2023-06-13 23:18:36 +00:00
|
|
|
use crate::{
|
|
|
|
run_pager,
|
|
|
|
util::{create_lscolors, create_map, map_into_value},
|
|
|
|
PagerConfig, StyleConfig,
|
|
|
|
};
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
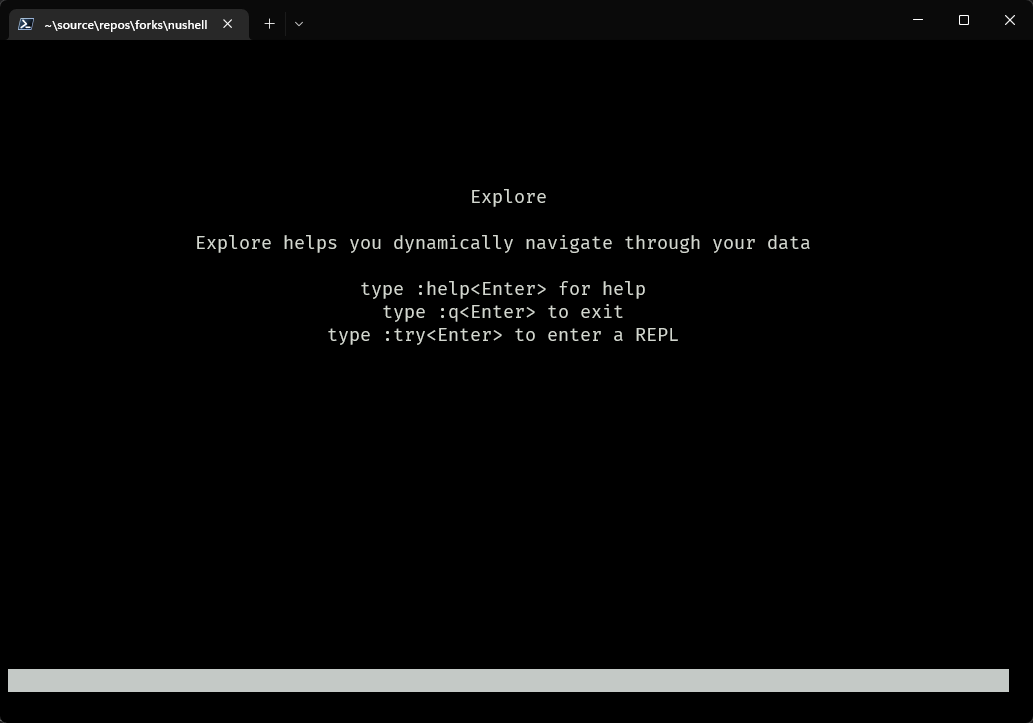
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
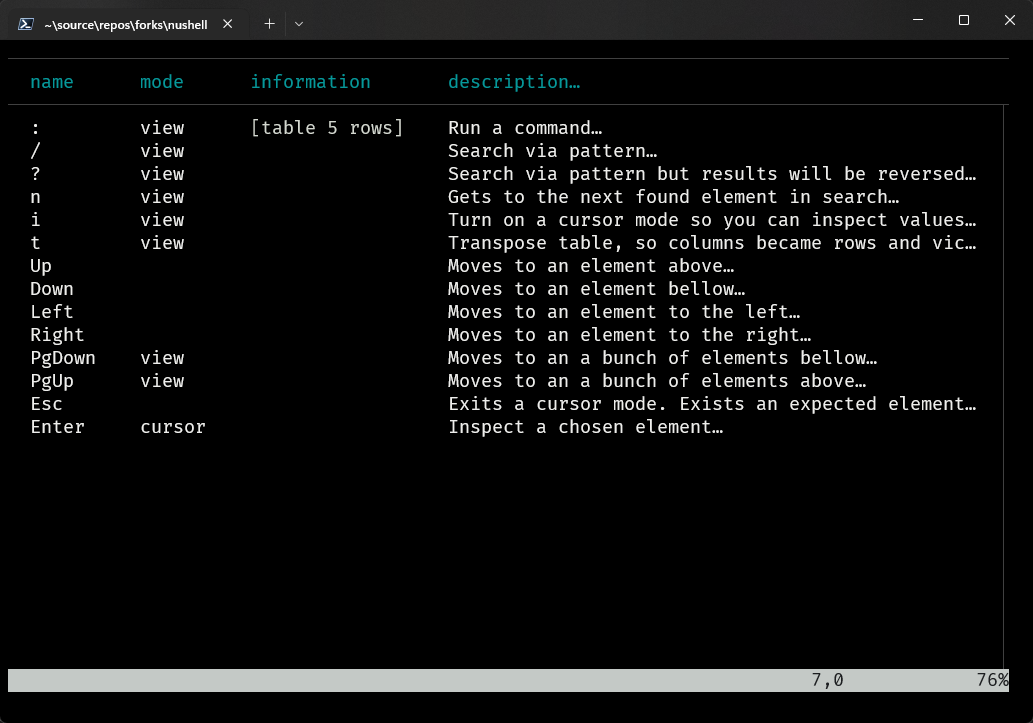
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
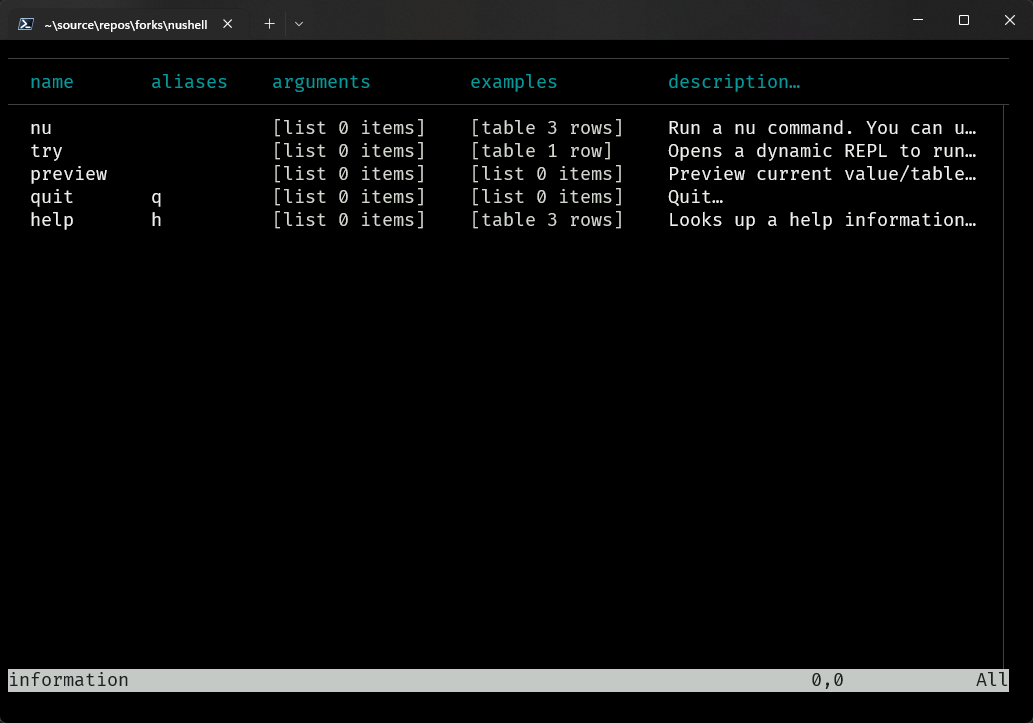
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
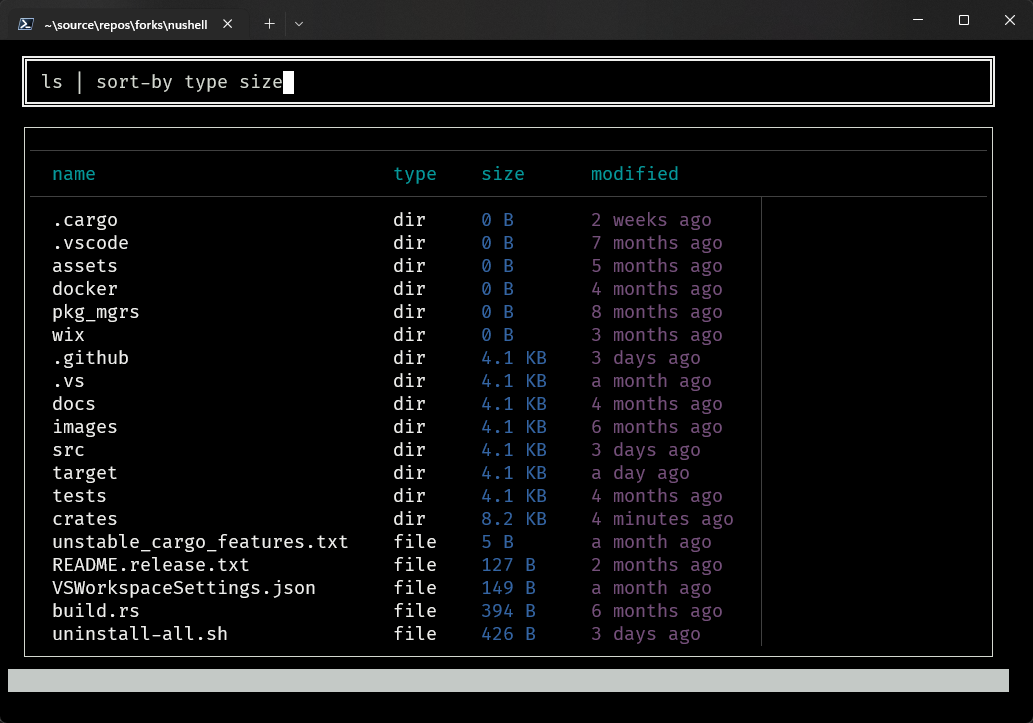
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
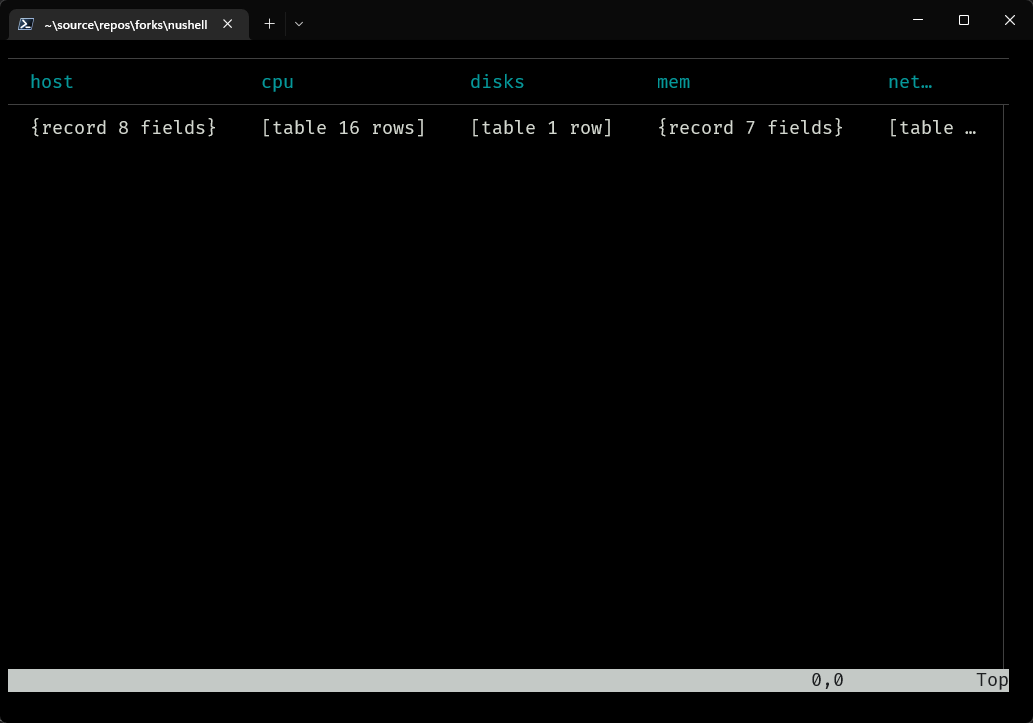
If you hit the `t` key it will now transpose the view to look like this.
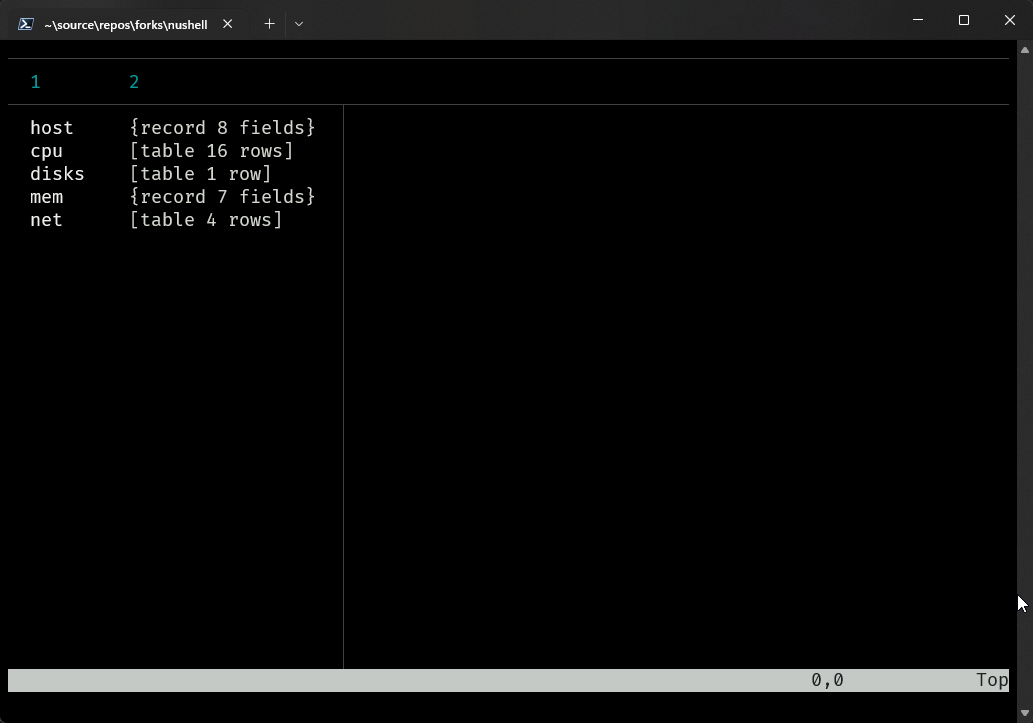
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
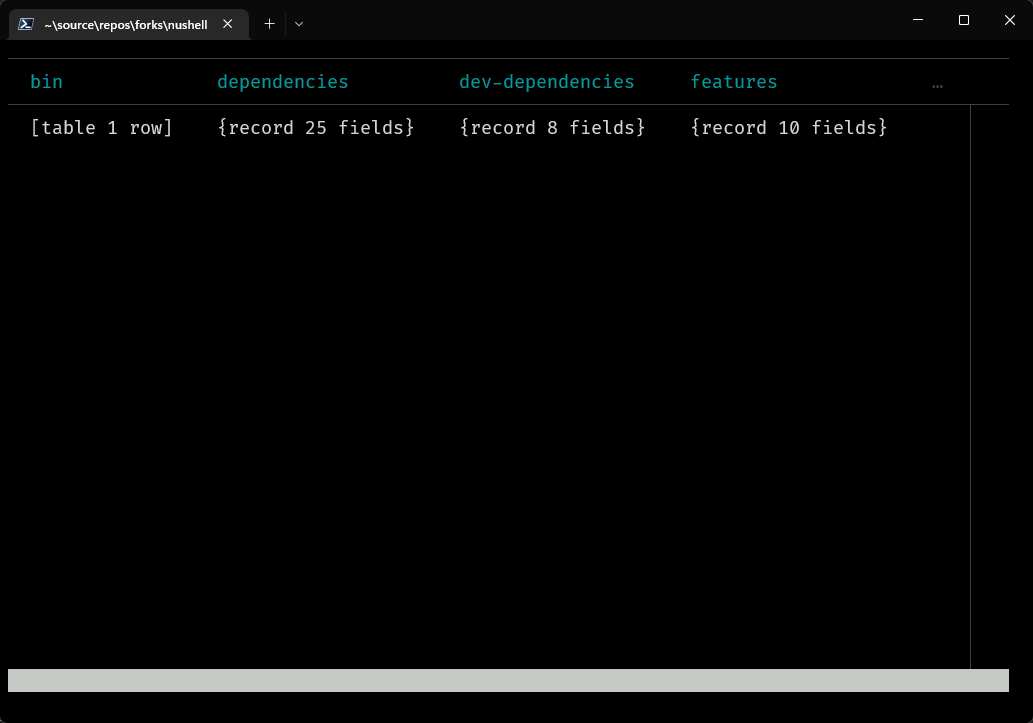
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
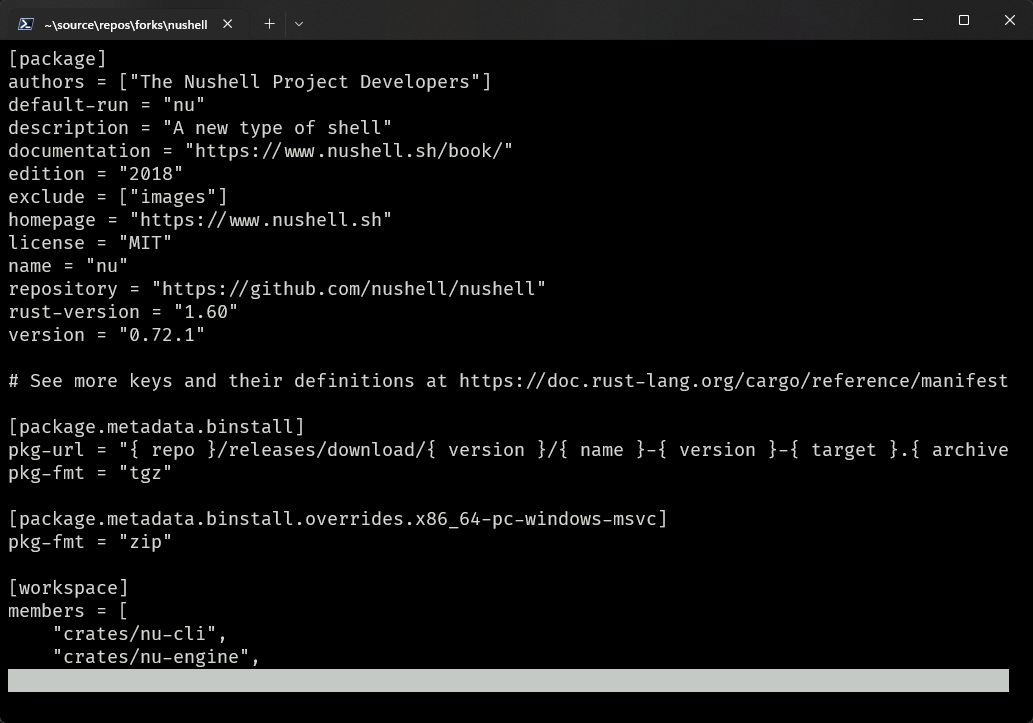
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
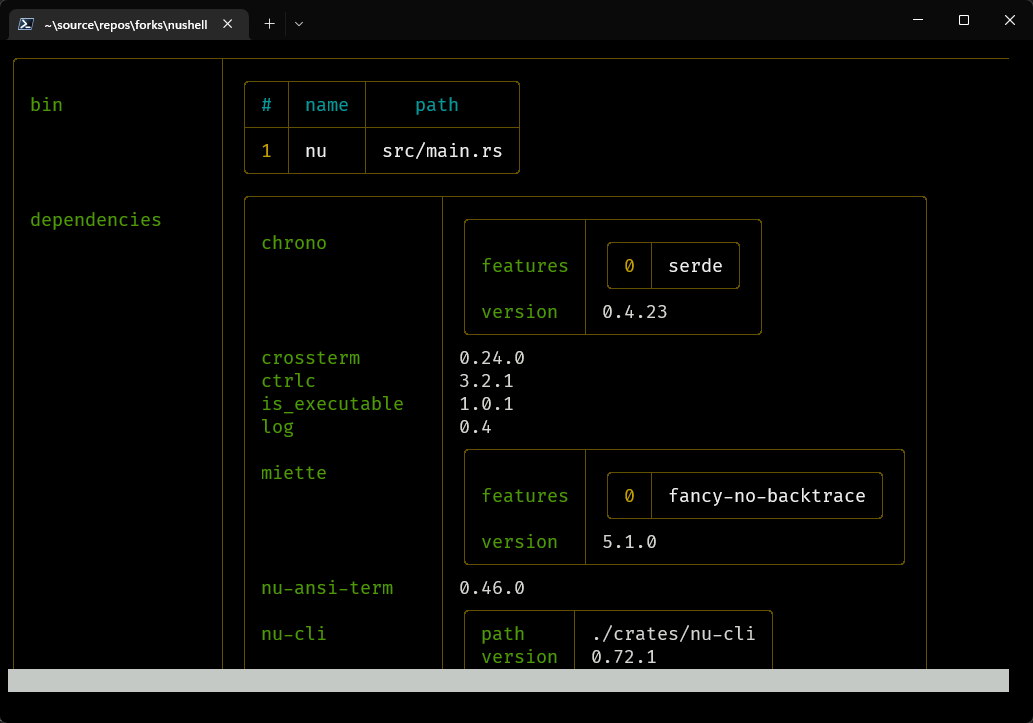
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
use nu_ansi_term::{Color, Style};
|
color_config now accepts closures as color values (#7141)
# Description
Closes #6909. You can now add closures to your `color_config` themes.
Whenever a value would be printed with `table`, the closure is run with
the value piped-in. The closure must return either a {fg,bg,attr} record
or a color name (`'light_red'` etc.). This returned style is used to
colour the value.
This is entirely backwards-compatible with existing config.nu files.
Example code excerpt:
```
let my_theme = {
header: green_bold
bool: { if $in { 'light_cyan' } else { 'light_red' } }
int: purple_bold
filesize: { |e| if $e == 0b { 'gray' } else if $e < 1mb { 'purple_bold' } else { 'cyan_bold' } }
duration: purple_bold
date: { (date now) - $in | if $in > 1wk { 'cyan_bold' } else if $in > 1day { 'green_bold' } else { 'yellow_bold' } }
range: yellow_bold
string: { if $in =~ '^#\w{6}$' { $in } else { 'white' } }
nothing: white
```
Example output with this in effect:
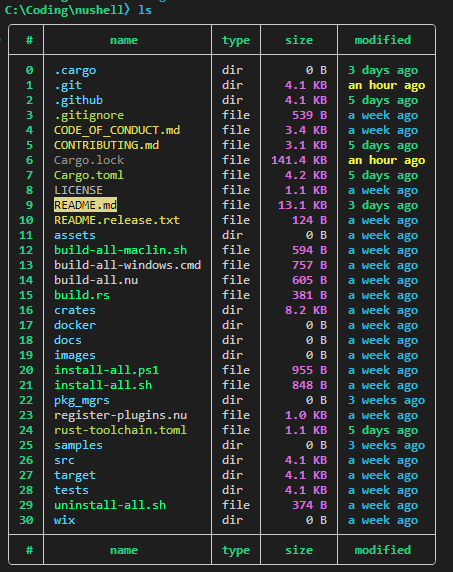
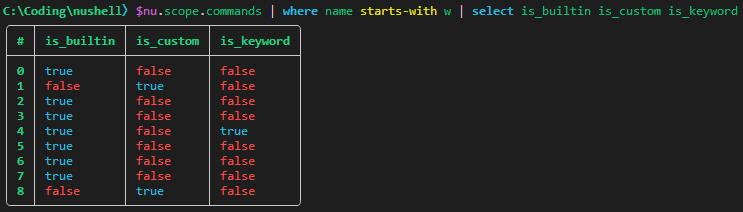
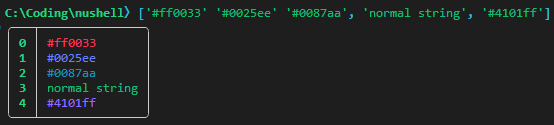
Slightly important notes:
* Some color_config names, namely "separator", "empty" and "hints", pipe
in `null` instead of a value.
* Currently, doing anything non-trivial inside a closure has an
understandably big perf hit. I currently do not actually recommend
something like `string: { if $in =~ '^#\w{6}$' { $in } else { 'white' }
}` for serious work, mainly because of the abundance of string-type data
in the world. Nevertheless, lesser-used types like "date" and "duration"
work well with this.
* I had to do some reorganisation in order to make it possible to call
`eval_block()` that late in table rendering. I invented a new struct
called "StyleComputer" which holds the engine_state and stack of the
initial `table` command (implicit or explicit).
* StyleComputer has a `compute()` method which takes a color_config name
and a nu value, and always returns the correct Style, so you don't have
to worry about A) the color_config value was set at all, B) whether it
was set to a closure or not, or C) which default style to use in those
cases.
* Currently, errors encountered during execution of the closures are
thrown in the garbage. Any other ideas are welcome. (Nonetheless, errors
result in a huge perf hit when they are encountered. I think what should
be done is to assume something terrible happened to the user's config
and invalidate the StyleComputer for that `table` run, thus causing
subsequent output to just be Style::default().)
* More thorough tests are forthcoming - ran into some difficulty using
`nu!` to take an alternative config, and for some reason `let-env config
=` statements don't seem to work inside `nu!` pipelines(???)
* The default config.nu has not been updated to make use of this yet. Do
tell if you think I should incorporate that into this.
# User-Facing Changes
See above.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace --features=extra -- -D warnings -D
clippy::unwrap_used -A clippy::needless_collect` to check that you're
using the standard code style
- `cargo test --workspace --features=extra` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2022-12-17 13:07:56 +00:00
|
|
|
use nu_color_config::{get_color_map, StyleComputer};
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
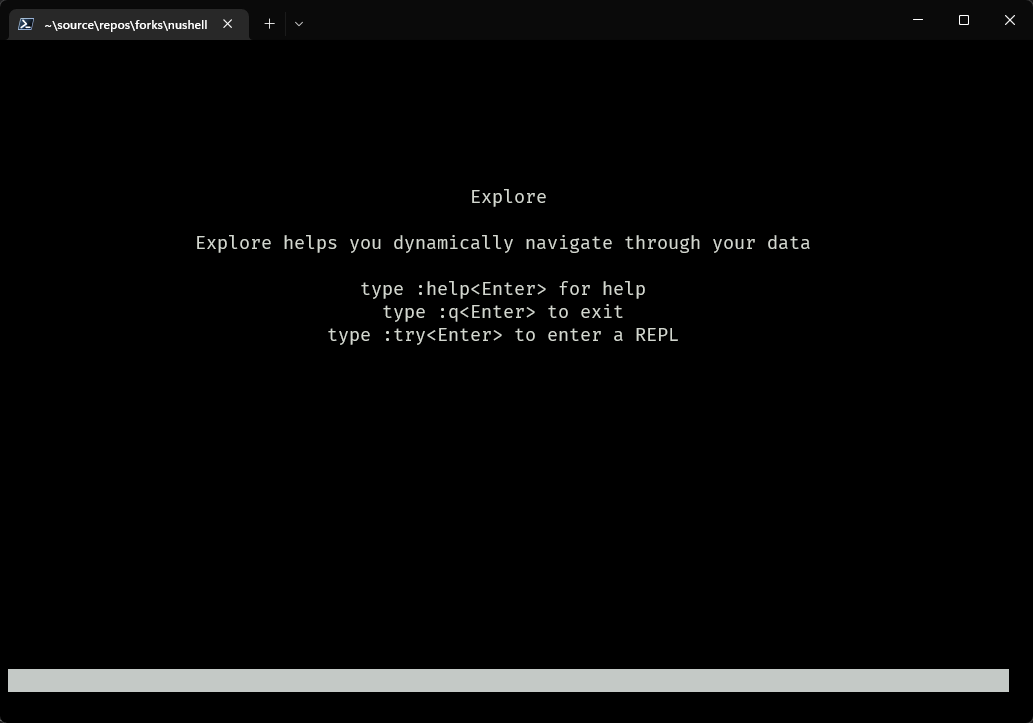
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
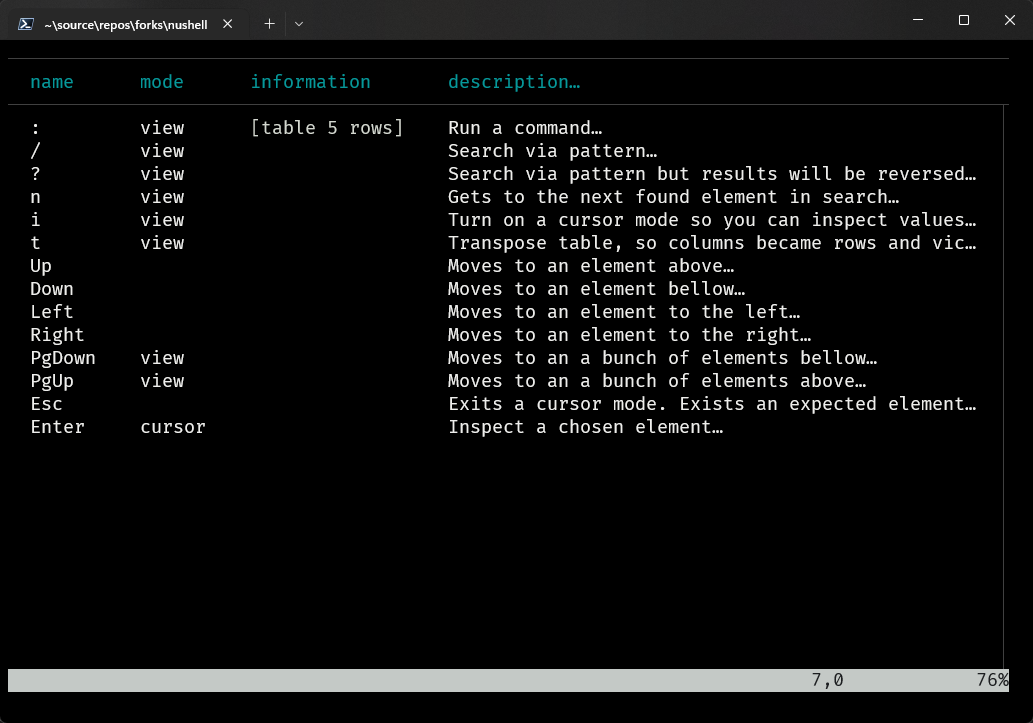
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
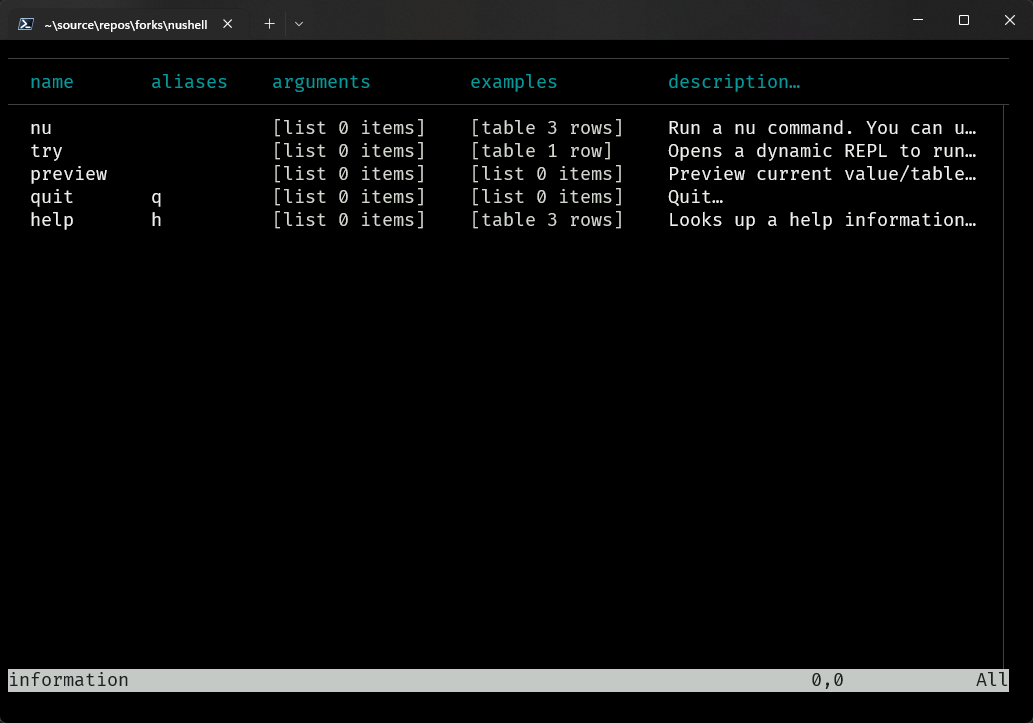
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
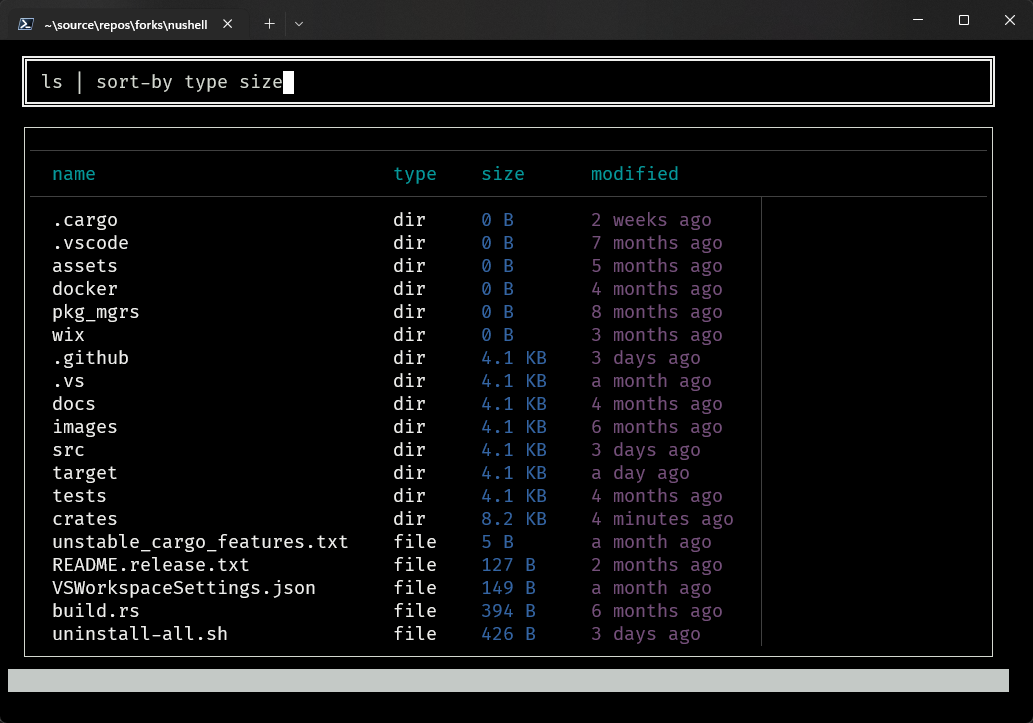
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
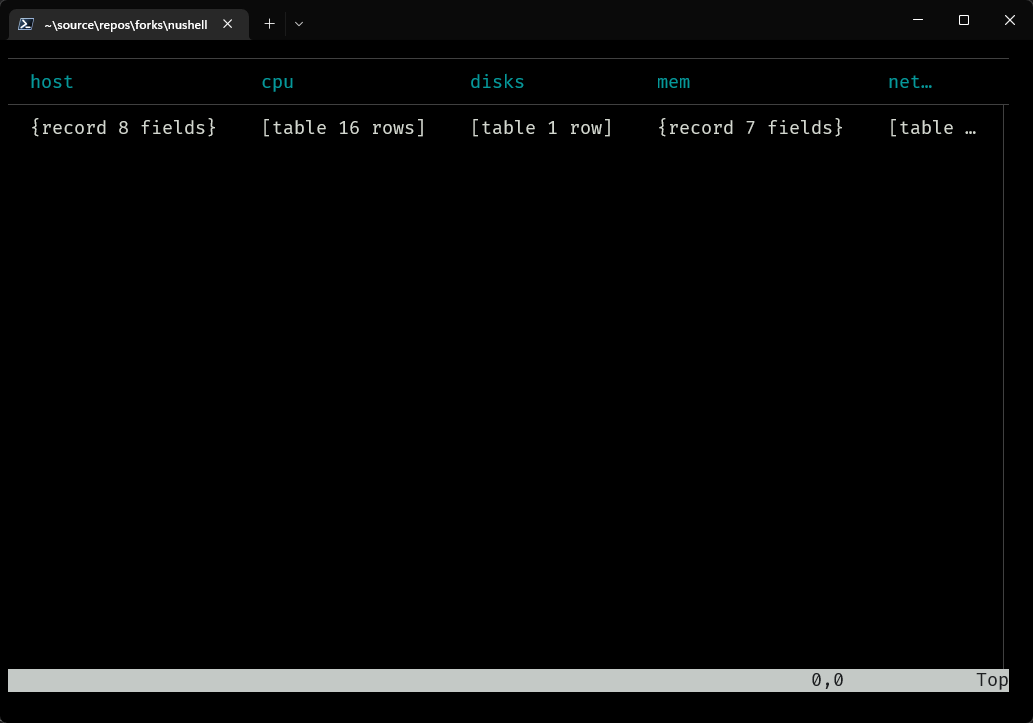
If you hit the `t` key it will now transpose the view to look like this.
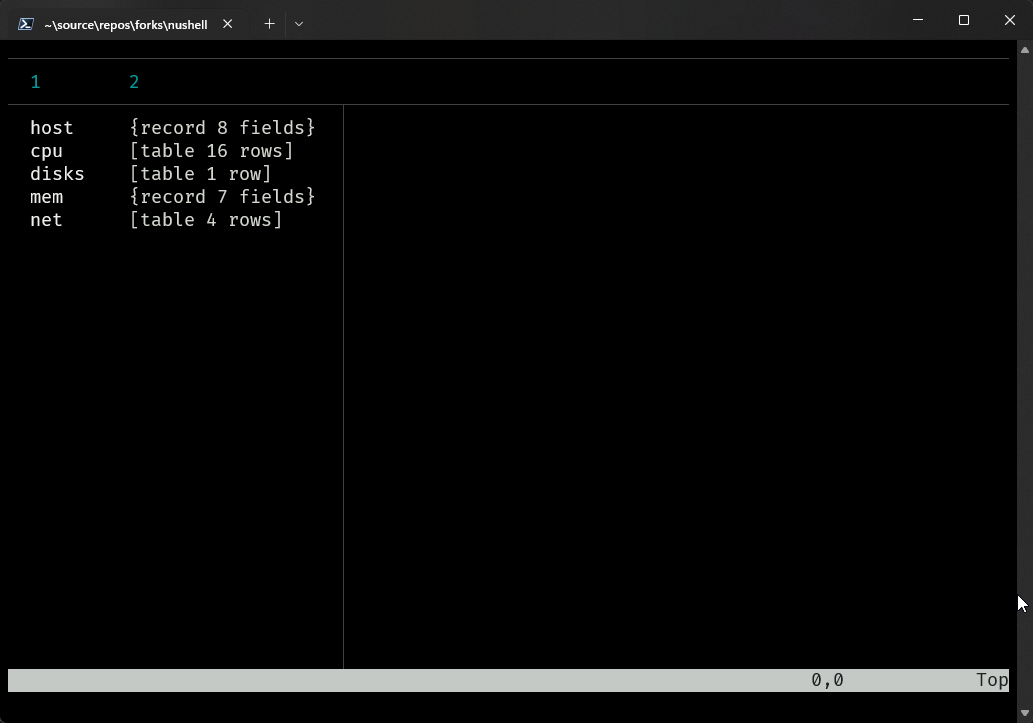
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
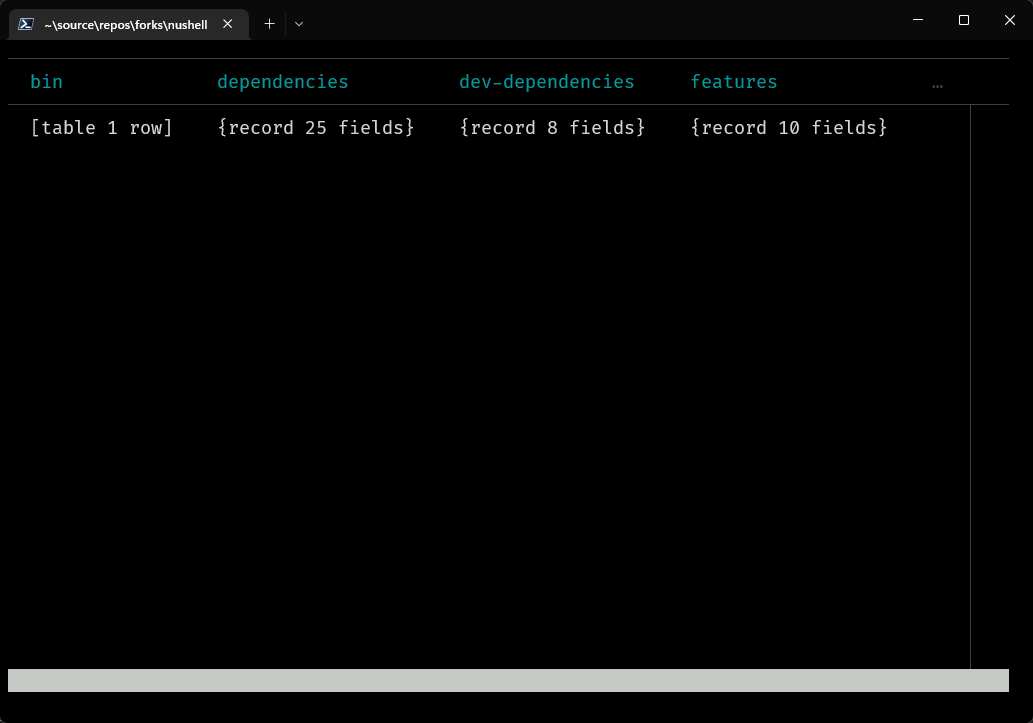
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
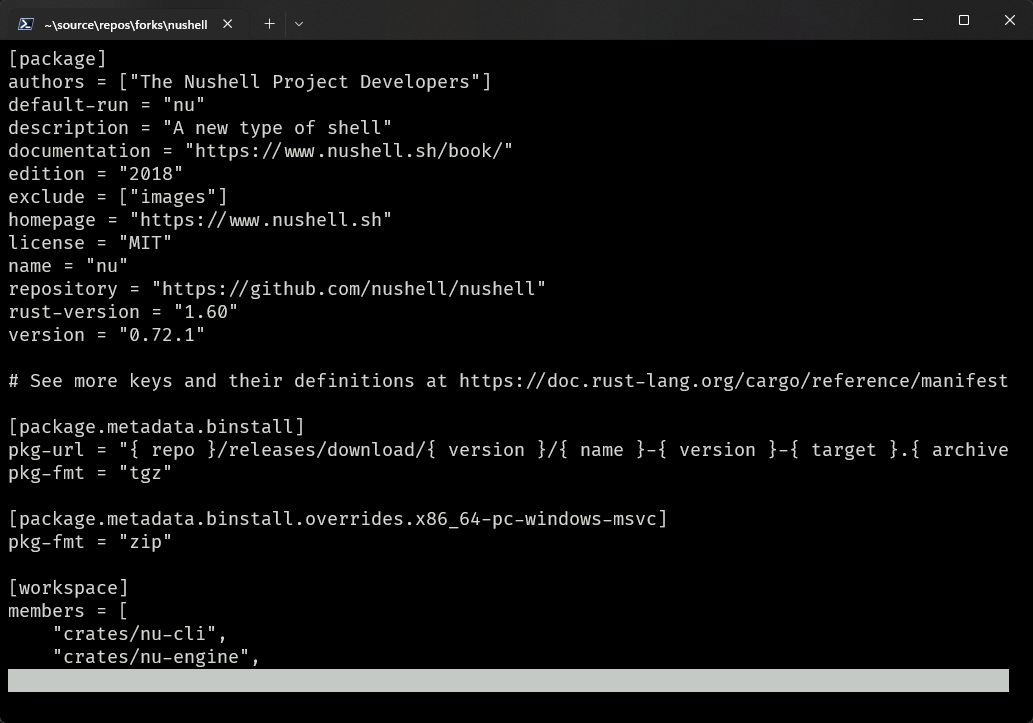
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
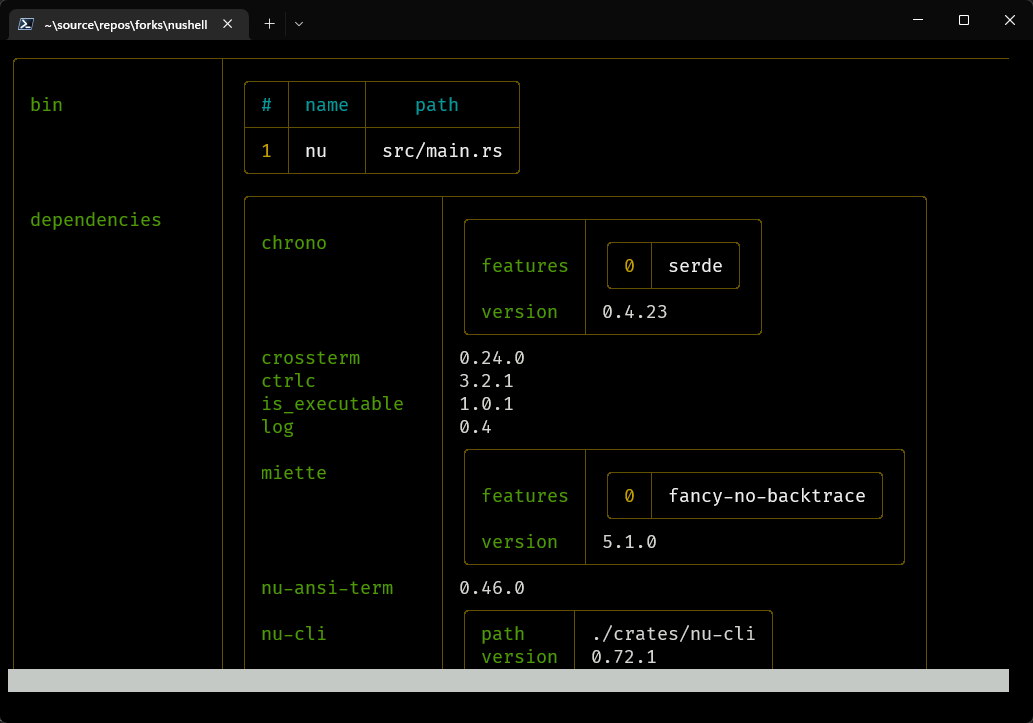
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
use nu_engine::CallExt;
|
|
|
|
use nu_protocol::{
|
|
|
|
ast::Call,
|
|
|
|
engine::{Command, EngineState, Stack},
|
2022-12-21 19:20:46 +00:00
|
|
|
Category, Example, PipelineData, ShellError, Signature, Span, SyntaxShape, Type, Value,
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
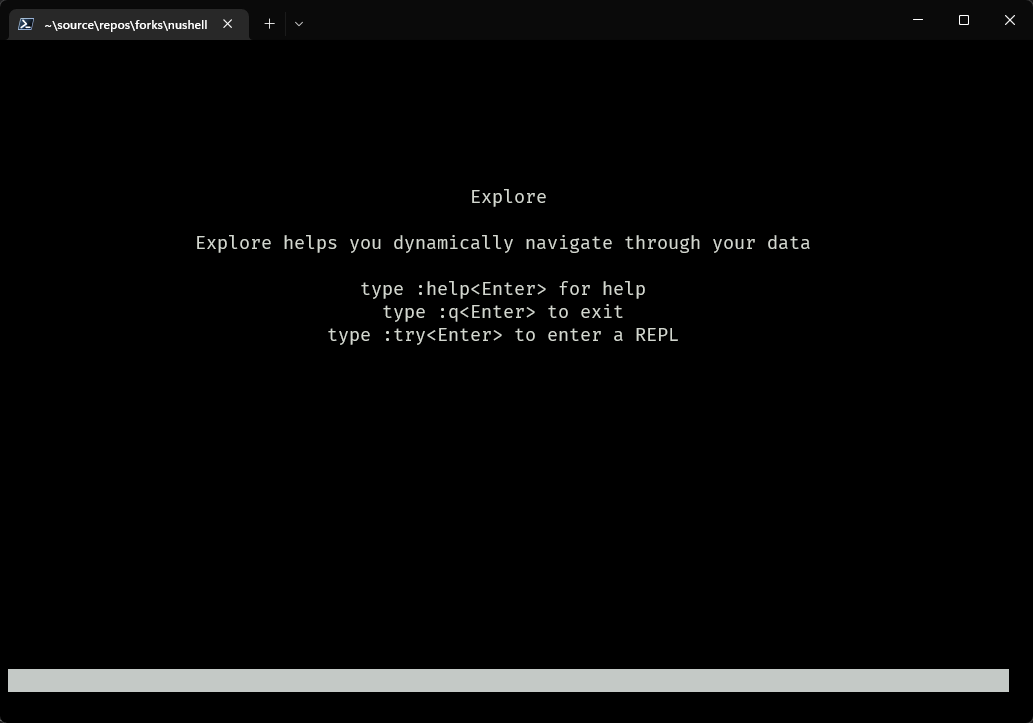
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
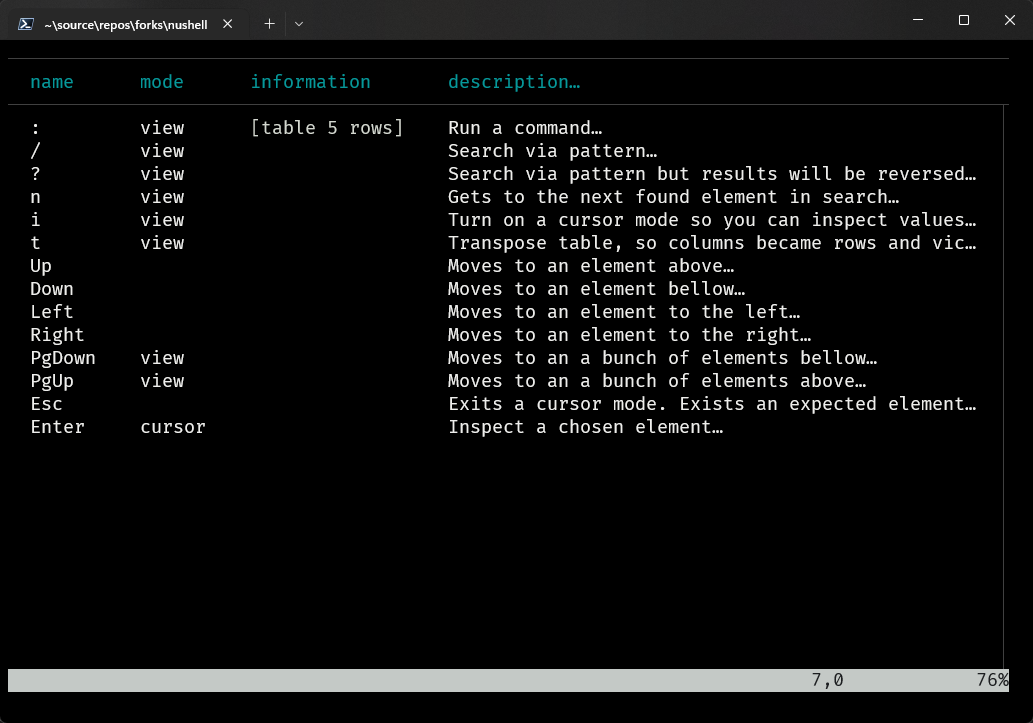
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
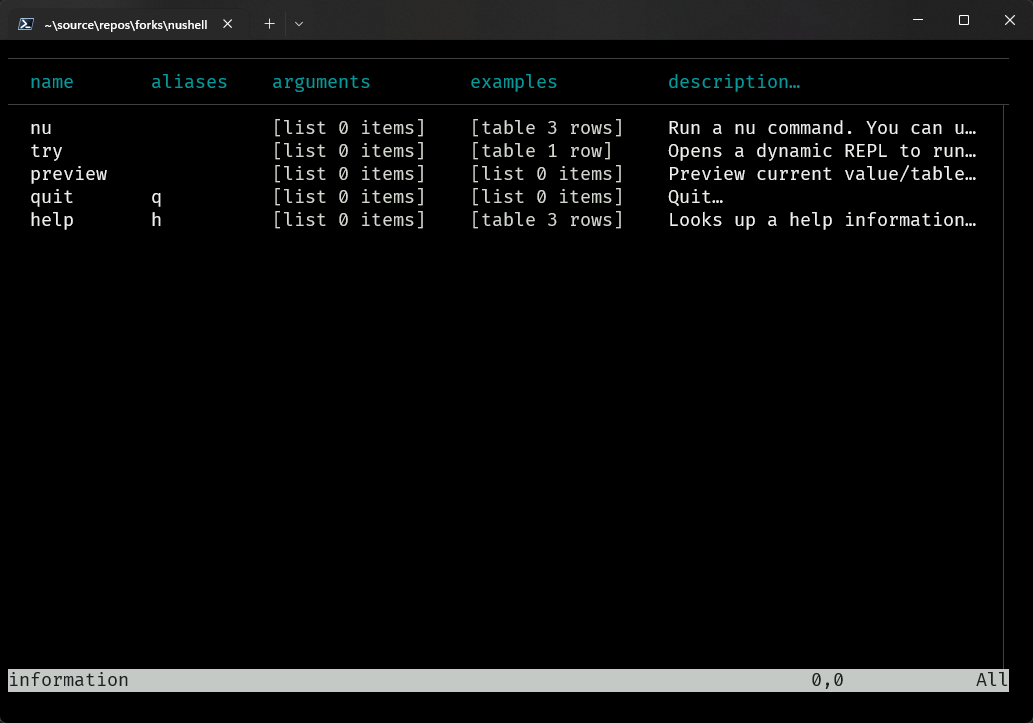
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
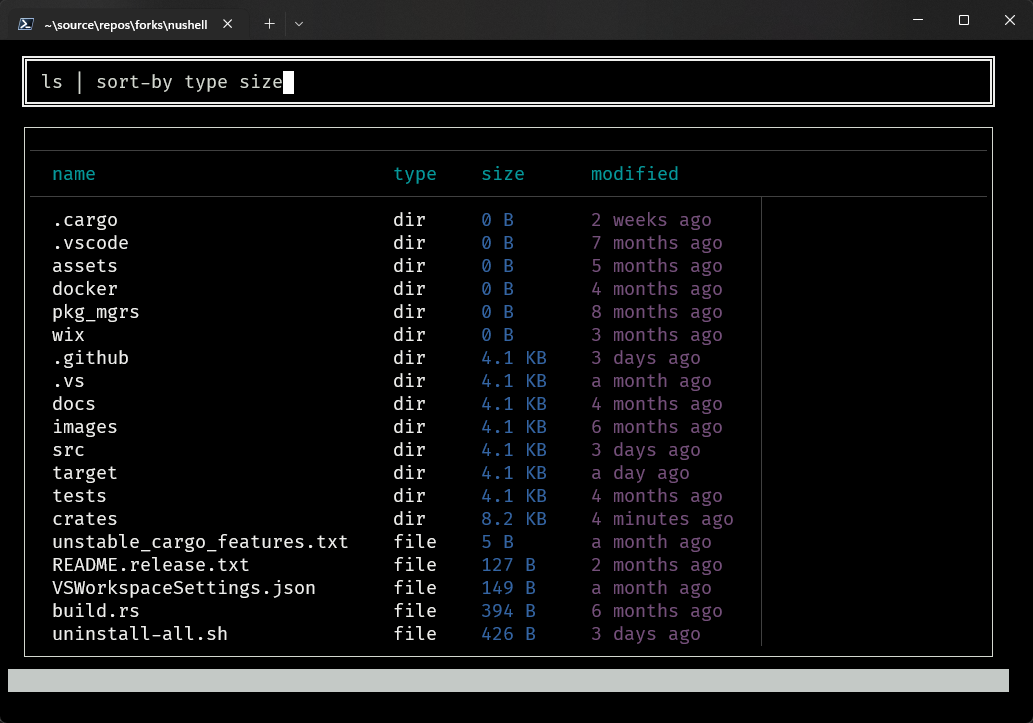
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
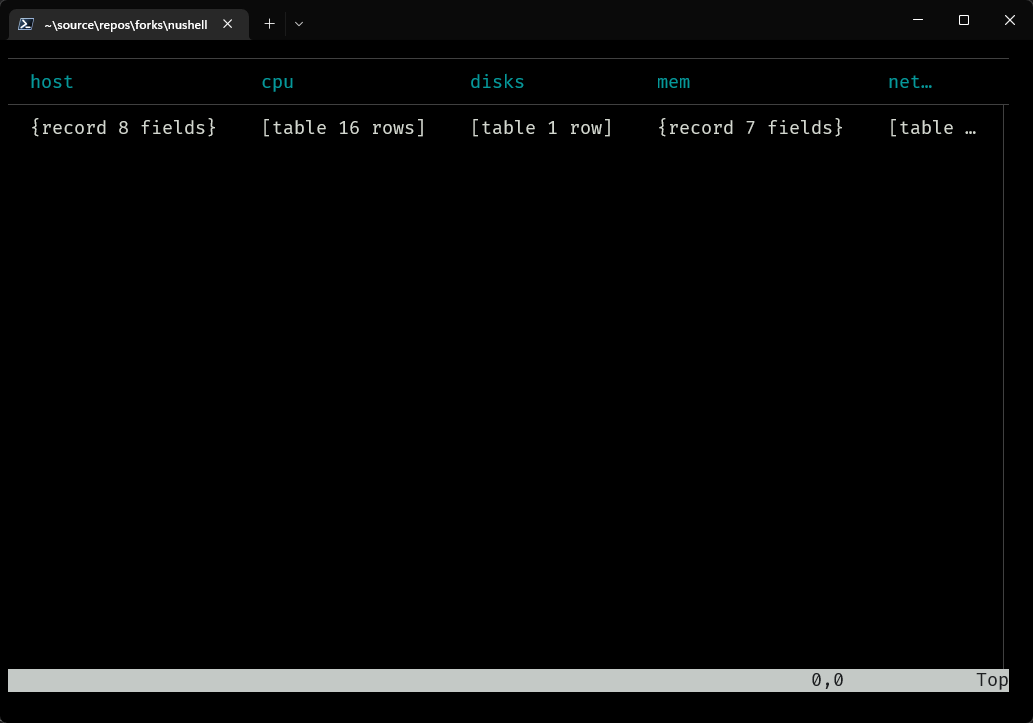
If you hit the `t` key it will now transpose the view to look like this.
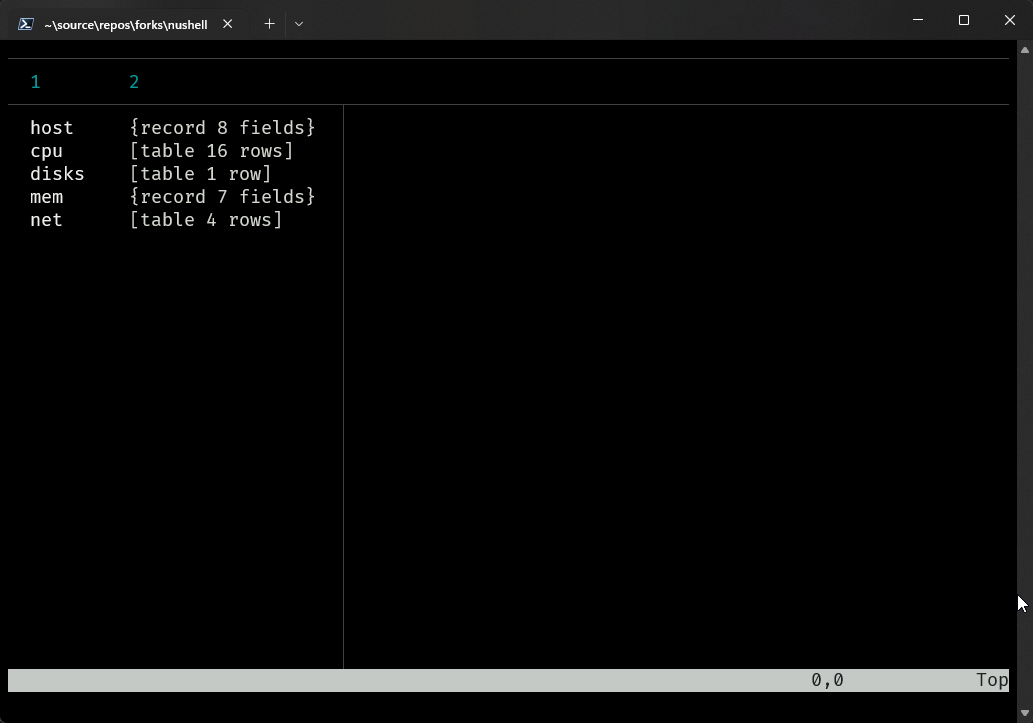
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
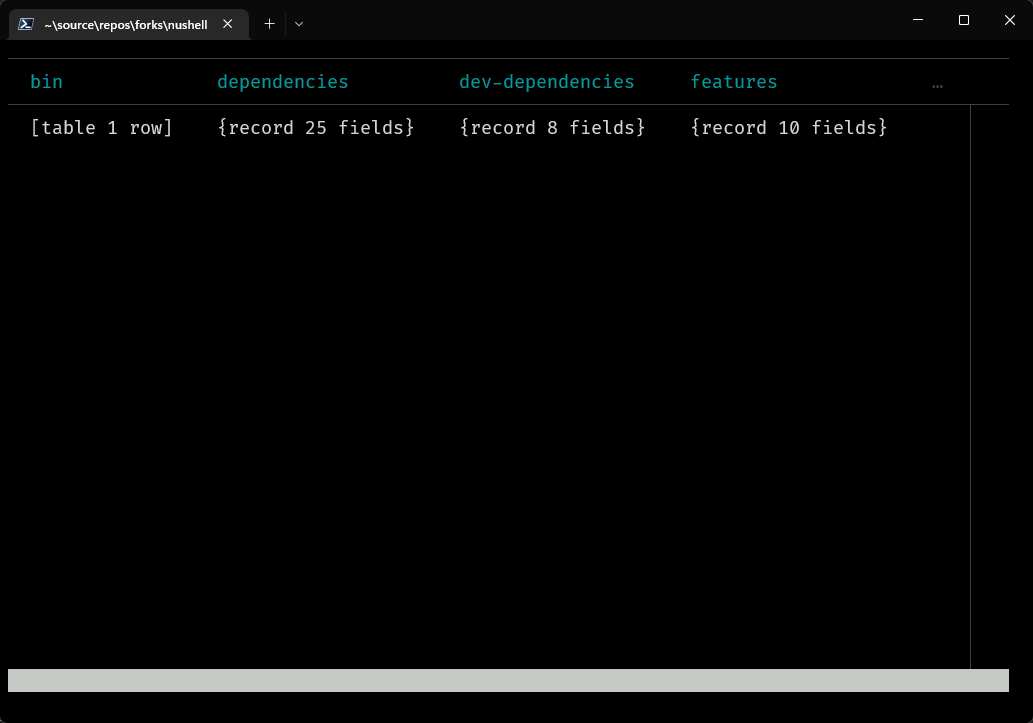
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
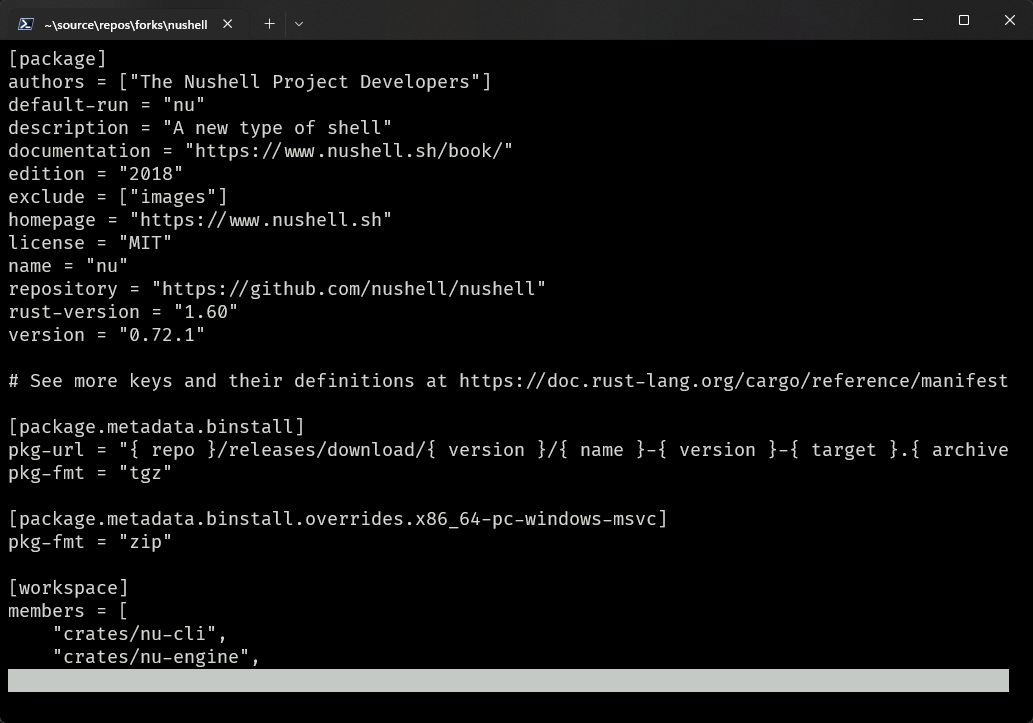
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
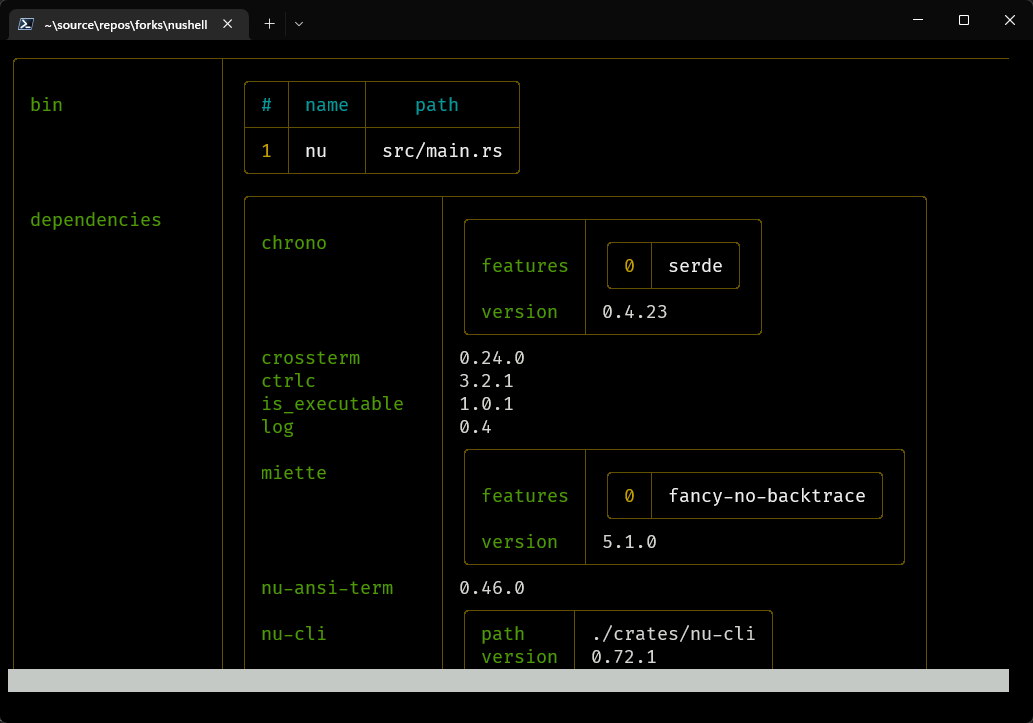
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
};
|
revert: move to ahash (#9464)
This PR reverts https://github.com/nushell/nushell/pull/9391
We try not to revert PRs like this, though after discussion with the
Nushell team, we decided to revert this one.
The main reason is that Nushell, as a codebase, isn't ready for these
kinds of optimisations. It's in the part of the development cycle where
our main focus should be on improving the algorithms inside of Nushell
itself. Once we have matured our algorithms, then we can look for
opportunities to switch out technologies we're using for alternate
forms.
Much of Nushell still has lots of opportunities for tuning the codebase,
paying down technical debt, and making the codebase generally cleaner
and more robust. This should be the focus. Performance improvements
should flow out of that work.
Said another, optimisation that isn't part of tuning the codebase is
premature at this stage. We need to focus on doing the hard work of
making the engine, parser, etc better.
# User-Facing Changes
Reverts the HashMap -> ahash change.
cc @FilipAndersson245
2023-06-18 03:27:57 +00:00
|
|
|
use std::collections::HashMap;
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
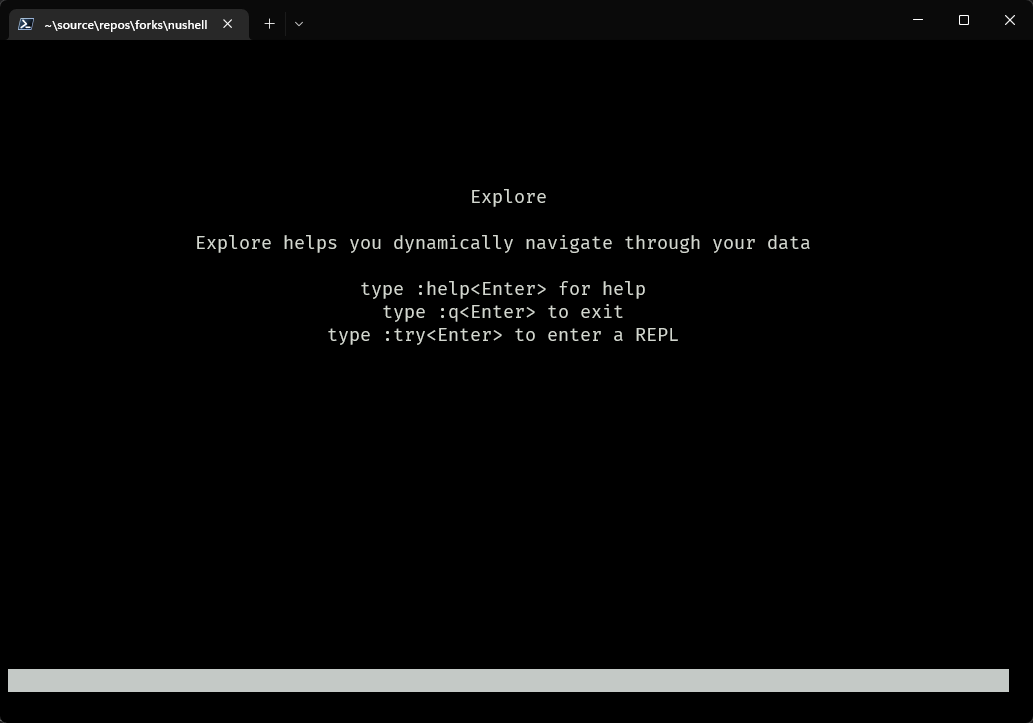
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
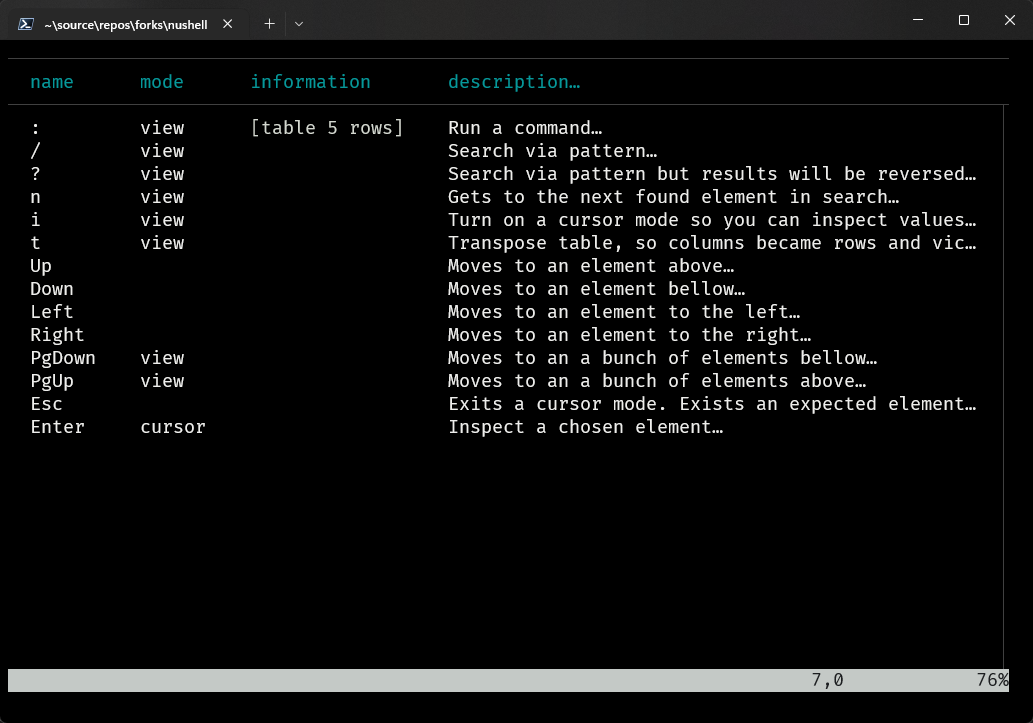
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
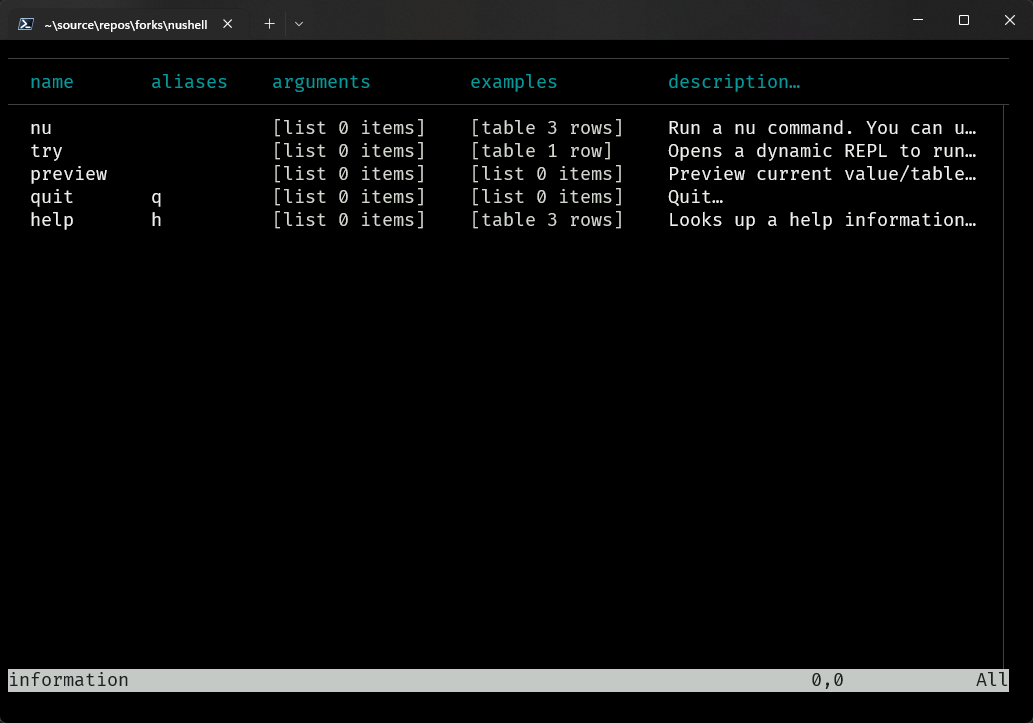
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
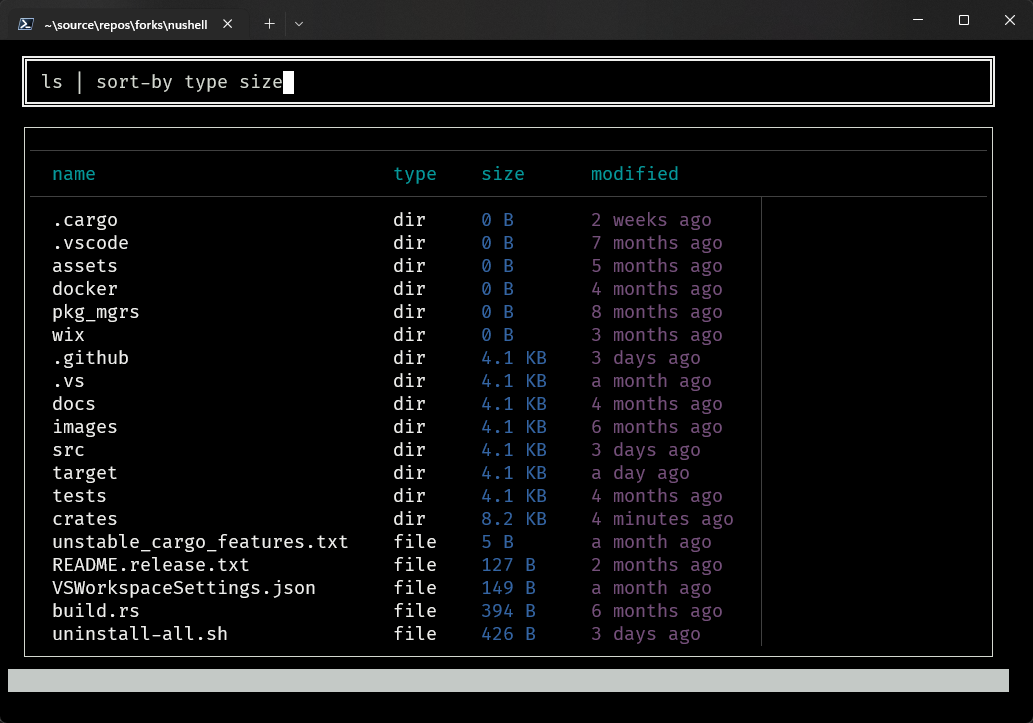
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
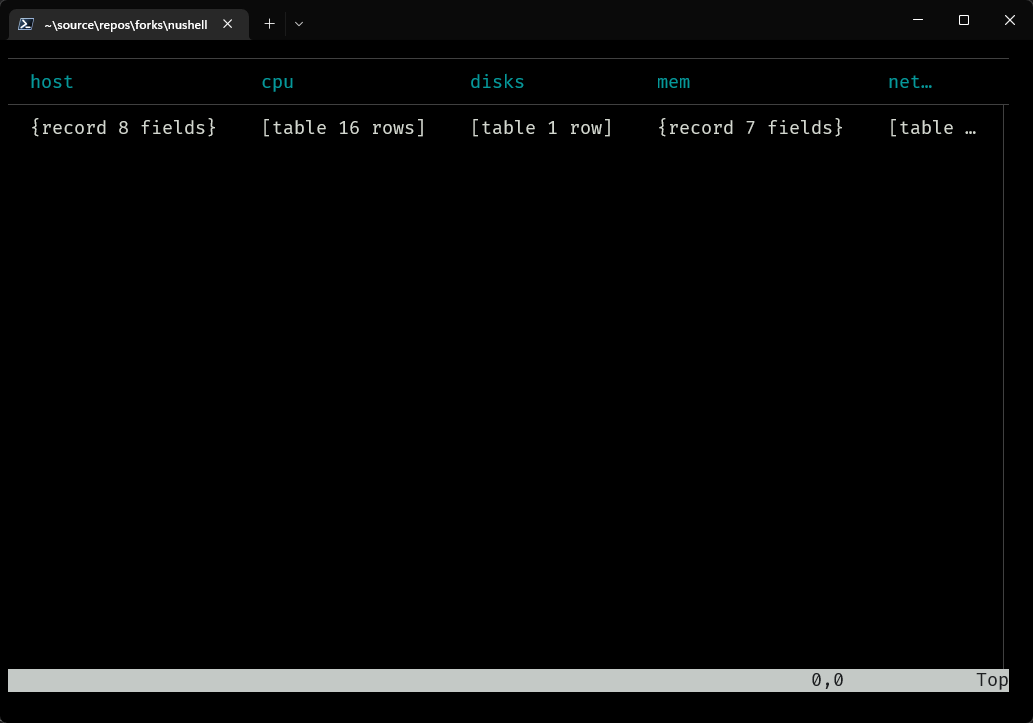
If you hit the `t` key it will now transpose the view to look like this.
