mirror of
https://github.com/photonstorm/phaser
synced 2025-02-18 15:08:31 +00:00
New Input handler implemented.
This commit is contained in:
parent
ce7bfd1fc2
commit
3cdd2baff0
31 changed files with 3048 additions and 2029 deletions
|
@ -49,7 +49,7 @@ module Phaser {
|
|||
/**
|
||||
* Game constructor
|
||||
*
|
||||
* Instantiate a new <code>Game</code> object.
|
||||
* Instantiate a new <code>Phaser.Game</code> object.
|
||||
*
|
||||
* @param callbackContext Which context will the callbacks be called with.
|
||||
* @param parent {string} ID of its parent DOM element.
|
||||
|
@ -59,14 +59,16 @@ module Phaser {
|
|||
* @param createCallback {function} Create callback invoked when create default screen.
|
||||
* @param updateCallback {function} Update callback invoked when update default screen.
|
||||
* @param renderCallback {function} Render callback invoked when render default screen.
|
||||
* @param destroyCallback {function} Destroy callback invoked when state is destroyed.
|
||||
*/
|
||||
constructor(callbackContext, parent?: string = '', width?: number = 800, height?: number = 600, initCallback = null, createCallback = null, updateCallback = null, renderCallback = null) {
|
||||
constructor(callbackContext, parent?: string = '', width?: number = 800, height?: number = 600, initCallback = null, createCallback = null, updateCallback = null, renderCallback = null, destroyCallback = null) {
|
||||
|
||||
this.callbackContext = callbackContext;
|
||||
this.onInitCallback = initCallback;
|
||||
this.onCreateCallback = createCallback;
|
||||
this.onUpdateCallback = updateCallback;
|
||||
this.onRenderCallback = renderCallback;
|
||||
this.onDestroyCallback = destroyCallback;
|
||||
|
||||
if (document.readyState === 'complete' || document.readyState === 'interactive')
|
||||
{
|
||||
|
@ -121,6 +123,12 @@ module Phaser {
|
|||
*/
|
||||
private _pendingState = null;
|
||||
|
||||
/**
|
||||
* The current State object (defaults to null)
|
||||
* @type {State}
|
||||
*/
|
||||
public state = null;
|
||||
|
||||
/**
|
||||
* Context for calling the callbacks.
|
||||
*/
|
||||
|
@ -156,6 +164,12 @@ module Phaser {
|
|||
*/
|
||||
public onPausedCallback = null;
|
||||
|
||||
/**
|
||||
* This will be called when the state is destroyed (i.e. swapping to a new state)
|
||||
* @type {function}
|
||||
*/
|
||||
public onDestroyCallback = null;
|
||||
|
||||
/**
|
||||
* Reference to the assets cache.
|
||||
* @type {Cache}
|
||||
|
@ -281,6 +295,7 @@ module Phaser {
|
|||
|
||||
this.framerate = 60;
|
||||
this.isBooted = true;
|
||||
this.input.start();
|
||||
|
||||
// Display the default game screen?
|
||||
if (this.onInitCallback == null && this.onCreateCallback == null && this.onUpdateCallback == null && this.onRenderCallback == null && this._pendingState == null)
|
||||
|
@ -423,13 +438,15 @@ module Phaser {
|
|||
* @param createCallback {function} Create callback invoked when create state.
|
||||
* @param updateCallback {function} Update callback invoked when update state.
|
||||
* @param renderCallback {function} Render callback invoked when render state.
|
||||
* @param destroyCallback {function} Destroy callback invoked when state is destroyed.
|
||||
*/
|
||||
public setCallbacks(initCallback = null, createCallback = null, updateCallback = null, renderCallback = null) {
|
||||
public setCallbacks(initCallback = null, createCallback = null, updateCallback = null, renderCallback = null, destroyCallback = null) {
|
||||
|
||||
this.onInitCallback = initCallback;
|
||||
this.onCreateCallback = createCallback;
|
||||
this.onUpdateCallback = updateCallback;
|
||||
this.onRenderCallback = renderCallback;
|
||||
this.onDestroyCallback = destroyCallback;
|
||||
|
||||
}
|
||||
|
||||
|
@ -447,47 +464,61 @@ module Phaser {
|
|||
return;
|
||||
}
|
||||
|
||||
// Destroy current state?
|
||||
if (this.onDestroyCallback !== null)
|
||||
{
|
||||
this.onDestroyCallback.call(this.callbackContext);
|
||||
}
|
||||
|
||||
this.input.reset();
|
||||
|
||||
// Prototype?
|
||||
if (typeof state === 'function')
|
||||
{
|
||||
state = new state(this);
|
||||
this.state = new state(this);
|
||||
}
|
||||
|
||||
// Ok, have we got the right functions?
|
||||
if (state['create'] || state['update'])
|
||||
if (this.state['create'] || this.state['update'])
|
||||
{
|
||||
this.callbackContext = state;
|
||||
this.callbackContext = this.state;
|
||||
|
||||
this.onInitCallback = null;
|
||||
this.onCreateCallback = null;
|
||||
this.onUpdateCallback = null;
|
||||
this.onRenderCallback = null;
|
||||
this.onPausedCallback = null;
|
||||
this.onDestroyCallback = null;
|
||||
|
||||
// Bingo, let's set them up
|
||||
if (state['init'])
|
||||
if (this.state['init'])
|
||||
{
|
||||
this.onInitCallback = state['init'];
|
||||
this.onInitCallback = this.state['init'];
|
||||
}
|
||||
|
||||
if (state['create'])
|
||||
if (this.state['create'])
|
||||
{
|
||||
this.onCreateCallback = state['create'];
|
||||
this.onCreateCallback = this.state['create'];
|
||||
}
|
||||
|
||||
if (state['update'])
|
||||
if (this.state['update'])
|
||||
{
|
||||
this.onUpdateCallback = state['update'];
|
||||
this.onUpdateCallback = this.state['update'];
|
||||
}
|
||||
|
||||
if (state['render'])
|
||||
if (this.state['render'])
|
||||
{
|
||||
this.onRenderCallback = state['render'];
|
||||
this.onRenderCallback = this.state['render'];
|
||||
}
|
||||
|
||||
if (state['paused'])
|
||||
if (this.state['paused'])
|
||||
{
|
||||
this.onPausedCallback = state['paused'];
|
||||
this.onPausedCallback = this.state['paused'];
|
||||
}
|
||||
|
||||
if (this.state['destroy'])
|
||||
{
|
||||
this.onDestroyCallback = this.state['destroy'];
|
||||
}
|
||||
|
||||
if (clearWorld)
|
||||
|
@ -522,6 +553,7 @@ module Phaser {
|
|||
this.onUpdateCallback = null;
|
||||
this.onRenderCallback = null;
|
||||
this.onPausedCallback = null;
|
||||
this.onDestroyCallback = null;
|
||||
this.cache = null;
|
||||
this.input = null;
|
||||
this.loader = null;
|
||||
|
|
|
@ -134,9 +134,17 @@
|
|||
<TypeScriptCompile Include="system\screens\PauseScreen.ts" />
|
||||
<TypeScriptCompile Include="system\screens\BootScreen.ts" />
|
||||
<TypeScriptCompile Include="system\input\MSPointer.ts" />
|
||||
<TypeScriptCompile Include="system\input\Gestures.ts" />
|
||||
<Content Include="system\input\Gestures.js">
|
||||
<DependentUpon>Gestures.ts</DependentUpon>
|
||||
</Content>
|
||||
<Content Include="system\input\MSPointer.js">
|
||||
<DependentUpon>MSPointer.ts</DependentUpon>
|
||||
</Content>
|
||||
<TypeScriptCompile Include="system\input\Pointer.ts" />
|
||||
<Content Include="system\input\Pointer.js">
|
||||
<DependentUpon>Pointer.ts</DependentUpon>
|
||||
</Content>
|
||||
<Content Include="system\screens\BootScreen.js">
|
||||
<DependentUpon>BootScreen.ts</DependentUpon>
|
||||
</Content>
|
||||
|
@ -233,9 +241,6 @@
|
|||
<Content Include="system\easing\Sinusoidal.js">
|
||||
<DependentUpon>Sinusoidal.ts</DependentUpon>
|
||||
</Content>
|
||||
<Content Include="system\input\Finger.js">
|
||||
<DependentUpon>Finger.ts</DependentUpon>
|
||||
</Content>
|
||||
<Content Include="system\input\Input.js">
|
||||
<DependentUpon>Input.ts</DependentUpon>
|
||||
</Content>
|
||||
|
@ -252,7 +257,6 @@
|
|||
<TypeScriptCompile Include="system\input\Mouse.ts" />
|
||||
<TypeScriptCompile Include="system\input\Keyboard.ts" />
|
||||
<TypeScriptCompile Include="system\input\Input.ts" />
|
||||
<TypeScriptCompile Include="system\input\Finger.ts" />
|
||||
<TypeScriptCompile Include="system\easing\Sinusoidal.ts" />
|
||||
<TypeScriptCompile Include="system\easing\Quintic.ts" />
|
||||
<TypeScriptCompile Include="system\easing\Quartic.ts" />
|
||||
|
|
|
@ -45,13 +45,14 @@ module Phaser {
|
|||
|
||||
// Consume default actions on the canvas
|
||||
this.canvas.style.msTouchAction = 'none';
|
||||
this.canvas.style['ms-touch-action'] = 'none';
|
||||
this.canvas.style['touch-action'] = 'none';
|
||||
this.canvas.style.backgroundColor = 'rgb(0,0,0)';
|
||||
|
||||
this.context = this.canvas.getContext('2d');
|
||||
|
||||
this.offset = this.getOffset(this.canvas);
|
||||
this.bounds = new Rectangle(this.offset.x, this.offset.y, width, height);
|
||||
this.bounds = new Quad(this.offset.x, this.offset.y, width, height);
|
||||
this.aspectRatio = width / height;
|
||||
this.scaleMode = StageScaleMode.NO_SCALE;
|
||||
this.scale = new StageScaleMode(this._game);
|
||||
|
@ -91,9 +92,9 @@ module Phaser {
|
|||
|
||||
/**
|
||||
* Bound of this stage.
|
||||
* @type {Rectangle}
|
||||
* @type {Quad}
|
||||
*/
|
||||
public bounds: Rectangle;
|
||||
public bounds: Quad;
|
||||
|
||||
/**
|
||||
* Asperct ratio, thus: width / height.
|
||||
|
@ -135,9 +136,9 @@ module Phaser {
|
|||
|
||||
/**
|
||||
* Offset from this stage to the canvas element.
|
||||
* @type {Point}
|
||||
* @type {MicroPoint}
|
||||
*/
|
||||
public offset: Point;
|
||||
public offset: MicroPoint;
|
||||
|
||||
/**
|
||||
* This object manages scaling of the game, see(StageScaleMode).
|
||||
|
@ -211,7 +212,7 @@ module Phaser {
|
|||
|
||||
}
|
||||
|
||||
private getOffset(element): Point {
|
||||
private getOffset(element): MicroPoint {
|
||||
|
||||
var box = element.getBoundingClientRect();
|
||||
|
||||
|
@ -220,7 +221,7 @@ module Phaser {
|
|||
var scrollTop = window.pageYOffset || element.scrollTop || document.body.scrollTop;
|
||||
var scrollLeft = window.pageXOffset || element.scrollLeft || document.body.scrollLeft;
|
||||
|
||||
return new Point(box.left + scrollLeft - clientLeft, box.top + scrollTop - clientTop);
|
||||
return new MicroPoint(box.left + scrollLeft - clientLeft, box.top + scrollTop - clientTop);
|
||||
|
||||
}
|
||||
|
||||
|
|
|
@ -43,87 +43,107 @@ module Phaser {
|
|||
* @type {Camera}
|
||||
*/
|
||||
public camera: Camera;
|
||||
|
||||
/**
|
||||
* Reference to the assets cache.
|
||||
* @type {Cache}
|
||||
*/
|
||||
public cache: Cache;
|
||||
|
||||
/**
|
||||
* Reference to the collision helper.
|
||||
* @type {Collision}
|
||||
*/
|
||||
public collision: Collision;
|
||||
|
||||
/**
|
||||
* Reference to the input manager
|
||||
* @type {Input}
|
||||
*/
|
||||
public input: Input;
|
||||
|
||||
/**
|
||||
* Reference to the assets loader.
|
||||
* @type {Loader}
|
||||
*/
|
||||
public loader: Loader;
|
||||
|
||||
/**
|
||||
* Reference to the math helper.
|
||||
* @type {GameMath}
|
||||
*/
|
||||
public math: GameMath;
|
||||
|
||||
/**
|
||||
* Reference to the motion helper.
|
||||
* @type {Motion}
|
||||
*/
|
||||
public motion: Motion;
|
||||
|
||||
/**
|
||||
* Reference to the sound manager.
|
||||
* @type {SoundManager}
|
||||
*/
|
||||
public sound: SoundManager;
|
||||
|
||||
/**
|
||||
* Reference to the stage.
|
||||
* @type {Stage}
|
||||
*/
|
||||
public stage: Stage;
|
||||
|
||||
/**
|
||||
* Reference to game clock.
|
||||
* @type {Time}
|
||||
*/
|
||||
public time: Time;
|
||||
|
||||
/**
|
||||
* Reference to the tween manager.
|
||||
* @type {TweenManager}
|
||||
*/
|
||||
public tweens: TweenManager;
|
||||
|
||||
/**
|
||||
* Reference to the world.
|
||||
* @type {World}
|
||||
*/
|
||||
public world: World;
|
||||
|
||||
// Override these in your own States
|
||||
|
||||
// Overload these in your own States
|
||||
/**
|
||||
* Override this method to add some load operations.
|
||||
* If you need to use the loader, you may need to use them here.
|
||||
*/
|
||||
public init() { }
|
||||
|
||||
/**
|
||||
* This method is called after the game engine successfully switches states.
|
||||
* Feel free to add any setup code here.(Do not load anything here, override init() instead)
|
||||
*/
|
||||
public create() { }
|
||||
|
||||
/**
|
||||
* Put update logic here.
|
||||
*/
|
||||
public update() { }
|
||||
|
||||
/**
|
||||
* Put render operations here.
|
||||
*/
|
||||
public render() { }
|
||||
|
||||
/**
|
||||
* This method will be called when game paused.
|
||||
*/
|
||||
public paused() { }
|
||||
|
||||
/**
|
||||
* This method will be called when the state is destroyed
|
||||
*/
|
||||
public destroy() { }
|
||||
|
||||
// Handy Proxy methods
|
||||
|
||||
/**
|
||||
|
|
|
@ -16,8 +16,7 @@ module Phaser {
|
|||
/**
|
||||
* GameObject constructor
|
||||
*
|
||||
* Create a new <code>GameObject</code> object at specific position with
|
||||
* specific width and height.
