: Some(
|
||||
"rustc",
|
||||
),
|
||||
message: "method `next` has an incompatible type for trait\nexpected type `fn(&mut ty::list_iter::ListIterator<\'list, M>) -> std::option::Option<&ty::Ref<M>>`\n found type `fn(&ty::list_iter::ListIterator<\'list, M>) -> std::option::Option<&\'list ty::Ref<M>>`",
|
||||
related_information: None,
|
||||
tags: None,
|
||||
},
|
||||
fixes: [],
|
||||
},
|
||||
fixes: [],
|
||||
}
|
||||
]
|
||||
|
|
|
@ -2,45 +2,47 @@
|
|||
source: crates/ra_cargo_watch/src/conv/test.rs
|
||||
expression: diag
|
||||
---
|
||||
MappedRustDiagnostic {
|
||||
location: Location {
|
||||
uri: "file:///test/runtime/compiler_support.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 47,
|
||||
character: 64,
|
||||
},
|
||||
end: Position {
|
||||
line: 47,
|
||||
character: 69,
|
||||
[
|
||||
MappedRustDiagnostic {
|
||||
location: Location {
|
||||
uri: "file:///test/runtime/compiler_support.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 47,
|
||||
character: 64,
|
||||
},
|
||||
end: Position {
|
||||
line: 47,
|
||||
character: 69,
|
||||
},
|
||||
},
|
||||
},
|
||||
},
|
||||
diagnostic: Diagnostic {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 47,
|
||||
character: 64,
|
||||
diagnostic: Diagnostic {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 47,
|
||||
character: 64,
|
||||
},
|
||||
end: Position {
|
||||
line: 47,
|
||||
character: 69,
|
||||
},
|
||||
},
|
||||
end: Position {
|
||||
line: 47,
|
||||
character: 69,
|
||||
},
|
||||
},
|
||||
severity: Some(
|
||||
Error,
|
||||
),
|
||||
code: Some(
|
||||
String(
|
||||
"E0308",
|
||||
severity: Some(
|
||||
Error,
|
||||
),
|
||||
),
|
||||
source: Some(
|
||||
"rustc",
|
||||
),
|
||||
message: "mismatched types\nexpected usize, found u32",
|
||||
related_information: None,
|
||||
tags: None,
|
||||
code: Some(
|
||||
String(
|
||||
"E0308",
|
||||
),
|
||||
),
|
||||
source: Some(
|
||||
"rustc",
|
||||
),
|
||||
message: "mismatched types\nexpected usize, found u32",
|
||||
related_information: None,
|
||||
tags: None,
|
||||
},
|
||||
fixes: [],
|
||||
},
|
||||
fixes: [],
|
||||
}
|
||||
]
|
||||
|
|
|
@ -2,83 +2,85 @@
|
|||
source: crates/ra_cargo_watch/src/conv/test.rs
|
||||
expression: diag
|
||||
---
|
||||
MappedRustDiagnostic {
|
||||
location: Location {
|
||||
uri: "file:///test/driver/subcommand/repl.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 290,
|
||||
character: 8,
|
||||
},
|
||||
end: Position {
|
||||
line: 290,
|
||||
character: 11,
|
||||
},
|
||||
},
|
||||
},
|
||||
diagnostic: Diagnostic {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 290,
|
||||
character: 8,
|
||||
},
|
||||
end: Position {
|
||||
line: 290,
|
||||
character: 11,
|
||||
},
|
||||
},
|
||||
severity: Some(
|
||||
Warning,
|
||||
),
|
||||
code: Some(
|
||||
String(
|
||||
"unused_variables",
|
||||
),
|
||||
),
|
||||
source: Some(
|
||||
"rustc",
|
||||
),
|
||||
message: "unused variable: `foo`\n#[warn(unused_variables)] on by default",
|
||||
related_information: None,
|
||||
tags: Some(
|
||||
[
|
||||
Unnecessary,