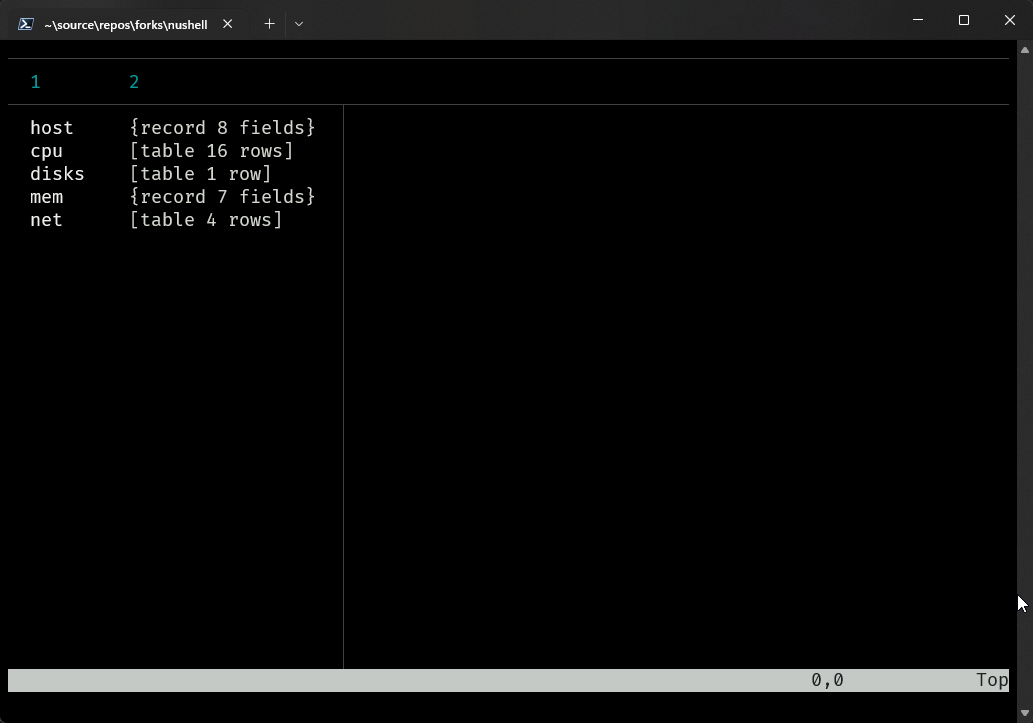
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
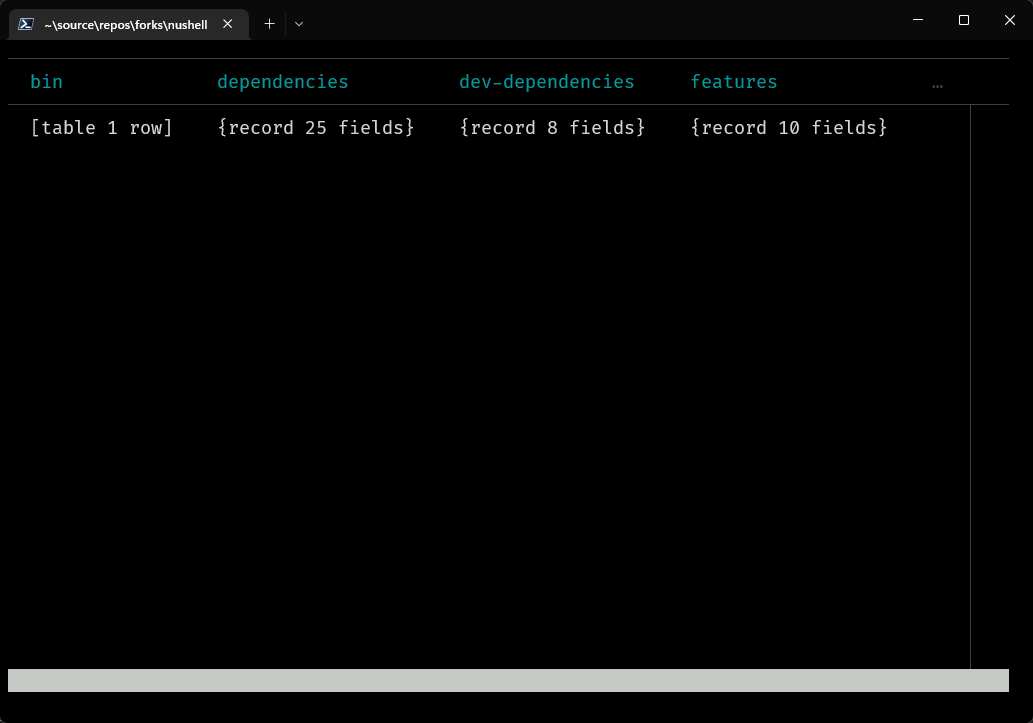
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
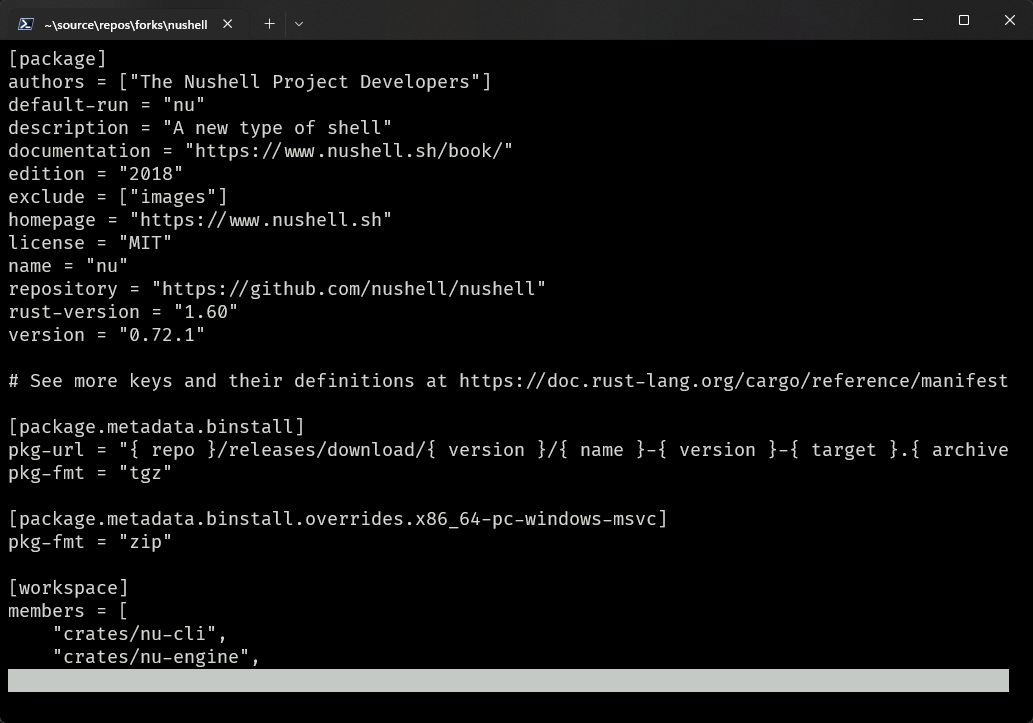
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
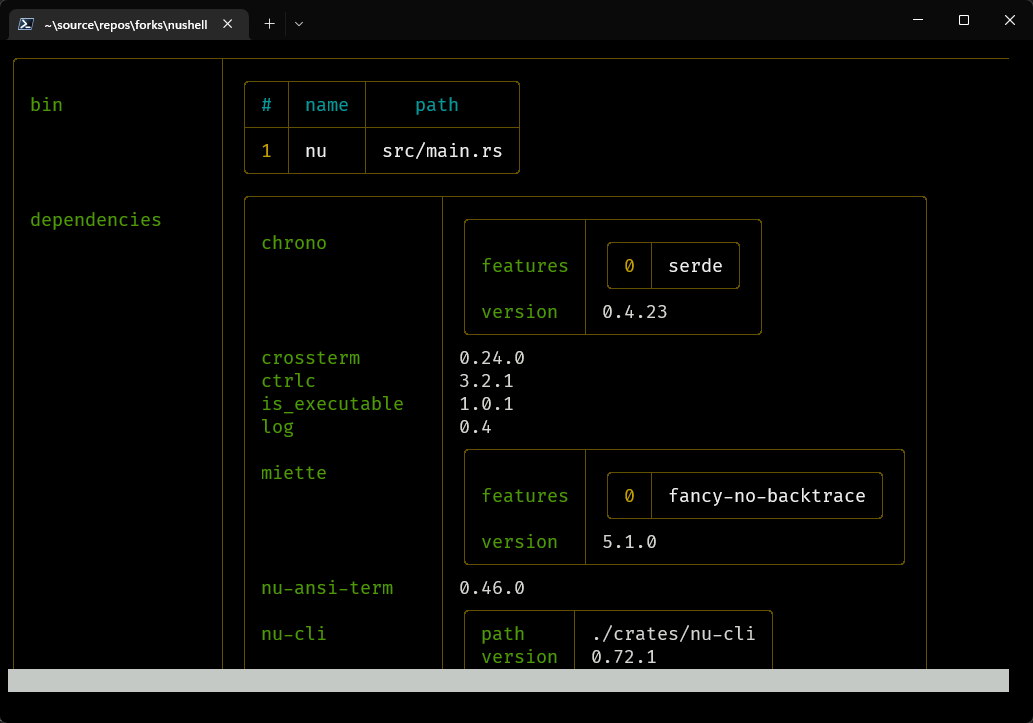
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
|
|
|
|
/// A `less` like program to render a [Value] as a table.
|
|
|
|
#[derive(Clone)]
|
|
|
|
pub struct Explore;
|
|
|
|
|
|
|
|
impl Command for Explore {
|
|
|
|
fn name(&self) -> &str {
|
|
|
|
"explore"
|
|
|
|
}
|
|
|
|
|
|
|
|
fn usage(&self) -> &str {
|
2023-03-01 05:33:02 +00:00
|
|
|
"Explore acts as a table pager, just like `less` does for text."
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
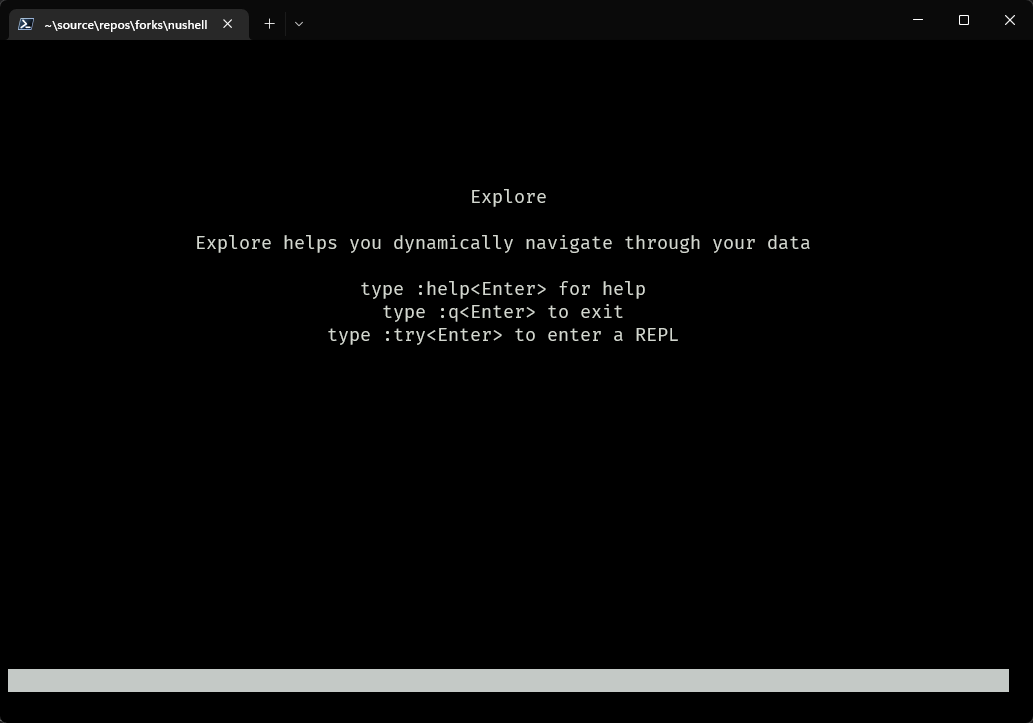
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
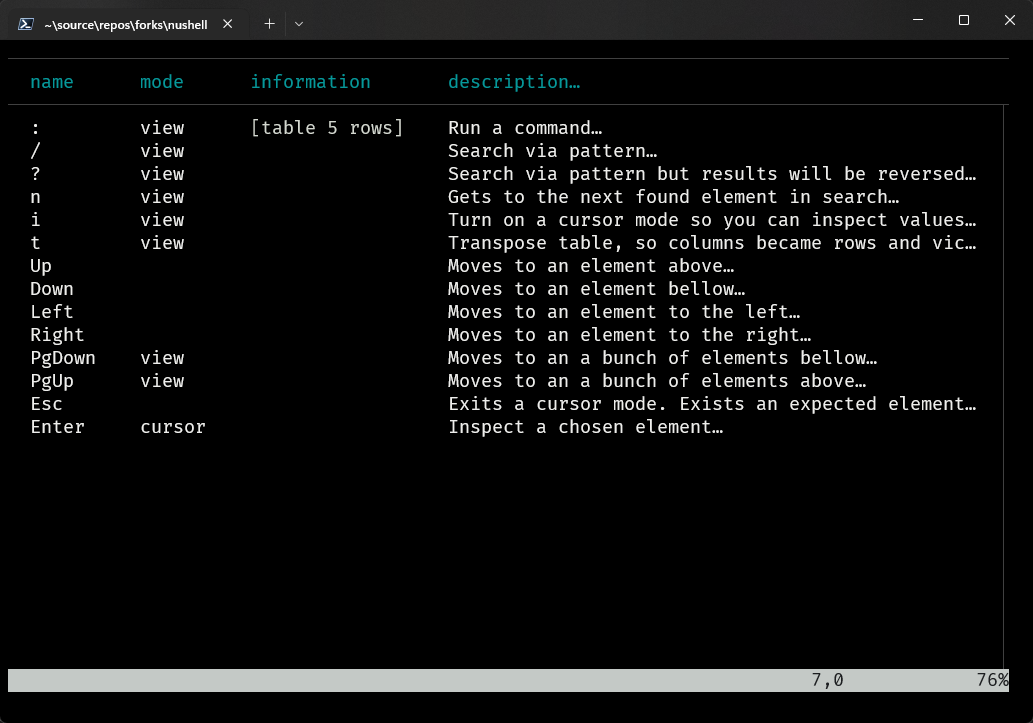
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
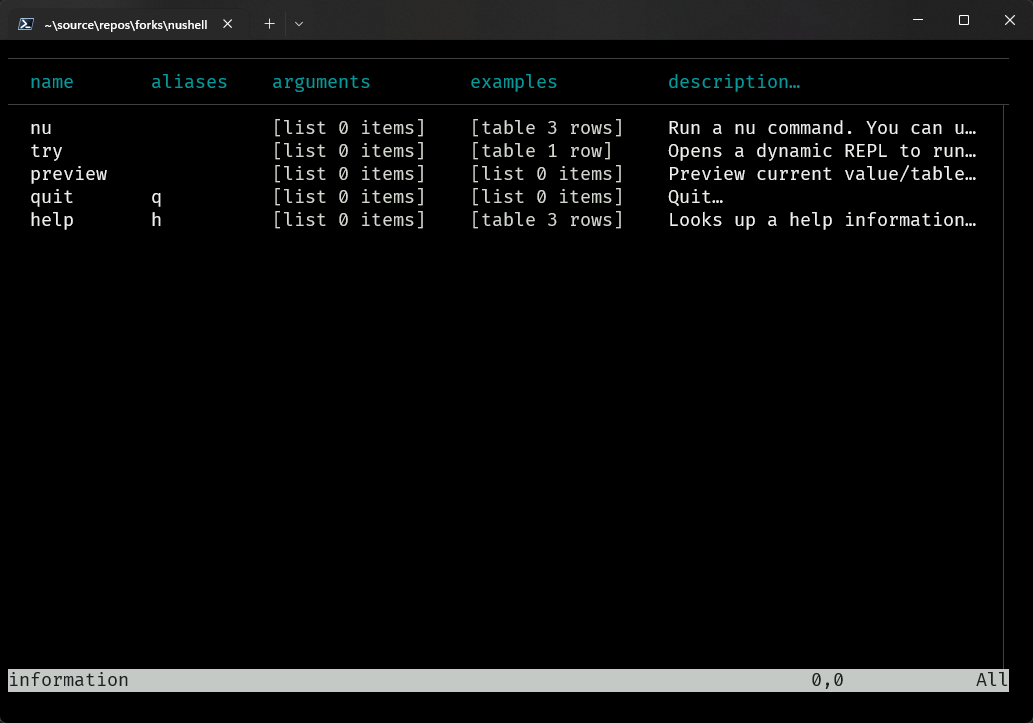
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
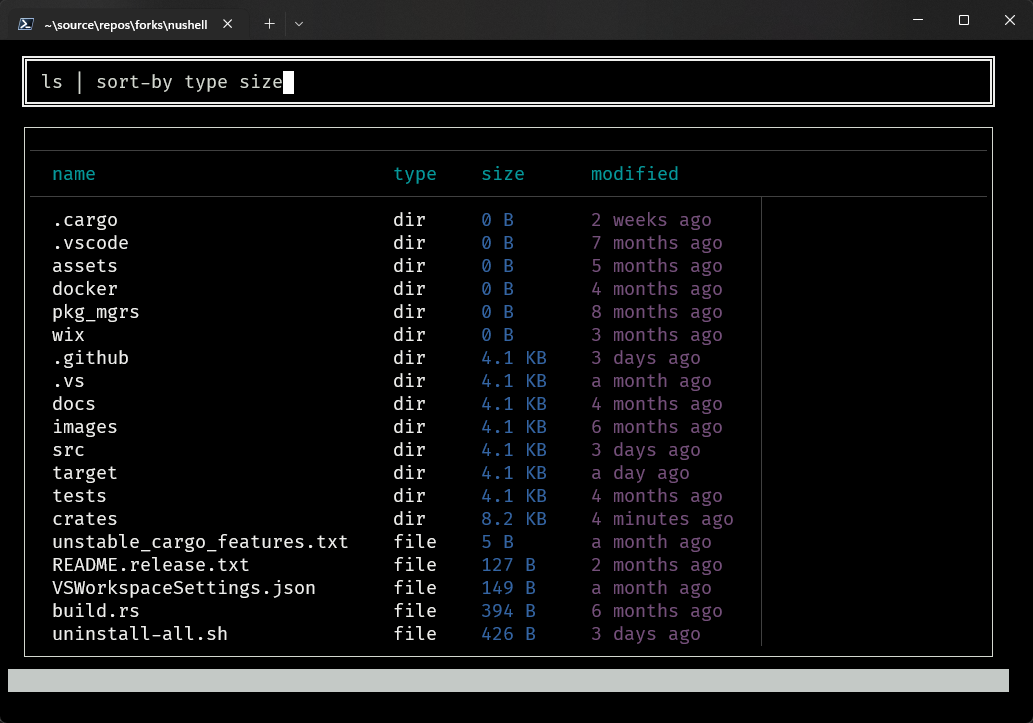
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
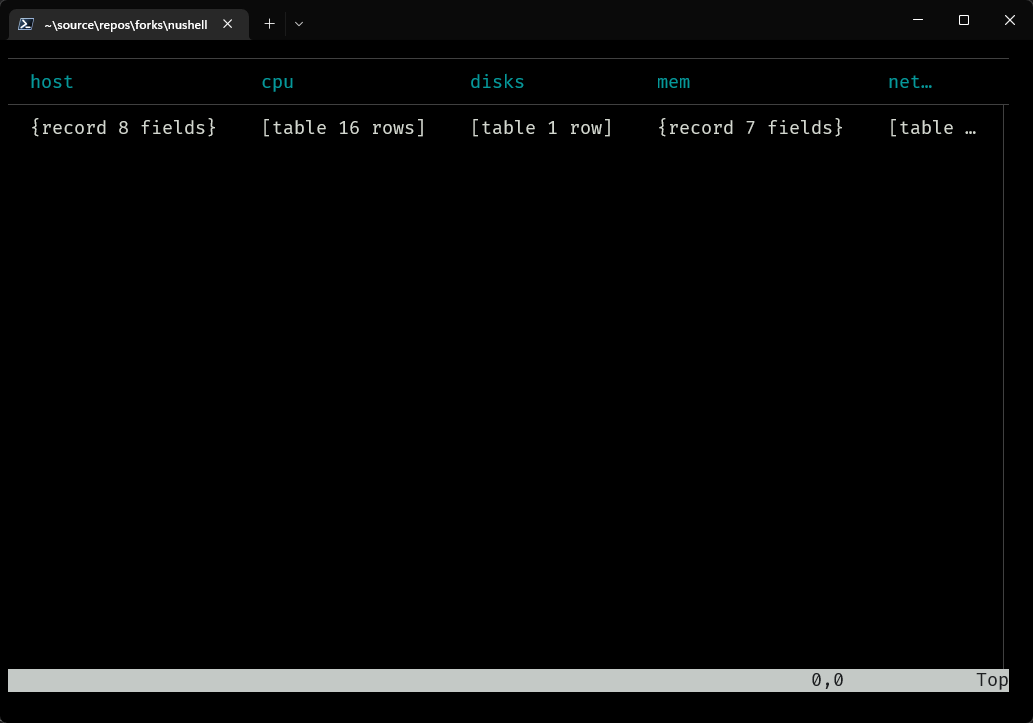
If you hit the `t` key it will now transpose the view to look like this.
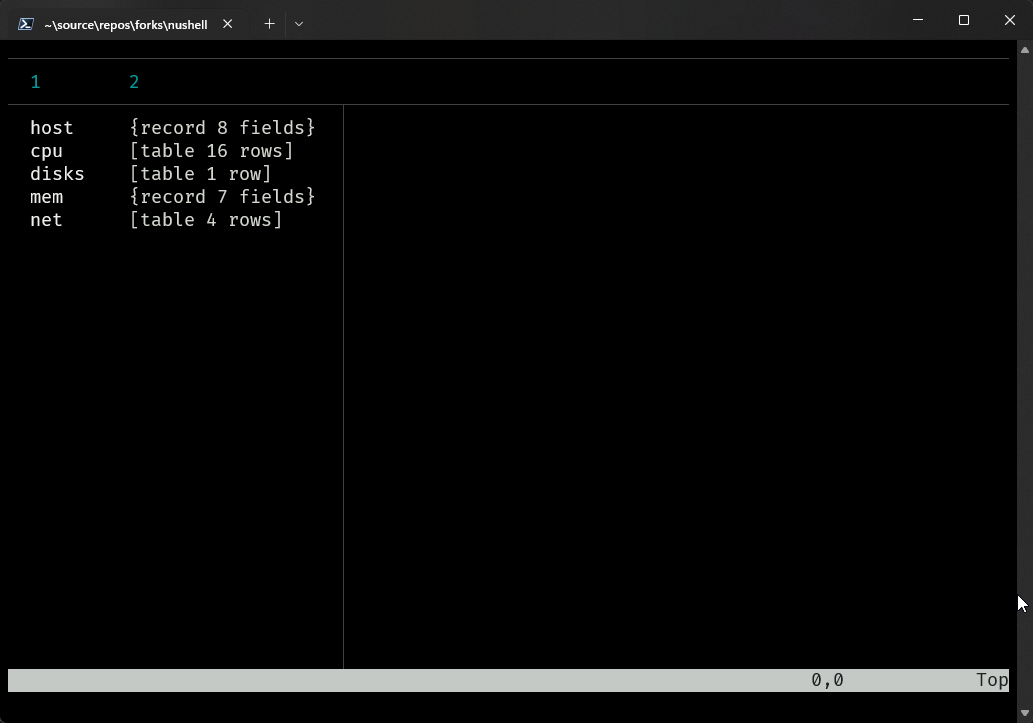
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
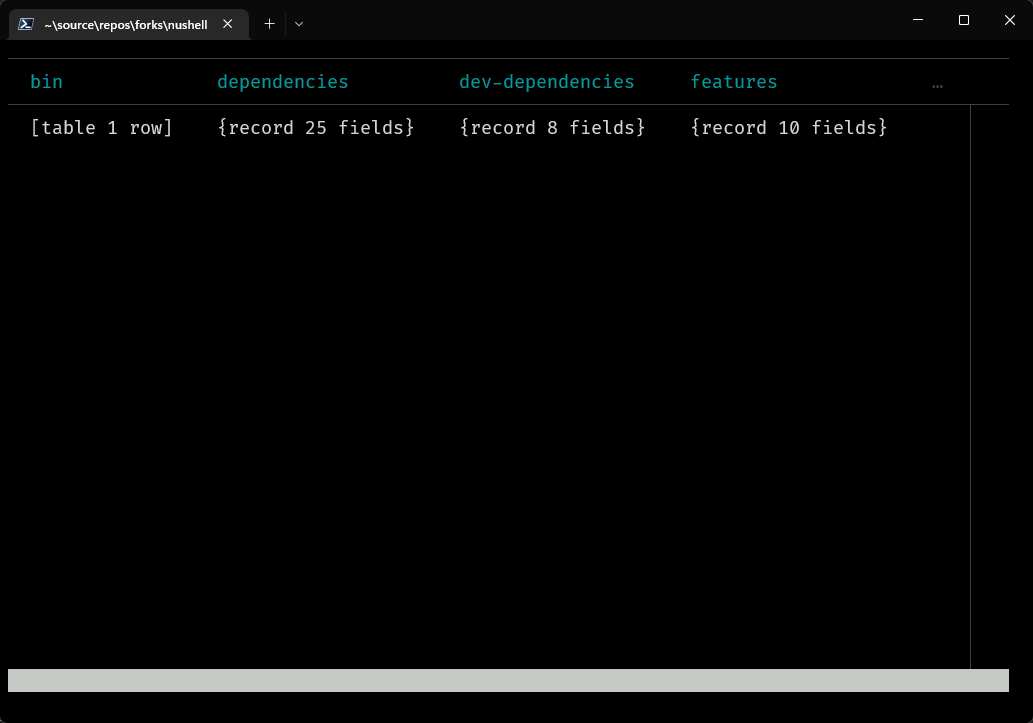
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
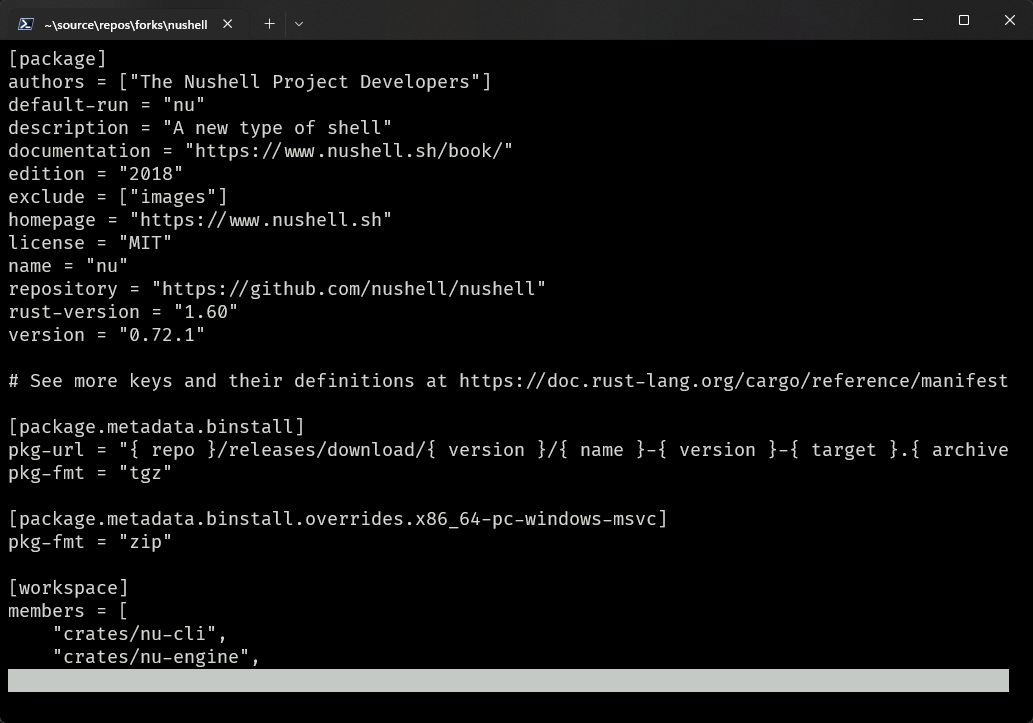
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
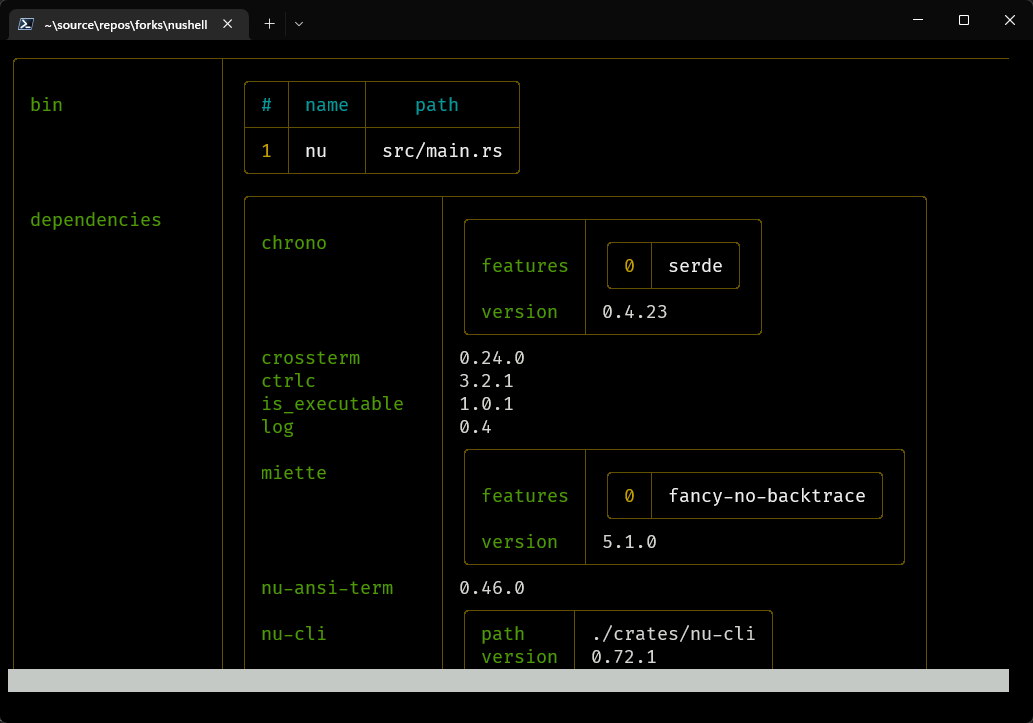
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
fn signature(&self) -> nu_protocol::Signature {
|
|
|
|
// todo: Fix error message when it's empty
|
|
|
|
// if we set h i short flags it panics????
|
|
|
|
|
|
|
|
Signature::build("explore")
|
2022-12-21 19:20:46 +00:00
|
|
|
.input_output_types(vec![(Type::Any, Type::Any)])
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
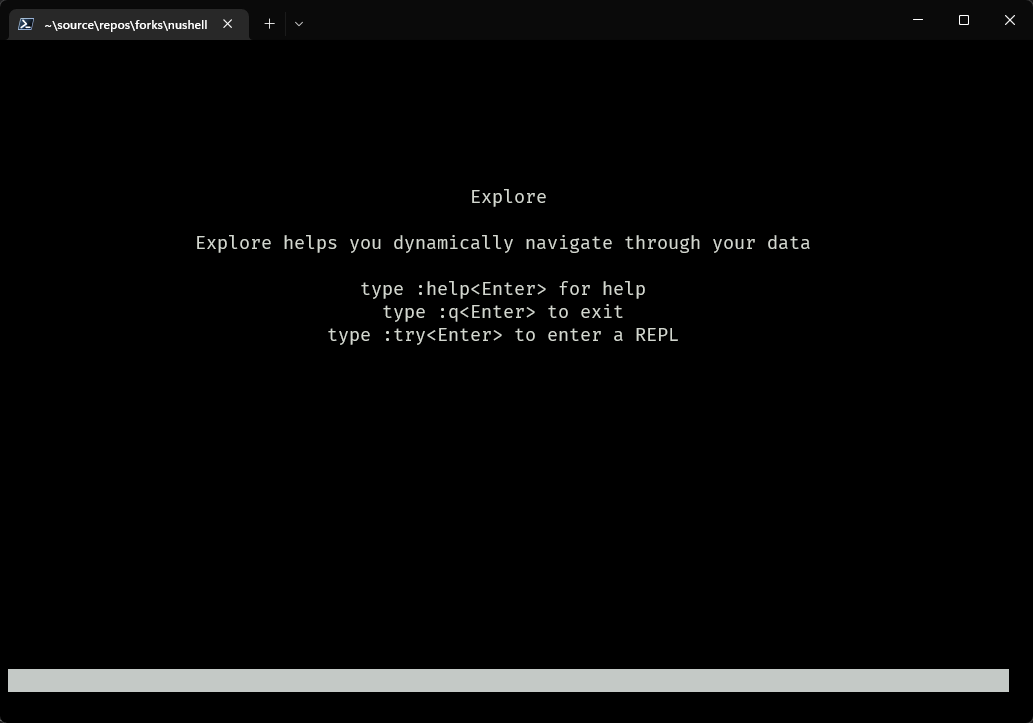
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
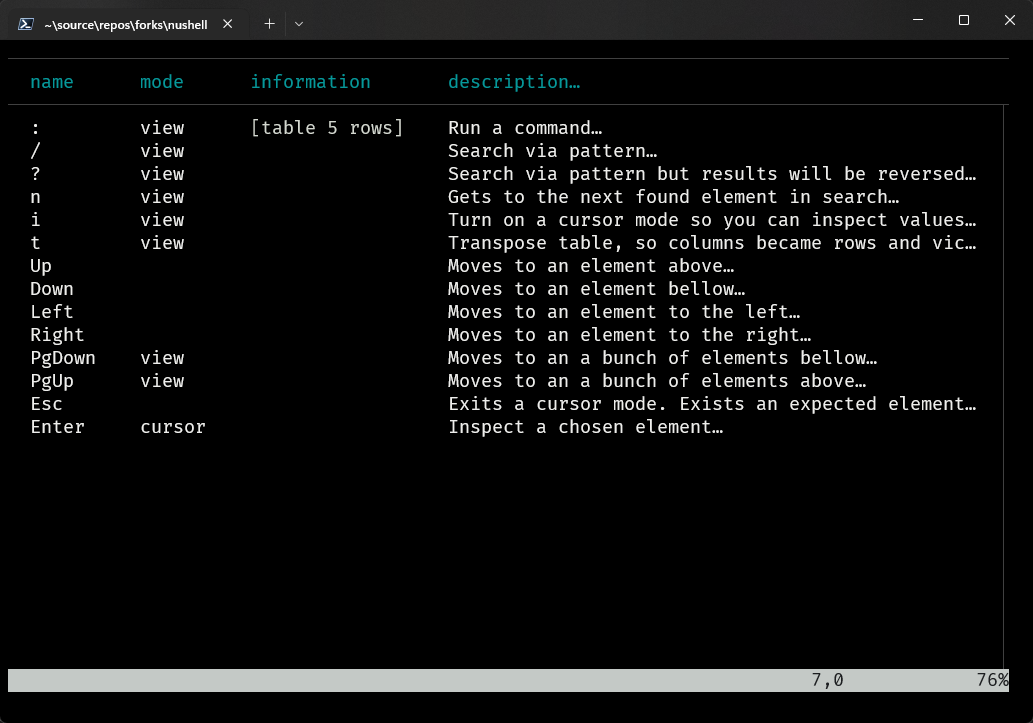
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
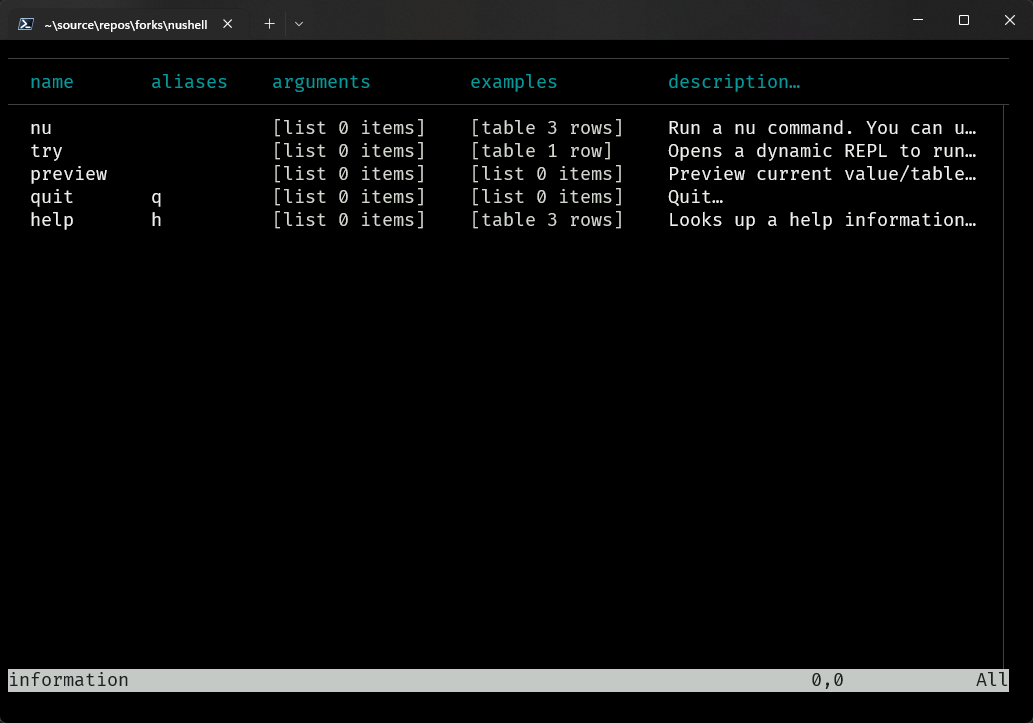
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
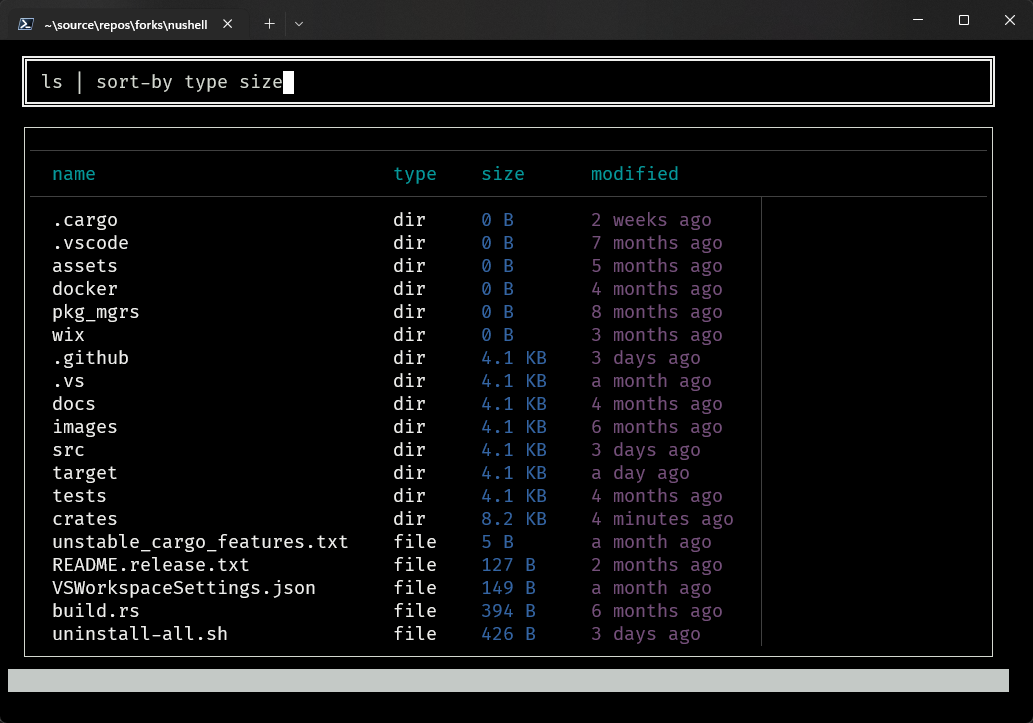
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
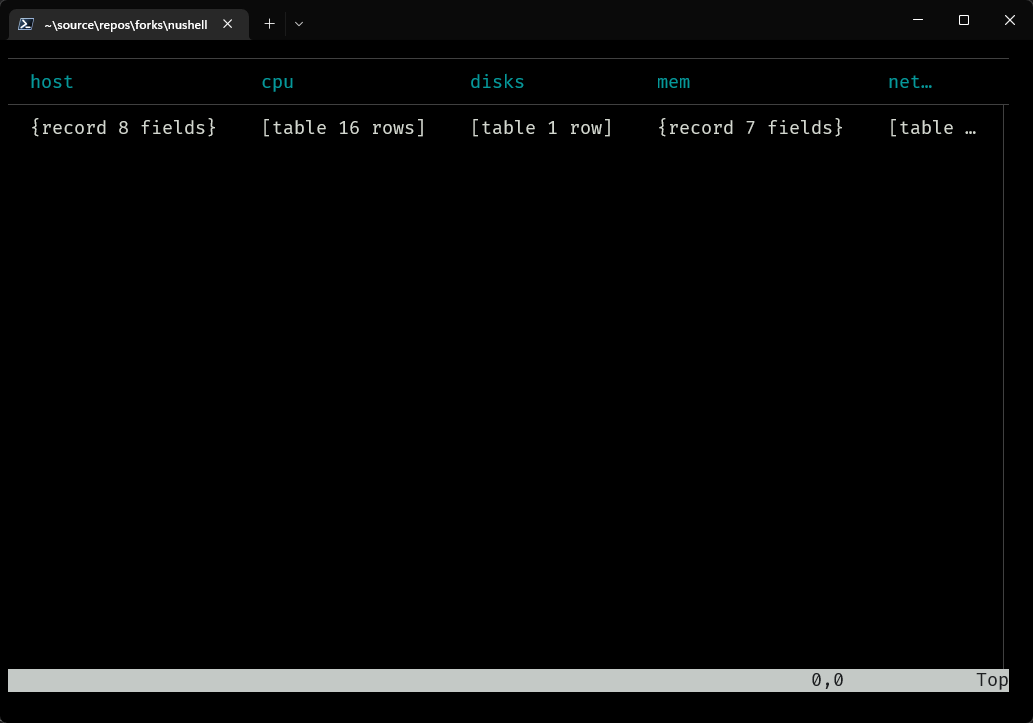
If you hit the `t` key it will now transpose the view to look like this.
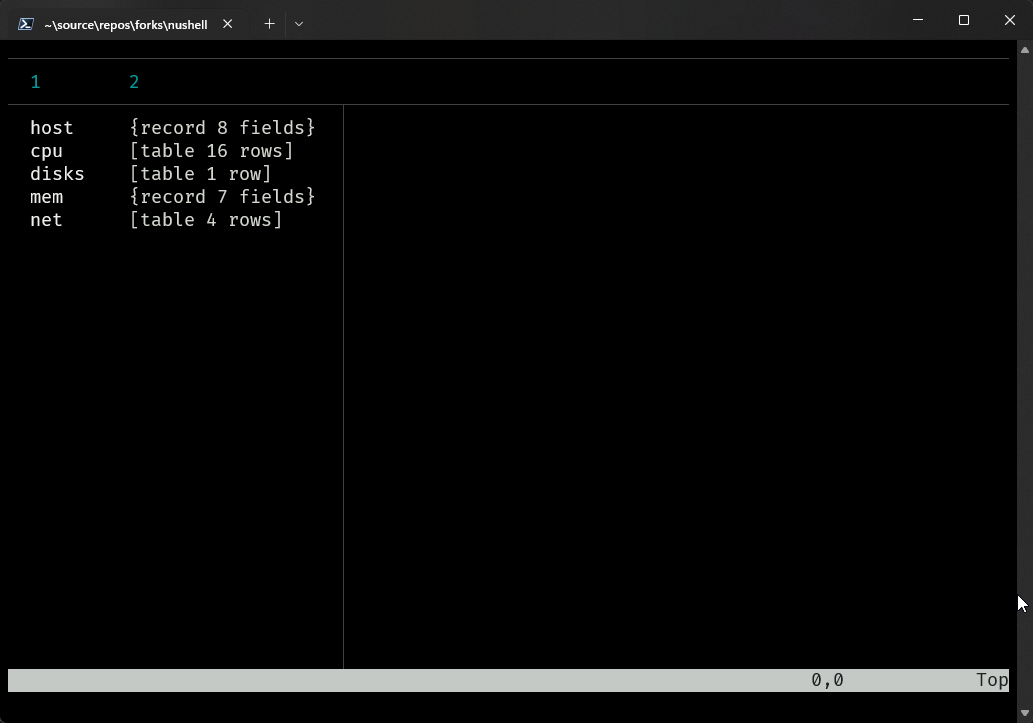
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
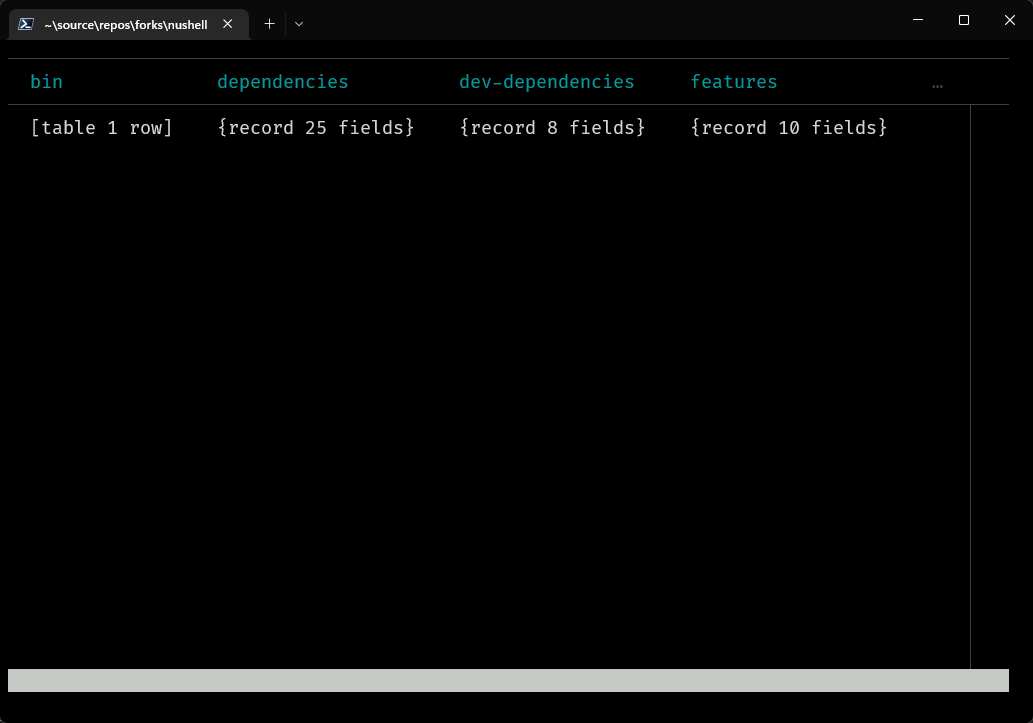
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
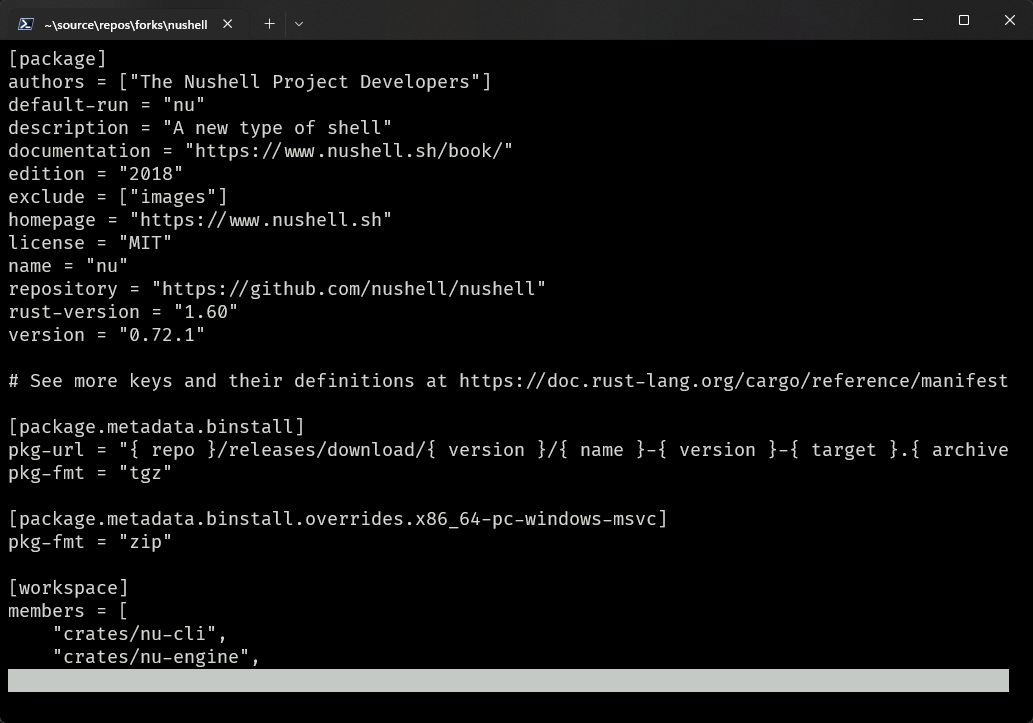
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
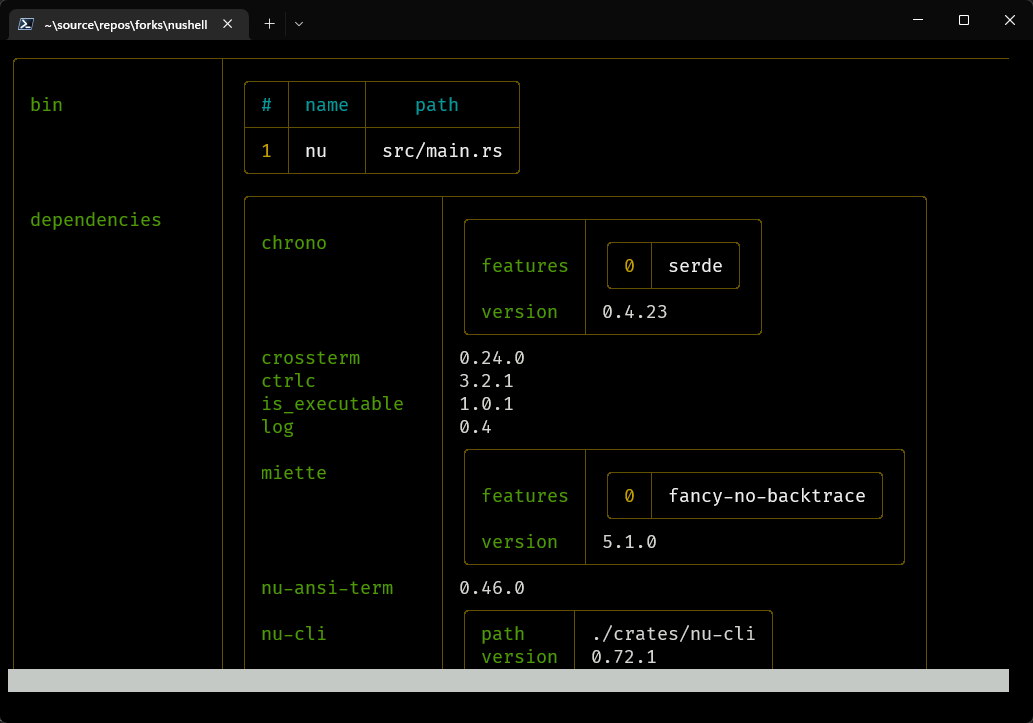
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
.named(
|
|
|
|
"head",
|
|
|
|
SyntaxShape::Boolean,
|
2022-12-02 14:01:02 +00:00
|
|
|
"Show or hide column headers (default true)",
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
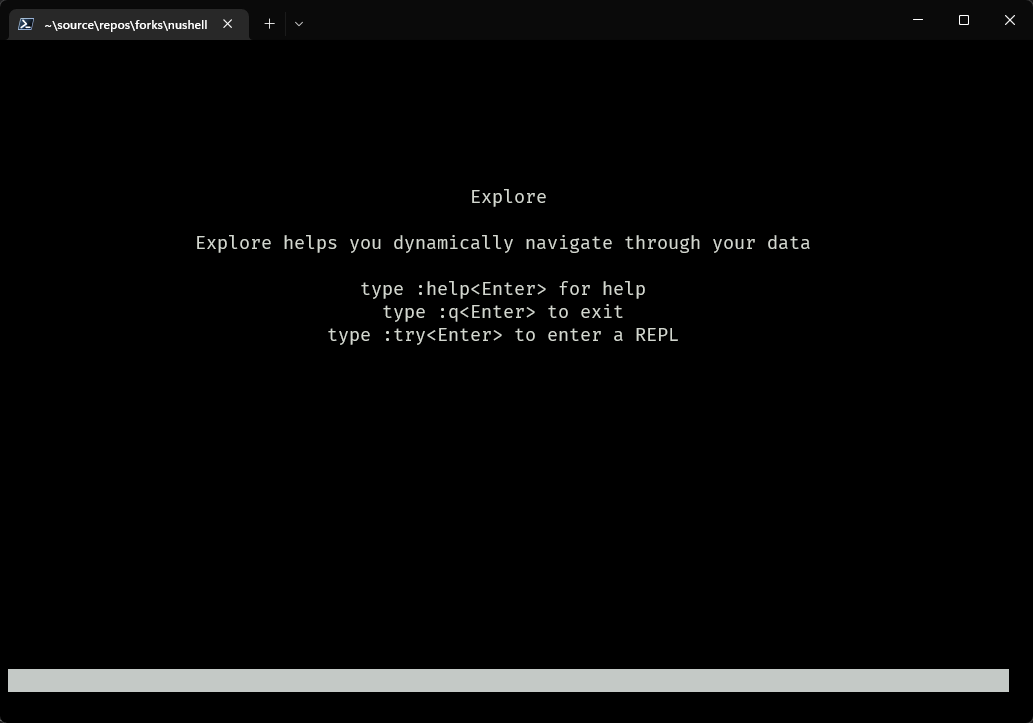
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
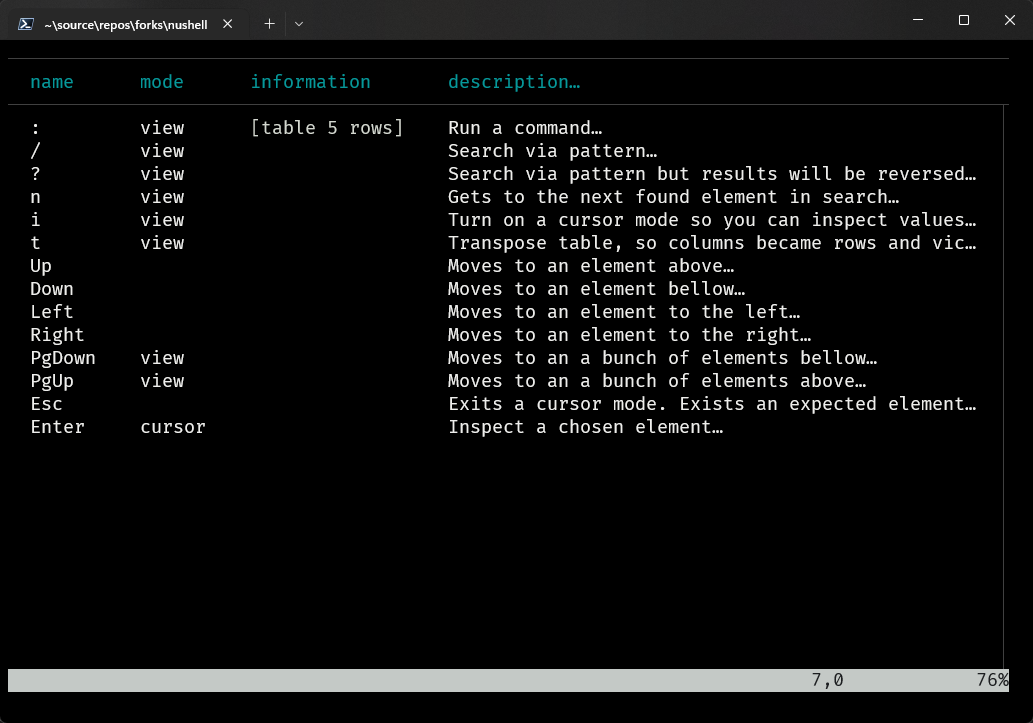
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
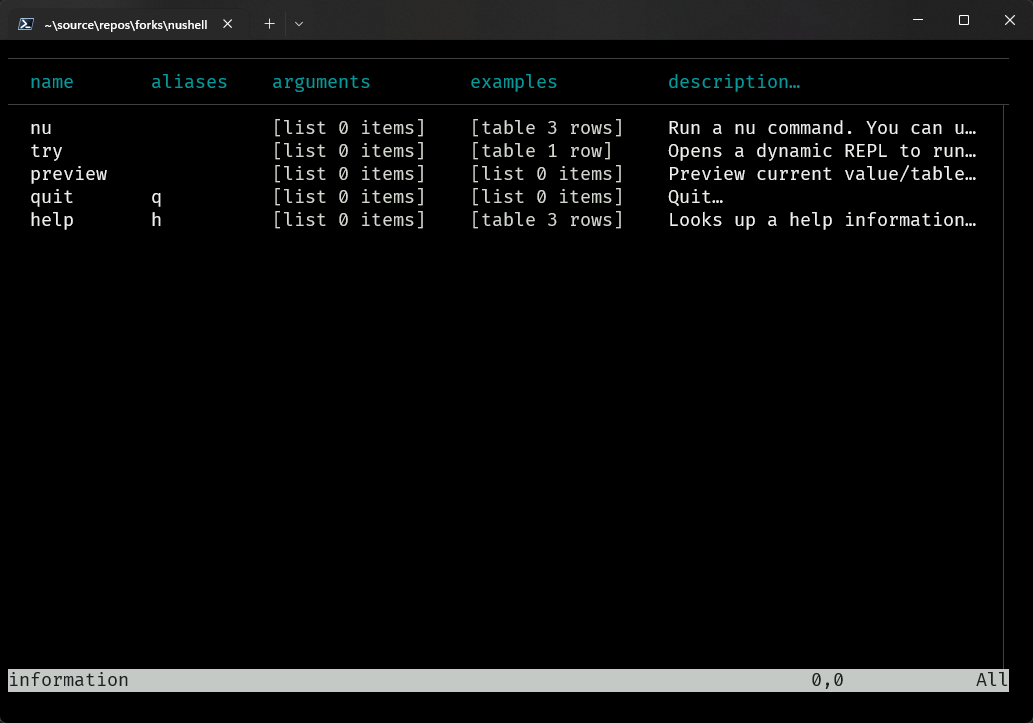
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
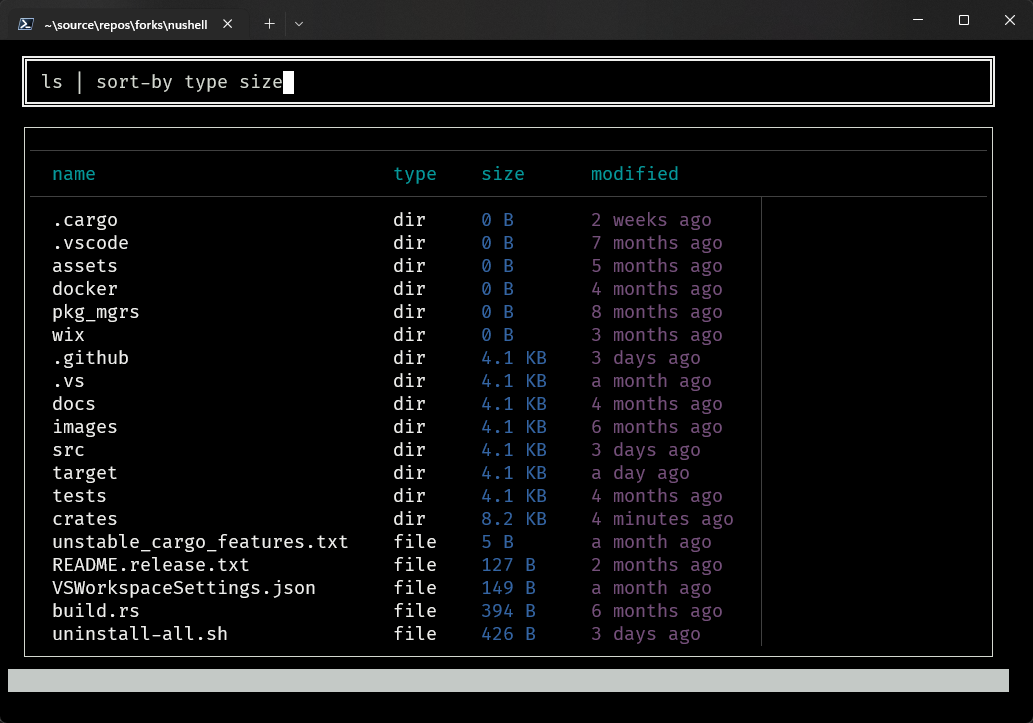
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
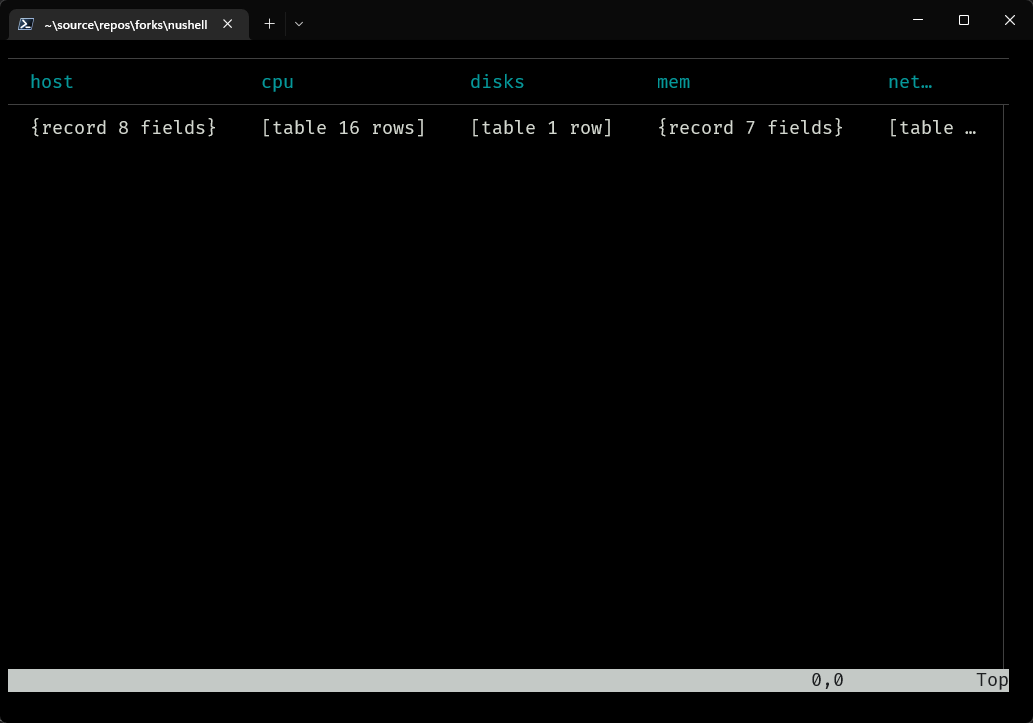
If you hit the `t` key it will now transpose the view to look like this.
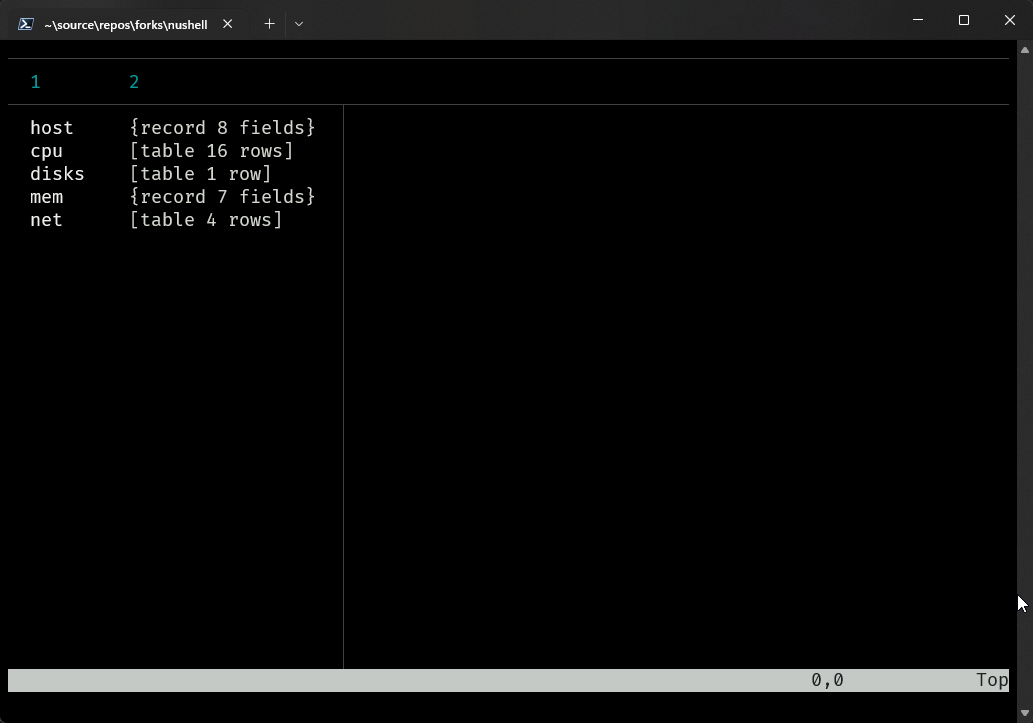
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
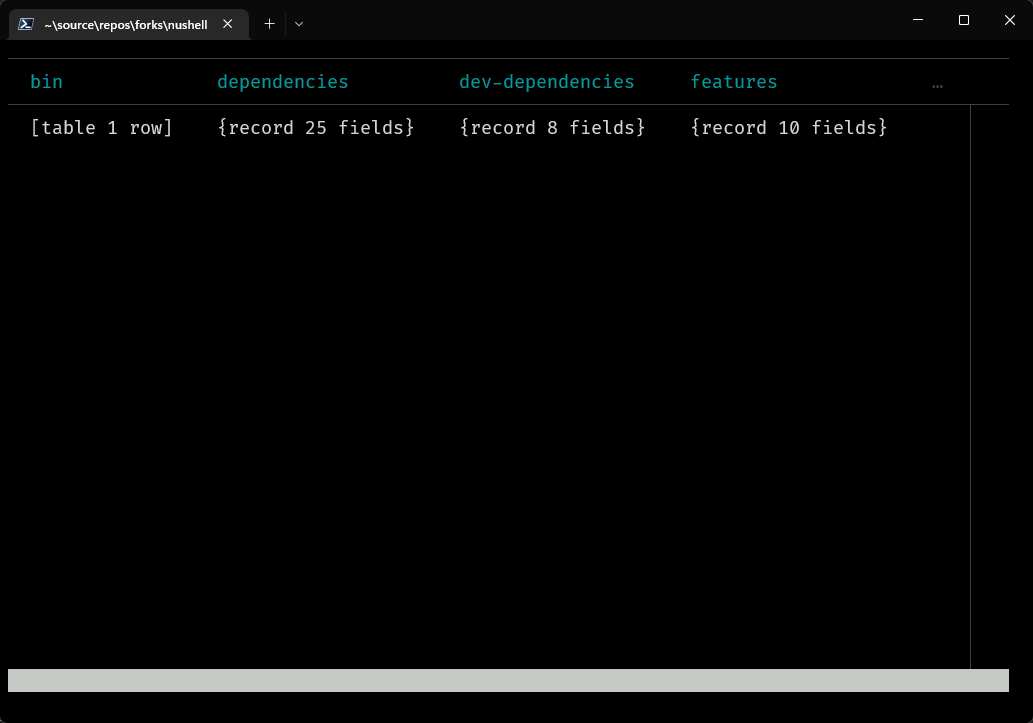
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
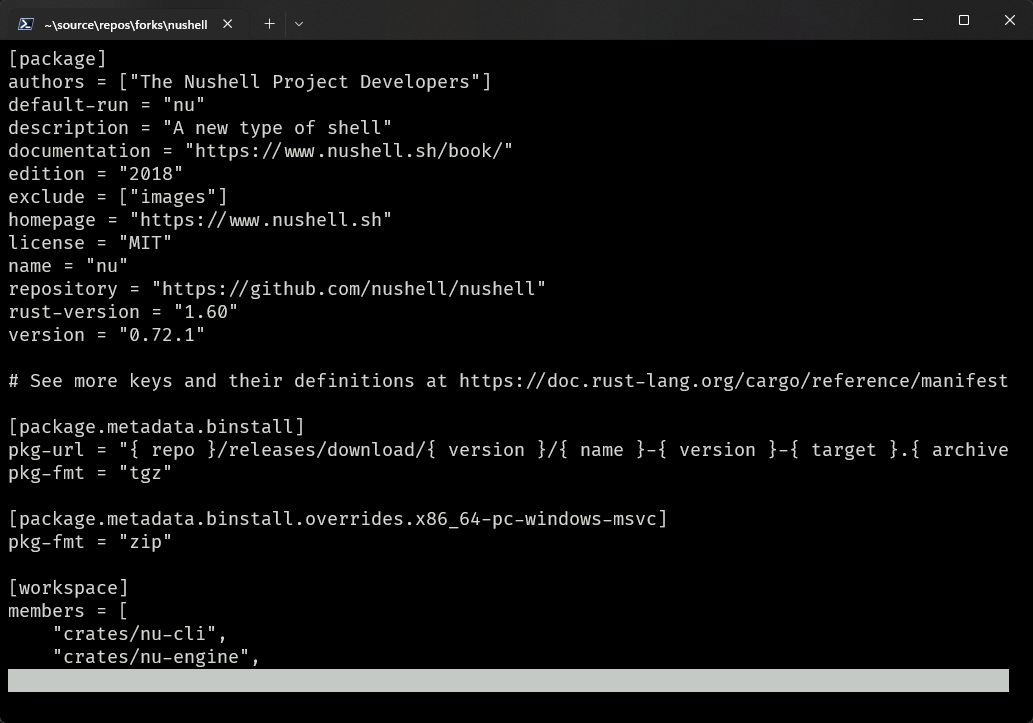
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
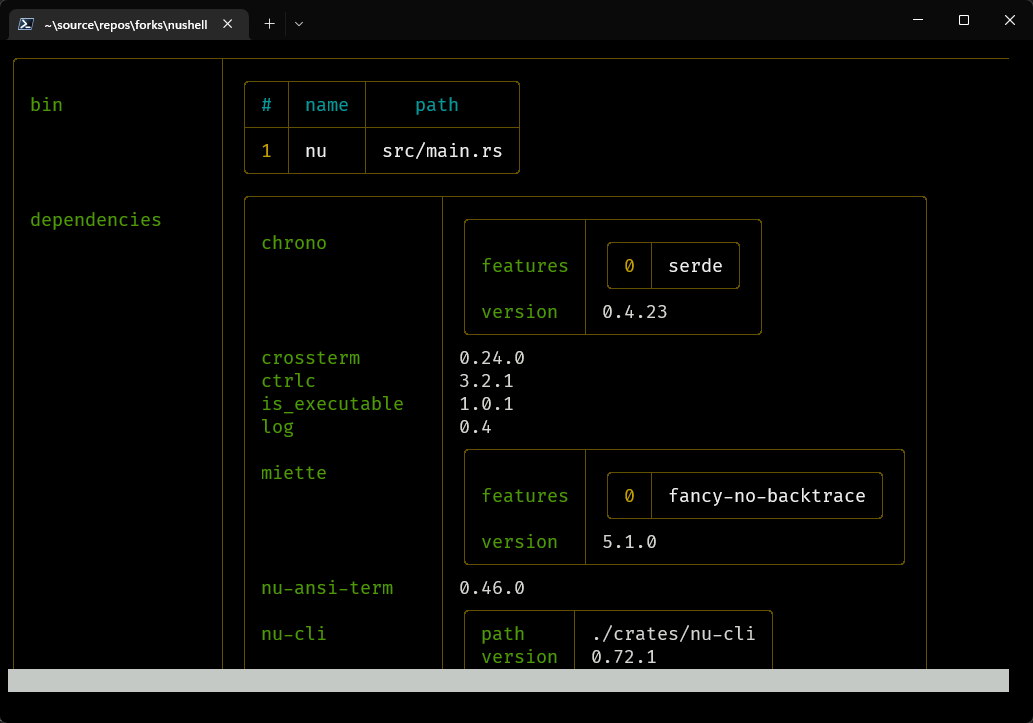
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
None,
|
|
|
|
)
|
2022-12-02 14:01:02 +00:00
|
|
|
.switch("index", "Show row indexes when viewing a list", Some('i'))
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
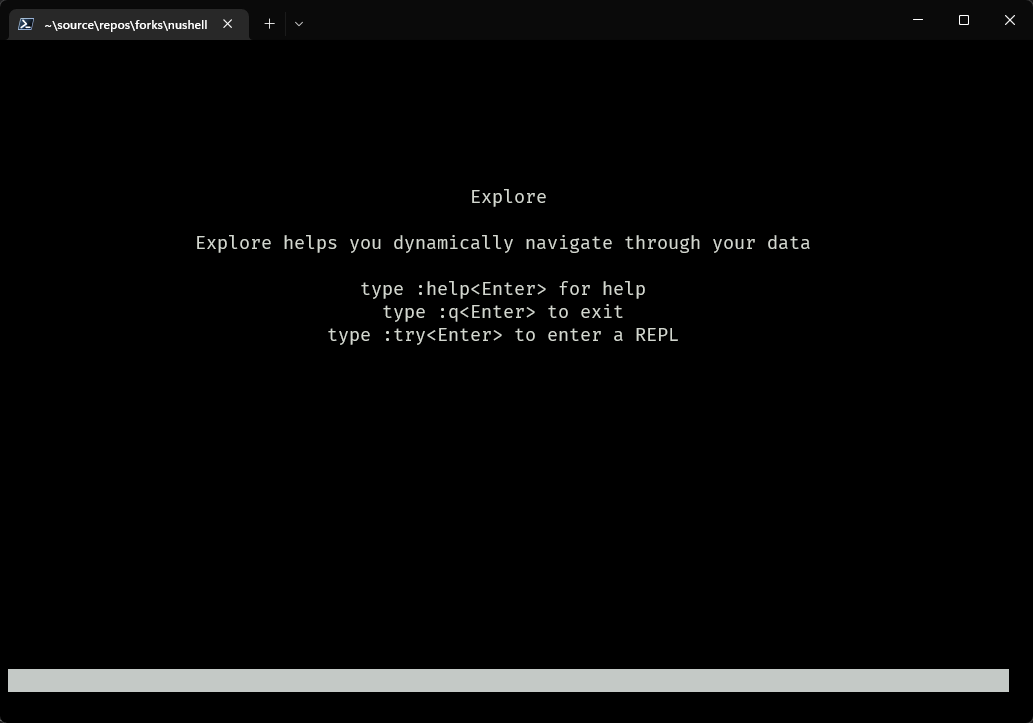
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
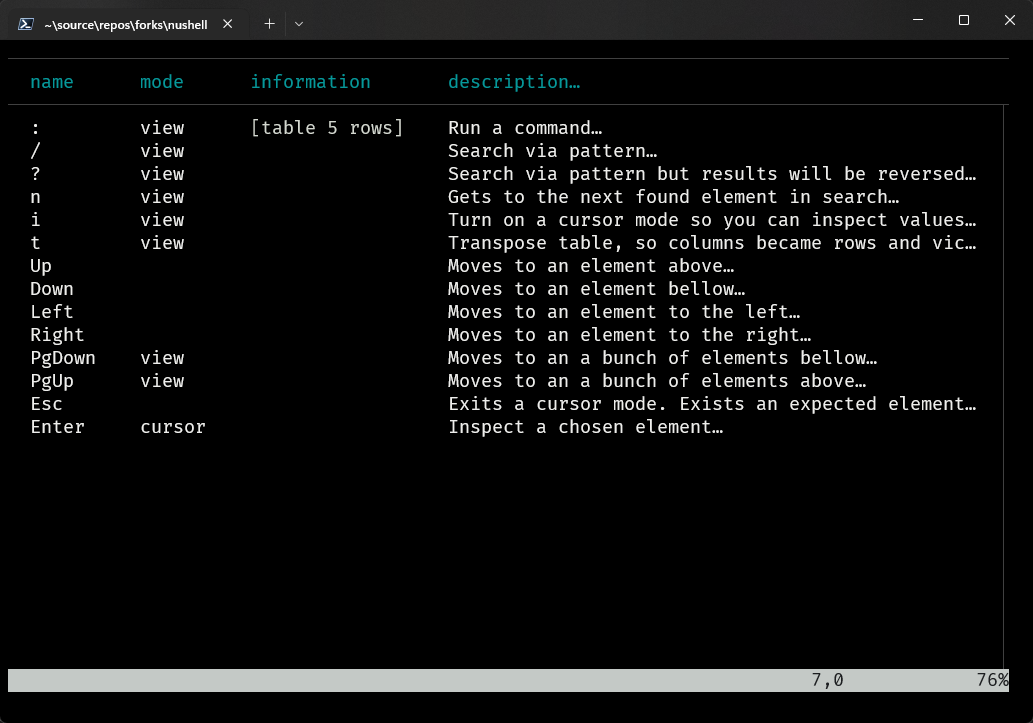
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
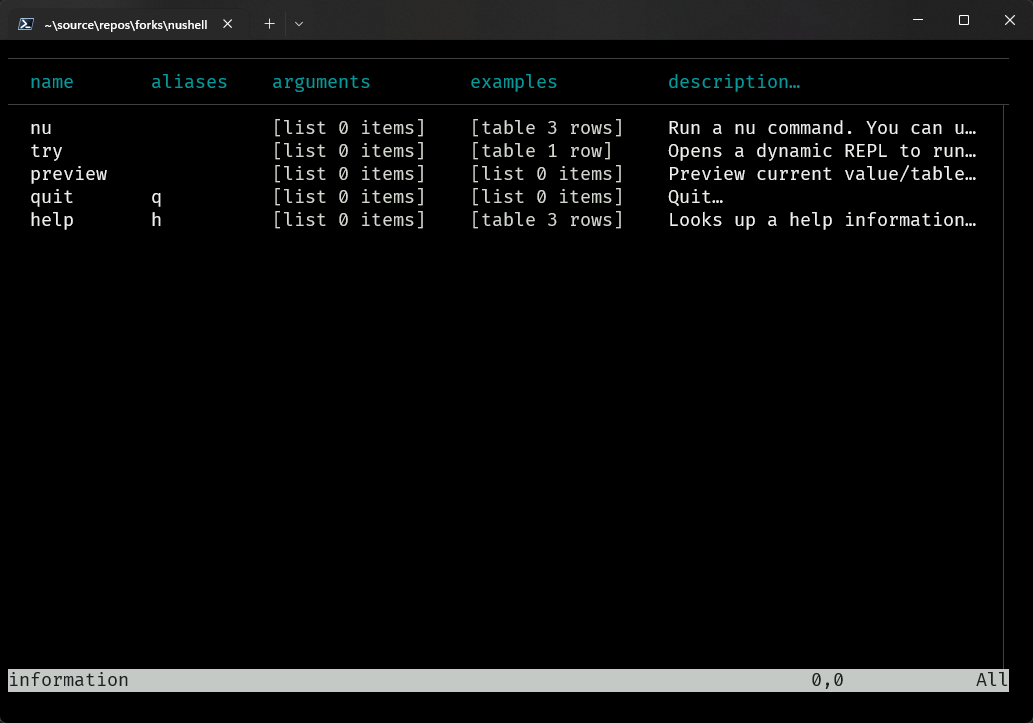
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
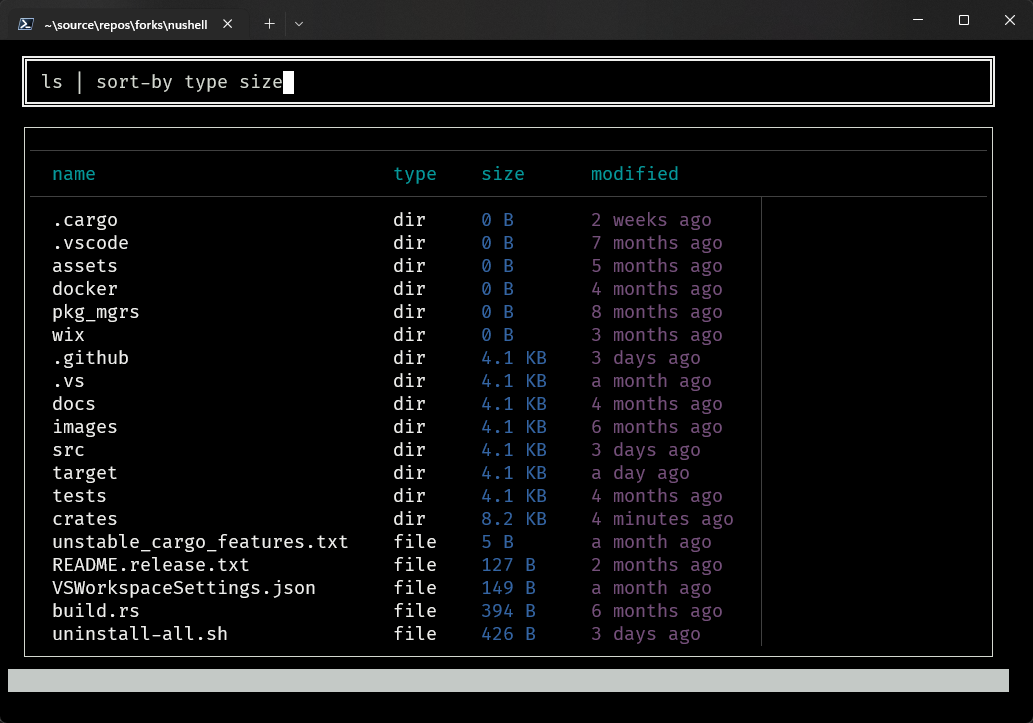
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
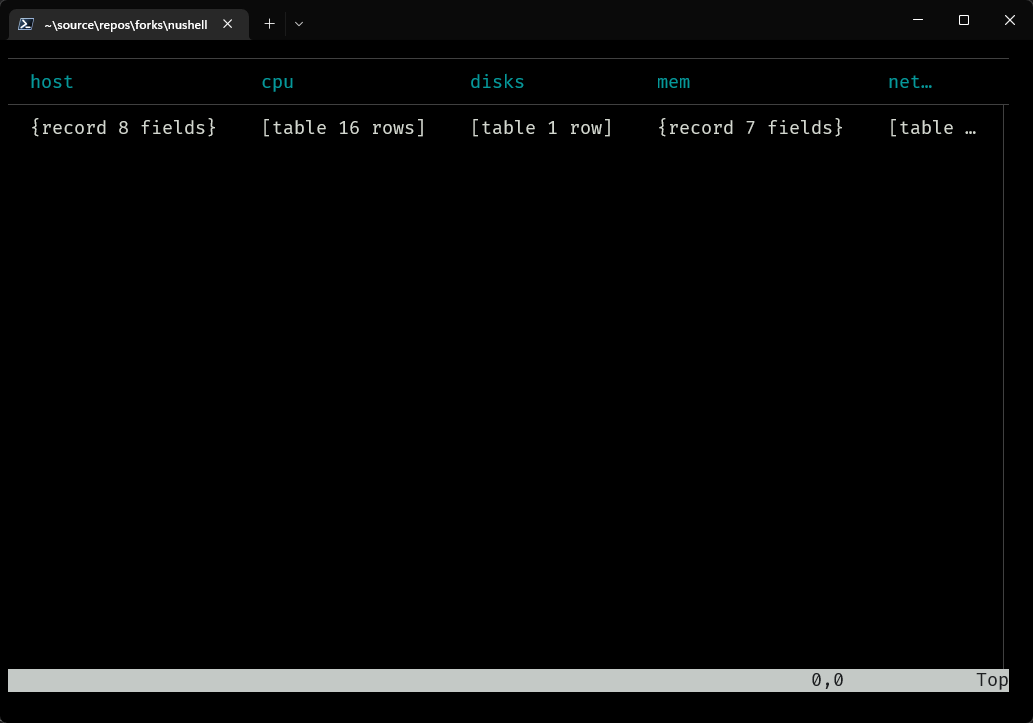
If you hit the `t` key it will now transpose the view to look like this.