|
||||
* Create a new <code>GameObject</code> object at specific position with specific width and height.
|
||||
*
|
||||
* @param [x] {number} The x position of the object.
|
||||
* @param [y] {number} The y position of the object.
|
||||
|
@ -78,52 +77,62 @@ module Phaser {
|
|||
* @type {number}
|
||||
*/
|
||||
public static ALIGN_TOP_LEFT: number = 0;
|
||||
|
||||
/**
|
||||
* Pivot position enum: at the top-center corner.
|
||||
* @type {number}
|
||||
*/
|
||||
public static ALIGN_TOP_CENTER: number = 1;
|
||||
|
||||
/**
|
||||
* Pivot position enum: at the top-right corner.
|
||||
* @type {number}
|
||||
*/
|
||||
public static ALIGN_TOP_RIGHT: number = 2;
|
||||
|
||||
/**
|
||||
* Pivot position enum: at the center-left corner.
|
||||
* @type {number}
|
||||
*/
|
||||
public static ALIGN_CENTER_LEFT: number = 3;
|
||||
|
||||
/**
|
||||
* Pivot position enum: at the center corner.
|
||||
* @type {number}
|
||||
*/
|
||||
public static ALIGN_CENTER: number = 4;
|
||||
|
||||
/**
|
||||
* Pivot position enum: at the center-right corner.
|
||||
* @type {number}
|
||||
*/
|
||||
public static ALIGN_CENTER_RIGHT: number = 5;
|
||||
|
||||
/**
|
||||
* Pivot position enum: at the bottom-left corner.
|
||||
* @type {number}
|
||||
*/
|
||||
public static ALIGN_BOTTOM_LEFT: number = 6;
|
||||
|
||||
/**
|
||||
* Pivot position enum: at the bottom-center corner.
|
||||
* @type {number}
|
||||
*/
|
||||
public static ALIGN_BOTTOM_CENTER: number = 7;
|
||||
|
||||
/**
|
||||
* Pivot position enum: at the bottom-right corner.
|
||||
* @type {number}
|
||||
*/
|
||||
public static ALIGN_BOTTOM_RIGHT: number = 8;
|
||||
|
||||
|
||||
/**
|
||||
* Enum value for outOfBoundsAction. Stop the object when is out of world bounds.
|
||||
* @type {number}
|
||||
*/
|
||||
public static OUT_OF_BOUNDS_STOP: number = 0;
|
||||
|
||||
/**
|
||||
* Enum value for outOfBoundsAction. Kill the object when is out of world bounds.
|
||||
* @type {number}
|
||||
|
@ -137,49 +146,58 @@ module Phaser {
|
|||
public _point: MicroPoint;
|
||||
|
||||
public cameraBlacklist: number[];
|
||||
|
||||
/**
|
||||
* Rectangle container of this object.
|
||||
* @type {Rectangle}
|
||||
*/
|
||||
public bounds: Rectangle;
|
||||
|
||||
/**
|
||||
* Bound of world.
|
||||
* @type {Quad}
|
||||
*/
|
||||
public worldBounds: Quad;
|
||||
|
||||
/**
|
||||
* What action will be performed when object is out of bounds.
|
||||
* This will default to GameObject.OUT_OF_BOUNDS_STOP.
|
||||
* @type {number}
|
||||
*/
|
||||
public outOfBoundsAction: number = 0;
|
||||
|
||||
/**
|
||||
* At which point the graphic of this object will align to.
|
||||
* Align of the object will default to GameObject.ALIGN_TOP_LEFT.
|
||||
* @type {number}
|
||||
*/
|
||||
public align: number;
|
||||
|
||||
/**
|
||||
* Oorientation of the object.
|
||||
* Orientation of the object.
|
||||
* @type {number}
|
||||
*/
|
||||
public facing: number;
|
||||
|
||||
/**
|
||||
* Set alpha to a number between 0 and 1 to change the opacity.
|
||||
* @type {number}
|
||||
*/
|
||||
public alpha: number;
|
||||
|
||||
/**
|
||||
* Scale factor of the object.
|
||||
* @type {MicroPoint}
|
||||
*/
|
||||
public scale: MicroPoint;
|
||||
|
||||
/**
|
||||
* Origin is the anchor point that the object will rotate by.
|
||||
* The origin will default to its center.
|
||||
* @type {MicroPoint}
|
||||
*/
|
||||
public origin: MicroPoint;
|
||||
|
||||
/**
|
||||
* Z-order value of the object.
|
||||
*/
|
||||
|
@ -218,54 +236,64 @@ module Phaser {
|
|||
* @type {MicroPoint}
|
||||
*/
|
||||
public velocity: MicroPoint;
|
||||
|
||||
/**
|
||||
* The virtual mass of the object.
|
||||
* @type {number}
|
||||
*/
|
||||
public mass: number;
|
||||
|
||||
/**
|
||||
* The bounciness of the object.
|
||||
* @type {number}
|
||||
*/
|
||||
public elasticity: number;
|
||||
|
||||
/**
|
||||
* How fast the speed of this object is changing.
|
||||
* @type {number}
|
||||
*/
|
||||
public acceleration: MicroPoint;
|
||||
|
||||
/**
|
||||
* This isn't drag exactly, more like deceleration that is only applied
|
||||
* when acceleration is not affecting the sprite.
|
||||
* @type {MicroPoint}
|
||||
*/
|
||||
public drag: MicroPoint;
|
||||
|
||||
/**
|
||||
* It will cap the speed automatically if you use the acceleration
|
||||
* to change its velocity.
|
||||
* @type {MicroPoint}
|
||||
*/
|
||||
public maxVelocity: MicroPoint;
|
||||
|
||||
/**
|
||||
* How fast this object is rotating.
|
||||
* @type {number}
|
||||
*/
|
||||
public angularVelocity: number;
|
||||
|
||||
/**
|
||||
* How fast angularVelocity of this object is changing.
|
||||
* @type {number}
|
||||
*/
|
||||
public angularAcceleration: number;
|
||||
|
||||
/**
|
||||
* Deacceleration of angularVelocity will be applied when it's rotating.
|
||||
* @type {number}
|
||||
*/
|
||||
public angularDrag: number;
|
||||
|
||||
/**
|
||||
* It will cap the rotate speed automatically if you use the angularAcceleration
|
||||
* to change its angularVelocity.
|
||||
* @type {number}
|
||||
*/
|
||||
public maxAngular: number;
|
||||
|
||||
/**
|
||||
* A point that can store numbers from 0 to 1 (for X and Y independently)
|
||||
* which governs how much this object is affected by the camera .
|
||||
|
@ -278,27 +306,32 @@ module Phaser {
|
|||
* @type {number}
|
||||
*/
|
||||
public health: number;
|
||||
|
||||
/**
|
||||
* Set this to false if you want to skip the automatic motion/movement stuff
|
||||
* (see updateMotion()).
|
||||
* @type {boolean}
|
||||
*/
|
||||
public moves: bool = true;
|
||||
|
||||
/**
|
||||
* Bit field of flags (use with UP, DOWN, LEFT, RIGHT, etc) indicating surface contacts.
|
||||
* @type {number}
|
||||
*/
|
||||
public touching: number;
|
||||
|
||||
/**
|
||||
* Bit field of flags (use with UP, DOWN, LEFT, RIGHT, etc) indicating surface contacts from the previous game loop step.
|
||||
* @type {number}
|
||||
*/
|
||||
public wasTouching: number;
|
||||
|
||||
/**
|
||||
* Bit field of flags (use with UP, DOWN, LEFT, RIGHT, etc) indicating collision directions.
|
||||
* @type {number}
|
||||
*/
|
||||
public allowCollisions: number;
|
||||
|
||||
/**
|
||||
* Important variable for collision processing.
|
||||
* @type {MicroPoint}
|
||||
|
@ -665,7 +698,7 @@ module Phaser {
|
|||
|
||||
/**
|
||||
* Handy for checking if this object is touching a particular surface.
|
||||
* For slightly better performance you can just & the value directly numbero <code>touching</code>.
|
||||
* For slightly better performance you can just & the value directly into <code>touching</code>.
|
||||
* However, this method is good for readability and accessibility.
|
||||
*
|
||||
* @param Direction {number} Any of the collision flags (e.g. LEFT, FLOOR, etc).
|
||||
|
@ -685,7 +718,7 @@ module Phaser {
|
|||
*/
|
||||
|
||||
/**
|
||||
* Handy function for checking if this object is just landed on a particular surface.
|
||||
* Handy function for checking if this object just landed on a particular surface.
|
||||
*
|
||||
* @param Direction {number} Any of the collision flags (e.g. LEFT, FLOOR, etc).
|
||||
*
|
||||
|
@ -730,7 +763,7 @@ module Phaser {
|
|||
|
||||
/**
|
||||
* If you do not wish this object to be visible to a specific camera, pass the camera here.
|
||||
|
||||
*
|
||||
* @param camera {Camera} The specific camera.
|
||||
*/
|
||||
public hideFromCamera(camera: Camera) {
|
||||
|
@ -758,7 +791,7 @@ module Phaser {
|
|||
}
|
||||
|
||||
/**
|
||||
* This will make the object not visible to any cameras.
|
||||
* This clears the camera black list, making the GameObject visible to all cameras.
|
||||
*/
|
||||
public clearCameraList() {
|
||||
|
||||
|
|
|
@ -47,6 +47,7 @@ module Phaser {
|
|||
* Texture of this sprite to be rendered.
|
||||
*/
|
||||
private _texture;
|
||||
|
||||
/**
|
||||
* Texture of this sprite is DynamicTexture? (default to false)
|
||||
* @type {boolean}
|
||||
|
@ -74,16 +75,25 @@ module Phaser {
|
|||
* @type {boolean}
|
||||
*/
|
||||
public renderDebug: bool = false;
|
||||
|
||||
/**
|
||||
* Color of the Sprite when no image is present. Format is a css color string.
|
||||
* @type {string}
|
||||
*/
|
||||
public fillColor: string = 'rgb(255,255,255)';
|
||||
|
||||
/**
|
||||
* Color of bound when render debug. (see renderDebug) Format is a css color string.
|
||||
* @type {string}
|
||||
*/
|
||||
public renderDebugColor: string = 'rgba(0,255,0,0.5)';
|
||||
|
||||
/**
|
||||
* Color of points when render debug. (see renderDebug) Format is a css color string.
|
||||
* @type {string}
|
||||
*/
|
||||
public renderDebugPointColor: string = 'rgba(255,255,255,1)';
|
||||
|
||||
/**
|
||||
* Flip the graphic vertically? (default to false)
|
||||
* @type {boolean}
|
||||
|
@ -143,12 +153,12 @@ module Phaser {
|
|||
* @param [color] {number} specifies the color of the generated block. (format is 0xAARRGGBB)
|
||||
* @return {Sprite} Sprite instance itself.
|
||||
*/
|
||||
public makeGraphic(width: number, height: number, color: number = 0xffffffff): Sprite {
|
||||
public makeGraphic(width: number, height: number, color: string = 'rgb(255,255,255)'): Sprite {
|
||||
|
||||
this._texture = null;
|
||||
this.width = width;
|
||||
this.height = height;
|
||||
|
||||
this.fillColor = color;
|
||||
this._dynamicTexture = false;
|
||||
|
||||
return this;
|
||||
|
@ -178,7 +188,7 @@ module Phaser {
|
|||
}
|
||||
|
||||
/**
|
||||
* Automatically called after update() by the game loop, this function just update animations.
|
||||
* Automatically called after update() by the game loop, this function just updates animations.
|
||||
*/
|
||||
public postUpdate() {
|
||||
|
||||
|
@ -353,7 +363,7 @@ module Phaser {
|
|||
}
|
||||
else
|
||||
{
|
||||
this._game.stage.context.fillStyle = 'rgb(255,255,255)';
|
||||
this._game.stage.context.fillStyle = this.fillColor;
|
||||
this._game.stage.context.fillRect(this._dx, this._dy, this._dw, this._dh);
|
||||
}
|
||||
|
||||
|
|
|
@ -113,6 +113,12 @@ module Phaser {
|
|||
*/
|
||||
public touch: bool = false;
|
||||
|
||||
/**
|
||||
* Is mspointer available?
|
||||
* @type {boolean}
|
||||
*/
|
||||
public mspointer: bool = false;
|
||||
|
||||
/**
|
||||
* Is css3D available?
|
||||
* @type {boolean}
|
||||
|
@ -313,6 +319,11 @@ module Phaser {
|
|||
this.touch = true;
|
||||
}
|
||||
|
||||
if (window.navigator.msPointerEnabled)
|
||||
{
|
||||
this.mspointer = true;
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
|
@ -502,6 +513,7 @@ module Phaser {
|
|||
output = output.concat('WebGL: ' + this.webGL + '\n');
|
||||
output = output.concat('Worker: ' + this.worker + '\n');
|
||||
output = output.concat('Touch: ' + this.touch + '\n');
|
||||
output = output.concat('MSPointer: ' + this.mspointer + '\n');
|
||||
output = output.concat('CSS 3D: ' + this.css3D + '\n');
|
||||
|
||||
output = output.concat('\n');
|
||||
|
|
|
@ -1,319 +0,0 @@
|
|||
/// <reference path="../../Game.ts" />
|
||||
|
||||
/**
|
||||
* Phaser - Finger
|
||||
*
|
||||
* A Finger object is used by the Touch manager and represents a single finger on the touch screen.
|
||||
*/
|
||||
|
||||
module Phaser {
|
||||
|
||||
export class Finger {
|
||||
|
||||
/**
|
||||
* Constructor
|
||||
* @param {Phaser.Game} game.
|
||||
* @return {Phaser.Finger} This object.
|
||||
*/
|
||||
constructor(game: Game) {
|
||||
|
||||
this._game = game;
|
||||
this.active = false;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @property _game
|
||||
* @type {Phaser.Game}
|
||||
* @private
|
||||
**/
|
||||
private _game: Game;
|
||||
|
||||
/**
|
||||
* An identification number for each touch point. When a touch point becomes active, it must be assigned an identifier that is distinct from any other active touch point. While the touch point remains active, all events that refer to it must assign it the same identifier.