|
||||
],
|
||||
),
|
||||
},
|
||||
fixes: [
|
||||
CodeAction {
|
||||
title: "consider prefixing with an underscore: \'_foo\'",
|
||||
kind: Some(
|
||||
"quickfix",
|
||||
),
|
||||
diagnostics: None,
|
||||
edit: Some(
|
||||
WorkspaceEdit {
|
||||
changes: Some(
|
||||
{
|
||||
"file:///test/driver/subcommand/repl.rs": [
|
||||
TextEdit {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 290,
|
||||
character: 8,
|
||||
},
|
||||
end: Position {
|
||||
line: 290,
|
||||
character: 11,
|
||||
},
|
||||
},
|
||||
new_text: "_foo",
|
||||
},
|
||||
],
|
||||
},
|
||||
),
|
||||
document_changes: None,
|
||||
[
|
||||
MappedRustDiagnostic {
|
||||
location: Location {
|
||||
uri: "file:///test/driver/subcommand/repl.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 290,
|
||||
character: 8,
|
||||
},
|
||||
),
|
||||
command: None,
|
||||
is_preferred: None,
|
||||
end: Position {
|
||||
line: 290,
|
||||
character: 11,
|
||||
},
|
||||
},
|
||||
},
|
||||
],
|
||||
}
|
||||
diagnostic: Diagnostic {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 290,
|
||||
character: 8,
|
||||
},
|
||||
end: Position {
|
||||
line: 290,
|
||||
character: 11,
|
||||
},
|
||||
},
|
||||
severity: Some(
|
||||
Warning,
|
||||
),
|
||||
code: Some(
|
||||
String(
|
||||
"unused_variables",
|
||||
),
|
||||
),
|
||||
source: Some(
|
||||
"rustc",
|
||||
),
|
||||
message: "unused variable: `foo`\n#[warn(unused_variables)] on by default",
|
||||
related_information: None,
|
||||
tags: Some(
|
||||
[
|
||||
Unnecessary,
|
||||
],
|
||||
),
|
||||
},
|
||||
fixes: [
|
||||
CodeAction {
|
||||
title: "consider prefixing with an underscore",
|
||||
kind: Some(
|
||||
"quickfix",
|
||||
),
|
||||
diagnostics: None,
|
||||
edit: Some(
|
||||
WorkspaceEdit {
|
||||
changes: Some(
|
||||
{
|
||||
"file:///test/driver/subcommand/repl.rs": [
|
||||
TextEdit {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 290,
|
||||
character: 8,
|
||||
},
|
||||
end: Position {
|
||||
line: 290,
|
||||
character: 11,
|
||||
},
|
||||
},
|
||||
new_text: "_foo",
|
||||
},
|
||||
],
|
||||
},
|
||||
),
|
||||
document_changes: None,
|
||||
},
|
||||
),
|
||||
command: None,
|
||||
is_preferred: None,
|
||||
},
|
||||
],
|
||||
},
|
||||
]
|
||||
|
|
|
@ -2,64 +2,66 @@
|
|||
source: crates/ra_cargo_watch/src/conv/test.rs
|
||||
expression: diag
|
||||
---
|
||||
MappedRustDiagnostic {
|
||||
location: Location {
|
||||
uri: "file:///test/compiler/ty/select.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 103,
|
||||
character: 17,
|
||||
},
|
||||
end: Position {
|
||||
line: 103,
|
||||
character: 29,
|
||||
[
|
||||
MappedRustDiagnostic {
|
||||
location: Location {
|
||||
uri: "file:///test/compiler/ty/select.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 103,
|
||||
character: 17,
|
||||
},
|
||||
end: Position {
|
||||
line: 103,
|
||||
character: 29,
|
||||
},
|
||||
},
|
||||
},
|
||||
},
|
||||
diagnostic: Diagnostic {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 103,
|
||||
character: 17,
|
||||
diagnostic: Diagnostic {