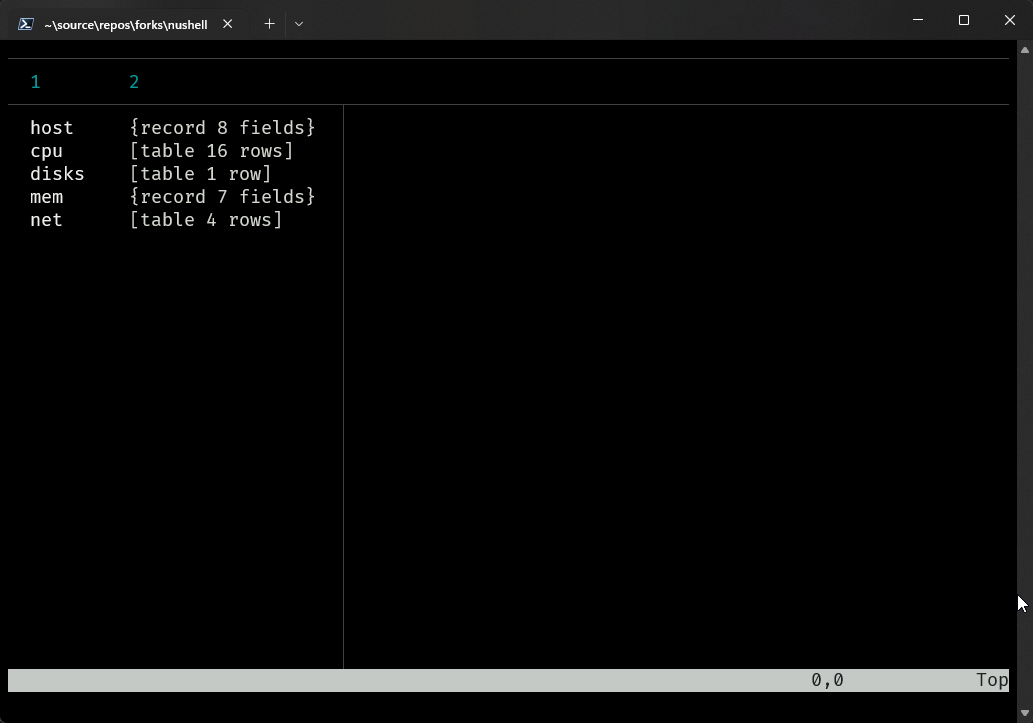
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
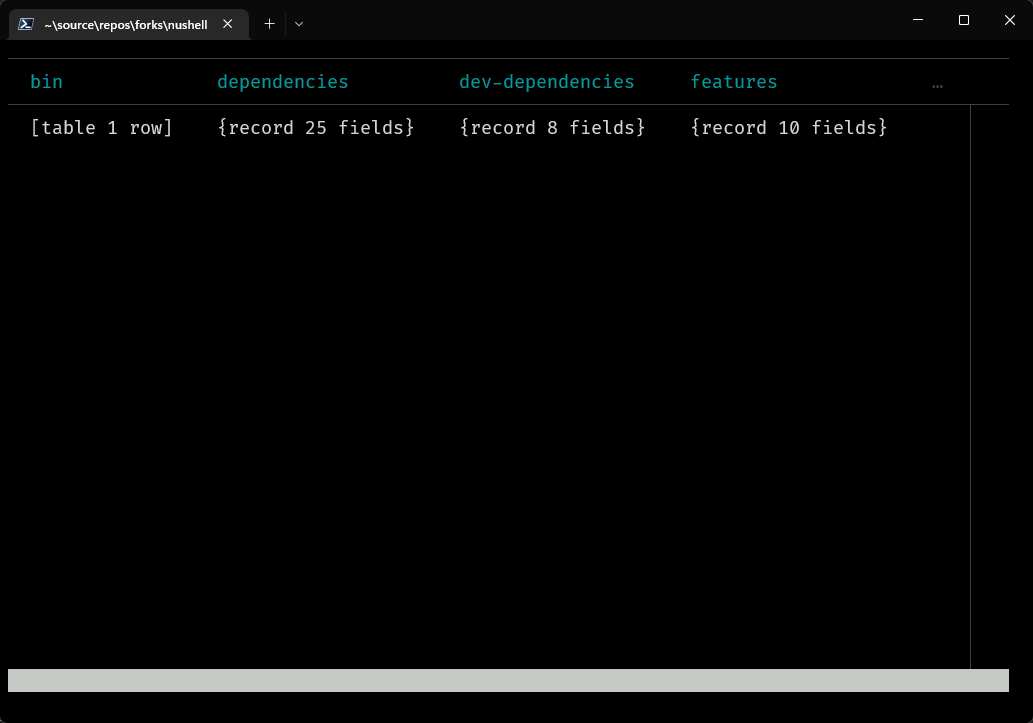
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
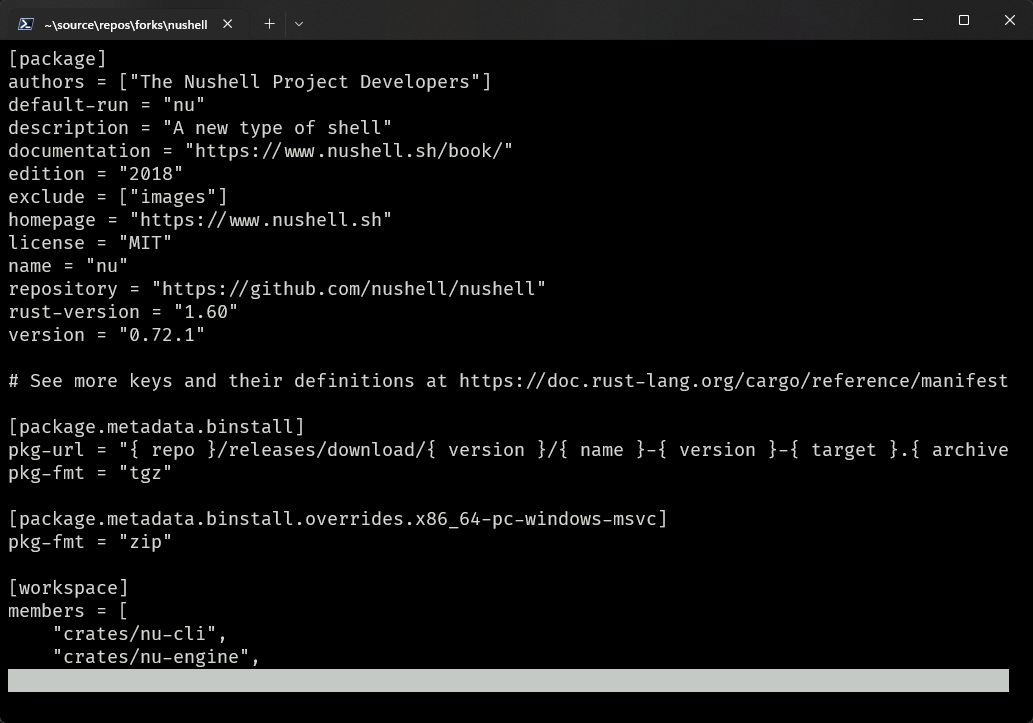
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
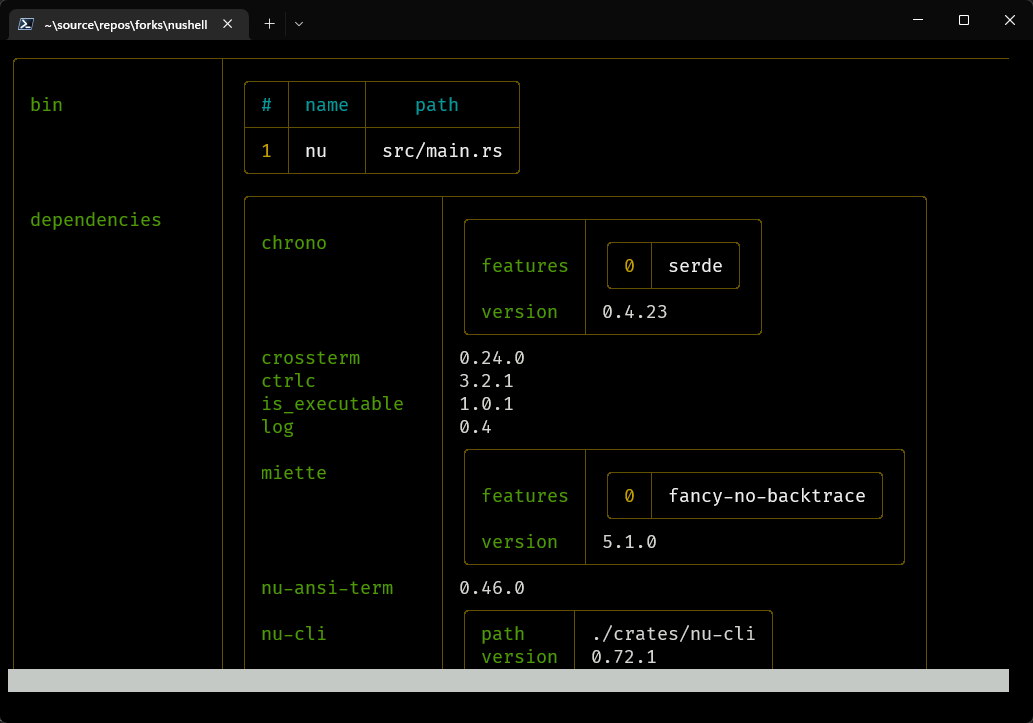
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
.switch(
|
|
|
|
"reverse",
|
2022-12-02 14:01:02 +00:00
|
|
|
"Start with the viewport scrolled to the bottom",
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
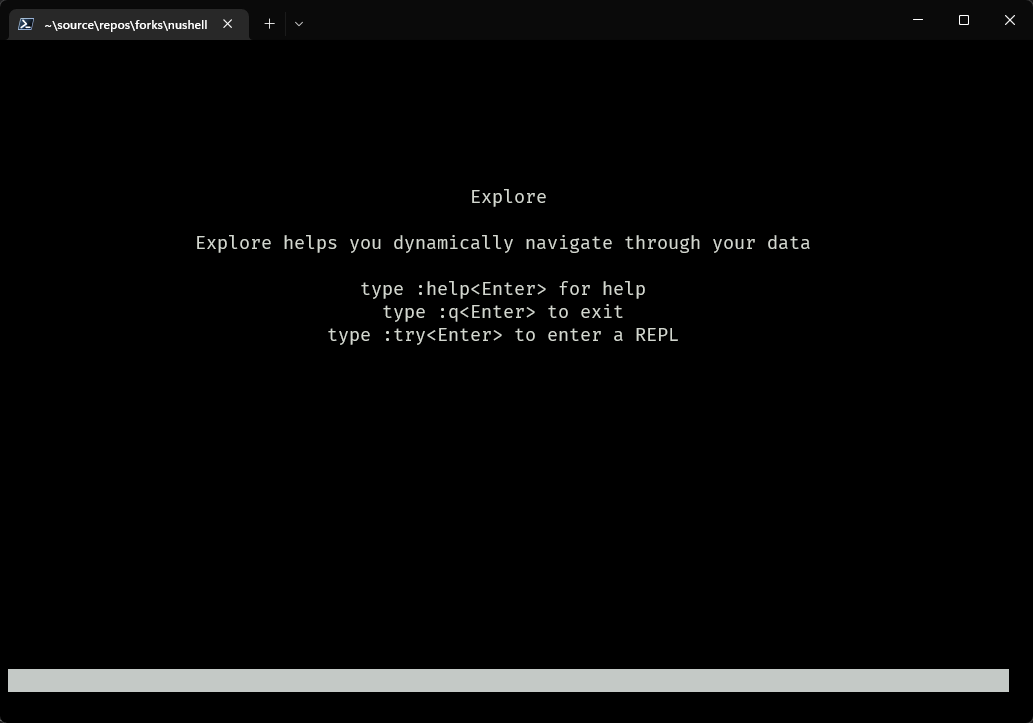
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
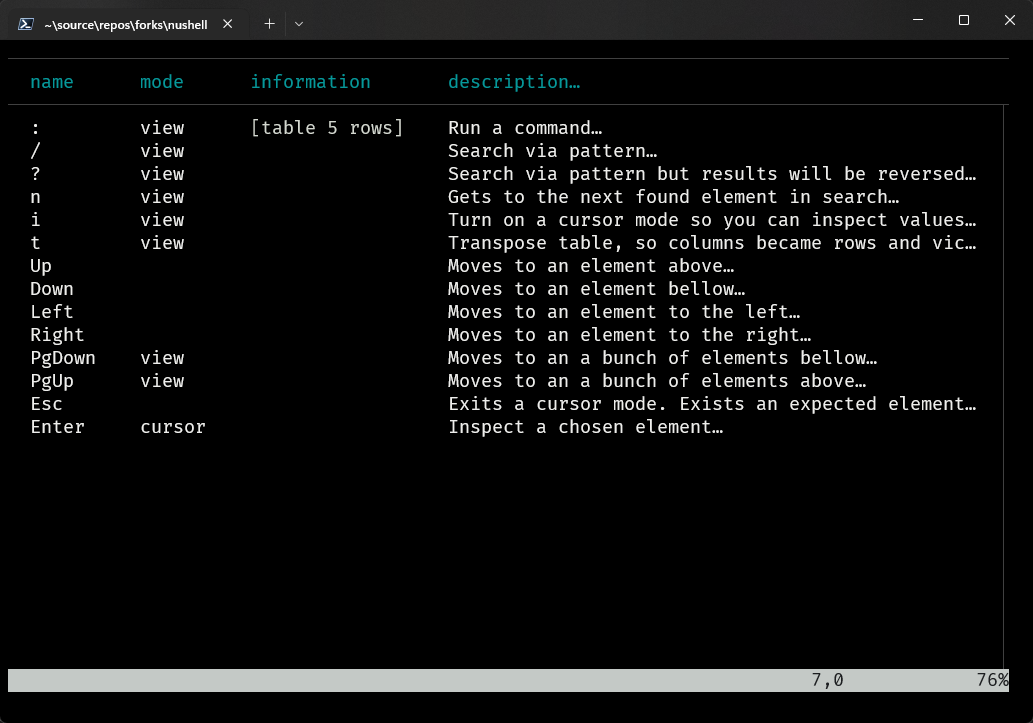
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
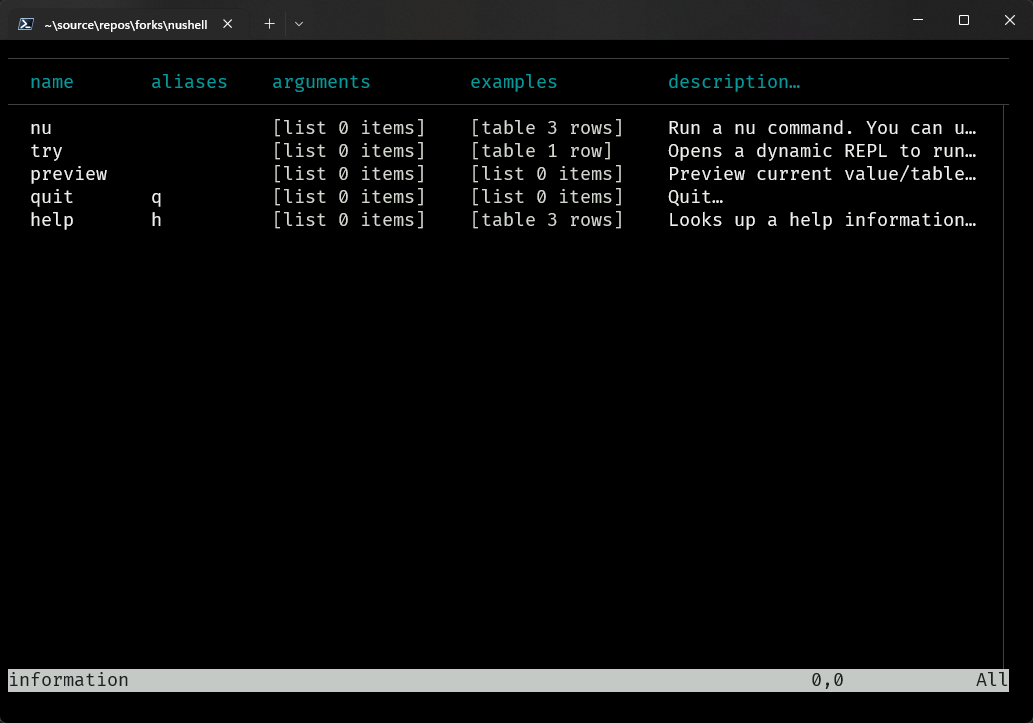
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
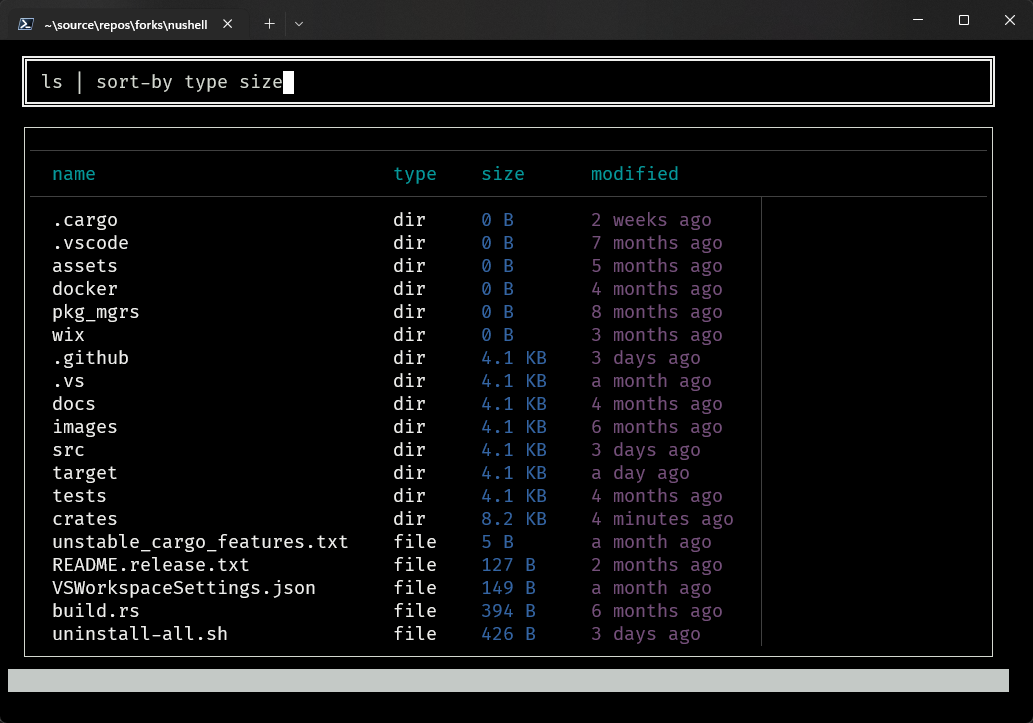
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
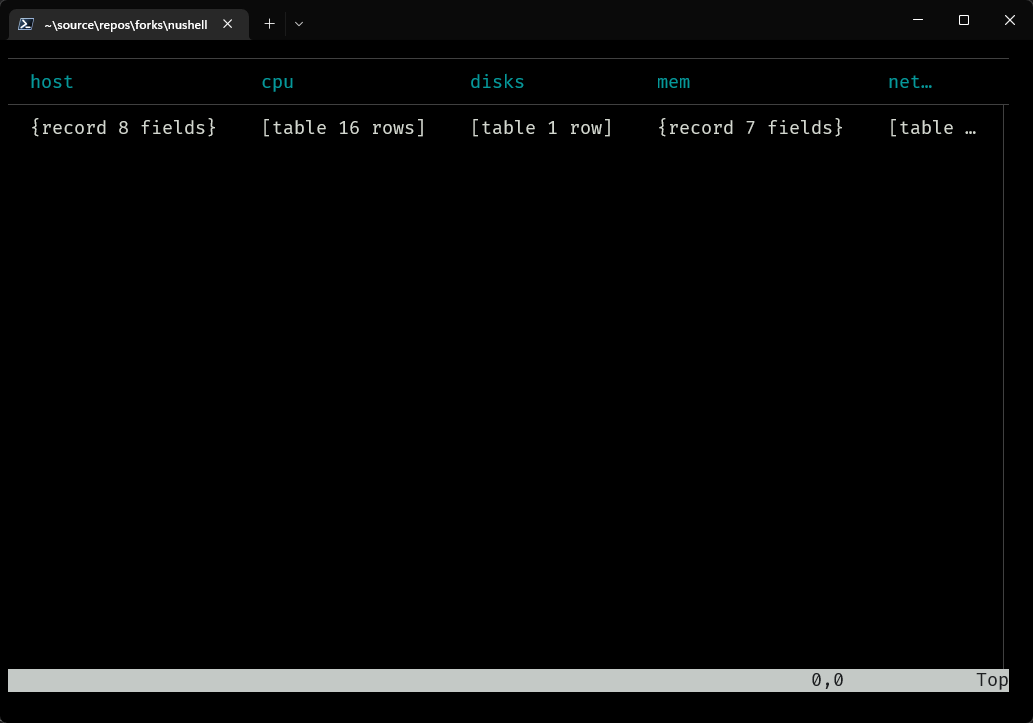
If you hit the `t` key it will now transpose the view to look like this.
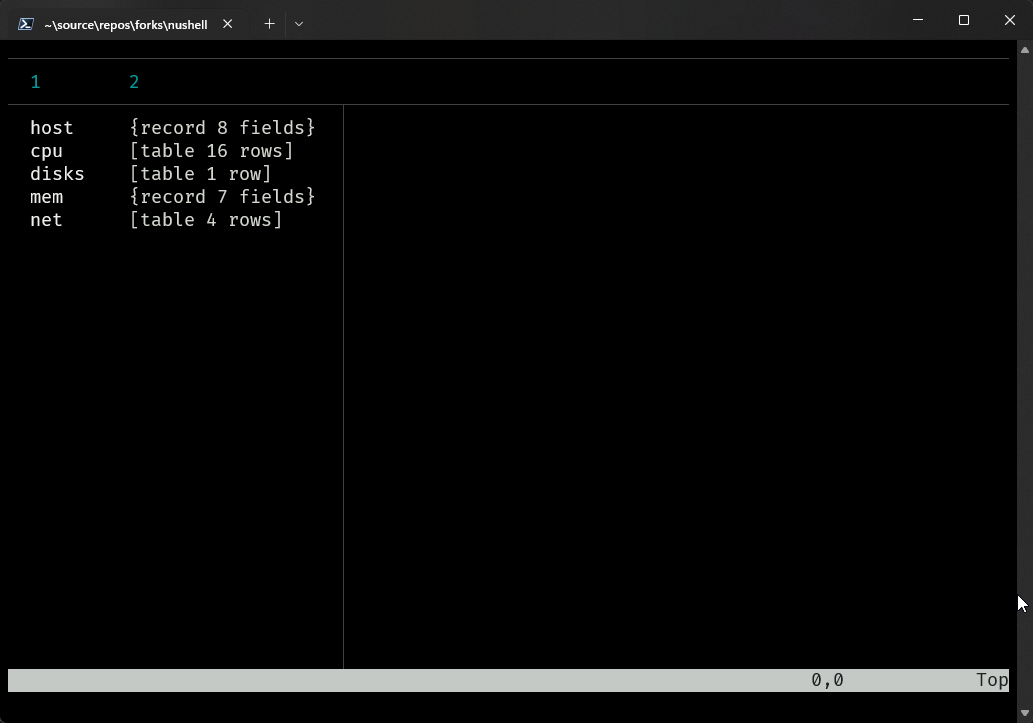
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
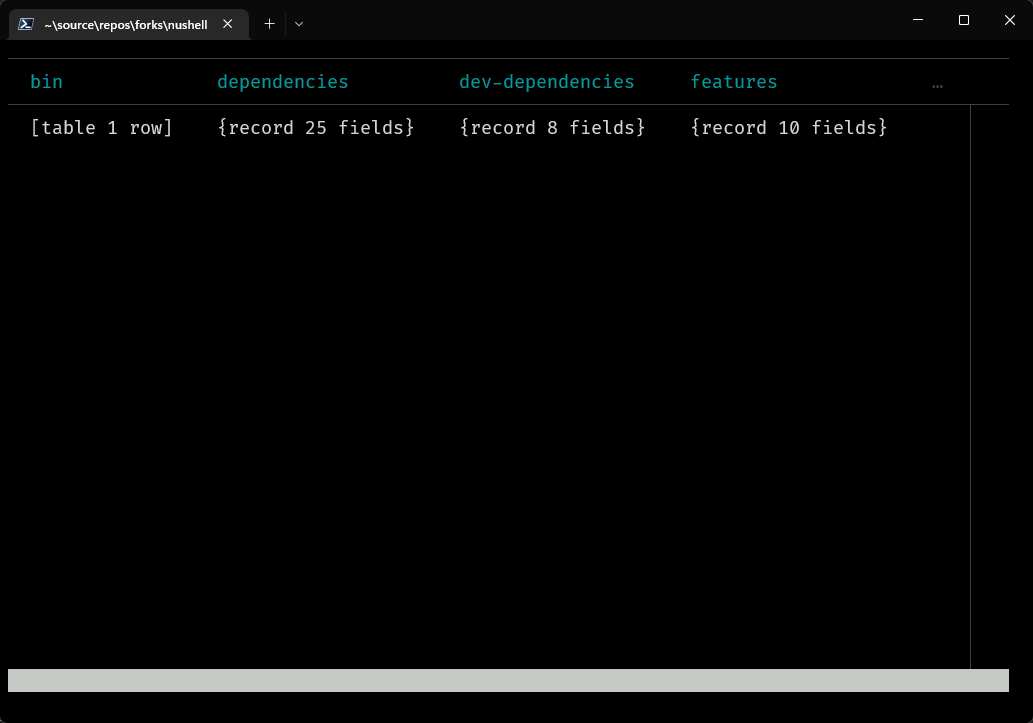
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
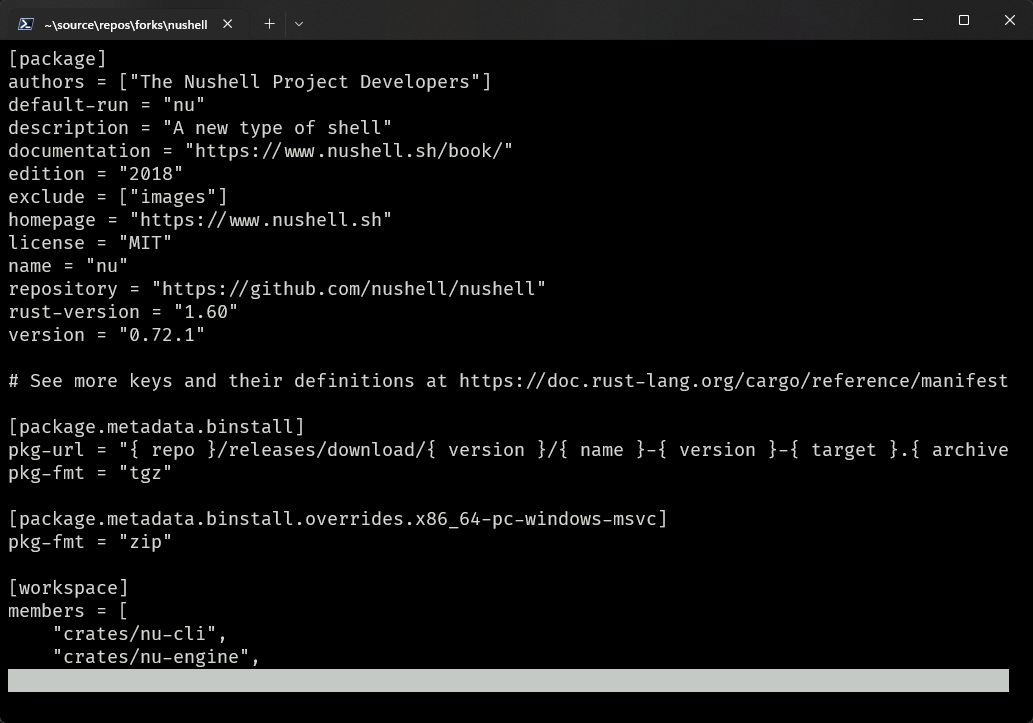
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
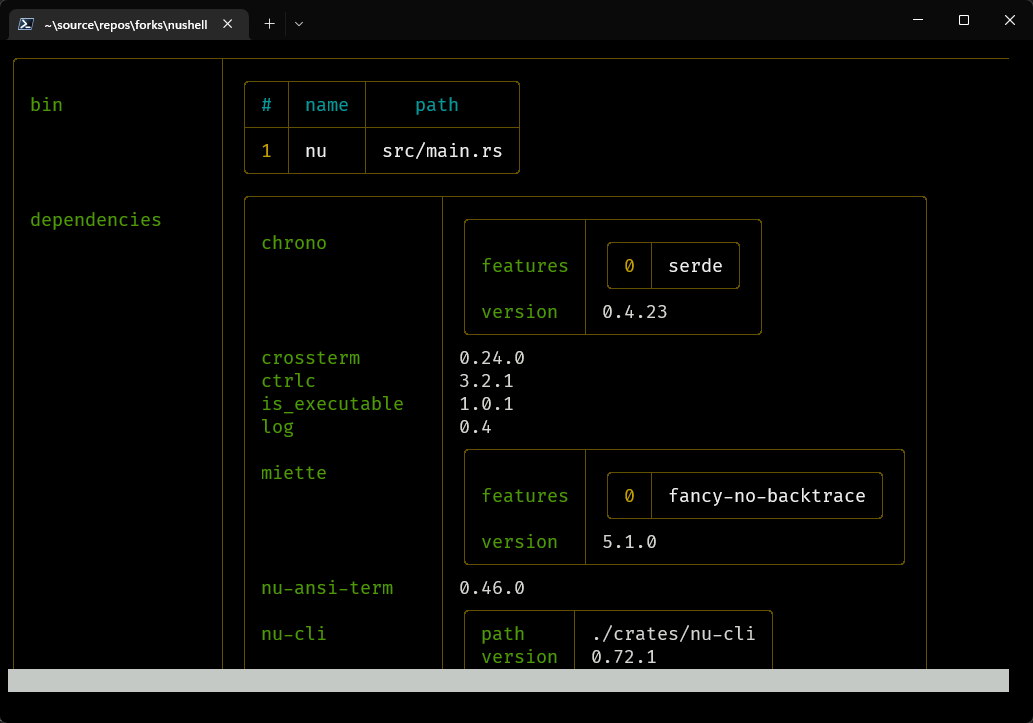
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
Some('r'),
|
|
|
|
)
|
2022-12-02 14:01:02 +00:00
|
|
|
.switch(
|
|
|
|
"peek",
|
|
|
|
"When quitting, output the value of the cell the cursor was on",
|
|
|
|
Some('p'),
|
|
|
|
)
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
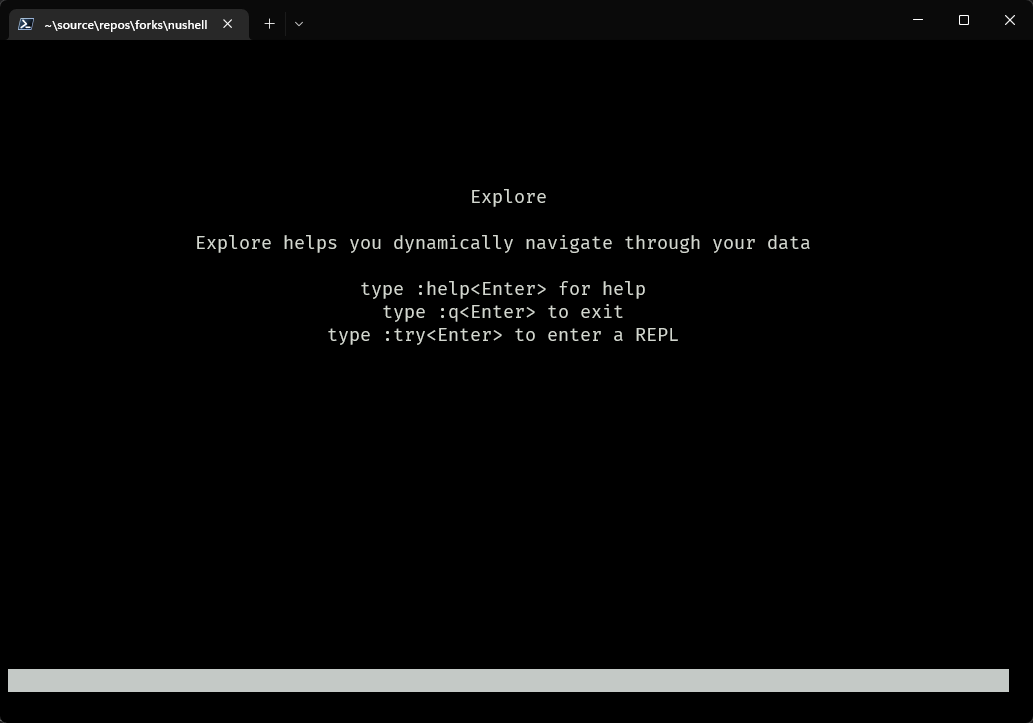
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
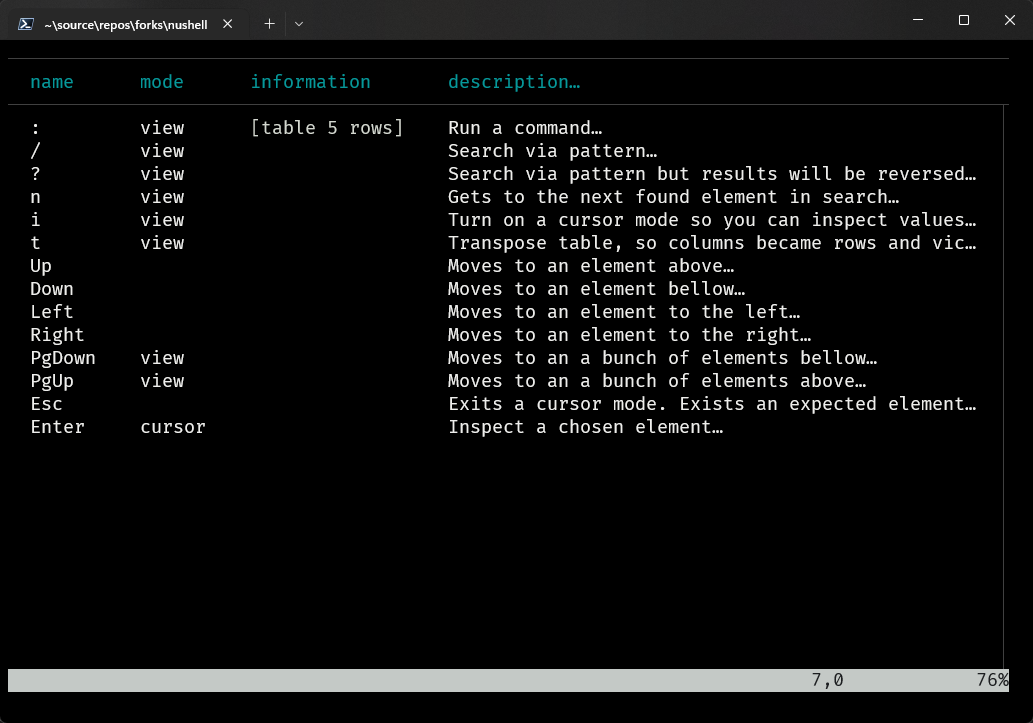
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
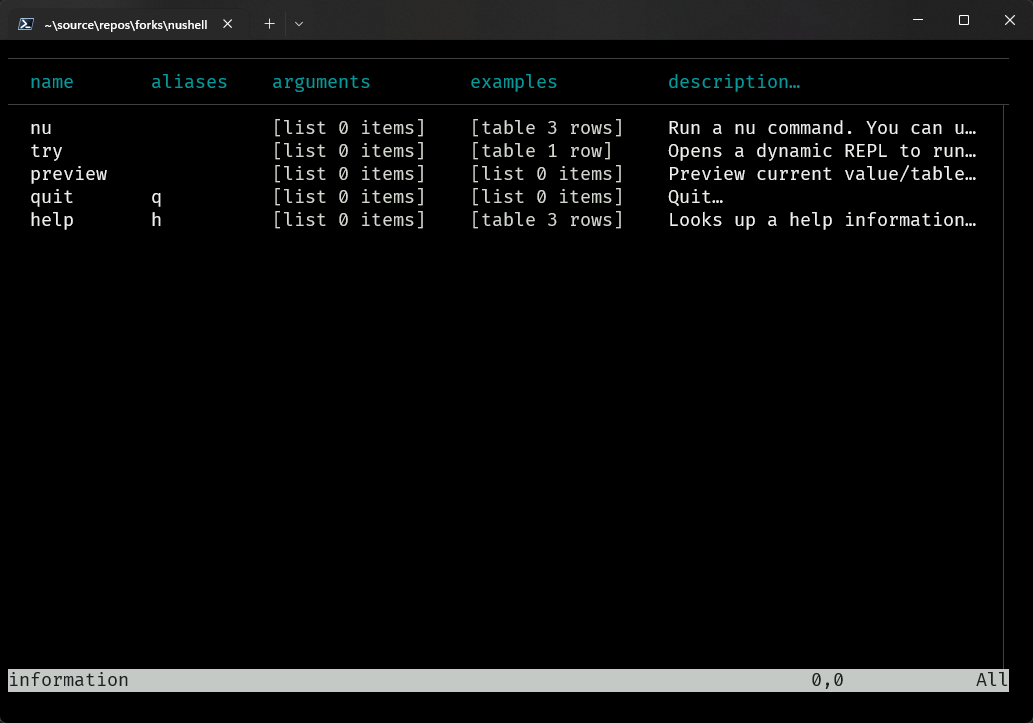
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
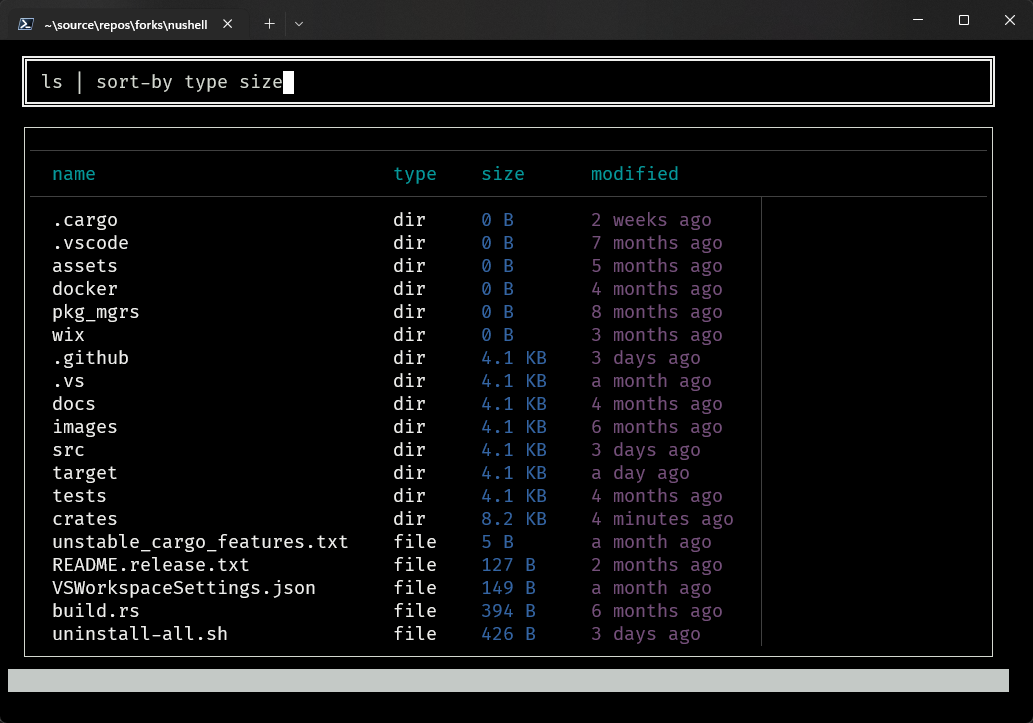
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
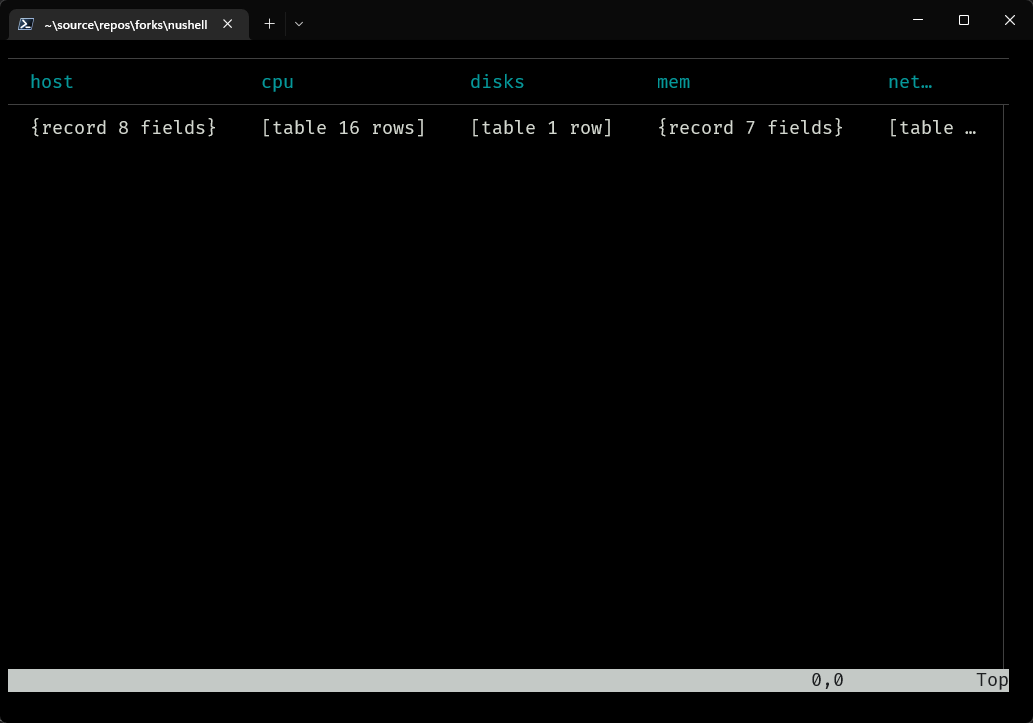
If you hit the `t` key it will now transpose the view to look like this.
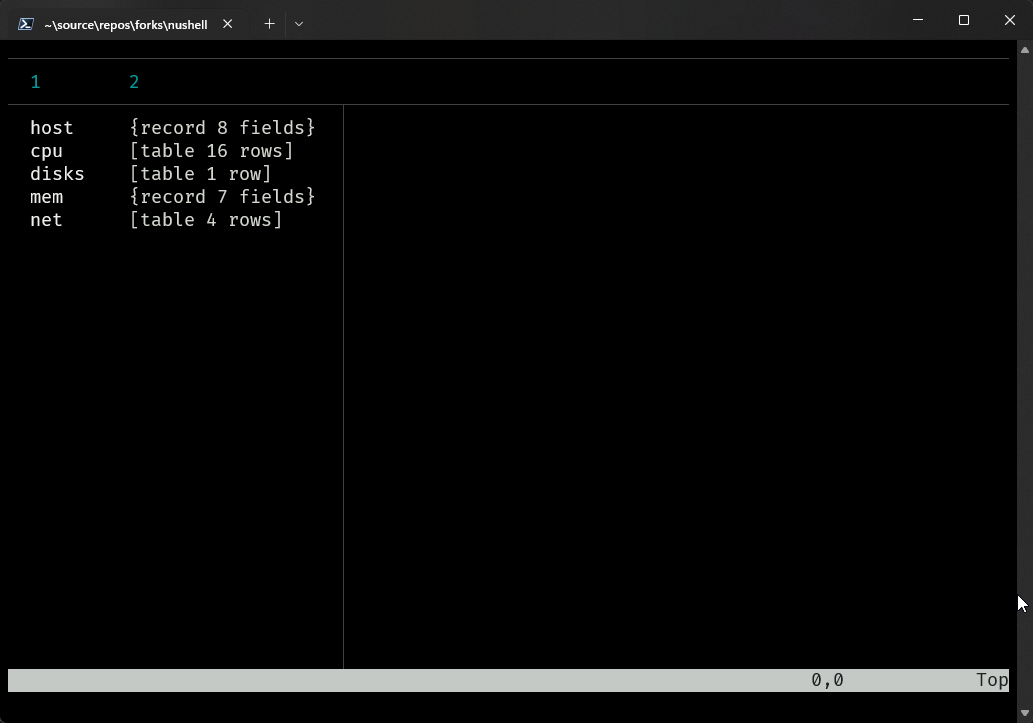
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
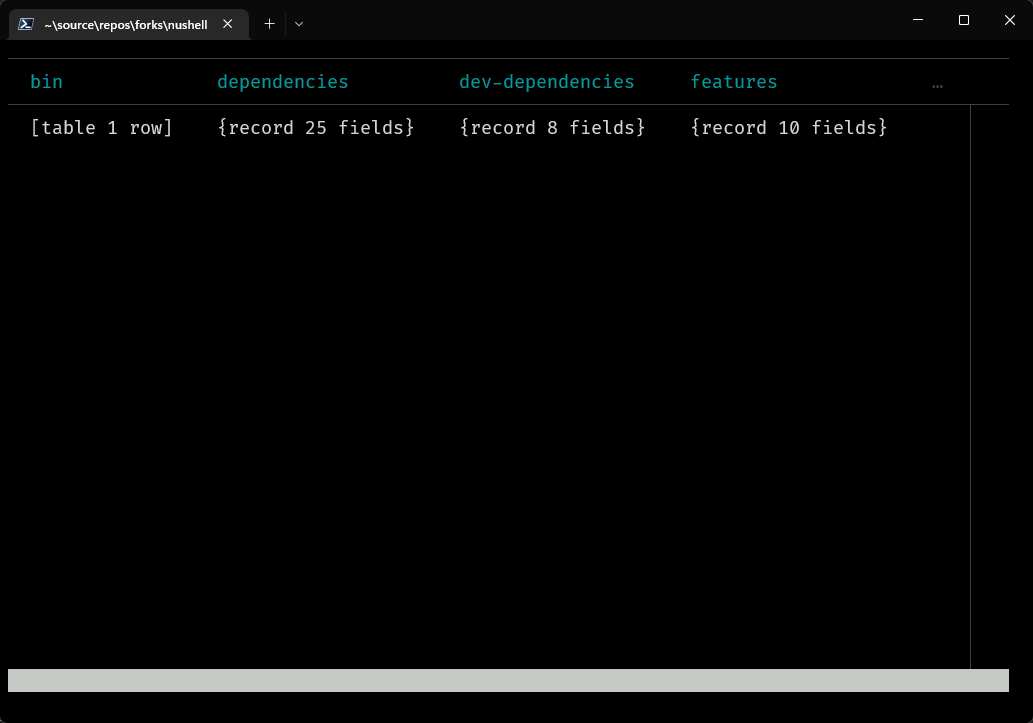
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
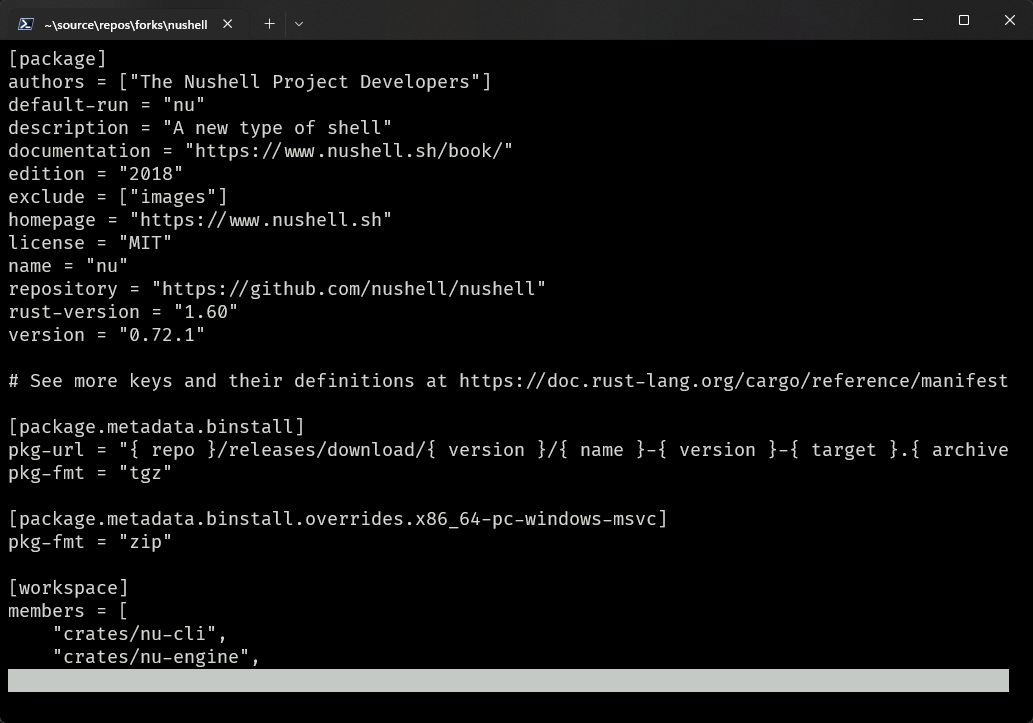
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
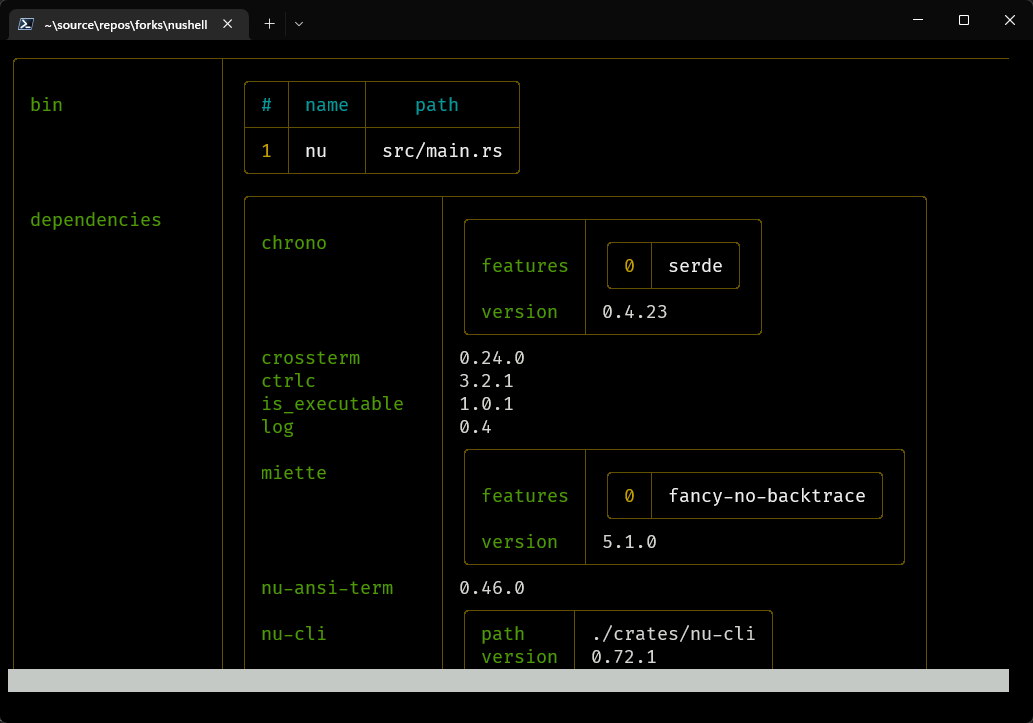
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
.category(Category::Viewers)
|
|
|
|
}
|
|
|
|
|
|
|
|
fn extra_usage(&self) -> &str {
|
2023-01-11 03:17:12 +00:00
|
|
|
r#"Press `:` then `h` to get a help menu."#
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
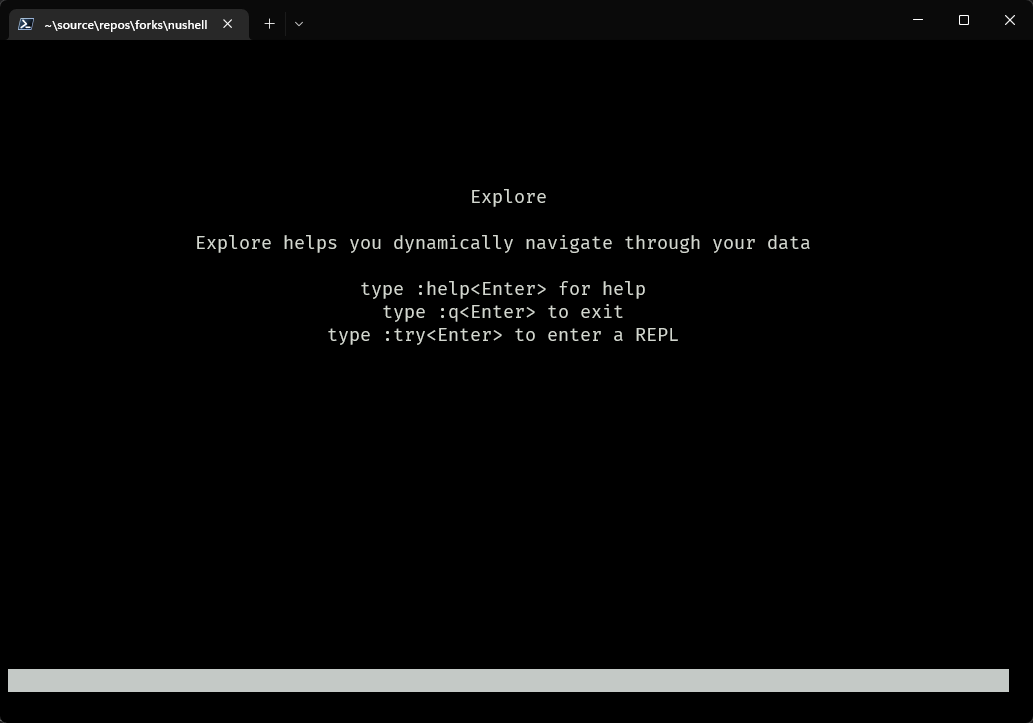
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
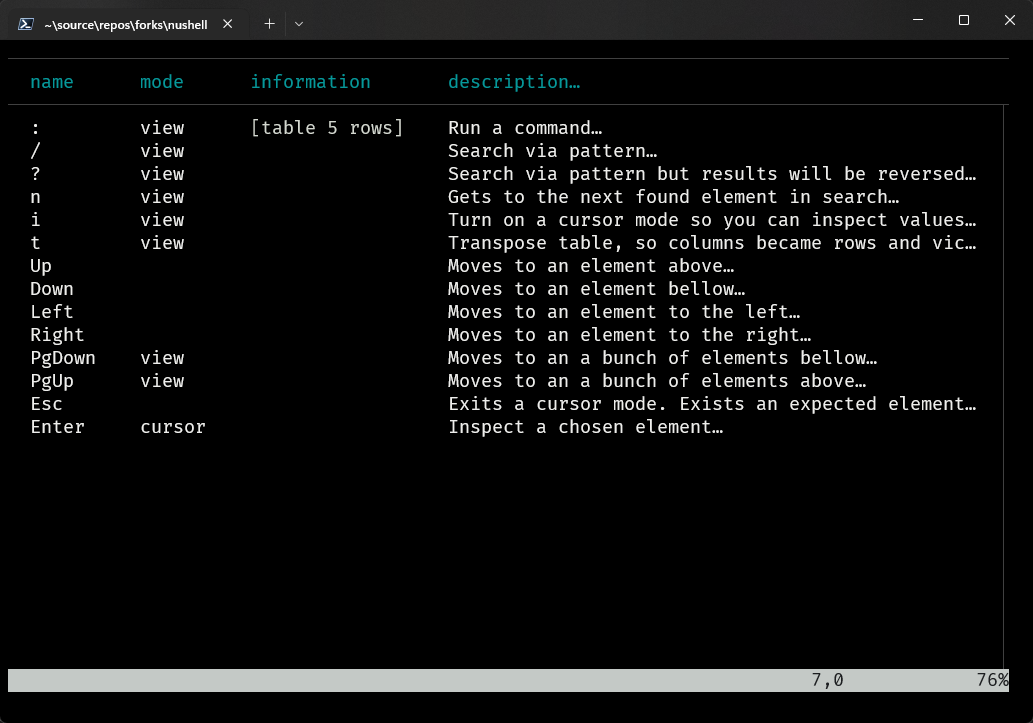
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
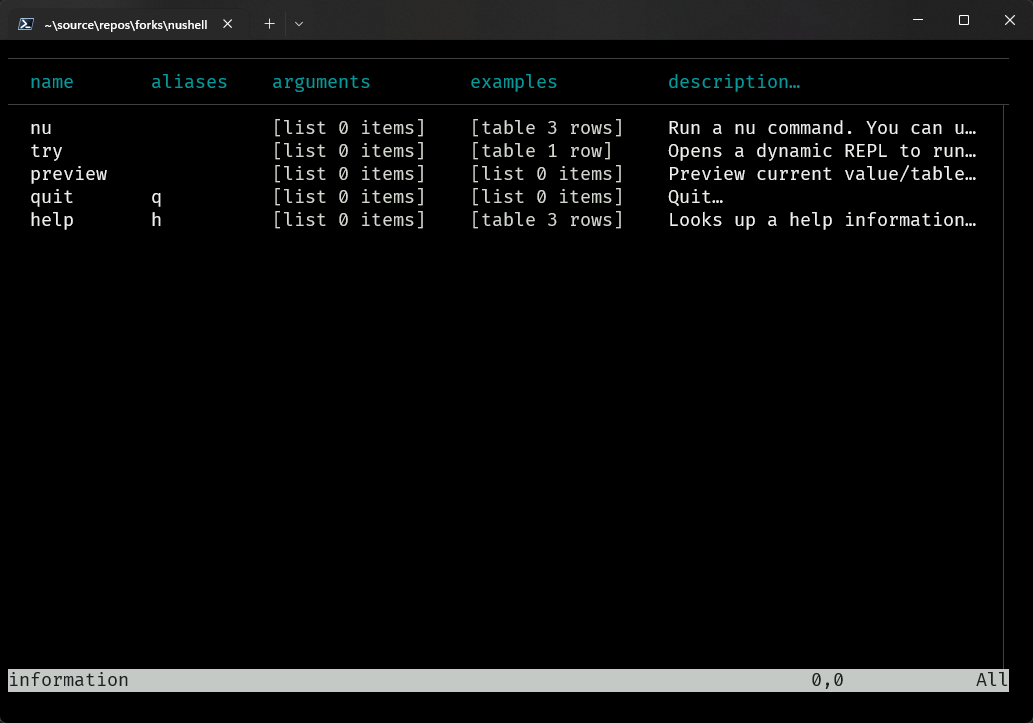
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
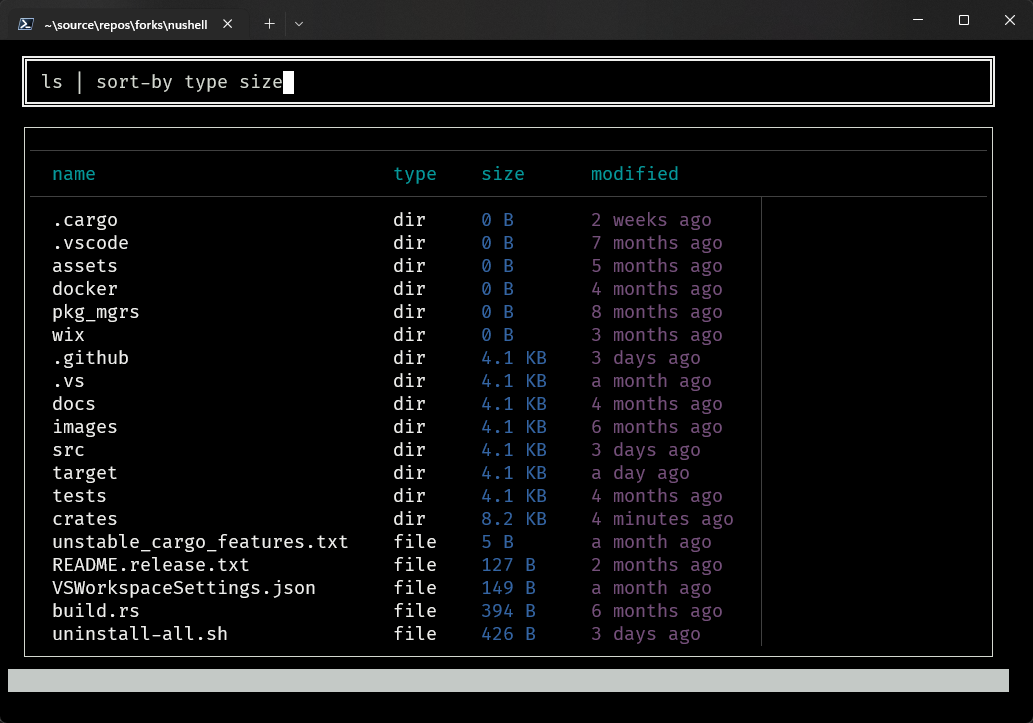
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
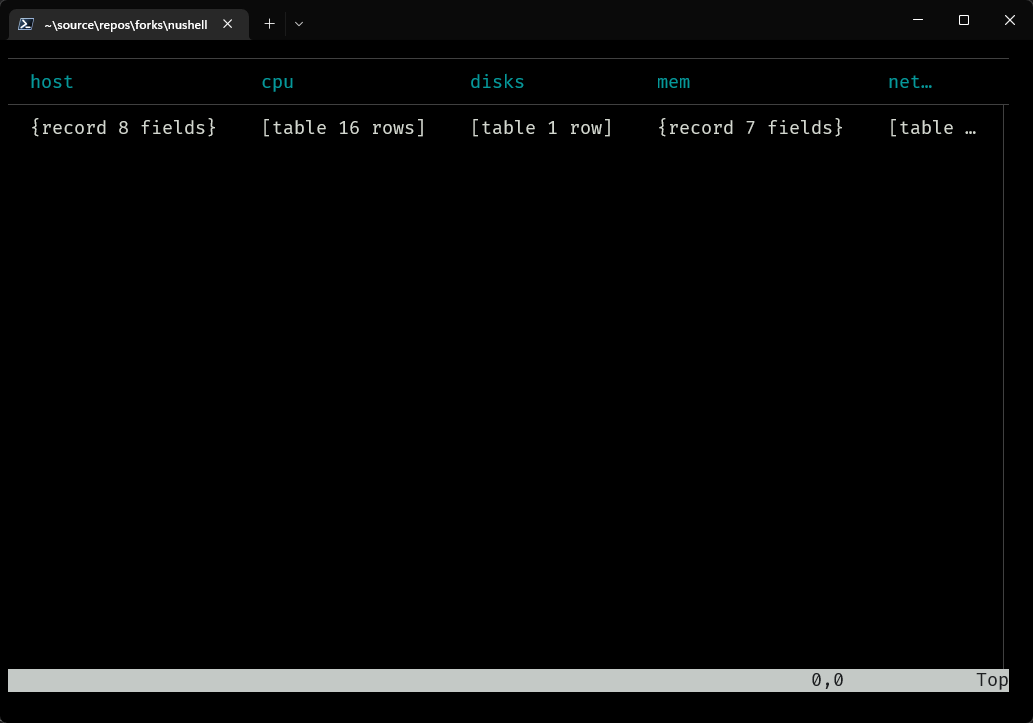
If you hit the `t` key it will now transpose the view to look like this.
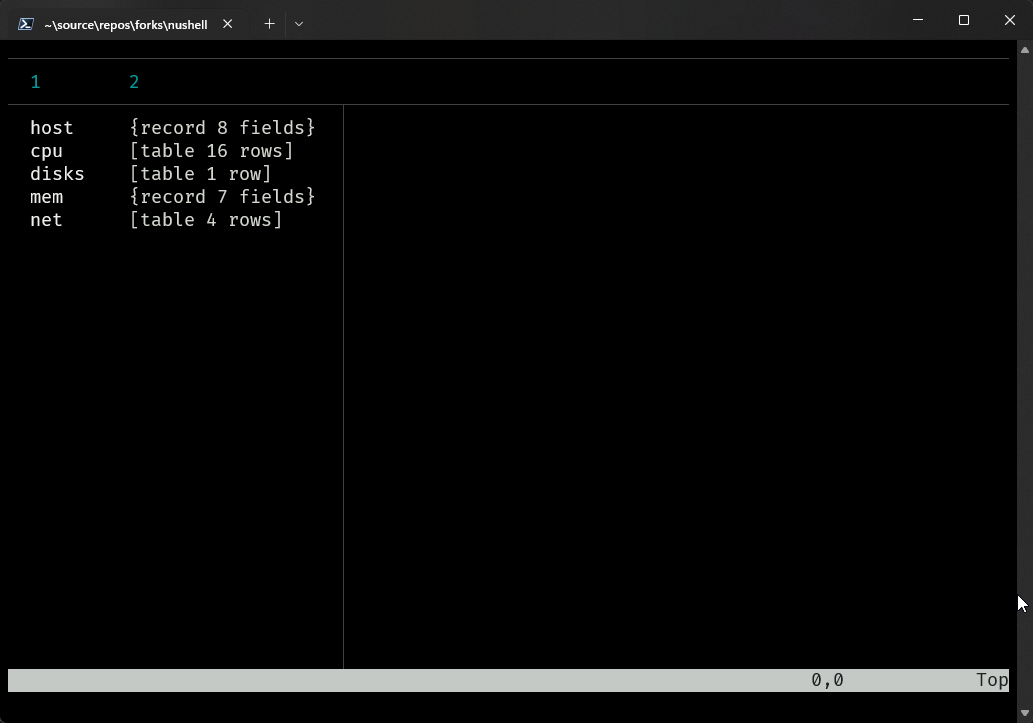
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
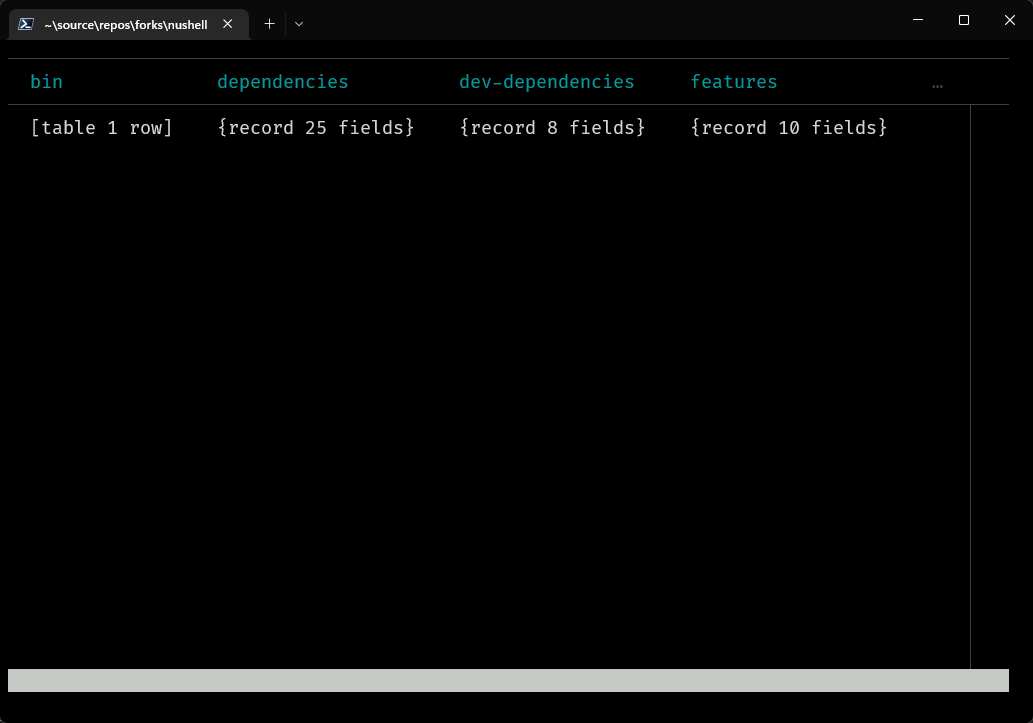
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
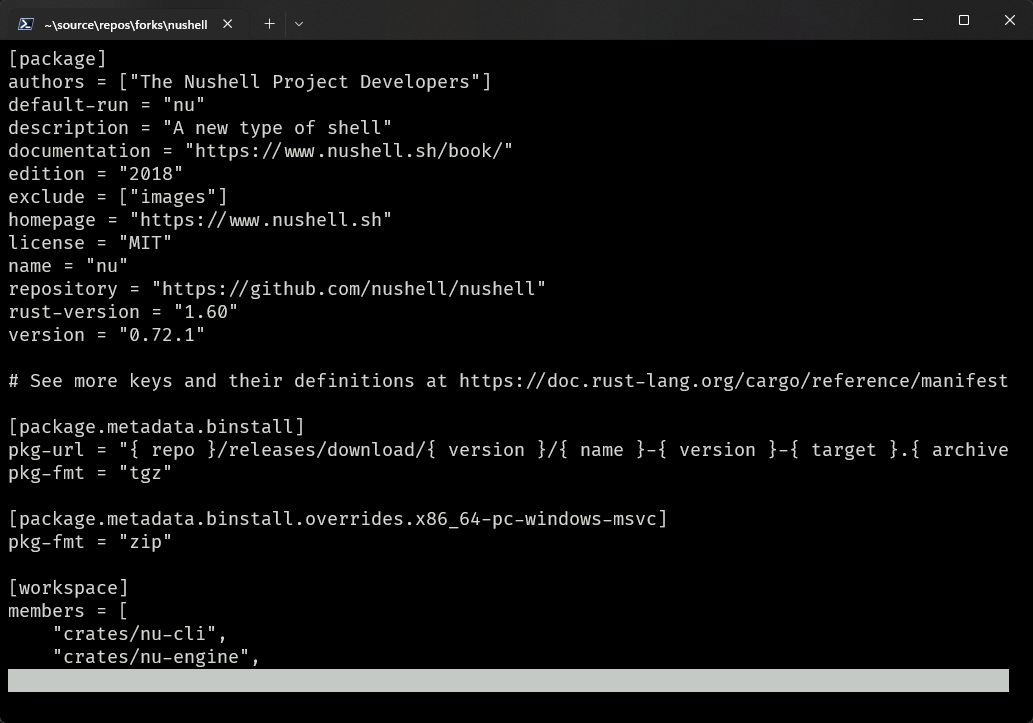
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
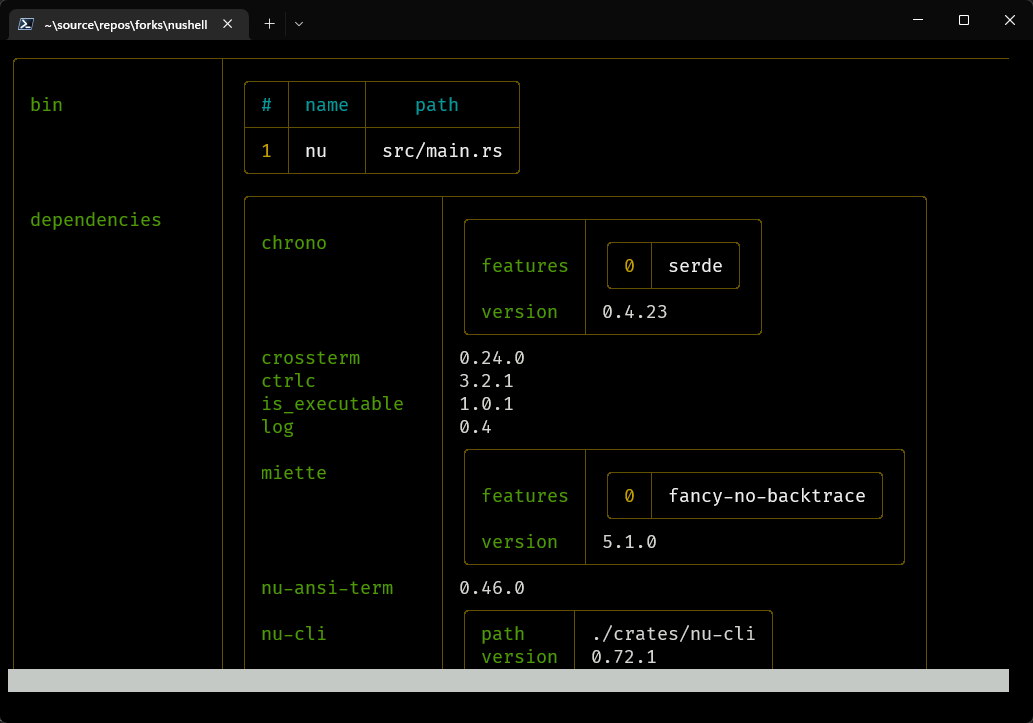
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
fn run(
|
|
|
|
&self,
|
|
|
|
engine_state: &EngineState,
|
|
|
|
stack: &mut Stack,
|
|
|
|
call: &Call,
|
|
|
|
input: PipelineData,
|
|
|
|
) -> Result<PipelineData, ShellError> {
|
|
|
|
let show_head: bool = call.get_flag(engine_state, stack, "head")?.unwrap_or(true);
|
|
|
|
let show_index: bool = call.has_flag("index");
|
|
|
|
let is_reverse: bool = call.has_flag("reverse");
|
|
|
|
let peek_value: bool = call.has_flag("peek");
|
|
|
|
|
|
|
|
let ctrlc = engine_state.ctrlc.clone();
|
2022-12-16 15:47:07 +00:00
|
|
|
let nu_config = engine_state.get_config();
|
color_config now accepts closures as color values (#7141)
# Description
Closes #6909. You can now add closures to your `color_config` themes.
Whenever a value would be printed with `table`, the closure is run with
the value piped-in. The closure must return either a {fg,bg,attr} record
or a color name (`'light_red'` etc.). This returned style is used to
colour the value.
This is entirely backwards-compatible with existing config.nu files.
Example code excerpt:
```
let my_theme = {
header: green_bold
bool: { if $in { 'light_cyan' } else { 'light_red' } }
int: purple_bold
filesize: { |e| if $e == 0b { 'gray' } else if $e < 1mb { 'purple_bold' } else { 'cyan_bold' } }
duration: purple_bold
date: { (date now) - $in | if $in > 1wk { 'cyan_bold' } else if $in > 1day { 'green_bold' } else { 'yellow_bold' } }
range: yellow_bold
string: { if $in =~ '^#\w{6}$' { $in } else { 'white' } }
nothing: white
```
Example output with this in effect:
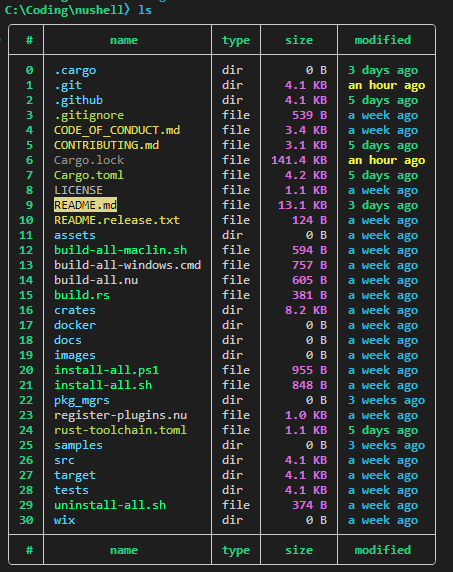
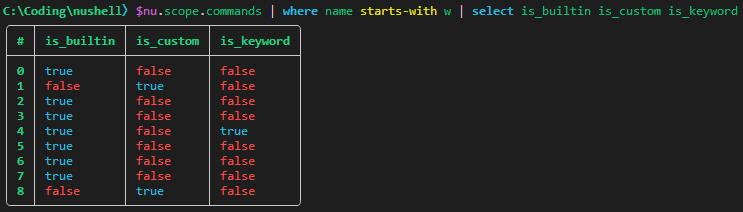
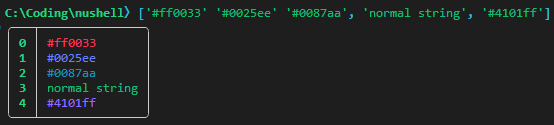
Slightly important notes:
* Some color_config names, namely "separator", "empty" and "hints", pipe
in `null` instead of a value.
* Currently, doing anything non-trivial inside a closure has an
understandably big perf hit. I currently do not actually recommend
something like `string: { if $in =~ '^#\w{6}$' { $in } else { 'white' }
}` for serious work, mainly because of the abundance of string-type data
in the world. Nevertheless, lesser-used types like "date" and "duration"
work well with this.
* I had to do some reorganisation in order to make it possible to call
`eval_block()` that late in table rendering. I invented a new struct
called "StyleComputer" which holds the engine_state and stack of the
initial `table` command (implicit or explicit).
* StyleComputer has a `compute()` method which takes a color_config name
and a nu value, and always returns the correct Style, so you don't have
to worry about A) the color_config value was set at all, B) whether it
was set to a closure or not, or C) which default style to use in those
cases.
* Currently, errors encountered during execution of the closures are
thrown in the garbage. Any other ideas are welcome. (Nonetheless, errors
result in a huge perf hit when they are encountered. I think what should
be done is to assume something terrible happened to the user's config
and invalidate the StyleComputer for that `table` run, thus causing
subsequent output to just be Style::default().)
* More thorough tests are forthcoming - ran into some difficulty using
`nu!` to take an alternative config, and for some reason `let-env config
=` statements don't seem to work inside `nu!` pipelines(???)
* The default config.nu has not been updated to make use of this yet. Do
tell if you think I should incorporate that into this.
# User-Facing Changes
See above.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace --features=extra -- -D warnings -D
clippy::unwrap_used -A clippy::needless_collect` to check that you're
using the standard code style
- `cargo test --workspace --features=extra` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2022-12-17 13:07:56 +00:00
|
|
|
let style_computer = StyleComputer::from_config(engine_state, stack);
|
2022-12-16 15:47:07 +00:00
|
|
|
|
|
|
|
let mut config = nu_config.explore.clone();
|
2022-12-18 14:43:15 +00:00
|
|
|
include_nu_config(&mut config, &style_computer);
|
2022-12-19 17:34:21 +00:00
|
|
|
update_config(&mut config, show_index, show_head);
|
|
|
|
prepare_default_config(&mut config);
|
2022-12-16 15:47:07 +00:00
|
|
|
|
|
|
|
let show_banner = is_need_banner(&config).unwrap_or(true);
|
2022-12-17 20:05:41 +00:00
|
|
|
let exit_esc = is_need_esc_exit(&config).unwrap_or(true);
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
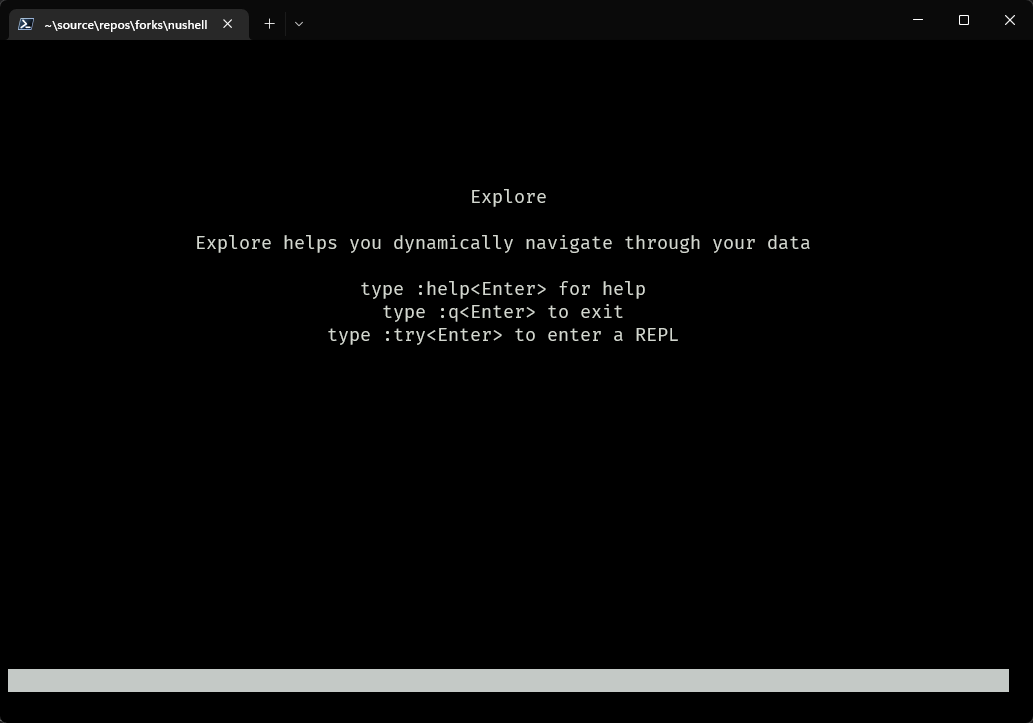
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
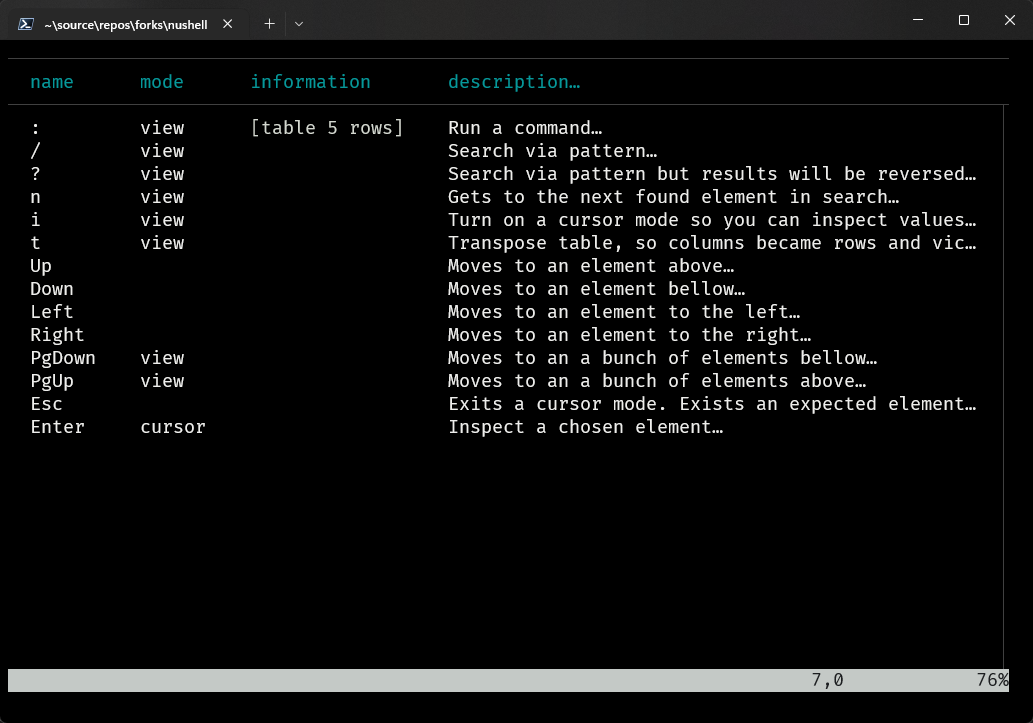
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
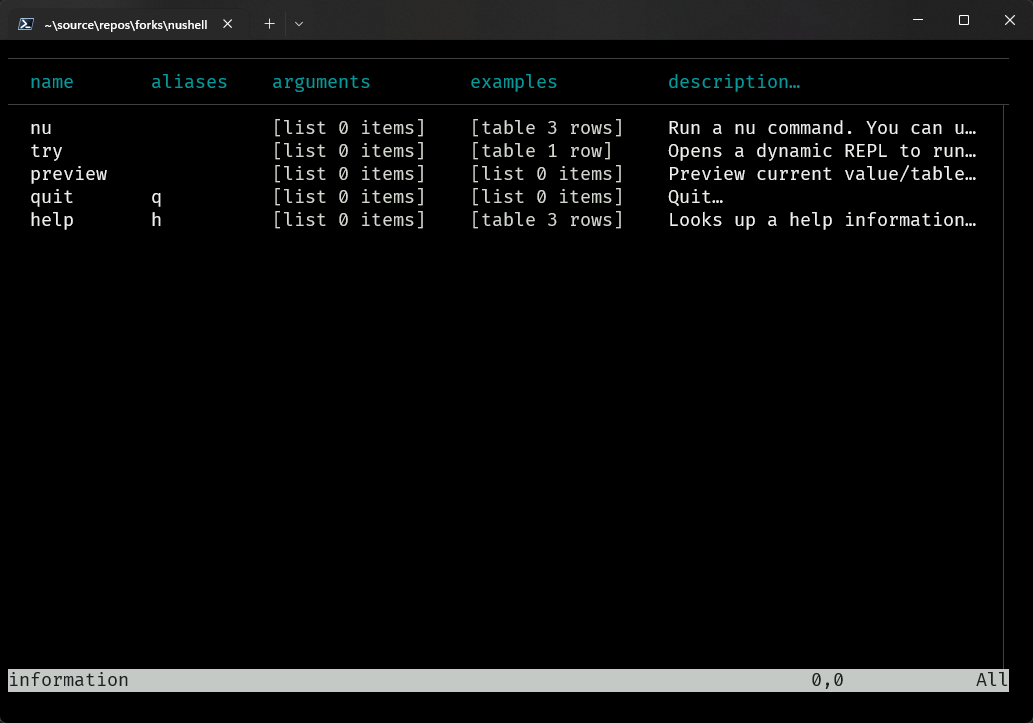
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
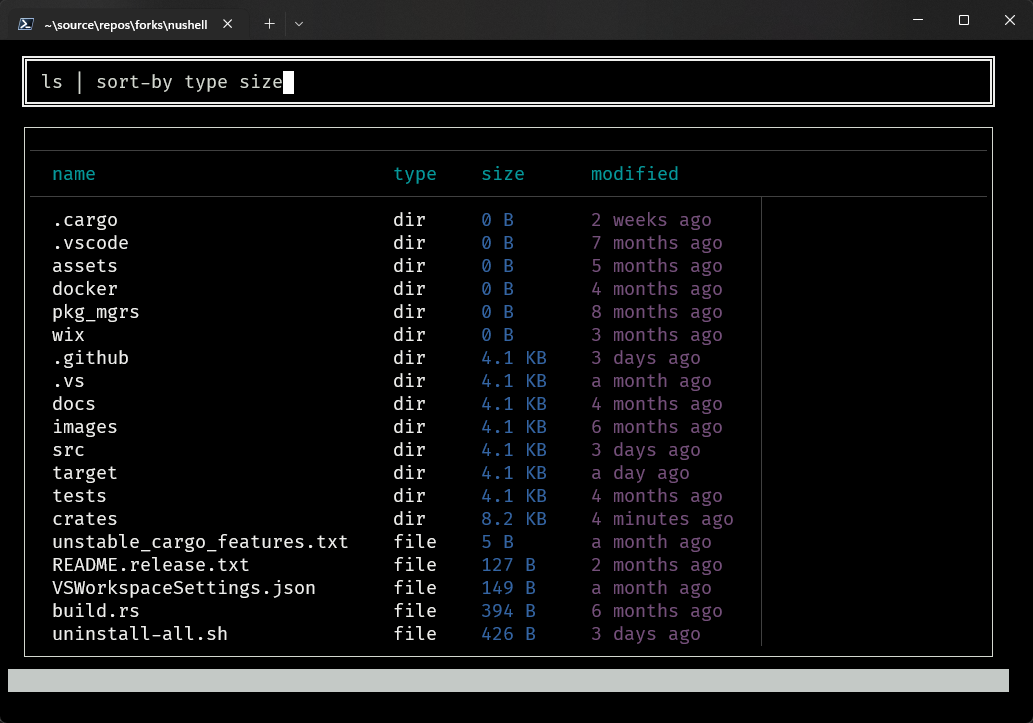
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
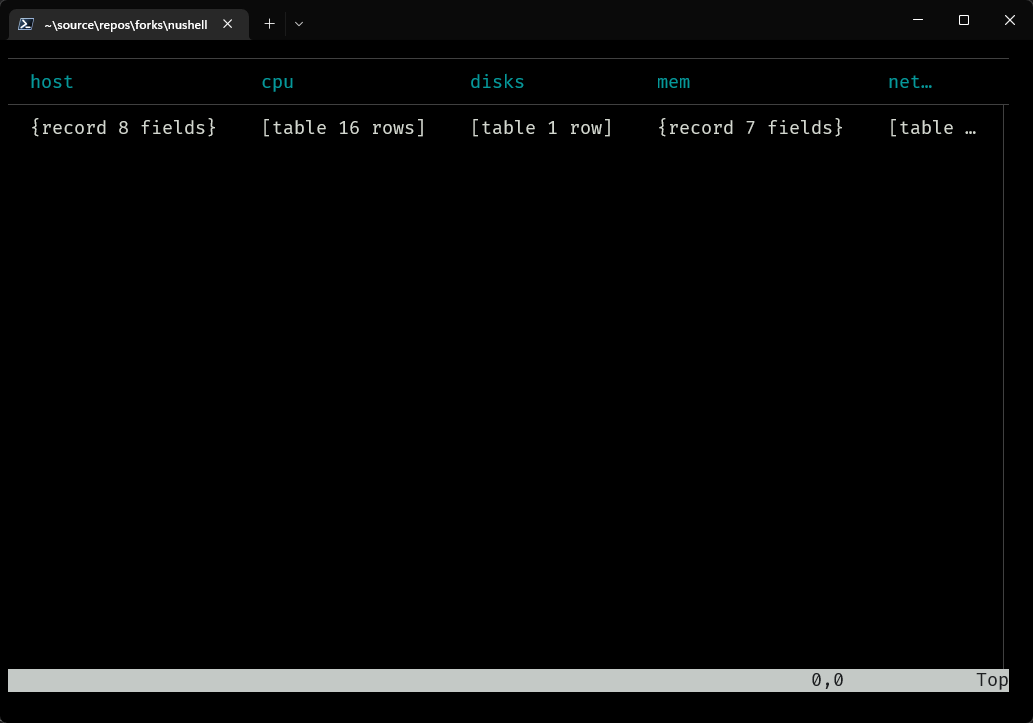
If you hit the `t` key it will now transpose the view to look like this.