|
||||
* @property identifier
|
||||
* @type {Number}
|
||||
*/
|
||||
public identifier: number;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property active
|
||||
* @type {Boolean}
|
||||
*/
|
||||
public active: bool;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property point
|
||||
* @type {Point}
|
||||
**/
|
||||
public point: Point = null;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property circle
|
||||
* @type {Circle}
|
||||
**/
|
||||
public circle: Circle = null;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property withinGame
|
||||
* @type {Boolean}
|
||||
*/
|
||||
public withinGame: bool = false;
|
||||
|
||||
/**
|
||||
* The horizontal coordinate of point relative to the viewport in pixels, excluding any scroll offset
|
||||
* @property clientX
|
||||
* @type {Number}
|
||||
*/
|
||||
public clientX: number = -1;
|
||||
|
||||
//
|
||||
/**
|
||||
* The vertical coordinate of point relative to the viewport in pixels, excluding any scroll offset
|
||||
* @property clientY
|
||||
* @type {Number}
|
||||
*/
|
||||
public clientY: number = -1;
|
||||
|
||||
//
|
||||
/**
|
||||
* The horizontal coordinate of point relative to the viewport in pixels, including any scroll offset
|
||||
* @property pageX
|
||||
* @type {Number}
|
||||
*/
|
||||
public pageX: number = -1;
|
||||
|
||||
/**
|
||||
* The vertical coordinate of point relative to the viewport in pixels, including any scroll offset
|
||||
* @property pageY
|
||||
* @type {Number}
|
||||
*/
|
||||
public pageY: number = -1;
|
||||
|
||||
/**
|
||||
* The horizontal coordinate of point relative to the screen in pixels
|
||||
* @property screenX
|
||||
* @type {Number}
|
||||
*/
|
||||
public screenX: number = -1;
|
||||
|
||||
/**
|
||||
* The vertical coordinate of point relative to the screen in pixels
|
||||
* @property screenY
|
||||
* @type {Number}
|
||||
*/
|
||||
public screenY: number = -1;
|
||||
|
||||
/**
|
||||
* The horizontal coordinate of point relative to the game element
|
||||
* @property x
|
||||
* @type {Number}
|
||||
*/
|
||||
public x: number = -1;
|
||||
|
||||
/**
|
||||
* The vertical coordinate of point relative to the game element
|
||||
* @property y
|
||||
* @type {Number}
|
||||
*/
|
||||
public y: number = -1;
|
||||
|
||||
/**
|
||||
* The Element on which the touch point started when it was first placed on the surface, even if the touch point has since moved outside the interactive area of that element.
|
||||
* @property target
|
||||
* @type {Any}
|
||||
*/
|
||||
public target;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property isDown
|
||||
* @type {Boolean}
|
||||
**/
|
||||
public isDown: bool = false;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property isUp
|
||||
* @type {Boolean}
|
||||
**/
|
||||
public isUp: bool = false;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property timeDown
|
||||
* @type {Number}
|
||||
**/
|
||||
public timeDown: number = 0;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property duration
|
||||
* @type {Number}
|
||||
**/
|
||||
public duration: number = 0;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property timeUp
|
||||
* @type {Number}
|
||||
**/
|
||||
public timeUp: number = 0;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property justPressedRate
|
||||
* @type {Number}
|
||||
**/
|
||||
public justPressedRate: number = 200;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property justReleasedRate
|
||||
* @type {Number}
|
||||
**/
|
||||
public justReleasedRate: number = 200;
|
||||
|
||||
/**
|
||||
*
|
||||
* @method start
|
||||
* @param {Any} event
|
||||
*/
|
||||
public start(event) {
|
||||
|
||||
this.identifier = event.identifier;
|
||||
this.target = event.target;
|
||||
|
||||
// populate geom objects
|
||||
if (this.point === null)
|
||||
{
|
||||
this.point = new Point();
|
||||
}
|
||||
|
||||
if (this.circle === null)
|
||||
{
|
||||
this.circle = new Circle(0, 0, 44);
|
||||
}
|
||||
|
||||
this.move(event);
|
||||
|
||||
this.active = true;
|
||||
this.withinGame = true;
|
||||
this.isDown = true;
|
||||
this.isUp = false;
|
||||
this.timeDown = this._game.time.now;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method move
|
||||
* @param {Any} event
|
||||
*/
|
||||
public move(event) {
|
||||
|
||||
this.clientX = event.clientX;
|
||||
this.clientY = event.clientY;
|
||||
this.pageX = event.pageX;
|
||||
this.pageY = event.pageY;
|
||||
this.screenX = event.screenX;
|
||||
this.screenY = event.screenY;
|
||||
|
||||
this.x = this.pageX - this._game.stage.offset.x;
|
||||
this.y = this.pageY - this._game.stage.offset.y;
|
||||
|
||||
this.point.setTo(this.x, this.y);
|
||||
this.circle.setTo(this.x, this.y, 44);
|
||||
|
||||
// Droppings history (used for gestures and motion tracking)
|
||||
|
||||
this.duration = this._game.time.now - this.timeDown;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method leave
|
||||
* @param {Any} event
|
||||
*/
|
||||
public leave(event) {
|
||||
|
||||
this.withinGame = false;
|
||||
this.move(event);
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method stop
|
||||
* @param {Any} event
|
||||
*/
|
||||
public stop(event) {
|
||||
|
||||
this.active = false;
|
||||
this.withinGame = false;
|
||||
|
||||
this.isDown = false;
|
||||
this.isUp = true;
|
||||
this.timeUp = this._game.time.now;
|
||||
this.duration = this.timeUp - this.timeDown;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method justPressed
|
||||
* @param {Number} [duration].
|
||||
* @return {Boolean}
|
||||
*/
|
||||
public justPressed(duration?: number = this.justPressedRate): bool {
|
||||
|
||||
if (this.isDown === true && (this.timeDown + duration) > this._game.time.now)
|
||||
{
|
||||
return true;
|
||||
}
|
||||
else
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method justReleased
|
||||
* @param {Number} [duration].
|
||||
* @return {Boolean}
|
||||
*/
|
||||
public justReleased(duration?: number = this.justReleasedRate): bool {
|
||||
|
||||
if (this.isUp === true && (this.timeUp + duration) > this._game.time.now)
|
||||
{
|
||||
return true;
|
||||
}
|
||||
else
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Returns a string representation of this object.
|
||||
* @method toString
|
||||
* @return {String} a string representation of the instance.
|
||||
**/
|
||||
public toString(): string {
|
||||
|
||||
return "[{Finger (identifer=" + this.identifier + " active=" + this.active + " duration=" + this.duration + " withinGame=" + this.withinGame + " x=" + this.x + " y=" + this.y + " clientX=" + this.clientX + " clientY=" + this.clientY + " screenX=" + this.screenX + " screenY=" + this.screenY + " pageX=" + this.pageX + " pageY=" + this.pageY + ")}]";
|
||||
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
}
|
63
Phaser/system/input/Gestures.ts
Normal file
63
Phaser/system/input/Gestures.ts
Normal file
|
@ -0,0 +1,63 @@
|
|||
/// <reference path="../../Game.ts" />
|
||||
/// <reference path="Pointer.ts" />
|
||||
|
||||
/**
|
||||
* Phaser - Gestures
|
||||
*
|
||||
* The Gesture class monitors for gestures and dispatches the resulting signals when they occur.
|
||||
* Note: Android 2.x only supports 1 touch event at once, no multi-touch
|
||||
*/
|
||||
|
||||
module Phaser {
|
||||
|
||||
export class Gestures {
|
||||
|
||||
/**
|
||||
* Constructor
|
||||
* @param {Game} game.
|
||||
* @return {Touch} This object.
|
||||
*/
|
||||
constructor(game: Game) {
|
||||
|
||||
this._game = game;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Local private reference to game.
|
||||
* @property _game
|
||||
* @type {Game}
|
||||
* @private
|
||||
**/
|
||||
private _game: Game;
|
||||
|
||||
private _p1: Pointer;
|
||||
private _p2: Pointer;
|
||||
private _p3: Pointer;
|
||||
private _p4: Pointer;
|
||||
private _p5: Pointer;
|
||||
private _p6: Pointer;
|
||||
private _p7: Pointer;
|
||||
private _p8: Pointer;
|
||||
private _p9: Pointer;
|
||||
private _p10: Pointer;
|
||||
|
||||
public start() {
|
||||
|
||||
// Local references to the Phaser.Input.pointer objects
|
||||
this._p1 = this._game.input.pointer1;
|
||||
this._p2 = this._game.input.pointer2;
|
||||
this._p3 = this._game.input.pointer3;
|
||||
this._p4 = this._game.input.pointer4;
|
||||
this._p5 = this._game.input.pointer5;
|
||||
this._p6 = this._game.input.pointer6;
|
||||
this._p7 = this._game.input.pointer7;
|
||||
this._p8 = this._game.input.pointer8;
|
||||
this._p9 = this._game.input.pointer9;
|
||||
this._p10 = this._game.input.pointer10;
|
||||
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
}
|
|
@ -1,11 +1,14 @@
|
|||
/// <reference path="../../Game.ts" />
|
||||
/// <reference path="../../Signal.ts" />
|
||||
/// <reference path="Pointer.ts" />
|
||||
/// <reference path="MSPointer.ts" />
|
||||
/// <reference path="Gestures.ts" />
|
||||
|
||||
/**
|
||||
* Phaser - Input
|
||||
*
|
||||
* A game specific Input manager that looks after the mouse, keyboard and touch objects. This is updated by the core game loop.
|
||||
* A game specific Input manager that looks after the mouse, keyboard and touch objects.
|
||||
* This is updated by the core game loop.
|
||||
*/
|
||||
|
||||
module Phaser {
|
||||
|
@ -16,53 +19,83 @@ module Phaser {
|
|||
|
||||
this._game = game;
|
||||
|
||||
this.pointer1 = new Pointer(this._game, 1);
|
||||
this.pointer2 = new Pointer(this._game, 2);
|
||||
this.pointer3 = new Pointer(this._game, 3);
|
||||
this.pointer4 = new Pointer(this._game, 4);
|
||||
this.pointer5 = new Pointer(this._game, 5);
|
||||
this.pointer6 = new Pointer(this._game, 6);
|
||||
this.pointer7 = new Pointer(this._game, 7);
|
||||
this.pointer8 = new Pointer(this._game, 8);
|
||||
this.pointer9 = new Pointer(this._game, 9);
|
||||
this.pointer10 = new Pointer(this._game, 10);
|
||||
|
||||
this.mouse = new Mouse(this._game);
|
||||
this.keyboard = new Keyboard(this._game);
|
||||
this.touch = new Touch(this._game);
|
||||
this.mspointer = new MSPointer(this._game);
|
||||
this.gestures = new Gestures(this._game);
|
||||
|
||||
this.onDown = new Phaser.Signal();
|
||||
this.onUp = new Phaser.Signal();
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Local private reference to game.
|
||||
*/
|
||||
private _game: Game;
|
||||
|
||||
/**
|
||||
*
|
||||
* @type {Mouse}
|
||||
*/
|
||||
* You can disable all Input by setting Input.disabled = true. While set all new input related events will be ignored.
|
||||
* If you need to disable just one type of input, for example mouse, use Input.mouse.disabled = true instead
|
||||
* @type {Boolean}
|
||||
*/
|
||||
public disabled: bool = false;
|
||||
|
||||
/**
|
||||
* Phaser.Mouse handler
|
||||
* @type {Mouse}
|
||||
*/
|
||||
public mouse: Mouse;
|
||||
|
||||
/**
|
||||
*
|
||||
* @type {Keyboard}
|
||||
*/
|
||||
* Phaser.Keyboard handler
|
||||
* @type {Keyboard}
|
||||
*/
|
||||
public keyboard: Keyboard;
|
||||
|
||||
/**
|
||||
*
|
||||
* @type {Touch}
|
||||
*/
|
||||
* Phaser.Touch handler
|
||||
* @type {Touch}
|
||||
*/
|
||||
public touch: Touch;
|
||||
|
||||
/**
|
||||
*
|
||||
* @type {MSPointer}
|
||||
*/
|
||||
* Phaser.MSPointer handler
|
||||
* @type {MSPointer}
|
||||
*/
|
||||
public mspointer: MSPointer;
|
||||
|
||||
/**
|
||||
*
|
||||
* @type {Number}
|
||||
*/
|
||||
public x: number = 0;
|
||||
* Phaser.Gestures handler
|
||||
* @type {Gestures}
|
||||
*/
|
||||
public gestures: Gestures;
|
||||
|
||||
/**
|
||||
*
|
||||
* @type {Number}
|
||||
*/
|
||||
public y: number = 0;
|
||||
* X coordinate of the most recent Pointer event
|
||||
* @type {Number}
|
||||
* @private
|
||||
*/
|
||||
private _x: number = 0;
|
||||
|
||||
/**
|
||||
* X coordinate of the most recent Pointer event
|
||||
* @type {Number}
|
||||
* @private
|
||||
*/
|
||||
private _y: number = 0;
|
||||
|
||||
/**
|
||||
*
|
||||
|
@ -89,27 +122,162 @@ module Phaser {
|
|||
public worldY: number = 0;
|
||||
|
||||
/**
|
||||
*
|
||||
* @type {Phaser.Signal}
|
||||
*/
|
||||
* The maximum number of Pointers allowed to be active at any one time.