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 103,
|
||||
character: 17,
|
||||
},
|
||||
end: Position {
|
||||
line: 103,
|
||||
character: 29,
|
||||
},
|
||||
},
|
||||
end: Position {
|
||||
line: 103,
|
||||
character: 29,
|
||||
},
|
||||
},
|
||||
severity: Some(
|
||||
Error,
|
||||
),
|
||||
code: Some(
|
||||
String(
|
||||
"E0061",
|
||||
severity: Some(
|
||||
Error,
|
||||
),
|
||||
),
|
||||
source: Some(
|
||||
"rustc",
|
||||
),
|
||||
message: "this function takes 2 parameters but 3 parameters were supplied\nexpected 2 parameters",
|
||||
related_information: Some(
|
||||
[
|
||||
DiagnosticRelatedInformation {
|
||||
location: Location {
|
||||
uri: "file:///test/compiler/ty/select.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 218,
|
||||
character: 4,
|
||||
},
|
||||
end: Position {
|
||||
line: 230,
|
||||
character: 5,
|
||||
code: Some(
|
||||
String(
|
||||
"E0061",
|
||||
),
|
||||
),
|
||||
source: Some(
|
||||
"rustc",
|
||||
),
|
||||
message: "this function takes 2 parameters but 3 parameters were supplied\nexpected 2 parameters",
|
||||
related_information: Some(
|
||||
[
|
||||
DiagnosticRelatedInformation {
|
||||
location: Location {
|
||||
uri: "file:///test/compiler/ty/select.rs",
|
||||
range: Range {
|
||||
start: Position {
|
||||
line: 218,
|
||||
character: 4,
|
||||
},
|
||||
end: Position {
|
||||
line: 230,
|
||||
character: 5,
|
||||
},
|
||||
},
|
||||
},
|
||||
message: "defined here",
|
||||
},
|
||||
message: "defined here",
|
||||
},
|
||||
],
|
||||
),
|
||||
tags: None,
|
||||
],
|
||||
),
|
||||
tags: None,
|
||||
},
|
||||
fixes: [],
|
||||
},
|
||||
fixes: [],
|
||||
}
|
||||
]
|
||||
|
|
|
@ -58,7 +58,7 @@ fn snap_rustc_incompatible_type_for_trait() {
|
|||
);
|
||||
|
||||
let workspace_root = PathBuf::from("/test/");
|
||||
let diag = map_rust_diagnostic_to_lsp(&diag, &workspace_root).expect("couldn't map diagnostic");
|
||||
let diag = map_rust_diagnostic_to_lsp(&diag, &workspace_root);
|
||||
insta::assert_debug_snapshot!(diag);
|
||||
}
|
||||
|
||||
|
@ -141,7 +141,7 @@ fn snap_rustc_unused_variable() {
|
|||
);
|
||||
|
||||
let workspace_root = PathBuf::from("/test/");
|
||||
let diag = map_rust_diagnostic_to_lsp(&diag, &workspace_root).expect("couldn't map diagnostic");
|
||||
let diag = map_rust_diagnostic_to_lsp(&diag, &workspace_root);
|
||||
insta::assert_debug_snapshot!(diag);
|
||||
}
|
||||
|
||||
|
@ -266,7 +266,7 @@ fn snap_rustc_wrong_number_of_parameters() {
|
|||
);
|
||||
|
||||
let workspace_root = PathBuf::from("/test/");
|
||||
let diag = map_rust_diagnostic_to_lsp(&diag, &workspace_root).expect("couldn't map diagnostic");
|
||||
let diag = map_rust_diagnostic_to_lsp(&diag, &workspace_root);
|
||||
insta::assert_debug_snapshot!(diag);
|
||||
}
|
||||
|
||||
|
@ -387,7 +387,7 @@ fn snap_clippy_pass_by_ref() {
|
|||
);
|
||||
|
||||
let workspace_root = PathBuf::from("/test/");
|
||||
let diag = map_rust_diagnostic_to_lsp(&diag, &workspace_root).expect("couldn't map diagnostic");
|
||||
let diag = map_rust_diagnostic_to_lsp(&diag, &workspace_root);
|
||||