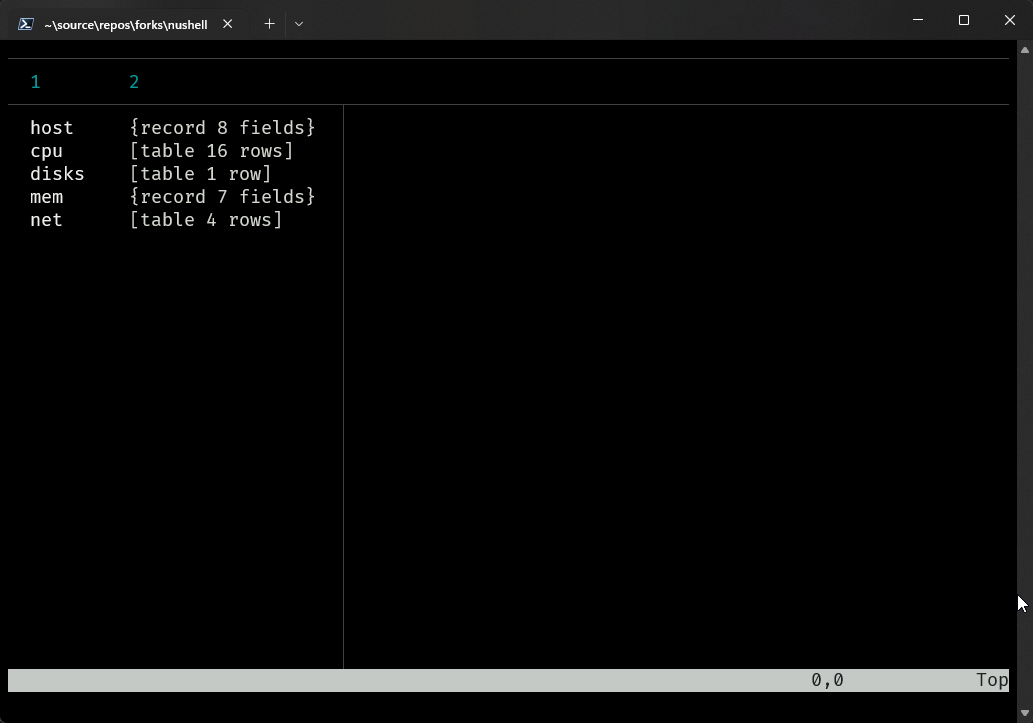
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
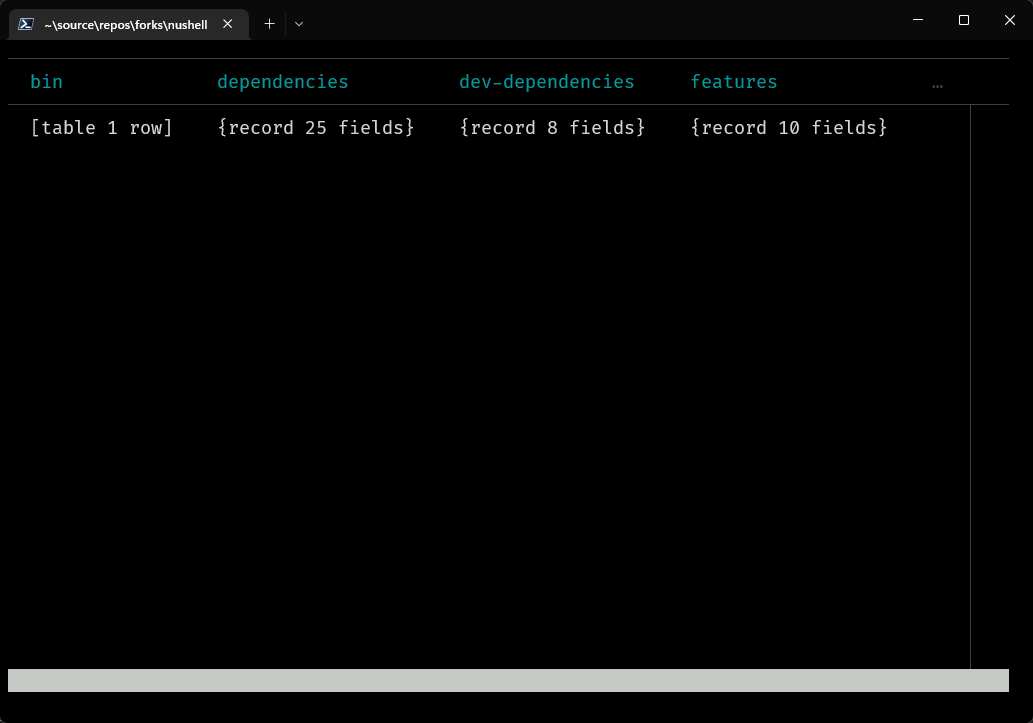
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
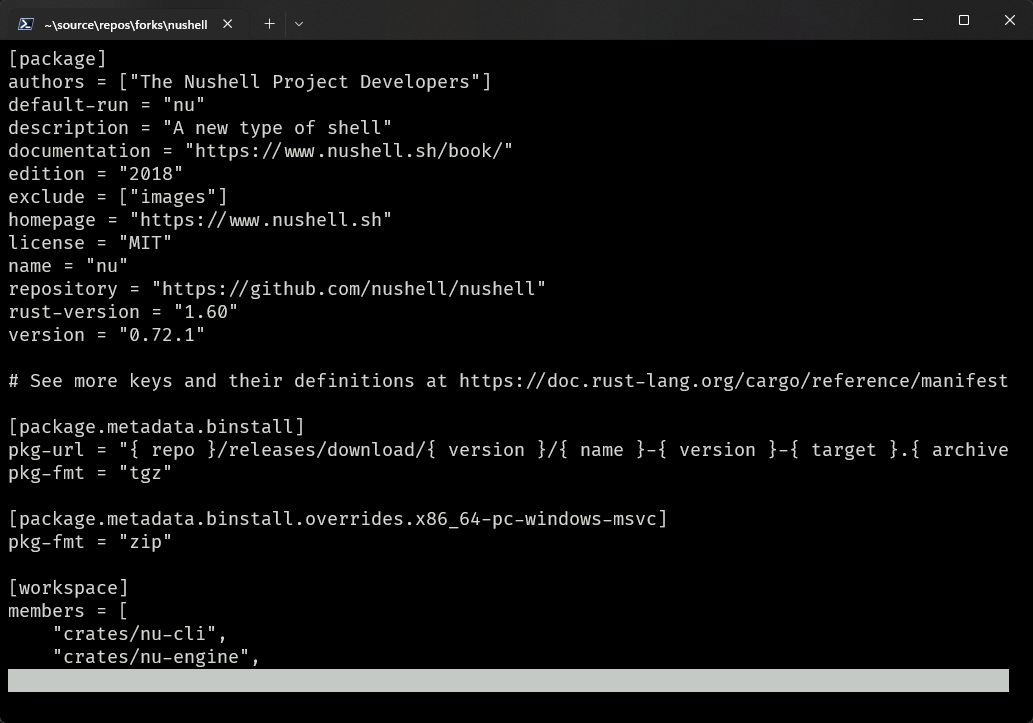
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
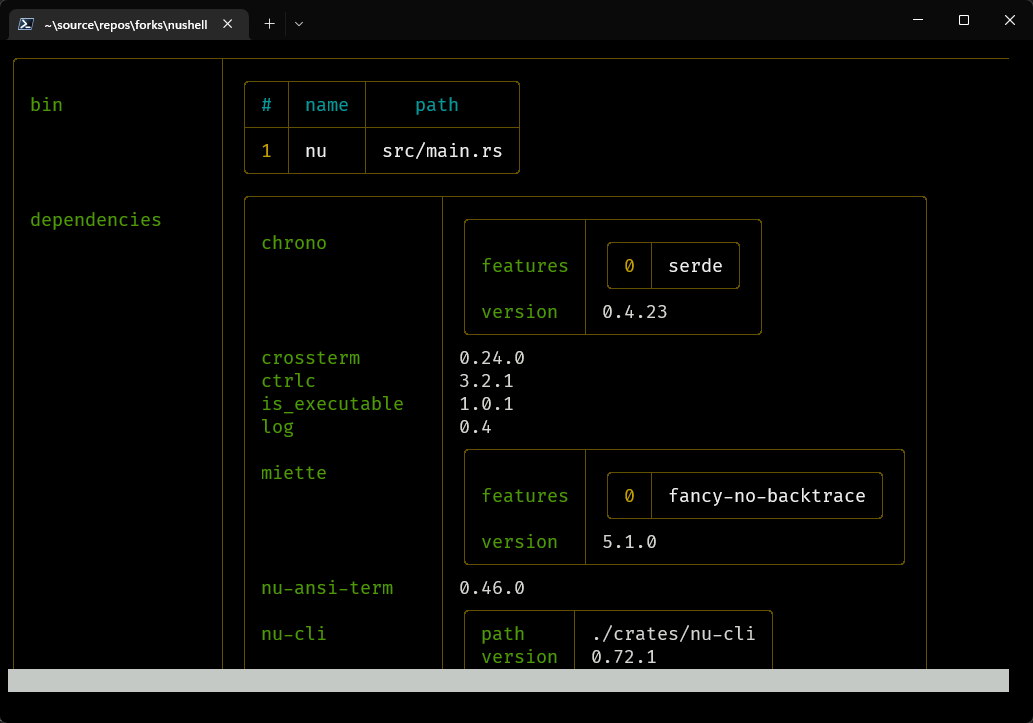
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
|
2022-12-16 15:47:07 +00:00
|
|
|
let style = style_from_config(&config);
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
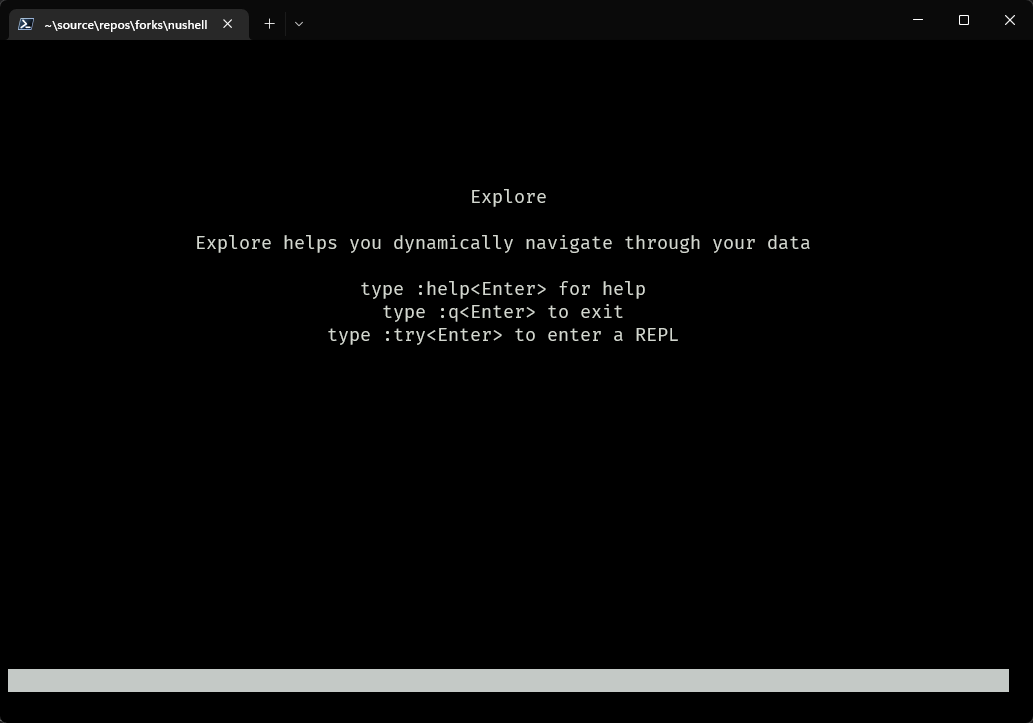
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
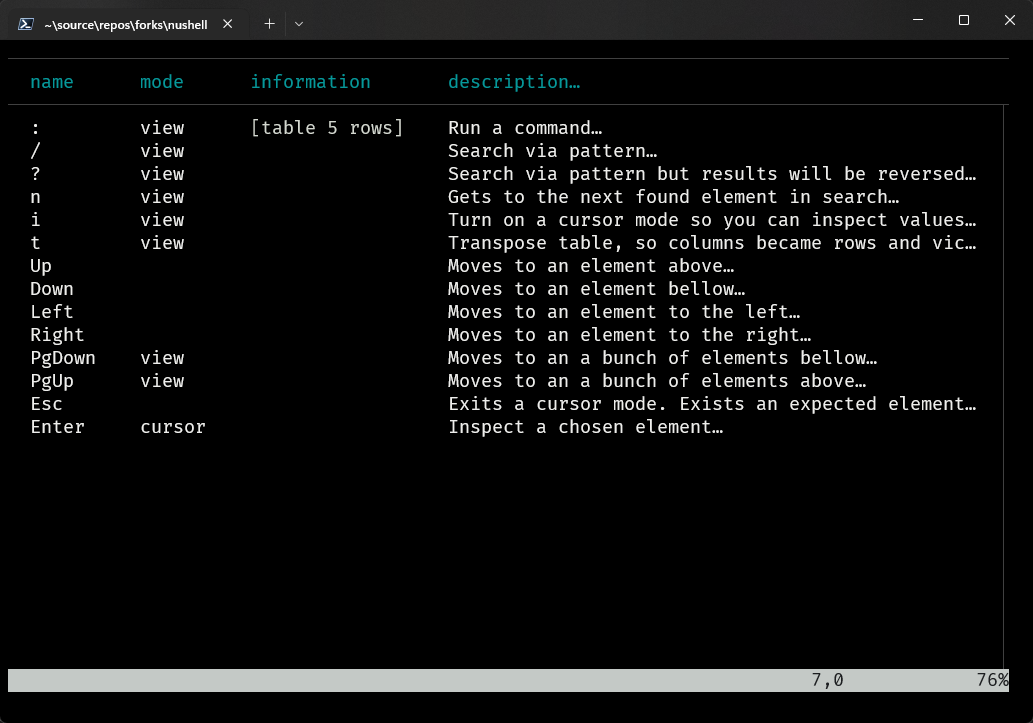
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
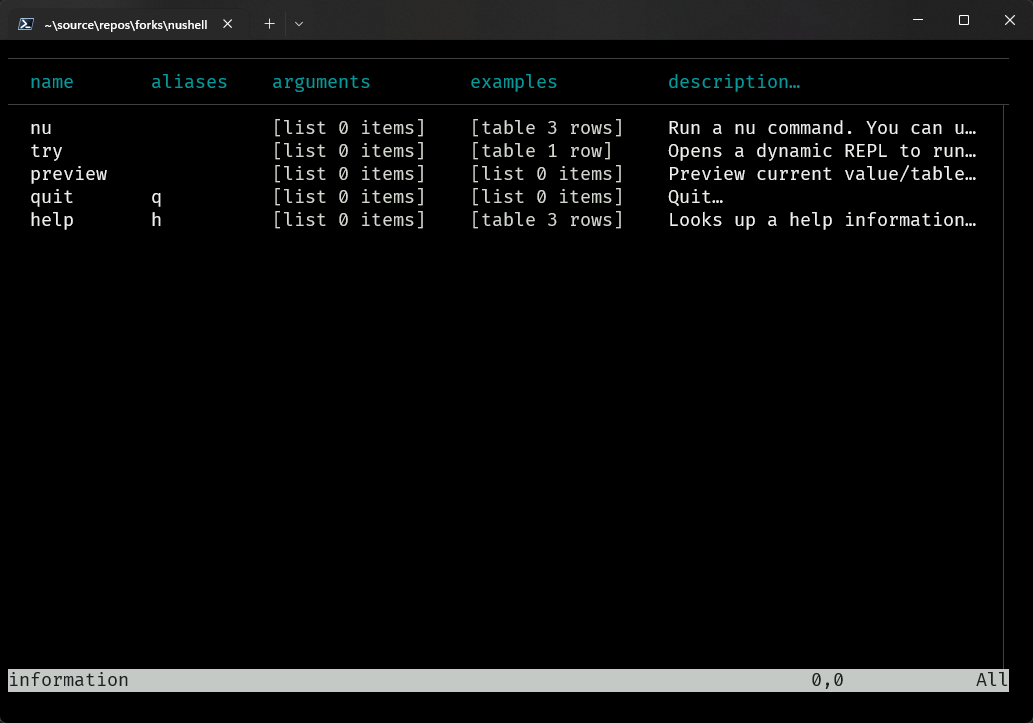
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
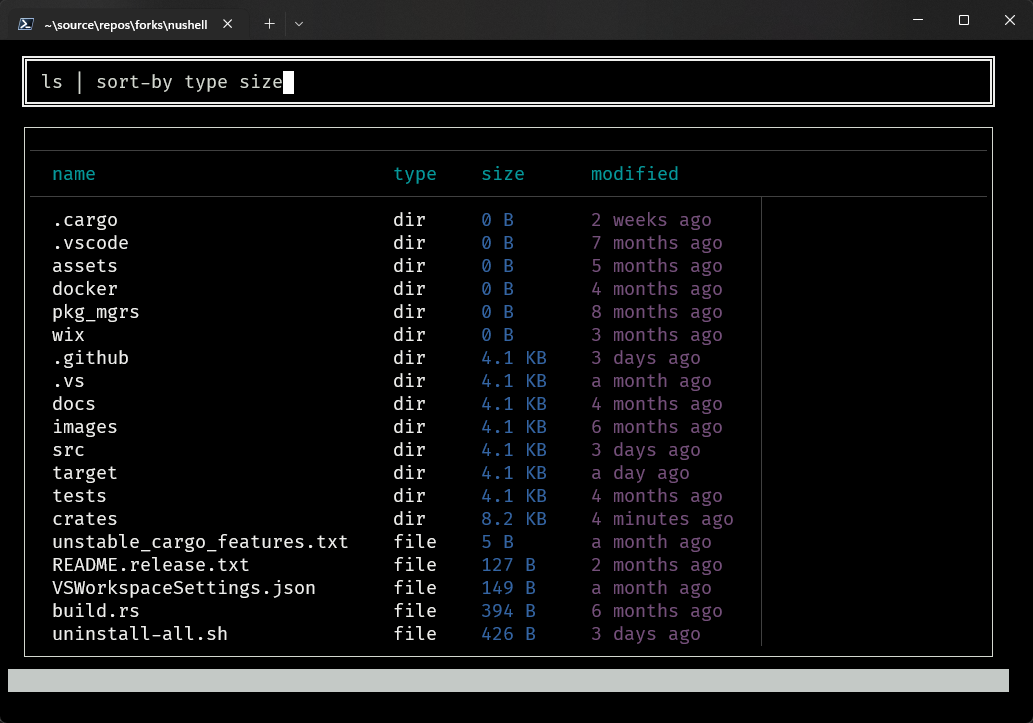
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
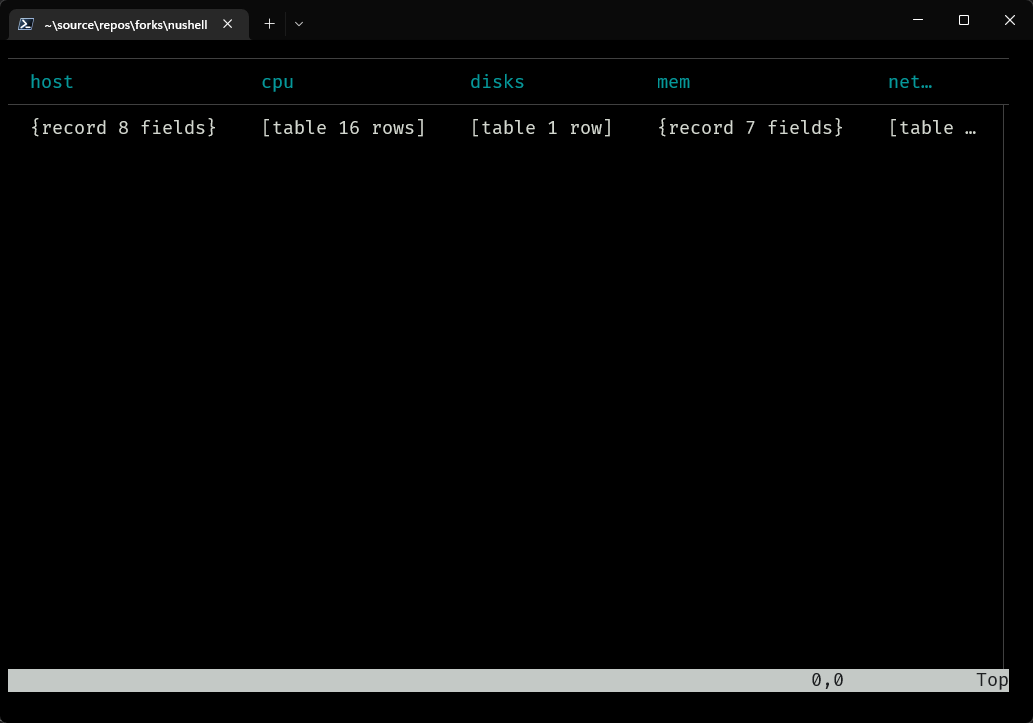
If you hit the `t` key it will now transpose the view to look like this.
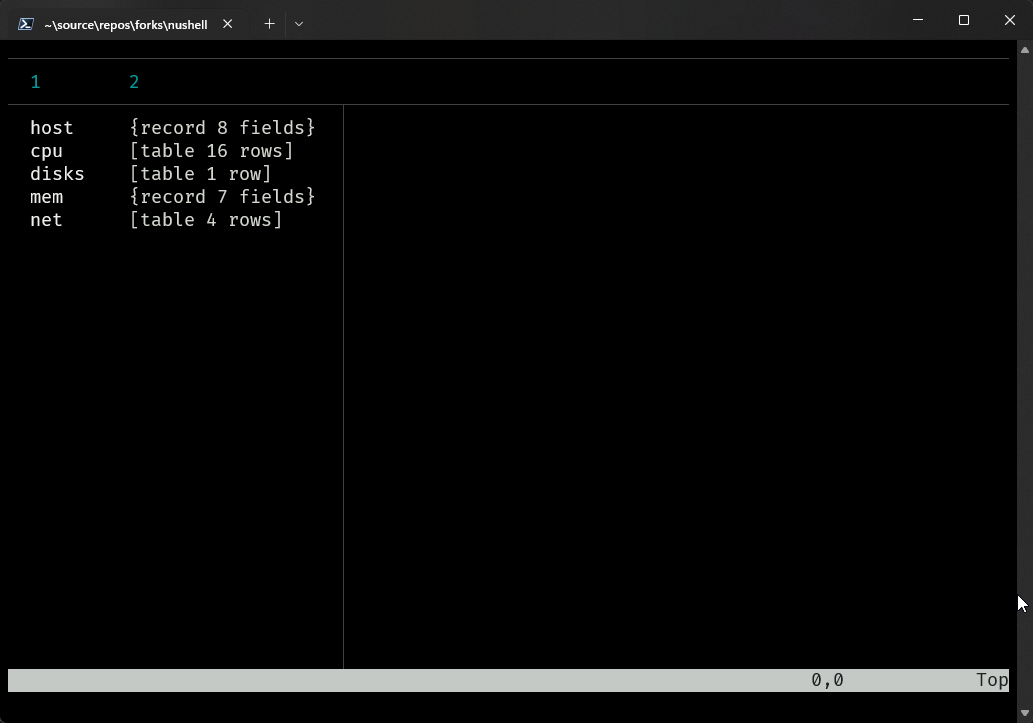
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
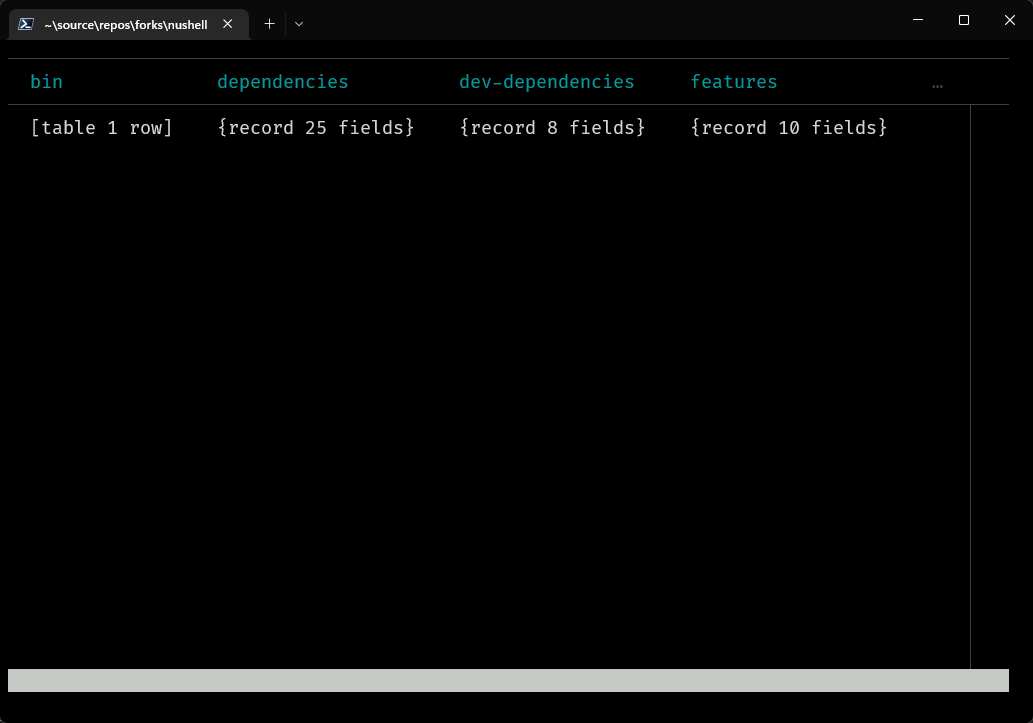
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
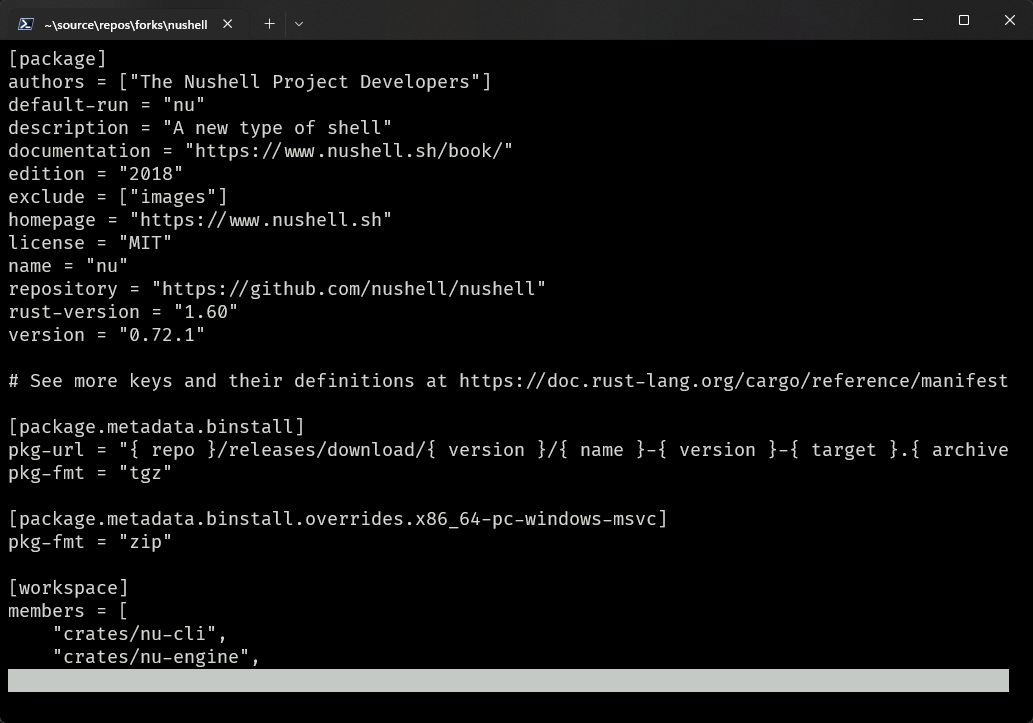
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
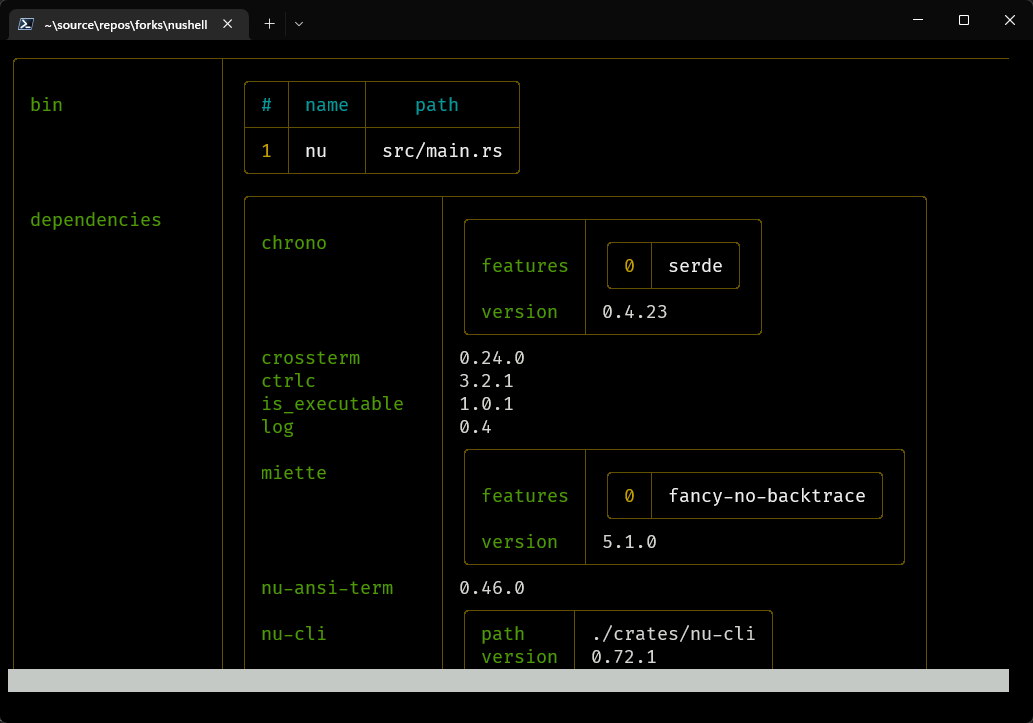
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
|
2023-06-13 23:18:36 +00:00
|
|
|
let lscolors = create_lscolors(engine_state, stack);
|
2022-12-18 14:43:15 +00:00
|
|
|
|
|
|
|
let mut config = PagerConfig::new(nu_config, &style_computer, &lscolors, config);
|
2022-12-16 15:47:07 +00:00
|
|
|
config.style = style;
|
|
|
|
config.reverse = is_reverse;
|
|
|
|
config.peek_value = peek_value;
|
|
|
|
config.reverse = is_reverse;
|
|
|
|
config.exit_esc = exit_esc;
|
|
|
|
config.show_banner = show_banner;
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
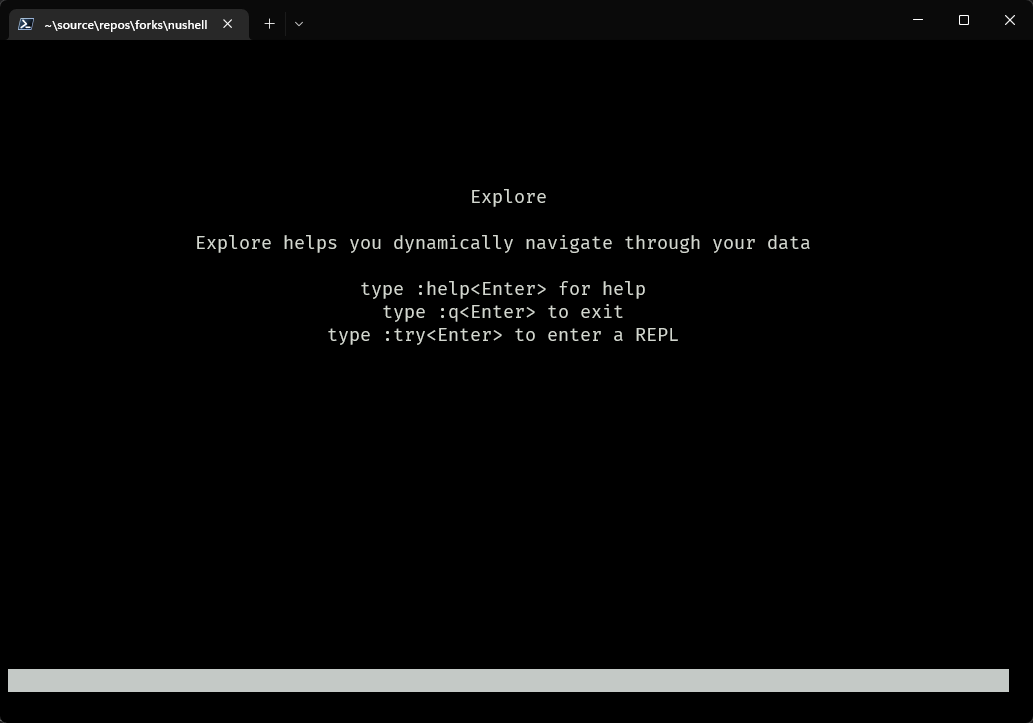
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
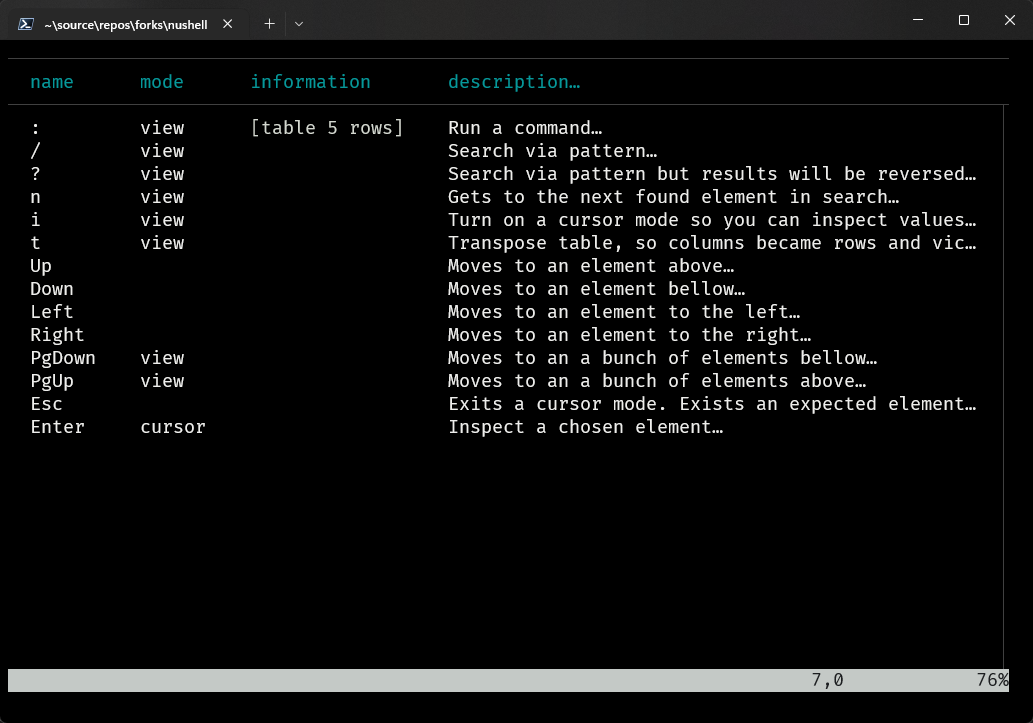
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
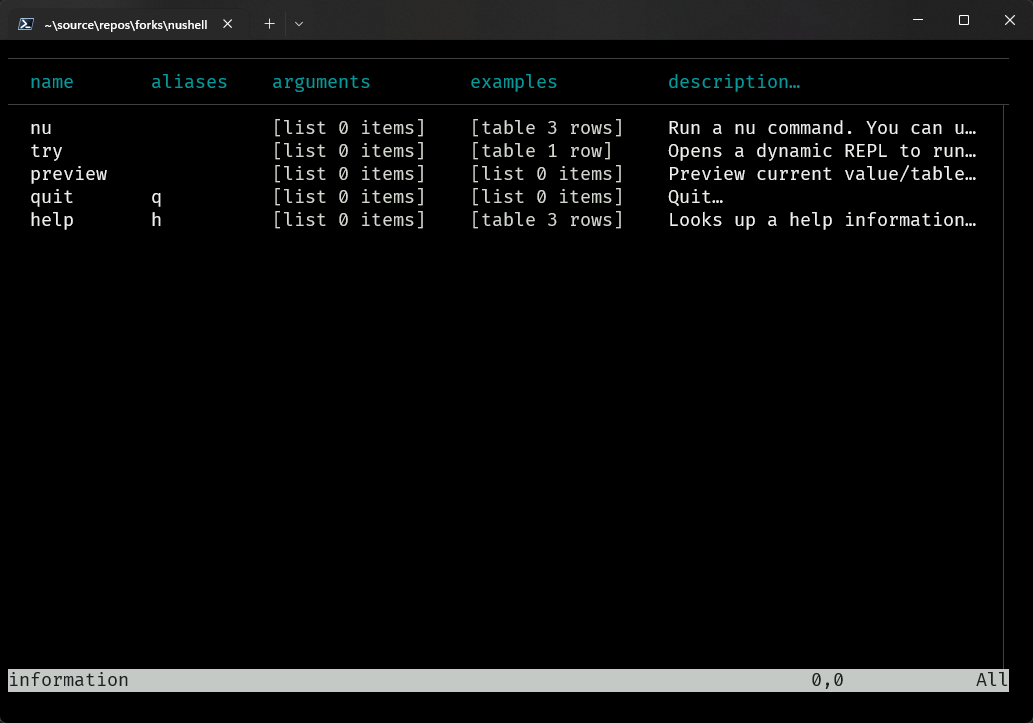
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
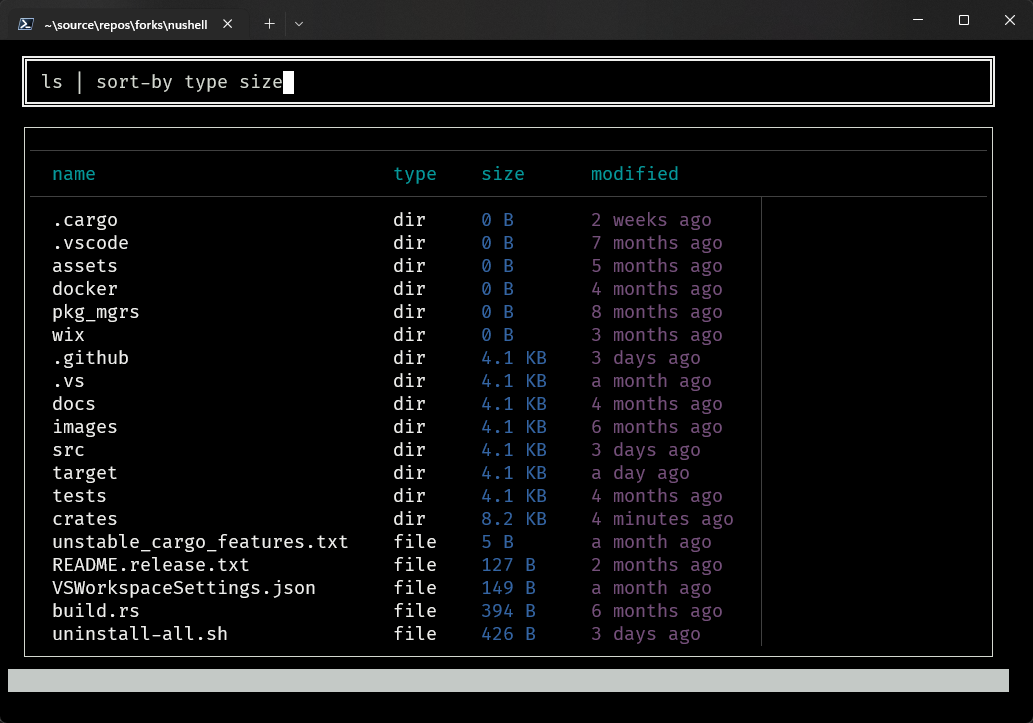
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
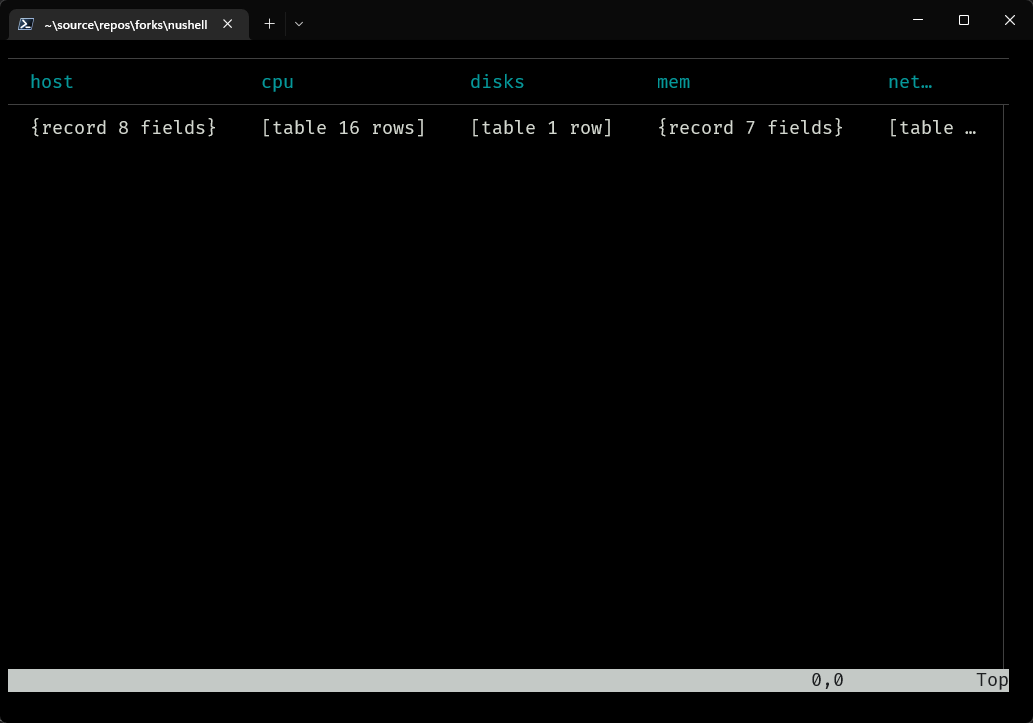
If you hit the `t` key it will now transpose the view to look like this.
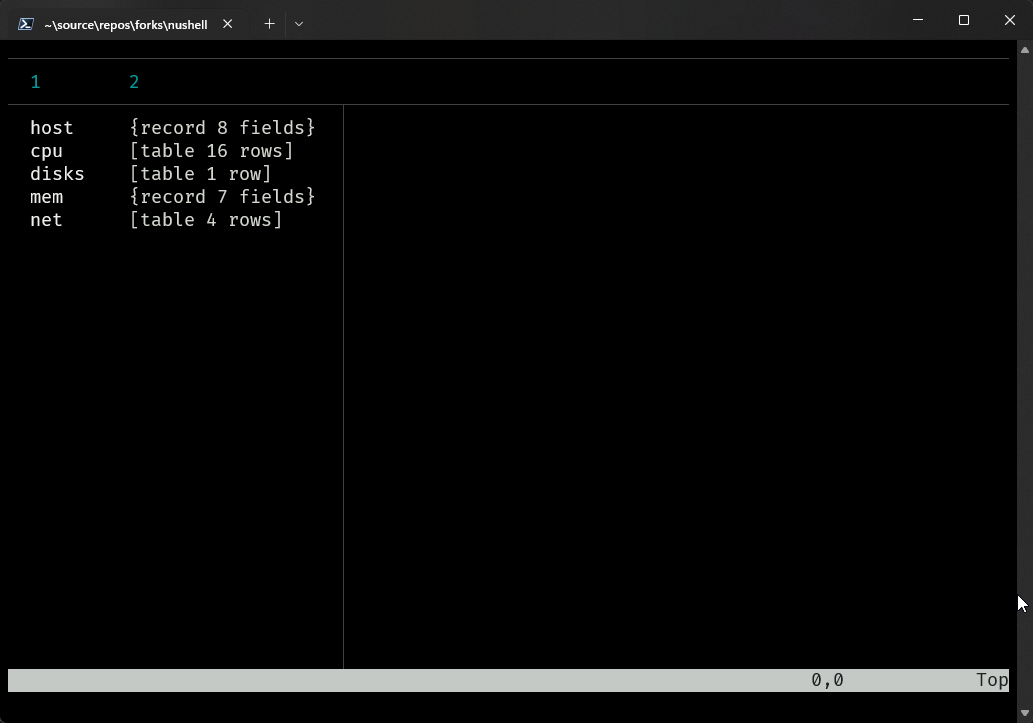
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
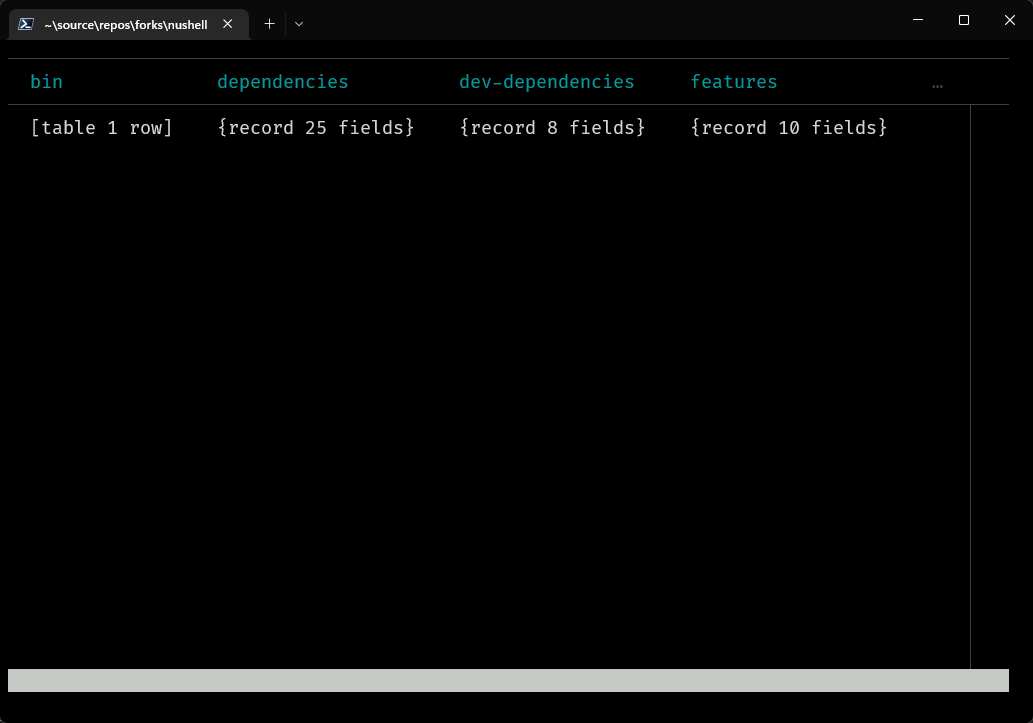
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
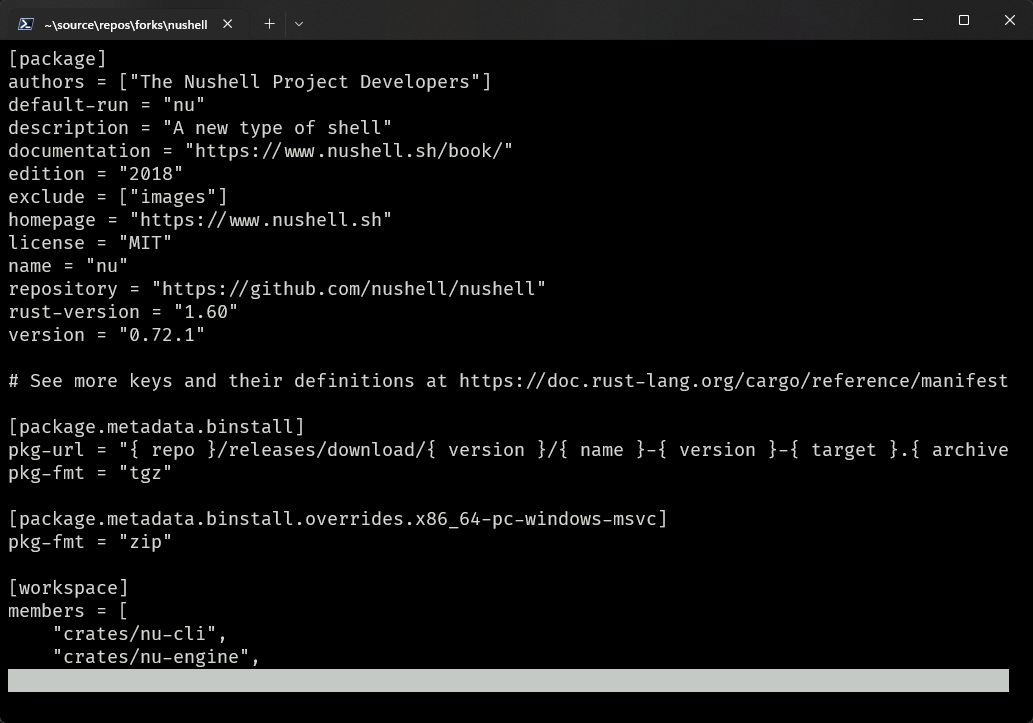
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
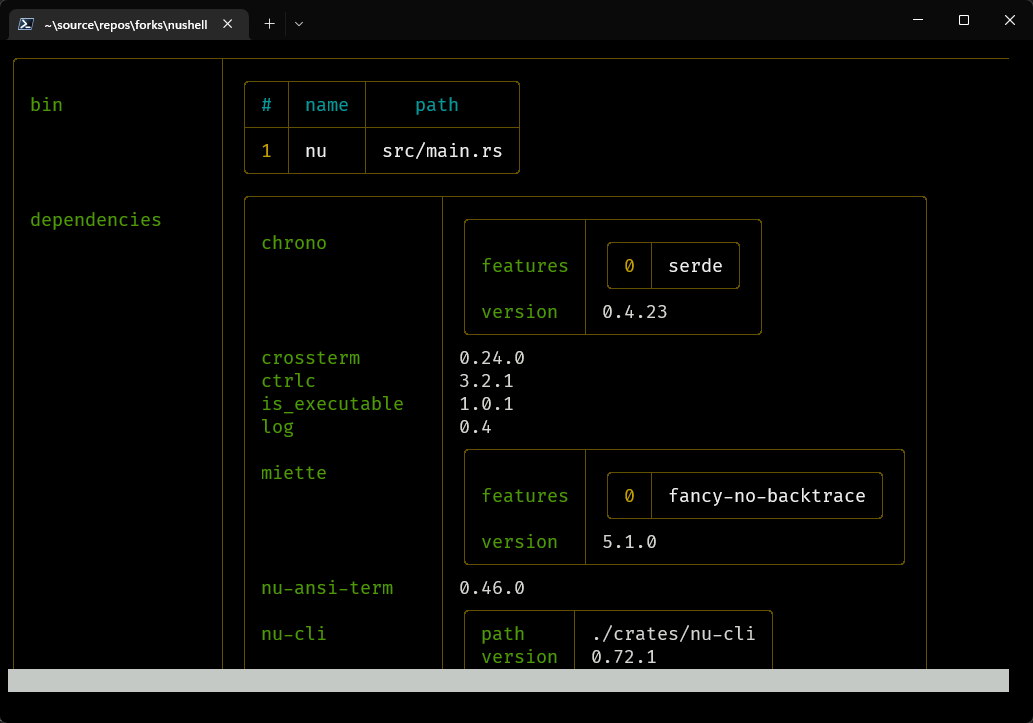
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
|
color_config now accepts closures as color values (#7141)
# Description
Closes #6909. You can now add closures to your `color_config` themes.
Whenever a value would be printed with `table`, the closure is run with
the value piped-in. The closure must return either a {fg,bg,attr} record
or a color name (`'light_red'` etc.). This returned style is used to
colour the value.
This is entirely backwards-compatible with existing config.nu files.
Example code excerpt:
```
let my_theme = {
header: green_bold
bool: { if $in { 'light_cyan' } else { 'light_red' } }
int: purple_bold
filesize: { |e| if $e == 0b { 'gray' } else if $e < 1mb { 'purple_bold' } else { 'cyan_bold' } }
duration: purple_bold
date: { (date now) - $in | if $in > 1wk { 'cyan_bold' } else if $in > 1day { 'green_bold' } else { 'yellow_bold' } }
range: yellow_bold
string: { if $in =~ '^#\w{6}$' { $in } else { 'white' } }
nothing: white
```
Example output with this in effect:
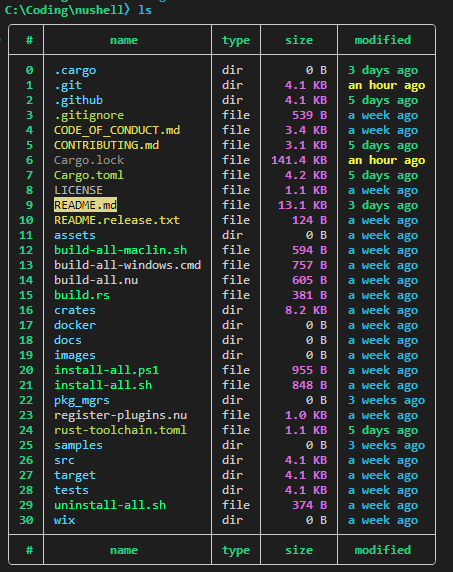
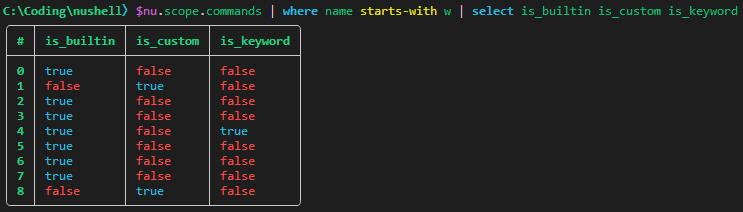
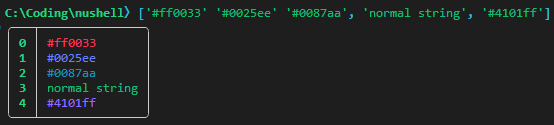
Slightly important notes:
* Some color_config names, namely "separator", "empty" and "hints", pipe
in `null` instead of a value.
* Currently, doing anything non-trivial inside a closure has an
understandably big perf hit. I currently do not actually recommend
something like `string: { if $in =~ '^#\w{6}$' { $in } else { 'white' }
}` for serious work, mainly because of the abundance of string-type data
in the world. Nevertheless, lesser-used types like "date" and "duration"
work well with this.
* I had to do some reorganisation in order to make it possible to call
`eval_block()` that late in table rendering. I invented a new struct
called "StyleComputer" which holds the engine_state and stack of the
initial `table` command (implicit or explicit).
* StyleComputer has a `compute()` method which takes a color_config name
and a nu value, and always returns the correct Style, so you don't have
to worry about A) the color_config value was set at all, B) whether it
was set to a closure or not, or C) which default style to use in those
cases.
* Currently, errors encountered during execution of the closures are
thrown in the garbage. Any other ideas are welcome. (Nonetheless, errors
result in a huge perf hit when they are encountered. I think what should
be done is to assume something terrible happened to the user's config
and invalidate the StyleComputer for that `table` run, thus causing
subsequent output to just be Style::default().)
* More thorough tests are forthcoming - ran into some difficulty using
`nu!` to take an alternative config, and for some reason `let-env config
=` statements don't seem to work inside `nu!` pipelines(???)
* The default config.nu has not been updated to make use of this yet. Do
tell if you think I should incorporate that into this.
# User-Facing Changes
See above.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace --features=extra -- -D warnings -D
clippy::unwrap_used -A clippy::needless_collect` to check that you're
using the standard code style
- `cargo test --workspace --features=extra` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2022-12-17 13:07:56 +00:00
|
|
|
let result = run_pager(engine_state, &mut stack.clone(), ctrlc, input, config);
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
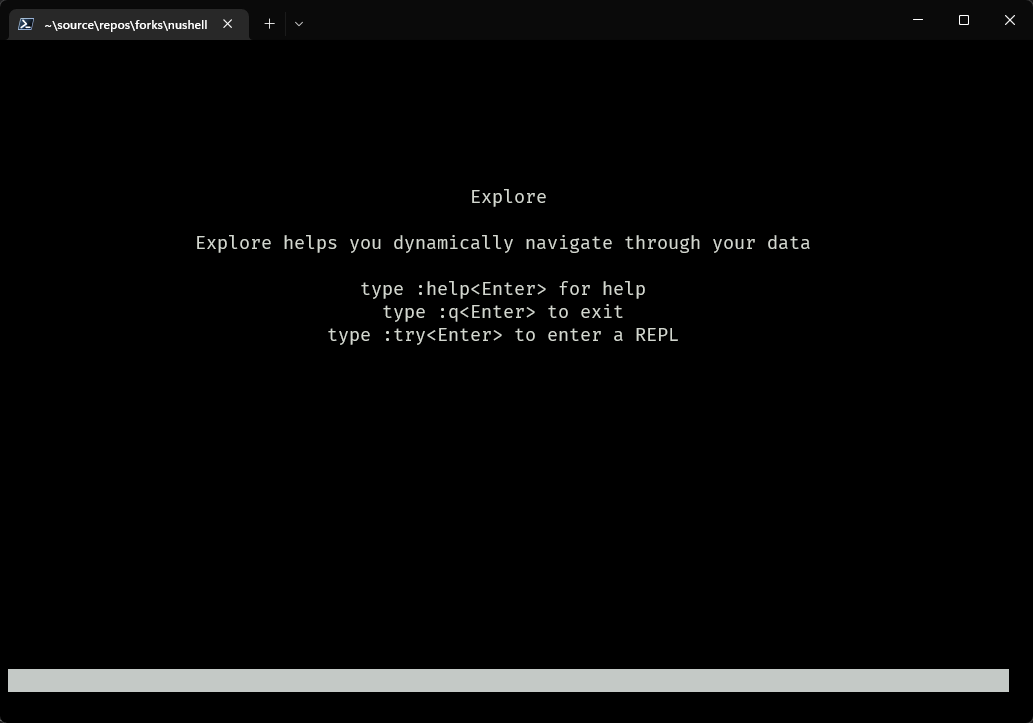
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
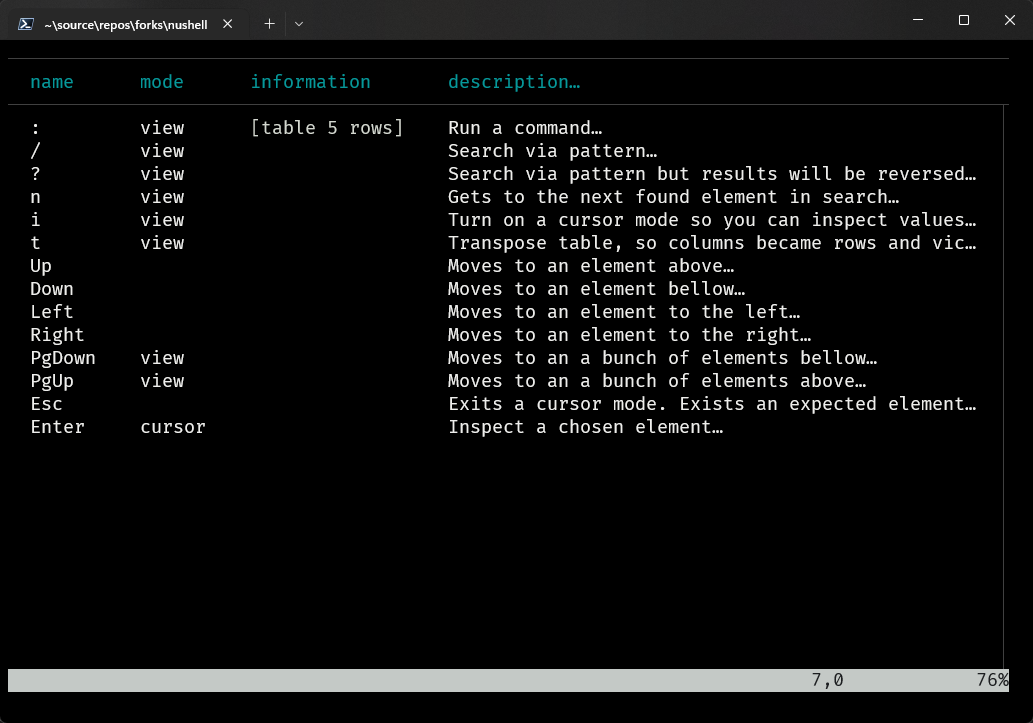
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
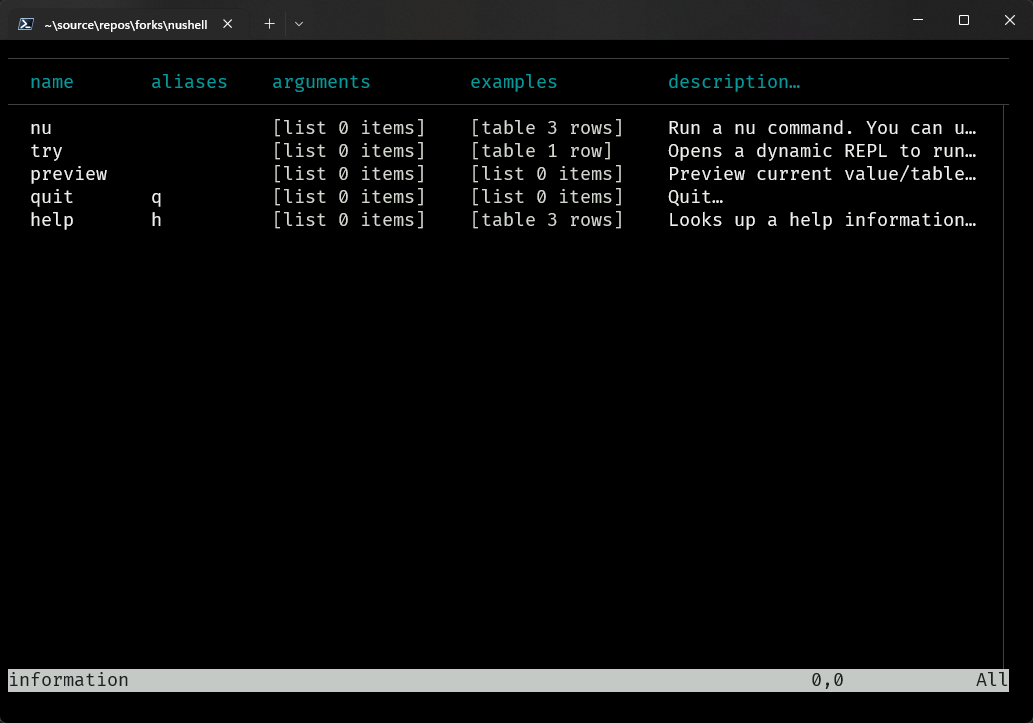
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
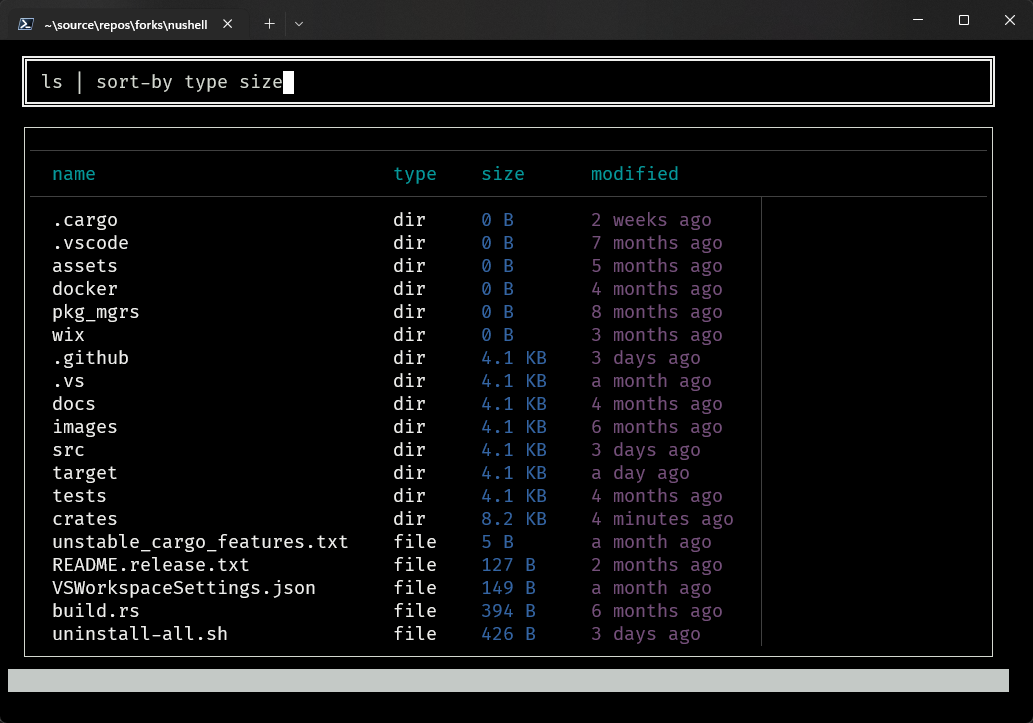
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
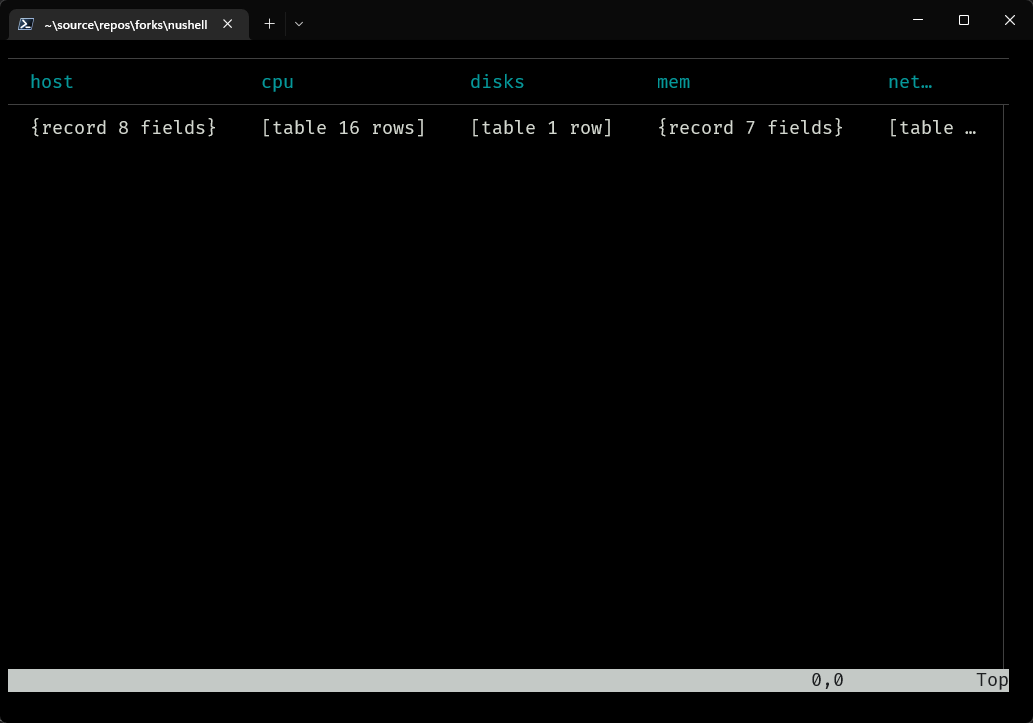
If you hit the `t` key it will now transpose the view to look like this.