|
||||
* For lots of games it's useful to set this to 1
|
||||
* @type {Number}
|
||||
*/
|
||||
public maxPointers: number = 10;
|
||||
|
||||
/**
|
||||
* A Signal dispatched when a mouse/Pointer object is pressed
|
||||
* @type {Phaser.Signal}
|
||||
*/
|
||||
public onDown: Phaser.Signal;
|
||||
|
||||
/**
|
||||
*
|
||||
* @type {Phaser.Signal}
|
||||
*/
|
||||
* A Signal dispatched when a mouse/Pointer object is released
|
||||
* @type {Phaser.Signal}
|
||||
*/
|
||||
public onUp: Phaser.Signal;
|
||||
|
||||
/**
|
||||
* A Signal dispatched when a Pointer object (including the mouse) is tapped: pressed and released quickly
|
||||
* @type {Phaser.Signal}
|
||||
*/
|
||||
public onTap: Phaser.Signal;
|
||||
|
||||
/**
|
||||
* A Signal dispatched when a Pointer object (including the mouse) is double tapped: pressed and released quickly twice in succession
|
||||
* @type {Phaser.Signal}
|
||||
*/
|
||||
public onDoubleTap: Phaser.Signal;
|
||||
|
||||
/**
|
||||
* A Signal dispatched when a Pointer object (including the mouse) is held down
|
||||
* @type {Phaser.Signal}
|
||||
*/
|
||||
public onHold: Phaser.Signal;
|
||||
|
||||
/**
|
||||
* A Pointer object
|
||||
* @property pointer1
|
||||
* @type {Pointer}
|
||||
**/
|
||||
public pointer1: Pointer;
|
||||
|
||||
/**
|
||||
* A Pointer object
|
||||
* @property pointer2
|
||||
* @type {Pointer}
|
||||
**/
|
||||
public pointer2: Pointer;
|
||||
|
||||
/**
|
||||
* A Pointer object
|
||||
* @property pointer3
|
||||
* @type {Pointer}
|
||||
**/
|
||||
public pointer3: Pointer;
|
||||
|
||||
/**
|
||||
* A Pointer object
|
||||
* @property pointer4
|
||||
* @type {Pointer}
|
||||
**/
|
||||
public pointer4: Pointer;
|
||||
|
||||
/**
|
||||
* A Pointer object
|
||||
* @property pointer5
|
||||
* @type {Pointer}
|
||||
**/
|
||||
public pointer5: Pointer;
|
||||
|
||||
/**
|
||||
* A Pointer object
|
||||
* @property pointer6
|
||||
* @type {Pointer}
|
||||
**/
|
||||
public pointer6: Pointer;
|
||||
|
||||
/**
|
||||
* A Pointer object
|
||||
* @property pointer7
|
||||
* @type {Pointer}
|
||||
**/
|
||||
public pointer7: Pointer;
|
||||
|
||||
/**
|
||||
* A Pointer object
|
||||
* @property pointer8
|
||||
* @type {Pointer}
|
||||
**/
|
||||
public pointer8: Pointer;
|
||||
|
||||
/**
|
||||
* A Pointer object
|
||||
* @property pointer9
|
||||
* @type {Pointer}
|
||||
**/
|
||||
public pointer9: Pointer;
|
||||
|
||||
/**
|
||||
* A Pointer object
|
||||
* @property pointer10
|
||||
* @type {Pointer}
|
||||
**/
|
||||
public pointer10: Pointer;
|
||||
|
||||
/**
|
||||
* The screen X coordinate
|
||||
* @property x
|
||||
* @type {Number}
|
||||
**/
|
||||
public get x(): number {
|
||||
|
||||
return this._x;
|
||||
|
||||
}
|
||||
|
||||
public set x(value: number) {
|
||||
|
||||
this._x = Math.round(value);
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* The screen Y coordinate
|
||||
* @property y
|
||||
* @type {Number}
|
||||
**/
|
||||
public get y(): number {
|
||||
|
||||
return this._y;
|
||||
|
||||
}
|
||||
|
||||
public set y(value: number) {
|
||||
|
||||
this._y = Math.round(value);
|
||||
|
||||
}
|
||||
|
||||
public start() {
|
||||
|
||||
this.mouse.start();
|
||||
this.keyboard.start();
|
||||
this.touch.start();
|
||||
this.mspointer.start();
|
||||
this.gestures.start();
|
||||
|
||||
}
|
||||
|
||||
public update() {
|
||||
|
||||
this.x = Math.round(this.x);
|
||||
this.y = Math.round(this.y);
|
||||
|
||||
this.worldX = this._game.camera.worldView.x + this.x;
|
||||
this.worldY = this._game.camera.worldView.y + this.y;
|
||||
//this.worldX = this._game.camera.worldView.x + this.x;
|
||||
//this.worldY = this._game.camera.worldView.y + this.y;
|
||||
|
||||
this.mouse.update();
|
||||
this.touch.update();
|
||||
|
||||
}
|
||||
|
||||
|
@ -117,7 +285,360 @@ module Phaser {
|
|||
|
||||
this.mouse.reset();
|
||||
this.keyboard.reset();
|
||||
this.touch.reset();
|
||||
|
||||
this.pointer1.reset();
|
||||
this.pointer2.reset();
|
||||
this.pointer3.reset();
|
||||
this.pointer4.reset();
|
||||
this.pointer5.reset();
|
||||
this.pointer6.reset();
|
||||
this.pointer7.reset();
|
||||
this.pointer8.reset();
|
||||
this.pointer9.reset();
|
||||
this.pointer10.reset();
|
||||
|
||||
this.onDown = new Phaser.Signal();
|
||||
this.onUp = new Phaser.Signal();
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Get the total number of inactive Pointers
|
||||
* @method totalInactivePointers
|
||||
* @return {Number} The number of Pointers currently inactive
|
||||
**/
|
||||
public get totalInactivePointers(): number {
|
||||
|
||||
return 10 - this.totalActivePointers;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Get the total number of active Pointers
|
||||
* @method totalActivePointers
|
||||
* @return {Number} The number of Pointers currently active
|
||||
**/
|
||||
public get totalActivePointers(): number {
|
||||
|
||||
var result: number = 0;
|
||||
|
||||
if (this.pointer1.active == true)
|
||||
{
|
||||
result++;
|
||||
}
|
||||
else if (this.pointer2.active == true)
|
||||
{
|
||||
result++;
|
||||
}
|
||||
else if (this.pointer3.active == true)
|
||||
{
|
||||
result++;
|
||||
}
|
||||
else if (this.pointer4.active == true)
|
||||
{
|
||||
result++;
|
||||
}
|
||||
else if (this.pointer5.active == true)
|
||||
{
|
||||
result++;
|
||||
}
|
||||
else if (this.pointer6.active == true)
|
||||
{
|
||||
result++;
|
||||
}
|
||||
else if (this.pointer7.active == true)
|
||||
{
|
||||
result++;
|
||||
}
|
||||
else if (this.pointer8.active == true)
|
||||
{
|
||||
result++;
|
||||
}
|
||||
else if (this.pointer9.active == true)
|
||||
{
|
||||
result++;
|
||||
}
|
||||
else if (this.pointer10.active == true)
|
||||
{
|
||||
result++;
|
||||
}
|
||||
|
||||
return result;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Find the first free Pointer object and start it, passing in the event data.
|
||||
* @method startPointer
|
||||
* @param {Any} event The event data from the Touch event
|
||||
* @return {Pointer} The Pointer object that was started or null if no Pointer object is available
|
||||
**/
|
||||
public startPointer(event):Pointer {
|
||||
|
||||
if (this.maxPointers < 10 && this.totalActivePointers == this.maxPointers)
|
||||
{
|
||||
return null;
|
||||
}
|
||||
|
||||
// Unrolled for speed
|
||||
if (this.pointer1.active == false)
|
||||
{
|
||||
return this.pointer1.start(event);
|
||||
}
|
||||
else if (this.pointer2.active == false)
|
||||
{
|
||||
return this.pointer2.start(event);
|
||||
}
|
||||
else if (this.pointer3.active == false)
|
||||
{
|
||||
return this.pointer3.start(event);
|
||||
}
|
||||
else if (this.pointer4.active == false)
|
||||
{
|
||||
return this.pointer4.start(event);
|
||||
}
|
||||
else if (this.pointer5.active == false)
|
||||
{
|
||||
return this.pointer5.start(event);
|
||||
}
|
||||
else if (this.pointer6.active == false)
|
||||
{
|
||||
return this.pointer6.start(event);
|
||||
}
|
||||
else if (this.pointer7.active == false)
|
||||
{
|
||||
return this.pointer7.start(event);
|
||||
}
|
||||
else if (this.pointer8.active == false)
|
||||
{
|
||||
return this.pointer8.start(event);
|
||||
}
|
||||
else if (this.pointer9.active == false)
|
||||
{
|
||||
return this.pointer9.start(event);
|
||||
}
|
||||
else if (this.pointer10.active == false)
|
||||
{
|
||||
return this.pointer10.start(event);
|
||||
}
|
||||
|
||||
return null;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Updates the matching Pointer object, passing in the event data.
|
||||
* @method updatePointer
|
||||
* @param {Any} event The event data from the Touch event
|
||||
* @return {Pointer} The Pointer object that was updated or null if no Pointer object is available
|
||||
**/
|
||||
public updatePointer(event):Pointer {
|
||||
|
||||
// Unrolled for speed
|
||||
if (this.pointer1.active == true && this.pointer1.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer1.move(event);
|
||||
}
|
||||
else if (this.pointer2.active == true && this.pointer2.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer2.move(event);
|
||||
}
|
||||
else if (this.pointer3.active == true && this.pointer3.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer3.move(event);
|
||||
}
|
||||
else if (this.pointer4.active == true && this.pointer4.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer4.move(event);
|
||||
}
|
||||
else if (this.pointer5.active == true && this.pointer5.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer5.move(event);
|
||||
}
|
||||
else if (this.pointer6.active == true && this.pointer6.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer6.move(event);
|
||||
}
|
||||
else if (this.pointer7.active == true && this.pointer7.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer7.move(event);
|
||||
}
|
||||
else if (this.pointer8.active == true && this.pointer8.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer8.move(event);
|
||||
}
|
||||
else if (this.pointer9.active == true && this.pointer9.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer9.move(event);
|
||||
}
|
||||
else if (this.pointer10.active == true && this.pointer10.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer10.move(event);
|
||||
}
|
||||
|
||||
return null;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Stops the matching Pointer object, passing in the event data.
|
||||
* @method stopPointer
|
||||
* @param {Any} event The event data from the Touch event
|
||||
* @return {Pointer} The Pointer object that was stopped or null if no Pointer object is available
|
||||
**/
|
||||
public stopPointer(event):Pointer {
|
||||
|
||||
// Unrolled for speed
|
||||
if (this.pointer1.active == true && this.pointer1.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer1.stop(event);
|
||||
}
|
||||
else if (this.pointer2.active == true && this.pointer2.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer2.stop(event);
|
||||
}
|
||||
else if (this.pointer3.active == true && this.pointer3.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer3.stop(event);
|
||||
}
|
||||
else if (this.pointer4.active == true && this.pointer4.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer4.stop(event);
|
||||
}
|
||||
else if (this.pointer5.active == true && this.pointer5.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer5.stop(event);
|
||||
}
|
||||
else if (this.pointer6.active == true && this.pointer6.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer6.stop(event);
|
||||
}
|
||||
else if (this.pointer7.active == true && this.pointer7.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer7.stop(event);
|
||||
}
|
||||
else if (this.pointer8.active == true && this.pointer8.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer8.stop(event);
|
||||
}
|
||||
else if (this.pointer9.active == true && this.pointer9.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer9.stop(event);
|
||||
}
|
||||
else if (this.pointer10.active == true && this.pointer10.identifier == event.identifier)
|
||||
{
|
||||
return this.pointer10.stop(event);
|
||||
}
|
||||
|
||||
return null;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Get the next Pointer object whos active property matches the given state
|
||||
* @method getPointer
|
||||
* @param {Boolean} state The state the Pointer should be in (false for inactive, true for active)
|
||||
* @return {Pointer} A Pointer object or null if no Pointer object matches the requested state.
|
||||
**/
|
||||
public getPointer(state: bool = false): Pointer {
|
||||
|
||||
// Unrolled for speed
|
||||
if (this.pointer1.active == state)
|
||||
{
|
||||
return this.pointer1;
|
||||
}
|
||||
else if (this.pointer2.active == state)
|
||||
{
|
||||
return this.pointer2;
|
||||
}
|
||||
else if (this.pointer3.active == state)
|
||||
{
|
||||
return this.pointer3;
|
||||
}
|
||||
else if (this.pointer4.active == state)
|
||||
{
|
||||
return this.pointer4;
|
||||
}
|
||||
else if (this.pointer5.active == state)
|
||||
{
|
||||
return this.pointer5;
|
||||
}
|
||||
else if (this.pointer6.active == state)
|
||||
{
|
||||
return this.pointer6;
|
||||
}
|
||||
else if (this.pointer7.active == state)
|
||||
{
|
||||
return this.pointer7;
|
||||
}
|
||||
else if (this.pointer8.active == state)
|
||||
{
|
||||
return this.pointer8;
|
||||
}
|
||||
else if (this.pointer9.active == state)
|
||||
{
|
||||
return this.pointer9;
|
||||
}
|
||||
else if (this.pointer10.active == state)
|
||||
{
|
||||
return this.pointer10;
|
||||
}
|
||||
|
||||
return null;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Get the Pointer object whos identified property matches the given identifier value
|
||||
* @method getPointerFromIdentifier
|
||||
* @param {Number} identifier The Pointer.identifier value to search for
|
||||
* @return {Pointer} A Pointer object or null if no Pointer object matches the requested identifier.
|
||||
**/
|
||||
public getPointerFromIdentifier(identifier: number): Pointer {
|
||||
|
||||
// Unrolled for speed
|
||||
if (this.pointer1.identifier == identifier)
|
||||
{
|
||||
return this.pointer1;
|
||||
}
|
||||
else if (this.pointer2.identifier == identifier)
|
||||
{
|
||||
return this.pointer2;
|
||||
}
|
||||
else if (this.pointer3.identifier == identifier)
|
||||
{
|
||||
return this.pointer3;
|
||||
}
|
||||
else if (this.pointer4.identifier == identifier)
|
||||
{
|
||||
return this.pointer4;
|
||||
}
|
||||
else if (this.pointer5.identifier == identifier)
|
||||
{
|
||||
return this.pointer5;
|
||||
}
|
||||
else if (this.pointer6.identifier == identifier)
|
||||
{
|
||||
return this.pointer6;
|
||||
}
|
||||
else if (this.pointer7.identifier == identifier)
|
||||
{
|
||||
return this.pointer7;
|
||||
}
|
||||
else if (this.pointer8.identifier == identifier)
|
||||
{
|
||||
return this.pointer8;
|
||||
}
|
||||
else if (this.pointer9.identifier == identifier)
|
||||
{
|
||||
return this.pointer9;
|
||||
}
|
||||
else if (this.pointer10.identifier == identifier)
|
||||
{
|
||||
return this.pointer10;
|
||||
}
|
||||
|
||||
return null;
|
||||
|
||||
}
|
||||
|
||||
|
@ -155,6 +676,34 @@ module Phaser {
|
|||
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method calculateDistance
|
||||
* @param {Finger} finger1
|
||||
* @param {Finger} finger2
|
||||
**/
|
||||
//public calculateDistance(finger1: Finger, finger2: Finger) {
|
||||
//}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method calculateAngle
|
||||
* @param {Finger} finger1
|
||||
* @param {Finger} finger2
|
||||
**/
|
||||
//public calculateAngle(finger1: Finger, finger2: Finger) {
|
||||
//}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method checkOverlap
|
||||
* @param {Finger} finger1
|
||||
* @param {Finger} finger2
|
||||
**/
|
||||
//public checkOverlap(finger1: Finger, finger2: Finger) {
|
||||
//}
|
||||
|
||||
|
||||
}
|
||||
|
||||
}
|
|
@ -15,7 +15,6 @@ module Phaser {
|
|||
constructor(game: Game) {
|
||||
|
||||
this._game = game;
|
||||
this.start();
|
||||
|
||||
}
|
||||
|
||||
|
@ -23,6 +22,12 @@ module Phaser {
|
|||
private _keys = {};
|
||||
private _capture = {};
|
||||
|
||||
/**
|
||||
* You can disable all Input by setting disabled = true. While set all new input related events will be ignored.