insta::assert_debug_snapshot!(diag);
|
||||
}
|
||||
|
||||
|
@ -431,7 +431,7 @@ fn snap_rustc_mismatched_type() {
|
|||
);
|
||||
|
||||
let workspace_root = PathBuf::from("/test/");
|
||||
let diag = map_rust_diagnostic_to_lsp(&diag, &workspace_root).expect("couldn't map diagnostic");
|
||||
let diag = map_rust_diagnostic_to_lsp(&diag, &workspace_root);
|
||||
insta::assert_debug_snapshot!(diag);
|
||||
}
|
||||
|
||||
|
@ -703,7 +703,7 @@ fn snap_handles_macro_location() {
|
|||
);
|
||||
|
||||
let workspace_root = PathBuf::from("/test/");
|
||||
let diag = map_rust_diagnostic_to_lsp(&diag, &workspace_root).expect("couldn't map diagnostic");
|
||||
let diag = map_rust_diagnostic_to_lsp(&diag, &workspace_root);
|
||||
insta::assert_debug_snapshot!(diag);
|
||||
}
|
||||
|
||||
|
@ -933,6 +933,140 @@ fn snap_macro_compiler_error() {
|
|||
);
|
||||
|
||||
let workspace_root = PathBuf::from("/test/");
|
||||
let diag = map_rust_diagnostic_to_lsp(&diag, &workspace_root).expect("couldn't map diagnostic");
|
||||
let diag = map_rust_diagnostic_to_lsp(&diag, &workspace_root);
|
||||
insta::assert_debug_snapshot!(diag);
|
||||
}
|
||||
|
||||
#[test]
|
||||
#[cfg(not(windows))]
|
||||
fn snap_multi_line_fix() {
|
||||
let diag = parse_diagnostic(
|
||||
r##"{
|
||||
"rendered": "warning: returning the result of a let binding from a block\n --> src/main.rs:4:5\n |\n3 | let a = (0..10).collect();\n | -------------------------- unnecessary let binding\n4 | a\n | ^\n |\n = note: `#[warn(clippy::let_and_return)]` on by default\n = help: for further information visit https://rust-lang.github.io/rust-clippy/master/index.html#let_and_return\nhelp: return the expression directly\n |\n3 | \n4 | (0..10).collect()\n |\n\n",
|
||||
"children": [
|
||||
{
|
||||
"children": [],
|
||||
"code": null,
|
||||
"level": "note",
|
||||
"message": "`#[warn(clippy::let_and_return)]` on by default",
|
||||
"rendered": null,
|
||||
"spans": []
|
||||
},
|
||||
{
|
||||
"children": [],
|
||||
"code": null,
|
||||
"level": "help",
|
||||
"message": "for further information visit https://rust-lang.github.io/rust-clippy/master/index.html#let_and_return",
|
||||
"rendered": null,
|
||||
"spans": []
|
||||
},
|
||||
{
|
||||
"children": [],
|
||||
"code": null,
|
||||
"level": "help",
|
||||
"message": "return the expression directly",
|
||||
"rendered": null,
|
||||
"spans": [
|
||||
{
|
||||
"byte_end": 55,
|
||||
"byte_start": 29,
|
||||
"column_end": 31,
|
||||
"column_start": 5,
|
||||
"expansion": null,
|
||||
"file_name": "src/main.rs",
|
||||
"is_primary": true,
|
||||
"label": null,
|
||||
"line_end": 3,
|
||||
"line_start": 3,
|
||||
"suggested_replacement": "",
|
||||
"suggestion_applicability": "MachineApplicable",
|
||||
"text": [
|
||||
{
|
||||
"highlight_end": 31,
|
||||
"highlight_start": 5,
|
||||
"text": " let a = (0..10).collect();"
|
||||
}
|
||||
]
|
||||
},
|
||||
{
|
||||
"byte_end": 61,
|
||||
"byte_start": 60,
|
||||
"column_end": 6,
|
||||
"column_start": 5,
|
||||
"expansion": null,
|
||||
"file_name": "src/main.rs",
|
||||
"is_primary": true,
|
||||