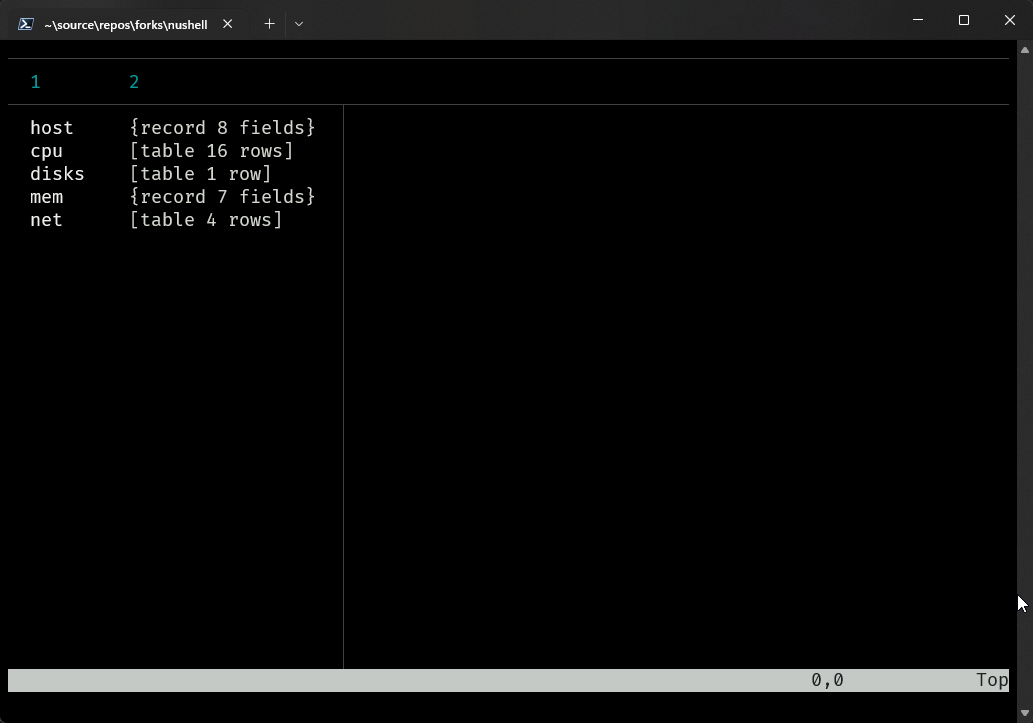
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
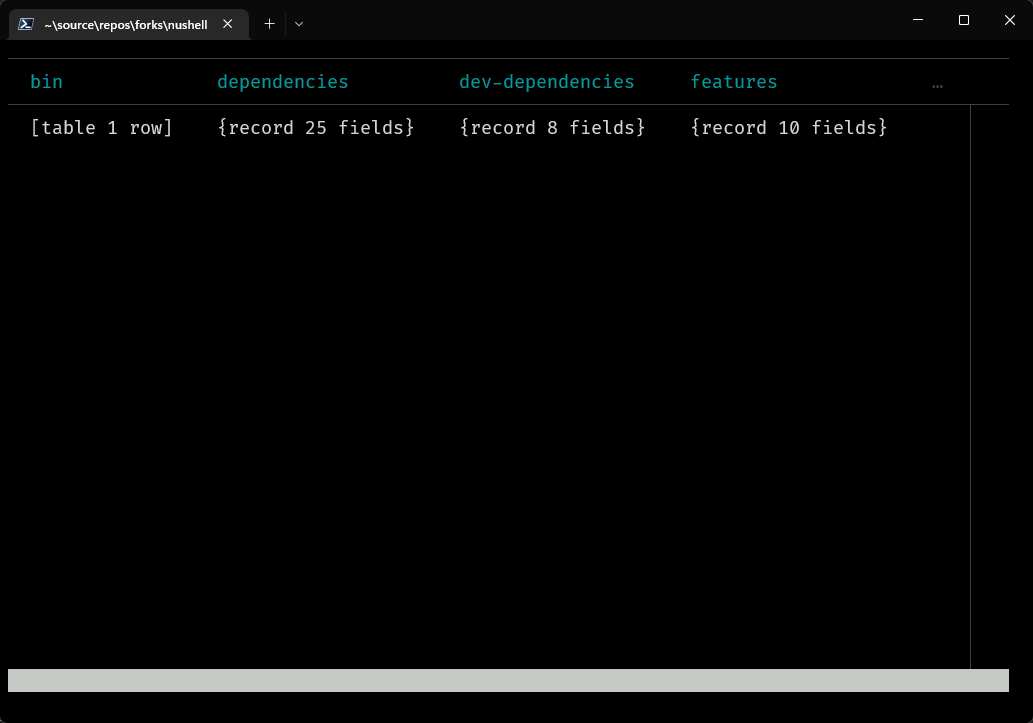
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
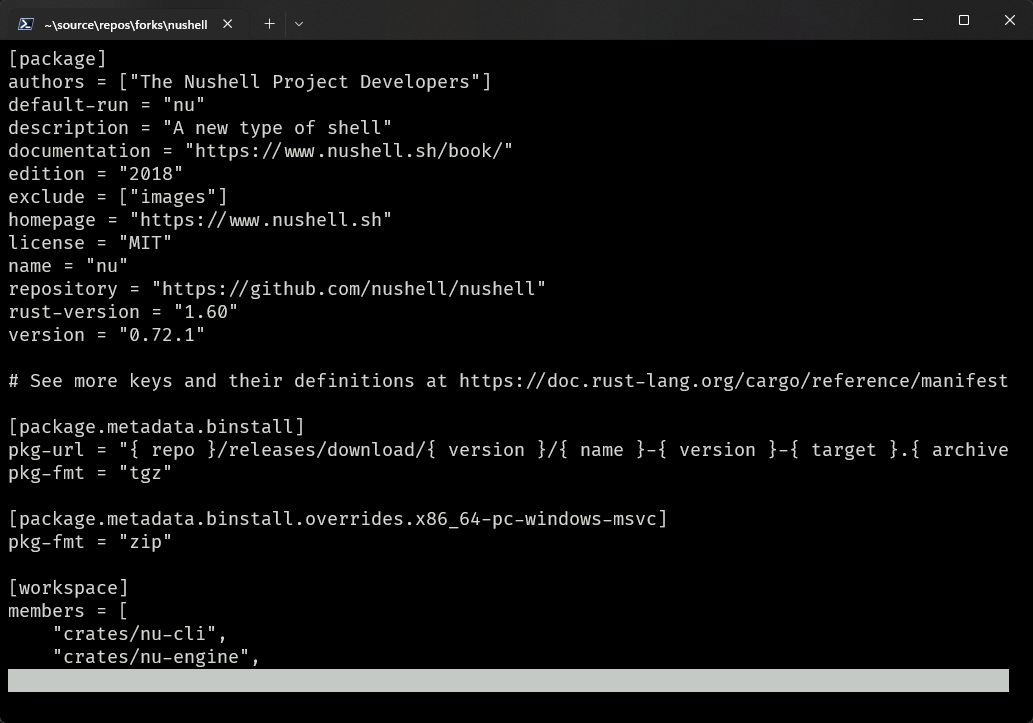
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
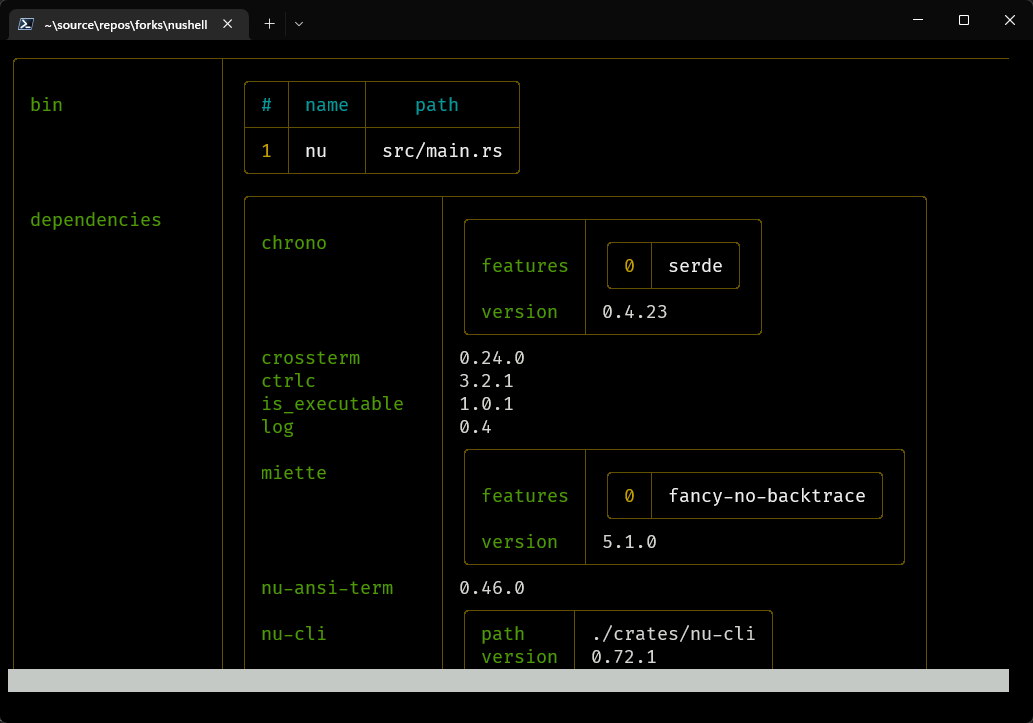
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
|
|
|
|
match result {
|
|
|
|
Ok(Some(value)) => Ok(PipelineData::Value(value, None)),
|
|
|
|
Ok(None) => Ok(PipelineData::Value(Value::default(), None)),
|
|
|
|
Err(err) => Ok(PipelineData::Value(
|
2023-09-03 14:27:29 +00:00
|
|
|
Value::error(err.into(), call.head),
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
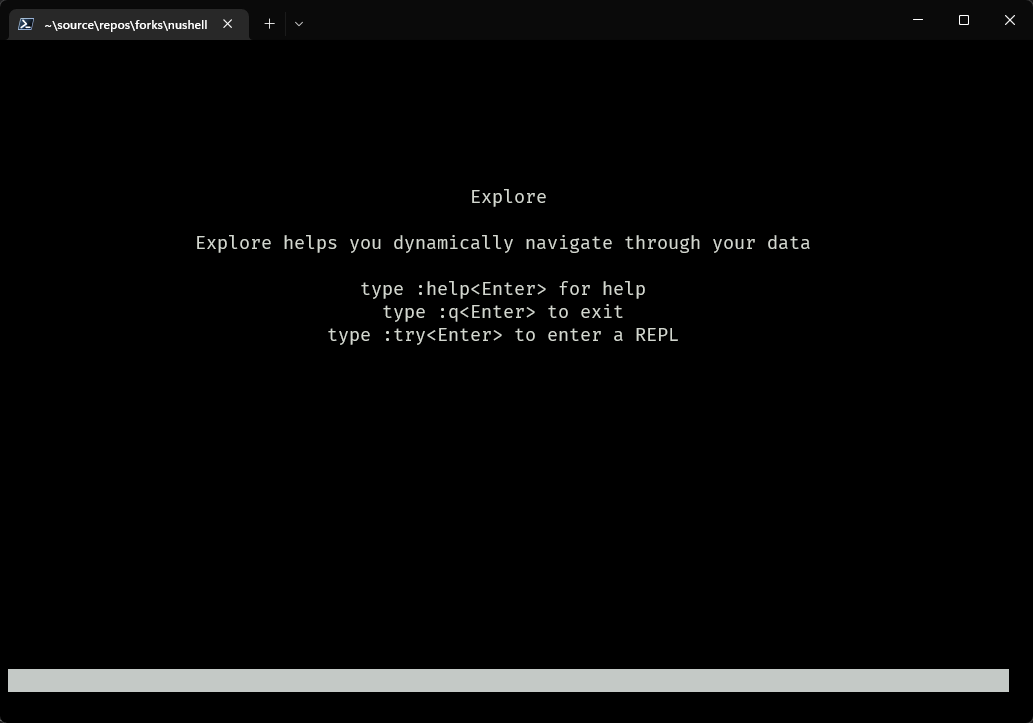
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
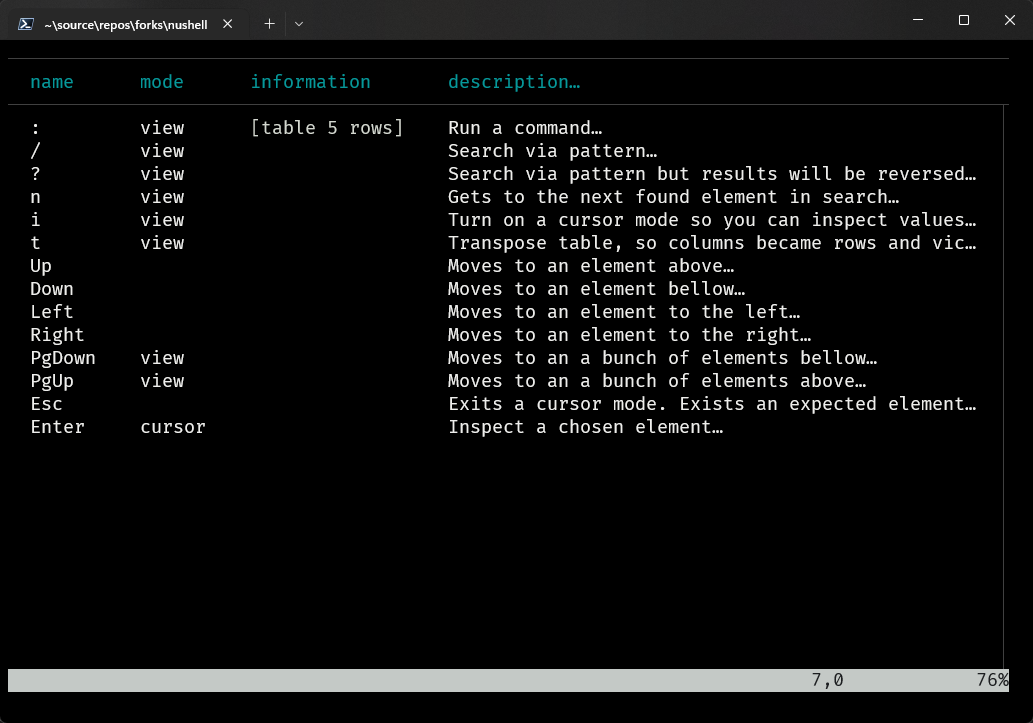
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
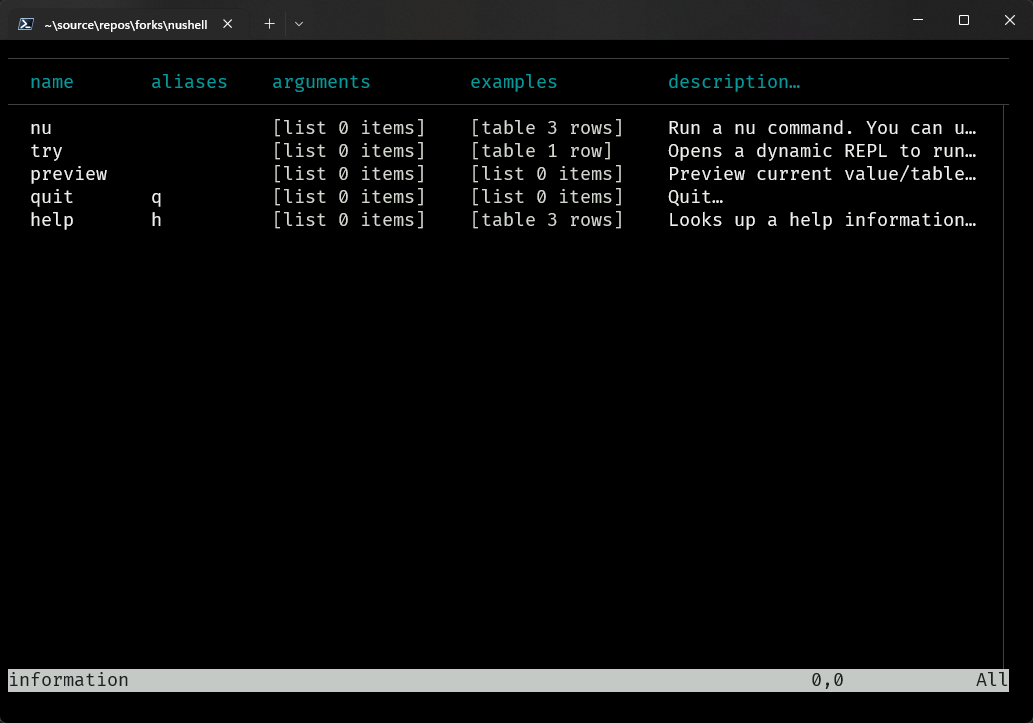
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
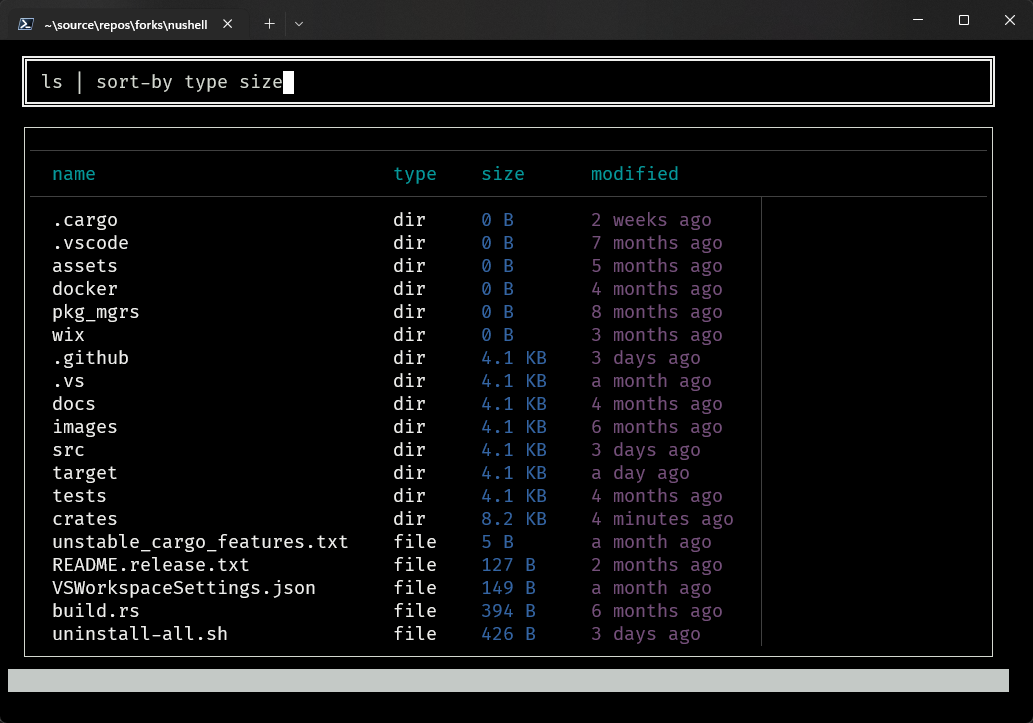
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
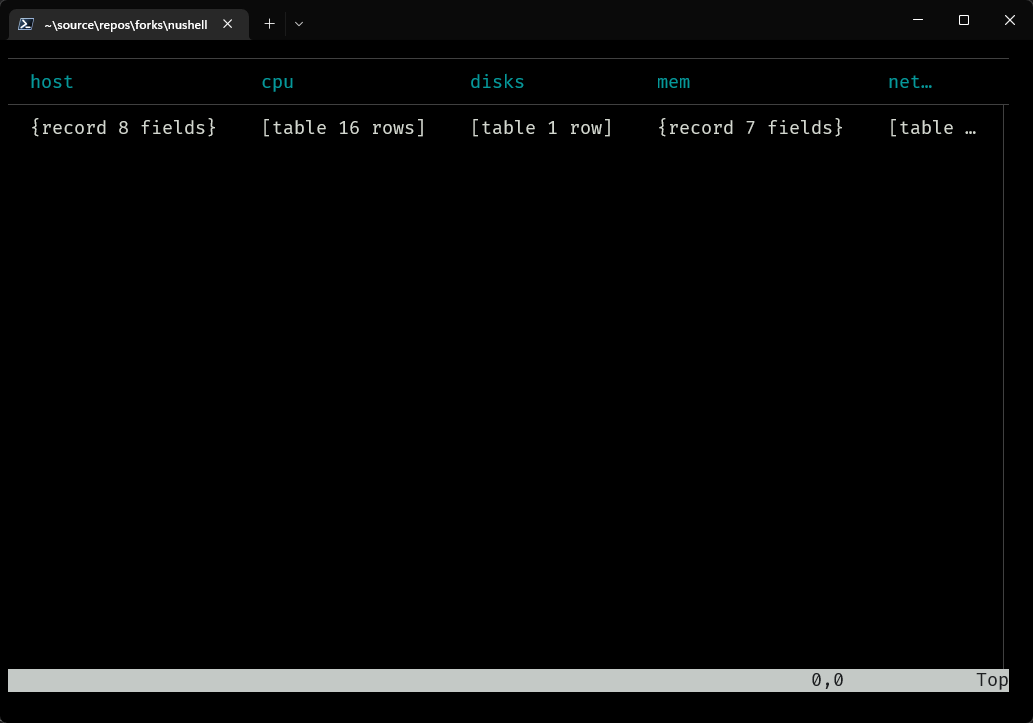
If you hit the `t` key it will now transpose the view to look like this.
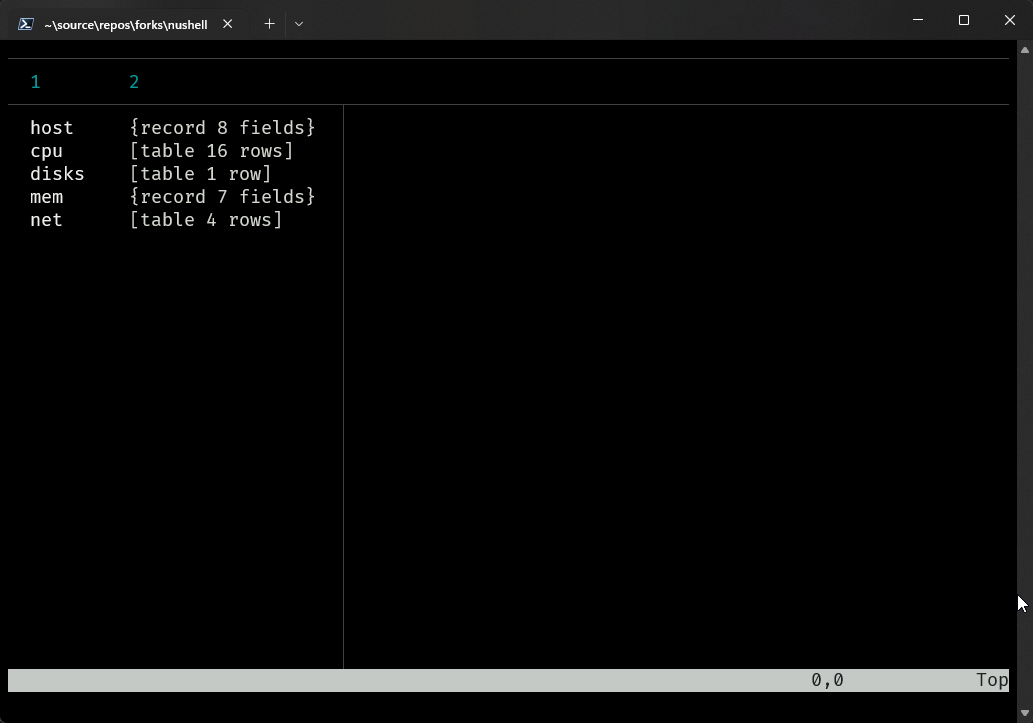
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
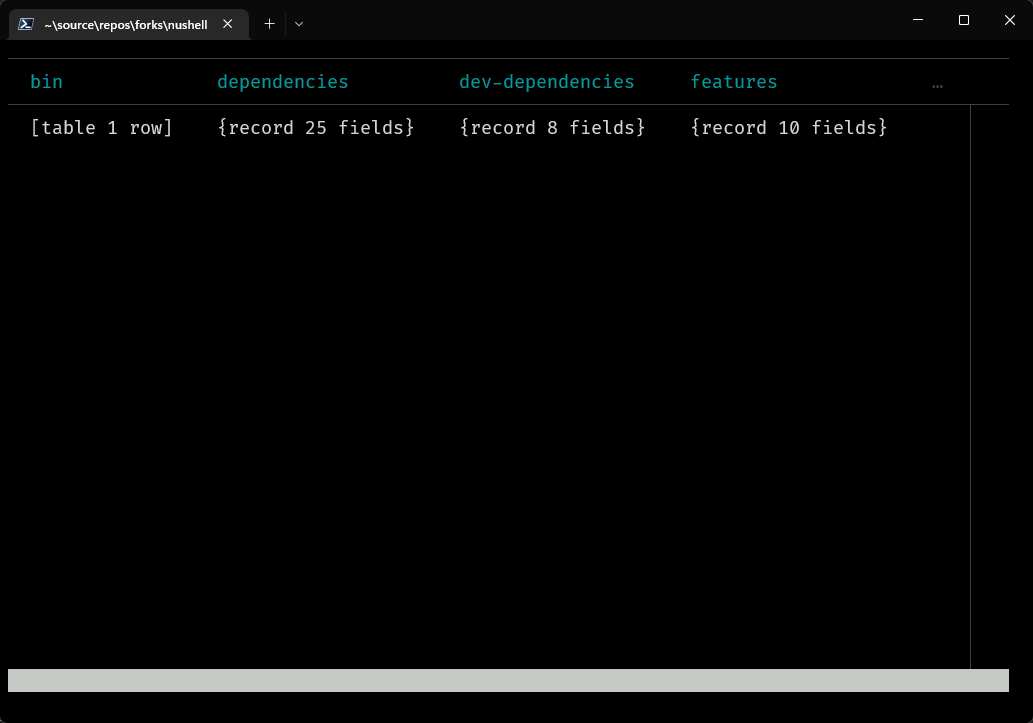
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
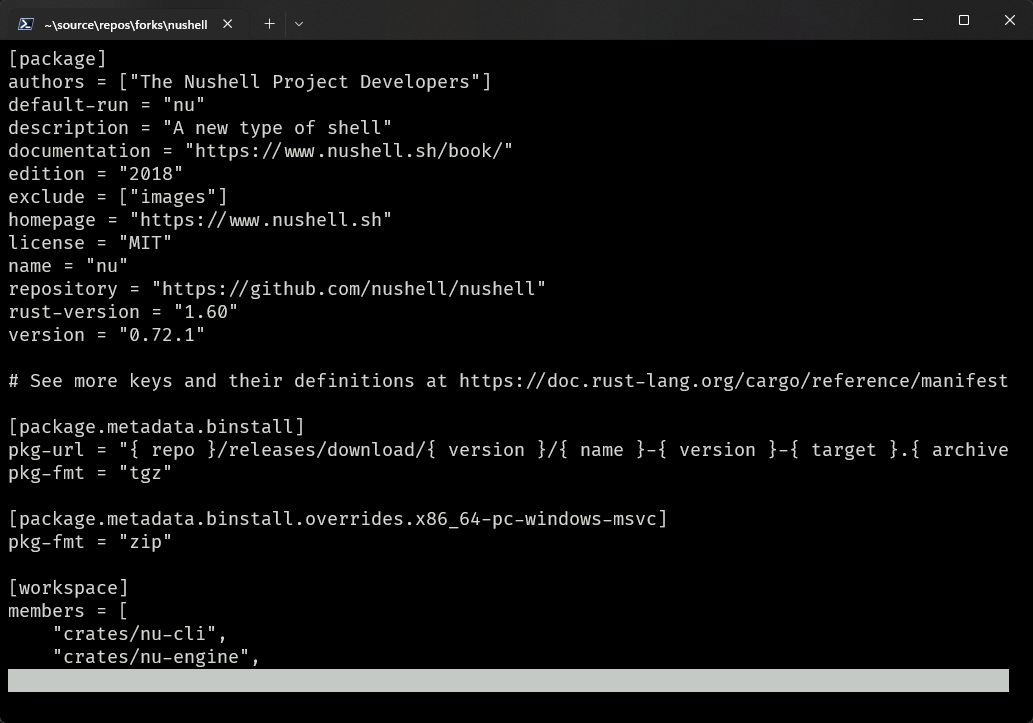
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
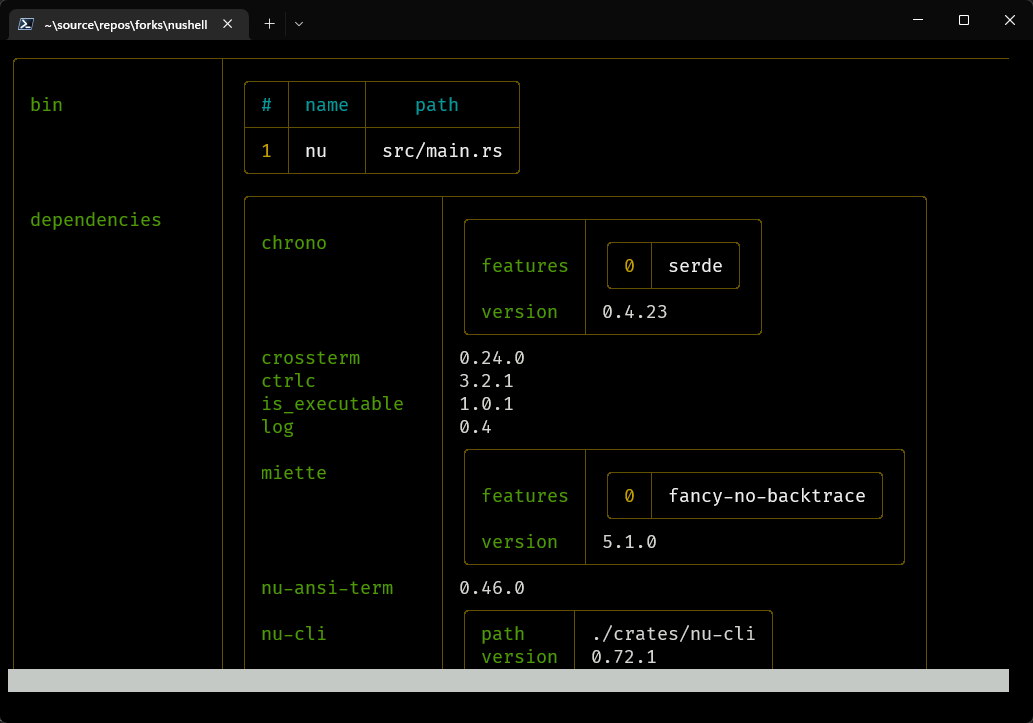
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
None,
|
|
|
|
)),
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
fn examples(&self) -> Vec<Example> {
|
|
|
|
vec![
|
|
|
|
Example {
|
2022-12-02 14:01:02 +00:00
|
|
|
description: "Explore the system information record",
|
|
|
|
example: r#"sys | explore"#,
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
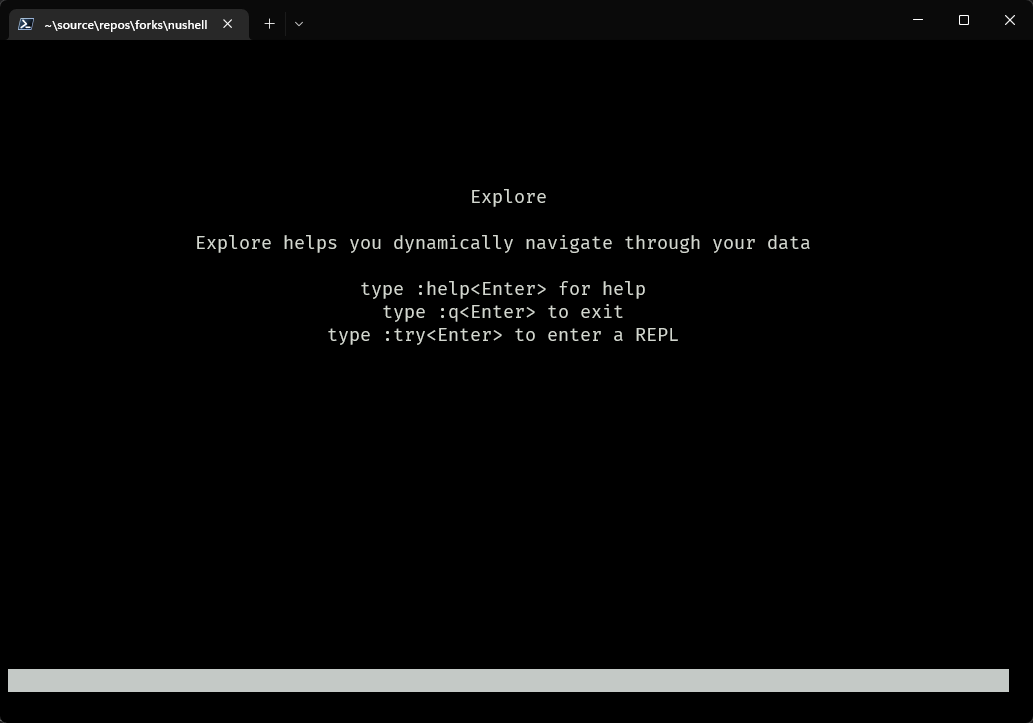
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
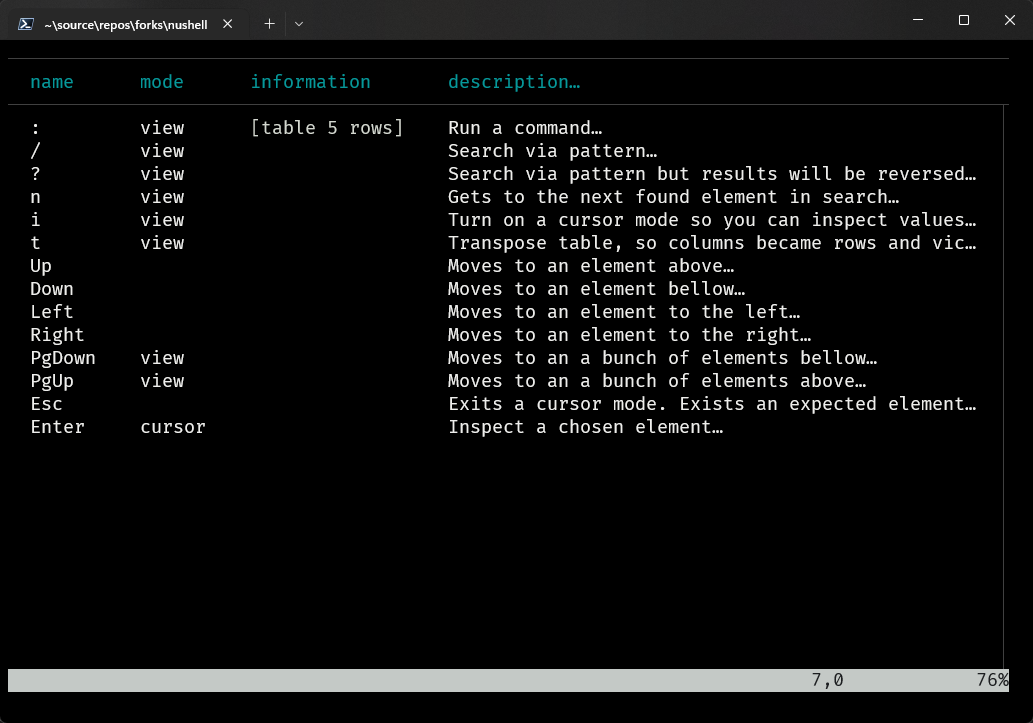
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
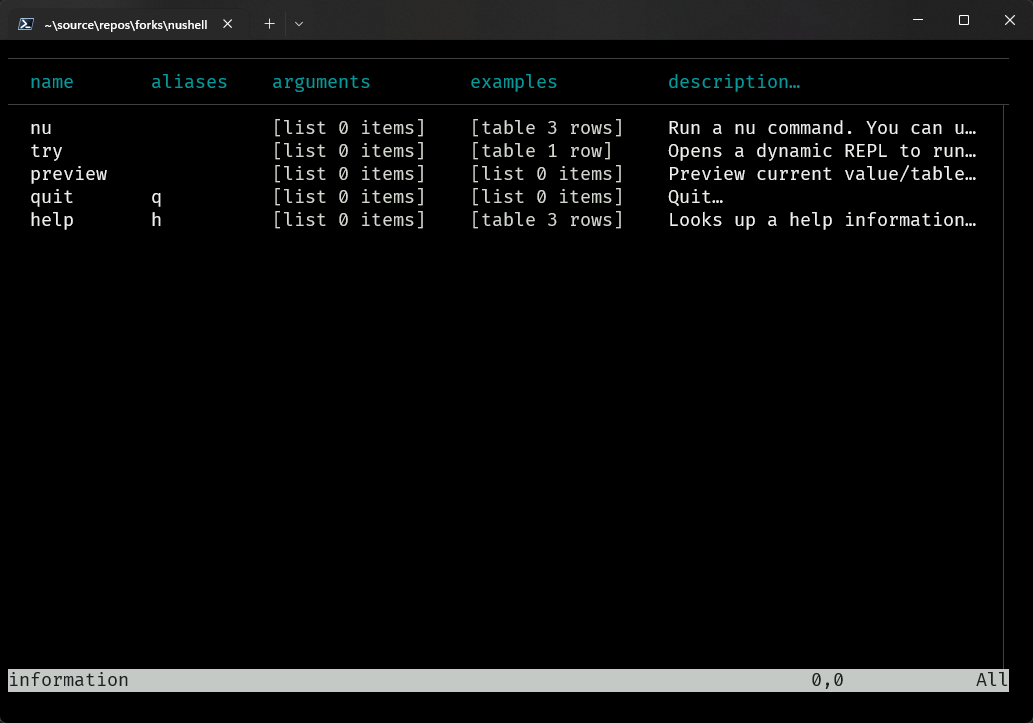
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
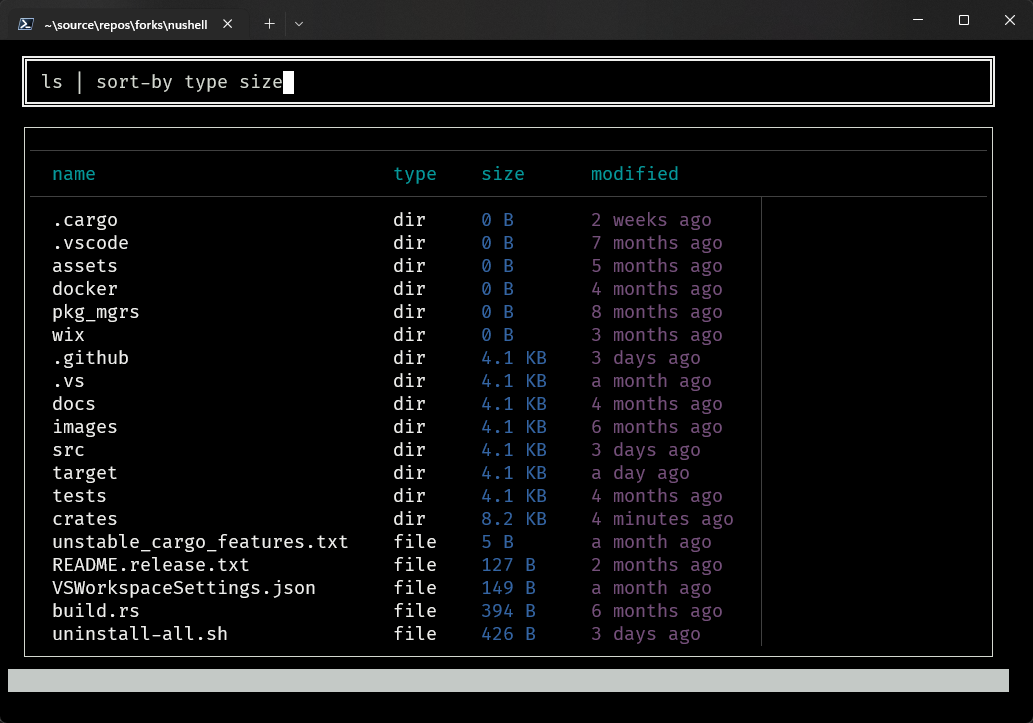
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
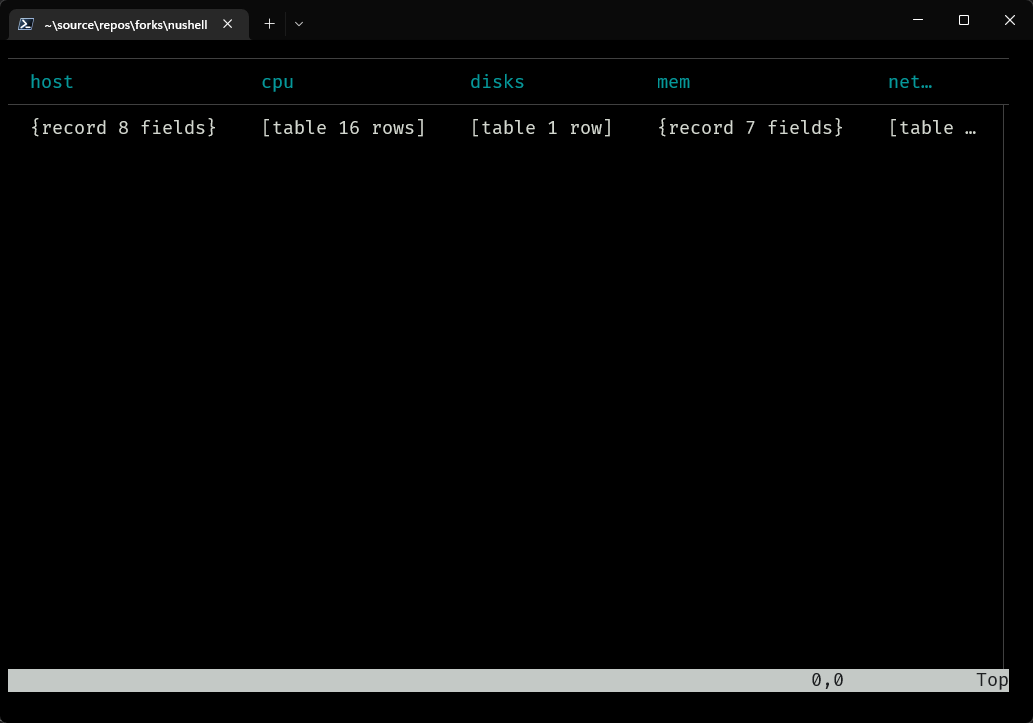
If you hit the `t` key it will now transpose the view to look like this.
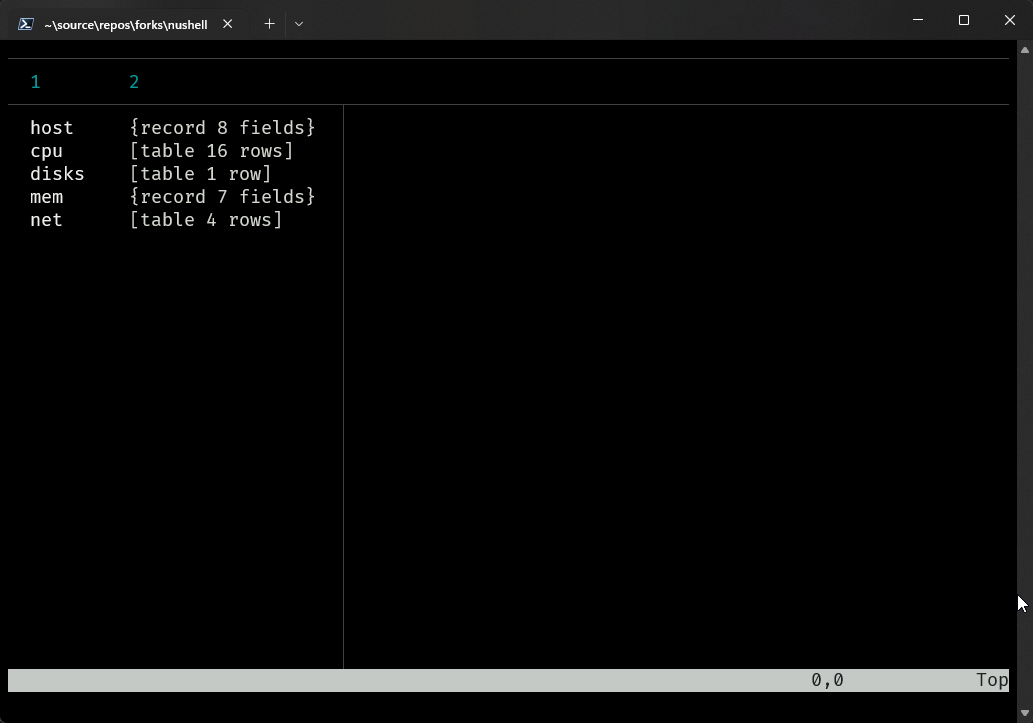
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
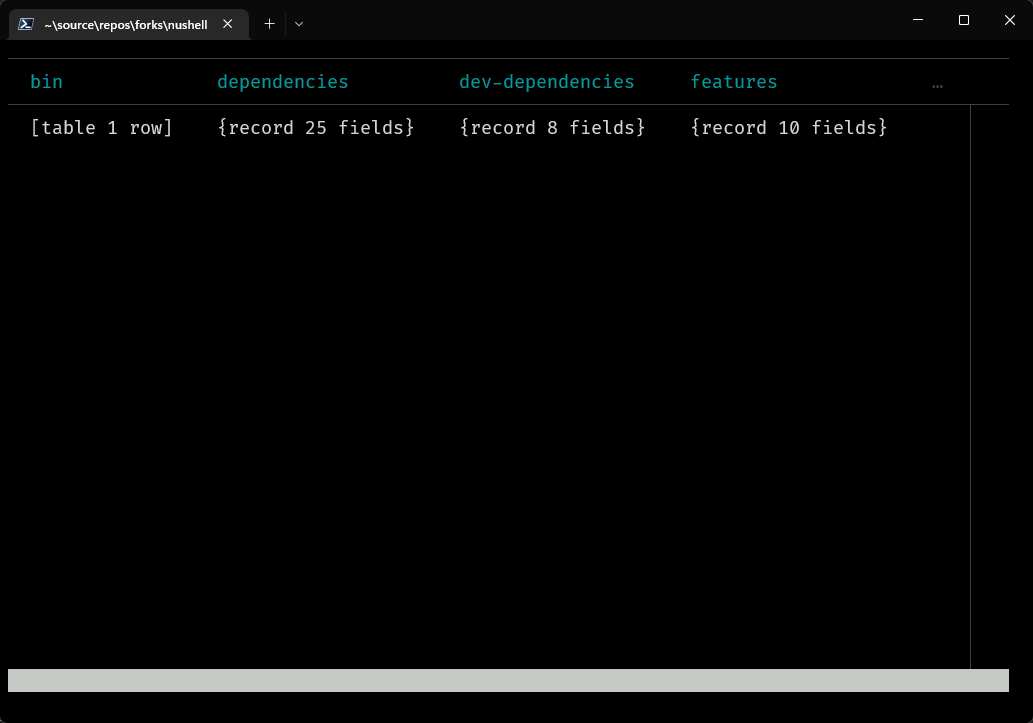
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
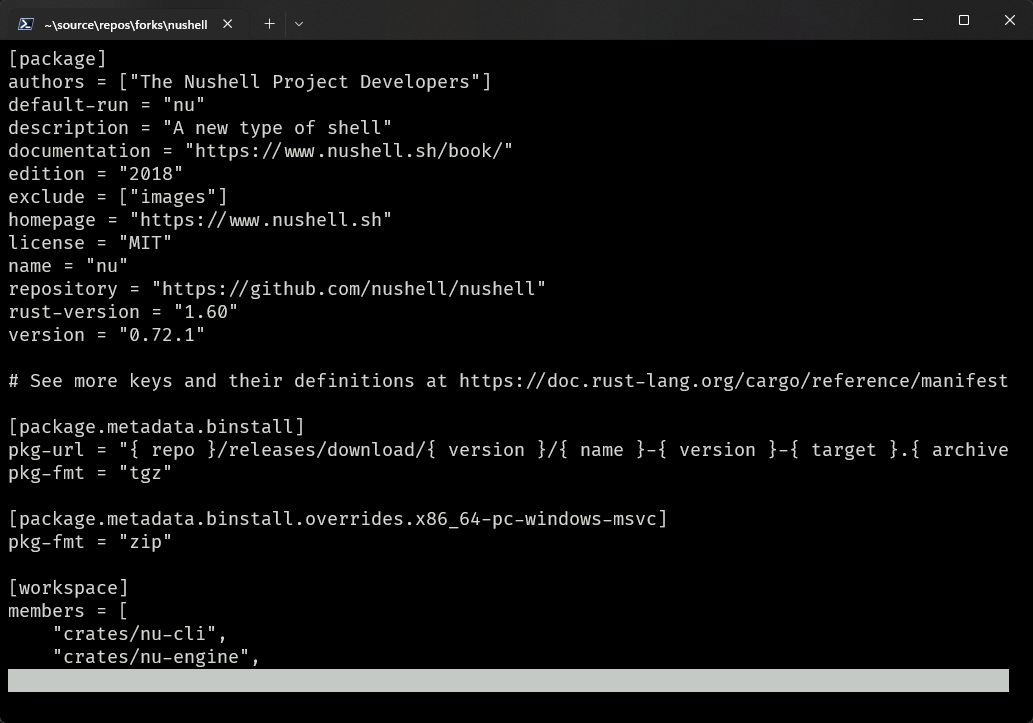
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
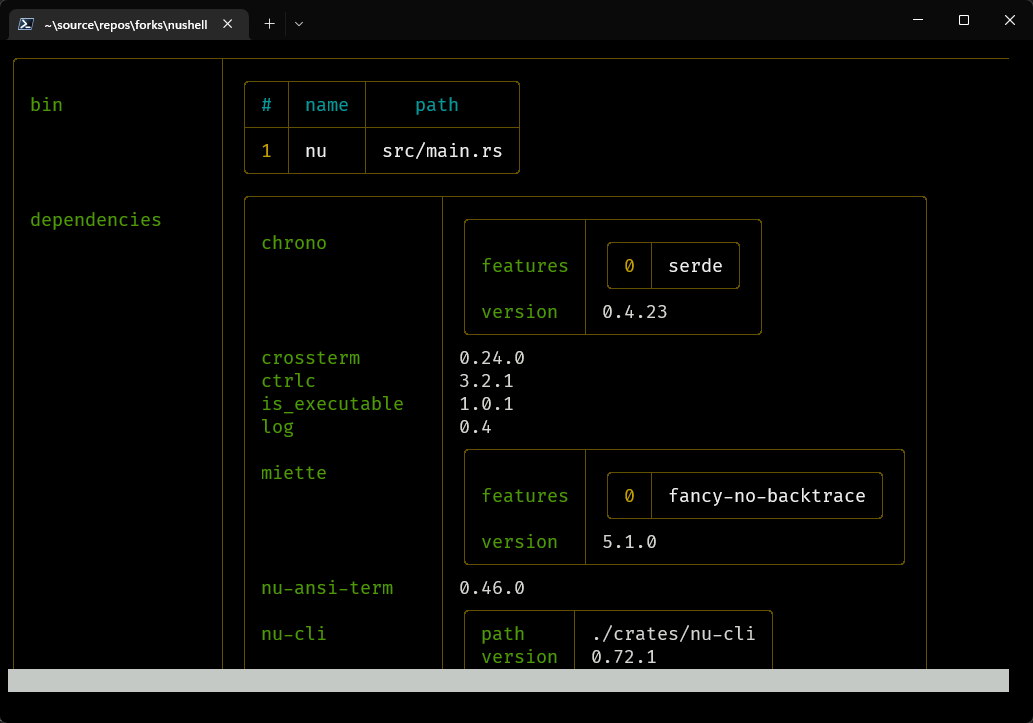
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
result: None,
|
|
|
|
},
|
|
|
|
Example {
|
2022-12-02 14:01:02 +00:00
|
|
|
description: "Explore the output of `ls` without column names",
|
|
|
|
example: r#"ls | explore --head false"#,
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
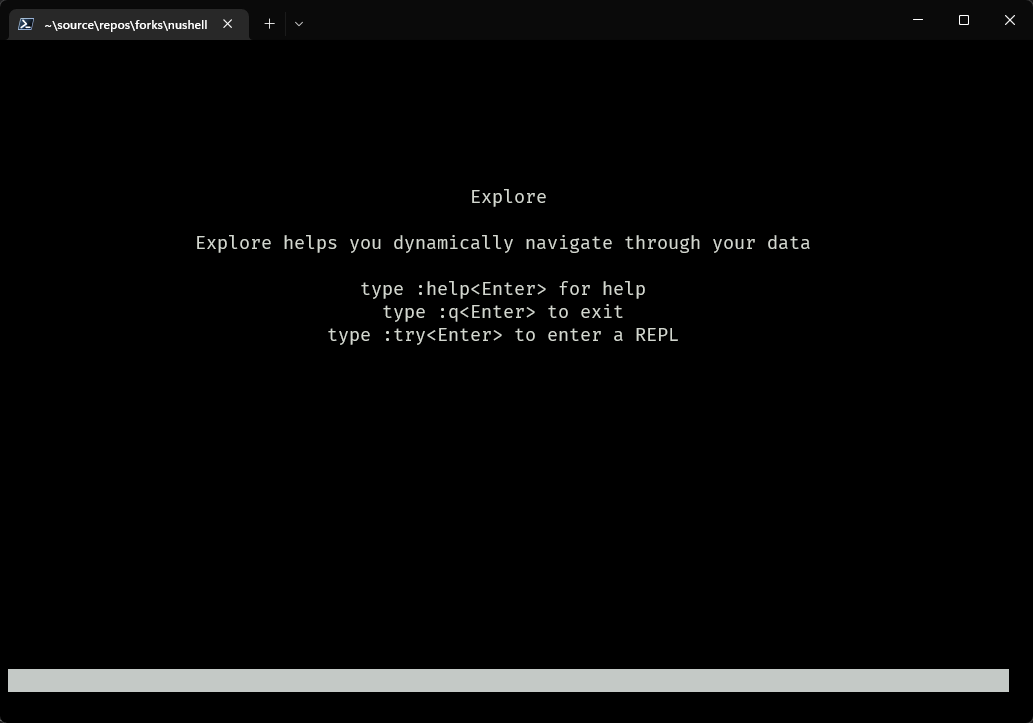
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
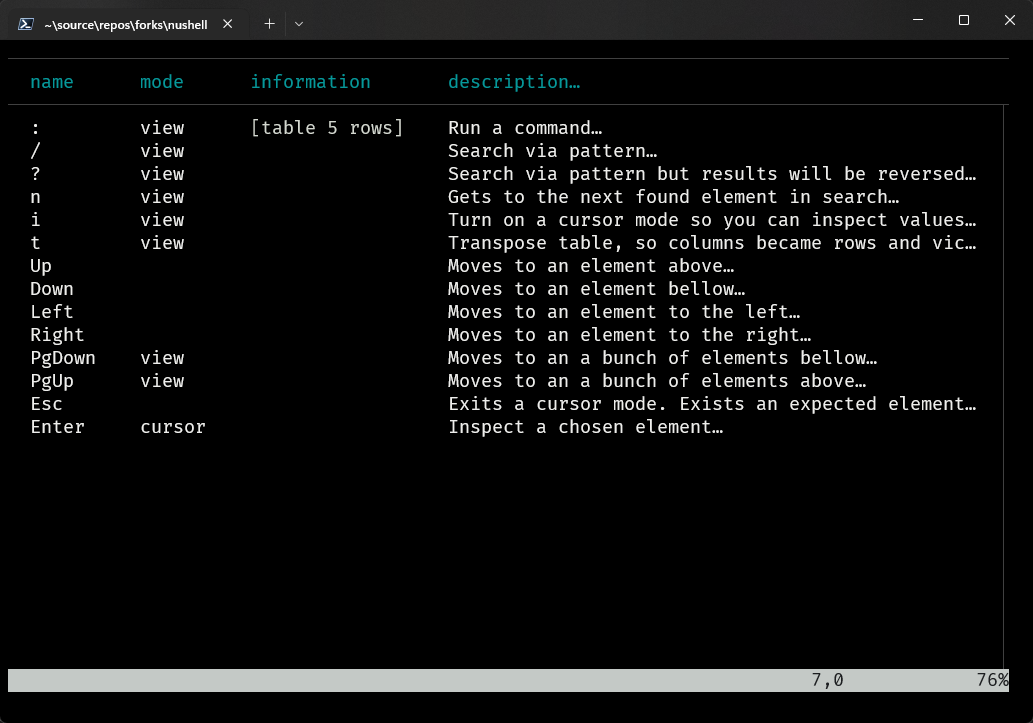
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
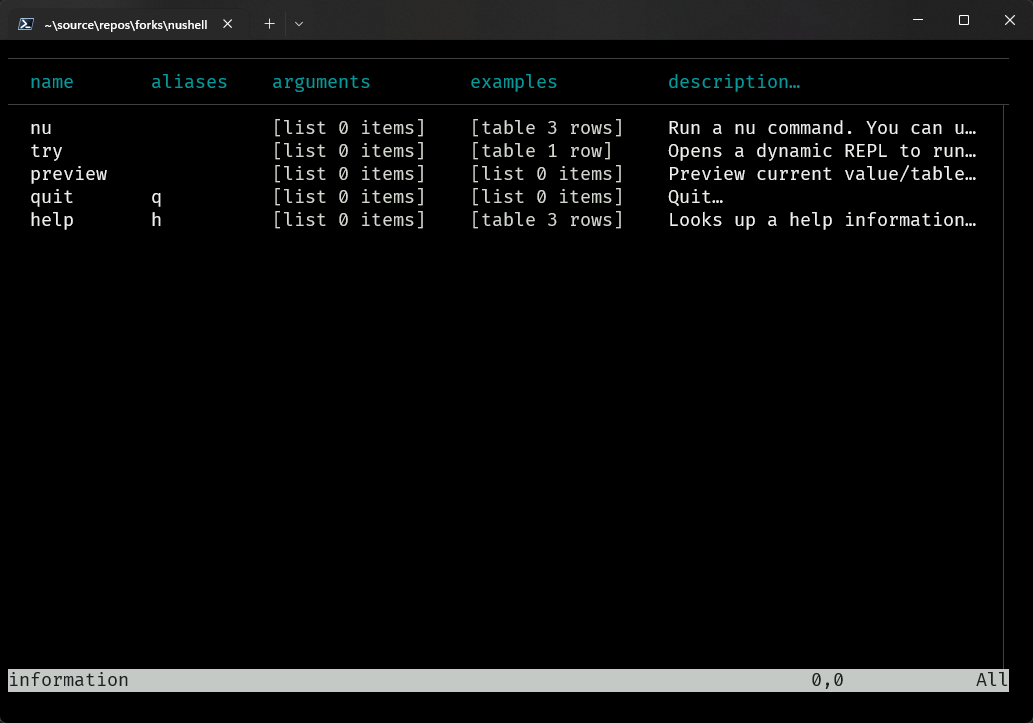
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
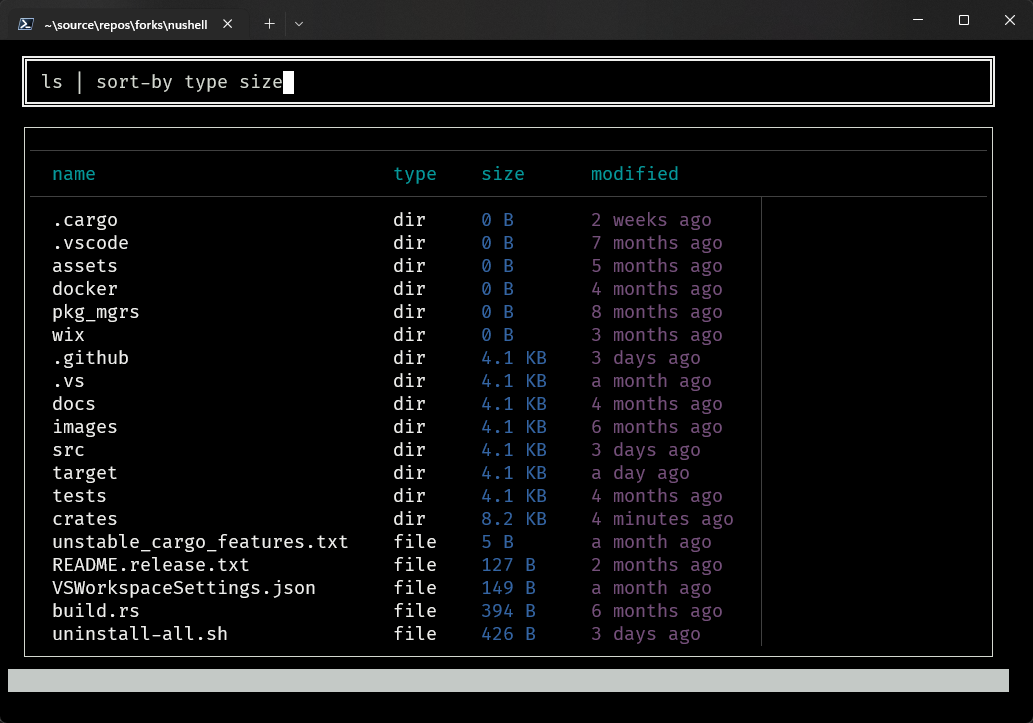
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
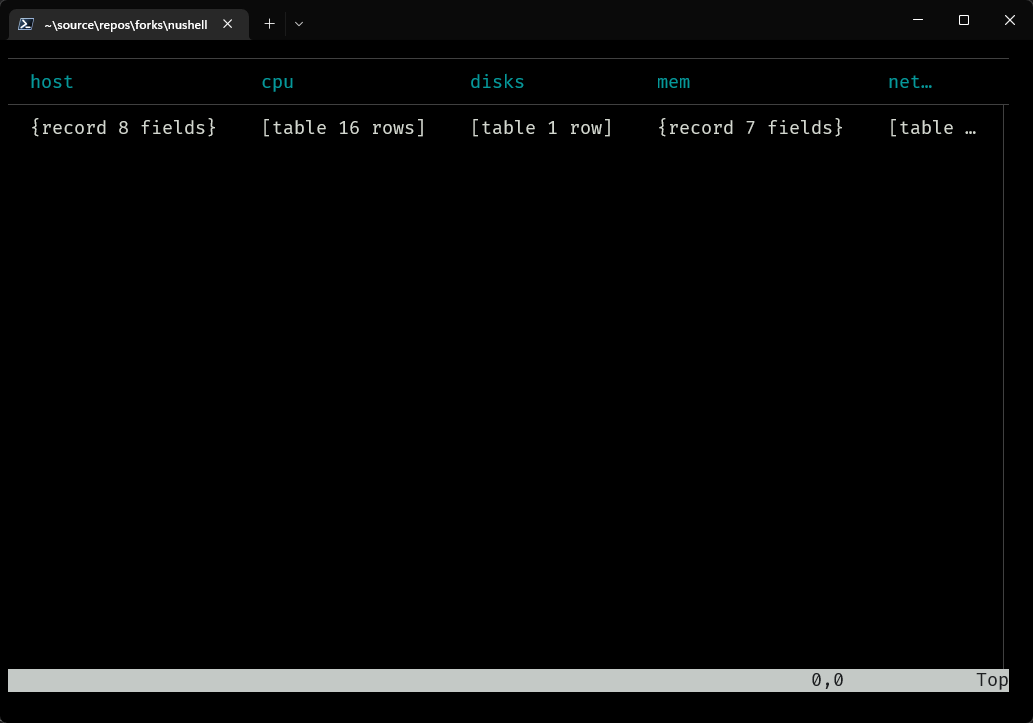
If you hit the `t` key it will now transpose the view to look like this.
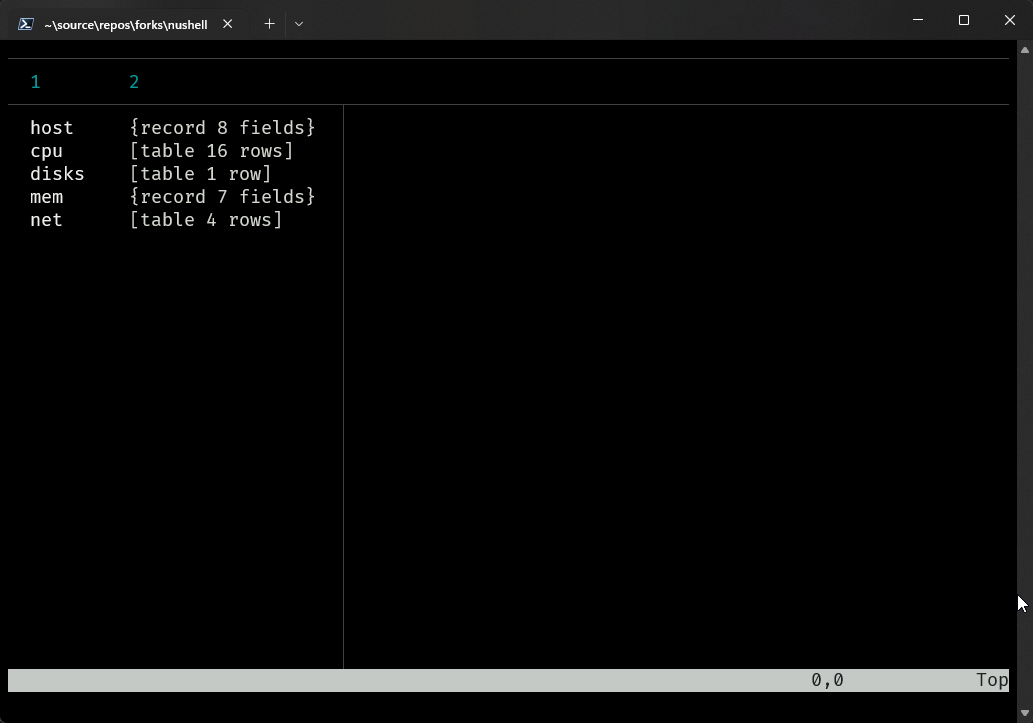
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
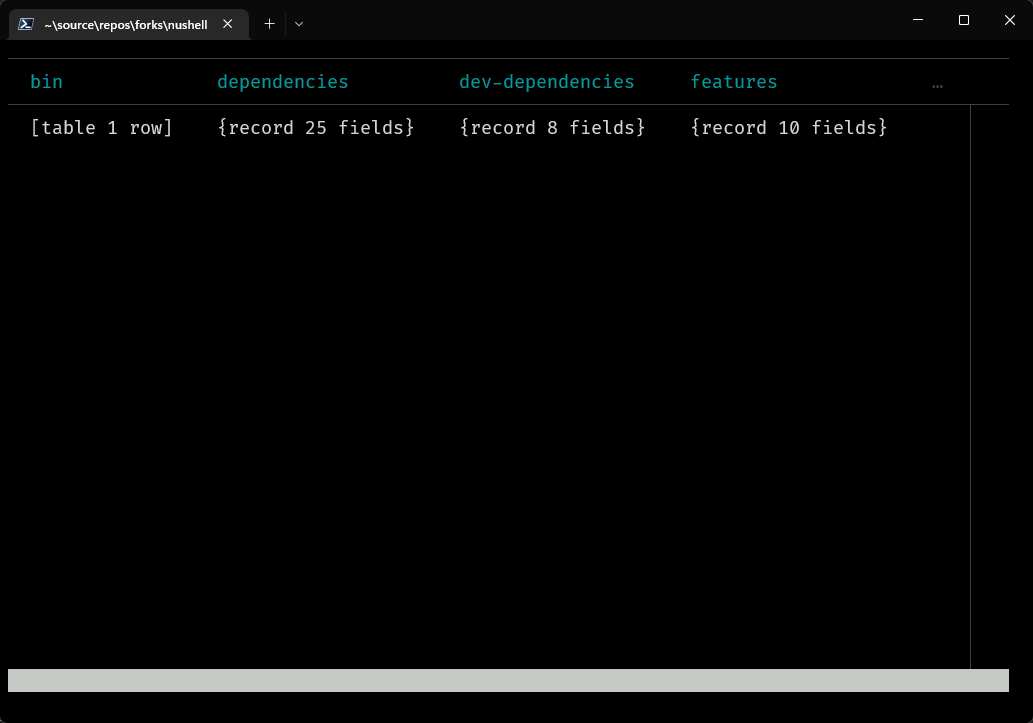
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
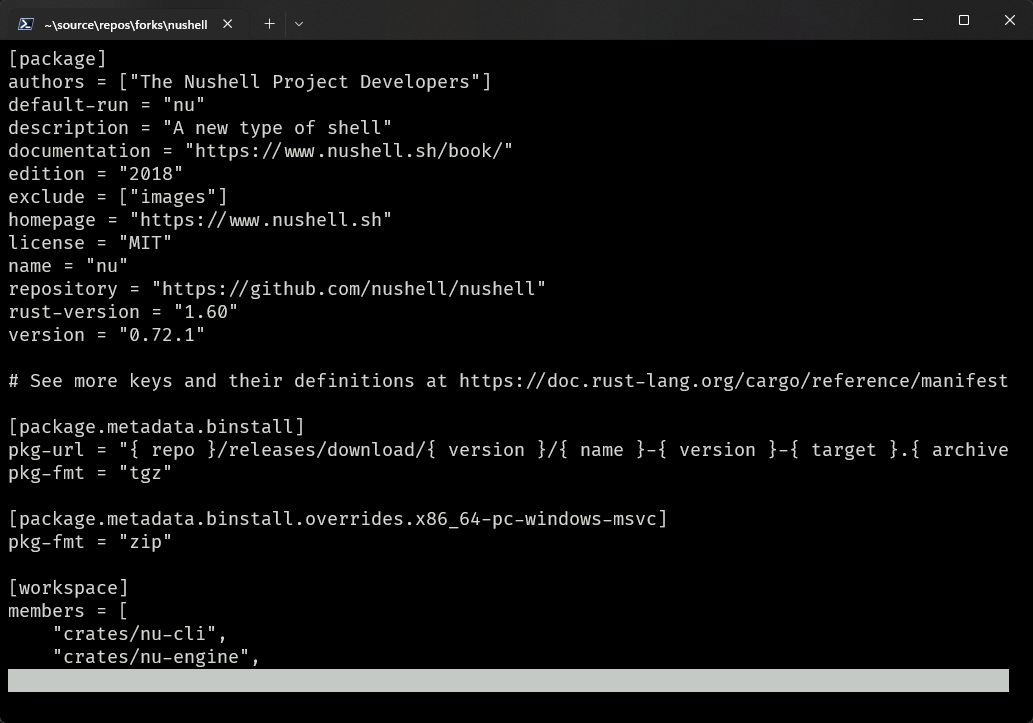
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
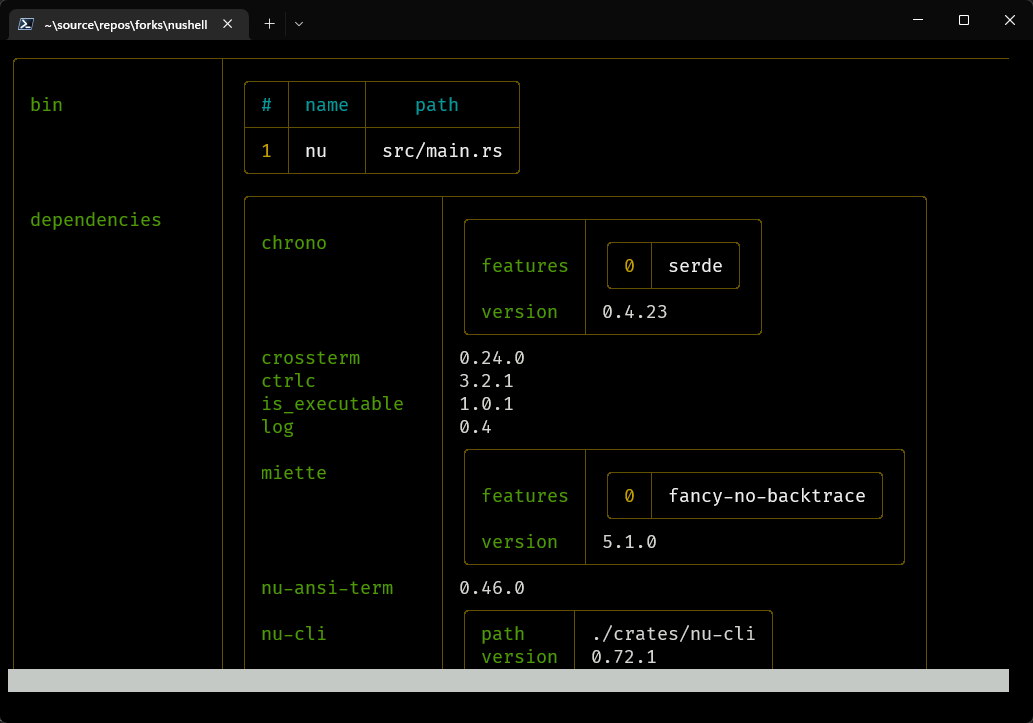
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
result: None,
|
|
|
|
},
|
|
|
|
Example {
|
2022-12-02 14:01:02 +00:00
|
|
|
description: "Explore a list of Markdown files' contents, with row indexes",
|
2023-04-05 23:36:00 +00:00
|
|
|
example: r#"glob *.md | each {|| open } | explore -i"#,
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
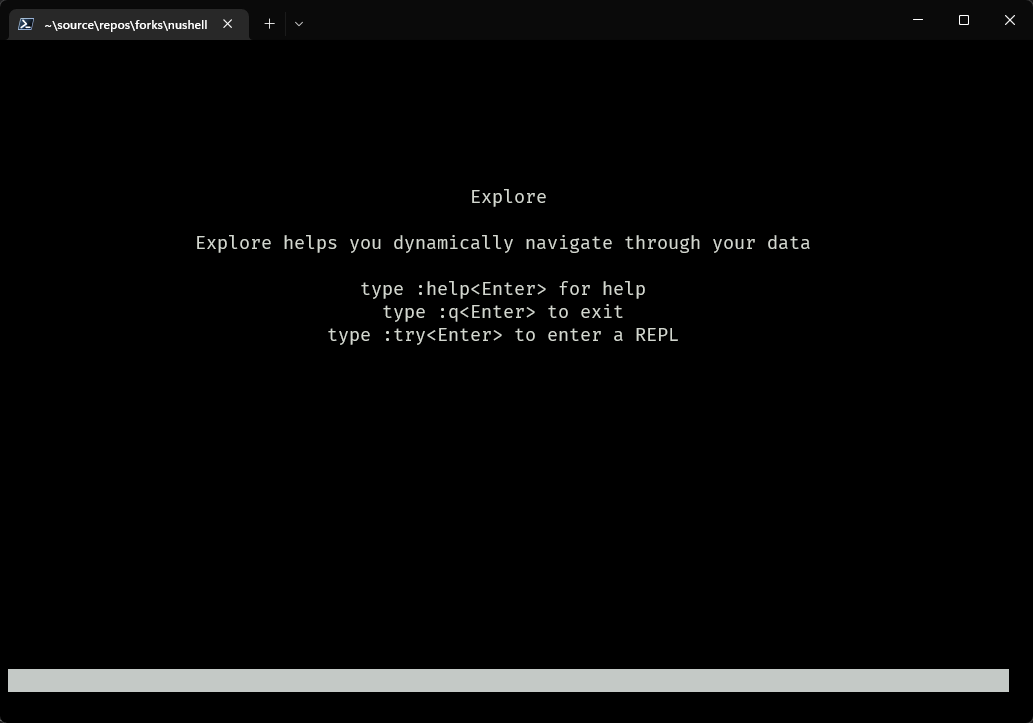
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
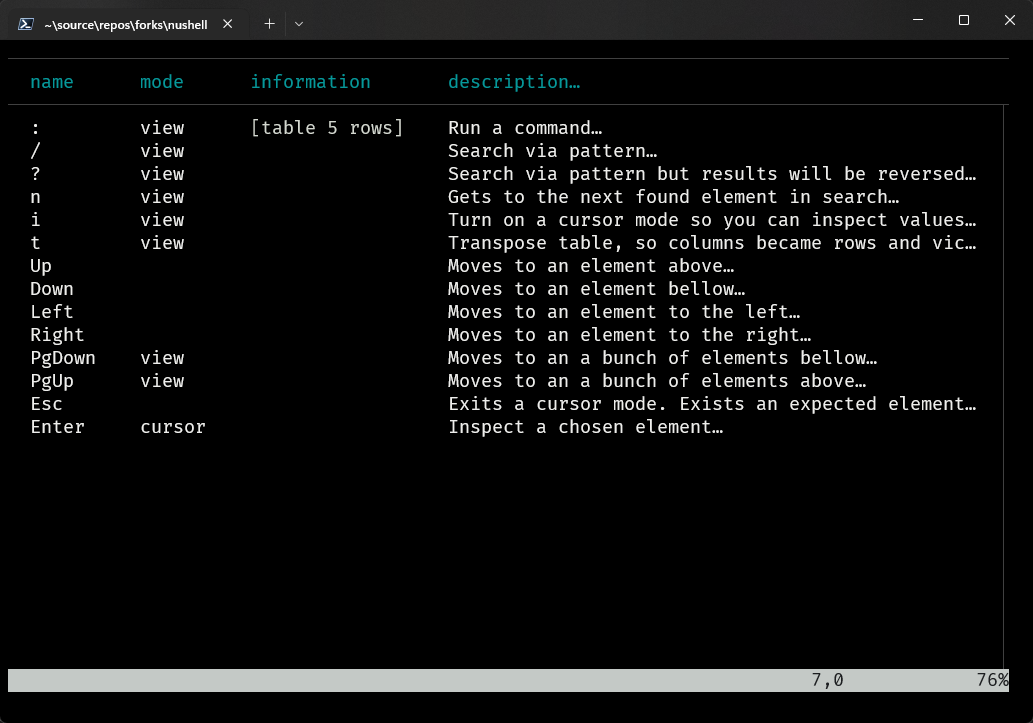
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
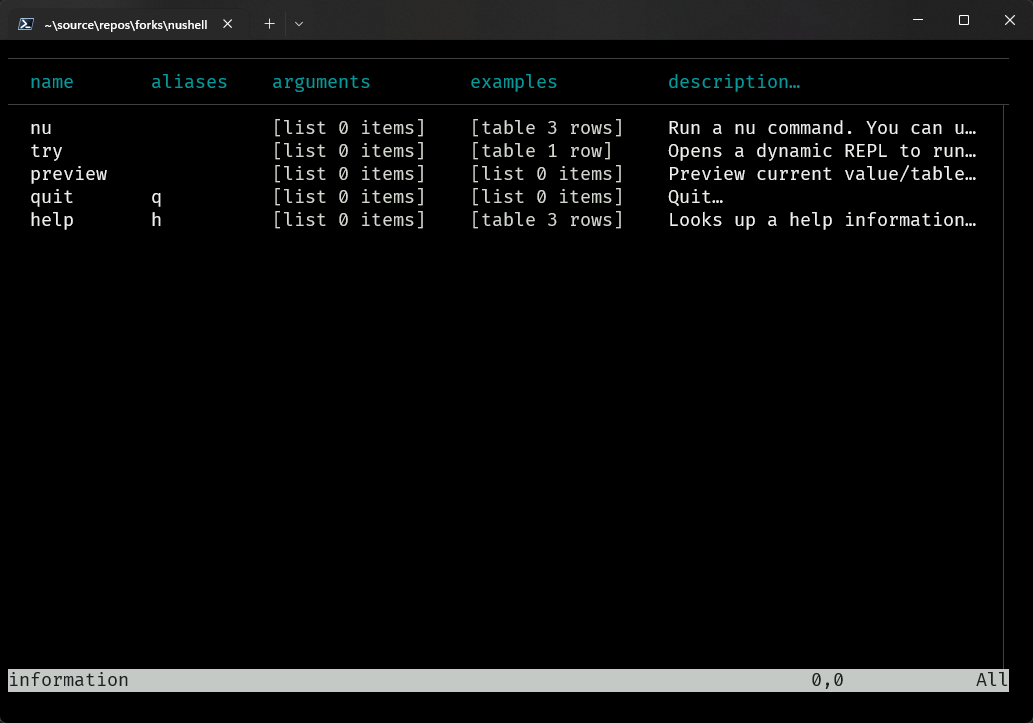
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
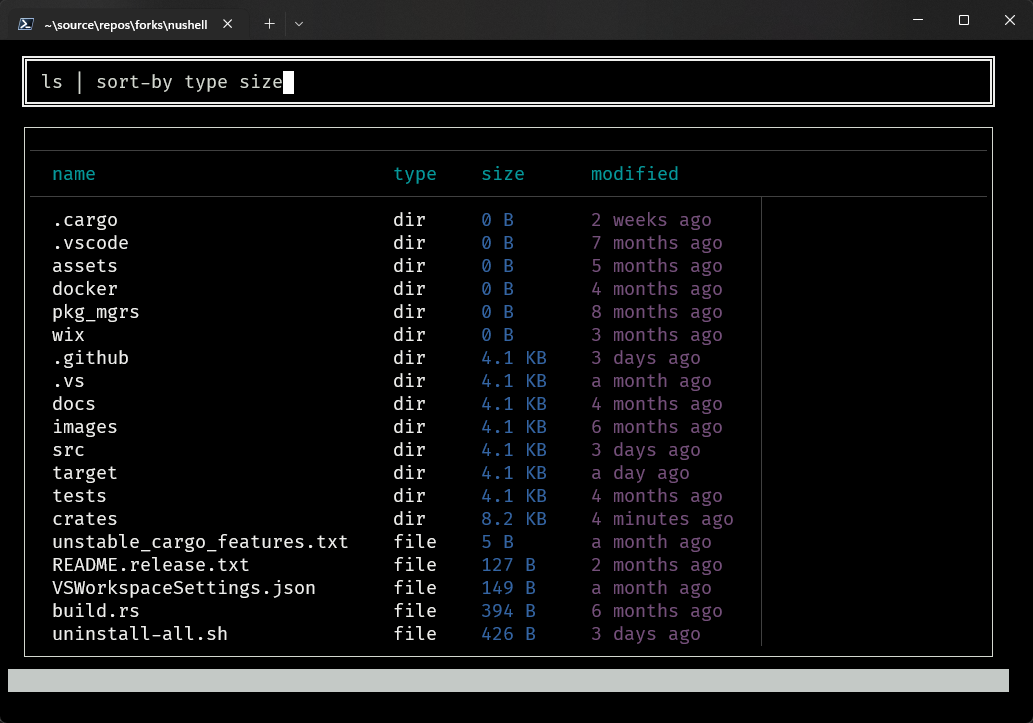
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
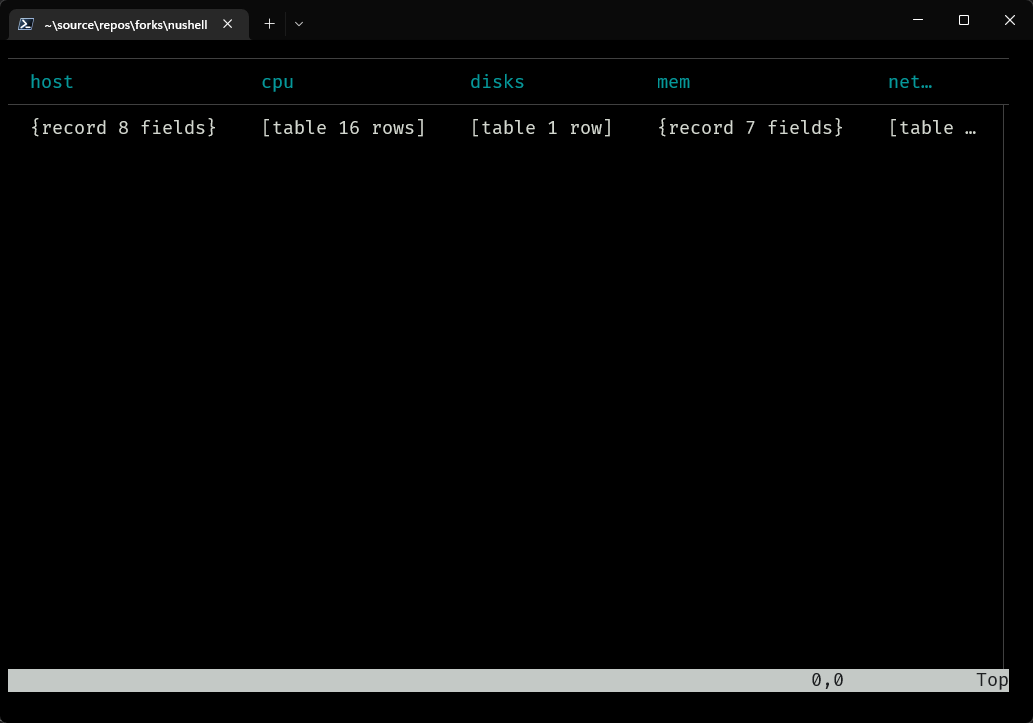
If you hit the `t` key it will now transpose the view to look like this.