|
||||
* @type {Boolean}
|
||||
*/
|
||||
public disabled: bool = false;
|
||||
|
||||
public start() {
|
||||
|
||||
document.body.addEventListener('keydown', (event: KeyboardEvent) => this.onKeyDown(event), false);
|
||||
|
@ -69,6 +74,11 @@ module Phaser {
|
|||
*/
|
||||
public onKeyDown(event: KeyboardEvent) {
|
||||
|
||||
if (this._game.input.disabled || this.disabled)
|
||||
{
|
||||
return;
|
||||
}
|
||||
|
||||
if (this._capture[event.keyCode])
|
||||
{
|
||||
event.preventDefault();
|
||||
|
@ -91,6 +101,11 @@ module Phaser {
|
|||
*/
|
||||
public onKeyUp(event: KeyboardEvent) {
|
||||
|
||||
if (this._game.input.disabled || this.disabled)
|
||||
{
|
||||
return;
|
||||
}
|
||||
|
||||
if (this._capture[event.keyCode])
|
||||
{
|
||||
event.preventDefault();
|
||||
|
|
|
@ -1,15 +1,12 @@
|
|||
/// <reference path="../../Game.ts" />
|
||||
/// <reference path="Finger.ts" />
|
||||
/// <reference path="Pointer.ts" />
|
||||
|
||||
/**
|
||||
* Phaser - MSPointer
|
||||
*
|
||||
* The MSPointer class handles touch interactions with the game and the resulting Finger objects.
|
||||
* The MSPointer class handles touch interactions with the game and the resulting Pointer objects.
|
||||
* It will work only in Internet Explorer 10 and Windows Store or Windows Phone 8 apps using JavaScript.
|
||||
* http://msdn.microsoft.com/en-us/library/ie/hh673557(v=vs.85).aspx
|
||||
*
|
||||
*
|
||||
* @todo Gestures (pinch, zoom, swipe)
|
||||
*/
|
||||
|
||||
module Phaser {
|
||||
|
@ -25,24 +22,6 @@ module Phaser {
|
|||
|
||||
this._game = game;
|
||||
|
||||
this.finger1 = new Finger(this._game);
|
||||
this.finger2 = new Finger(this._game);
|
||||
this.finger3 = new Finger(this._game);
|
||||
this.finger4 = new Finger(this._game);
|
||||
this.finger5 = new Finger(this._game);
|
||||
this.finger6 = new Finger(this._game);
|
||||
this.finger7 = new Finger(this._game);
|
||||
this.finger8 = new Finger(this._game);
|
||||
this.finger9 = new Finger(this._game);
|
||||
this.finger10 = new Finger(this._game);
|
||||
|
||||
this._fingers = [this.finger1, this.finger2, this.finger3, this.finger4, this.finger5, this.finger6, this.finger7, this.finger8, this.finger9, this.finger10];
|
||||
|
||||
this.touchDown = new Signal();
|
||||
this.touchUp = new Signal();
|
||||
|
||||
this.start();
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
|
@ -53,121 +32,11 @@ module Phaser {
|
|||
**/
|
||||
private _game: Game;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property x
|
||||
* @type Number
|
||||
**/
|
||||
public x: number = 0;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property y
|
||||
* @type Number
|
||||
**/
|
||||
public y: number = 0;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property _fingers
|
||||
* @type Array
|
||||
* @private
|
||||
**/
|
||||
private _fingers: Finger[];
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger1
|
||||
* @type Finger
|
||||
**/
|
||||
public finger1: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger2
|
||||
* @type Finger
|
||||
**/
|
||||
public finger2: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger3
|
||||
* @type Finger
|
||||
**/
|
||||
public finger3: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger4
|
||||
* @type Finger
|
||||
**/
|
||||
public finger4: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger5
|
||||
* @type Finger
|
||||
**/
|
||||
public finger5: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger6
|
||||
* @type Finger
|
||||
**/
|
||||
public finger6: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger7
|
||||
* @type Finger
|
||||
**/
|
||||
public finger7: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger8
|
||||
* @type Finger
|
||||
**/
|
||||
public finger8: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger9
|
||||
* @type Finger
|
||||
**/
|
||||
public finger9: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger10
|
||||
* @type Finger
|
||||
**/
|
||||
public finger10: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property latestFinger
|
||||
* @type Finger
|
||||
**/
|
||||
public latestFinger: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property isDown
|
||||
* @type Boolean
|
||||
**/
|
||||
public isDown: bool = false;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property isUp
|
||||
* @type Boolean
|
||||
**/
|
||||
public isUp: bool = true;
|
||||
|
||||
public touchDown: Signal;
|
||||
public touchUp: Signal;
|
||||
/**
|
||||
* You can disable all Input by setting disabled = true. While set all new input related events will be ignored.
|
||||
* @type {Boolean}
|
||||
*/
|
||||
public disabled: bool = false;
|
||||
|
||||
/**
|
||||
*
|
||||
|
@ -175,7 +44,7 @@ module Phaser {
|
|||
*/
|
||||
public start() {
|
||||
|
||||
if (navigator.msMaxTouchPoints)
|
||||
if (this._game.device.mspointer == true)
|
||||
{
|
||||
this._game.stage.canvas.addEventListener('MSPointerDown', (event) => this.onPointerDown(event), false);
|
||||
this._game.stage.canvas.addEventListener('MSPointerMove', (event) => this.onPointerMove(event), false);
|
||||
|
@ -191,25 +60,16 @@ module Phaser {
|
|||
**/
|
||||
private onPointerDown(event) {
|
||||
|
||||
event.preventDefault();
|
||||
|
||||
for (var f = 0; f < this._fingers.length; f++)
|
||||
if (this._game.input.disabled || this.disabled)
|
||||
{
|
||||
if (this._fingers[f].active === false)
|
||||
{
|
||||
event.identifier = event.pointerId;
|
||||
this._fingers[f].start(event);
|
||||
this.x = this._fingers[f].x;
|
||||
this.y = this._fingers[f].y;
|
||||
this._game.input.x = this.x * this._game.input.scaleX;
|
||||
this._game.input.y = this.y * this._game.input.scaleY;
|
||||
this.touchDown.dispatch(this._fingers[f].x, this._fingers[f].y, this._fingers[f].timeDown, this._fingers[f].timeUp, this._fingers[f].duration);
|
||||
this._game.input.onDown.dispatch(this._game.input.x, this._game.input.y, this._fingers[f].timeDown);
|
||||
this.isDown = true;
|
||||
this.isUp = false;
|
||||
break;
|
||||
}
|
||||
return;
|
||||
}
|
||||
|
||||
event.preventDefault();
|
||||
event.identifier = event.pointerId;
|
||||
|
||||
this._game.input.startPointer(event);
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
|
@ -219,22 +79,16 @@ module Phaser {
|
|||
**/
|
||||
private onPointerMove(event) {
|
||||
|
||||
event.preventDefault();
|
||||
|
||||
for (var f = 0; f < this._fingers.length; f++)
|
||||
if (this._game.input.disabled || this.disabled)
|
||||
{
|
||||
if (this._fingers[f].identifier === event.pointerId &&
|
||||
this._fingers[f].active === true)
|
||||
{
|
||||
event.identifier = event.pointerId;
|
||||
this._fingers[f].move(event);
|
||||
this.x = this._fingers[f].x;
|
||||
this.y = this._fingers[f].y;
|
||||
this._game.input.x = this.x * this._game.input.scaleX;
|
||||
this._game.input.y = this.y * this._game.input.scaleY;
|
||||
break;
|
||||
}
|
||||
return;
|
||||
}
|
||||
|
||||
event.preventDefault();
|
||||
event.identifier = event.pointerId;
|
||||
|
||||
this._game.input.updatePointer(event);
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
|
@ -244,61 +98,15 @@ module Phaser {
|
|||
**/
|
||||
private onPointerUp(event) {
|
||||
|
||||
event.preventDefault();
|
||||
|
||||
for (var f = 0; f < this._fingers.length; f++)
|
||||
if (this._game.input.disabled || this.disabled)
|
||||
{
|
||||
|
||||
if (this._fingers[f].identifier === event.pointerId)
|
||||
{
|
||||
event.identifier = event.pointerId;
|
||||
this._fingers[f].stop(event);
|
||||
this.x = this._fingers[f].x;
|
||||
this.y = this._fingers[f].y;
|
||||
this._game.input.x = this.x * this._game.input.scaleX;
|
||||
this._game.input.y = this.y * this._game.input.scaleY;
|
||||
this.touchUp.dispatch(this._fingers[f].x, this._fingers[f].y, this._fingers[f].timeDown, this._fingers[f].timeUp, this._fingers[f].duration);
|
||||
this._game.input.onUp.dispatch(this._game.input.x, this._game.input.y, this._fingers[f].timeUp);
|
||||
this.isDown = false;
|
||||
this.isUp = true;
|
||||
break;
|
||||
}
|
||||
return;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method calculateDistance
|
||||
* @param {Finger} finger1
|
||||
* @param {Finger} finger2
|
||||
**/
|
||||
public calculateDistance(finger1: Finger, finger2: Finger) {
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method calculateAngle
|
||||
* @param {Finger} finger1
|
||||
* @param {Finger} finger2
|
||||
**/
|
||||
public calculateAngle(finger1: Finger, finger2: Finger) {
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method checkOverlap
|
||||
* @param {Finger} finger1
|
||||
* @param {Finger} finger2
|
||||
**/
|
||||
public checkOverlap(finger1: Finger, finger2: Finger) {
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method update
|
||||
*/
|
||||
public update() {
|
||||
event.preventDefault();
|
||||
event.identifier = event.pointerId;
|
||||
|
||||
this._game.input.stopPointer(event);
|
||||
|
||||
}
|
||||
|
||||
|
@ -310,17 +118,6 @@ module Phaser {
|
|||
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method reset
|
||||
**/
|
||||
public reset() {
|
||||
|
||||
this.isDown = false;
|
||||
this.isUp = false;
|
||||
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
}
|
|
@ -13,7 +13,6 @@ module Phaser {
|
|||
constructor(game: Game) {
|
||||
|
||||
this._game = game;
|
||||
this.start();
|
||||
|
||||
}
|
||||
|
||||
|
@ -28,22 +27,32 @@ module Phaser {
|
|||
public static MIDDLE_BUTTON: number = 1;
|
||||
public static RIGHT_BUTTON: number = 2;
|
||||
|
||||
/**
|
||||
* You can disable all Input by setting disabled = true. While set all new input related events will be ignored.
|
||||
* @type {Boolean}
|
||||
*/
|
||||
public disabled: bool = false;
|
||||
|
||||
/**
|
||||
* @type {Boolean}
|
||||
*/
|
||||
public isDown: bool = false;
|
||||
|
||||
/**
|
||||
* @type {Boolean}
|
||||
*/
|
||||
public isUp: bool = true;
|
||||
|
||||
/**
|
||||
* @type {Number}
|
||||
*/
|
||||
public timeDown: number = 0;
|
||||
|
||||
/**
|
||||
* @type {Number}
|
||||
*/
|
||||
public duration: number = 0;
|
||||
|
||||
/**
|
||||
* @type {Number}
|
||||
*/
|
||||
|
@ -69,6 +78,11 @@ module Phaser {
|
|||
*/
|
||||
public onMouseDown(event: MouseEvent) {
|
||||
|
||||
if (this._game.input.disabled || this.disabled)
|
||||
{
|
||||
return;
|
||||
}
|
||||
|
||||
this.button = event.button;
|
||||
|
||||
this._x = event.clientX - this._game.stage.x;
|
||||
|
@ -102,6 +116,11 @@ module Phaser {
|
|||
*/
|
||||
public onMouseMove(event: MouseEvent) {
|
||||
|
||||
if (this._game.input.disabled || this.disabled)
|
||||
{
|
||||
return;
|
||||
}
|
||||
|
||||
this.button = event.button;
|
||||
|
||||
this._x = event.clientX - this._game.stage.x;
|
||||
|
@ -117,6 +136,11 @@ module Phaser {
|
|||
*/
|
||||
public onMouseUp(event: MouseEvent) {
|
||||
|
||||
if (this._game.input.disabled || this.disabled)
|
||||
{
|
||||
return;
|
||||
}
|
||||
|
||||
this.button = event.button;
|
||||
this.isDown = false;
|
||||
this.isUp = true;
|
||||
|
|
430
Phaser/system/input/Pointer.ts
Normal file
430
Phaser/system/input/Pointer.ts
Normal file
|
@ -0,0 +1,430 @@
|
|||
/// <reference path="../../Game.ts" />
|
||||
|
||||
/**
|
||||
* Phaser - Pointer
|
||||
*
|
||||
* A Pointer object is used by the Touch and MSPoint managers and represents a single finger on the touch screen.
|
||||
*/
|
||||
|
||||
module Phaser {
|
||||
|
||||
export class Pointer {
|
||||
|
||||
/**
|
||||
* Constructor
|
||||
* @param {Phaser.Game} game.
|
||||
* @return {Phaser.Pointer} This object.
|
||||
*/
|
||||
constructor(game: Game, id: number) {
|
||||
|
||||
this._game = game;
|
||||
|
||||
this.id = id;
|
||||
this.active = false;
|
||||
this.pointA = new Point();
|
||||
this.pointB = new Point();
|
||||
this.circle = new Circle(0, 0, 44);
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Local private reference to game.
|
||||
* @property _game
|
||||
* @type {Phaser.Game}
|
||||
* @private
|
||||
**/
|
||||
private _game: Game;
|
||||
|
||||
/**
|
||||
* The Pointer ID (a number between 1 and 10)
|
||||
* @property id
|
||||
* @type {Number}
|
||||
*/
|
||||
public id: number;
|
||||
|
||||
/**
|
||||
* An identification number for each touch point.
|
||||
* When a touch point becomes active, it is assigned an identifier that is distinct from any other active touch point.
|
||||
* While the touch point remains active, all events that refer to it are assigned the same identifier.
|
||||
* @property identifier
|
||||
* @type {Number}
|
||||
*/
|
||||
public identifier: number;
|
||||
|
||||
/**
|
||||
* Is this Pointer active or not? An active Pointer is one that is in contact with the touch screen.
|
||||
* @property active
|
||||
* @type {Boolean}
|
||||
*/
|
||||
public active: bool;
|
||||
|
||||
/**
|
||||
* A Point object representing the x/y screen coordinates of the Pointer.