"label": null,
|
||||
"line_end": 4,
|
||||
"line_start": 4,
|
||||
"suggested_replacement": "(0..10).collect()",
|
||||
"suggestion_applicability": "MachineApplicable",
|
||||
"text": [
|
||||
{
|
||||
"highlight_end": 6,
|
||||
"highlight_start": 5,
|
||||
"text": " a"
|
||||
}
|
||||
]
|
||||
}
|
||||
]
|
||||
}
|
||||
],
|
||||
"code": {
|
||||
"code": "clippy::let_and_return",
|
||||
"explanation": null
|
||||
},
|
||||
"level": "warning",
|
||||
"message": "returning the result of a let binding from a block",
|
||||
"spans": [
|
||||
{
|
||||
"byte_end": 55,
|
||||
"byte_start": 29,
|
||||
"column_end": 31,
|
||||
"column_start": 5,
|
||||
"expansion": null,
|
||||
"file_name": "src/main.rs",
|
||||
"is_primary": false,
|
||||
"label": "unnecessary let binding",
|
||||
"line_end": 3,
|
||||
"line_start": 3,
|
||||
"suggested_replacement": null,
|
||||
"suggestion_applicability": null,
|
||||
"text": [
|
||||
{
|
||||
"highlight_end": 31,
|
||||
"highlight_start": 5,
|
||||
"text": " let a = (0..10).collect();"
|
||||
}
|
||||
]
|
||||
},
|
||||
{
|
||||
"byte_end": 61,
|
||||
"byte_start": 60,
|
||||
"column_end": 6,
|
||||
"column_start": 5,
|
||||
"expansion": null,
|
||||
"file_name": "src/main.rs",
|
||||
"is_primary": true,
|
||||
"label": null,
|
||||
"line_end": 4,
|
||||
"line_start": 4,
|
||||
"suggested_replacement": null,
|
||||
"suggestion_applicability": null,
|
||||
"text": [
|
||||
{
|
||||
"highlight_end": 6,
|
||||
"highlight_start": 5,
|
||||
"text": " a"
|
||||
}
|
||||
]
|
||||
}
|
||||
]
|
||||
}
|
||||
"##,
|
||||
);
|
||||
|
||||
let workspace_root = PathBuf::from("/test/");
|
||||
let diag = map_rust_diagnostic_to_lsp(&diag, &workspace_root);
|
||||
insta::assert_debug_snapshot!(diag);
|
||||
}
|
||||
|
|
|
@ -197,23 +197,23 @@ impl CheckWatcherThread {
|
|||
}
|
||||
|
||||
CheckEvent::Msg(Message::CompilerMessage(msg)) => {
|
||||
let map_result =
|
||||
match map_rust_diagnostic_to_lsp(&msg.message, &self.workspace_root) {
|
||||
Some(map_result) => map_result,
|
||||
None => return,
|
||||
};
|
||||
let map_result = map_rust_diagnostic_to_lsp(&msg.message, &self.workspace_root);
|
||||
if map_result.is_empty() {
|
||||
return;
|
||||
}
|
||||
|
||||
let MappedRustDiagnostic { location, diagnostic, fixes } = map_result;
|
||||
let fixes = fixes
|
||||
.into_iter()
|
||||
.map(|fix| {
|
||||
CodeAction { diagnostics: Some(vec![diagnostic.clone()]), ..fix }.into()
|
||||
})
|
||||
.collect();
|
||||
for MappedRustDiagnostic { location, diagnostic, fixes } in map_result {
|
||||
let fixes = fixes
|
||||
.into_iter()
|
||||
.map(|fix| {
|
||||
CodeAction { diagnostics: Some(vec![diagnostic.clone()]), ..fix }.into()
|
||||
})
|
||||
.collect();
|
||||
|
||||
task_send
|
||||
.send(CheckTask::AddDiagnostic { url: location.uri, diagnostic, fixes })
|
||||
.unwrap();
|
||||
task_send
|
||||
.send(CheckTask::AddDiagnostic { url: location.uri, diagnostic, fixes })
|
||||
.unwrap();
|
||||
}
|
||||
}
|
||||
|
||||
CheckEvent::Msg(Message::BuildScriptExecuted(_msg)) => {}
|
||||
|
|
Loading…
Reference in a new issue