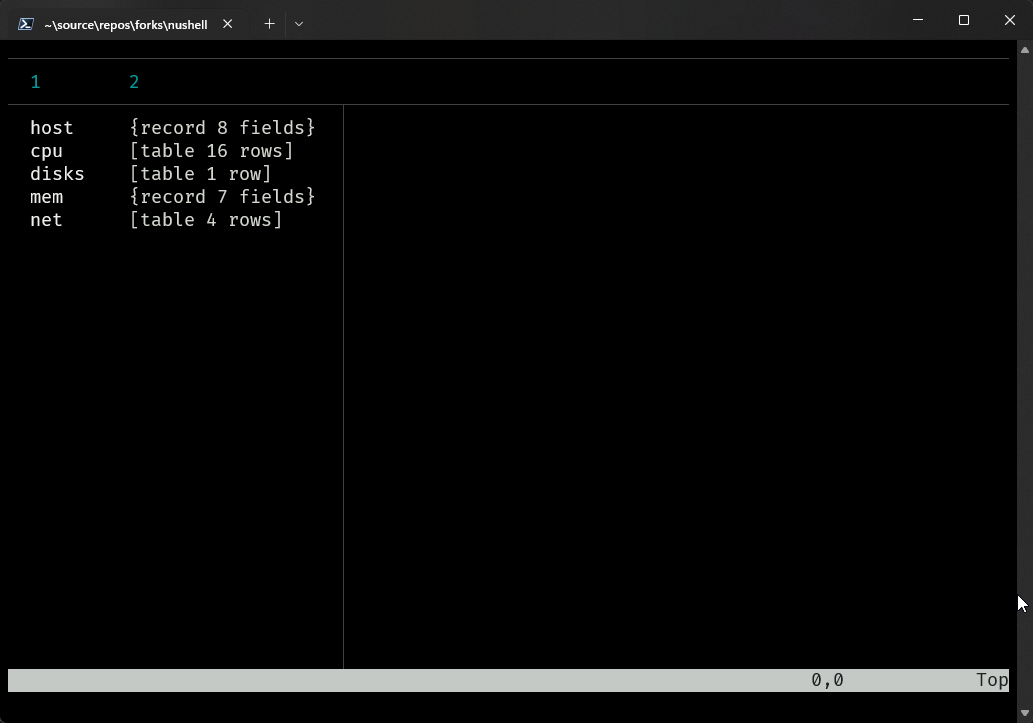
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
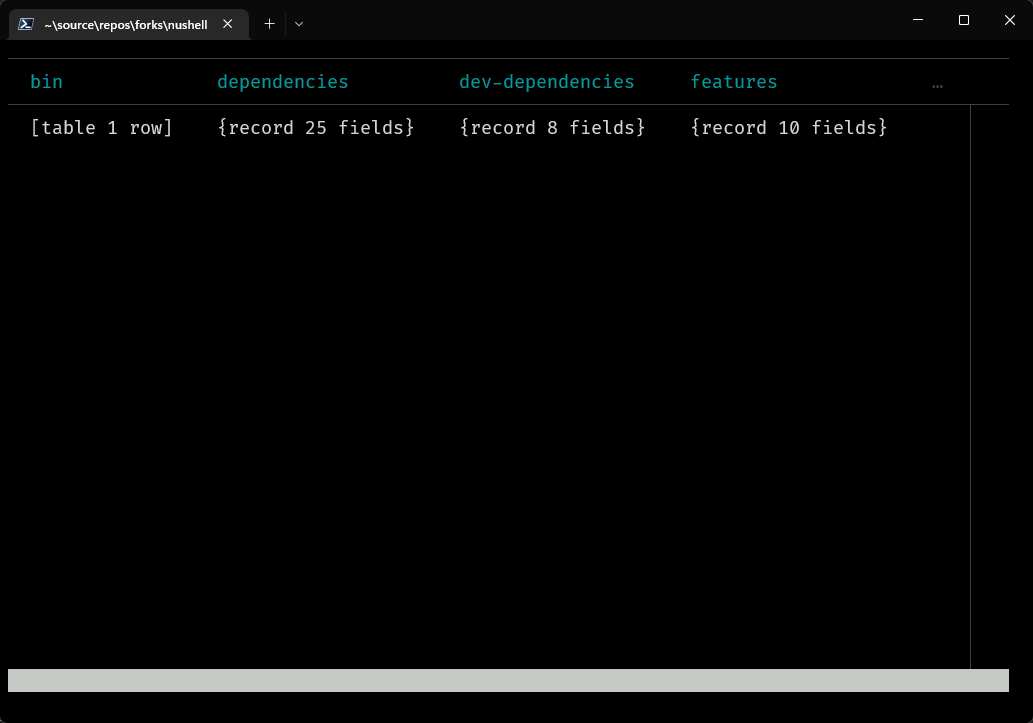
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
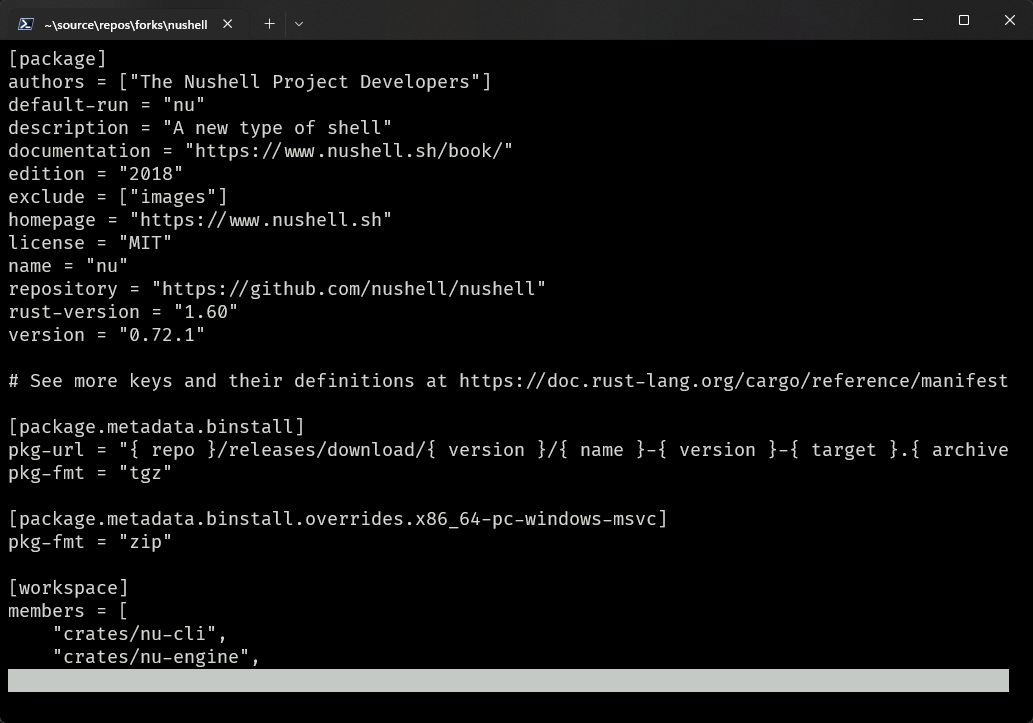
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
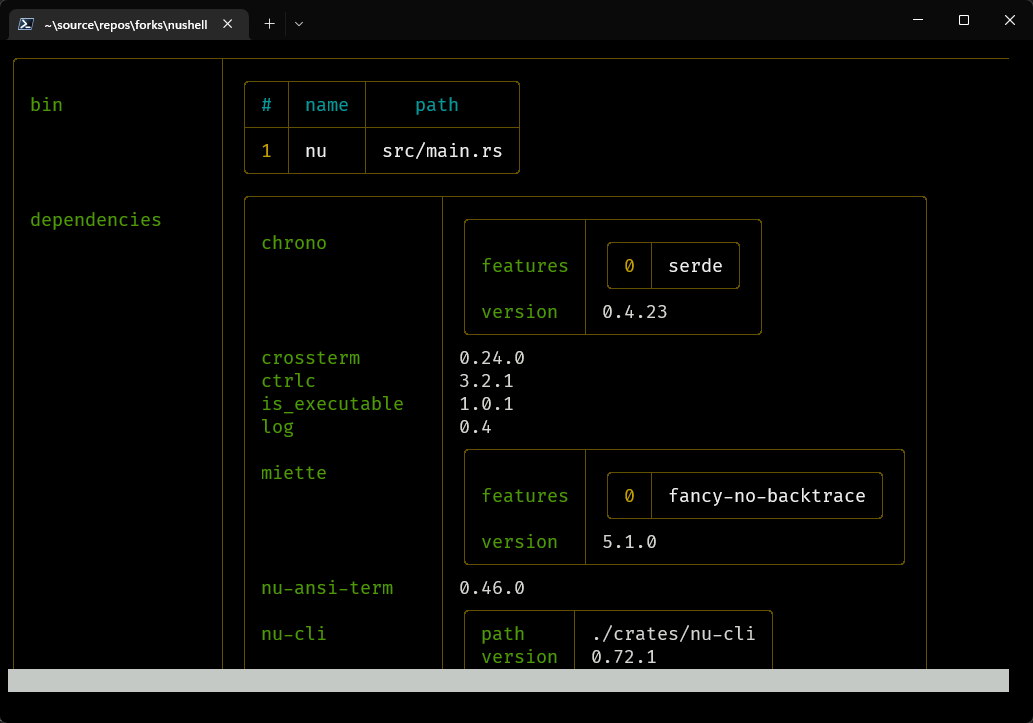
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
result: None,
|
|
|
|
},
|
|
|
|
Example {
|
2022-12-02 14:01:02 +00:00
|
|
|
description:
|
|
|
|
"Explore a JSON file, then save the last visited sub-structure to a file",
|
|
|
|
example: r#"open file.json | explore -p | to json | save part.json"#,
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
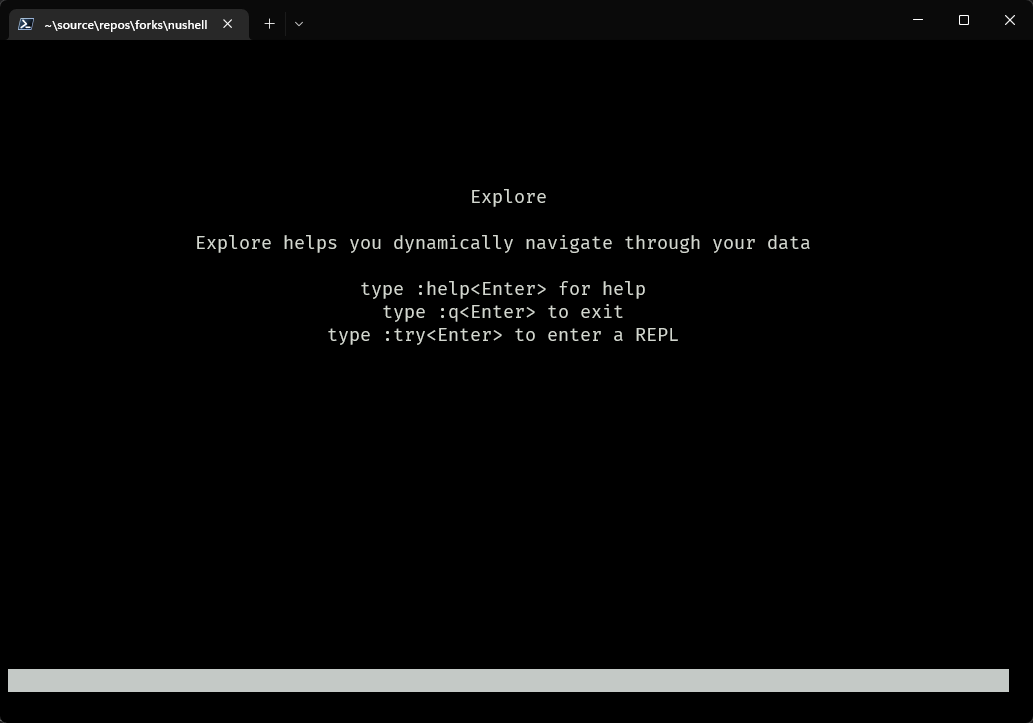
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
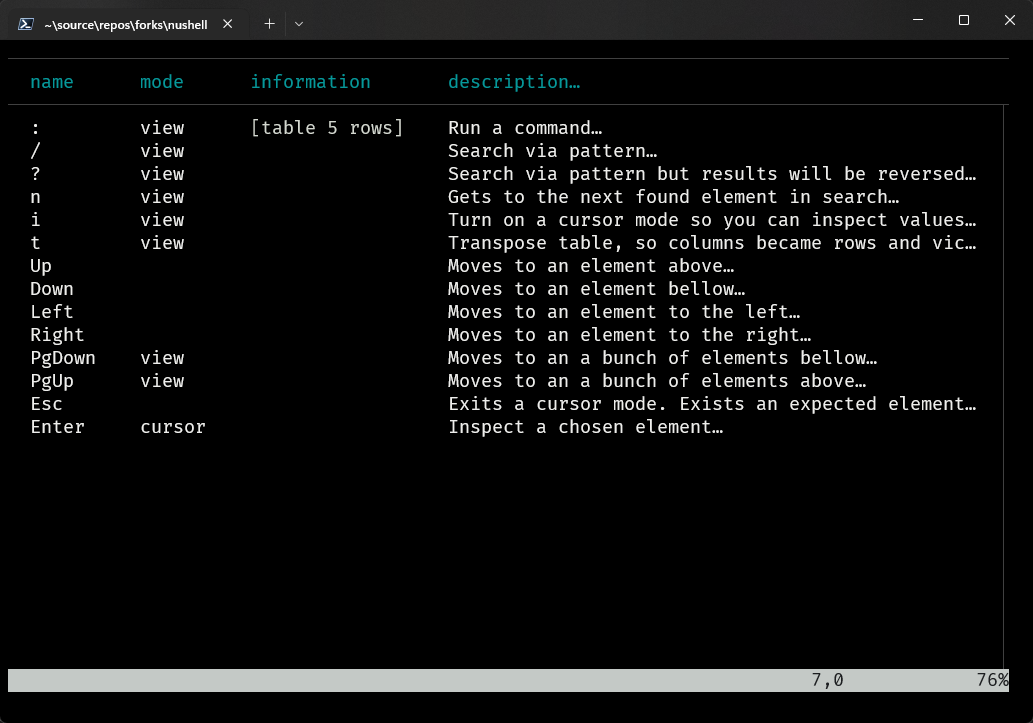
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
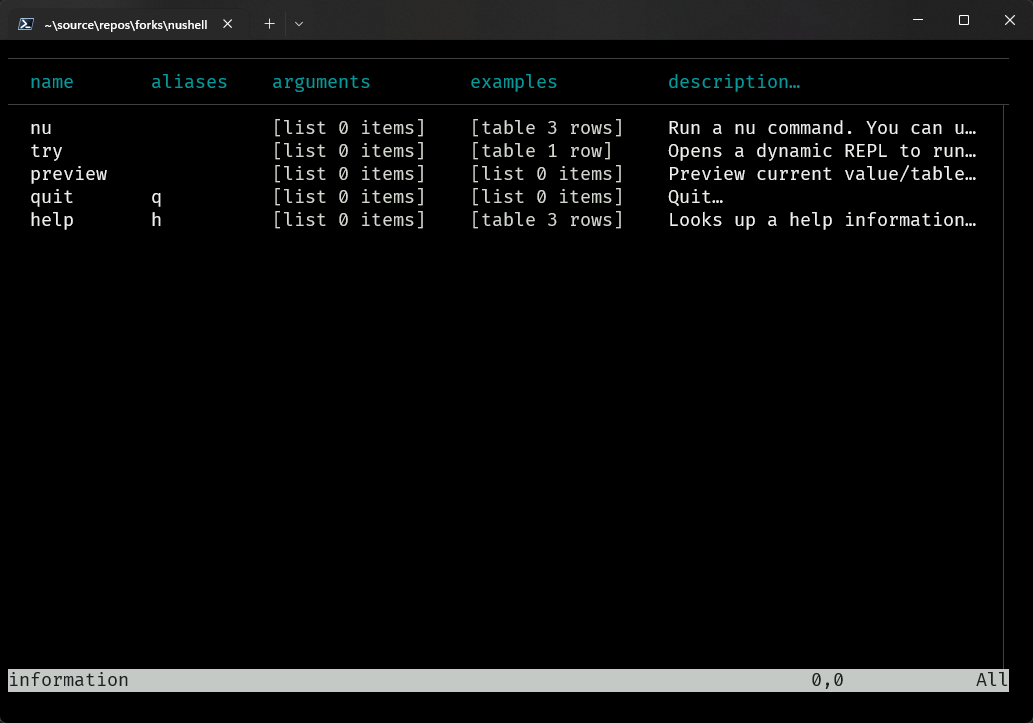
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
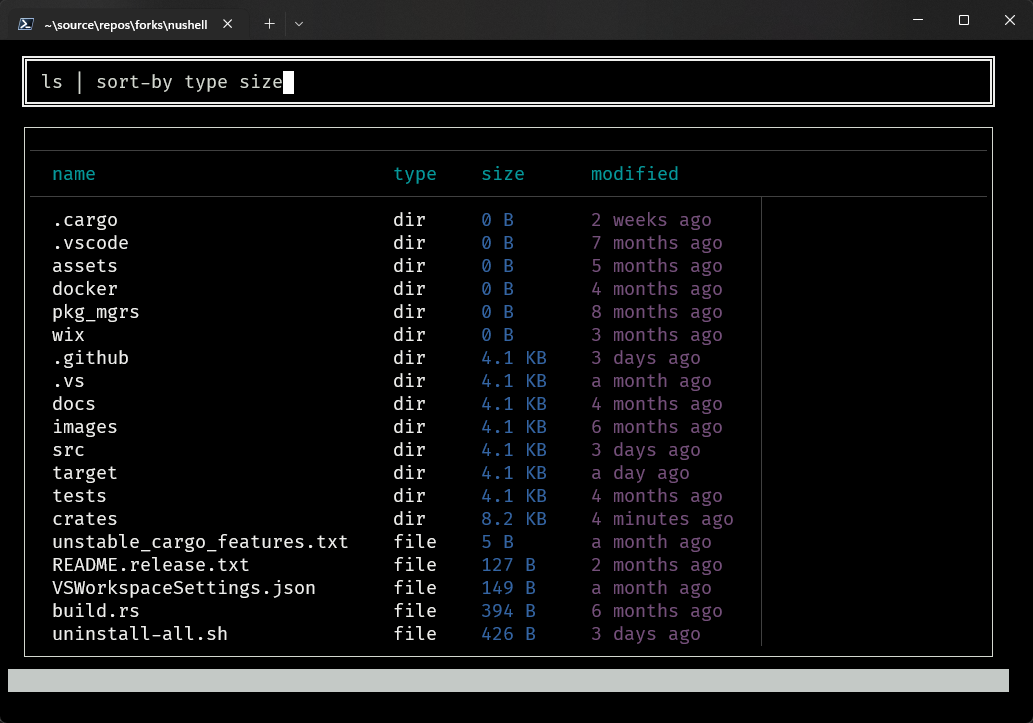
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
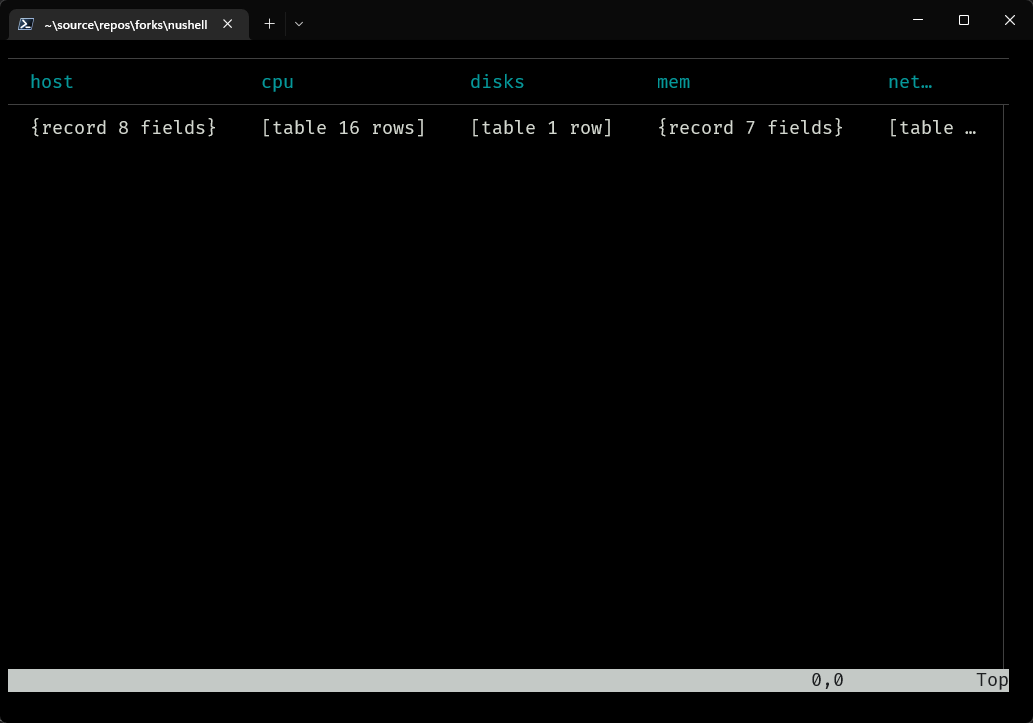
If you hit the `t` key it will now transpose the view to look like this.
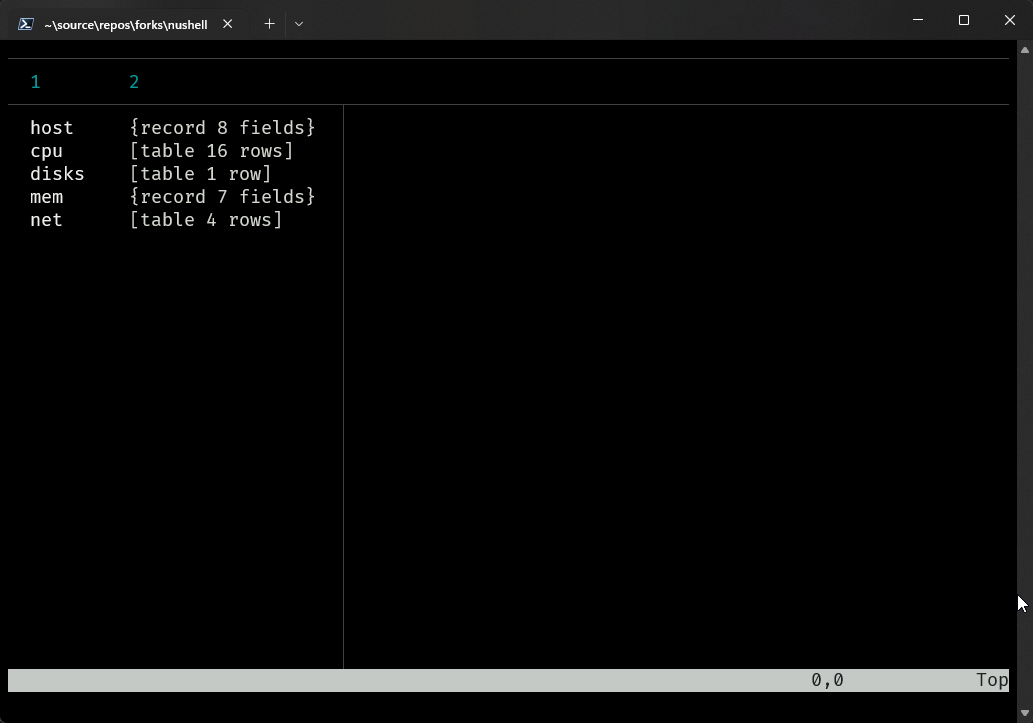
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
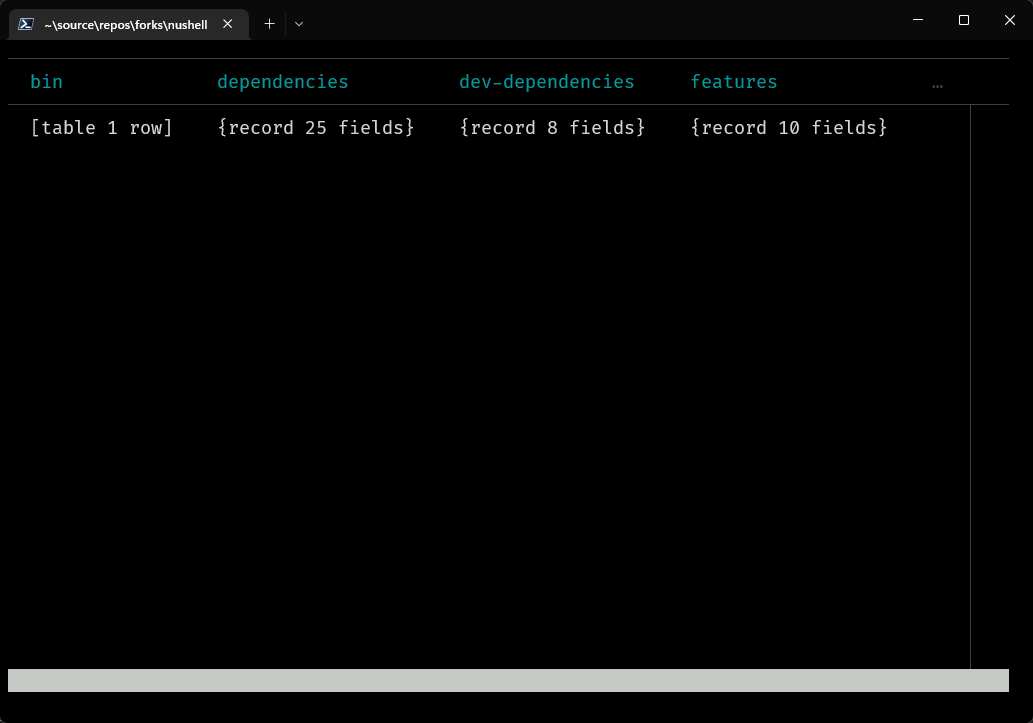
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
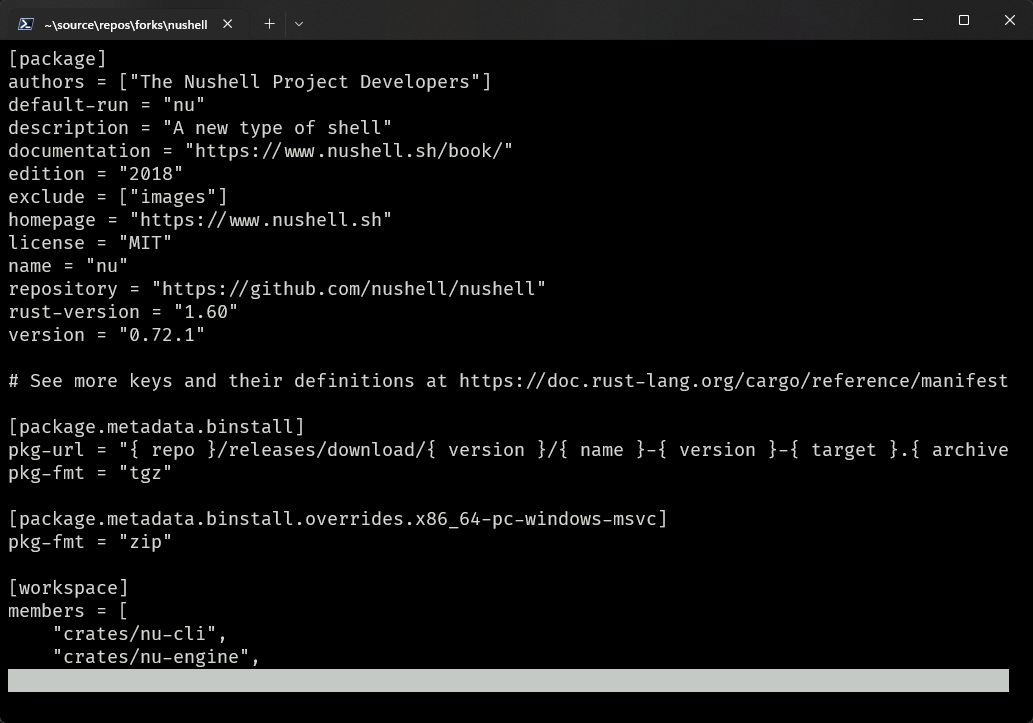
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
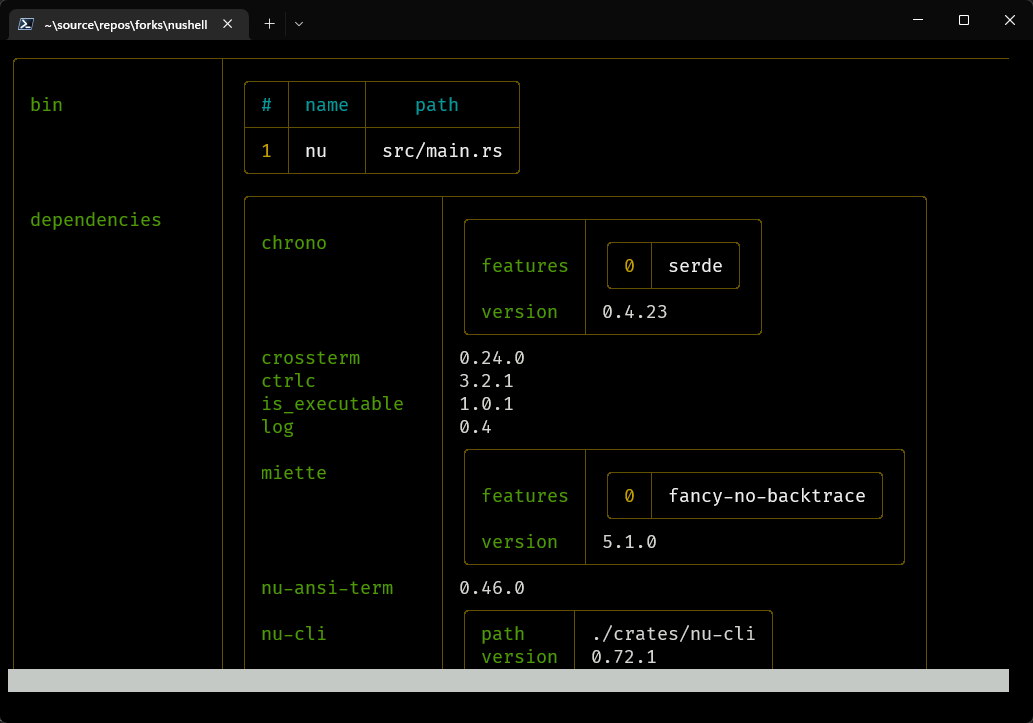
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
result: None,
|
|
|
|
},
|
|
|
|
]
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
color_config now accepts closures as color values (#7141)
# Description
Closes #6909. You can now add closures to your `color_config` themes.
Whenever a value would be printed with `table`, the closure is run with
the value piped-in. The closure must return either a {fg,bg,attr} record
or a color name (`'light_red'` etc.). This returned style is used to
colour the value.
This is entirely backwards-compatible with existing config.nu files.
Example code excerpt:
```
let my_theme = {
header: green_bold
bool: { if $in { 'light_cyan' } else { 'light_red' } }
int: purple_bold
filesize: { |e| if $e == 0b { 'gray' } else if $e < 1mb { 'purple_bold' } else { 'cyan_bold' } }
duration: purple_bold
date: { (date now) - $in | if $in > 1wk { 'cyan_bold' } else if $in > 1day { 'green_bold' } else { 'yellow_bold' } }
range: yellow_bold
string: { if $in =~ '^#\w{6}$' { $in } else { 'white' } }
nothing: white
```
Example output with this in effect:
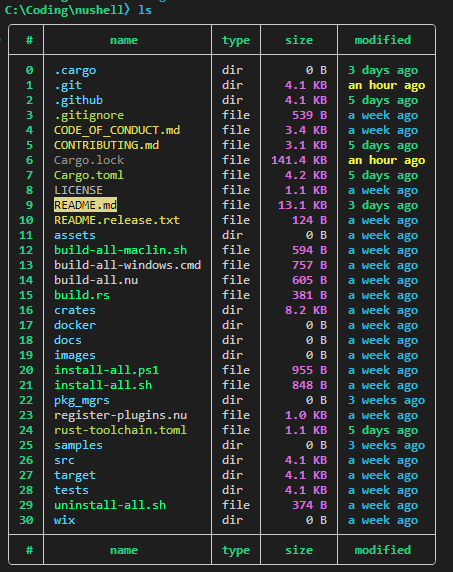
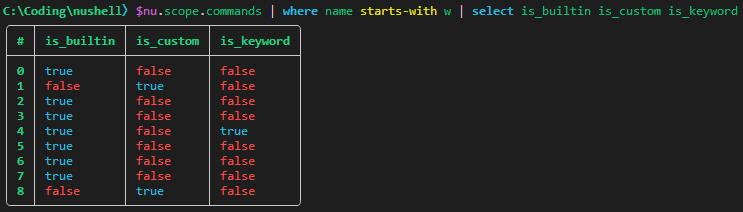
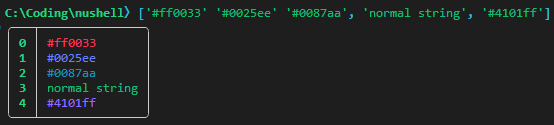
Slightly important notes:
* Some color_config names, namely "separator", "empty" and "hints", pipe
in `null` instead of a value.
* Currently, doing anything non-trivial inside a closure has an
understandably big perf hit. I currently do not actually recommend
something like `string: { if $in =~ '^#\w{6}$' { $in } else { 'white' }
}` for serious work, mainly because of the abundance of string-type data
in the world. Nevertheless, lesser-used types like "date" and "duration"
work well with this.
* I had to do some reorganisation in order to make it possible to call
`eval_block()` that late in table rendering. I invented a new struct
called "StyleComputer" which holds the engine_state and stack of the
initial `table` command (implicit or explicit).
* StyleComputer has a `compute()` method which takes a color_config name
and a nu value, and always returns the correct Style, so you don't have
to worry about A) the color_config value was set at all, B) whether it
was set to a closure or not, or C) which default style to use in those
cases.
* Currently, errors encountered during execution of the closures are
thrown in the garbage. Any other ideas are welcome. (Nonetheless, errors
result in a huge perf hit when they are encountered. I think what should
be done is to assume something terrible happened to the user's config
and invalidate the StyleComputer for that `table` run, thus causing
subsequent output to just be Style::default().)
* More thorough tests are forthcoming - ran into some difficulty using
`nu!` to take an alternative config, and for some reason `let-env config
=` statements don't seem to work inside `nu!` pipelines(???)
* The default config.nu has not been updated to make use of this yet. Do
tell if you think I should incorporate that into this.
# User-Facing Changes
See above.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace --features=extra -- -D warnings -D
clippy::unwrap_used -A clippy::needless_collect` to check that you're
using the standard code style
- `cargo test --workspace --features=extra` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2022-12-17 13:07:56 +00:00
|
|
|
// For now, this doesn't use StyleComputer.
|
|
|
|
// As such, closures can't be given as styles for Explore.
|
2022-12-16 15:47:07 +00:00
|
|
|
fn is_need_banner(config: &HashMap<String, Value>) -> Option<bool> {
|
|
|
|
config.get("help_banner").and_then(|v| v.as_bool().ok())
|
|
|
|
}
|
|
|
|
|
|
|
|
fn is_need_esc_exit(config: &HashMap<String, Value>) -> Option<bool> {
|
|
|
|
config.get("exit_esc").and_then(|v| v.as_bool().ok())
|
|
|
|
}
|
|
|
|
|
|
|
|
fn update_config(config: &mut HashMap<String, Value>, show_index: bool, show_head: bool) {
|
|
|
|
let mut hm = config.get("table").and_then(create_map).unwrap_or_default();
|
|
|
|
if show_index {
|
|
|
|
insert_bool(&mut hm, "show_index", show_index);
|
|
|
|
}
|
|
|
|
|
|
|
|
if show_head {
|
|
|
|
insert_bool(&mut hm, "show_head", show_head);
|
|
|
|
}
|
|
|
|
|
|
|
|
config.insert(String::from("table"), map_into_value(hm));
|
|
|
|
}
|
|
|
|
|
|
|
|
fn style_from_config(config: &HashMap<String, Value>) -> StyleConfig {
|
|
|
|
let mut style = StyleConfig::default();
|
|
|
|
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
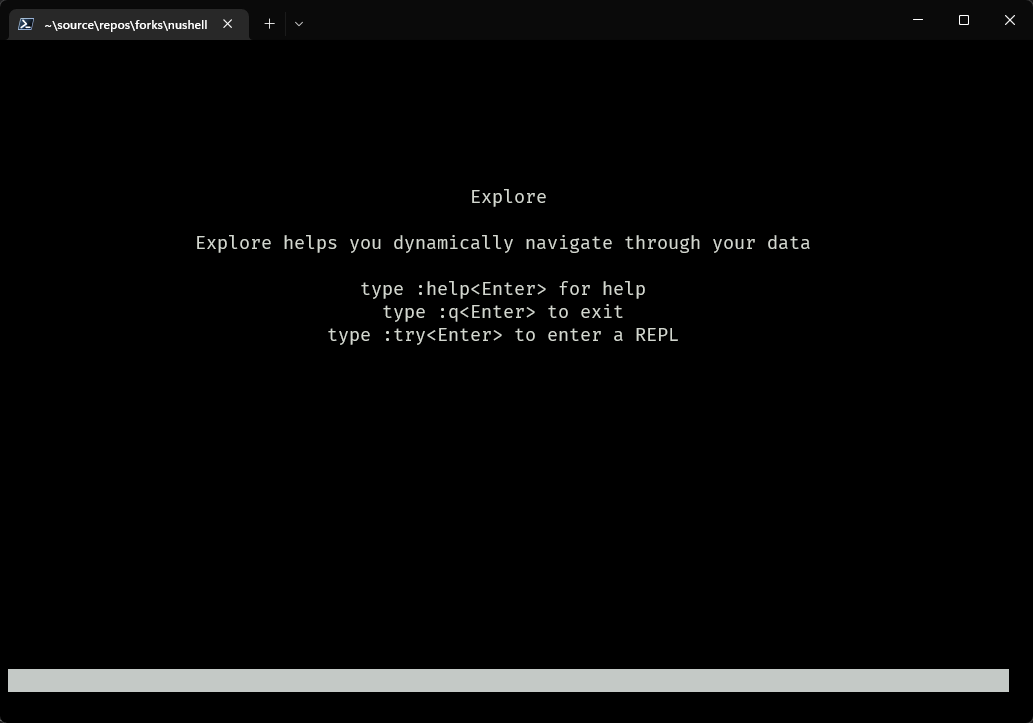
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
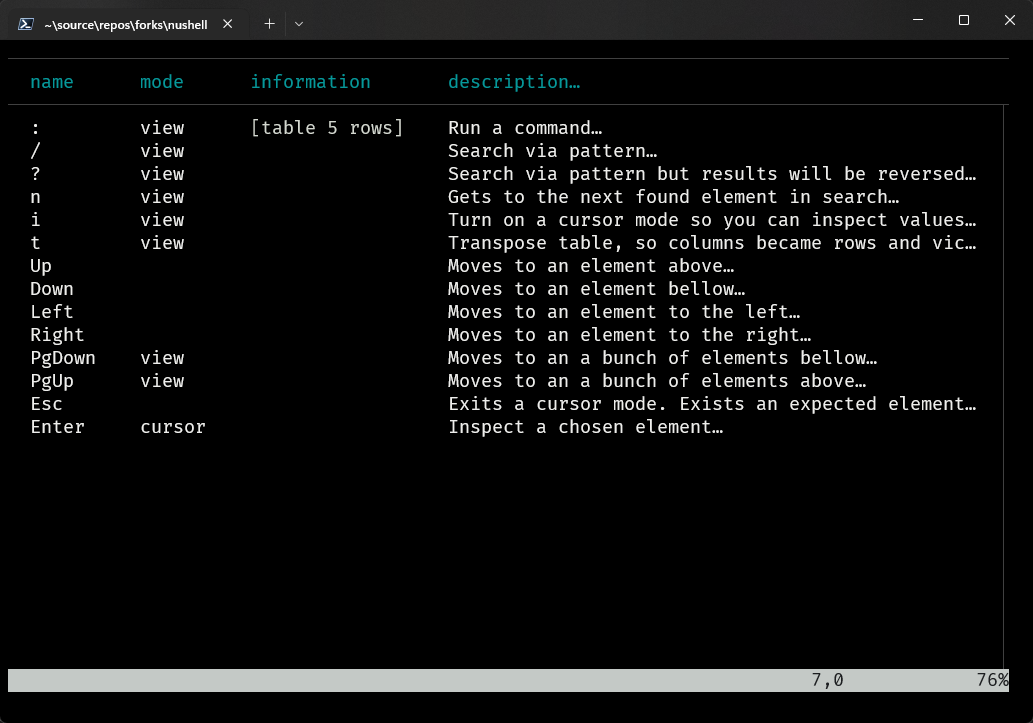
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
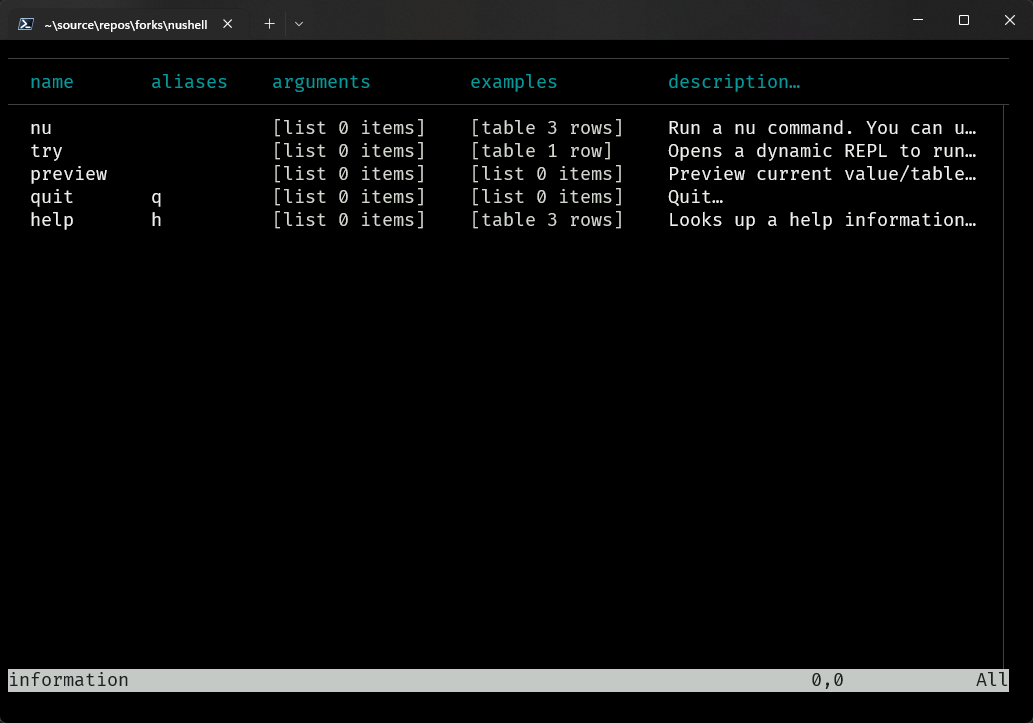
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
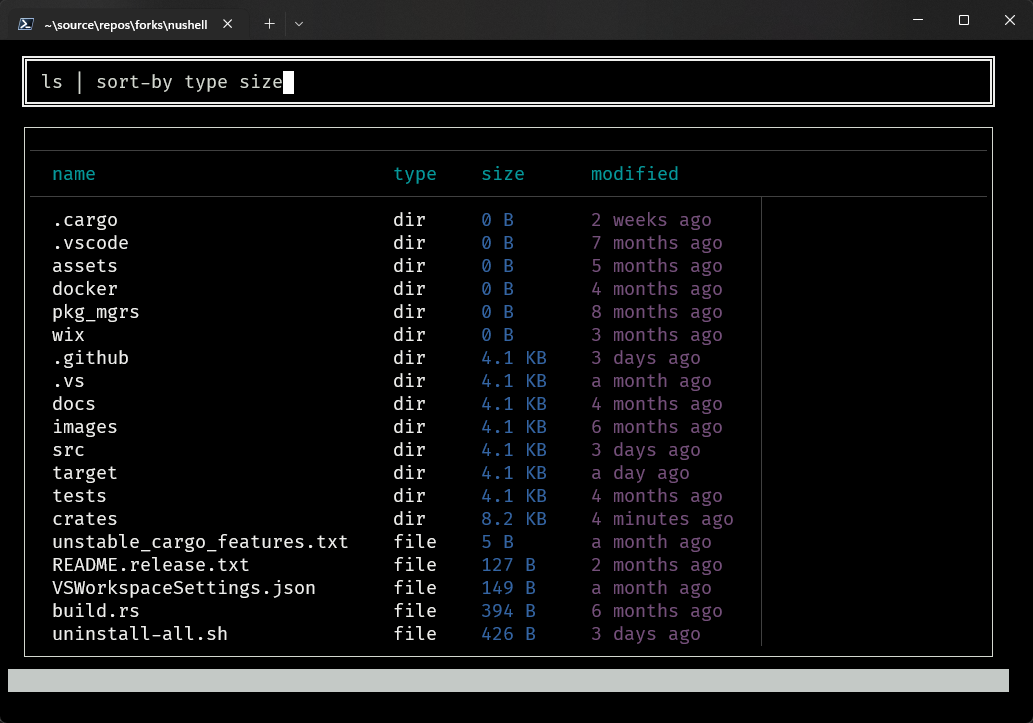
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
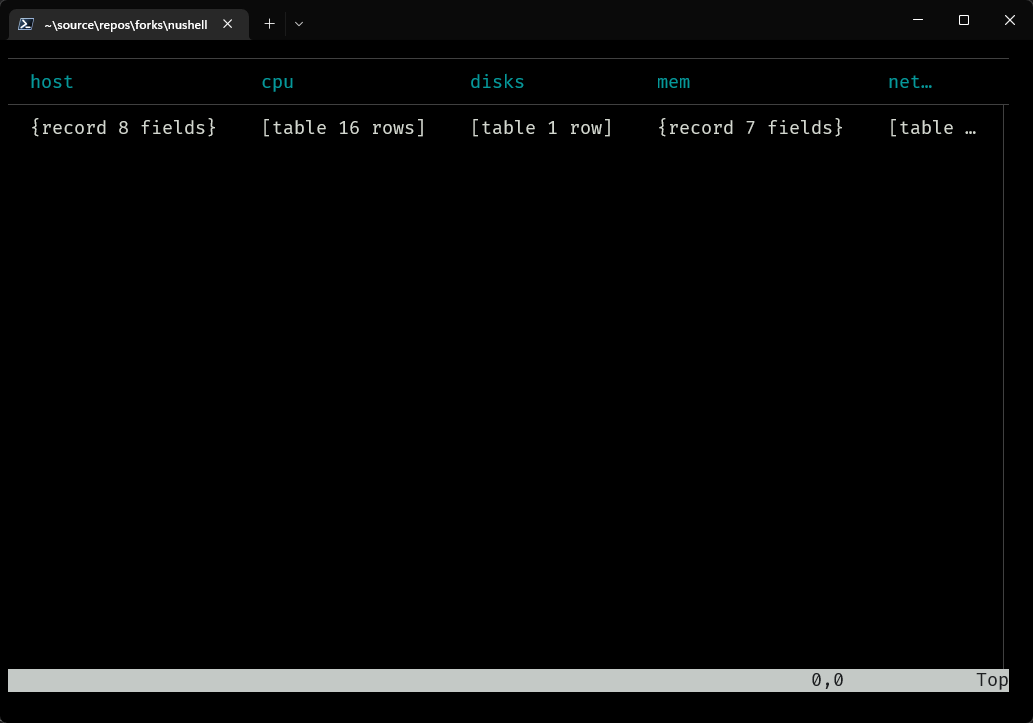
If you hit the `t` key it will now transpose the view to look like this.
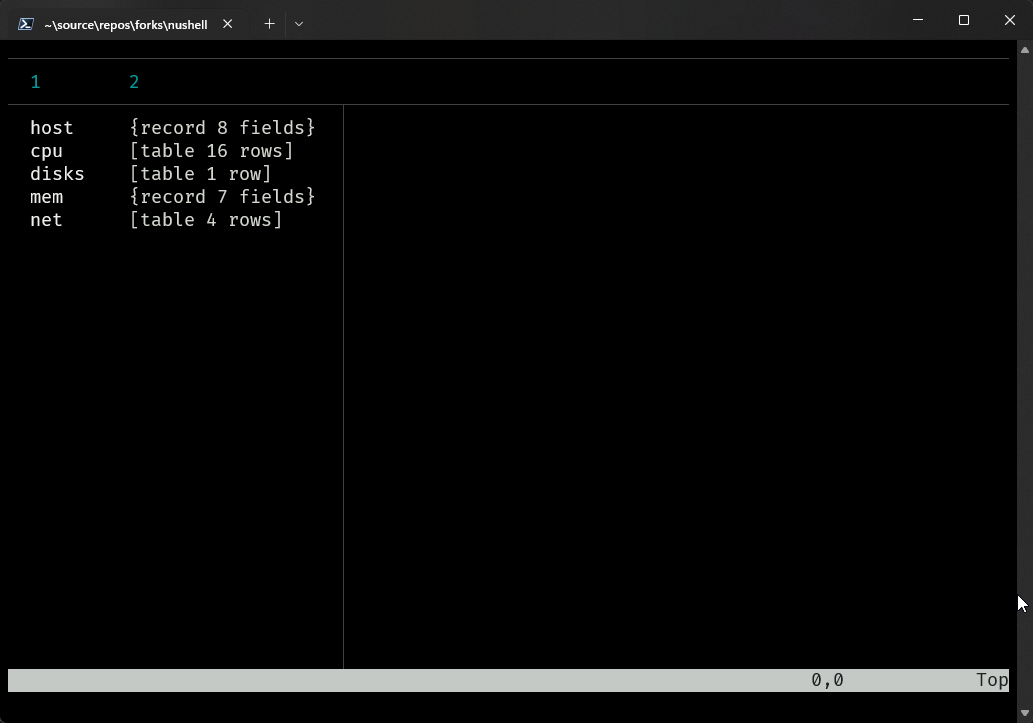
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
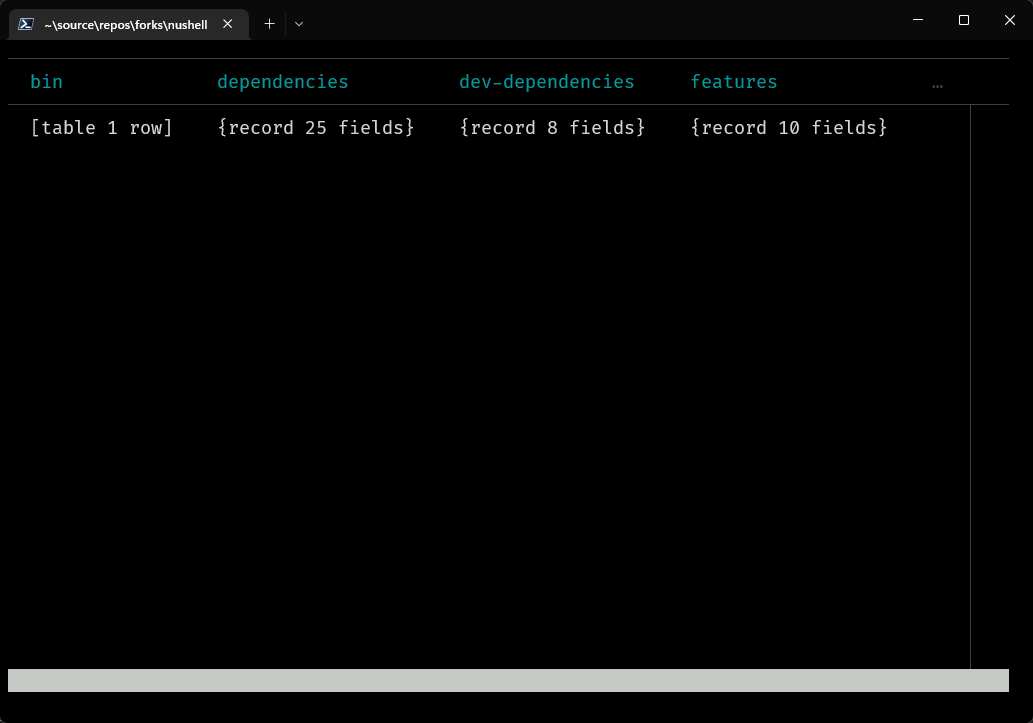
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
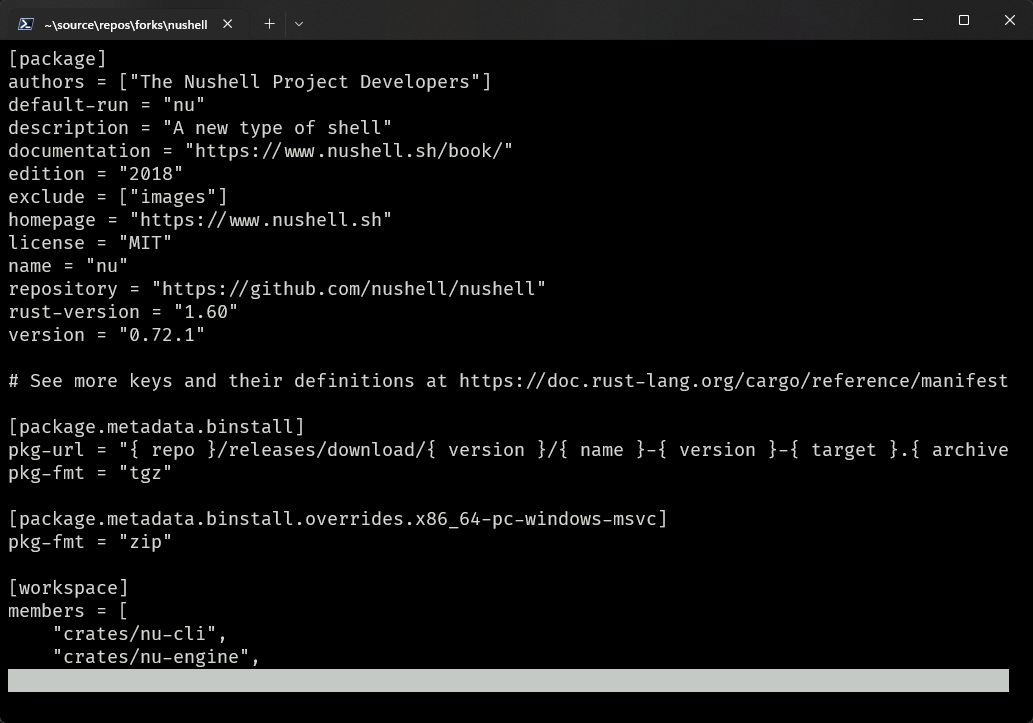
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
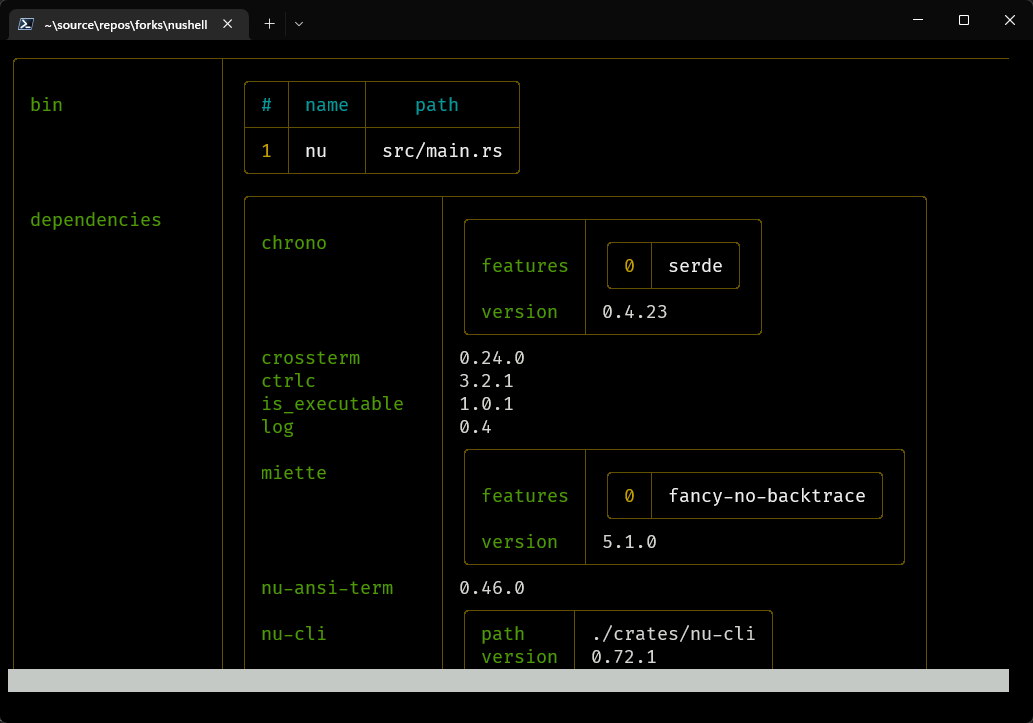
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
let colors = get_color_map(config);
|
|
|
|
|
2022-12-16 15:47:07 +00:00
|
|
|
if let Some(s) = colors.get("status_bar_text") {
|
|
|
|
style.status_bar_text = *s;
|
|
|
|
}
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
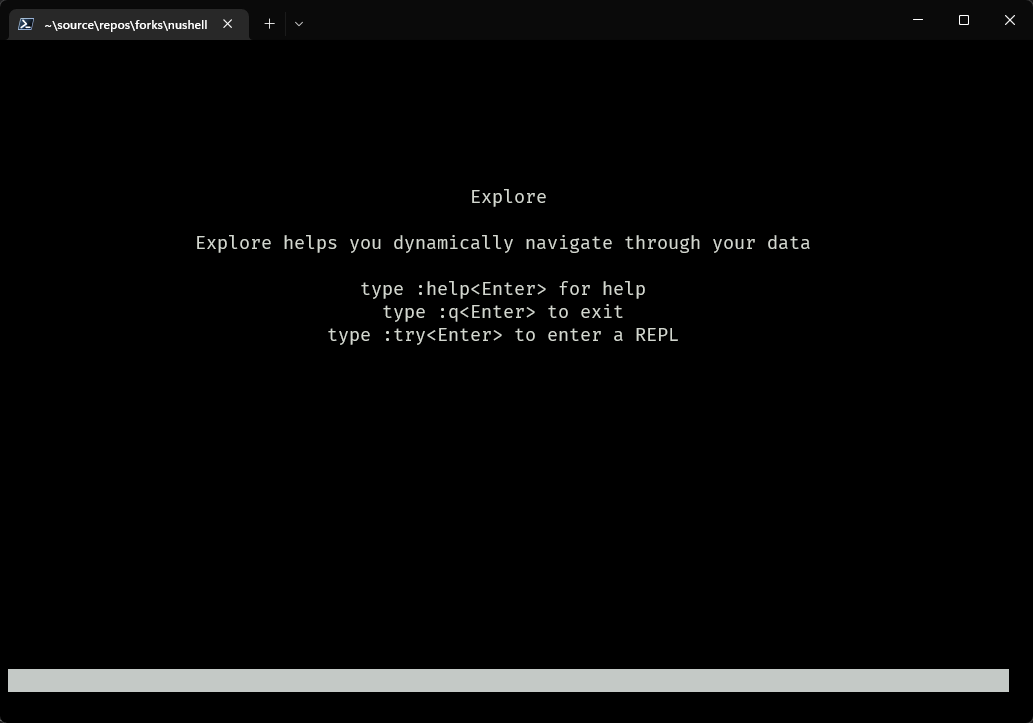
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
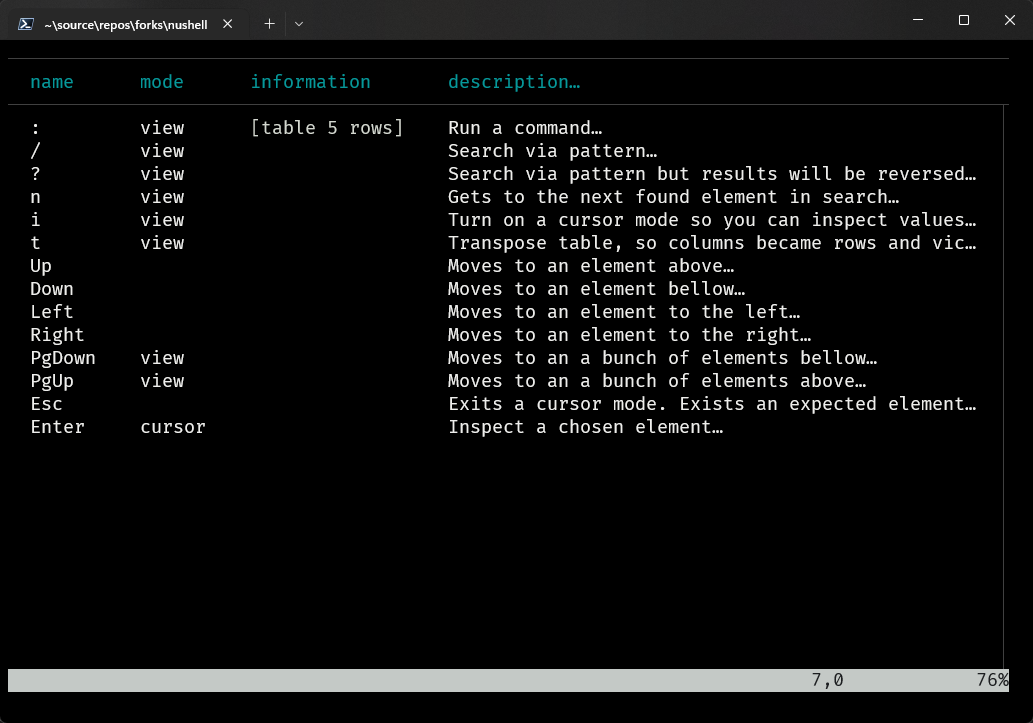
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
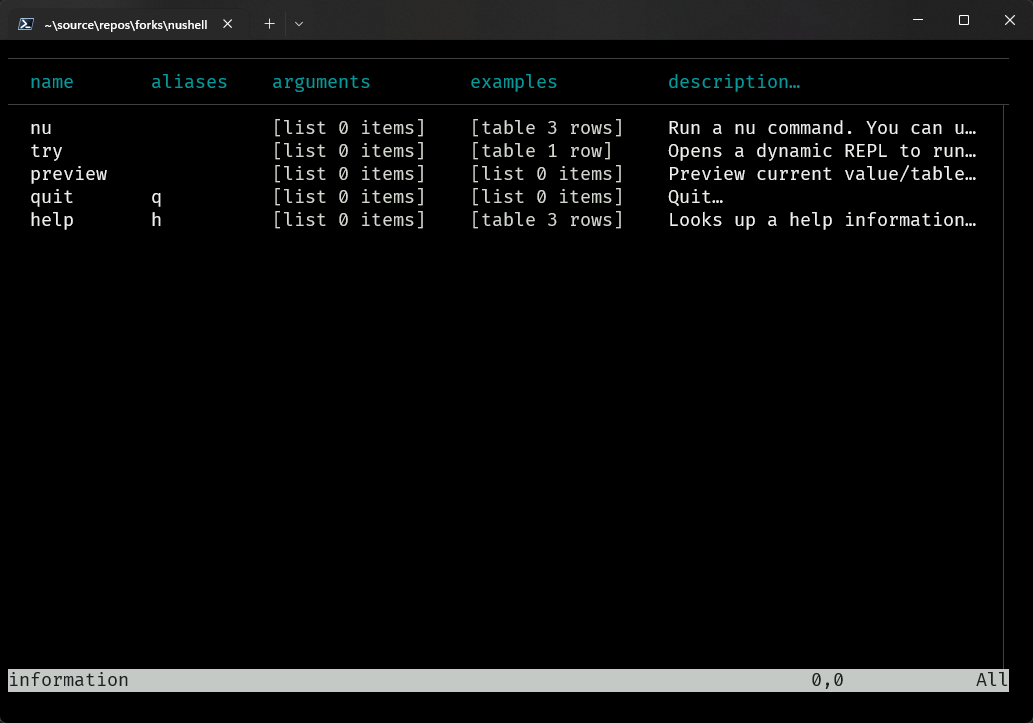
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
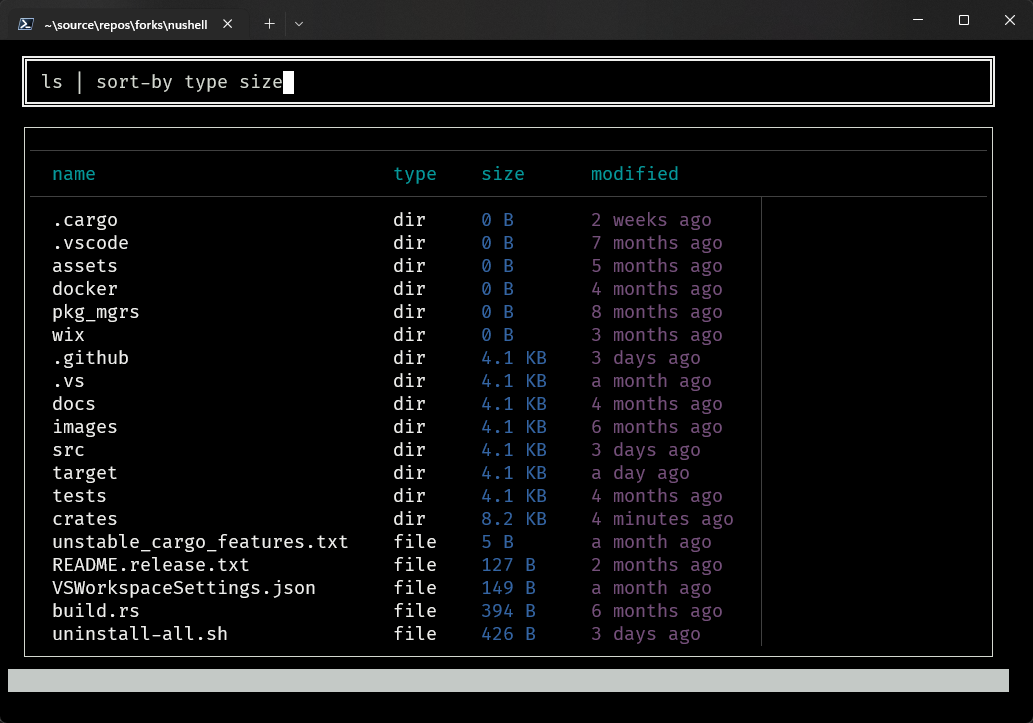
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
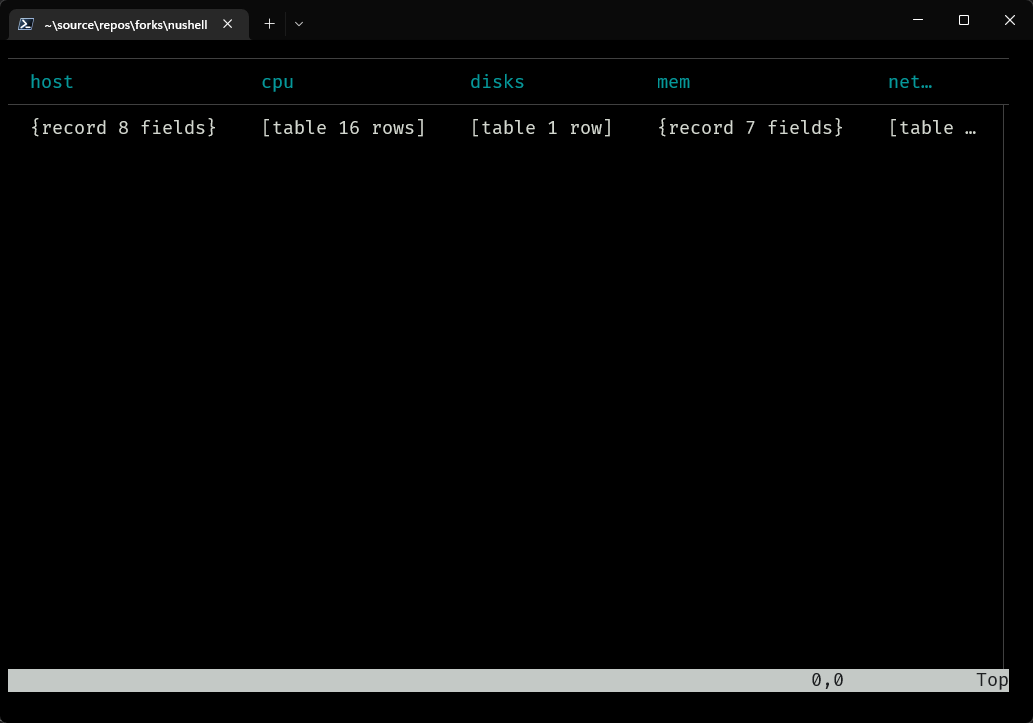
If you hit the `t` key it will now transpose the view to look like this.
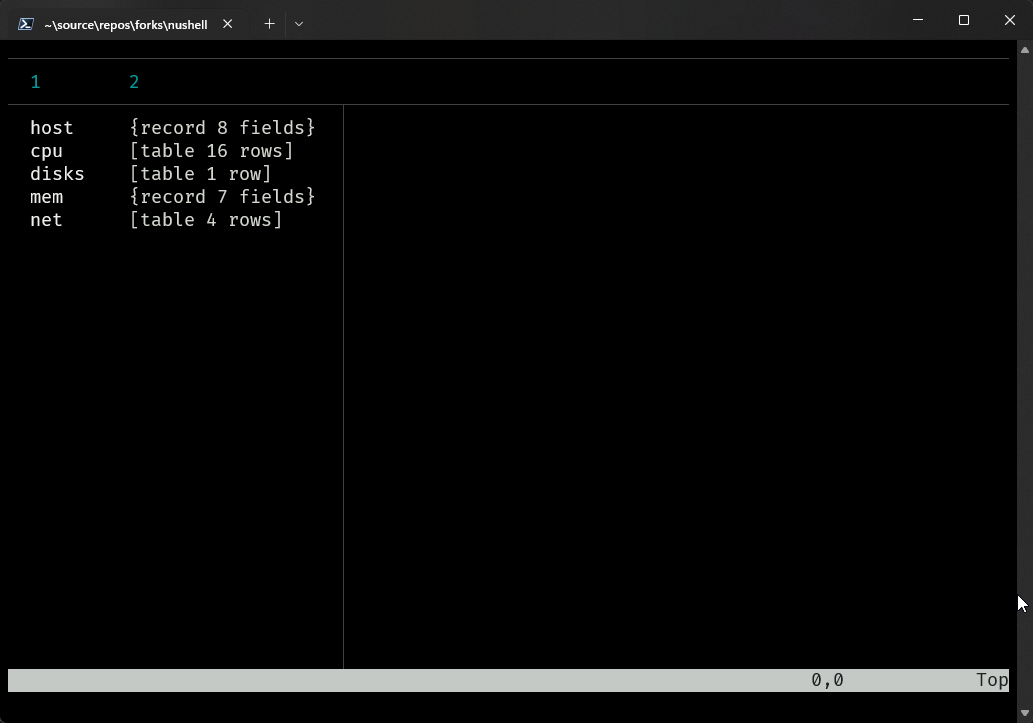
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
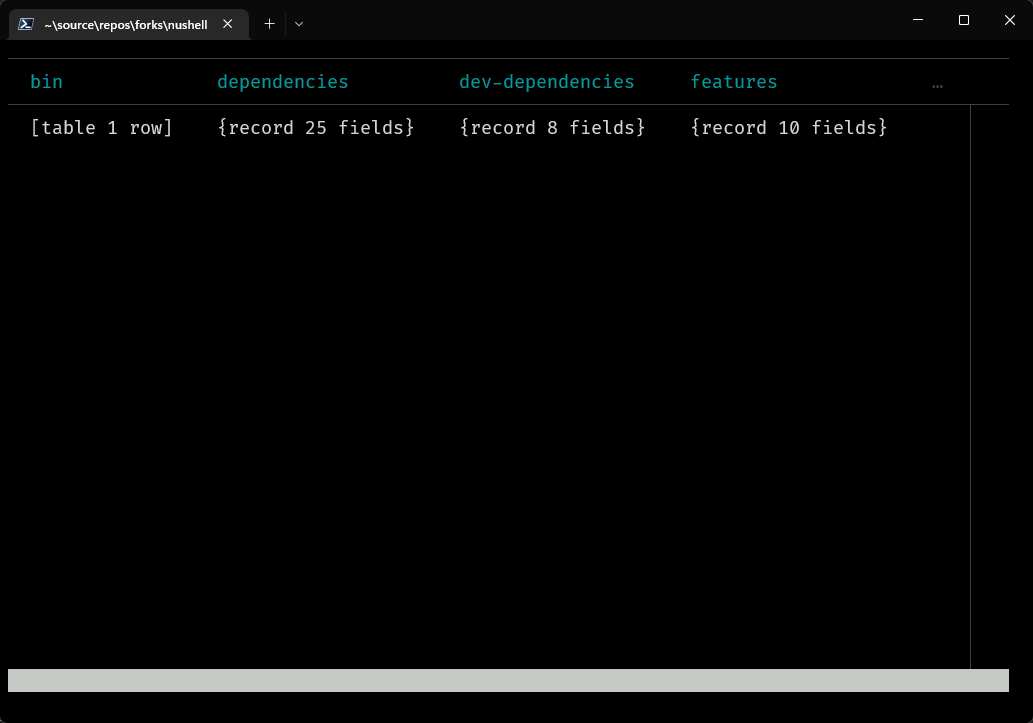
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
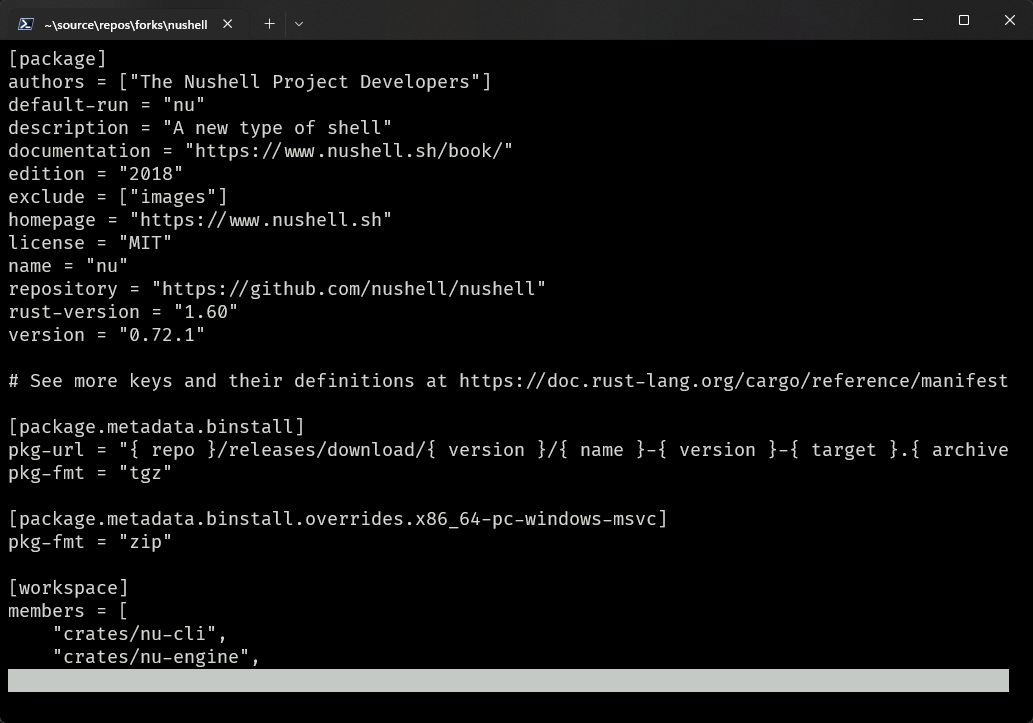
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
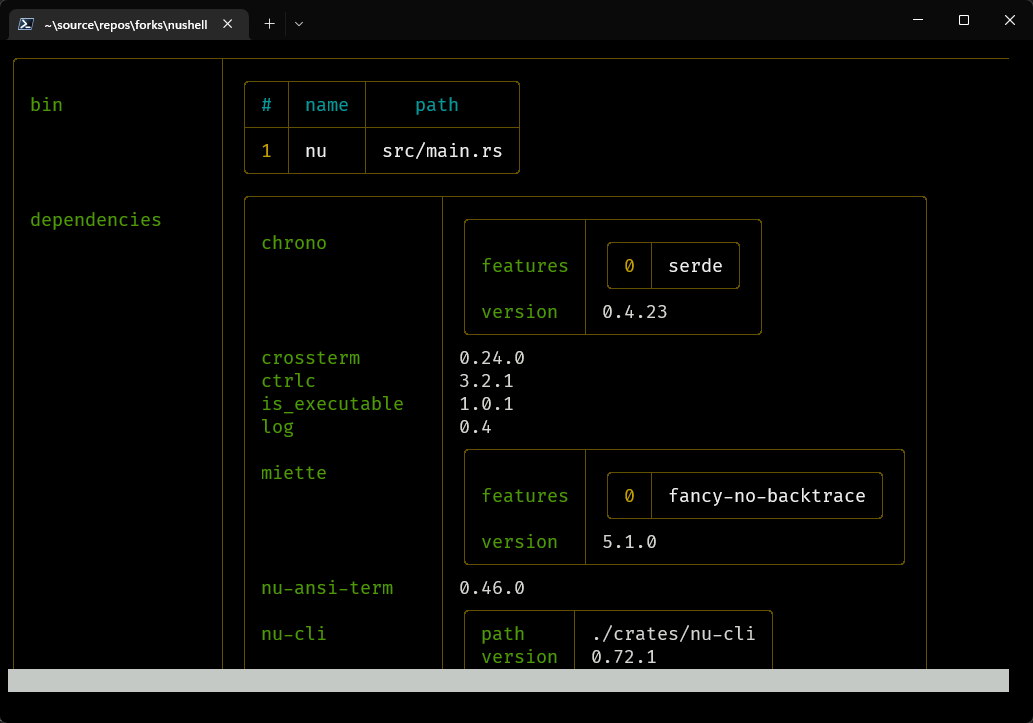
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
|
2022-12-16 15:47:07 +00:00
|
|
|
if let Some(s) = colors.get("status_bar_background") {
|
|
|
|
style.status_bar_background = *s;
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
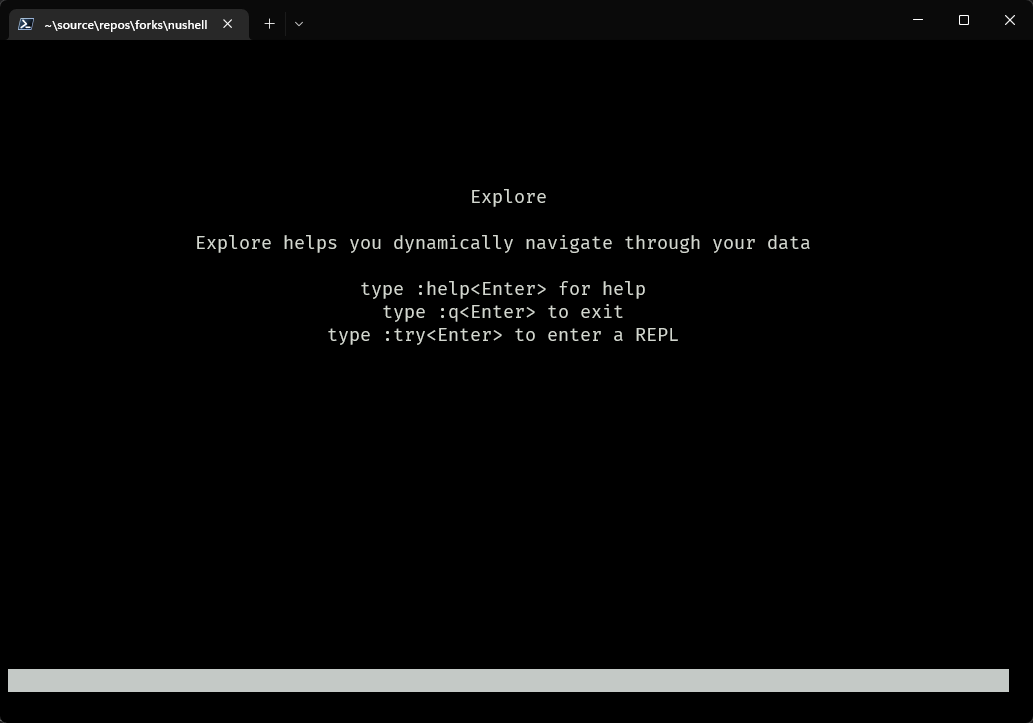
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
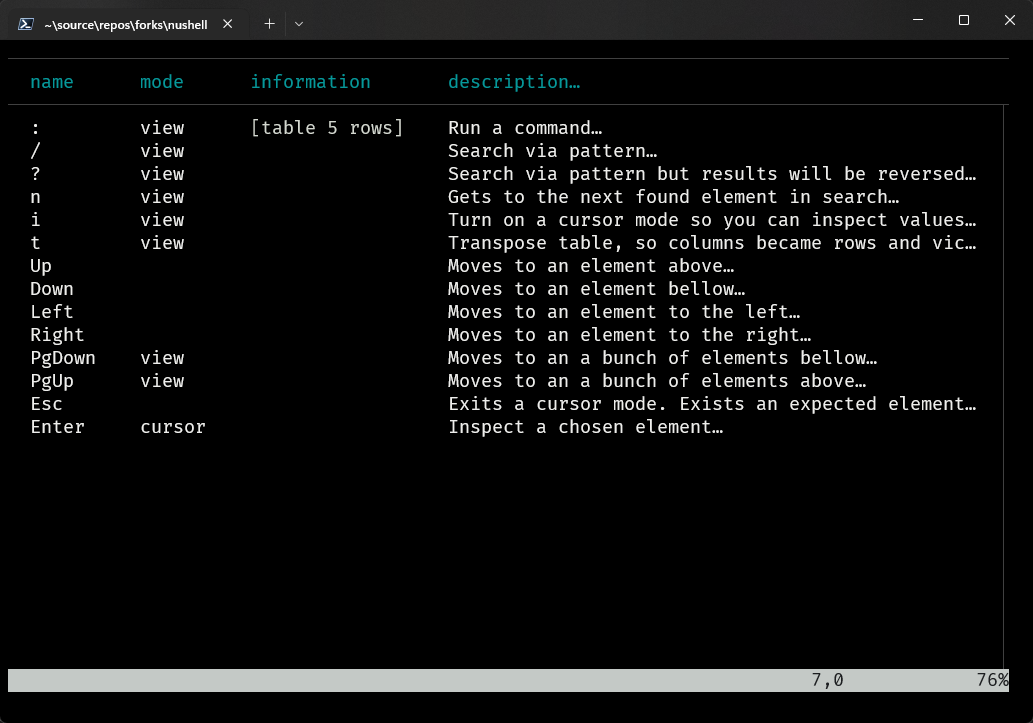
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
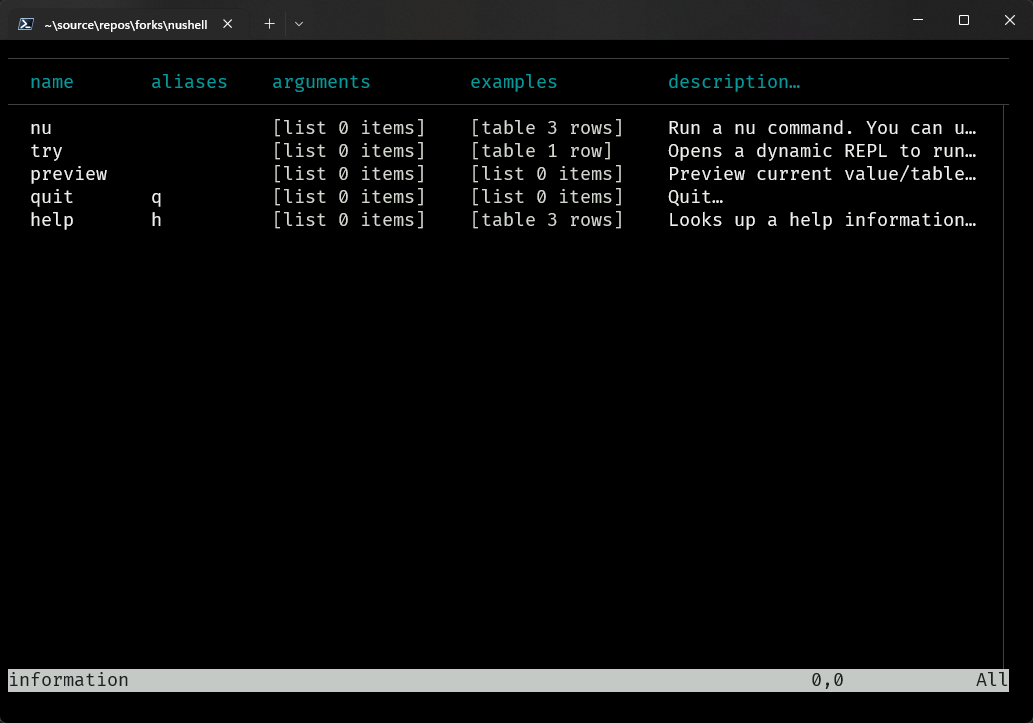
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
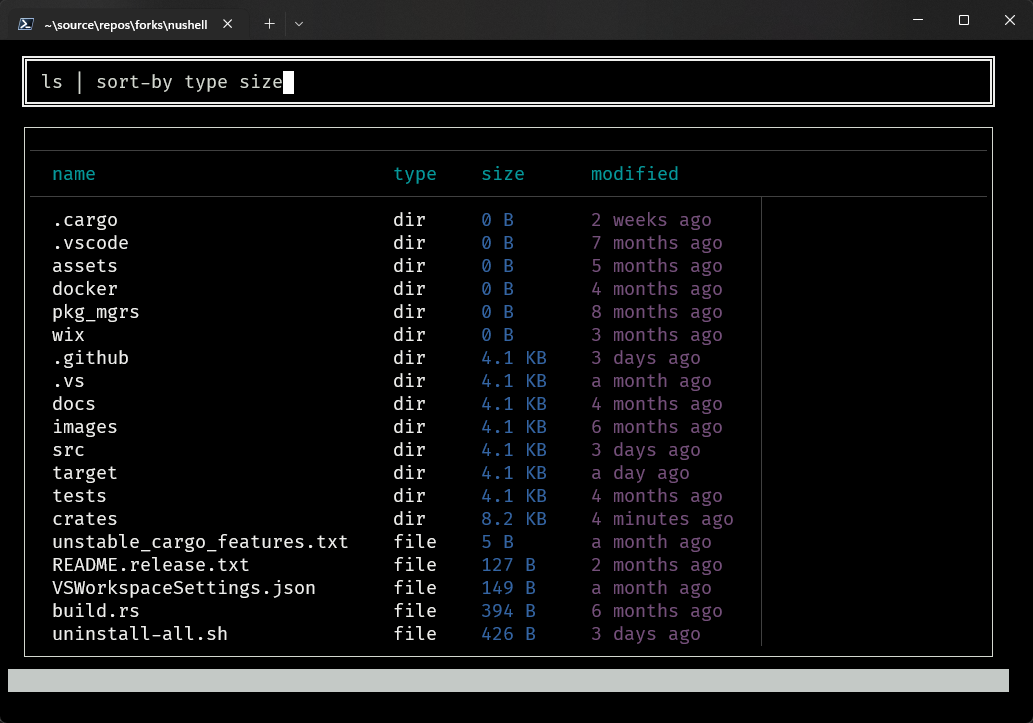
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
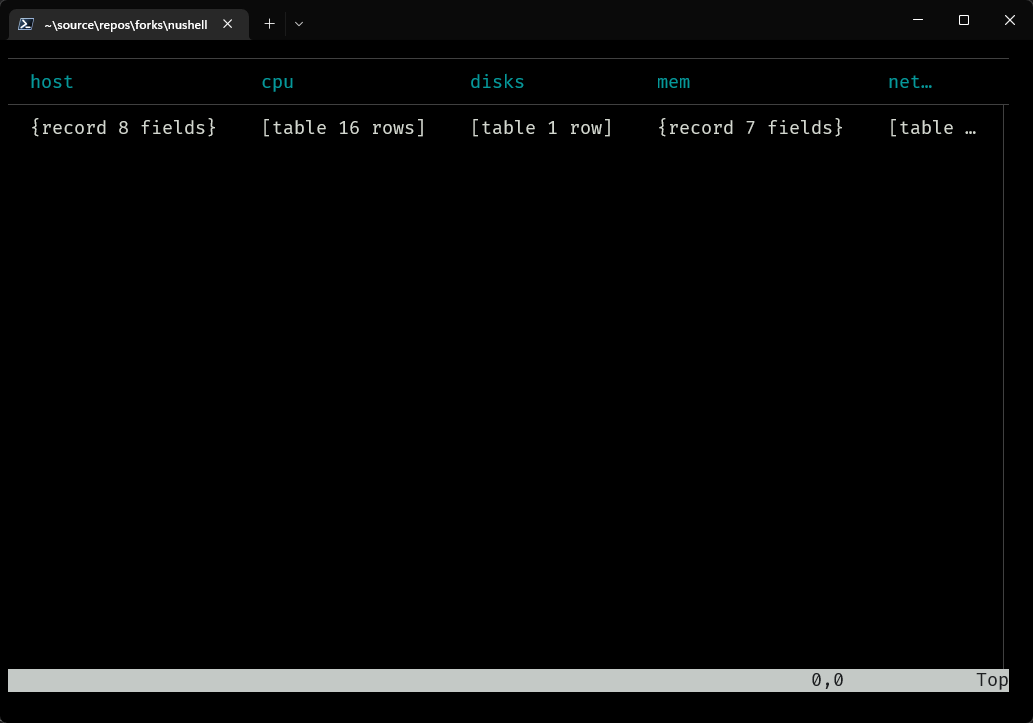
If you hit the `t` key it will now transpose the view to look like this.