|
||||
* @property pointA
|
||||
* @type {Point}
|
||||
**/
|
||||
public pointA: Point = null;
|
||||
|
||||
/**
|
||||
* A Point object representing the x/y screen coordinates of the Pointer.
|
||||
* @property pointB
|
||||
* @type {Point}
|
||||
**/
|
||||
public pointB: Point = null;
|
||||
|
||||
/**
|
||||
* A Circle object centered on the x/y screen coordinates of the Pointer.
|
||||
* Default size of 44px (Apple's recommended "finger tip" size)
|
||||
* @property circle
|
||||
* @type {Circle}
|
||||
**/
|
||||
public circle: Circle = null;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property withinGame
|
||||
* @type {Boolean}
|
||||
*/
|
||||
public withinGame: bool = false;
|
||||
|
||||
/**
|
||||
* The horizontal coordinate of point relative to the viewport in pixels, excluding any scroll offset
|
||||
* @property clientX
|
||||
* @type {Number}
|
||||
*/
|
||||
public clientX: number = -1;
|
||||
|
||||
/**
|
||||
* The vertical coordinate of point relative to the viewport in pixels, excluding any scroll offset
|
||||
* @property clientY
|
||||
* @type {Number}
|
||||
*/
|
||||
public clientY: number = -1;
|
||||
|
||||
/**
|
||||
* The horizontal coordinate of point relative to the viewport in pixels, including any scroll offset
|
||||
* @property pageX
|
||||
* @type {Number}
|
||||
*/
|
||||
public pageX: number = -1;
|
||||
|
||||
/**
|
||||
* The vertical coordinate of point relative to the viewport in pixels, including any scroll offset
|
||||
* @property pageY
|
||||
* @type {Number}
|
||||
*/
|
||||
public pageY: number = -1;
|
||||
|
||||
/**
|
||||
* The horizontal coordinate of point relative to the screen in pixels
|
||||
* @property screenX
|
||||
* @type {Number}
|
||||
*/
|
||||
public screenX: number = -1;
|
||||
|
||||
/**
|
||||
* The vertical coordinate of point relative to the screen in pixels
|
||||
* @property screenY
|
||||
* @type {Number}
|
||||
*/
|
||||
public screenY: number = -1;
|
||||
|
||||
/**
|
||||
* The horizontal coordinate of point relative to the game element
|
||||
* @property x
|
||||
* @type {Number}
|
||||
*/
|
||||
public x: number = -1;
|
||||
|
||||
/**
|
||||
* The vertical coordinate of point relative to the game element
|
||||
* @property y
|
||||
* @type {Number}
|
||||
*/
|
||||
public y: number = -1;
|
||||
|
||||
/**
|
||||
* The Element on which the touch point started when it was first placed on the surface, even if the touch point has since moved outside the interactive area of that element.
|
||||
* @property target
|
||||
* @type {Any}
|
||||
*/
|
||||
public target;
|
||||
|
||||
/**
|
||||
* If the Pointer is touching the touchscreen isDown is set to true
|
||||
* @property isDown
|
||||
* @type {Boolean}
|
||||
**/
|
||||
public isDown: bool = false;
|
||||
|
||||
/**
|
||||
* If the Pointer is not touching the touchscreen isUp is set to true
|
||||
* @property isUp
|
||||
* @type {Boolean}
|
||||
**/
|
||||
public isUp: bool = true;
|
||||
|
||||
/**
|
||||
* A timestamp representing when the Pointer first touched the touchscreen.
|
||||
* @property timeDown
|
||||
* @type {Number}
|
||||
**/
|
||||
public timeDown: number = 0;
|
||||
|
||||
/**
|
||||
* A timestamp representing when the Pointer left the touchscreen.
|
||||
* @property timeUp
|
||||
* @type {Number}
|
||||
**/
|
||||
public timeUp: number = 0;
|
||||
|
||||
/**
|
||||
* The number of milliseconds below which the Pointer is considered justPressed
|
||||
* @property justPressedRate
|
||||
* @type {Number}
|
||||
**/
|
||||
public justPressedRate: number = 200;
|
||||
|
||||
/**
|
||||
* The number of milliseconds below which the Pointer is considered justReleased
|
||||
* @property justReleasedRate
|
||||
* @type {Number}
|
||||
**/
|
||||
public justReleasedRate: number = 200;
|
||||
|
||||
/**
|
||||
* The total number of times this Pointer has been touched to the touchscreen
|
||||
* @property totalTouches
|
||||
* @type {Number}
|
||||
**/
|
||||
public totalTouches: number = 0;
|
||||
|
||||
/**
|
||||
* How long the Pointer has been depressed on the touchscreen.
|
||||
* @property duration
|
||||
* @type {Number}
|
||||
**/
|
||||
public get duration(): number {
|
||||
|
||||
return this._game.time.now - this.timeDown;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Gets the X value of this Pointer in world coordinate space
|
||||
* @param {Camera} [camera]
|
||||
*/
|
||||
public getWorldX(camera?: Camera = this._game.camera) {
|
||||
|
||||
return camera.worldView.x + this.x;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Gets the Y value of this Pointer in world coordinate space
|
||||
* @param {Camera} [camera]
|
||||
*/
|
||||
public getWorldY(camera?: Camera = this._game.camera) {
|
||||
|
||||
return camera.worldView.y + this.y;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Called when the Pointer is pressed onto the touchscreen
|
||||
* @method start
|
||||
* @param {Any} event
|
||||
*/
|
||||
public start(event): Pointer {
|
||||
|
||||
this.identifier = event.identifier;
|
||||
this.target = event.target;
|
||||
|
||||
this.move(event);
|
||||
|
||||
this.pointA.setTo(this.x, this.y);
|
||||
|
||||
this.active = true;
|
||||
this.withinGame = true;
|
||||
this.isDown = true;
|
||||
this.isUp = false;
|
||||
this.timeDown = this._game.time.now;
|
||||
|
||||
this._game.input.x = this.x * this._game.input.scaleX;
|
||||
this._game.input.y = this.y * this._game.input.scaleY;
|
||||
this._game.input.onDown.dispatch(this);
|
||||
|
||||
this.totalTouches++;
|
||||
|
||||
return this;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Called when the Pointer is moved on the touchscreen
|
||||
* @method move
|
||||
* @param {Any} event
|
||||
*/
|
||||
public move(event): Pointer {
|
||||
|
||||
this.clientX = event.clientX;
|
||||
this.clientY = event.clientY;
|
||||
this.pageX = event.pageX;
|
||||
this.pageY = event.pageY;
|
||||
this.screenX = event.screenX;
|
||||
this.screenY = event.screenY;
|
||||
|
||||
this.x = this.pageX - this._game.stage.offset.x;
|
||||
this.y = this.pageY - this._game.stage.offset.y;
|
||||
|
||||
this.pointB.setTo(this.x, this.y);
|
||||
this.circle.setTo(this.x, this.y, 44);
|
||||
|
||||
this._game.input.x = this.x * this._game.input.scaleX;
|
||||
this._game.input.y = this.y * this._game.input.scaleY;
|
||||
|
||||
// Droppings history (used for gestures and motion tracking)
|
||||
|
||||
return this;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Called when the Pointer leaves the target area
|
||||
* @method leave
|
||||
* @param {Any} event
|
||||
*/
|
||||
public leave(event) {
|
||||
|
||||
this.withinGame = false;
|
||||
this.move(event);
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Called when the Pointer leaves the touchscreen
|
||||
* @method stop
|
||||
* @param {Any} event
|
||||
*/
|
||||
public stop(event): Pointer {
|
||||
|
||||
this.active = false;
|
||||
this.withinGame = false;
|
||||
|
||||
this.isDown = false;
|
||||
this.isUp = true;
|
||||
this.timeUp = this._game.time.now;
|
||||
|
||||
this._game.input.onUp.dispatch(this);
|
||||
|
||||
return this;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* The Pointer is considered justPressed if the time it was pressed onto the touchscreen is less than justPressedRate
|
||||
* @method justPressed
|
||||
* @param {Number} [duration].
|
||||
* @return {Boolean}
|
||||
*/
|
||||
public justPressed(duration?: number = this.justPressedRate): bool {
|
||||
|
||||
if (this.isDown === true && (this.timeDown + duration) > this._game.time.now)
|
||||
{
|
||||
return true;
|
||||
}
|
||||
else
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* The Pointer is considered justReleased if the time it left the touchscreen is less than justReleasedRate
|
||||
* @method justReleased
|
||||
* @param {Number} [duration].
|
||||
* @return {Boolean}
|
||||
*/
|
||||
public justReleased(duration?: number = this.justReleasedRate): bool {
|
||||
|
||||
if (this.isUp === true && (this.timeUp + duration) > this._game.time.now)
|
||||
{
|
||||
return true;
|
||||
}
|
||||
else
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
public reset() {
|
||||
|
||||
this.active = false;
|
||||
this.identifier = null;
|
||||
this.isDown = false;
|
||||
this.isUp = true;
|
||||
this.totalTouches = 0;
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Renders the Pointer.circle object onto the stage in green if down or red if up.
|
||||
* @method renderDebug
|
||||
*/
|
||||
public renderDebug(hideIfUp: bool = false) {
|
||||
|
||||
if (hideIfUp == true && this.isUp == true)
|
||||
{
|
||||
return;
|
||||
}
|
||||
|
||||
this._game.stage.context.beginPath();
|
||||
this._game.stage.context.arc(this.x, this.y, this.circle.radius * 2, 0, Math.PI * 2);
|
||||
|
||||
if (this.active)
|
||||
{
|
||||
this._game.stage.context.fillStyle = 'rgb(0,255,0)';
|
||||
this._game.stage.context.strokeStyle = 'rgb(0,255,0)';
|
||||
}
|
||||
else
|
||||
{
|
||||
this._game.stage.context.fillStyle = 'rgb(100,0,0)';
|
||||
this._game.stage.context.strokeStyle = 'rgb(100,0,0)';
|
||||
}
|
||||
|
||||
this._game.stage.context.fill();
|
||||
this._game.stage.context.closePath();
|
||||
|
||||
// Render the points
|
||||
this._game.stage.context.beginPath();
|
||||
this._game.stage.context.moveTo(this.pointA.x, this.pointA.y);
|
||||
this._game.stage.context.lineTo(this.pointB.x, this.pointB.y);
|
||||
this._game.stage.context.lineWidth = 2;
|
||||
this._game.stage.context.stroke();
|
||||
this._game.stage.context.closePath();
|
||||
|
||||
// Render the text
|
||||
this._game.stage.context.fillStyle = 'rgb(255,255,255)';
|
||||
this._game.stage.context.font = 'Arial 16px';
|
||||
this._game.stage.context.fillText('ID: ' + this.id + " Active: " + this.active, this.x, this.y - 100);
|
||||
this._game.stage.context.fillText('Screen X: ' + this.x + " Screen Y: " + this.y, this.x, this.y - 80);
|
||||
this._game.stage.context.fillText('Duration: ' + this.duration + " ms", this.x, this.y - 60);
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Returns a string representation of this object.
|
||||
* @method toString
|
||||
* @return {String} a string representation of the instance.
|
||||
**/
|
||||
public toString(): string {
|
||||
|
||||
return "[{Pointer (id=" + this.id + " identifer=" + this.identifier + " active=" + this.active + " duration=" + this.duration + " withinGame=" + this.withinGame + " x=" + this.x + " y=" + this.y + " clientX=" + this.clientX + " clientY=" + this.clientY + " screenX=" + this.screenX + " screenY=" + this.screenY + " pageX=" + this.pageX + " pageY=" + this.pageY + ")}]";
|
||||
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
}
|
|
@ -1,20 +1,14 @@
|
|||
/// <reference path="../../Game.ts" />
|
||||
/// <reference path="Finger.ts" />
|
||||
/// <reference path="Pointer.ts" />
|
||||
|
||||
/**
|
||||
* Phaser - Touch
|
||||
*
|
||||
* The Touch class handles touch interactions with the game and the resulting Finger objects.
|
||||
* The Touch class handles touch interactions with the game and the resulting Pointer objects.
|
||||
* http://www.w3.org/TR/touch-events/
|
||||
* https://developer.mozilla.org/en-US/docs/DOM/TouchList
|
||||
* http://www.html5rocks.com/en/mobile/touchandmouse/
|
||||
* Note: Android 2.x only supports 1 touch event at once, no multi-touch
|
||||
*
|
||||
* @todo Try and resolve update lag in Chrome/Android
|
||||
* Gestures (pinch, zoom, swipe)
|
||||
* GameObject Touch
|
||||
* Touch point within GameObject
|
||||
* Input Zones (mouse and touch) - lock entities within them + axis aligned drags
|
||||
*/
|
||||
|
||||
module Phaser {
|
||||
|
@ -30,24 +24,6 @@ module Phaser {
|
|||
|
||||
this._game = game;
|
||||
|
||||
this.finger1 = new Finger(this._game);
|
||||
this.finger2 = new Finger(this._game);
|
||||
this.finger3 = new Finger(this._game);
|
||||
this.finger4 = new Finger(this._game);
|
||||
this.finger5 = new Finger(this._game);
|
||||
this.finger6 = new Finger(this._game);
|
||||
this.finger7 = new Finger(this._game);
|
||||
this.finger8 = new Finger(this._game);
|
||||
this.finger9 = new Finger(this._game);
|
||||
this.finger10 = new Finger(this._game);
|
||||
|
||||
this._fingers = [this.finger1, this.finger2, this.finger3, this.finger4, this.finger5, this.finger6, this.finger7, this.finger8, this.finger9, this.finger10];
|
||||
|
||||
this.touchDown = new Signal();
|
||||
this.touchUp = new Signal();
|
||||
|
||||
this.start();
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
|
@ -59,120 +35,10 @@ module Phaser {
|
|||
private _game: Game;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property x
|
||||
* @type {Number}
|
||||
**/
|
||||
public x: number = 0;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property y
|
||||
* @type {Number}
|
||||
**/
|
||||
public y: number = 0;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property _fingers
|
||||
* @type {Array}
|
||||
* @private
|
||||
**/
|
||||
private _fingers: Finger[];
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger1
|
||||
* @type {Finger}
|
||||
**/
|
||||
public finger1: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger2
|
||||
* @type {Finger}
|
||||
**/
|
||||
public finger2: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger3
|
||||
* @type {Finger}
|
||||
**/
|
||||
public finger3: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger4
|
||||
* @type {Finger}
|
||||
**/
|
||||
public finger4: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger5
|
||||
* @type {Finger}
|
||||
**/
|
||||
public finger5: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger6
|
||||
* @type {Finger}
|
||||
**/
|
||||
public finger6: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger7
|
||||
* @type {Finger}
|
||||
**/
|
||||
public finger7: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger8
|
||||
* @type {Finger}
|
||||
**/
|
||||
public finger8: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger9
|
||||
* @type {Finger}
|
||||
**/
|
||||
public finger9: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property finger10
|
||||
* @type {Finger}
|
||||
**/
|
||||
public finger10: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property latestFinger
|
||||
* @type {Finger}
|
||||
**/
|
||||
public latestFinger: Finger;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property isDown
|
||||
* You can disable all Input by setting disabled = true. While set all new input related events will be ignored.