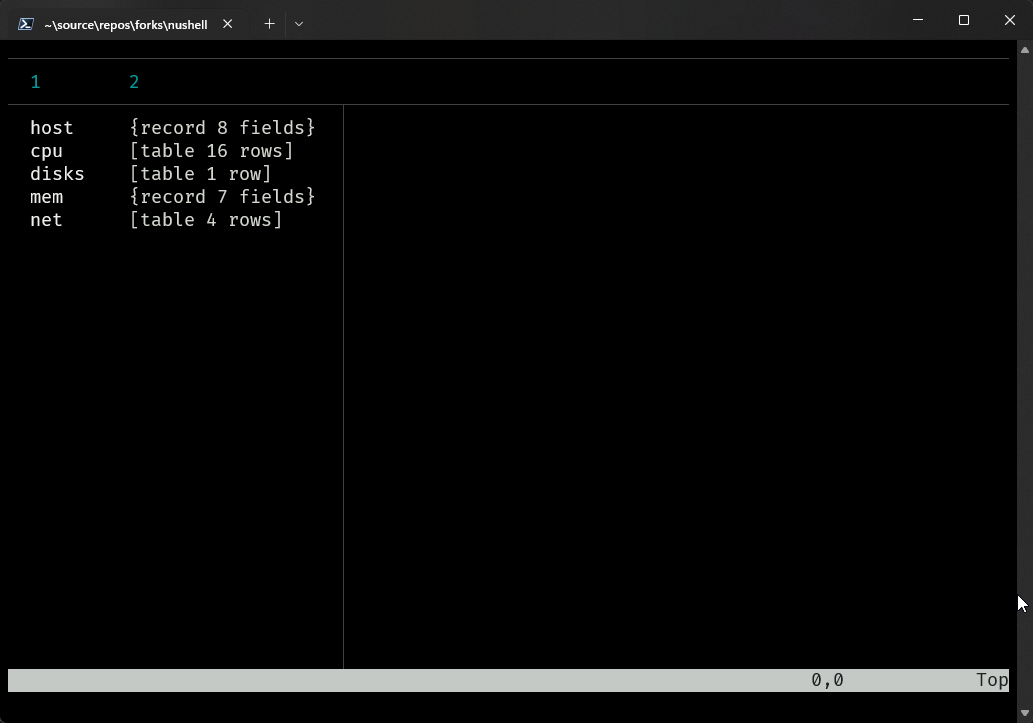
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
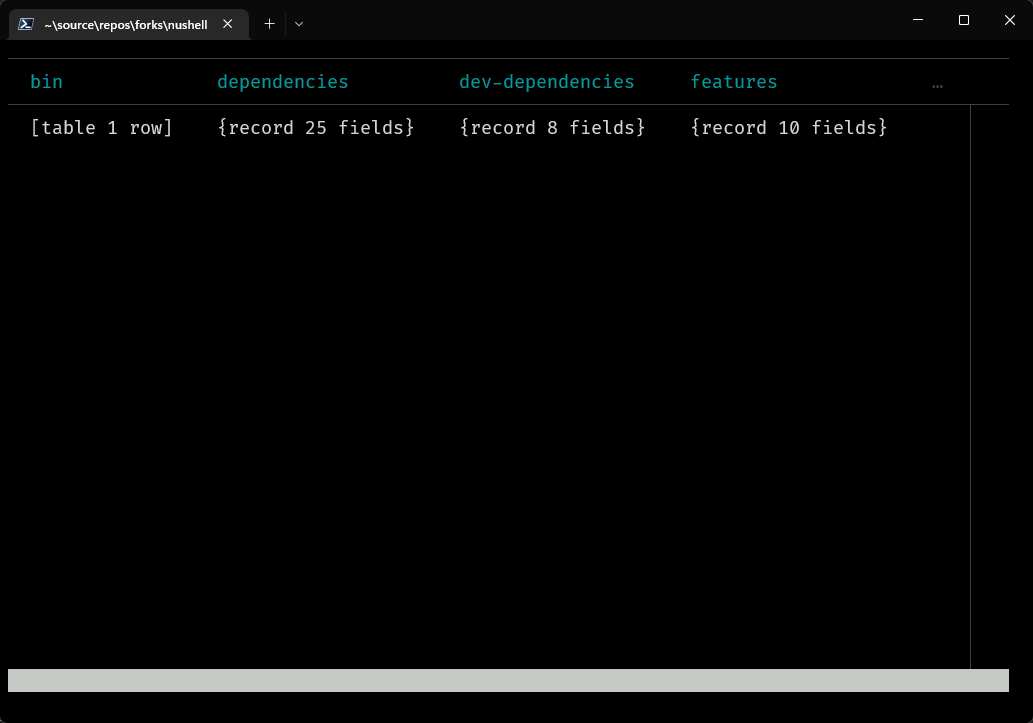
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
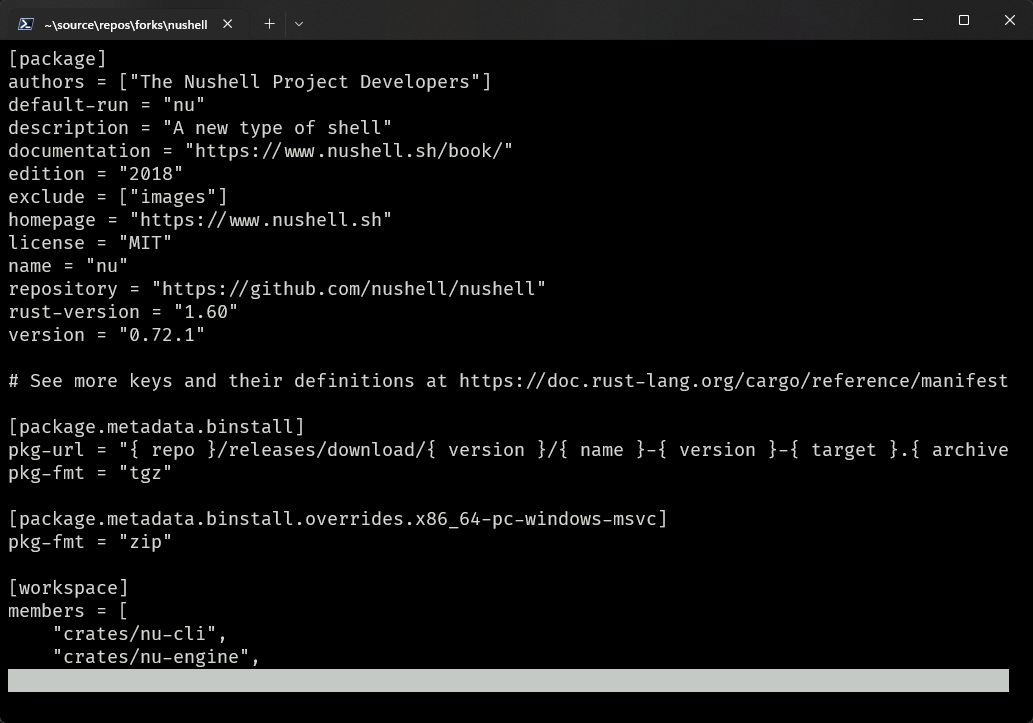
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
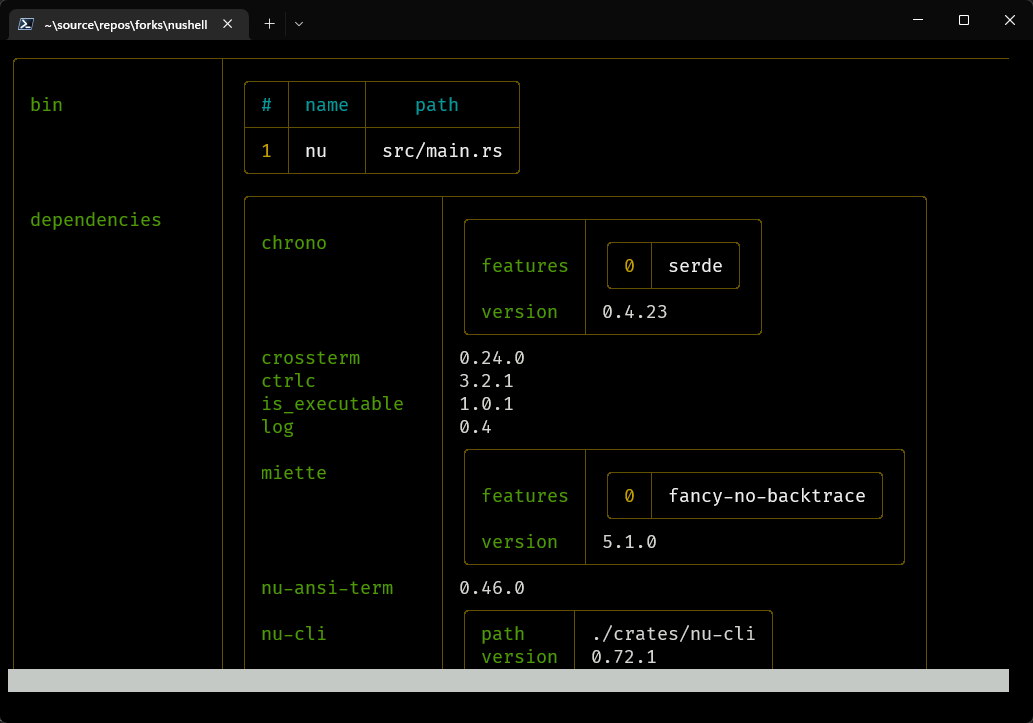
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
}
|
|
|
|
|
2022-12-16 15:47:07 +00:00
|
|
|
if let Some(s) = colors.get("command_bar_text") {
|
|
|
|
style.cmd_bar_text = *s;
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
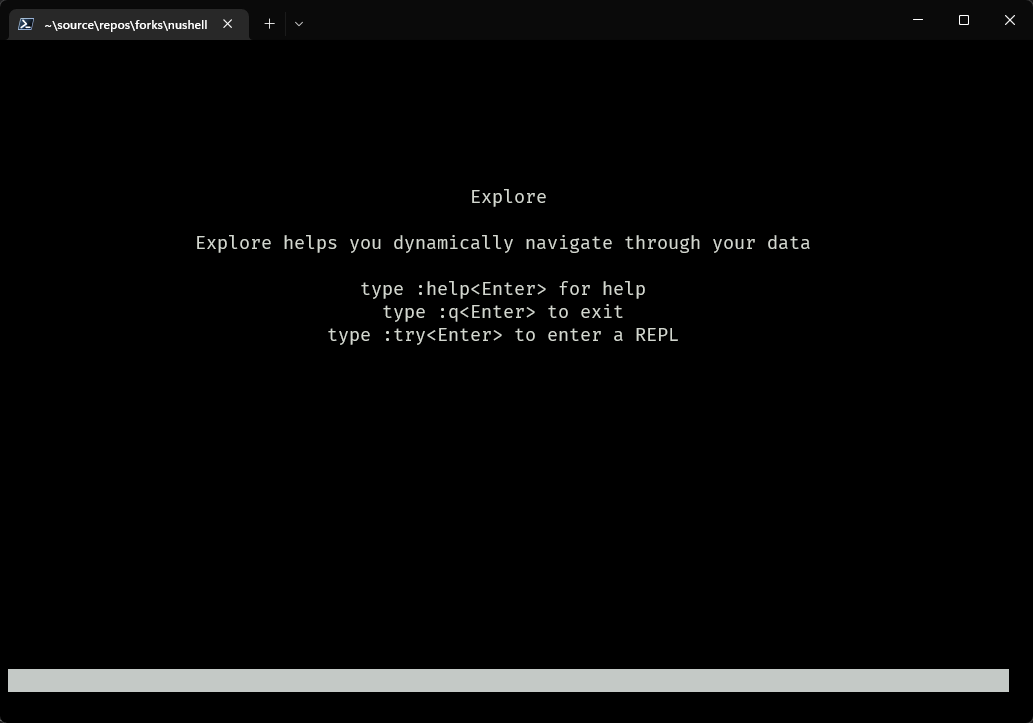
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
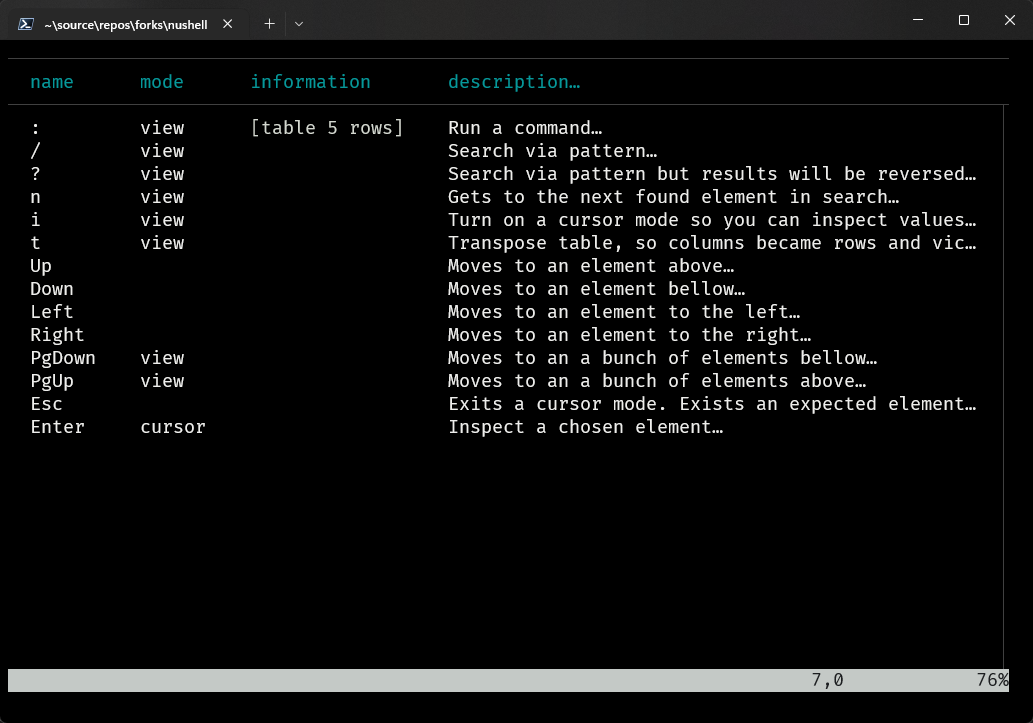
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
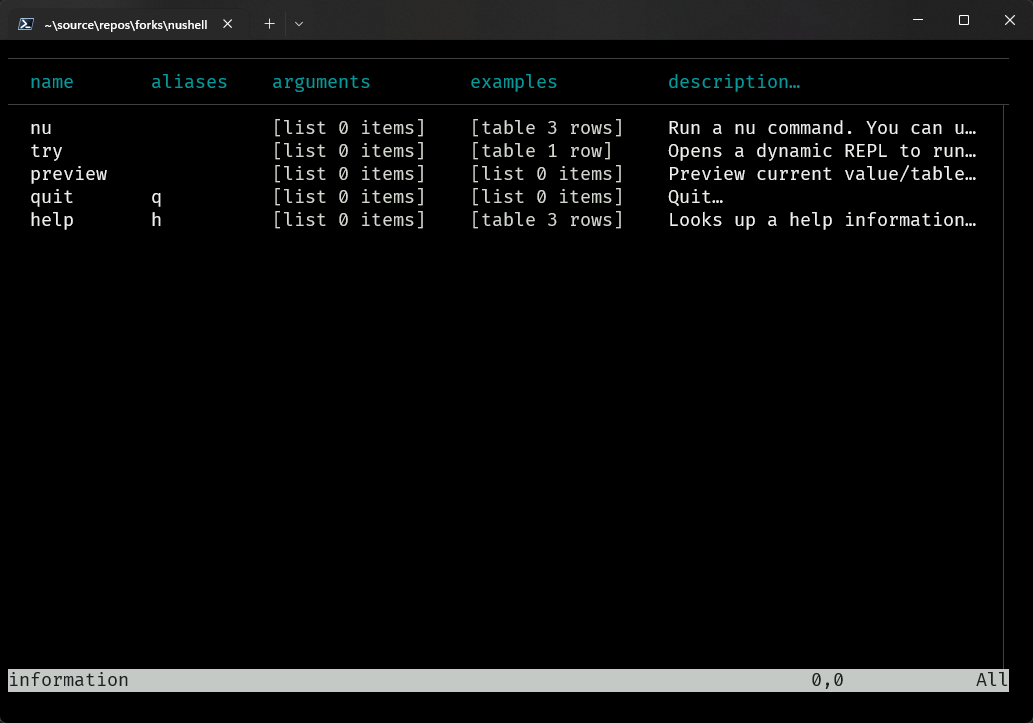
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
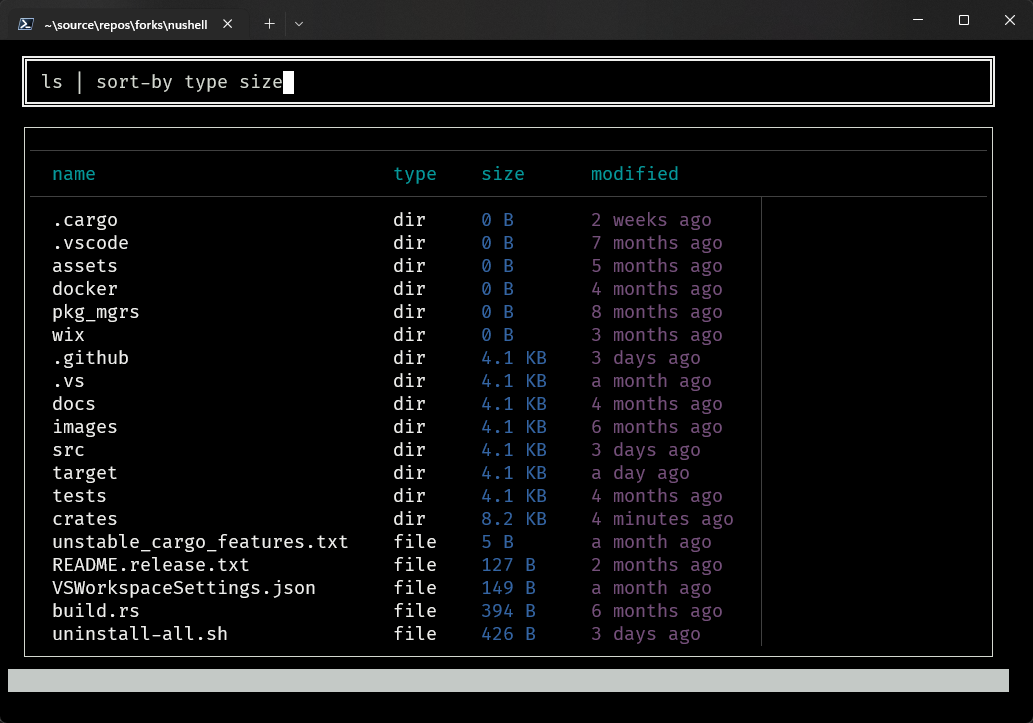
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
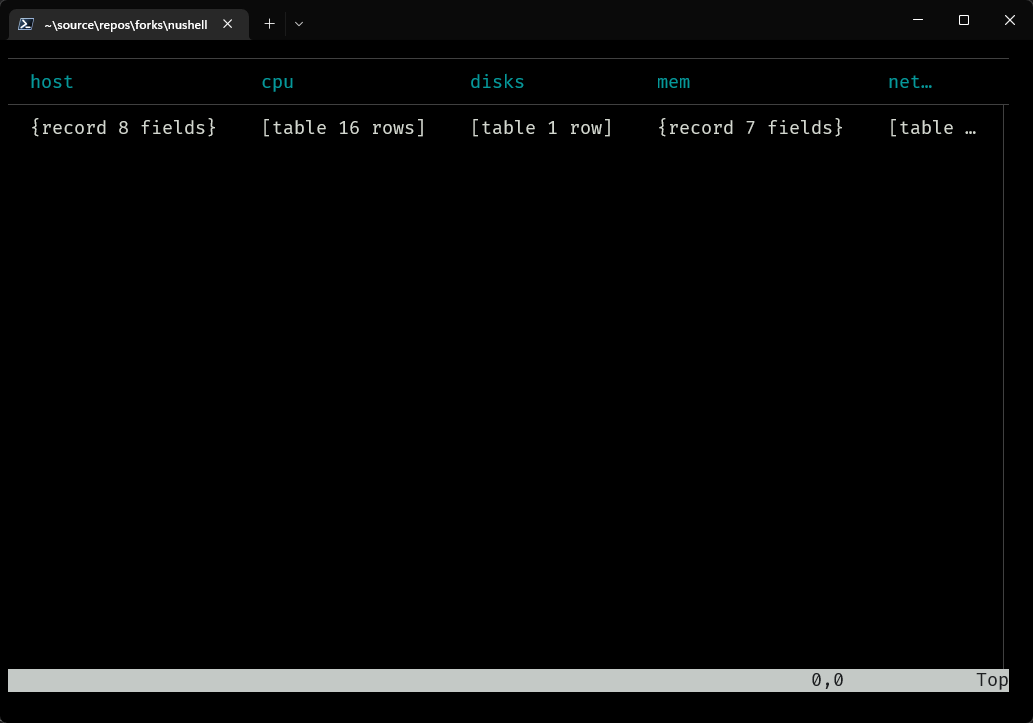
If you hit the `t` key it will now transpose the view to look like this.
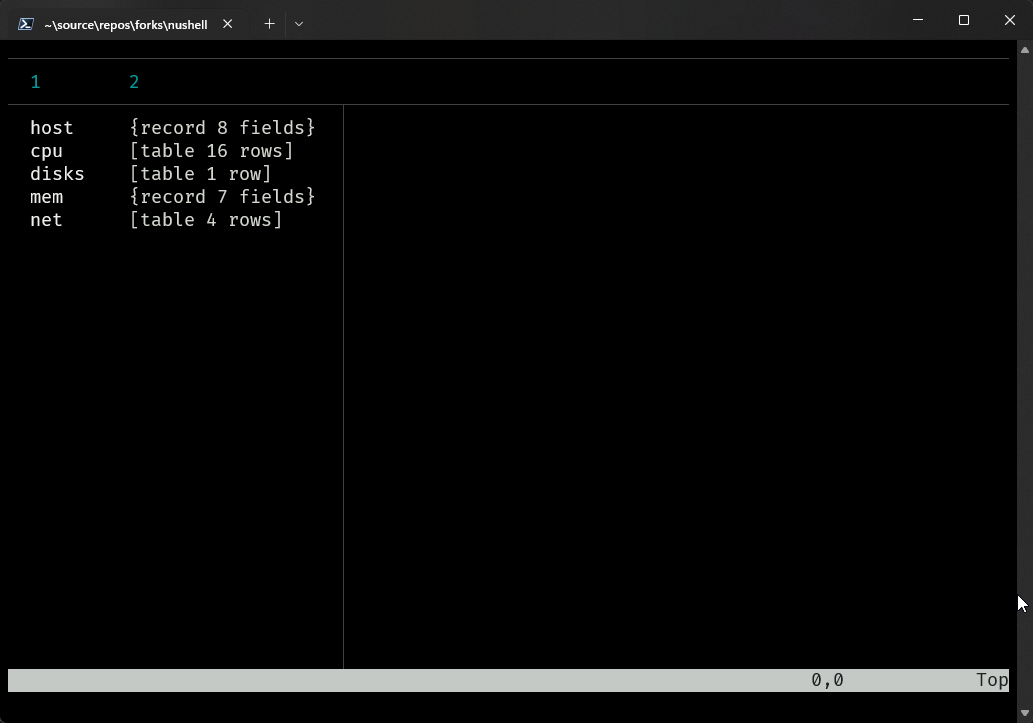
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
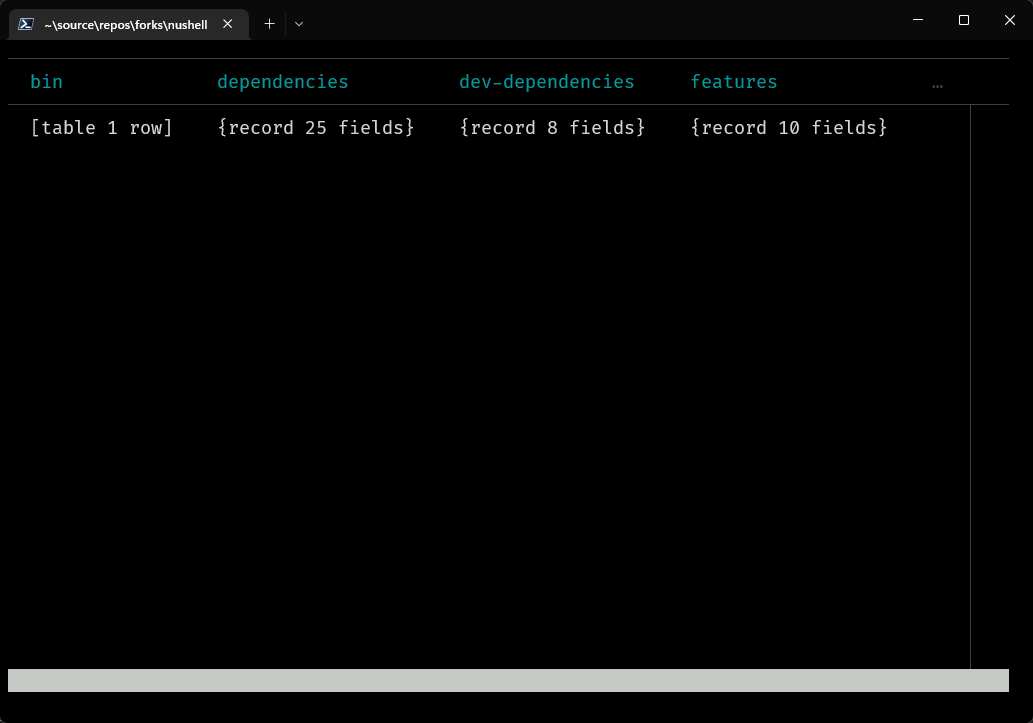
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
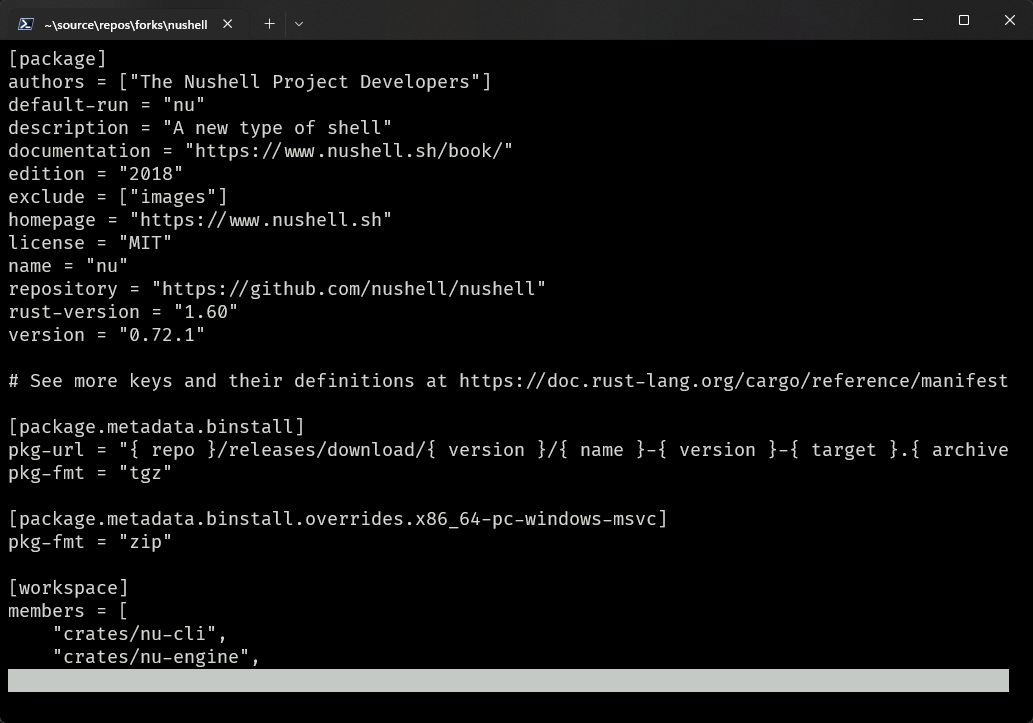
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
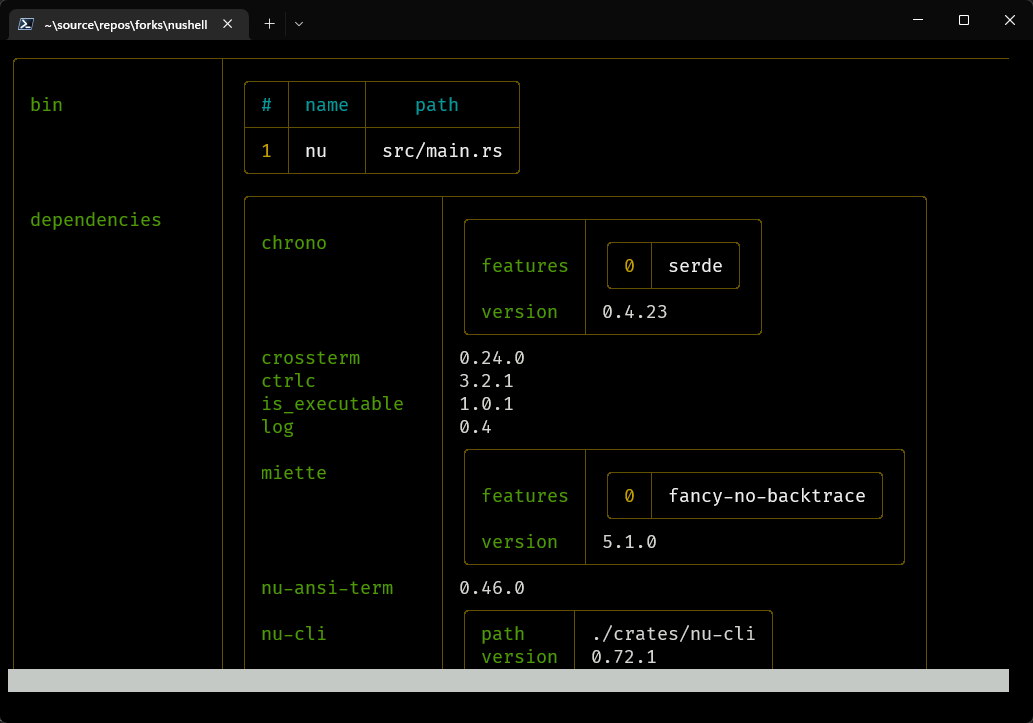
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
}
|
|
|
|
|
2022-12-16 15:47:07 +00:00
|
|
|
if let Some(s) = colors.get("command_bar_background") {
|
|
|
|
style.cmd_bar_background = *s;
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
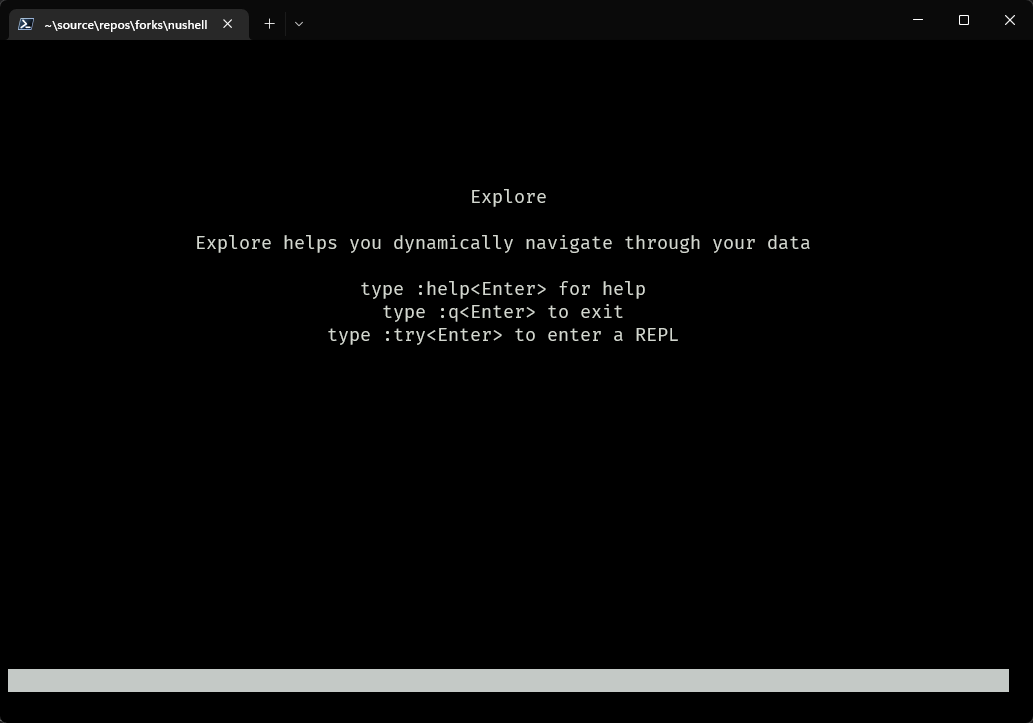
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
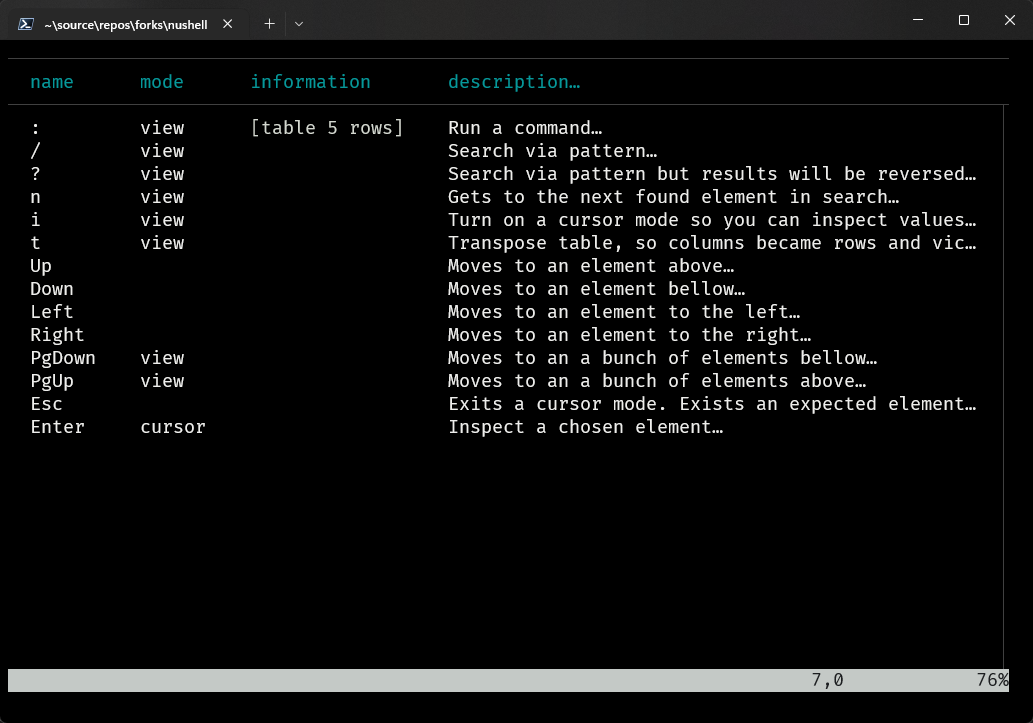
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
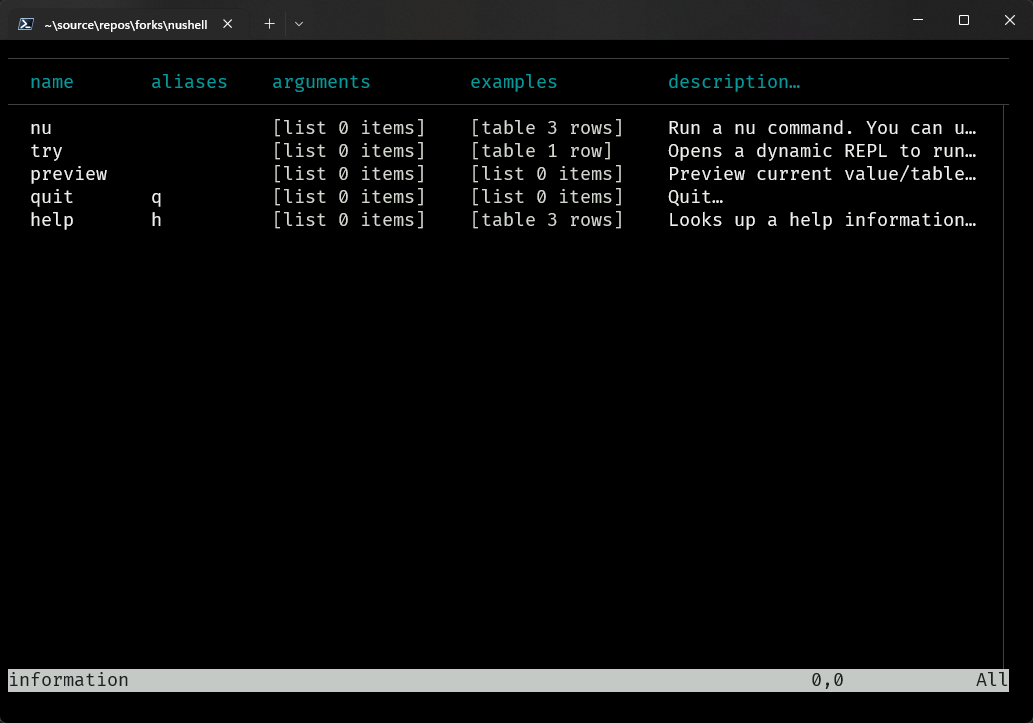
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
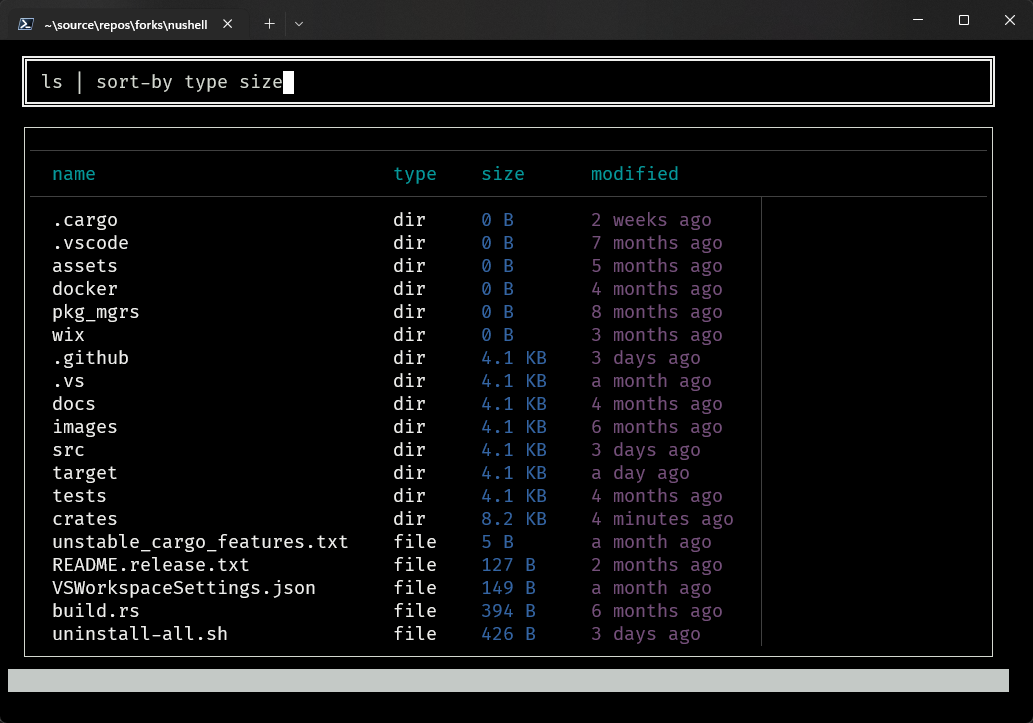
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
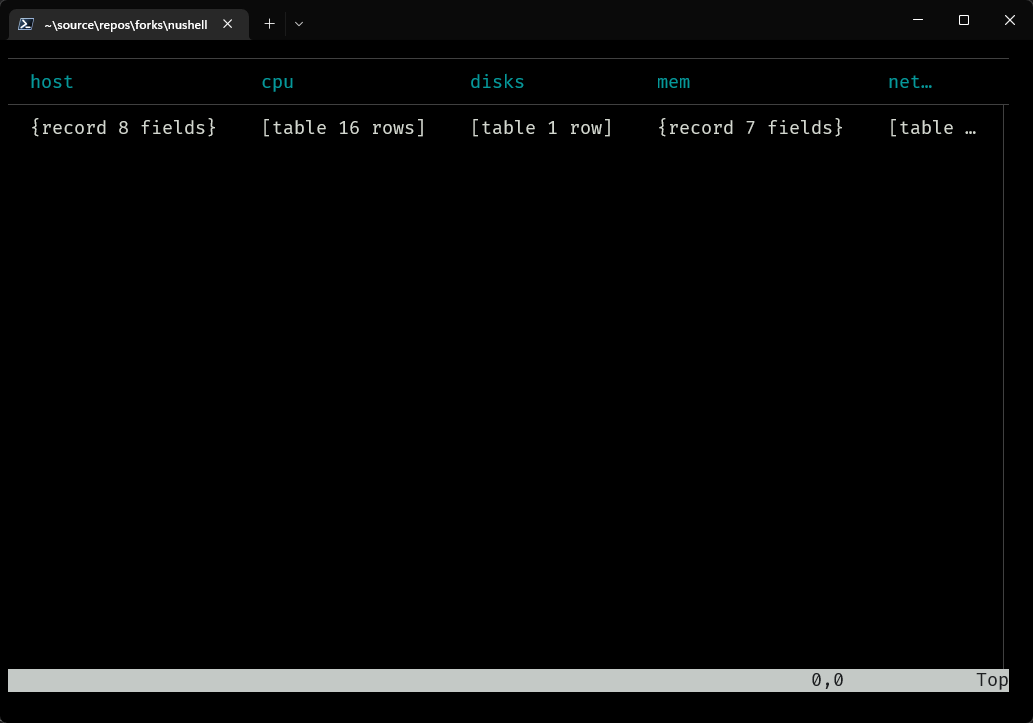
If you hit the `t` key it will now transpose the view to look like this.
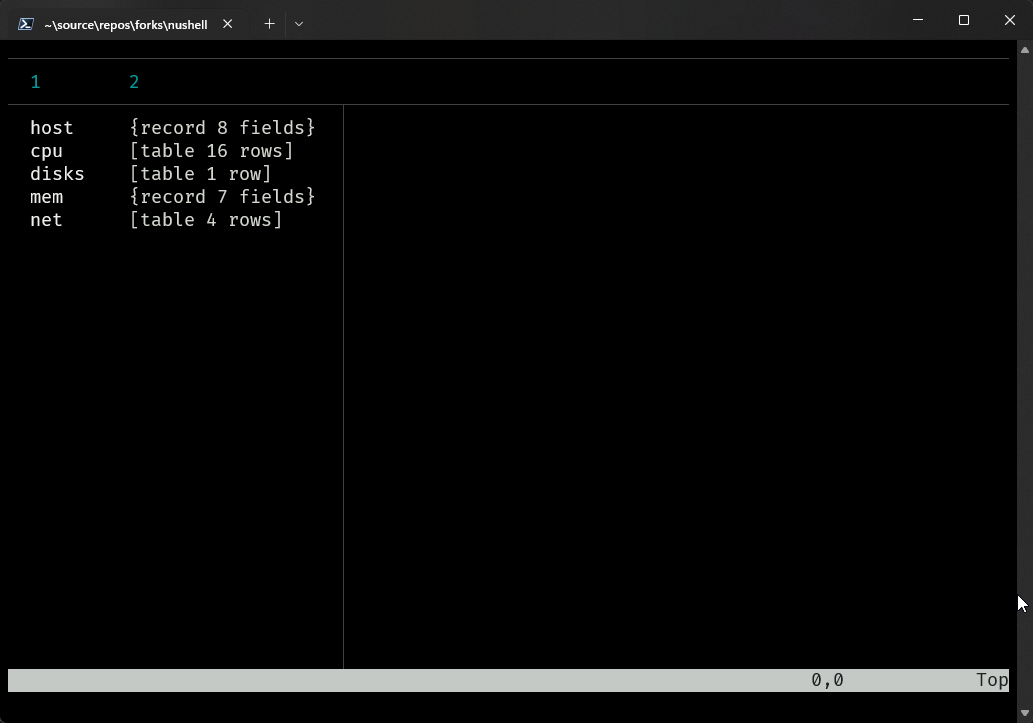
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
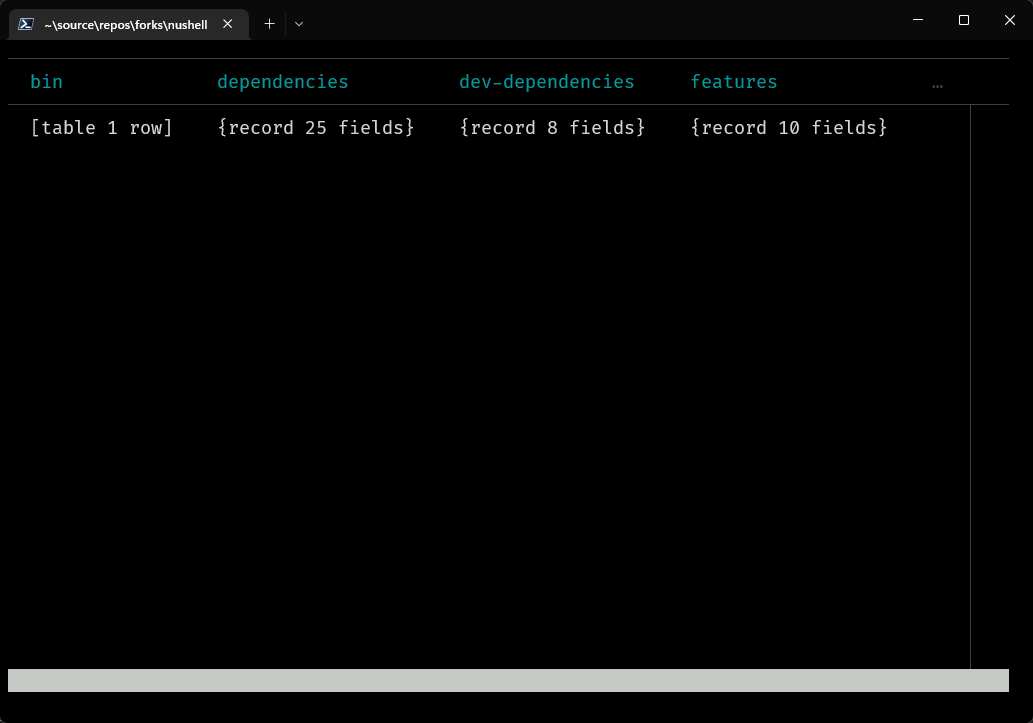
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
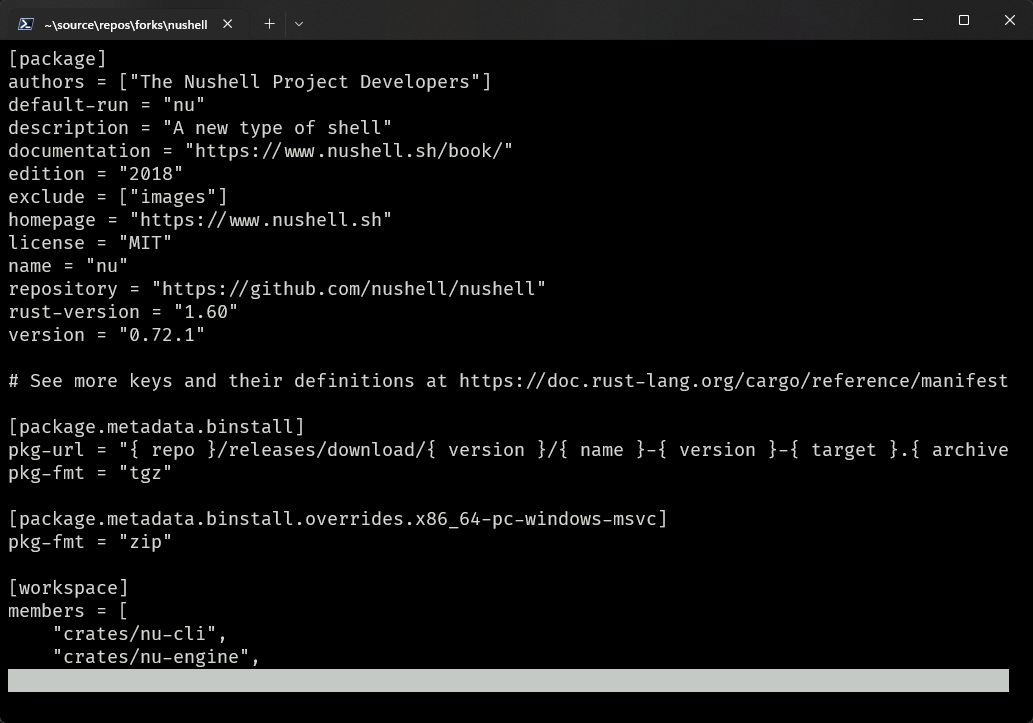
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
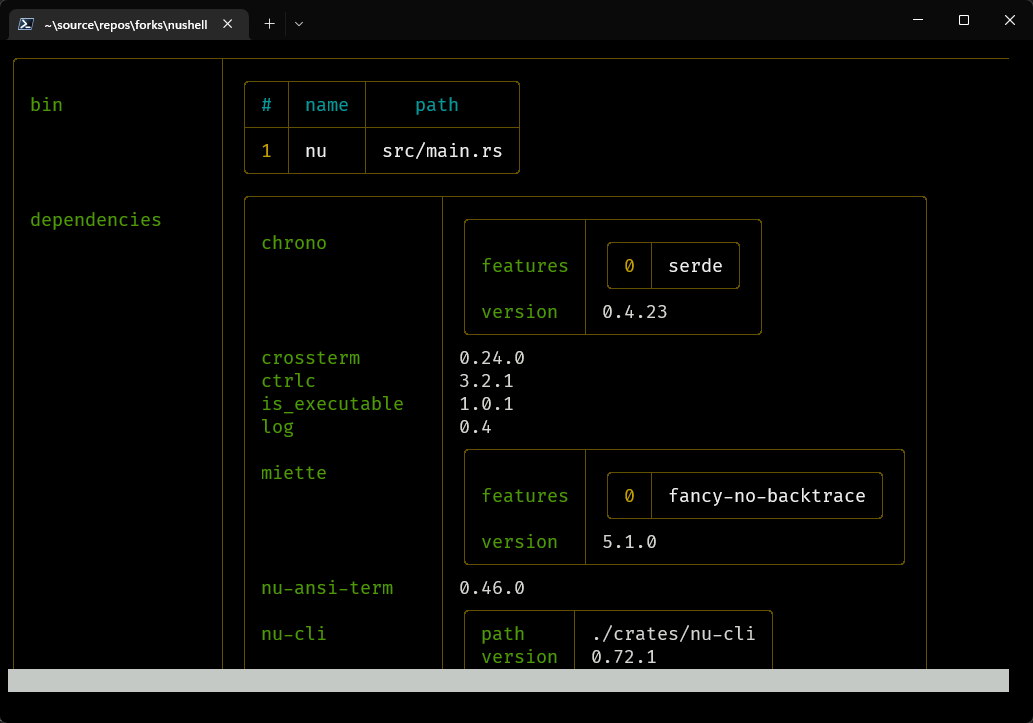
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
}
|
|
|
|
|
2022-12-16 15:47:07 +00:00
|
|
|
if let Some(hm) = config.get("status").and_then(create_map) {
|
|
|
|
let colors = get_color_map(&hm);
|
|
|
|
|
|
|
|
if let Some(s) = colors.get("info") {
|
|
|
|
style.status_info = *s;
|
|
|
|
}
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
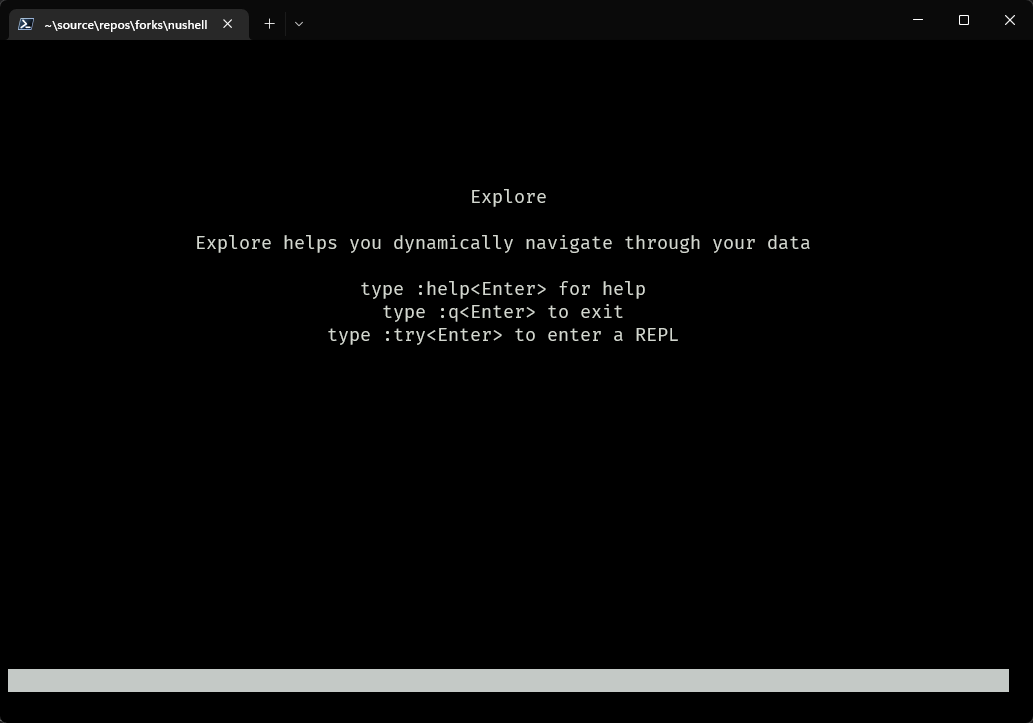
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
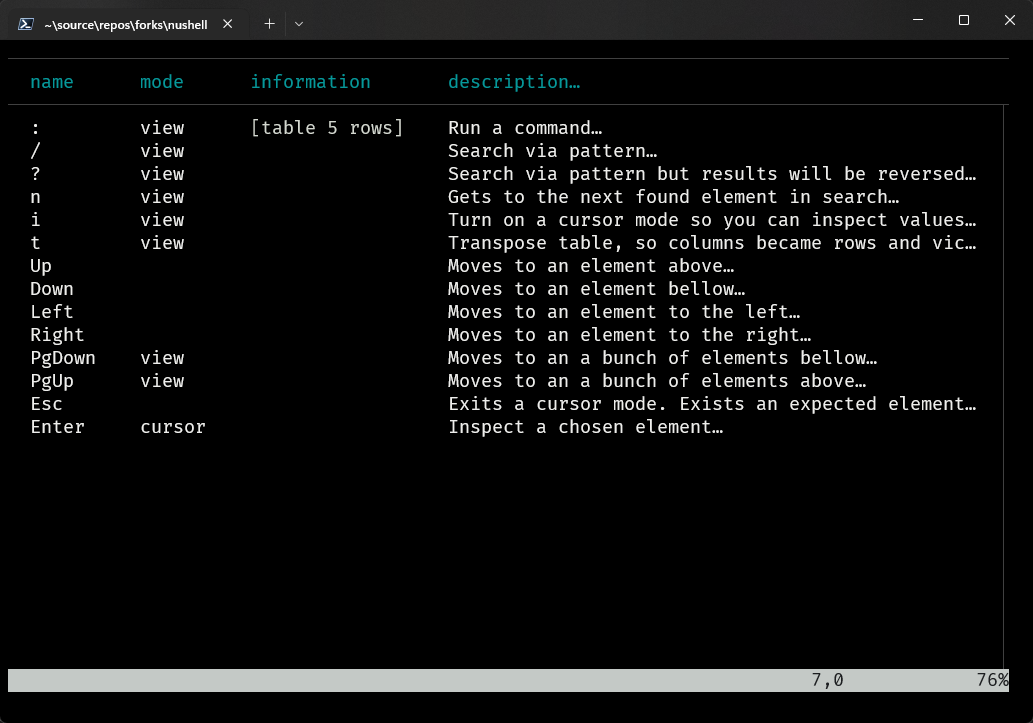
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
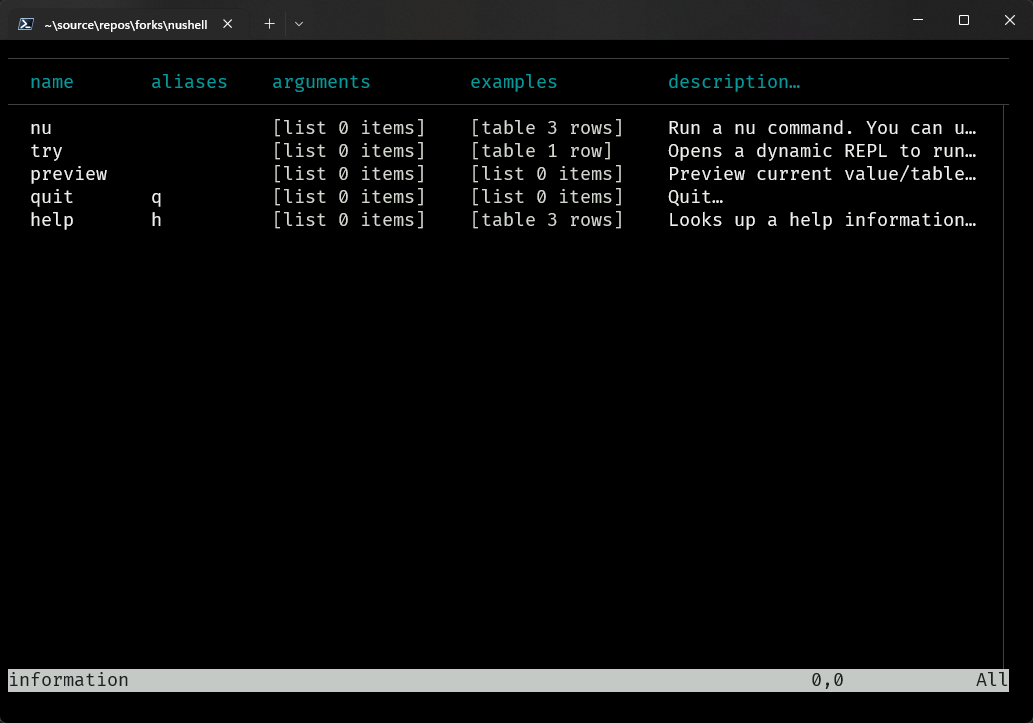
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
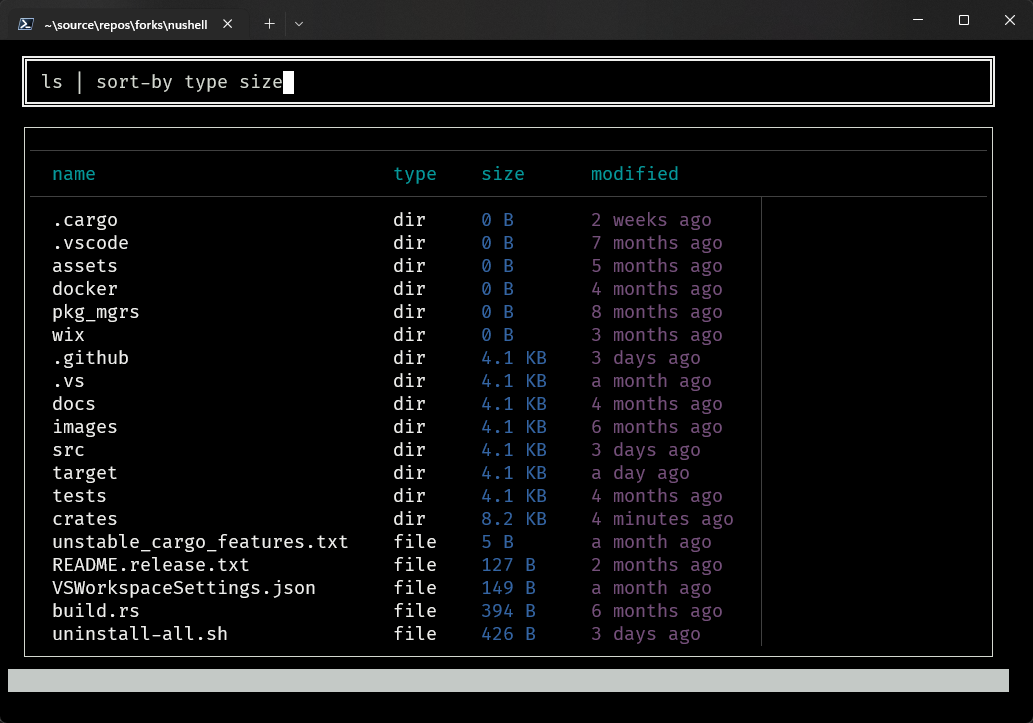
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
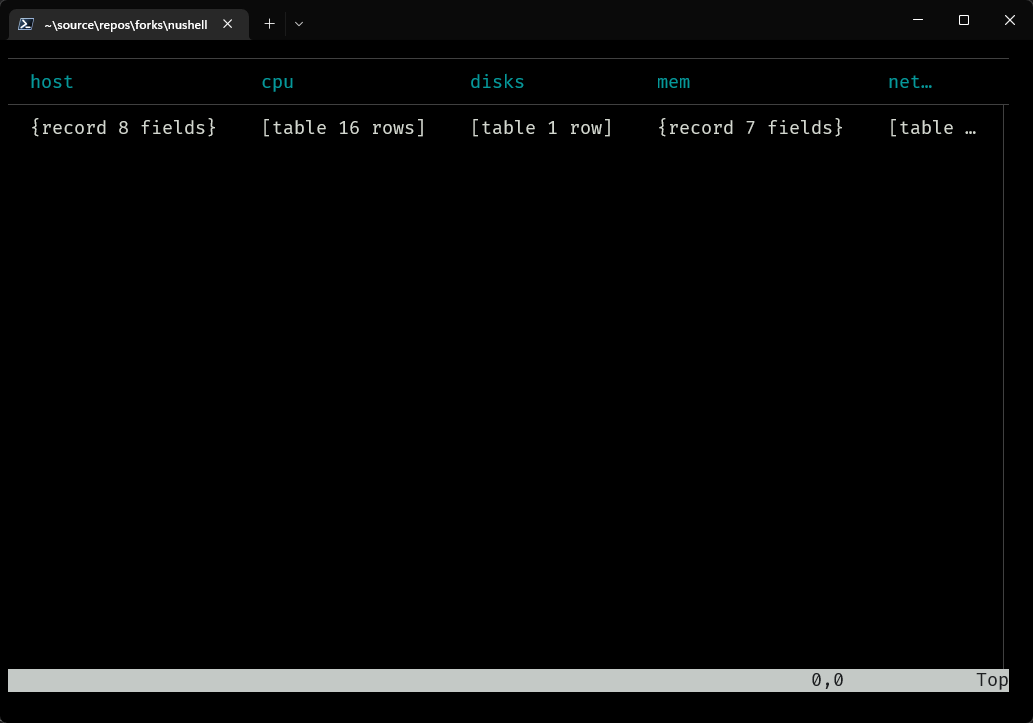
If you hit the `t` key it will now transpose the view to look like this.
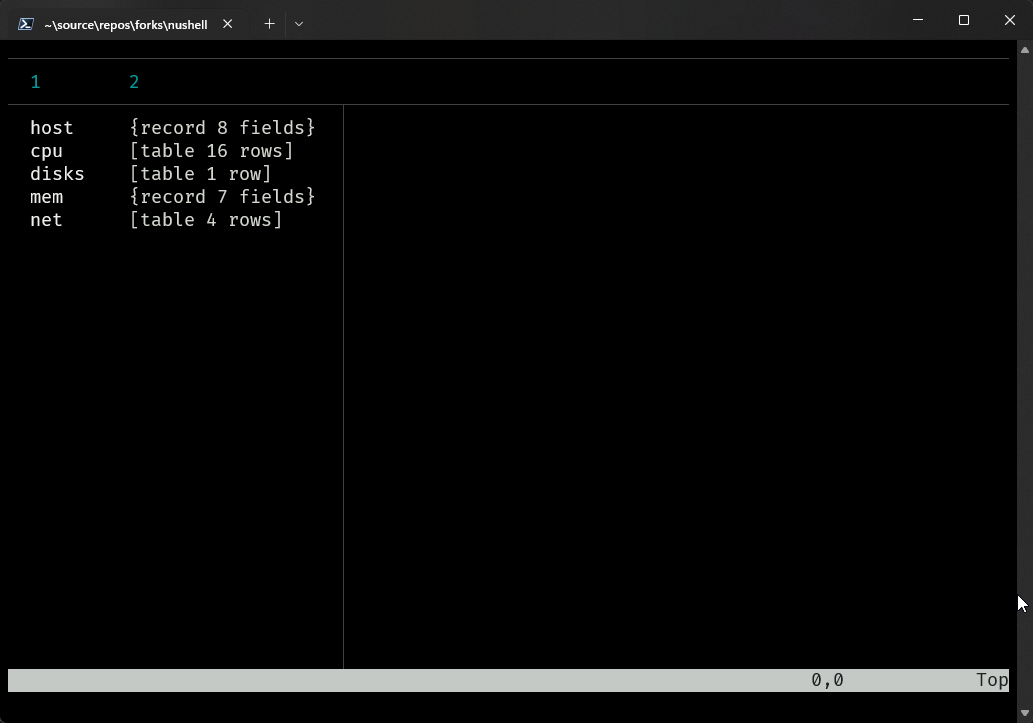
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
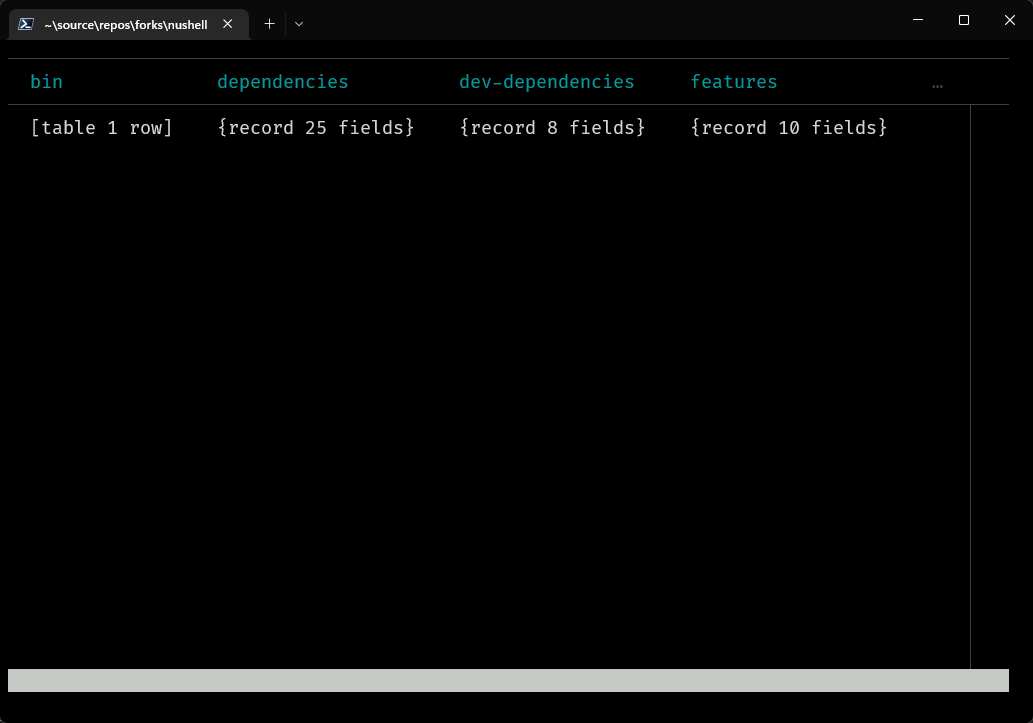
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
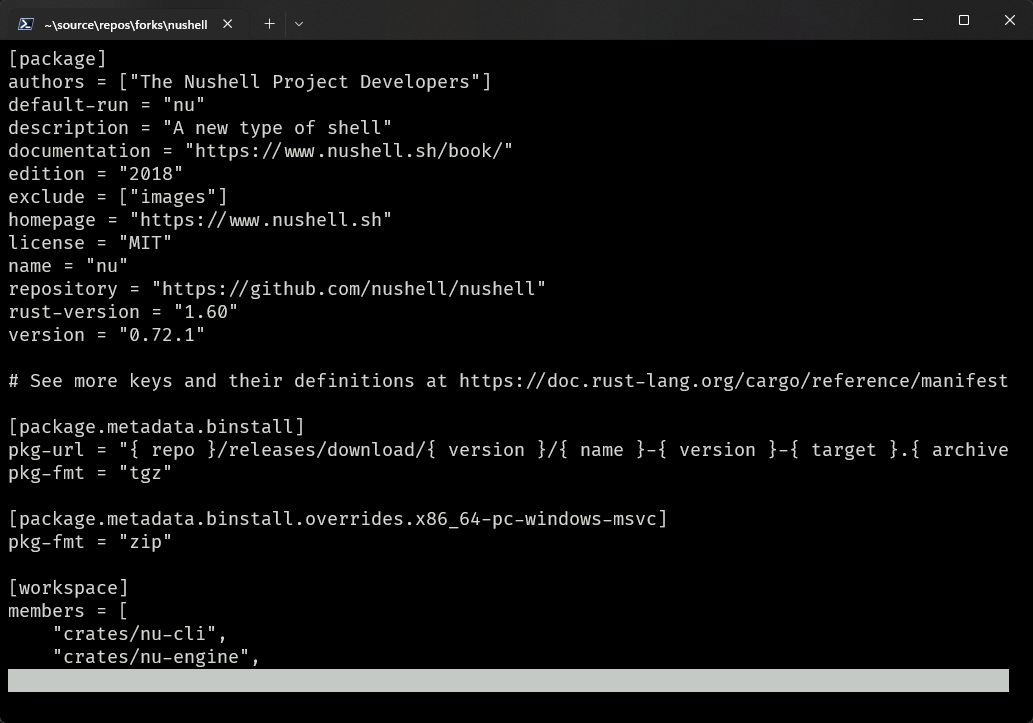
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
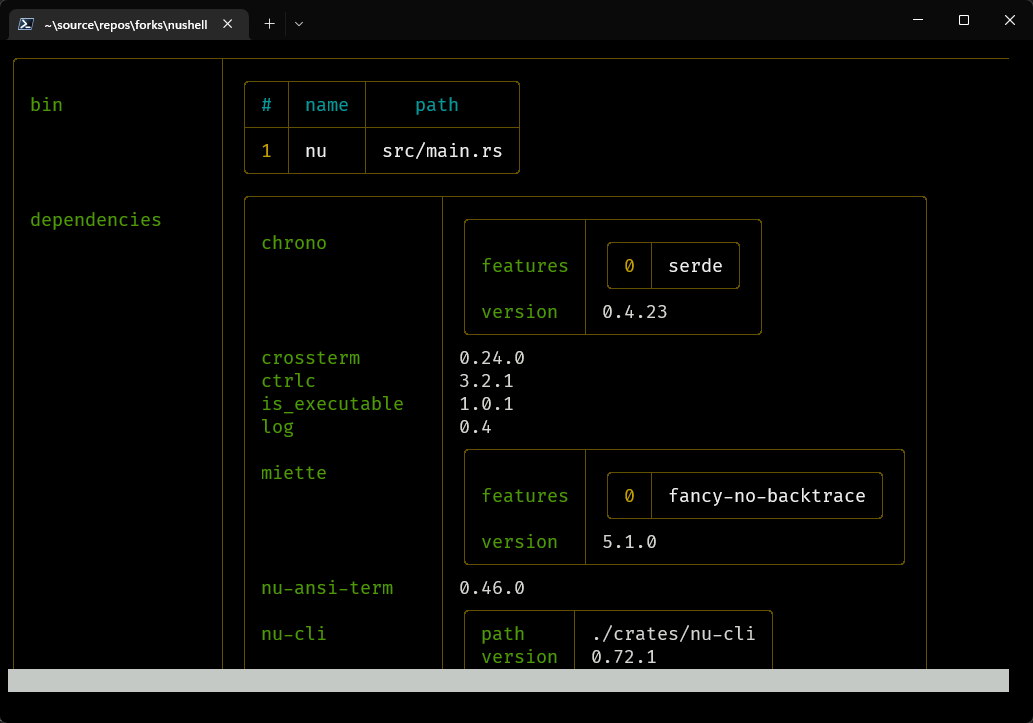
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
|
2022-12-16 15:47:07 +00:00
|
|
|
if let Some(s) = colors.get("warn") {
|
|
|
|
style.status_warn = *s;
|
|
|
|
}
|
|
|
|
|
|
|
|
if let Some(s) = colors.get("error") {
|
|
|
|
style.status_error = *s;
|
|
|
|
}
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
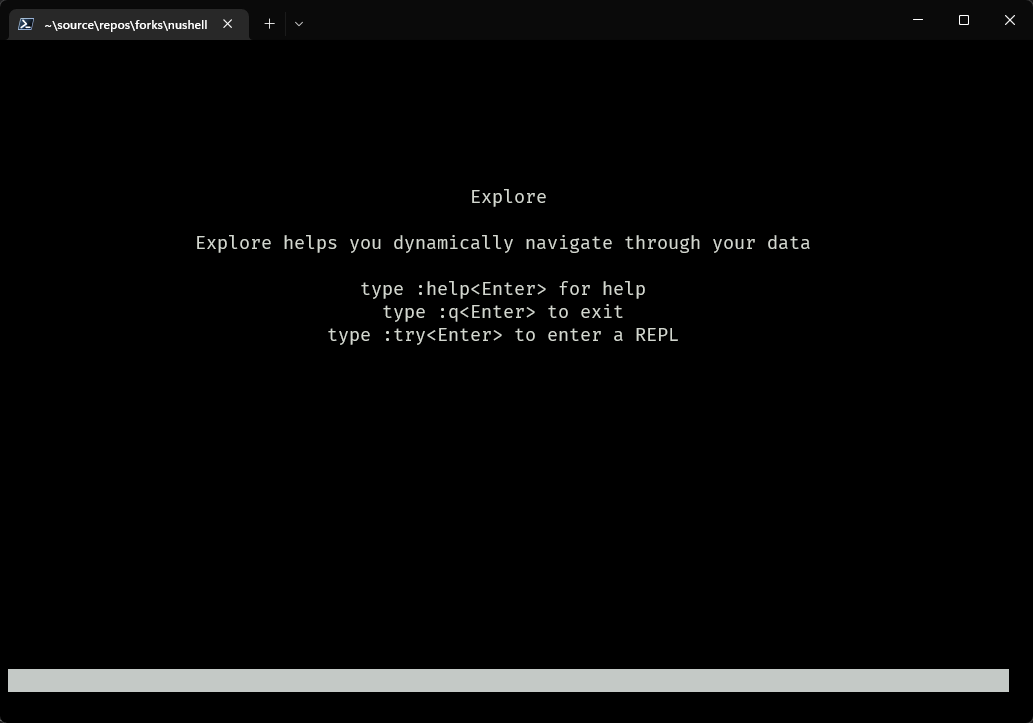
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
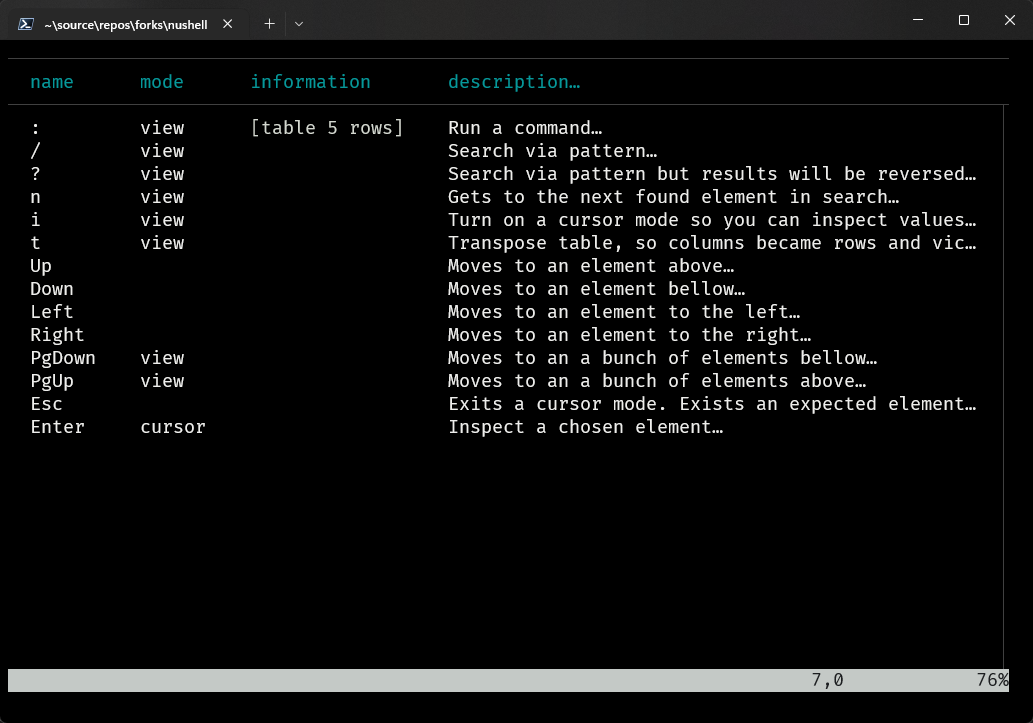
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
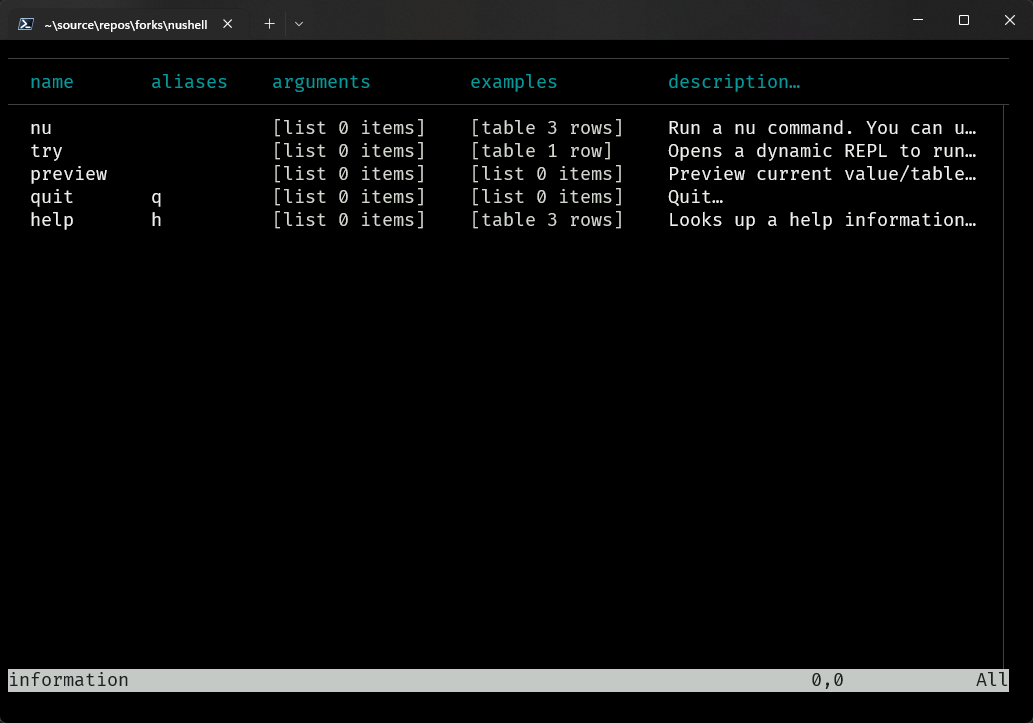
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
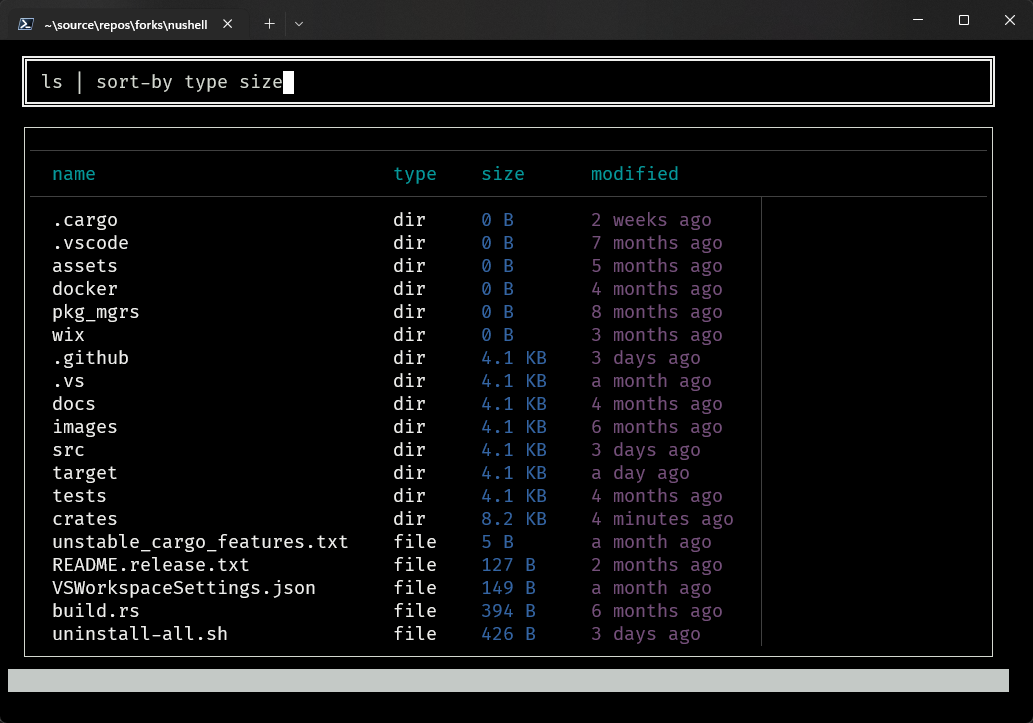
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
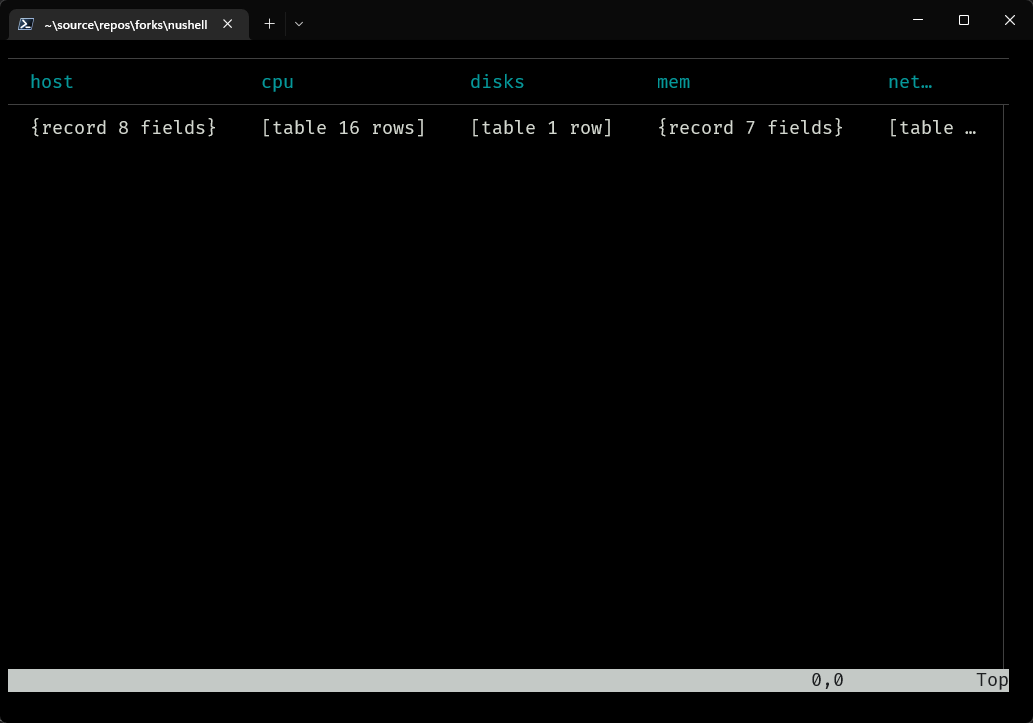
If you hit the `t` key it will now transpose the view to look like this.