|
||||
* @type {Boolean}
|
||||
**/
|
||||
public isDown: bool = false;
|
||||
|
||||
/**
|
||||
*
|
||||
* @property isUp
|
||||
* @type {Boolean}
|
||||
**/
|
||||
public isUp: bool = true;
|
||||
|
||||
public touchDown: Signal;
|
||||
public touchUp: Signal;
|
||||
*/
|
||||
public disabled: bool = false;
|
||||
|
||||
/**
|
||||
*
|
||||
|
@ -209,91 +75,71 @@ module Phaser {
|
|||
**/
|
||||
private onTouchStart(event) {
|
||||
|
||||
event.preventDefault();
|
||||
if (this._game.input.disabled || this.disabled)
|
||||
{
|
||||
return;
|
||||
}
|
||||
|
||||
// A list of all the touch points that BECAME active with the current event
|
||||
// https://developer.mozilla.org/en-US/docs/DOM/TouchList
|
||||
event.preventDefault();
|
||||
|
||||
// event.targetTouches = list of all touches on the TARGET ELEMENT (i.e. game dom element)
|
||||
// event.touches = list of all touches on the ENTIRE DOCUMENT, not just the target element
|
||||
// event.changedTouches = the touches that CHANGED in this event, not the total number of them
|
||||
for (var i = 0; i < event.changedTouches.length; i++)
|
||||
{
|
||||
for (var f = 0; f < this._fingers.length; f++)
|
||||
{
|
||||
if (this._fingers[f].active === false)
|
||||
{
|
||||
this._fingers[f].start(event.changedTouches[i]);
|
||||
this.x = this._fingers[f].x;
|
||||
this.y = this._fingers[f].y;
|
||||
this._game.input.x = this.x * this._game.input.scaleX;
|
||||
this._game.input.y = this.y * this._game.input.scaleY;
|
||||
this.touchDown.dispatch(this._fingers[f].x, this._fingers[f].y, this._fingers[f].timeDown, this._fingers[f].timeUp, this._fingers[f].duration);
|
||||
this._game.input.onDown.dispatch(this._game.input.x, this._game.input.y, this._fingers[f].timeDown);
|
||||
this.isDown = true;
|
||||
this.isUp = false;
|
||||
break;
|
||||
}
|
||||
}
|
||||
this._game.input.startPointer(event.changedTouches[i]);
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* Doesn't appear to be supported by most browsers yet
|
||||
* Touch cancel - touches that were disrupted (perhaps by moving into a plugin or browser chrome)
|
||||
* Occurs for example on iOS when you put down 4 fingers and the app selector UI appears
|
||||
* @method onTouchCancel
|
||||
* @param {Any} event
|
||||
**/
|
||||
private onTouchCancel(event) {
|
||||
|
||||
if (this._game.input.disabled || this.disabled)
|
||||
{
|
||||
return;
|
||||
}
|
||||
|
||||
event.preventDefault();
|
||||
|
||||
// Touch cancel - touches that were disrupted (perhaps by moving into a plugin or browser chrome)
|
||||
// http://www.w3.org/TR/touch-events/#dfn-touchcancel
|
||||
// event.changedTouches = the touches that CHANGED in this event, not the total number of them
|
||||
for (var i = 0; i < event.changedTouches.length; i++)
|
||||
{
|
||||
for (var f = 0; f < this._fingers.length; f++)
|
||||
{
|
||||
if (this._fingers[f].identifier === event.changedTouches[i].identifier)
|
||||
{
|
||||
this._fingers[f].stop(event.changedTouches[i]);
|
||||
break;
|
||||
}
|
||||
}
|
||||
this._game.input.stopPointer(event.changedTouches[i]);
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* For touch enter and leave its a list of the touch points that have entered or left the target
|
||||
* Doesn't appear to be supported by most browsers yet
|
||||
* @method onTouchEnter
|
||||
* @param {Any} event
|
||||
**/
|
||||
private onTouchEnter(event) {
|
||||
|
||||
if (this._game.input.disabled || this.disabled)
|
||||
{
|
||||
return;
|
||||
}
|
||||
|
||||
event.preventDefault();
|
||||
|
||||
// For touch enter and leave its a list of the touch points that have entered or left the target
|
||||
|
||||
// event.targetTouches = list of all touches on the TARGET ELEMENT (i.e. game dom element)
|
||||
// event.touches = list of all touches on the ENTIRE DOCUMENT, not just the target element
|
||||
// event.changedTouches = the touches that CHANGED in this event, not the total number of them
|
||||
for (var i = 0; i < event.changedTouches.length; i++)
|
||||
{
|
||||
for (var f = 0; f < this._fingers.length; f++)
|
||||
{
|
||||
if (this._fingers[f].active === false)
|
||||
{
|
||||
this._fingers[f].start(event.changedTouches[i]);
|
||||
break;
|
||||
}
|
||||
}
|
||||
console.log('touch enter');
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
* For touch enter and leave its a list of the touch points that have entered or left the target
|
||||
* Doesn't appear to be supported by most browsers yet
|
||||
* @method onTouchLeave
|
||||
* @param {Any} event
|
||||
|
@ -302,19 +148,9 @@ module Phaser {
|
|||
|
||||
event.preventDefault();
|
||||
|
||||
// For touch enter and leave its a list of the touch points that have entered or left the target
|
||||
|
||||
// event.changedTouches = the touches that CHANGED in this event, not the total number of them
|
||||
for (var i = 0; i < event.changedTouches.length; i++)
|
||||
{
|
||||
for (var f = 0; f < this._fingers.length; f++)
|
||||
{
|
||||
if (this._fingers[f].identifier === event.changedTouches[i].identifier)
|
||||
{
|
||||
this._fingers[f].leave(event.changedTouches[i]);
|
||||
break;
|
||||
}
|
||||
}
|
||||
console.log('touch leave');
|
||||
}
|
||||
|
||||
}
|
||||
|
@ -328,23 +164,9 @@ module Phaser {
|
|||
|
||||
event.preventDefault();
|
||||
|
||||
// event.targetTouches = list of all touches on the TARGET ELEMENT (i.e. game dom element)
|
||||
// event.touches = list of all touches on the ENTIRE DOCUMENT, not just the target element
|
||||
// event.changedTouches = the touches that CHANGED in this event, not the total number of them
|
||||
for (var i = 0; i < event.changedTouches.length; i++)
|
||||
{
|
||||
for (var f = 0; f < this._fingers.length; f++)
|
||||
{
|
||||
if (this._fingers[f].identifier === event.changedTouches[i].identifier)
|
||||
{
|
||||
this._fingers[f].move(event.changedTouches[i]);
|
||||
this.x = this._fingers[f].x;
|
||||
this.y = this._fingers[f].y;
|
||||
this._game.input.x = this.x * this._game.input.scaleX;
|
||||
this._game.input.y = this.y * this._game.input.scaleY;
|
||||
break;
|
||||
}
|
||||
}
|
||||
this._game.input.updatePointer(event.changedTouches[i]);
|
||||
}
|
||||
|
||||
}
|
||||
|
@ -364,60 +186,9 @@ module Phaser {
|
|||
// event.changedTouches = the touches that CHANGED in this event, not the total number of them
|
||||
for (var i = 0; i < event.changedTouches.length; i++)
|
||||
{
|
||||
for (var f = 0; f < this._fingers.length; f++)
|
||||
{
|
||||
if (this._fingers[f].identifier === event.changedTouches[i].identifier)
|
||||
{
|
||||
this._fingers[f].stop(event.changedTouches[i]);
|
||||
this.x = this._fingers[f].x;
|
||||
this.y = this._fingers[f].y;
|
||||
this._game.input.x = this.x * this._game.input.scaleX;
|
||||
this._game.input.y = this.y * this._game.input.scaleY;
|
||||
this.touchUp.dispatch(this._fingers[f].x, this._fingers[f].y, this._fingers[f].timeDown, this._fingers[f].timeUp, this._fingers[f].duration);
|
||||
this._game.input.onUp.dispatch(this._game.input.x, this._game.input.y, this._fingers[f].timeUp);
|
||||
this.isDown = false;
|
||||
this.isUp = true;
|
||||
break;
|
||||
}
|
||||
}
|
||||
this._game.input.stopPointer(event.changedTouches[i]);
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method calculateDistance
|
||||
* @param {Finger} finger1
|
||||
* @param {Finger} finger2
|
||||
**/
|
||||
public calculateDistance(finger1: Finger, finger2: Finger) {
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method calculateAngle
|
||||
* @param {Finger} finger1
|
||||
* @param {Finger} finger2
|
||||
**/
|
||||
public calculateAngle(finger1: Finger, finger2: Finger) {
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method checkOverlap
|
||||
* @param {Finger} finger1
|
||||
* @param {Finger} finger2
|
||||
**/
|
||||
public checkOverlap(finger1: Finger, finger2: Finger) {
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method update
|
||||
*/
|
||||
public update() {
|
||||
|
||||
|
||||
}
|
||||
|
||||
/**
|
||||
|
@ -435,17 +206,6 @@ module Phaser {
|
|||
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* @method reset
|
||||
**/
|
||||
public reset() {
|
||||
|
||||
this.isDown = false;
|
||||
this.isUp = false;
|
||||
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
}
|
36
README.md
36
README.md
|
@ -15,16 +15,18 @@ Try out the [Phaser Test Suite](http://gametest.mobi/phaser/)
|
|||
|
||||
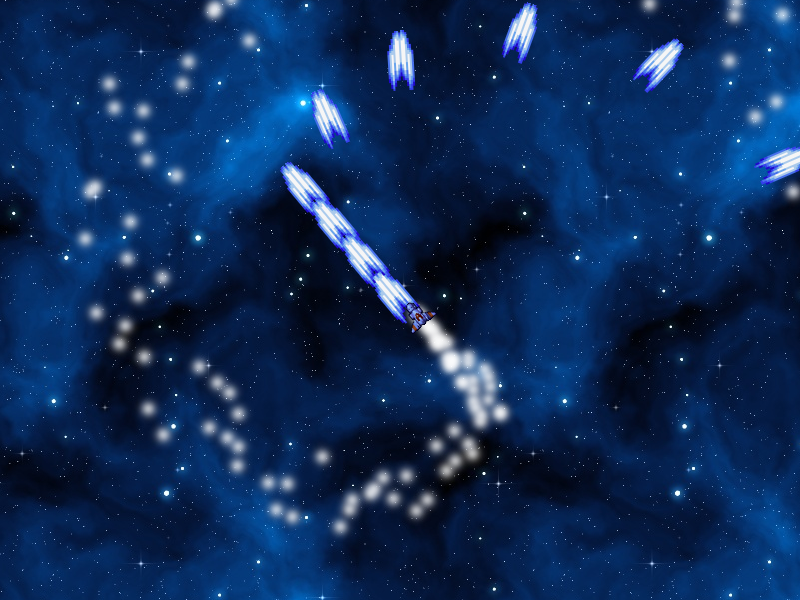
|
||||
|
||||
"Being negative is not how we make progress" - Larry Page, Google
|
||||
|
||||
Latest Update
|
||||
-------------
|
||||
|
||||
V0.9.6
|
||||
|
||||
* Documentation! Every class now has documentation for nearly every single function - if you spot a typo, please shout! (thanks pixelpicosean)
|
||||
* Virtually every class now has documentation - if you spot a typo or something missing please shout (thanks pixelpicosean)
|
||||
* Grunt file updated to produce the new Special FX JS file (thanks HackManiac)
|
||||
* Fixed issue stopping Phaser working on iOS 5 (i.e. iPad 1)
|
||||
* Fixed issue stopping Phaser working on iOS 5 (iPad 1)
|
||||
* Created new mobile test folder, updated index.php to use mobile CSS and made some mobile specific tests
|
||||
* Fixed a few speed issues on Android 2.x stock browser, but it's still tricky to get a fast game out of it
|
||||
* Fixed a few speed issues on Android 2.x stock browser
|
||||
* Moved Camera context save/restore back inside parameter checks (sped-up Samsung S3 stock browser)
|
||||
* Fixed bug with StageScaleMode.checkOrientation not respecting the NO_SCALE value
|
||||
* Added MSPointer support (thanks Diego Bezerra)
|
||||
|
@ -38,10 +40,32 @@ V0.9.6
|
|||
* Updated RequestAnimationFrame setTimeout to use fixed timestep and re-ordered callback sequence. Android 2/iOS5 performance much better now
|
||||
* Removed Stage.ORIENTATION_LANDSCAPE statics because the values should be taken from Stage.scale.isPortrait / isLandscape
|
||||
* Removed Stage.maxScaleX/Y and moved them into StageScaleMode.minWidth, minHeight, maxWidth and maxHeight
|
||||
* Fixed Stage.scale so that it resizes without needing an orientation change
|
||||
* Fixed Stage.scale so that it resizes without needing an orientation change first
|
||||
* Added StageScaleMode.startFullscreen(), stopFullScreen() and isFullScreen for making use of the FullScreen API on desktop browsers
|
||||
* Swapped Stage.offset from Point to MicroPoint
|
||||
* Swapped Stage.bounds from Rectangle to Quad
|
||||
* Added State.destroy support. A states destroy function is called when you switch to a new state (thanks JesseFreeman)
|
||||
* Added Sprite.fillColor, used in the Sprite render if no image is loaded (set via the property or Sprite.makeGraphic) (thanks JesseFreeman)
|
||||
* Renamed Phaser.Finger to Phaser.Pointer
|
||||
* Updated all of the Input classes so they now use Input.pointers 1 through 10
|
||||
* Updated Touch and MSPointer to allow multi-touch support (when the hardware supports it) and created new tests to show this
|
||||
* Added Input.getPointer, Input.getPointerFromIdentifier, Input.totalActivePointers and Input.totalInactivePointers
|
||||
* Added Input.startPointer, Input.updatePointer and Input.stopPointer
|
||||
* Phaser Input now confirmed working on Windows Phone 8 (Nokia Lumia 920)
|
||||
* Added Input.maxPointers to allow you to limit the number of fingers your game will listen for on multi-touch systems
|
||||
* Pointer.totalTouches value keeps a running total of the number of times the Pointer has been pressed
|
||||
* Added Pointer.pointA and pointB - pointA is placed on touch, pointB is moved on update, useful for tracking distance/direction/gestures
|
||||
* Added Game.state - now contains a reference to the current state object (if any was given)
|
||||
* Moved the Input start events from the constructors to a single Input.start method
|
||||
* Added Input.disabled boolean to globally turn off all input event processing.
|
||||
* Added Input.Mouse.disabled, Input.Touch.disabled, Input.MSPointer.disabled and Input.Keyboard.disabled
|
||||
* Added Device.mspointer boolean. true if MSPointer is available on the device.
|
||||
|
||||
|
||||
* TODO: Check that tween pausing works with the new performance.now
|
||||
* TODO: Game.Time should monitor pause duration
|
||||
* Added StageScaleMode.startFullscreen(), stopFullScreen() and isFullScreen for making use of the FullScreen API on desktop browsers
|
||||
* TODO: Investigate bug re: tilemap collision and animation frames
|
||||
* TODO: Update tests that use arrow keys and include touch/mouse support
|
||||
|
||||
|
||||
Requirements
|
||||
|
@ -140,9 +164,9 @@ Work in Progress
|
|||
We've a number of features that we know Phaser is lacking, here is our current priority list:
|
||||
|
||||
* Better sound controls
|
||||
* MSPointer support
|
||||
* Text Rendering
|
||||
* Buttons
|
||||
* Google Play Game Services
|
||||
|
||||
Beyond this there are lots of other things we plan to add such as WebGL support, Spine animation format support, sloped collision tiles, path finding and support for custom plugins. But the list above are priority items, and by no means exhaustive either! However we do feel that the core structure of Phaser is now tightly locked down, so safe to use for small scale production games.
|
||||
|
||||
|
|
|
@ -119,6 +119,10 @@
|
|||
<Content Include="input\multitouch.js">
|
||||
<DependentUpon>multitouch.ts</DependentUpon>
|
||||
</Content>
|
||||
<TypeScriptCompile Include="input\single touch.ts" />
|
||||
<Content Include="input\single touch.js">
|
||||
<DependentUpon>single touch.ts</DependentUpon>
|
||||
</Content>
|
||||
<Content Include="misc\bootscreen.js">
|
||||
<DependentUpon>bootscreen.ts</DependentUpon>
|
||||
</Content>
|
||||
|
|
|
@ -1,7 +1,7 @@
|
|||
/// <reference path="../../Phaser/Game.ts" />
|
||||
(function () {
|
||||
// Here we create a quite tiny game (320x240 in size)
|
||||
var myGame = new Phaser.Game(this, 'game', 320, 240, init, create, update);
|
||||
var myGame = new Phaser.Game(this, 'game', 320, 240, init, create, update, render);
|
||||
function init() {
|
||||
// This sets a limit on the up-scale
|
||||
myGame.stage.scale.maxWidth = 640;
|
||||
|
@ -16,7 +16,6 @@
|
|||
for(var i = 0; i < 1000; i++) {
|
||||
myGame.createSprite(myGame.world.randomX, myGame.world.randomY, 'melon');
|
||||
}
|
||||
myGame.onRenderCallback = render;
|
||||
}
|
||||
function update() {
|
||||
if(myGame.input.keyboard.isDown(Phaser.Keyboard.LEFT)) {
|
||||
|
|
|
@ -3,7 +3,7 @@
|
|||
(function () {
|
||||
|
||||
// Here we create a quite tiny game (320x240 in size)
|
||||
var myGame = new Phaser.Game(this, 'game', 320, 240, init, create, update);
|
||||
var myGame = new Phaser.Game(this, 'game', 320, 240, init, create, update, render);
|
||||
|
||||
function init() {
|
||||
|
||||
|
@ -29,8 +29,6 @@
|
|||
myGame.createSprite(myGame.world.randomX, myGame.world.randomY, 'melon');
|
||||
}
|
||||
|
||||
myGame.onRenderCallback = render;
|
||||
|
||||
}
|
||||
|
||||
function update() {
|
||||
|
|
|
@ -1,16 +1,23 @@
|
|||
/// <reference path="../../Phaser/Game.ts" />
|
||||
(function () {
|
||||
// Here we create a quite tiny game (320x240 in size)
|
||||
var myGame = new Phaser.Game(this, 'game', 800, 600, init, create, update);
|
||||
var myGame = new Phaser.Game(this, 'game', 800, 600, init, create, update, render);
|
||||
function init() {
|
||||
// Tell Phaser that we want it to scale up to whatever the browser can handle, but to do it proportionally
|
||||
myGame.stage.scaleMode = Phaser.StageScaleMode.SHOW_ALL;
|
||||
myGame.loader.addImageFile('dragonsun', 'assets/pics/cougar_dragonsun.png');
|
||||
myGame.loader.load();
|
||||
}
|
||||
function create() {
|
||||
myGame.onRenderCallback = render;
|
||||
console.log('dragons 8');
|
||||
myGame.input.onDown.add(test1, this);
|
||||
}
|
||||
function test1() {
|
||||
console.log('down');
|
||||
}
|
||||
function update() {
|
||||
}
|
||||
function render() {
|
||||
myGame.input.pointer1.renderDebug();
|
||||
myGame.input.pointer2.renderDebug();
|
||||
myGame.input.pointer3.renderDebug();
|
||||
myGame.input.pointer4.renderDebug();
|
||||
}
|
||||
})();
|
||||
|
|
|
@ -2,29 +2,38 @@
|
|||
|
||||
(function () {
|
||||
|
||||
// Here we create a quite tiny game (320x240 in size)
|
||||
var myGame = new Phaser.Game(this, 'game', 800, 600, init, create, update);
|
||||
var myGame = new Phaser.Game(this, 'game', 800, 600, init, create, update, render);
|
||||
|
||||
function init() {
|
||||
|
||||
// Tell Phaser that we want it to scale up to whatever the browser can handle, but to do it proportionally
|
||||
myGame.stage.scaleMode = Phaser.StageScaleMode.SHOW_ALL;
|
||||
myGame.loader.addImageFile('dragonsun', 'assets/pics/cougar_dragonsun.png');
|
||||
|
||||
myGame.loader.load();
|
||||
|
||||
}
|
||||
|
||||
function create() {
|
||||
|
||||
myGame.onRenderCallback = render;
|
||||
console.log('dragons 8');
|
||||
|
||||
myGame.input.onDown.add(test1, this);
|
||||
|
||||
}
|
||||
|
||||
function update() {
|
||||
function test1() {
|
||||
console.log('down');
|
||||
}
|
||||
|
||||
function update() {
|
||||
|
||||
}
|
||||
|
||||
function render() {
|
||||
|
||||
myGame.input.pointer1.renderDebug();
|
||||
myGame.input.pointer2.renderDebug();
|
||||
myGame.input.pointer3.renderDebug();
|
||||
myGame.input.pointer4.renderDebug();
|
||||
|
||||
}
|
||||
|
||||
|
|
19
Tests/input/single touch.js
Normal file
19
Tests/input/single touch.js
Normal file
|
@ -0,0 +1,19 @@
|
|||
/// <reference path="../../Phaser/Game.ts" />
|
||||
(function () {
|
||||
var myGame = new Phaser.Game(this, 'game', 800, 600, init, create, update, render);
|
||||
function init() {
|
||||
}
|
||||
function create() {
|
||||
// We lock the game to allowing only 1 Pointer active
|
||||
// This means on multi-touch systems it will ignore any extra fingers placed down beyond the first
|
||||
myGame.input.maxPointers = 1;
|
||||
}
|
||||
function update() {
|
||||
}
|
||||
function render() {
|
||||
myGame.input.renderDebugInfo(16, 16);
|
||||
myGame.input.pointer1.renderDebug(true);
|
||||
myGame.input.pointer2.renderDebug(true);
|
||||
myGame.input.pointer3.renderDebug(true);
|
||||
}
|
||||
})();
|
31
Tests/input/single touch.ts
Normal file
31
Tests/input/single touch.ts
Normal file
|
@ -0,0 +1,31 @@
|
|||
/// <reference path="../../Phaser/Game.ts" />
|
||||
|
||||
(function () {
|
||||
|
||||
var myGame = new Phaser.Game(this, 'game', 800, 600, init, create, update, render);
|
||||
|
||||
function init() {
|
||||
}
|
||||
|
||||
function create() {
|
||||
|
||||
// We lock the game to allowing only 1 Pointer active
|
||||
// This means on multi-touch systems it will ignore any extra fingers placed down beyond the first
|
||||
myGame.input.maxPointers = 1;
|
||||
|
||||
}
|
||||
|
||||
function update() {
|
||||
}
|
||||
|
||||
function render() {
|
||||
|
||||
myGame.input.renderDebugInfo(16, 16);
|
||||
|
||||
myGame.input.pointer1.renderDebug(true);
|
||||
myGame.input.pointer2.renderDebug(true);
|
||||
myGame.input.pointer3.renderDebug(true);
|
||||
|
||||
}
|
||||
|
||||
})();
|
|
@ -1,6 +1,6 @@
|
|||
/// <reference path="../../Phaser/Game.ts" />
|
||||
(function () {
|
||||
var myGame = new Phaser.Game(this, 'game', 320, 460, init, create, update);
|
||||
var myGame = new Phaser.Game(this, 'game', 320, 460, init, create, update, render);
|
||||
function init() {
|
||||
myGame.loader.addImageFile('bunny', 'assets/sprites/wabbit.png');
|
||||
myGame.loader.load();
|
||||
|
@ -15,12 +15,11 @@
|
|||
minY = 0;
|
||||
maxX = myGame.stage.width - 26;
|
||||
maxY = myGame.stage.height - 37;
|
||||
myGame.input.touch.touchDown.add(addBunnies, this);
|
||||
myGame.input.onDown.add(addBunnies, this);
|
||||
// This will really help on slow Android phones
|
||||
myGame.framerate = 30;
|
||||
// Make sure the camera doesn't clip anything
|
||||
myGame.camera.disableClipping = true;
|
||||
myGame.onRenderCallback = render;
|
||||
myGame.stage.context.fillStyle = 'rgb(255,0,0)';
|
||||
myGame.stage.context.font = '20px Arial';
|
||||
addBunnies();
|
||||
|
|
|
@ -2,7 +2,7 @@
|
|||
|
||||
(function () {
|
||||
|
||||
var myGame = new Phaser.Game(this, 'game', 320, 460, init, create, update);
|
||||
var myGame = new Phaser.Game(this, 'game', 320, 460, init, create, update, render);
|
||||
|
||||
function init() {
|
||||
|
||||
|
@ -26,14 +26,13 @@
|
|||
maxX = myGame.stage.width - 26;
|
||||
maxY = myGame.stage.height - 37;
|
||||
|
||||
myGame.input.touch.touchDown.add(addBunnies, this);
|
||||
myGame.input.onDown.add(addBunnies, this);
|
||||
|
||||
// This will really help on slow Android phones
|
||||
myGame.framerate = 30;
|
||||
// Make sure the camera doesn't clip anything
|
||||
myGame.camera.disableClipping = true;
|
||||
|
||||
myGame.onRenderCallback = render;
|
||||
myGame.stage.context.fillStyle = 'rgb(255,0,0)';
|
||||
myGame.stage.context.font = '20px Arial';
|
||||
|
||||
|
|
|
@ -14,7 +14,7 @@
|
|||
|
||||
* {
|
||||
user-select: none;
|
||||
-webkit-tap-highlight-color: rgb(0, 0, 0, 0);
|
||||
-webkit-tap-highlight-color: rgba(0, 0, 0, 0);
|
||||
-webkit-touch-callout: none;
|
||||
-webkit-user-select: none;
|
||||
-moz-user-select: none;
|
||||
|
|
1015
Tests/phaser.js
1015
Tests/phaser.js
File diff suppressed because it is too large
Load diff
|
@ -3,10 +3,12 @@
|
|||
var myGame = new Phaser.Game(this, 'game', 800, 600, init, create, update);
|
||||
function init() {
|
||||
myGame.loader.addTextureAtlas('bot', 'assets/sprites/running_bot.png', 'assets/sprites/running_bot.json');
|
||||
myGame.loader.addTextureAtlas('atlas', 'assets/pics/texturepacker_test.png', 'assets/pics/texturepacker_test.json');
|
||||
myGame.loader.load();
|
||||
}
|
||||
var bot;
|
||||
var bot2;
|
||||
var car;
|
||||
function create() {
|
||||
// This bot will flip properly when he reaches the edge
|
||||
bot = myGame.createSprite(myGame.stage.width, 300, 'bot');
|
||||
|
@ -18,6 +20,10 @@
|
|||
bot2.animations.add('run');
|
||||
bot2.animations.play('run', 10, true);
|
||||
bot2.velocity.x = -150;
|
||||
// Flip a static sprite (not an animation)
|
||||
car = myGame.createSprite(100, 400, 'atlas');
|
||||
car.frameName = 'supercars_parsec.png';
|
||||
car.flipped = true;
|
||||
}
|
||||
function update() {
|
||||
if(bot.x < -bot.width) {
|
||||
|
|
|
@ -7,12 +7,14 @@
|
|||
function init() {
|
||||
|
||||
myGame.loader.addTextureAtlas('bot', 'assets/sprites/running_bot.png', 'assets/sprites/running_bot.json');
|
||||
myGame.loader.addTextureAtlas('atlas', 'assets/pics/texturepacker_test.png', 'assets/pics/texturepacker_test.json');
|
||||
myGame.loader.load();
|
||||
|
||||
}
|
||||
|
||||
var bot: Phaser.Sprite;
|
||||
var bot2: Phaser.Sprite;
|
||||
var car: Phaser.Sprite;
|
||||
|
||||
function create() {
|
||||
|
||||
|
@ -28,6 +30,11 @@
|
|||
bot2.animations.play('run', 10, true);
|
||||
bot2.velocity.x = -150;
|
||||
|
||||
// Flip a static sprite (not an animation)
|
||||
car = myGame.createSprite(100, 400, 'atlas');
|
||||
car.frameName = 'supercars_parsec.png';
|
||||
car.flipped = true;
|
||||
|
||||
}
|
||||
|
||||
function update() {
|
||||
|
|
664
build/phaser.d.ts
vendored
664
build/phaser.d.ts
vendored
File diff suppressed because it is too large
Load diff
1015
build/phaser.js
1015
build/phaser.js
File diff suppressed because it is too large
Load diff
Loading…
Add table
Reference in a new issue