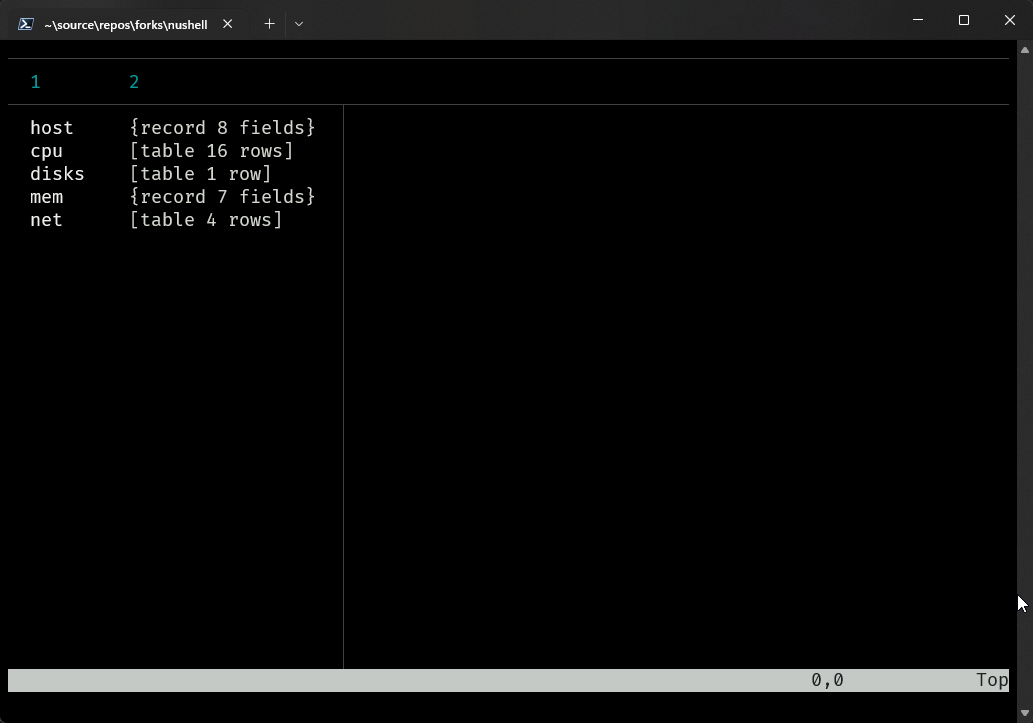
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
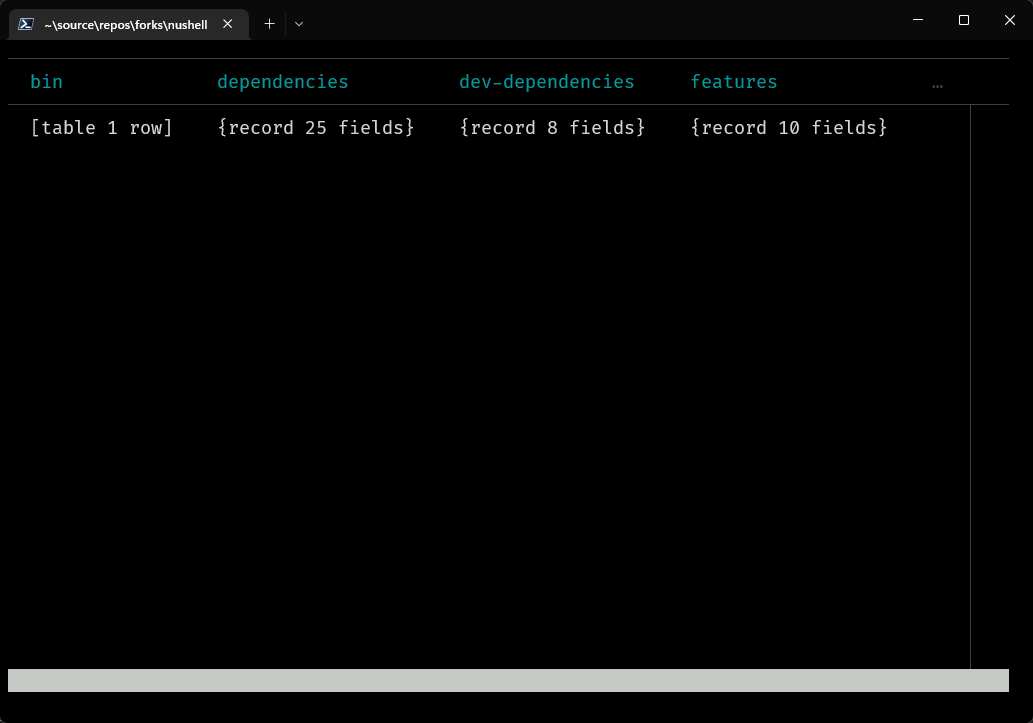
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
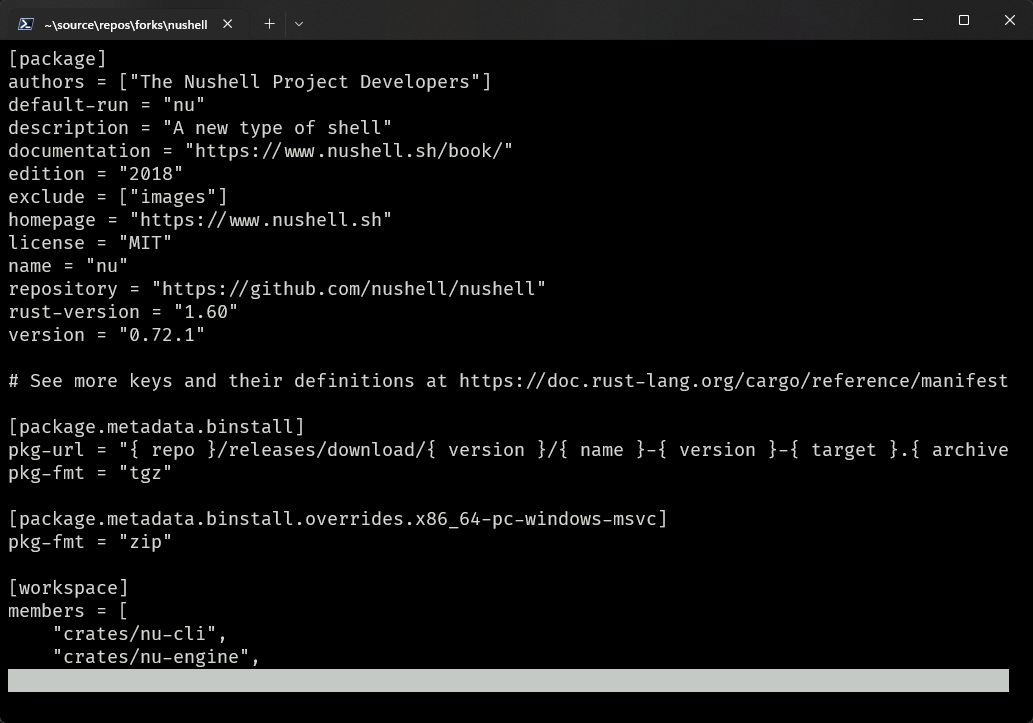
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
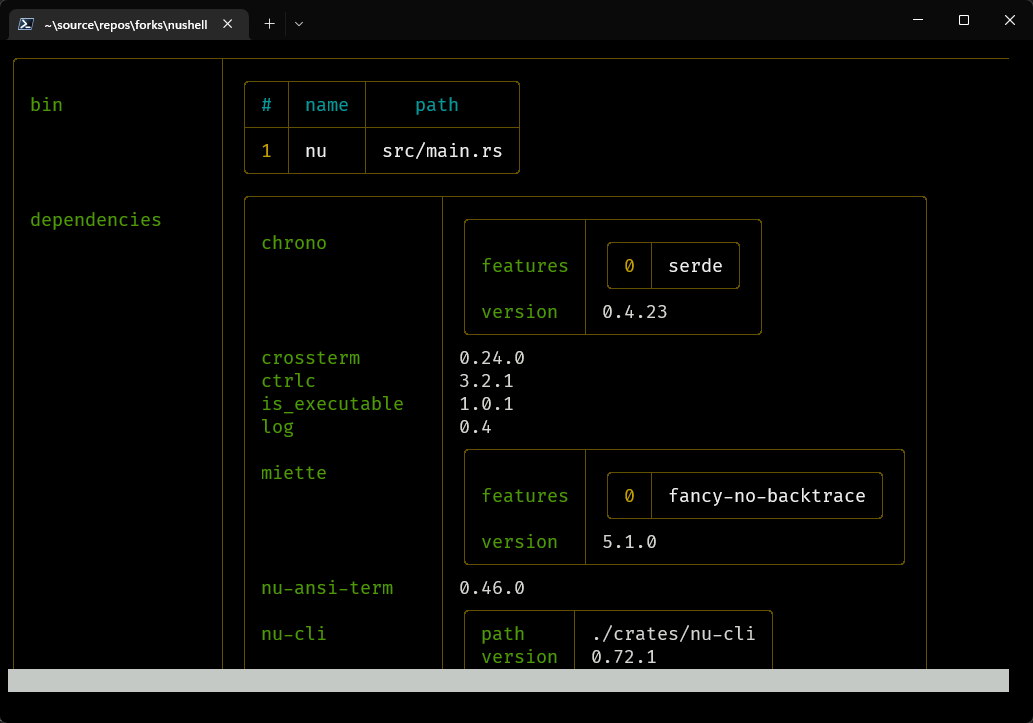
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
}
|
|
|
|
|
2022-12-16 15:47:07 +00:00
|
|
|
style
|
|
|
|
}
|
|
|
|
|
|
|
|
fn prepare_default_config(config: &mut HashMap<String, Value>) {
|
|
|
|
const STATUS_BAR: Style = color(
|
|
|
|
Some(Color::Rgb(29, 31, 33)),
|
|
|
|
Some(Color::Rgb(196, 201, 198)),
|
|
|
|
);
|
|
|
|
|
|
|
|
const INPUT_BAR: Style = color(Some(Color::Rgb(196, 201, 198)), None);
|
|
|
|
|
|
|
|
const HIGHLIGHT: Style = color(Some(Color::Black), Some(Color::Yellow));
|
|
|
|
|
|
|
|
const STATUS_ERROR: Style = color(Some(Color::White), Some(Color::Red));
|
|
|
|
|
|
|
|
const STATUS_INFO: Style = color(None, None);
|
|
|
|
|
|
|
|
const STATUS_WARN: Style = color(None, None);
|
|
|
|
|
|
|
|
const TABLE_SPLIT_LINE: Style = color(Some(Color::Rgb(64, 64, 64)), None);
|
|
|
|
|
|
|
|
const TABLE_LINE_HEADER_TOP: bool = true;
|
|
|
|
|
|
|
|
const TABLE_LINE_HEADER_BOTTOM: bool = true;
|
|
|
|
|
|
|
|
const TABLE_LINE_INDEX: bool = true;
|
|
|
|
|
|
|
|
const TABLE_LINE_SHIFT: bool = true;
|
|
|
|
|
|
|
|
const TABLE_SELECT_CURSOR: bool = true;
|
|
|
|
|
|
|
|
const TABLE_SELECT_CELL: Style = color(None, None);
|
|
|
|
|
|
|
|
const TABLE_SELECT_ROW: Style = color(None, None);
|
|
|
|
|
|
|
|
const TABLE_SELECT_COLUMN: Style = color(None, None);
|
|
|
|
|
|
|
|
const TRY_BORDER_COLOR: Style = color(None, None);
|
|
|
|
|
|
|
|
const CONFIG_CURSOR_COLOR: Style = color(Some(Color::Black), Some(Color::LightYellow));
|
|
|
|
|
|
|
|
insert_style(config, "status_bar_background", STATUS_BAR);
|
|
|
|
insert_style(config, "command_bar_text", INPUT_BAR);
|
|
|
|
insert_style(config, "highlight", HIGHLIGHT);
|
|
|
|
|
|
|
|
// because how config works we need to parse a string into Value::Record
|
|
|
|
|
|
|
|
{
|
|
|
|
let mut hm = config
|
|
|
|
.get("status")
|
|
|
|
.and_then(parse_hash_map)
|
|
|
|
.unwrap_or_default();
|
|
|
|
|
|
|
|
insert_style(&mut hm, "info", STATUS_INFO);
|
|
|
|
insert_style(&mut hm, "warn", STATUS_WARN);
|
|
|
|
insert_style(&mut hm, "error", STATUS_ERROR);
|
|
|
|
|
|
|
|
config.insert(String::from("status"), map_into_value(hm));
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
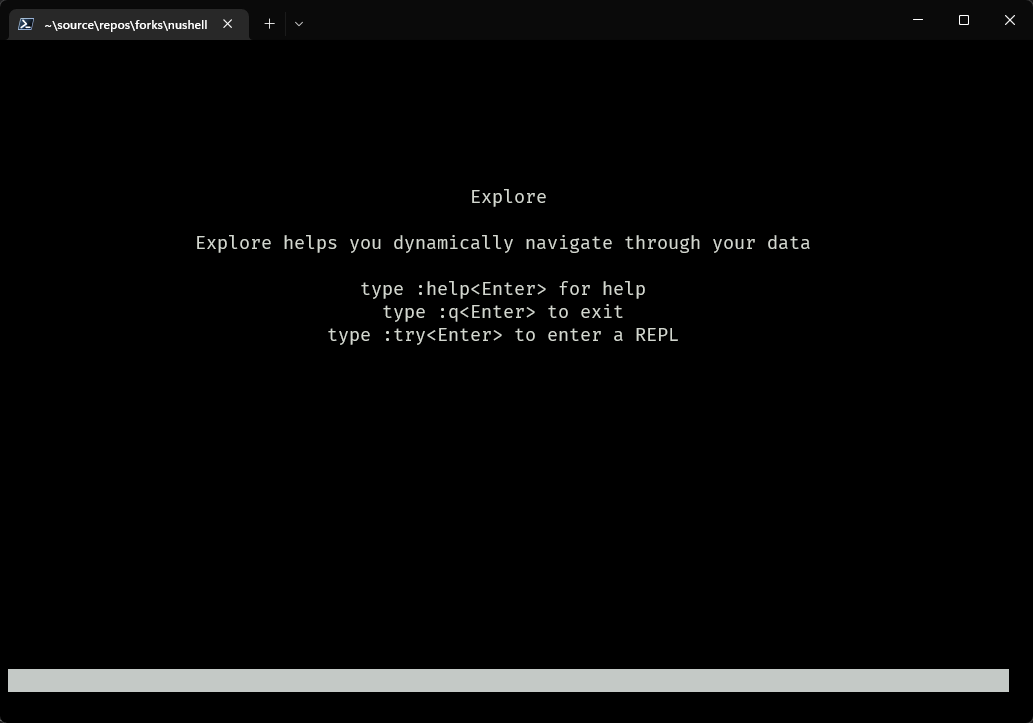
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
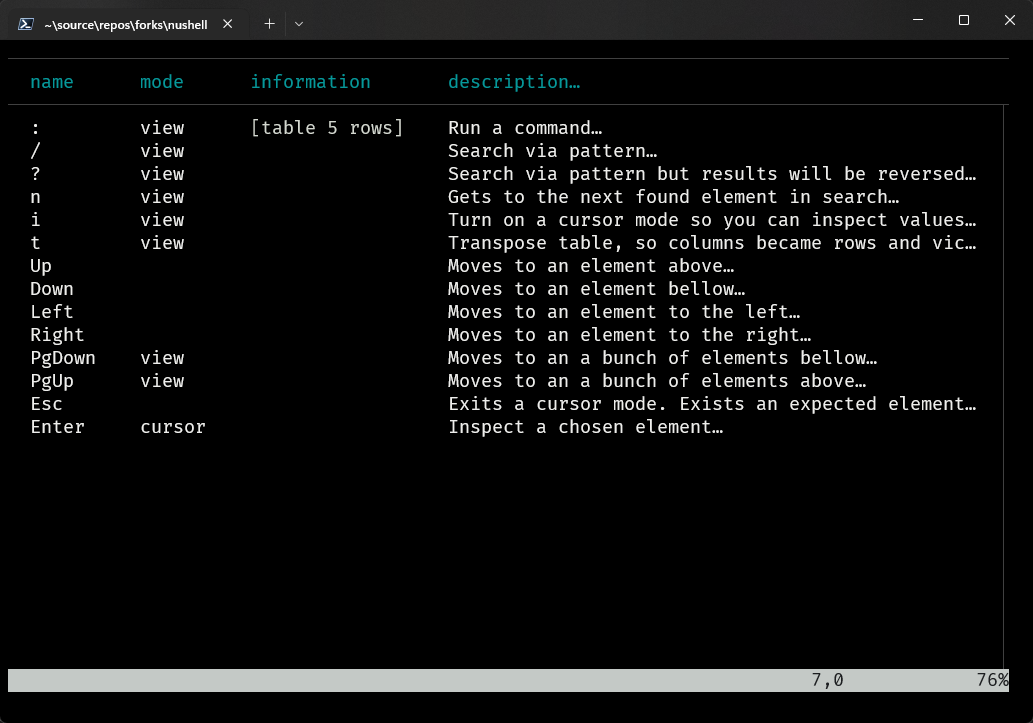
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
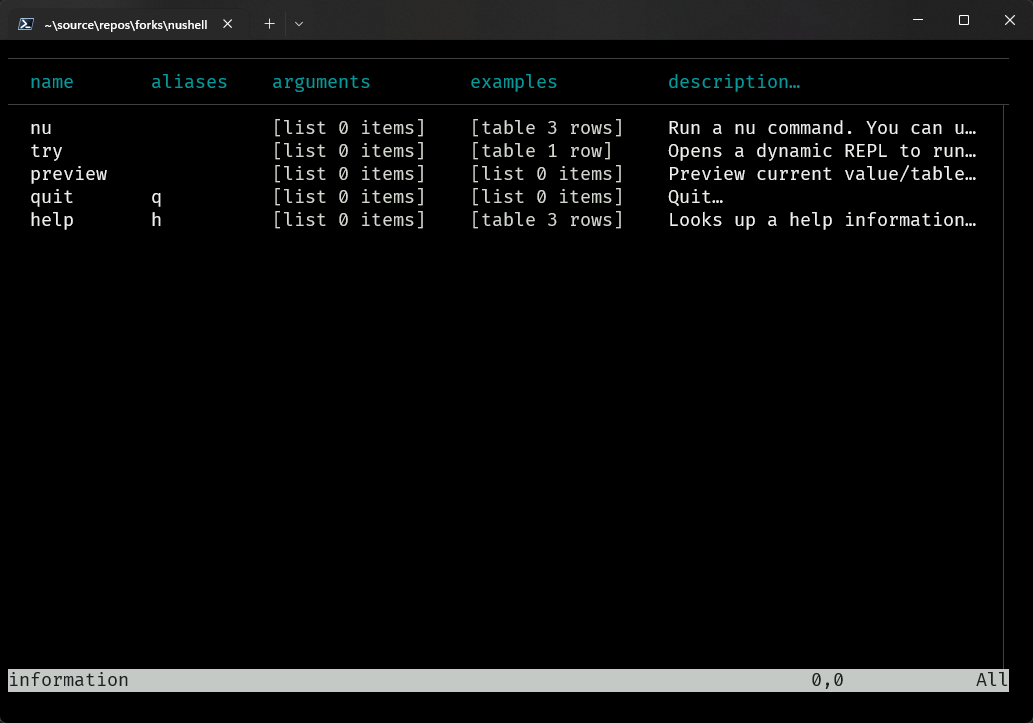
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
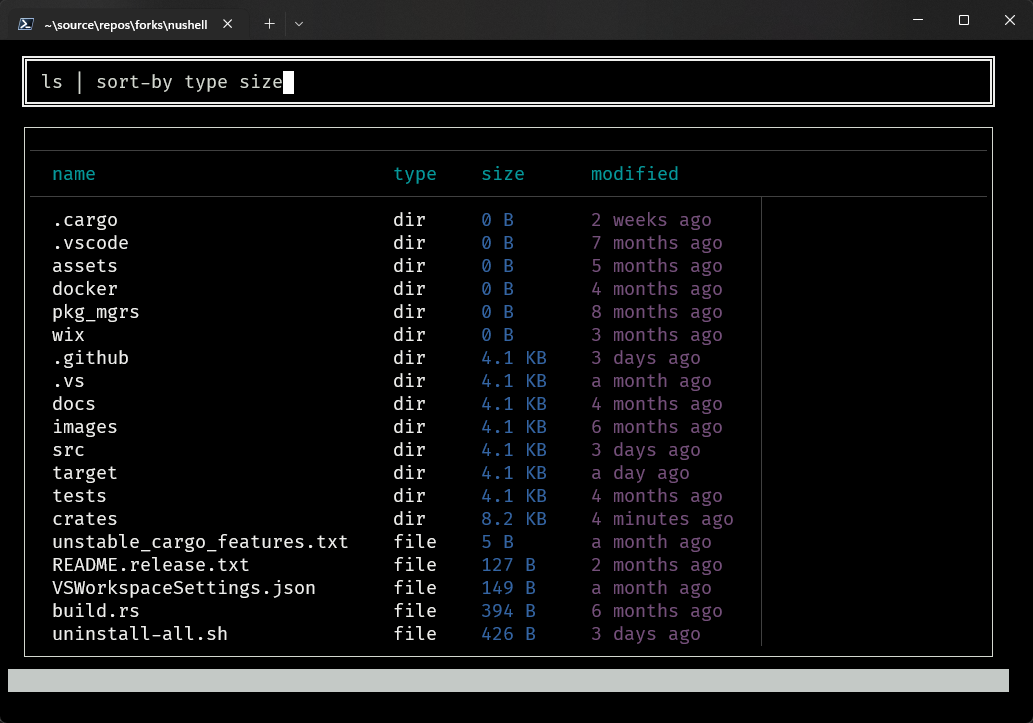
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
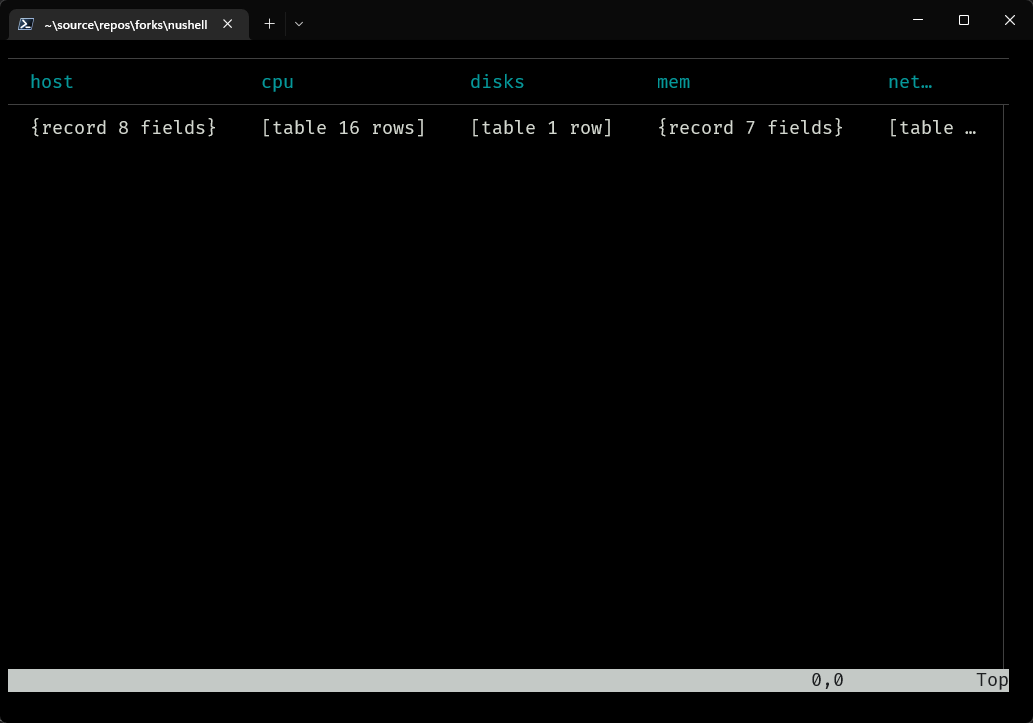
If you hit the `t` key it will now transpose the view to look like this.
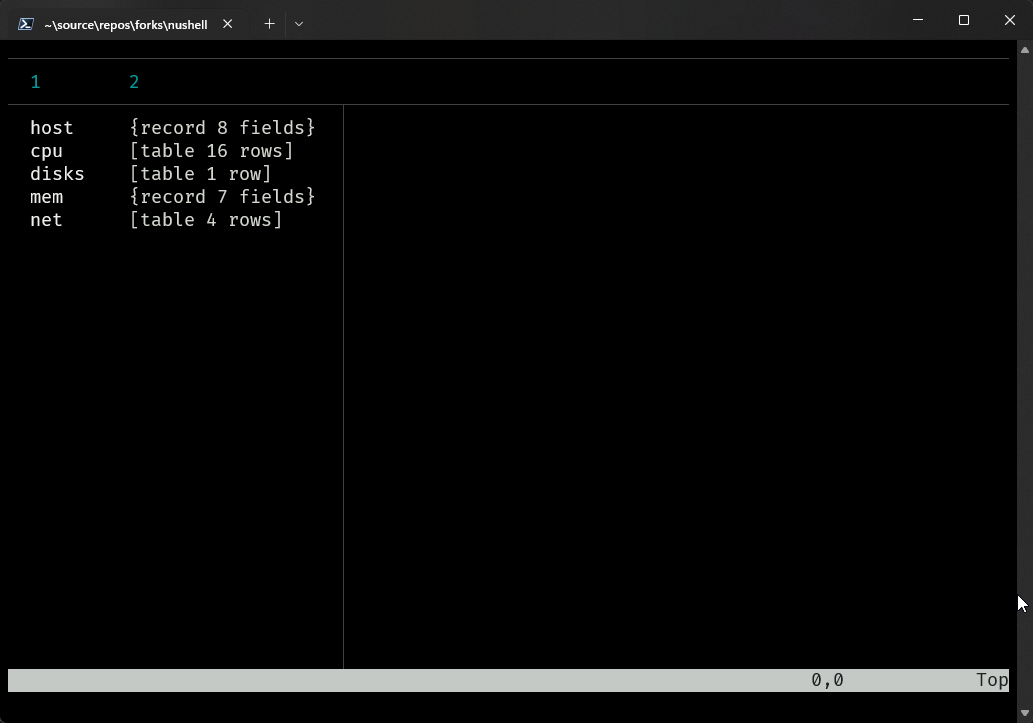
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
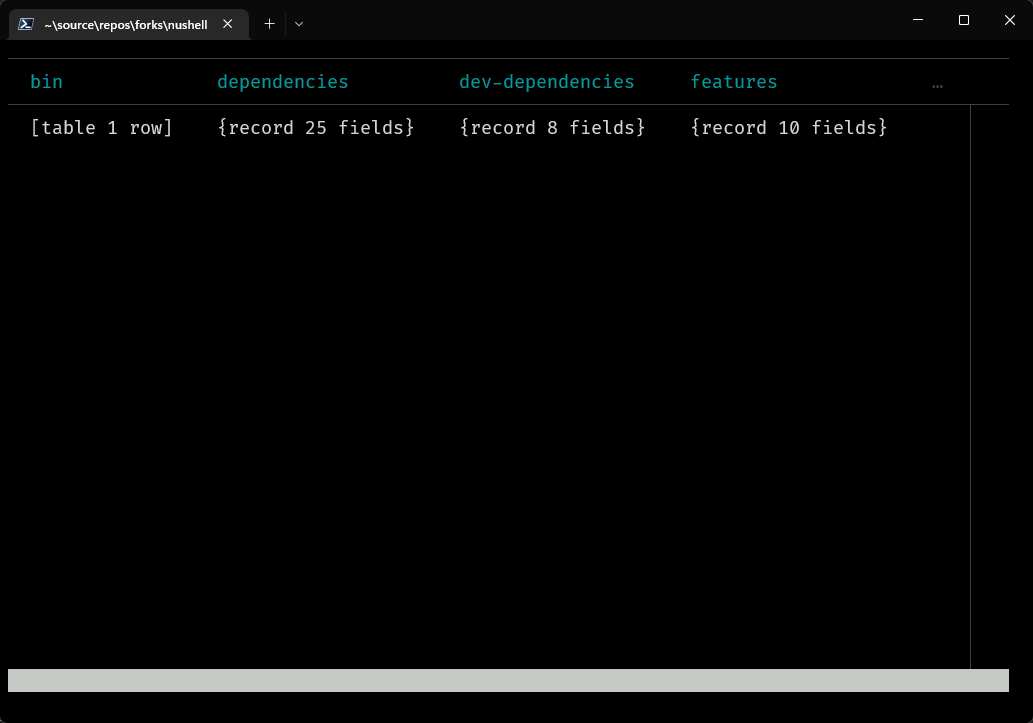
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
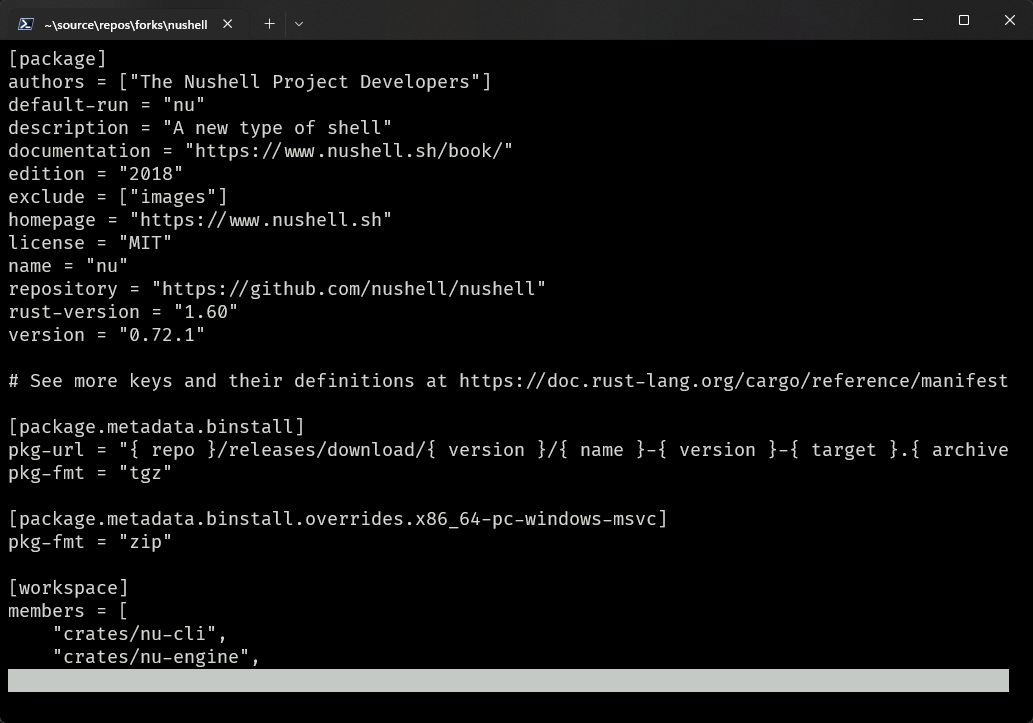
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
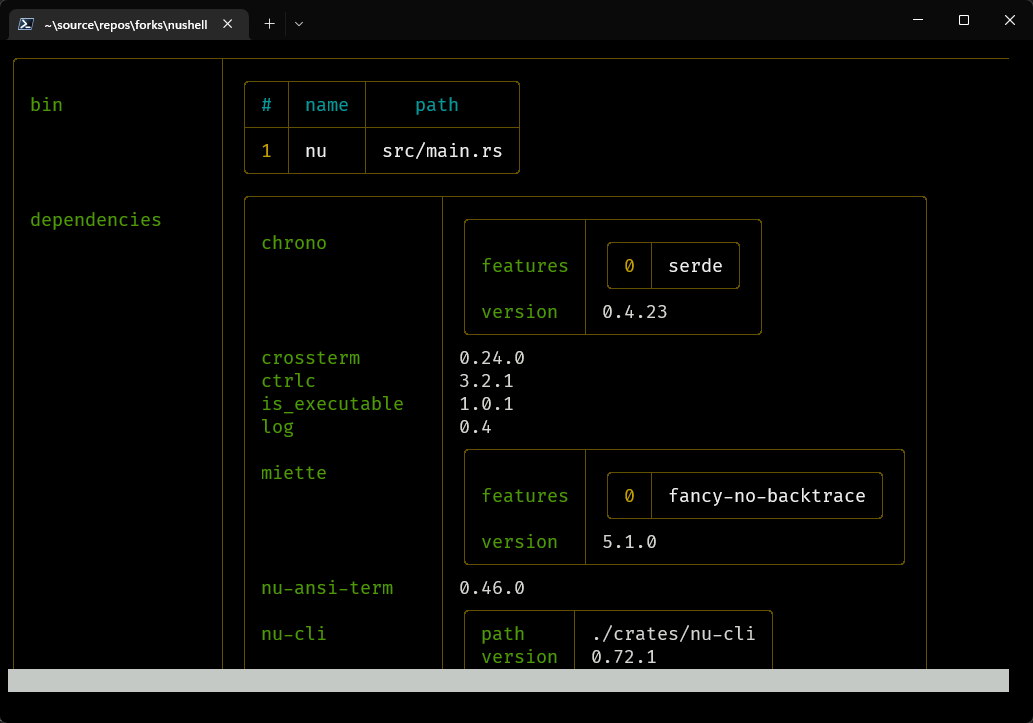
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
}
|
2022-12-18 14:43:15 +00:00
|
|
|
|
2022-12-16 15:47:07 +00:00
|
|
|
{
|
|
|
|
let mut hm = config
|
|
|
|
.get("table")
|
|
|
|
.and_then(parse_hash_map)
|
|
|
|
.unwrap_or_default();
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
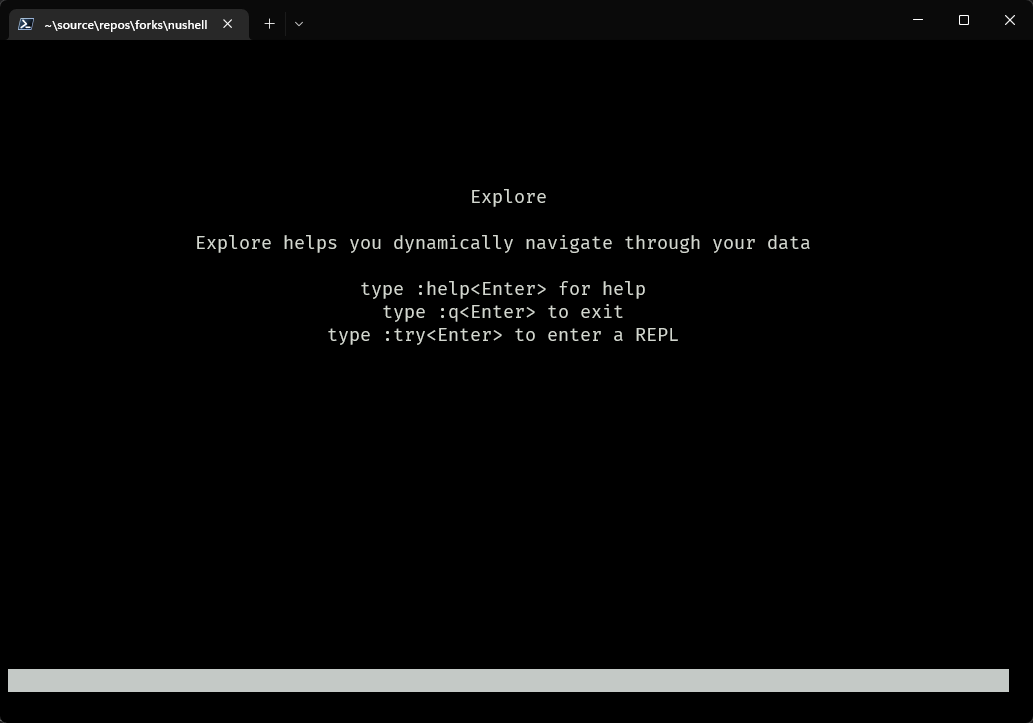
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
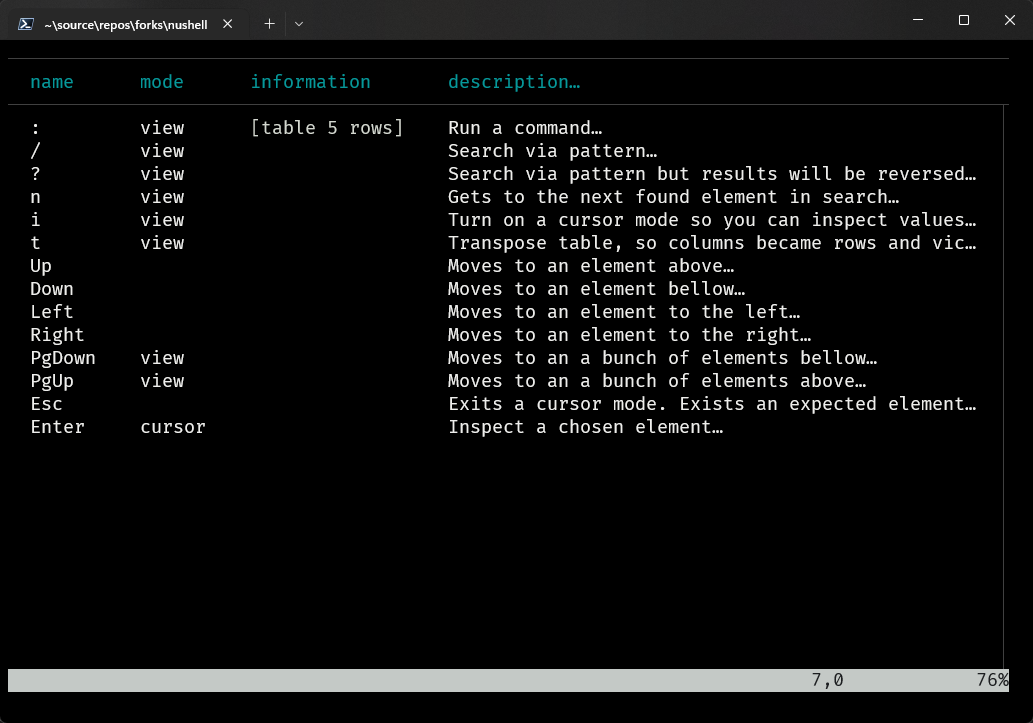
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
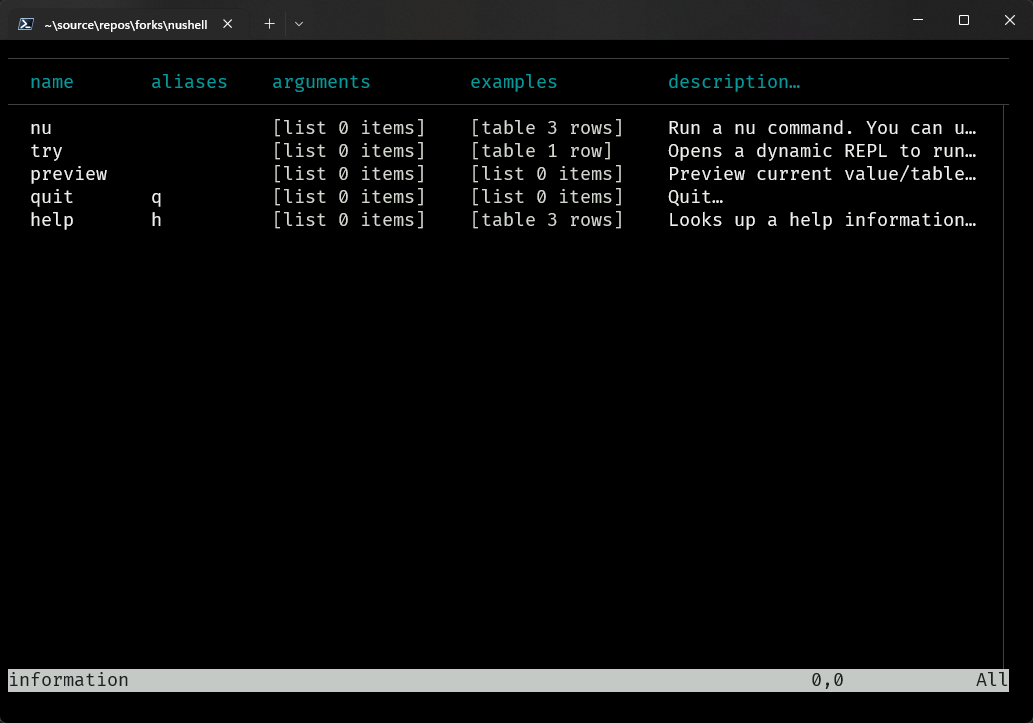
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
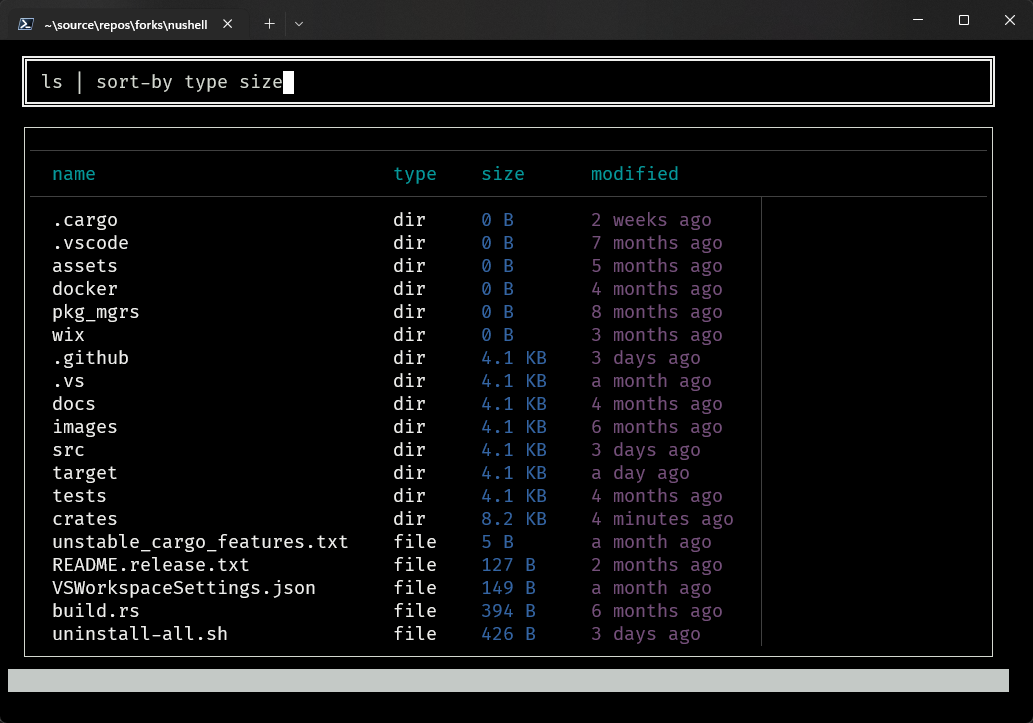
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
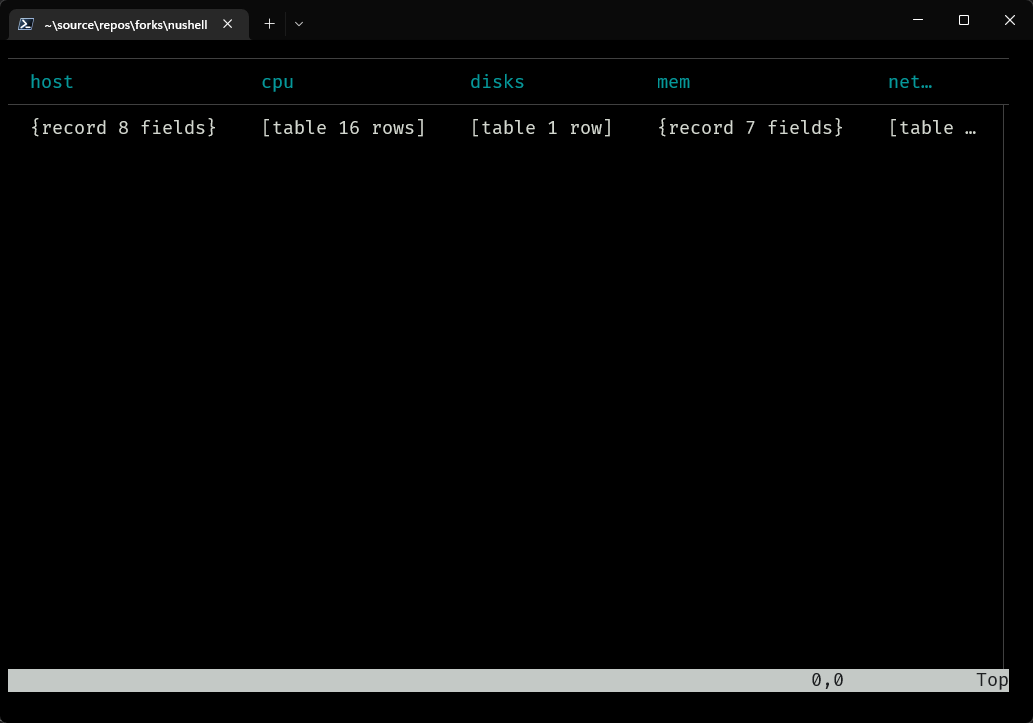
If you hit the `t` key it will now transpose the view to look like this.
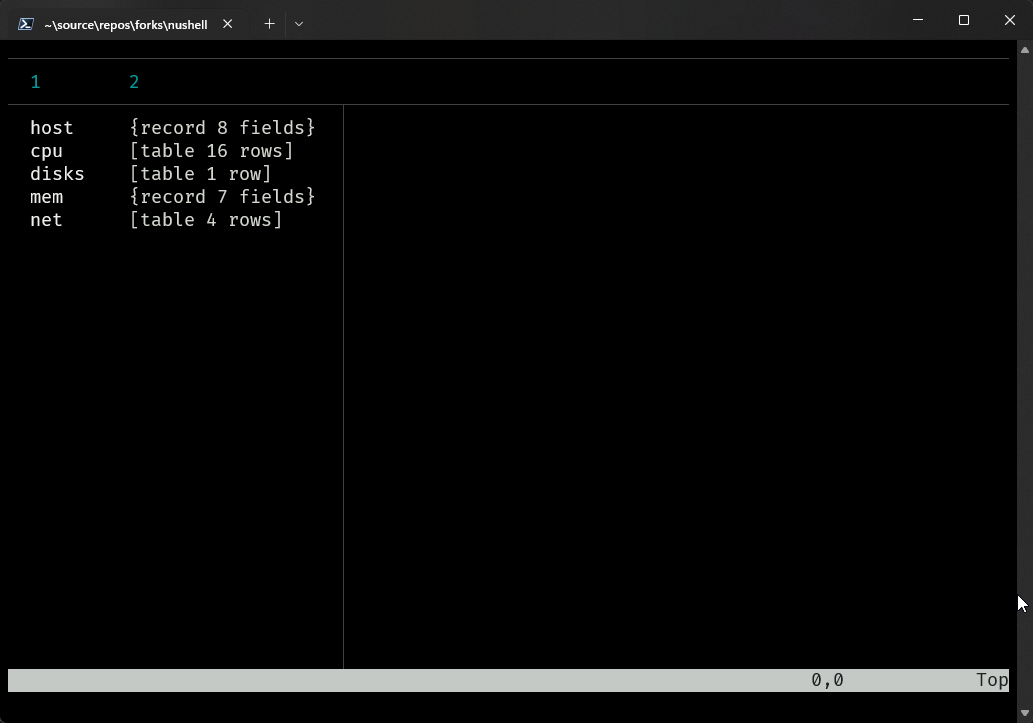
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
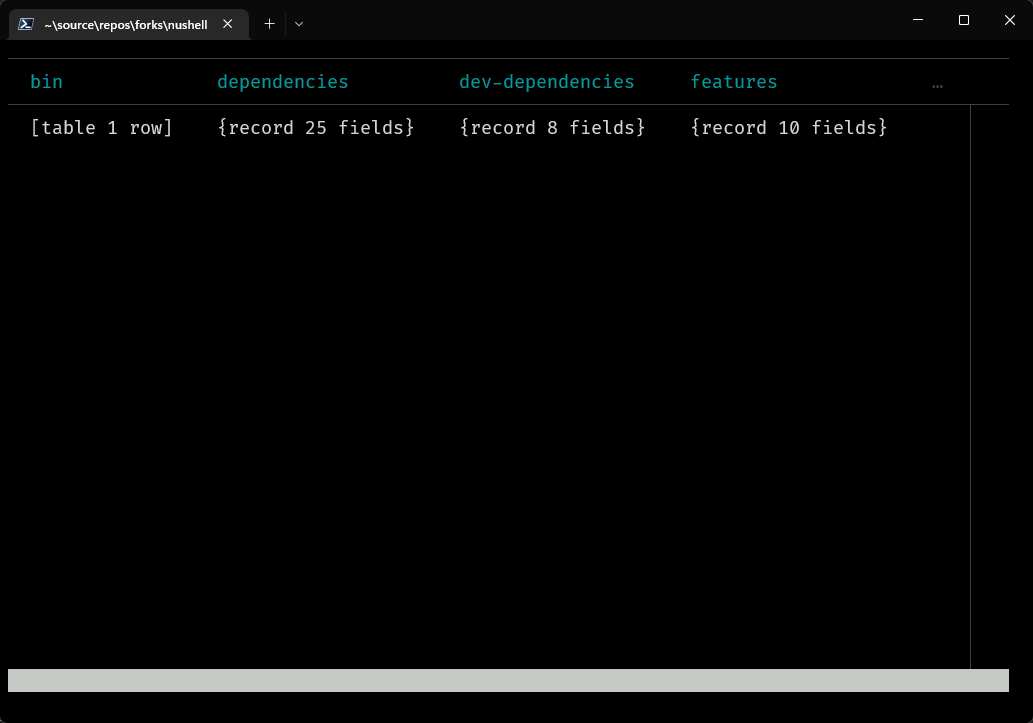
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
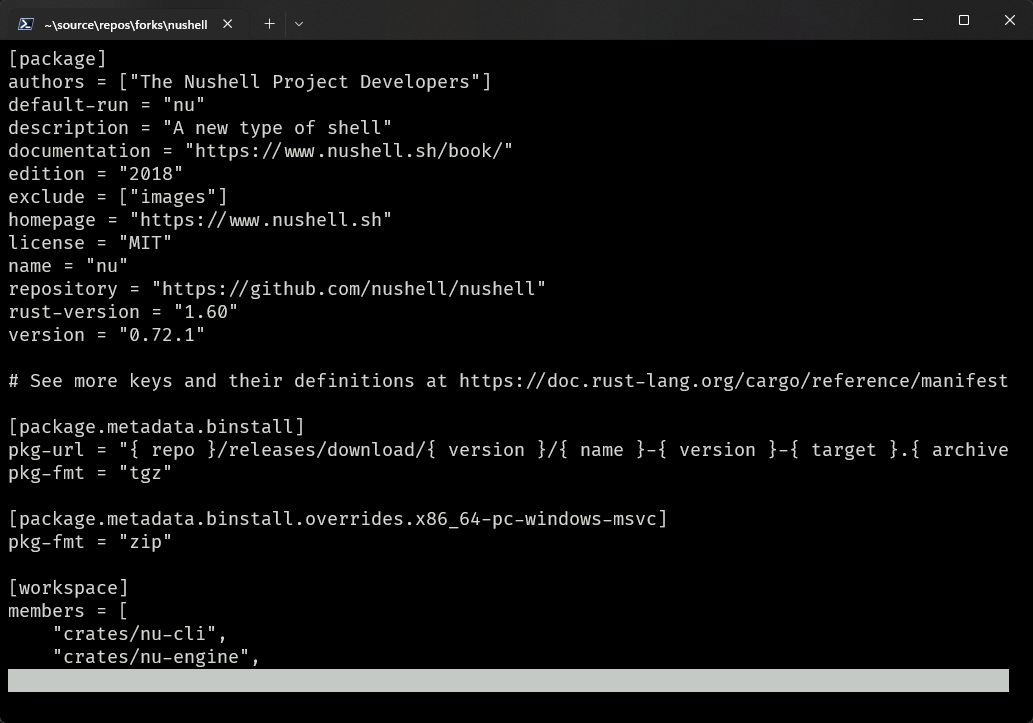
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
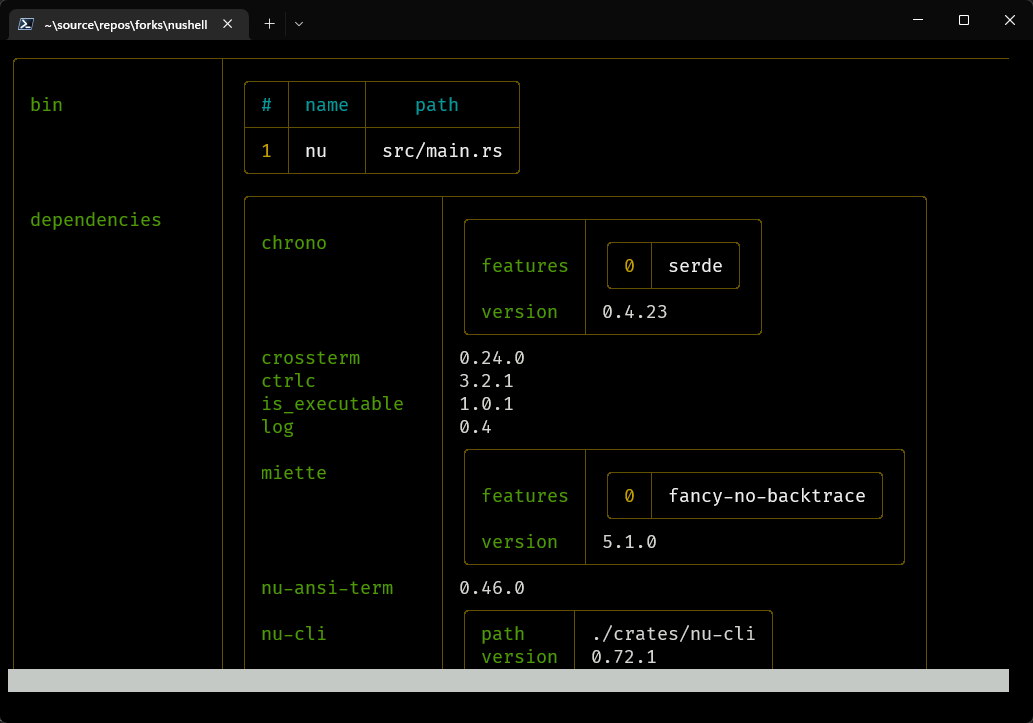
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
|
2022-12-16 15:47:07 +00:00
|
|
|
insert_style(&mut hm, "split_line", TABLE_SPLIT_LINE);
|
|
|
|
insert_style(&mut hm, "selected_cell", TABLE_SELECT_CELL);
|
|
|
|
insert_style(&mut hm, "selected_row", TABLE_SELECT_ROW);
|
|
|
|
insert_style(&mut hm, "selected_column", TABLE_SELECT_COLUMN);
|
|
|
|
insert_bool(&mut hm, "cursor", TABLE_SELECT_CURSOR);
|
|
|
|
insert_bool(&mut hm, "line_head_top", TABLE_LINE_HEADER_TOP);
|
|
|
|
insert_bool(&mut hm, "line_head_bottom", TABLE_LINE_HEADER_BOTTOM);
|
|
|
|
insert_bool(&mut hm, "line_shift", TABLE_LINE_SHIFT);
|
|
|
|
insert_bool(&mut hm, "line_index", TABLE_LINE_INDEX);
|
|
|
|
|
|
|
|
config.insert(String::from("table"), map_into_value(hm));
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
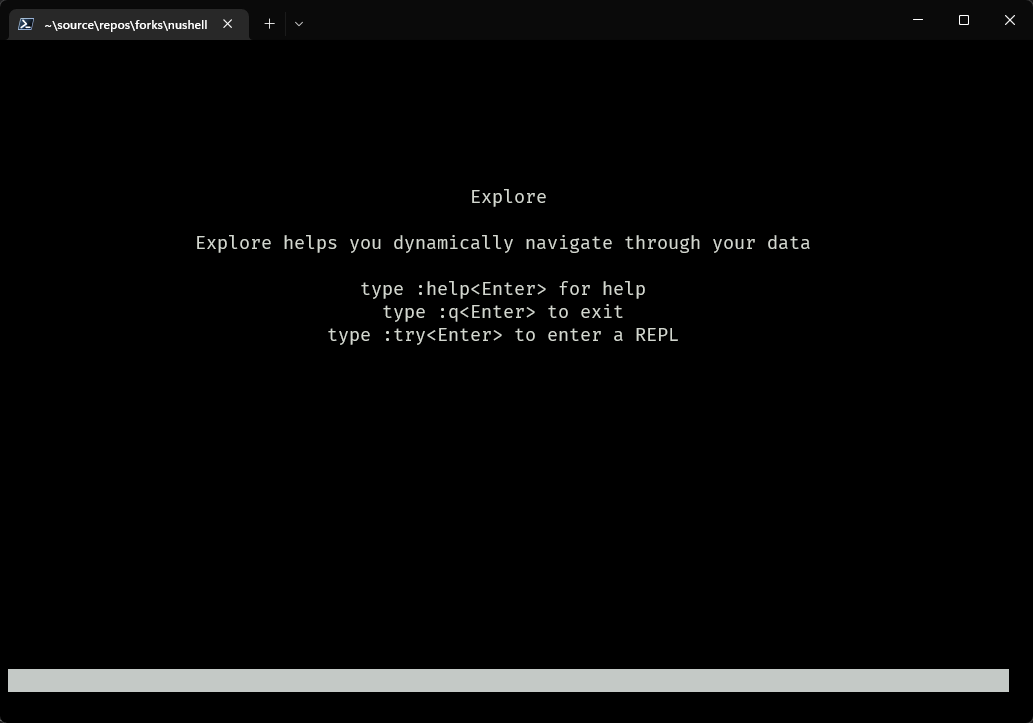
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
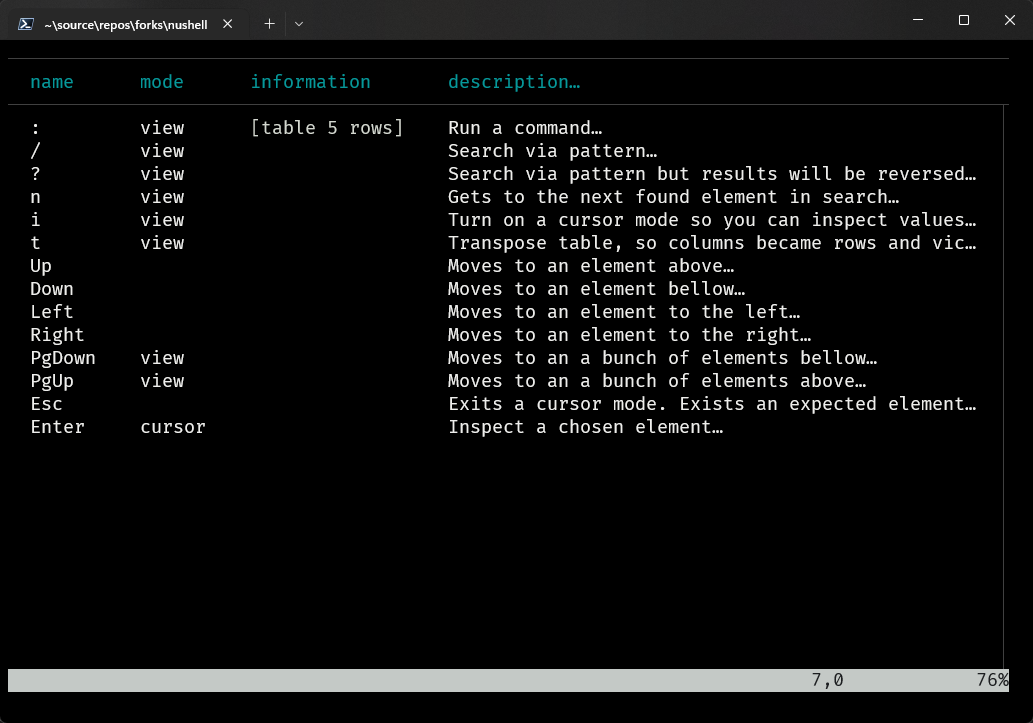
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
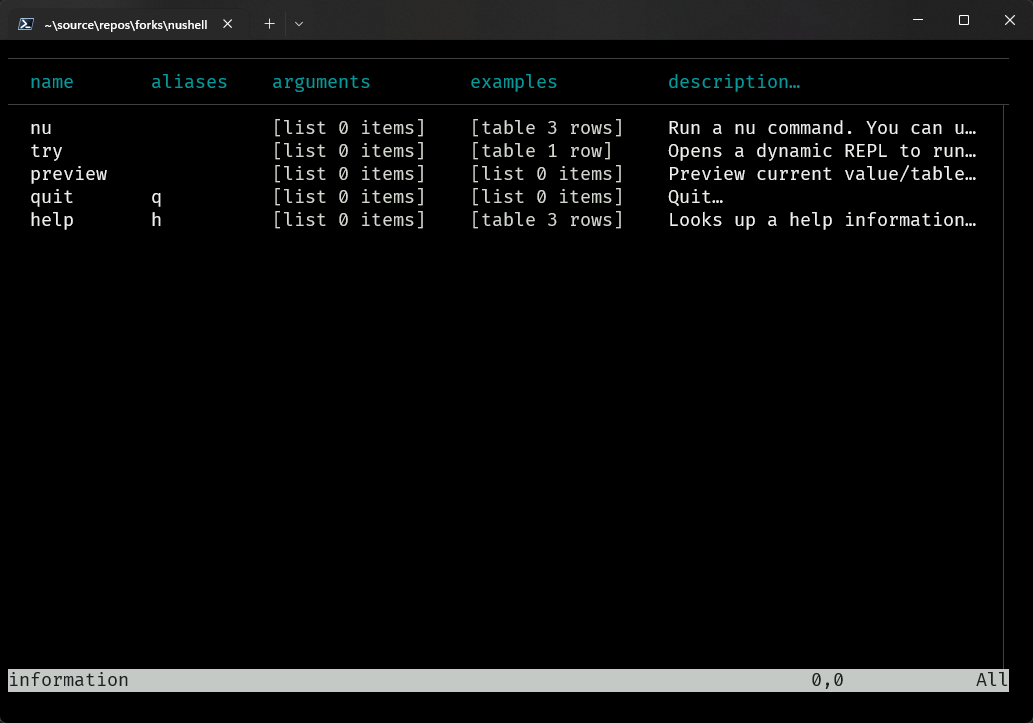
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
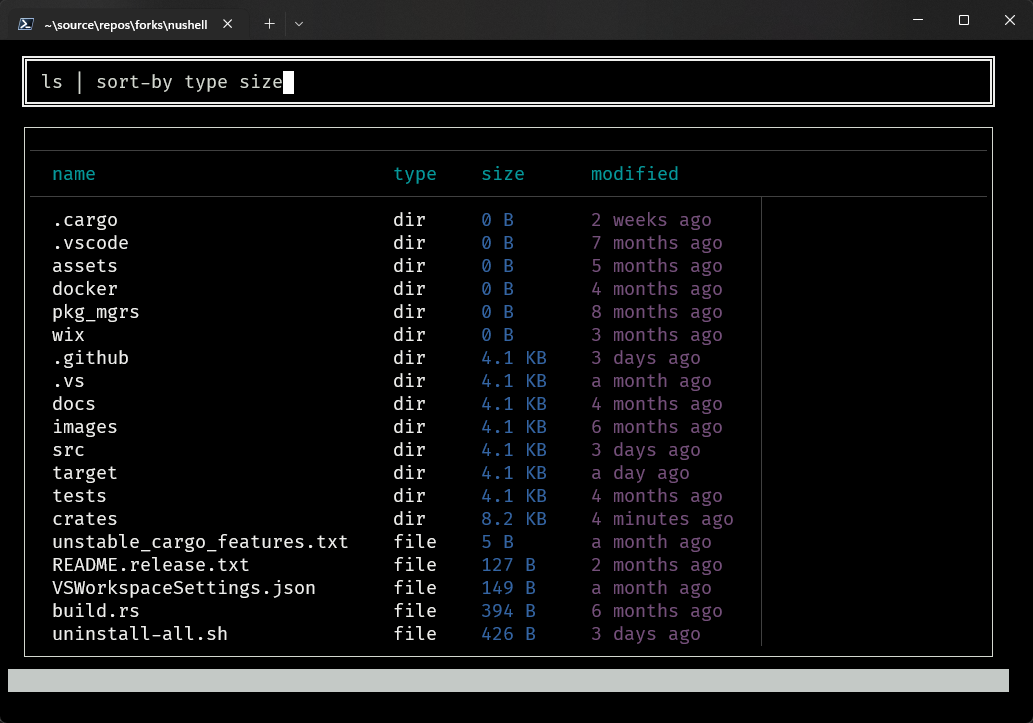
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
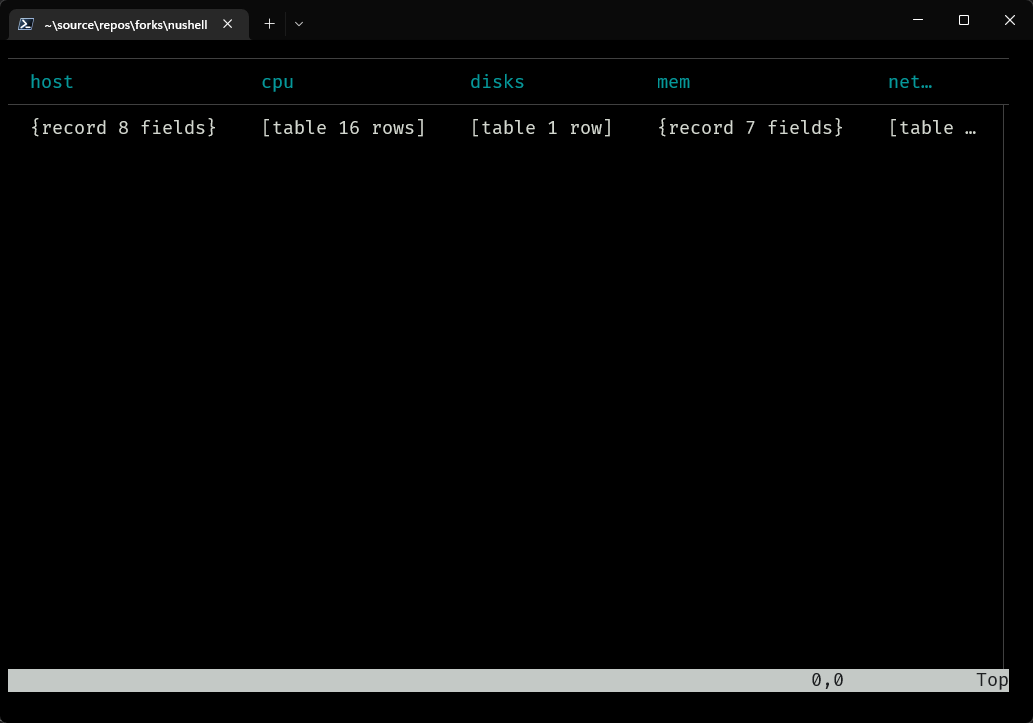
If you hit the `t` key it will now transpose the view to look like this.
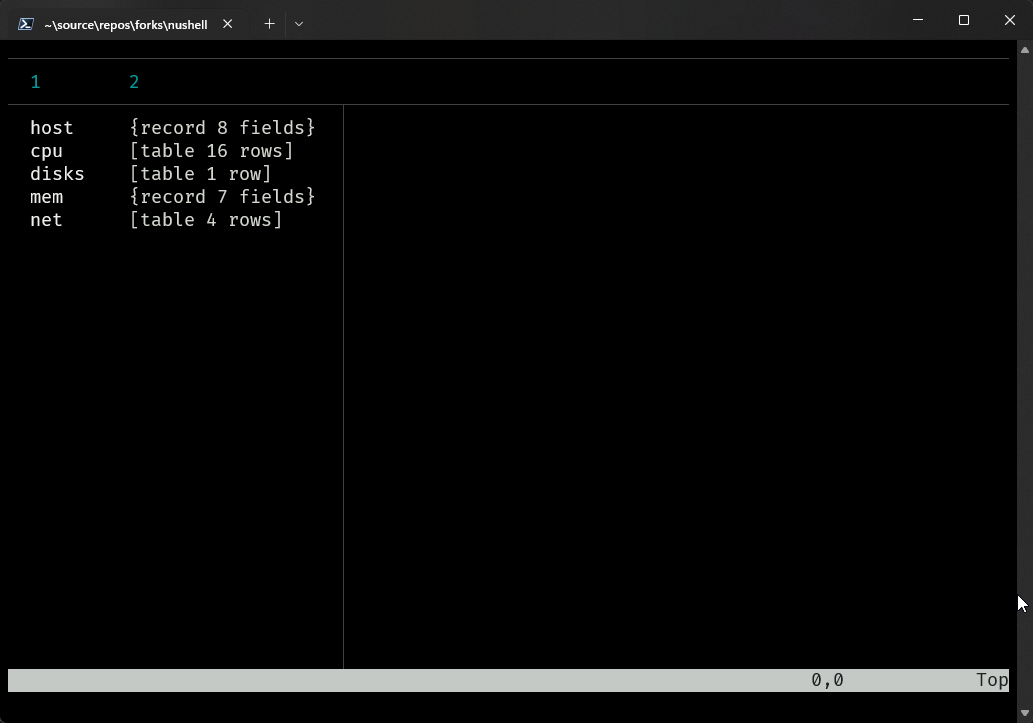
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
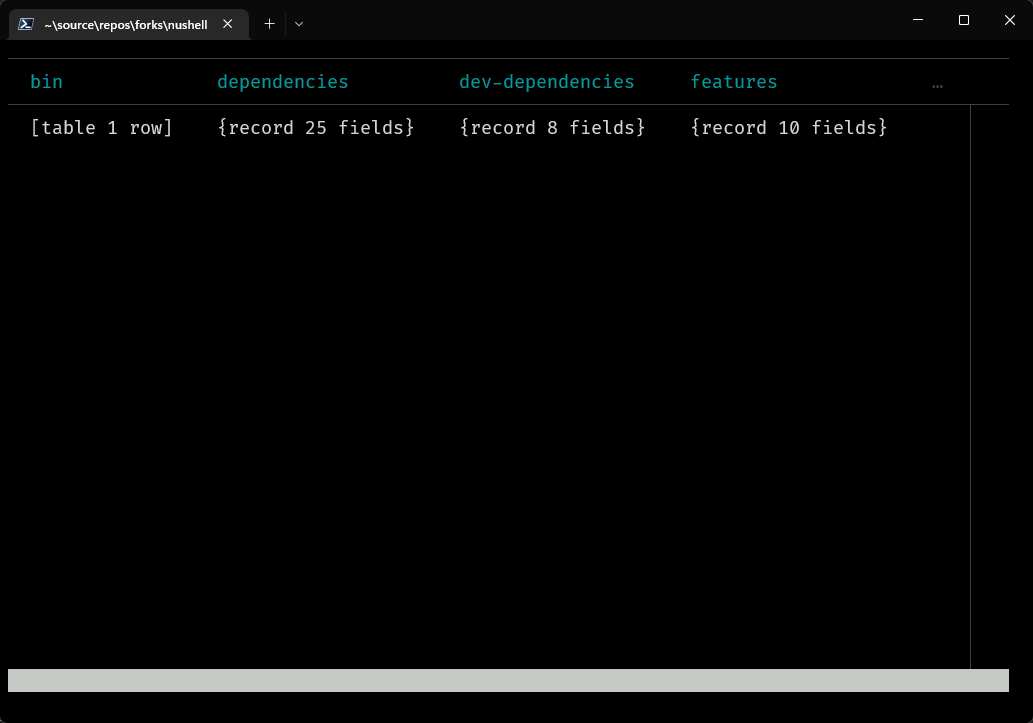
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
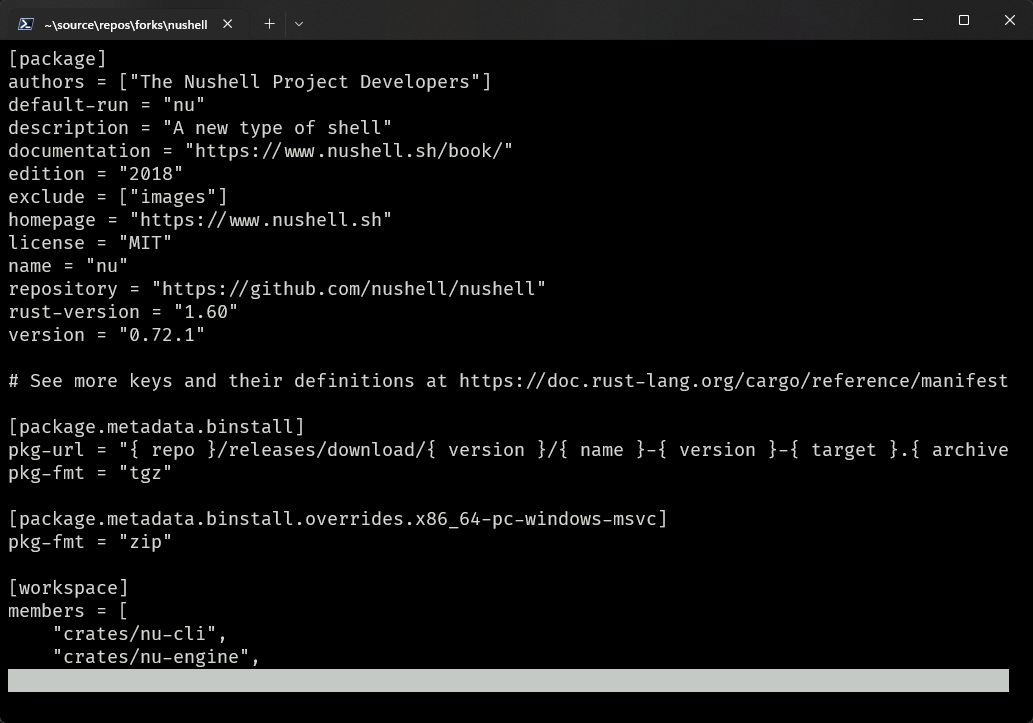
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
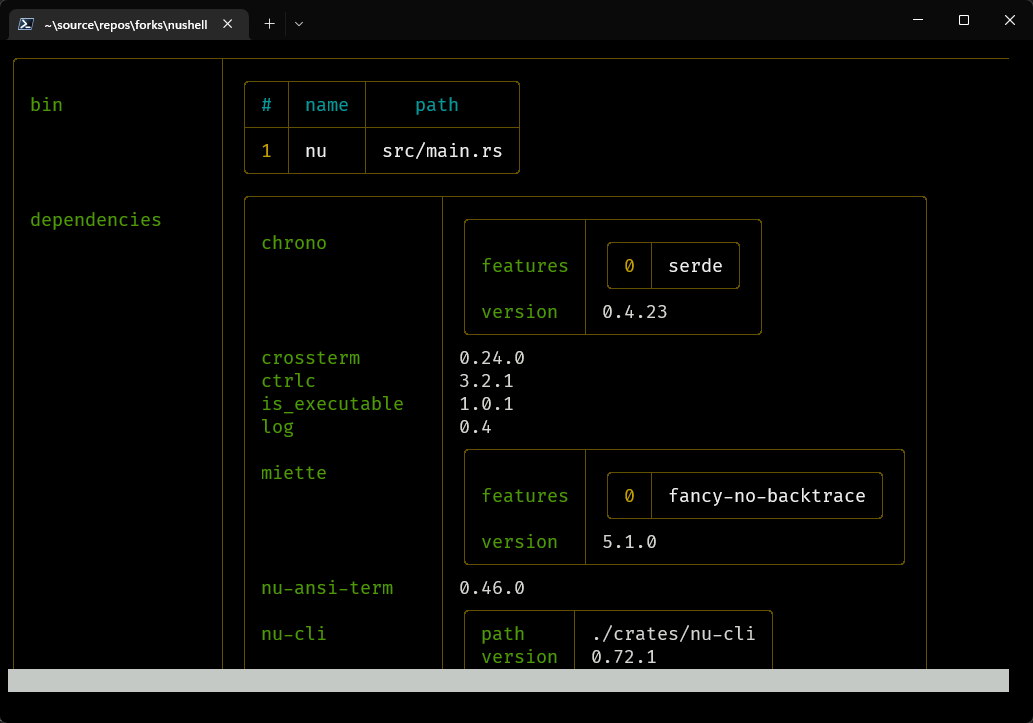
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
}
|
|
|
|
|
2022-12-16 15:47:07 +00:00
|
|
|
{
|
|
|
|
let mut hm = config
|
|
|
|
.get("try")
|
|
|
|
.and_then(parse_hash_map)
|
|
|
|
.unwrap_or_default();
|
|
|
|
|
|
|
|
insert_style(&mut hm, "border_color", TRY_BORDER_COLOR);
|
|
|
|
|
|
|
|
config.insert(String::from("try"), map_into_value(hm));
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
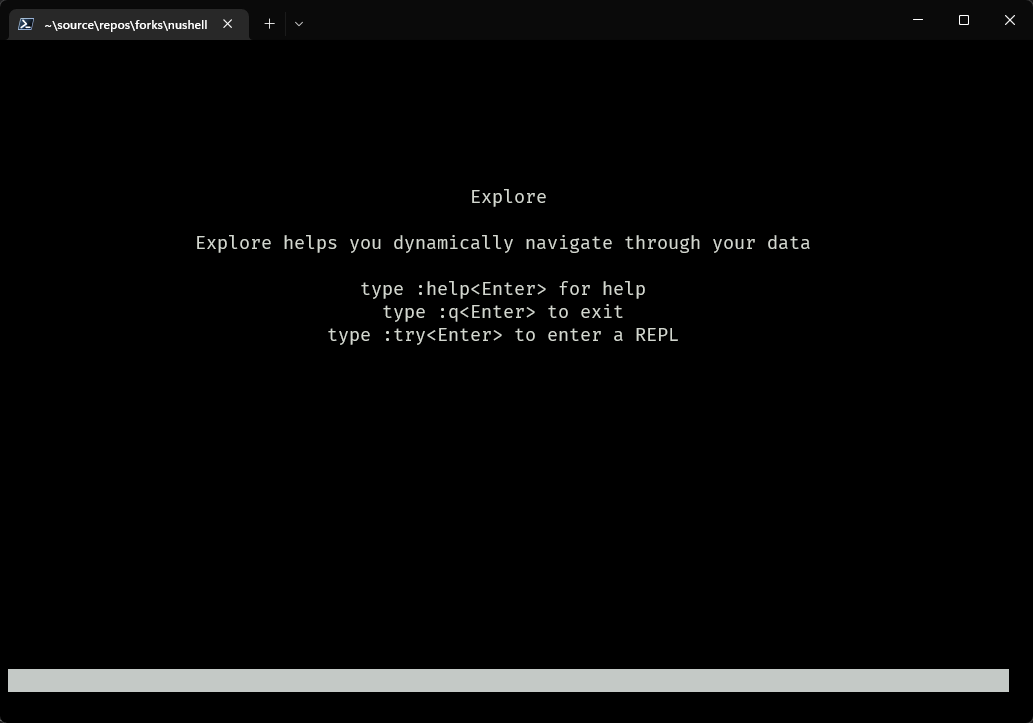
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
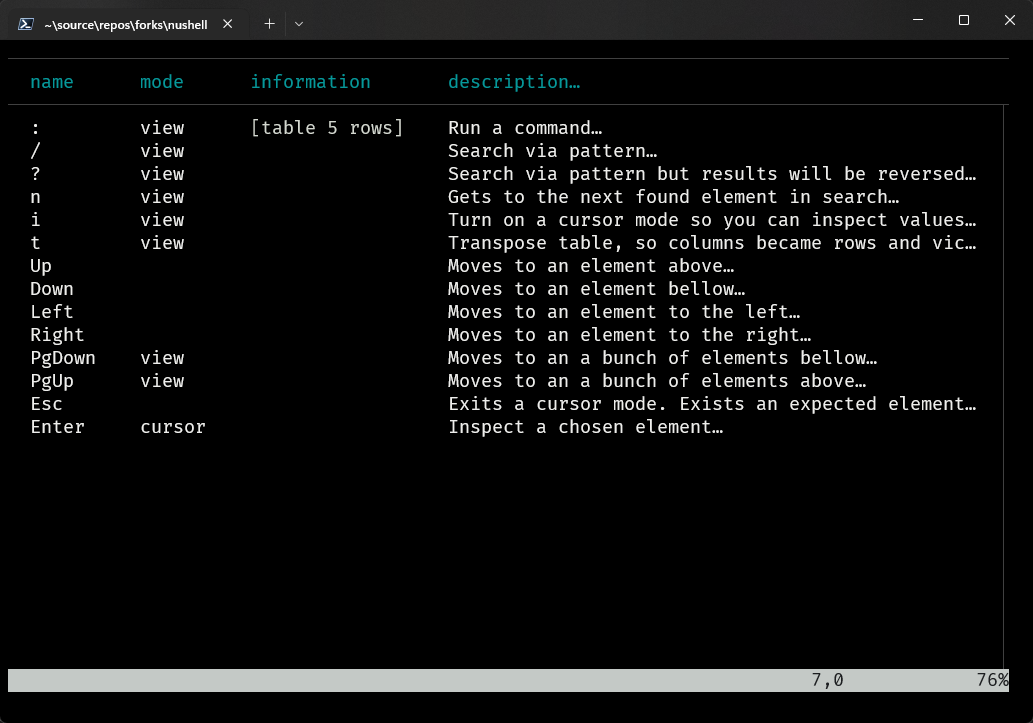
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
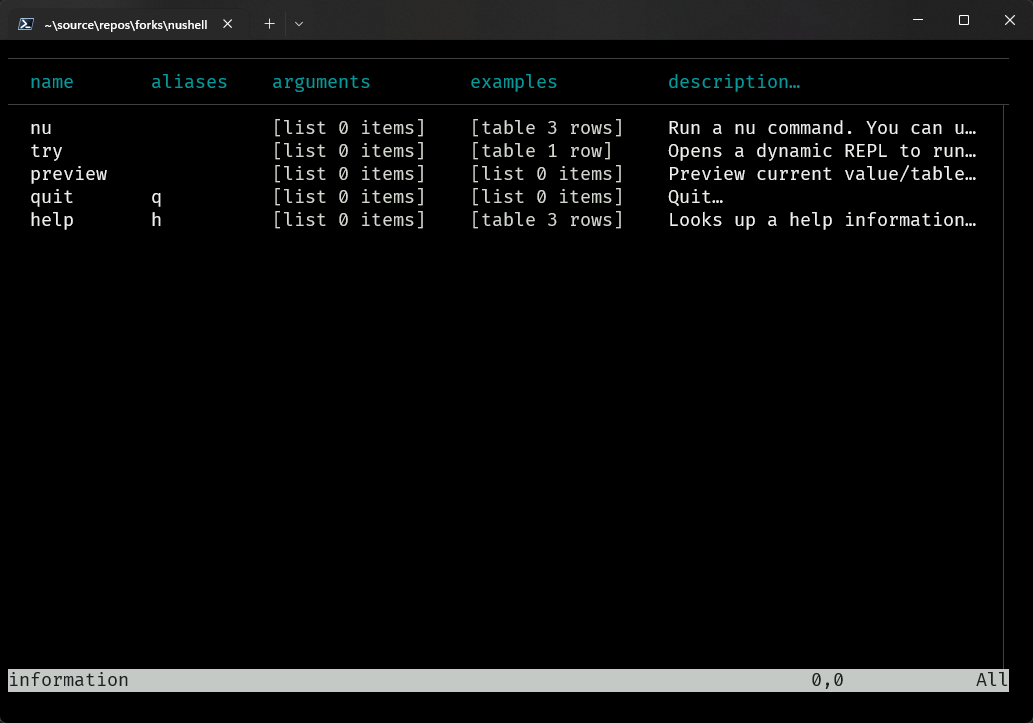
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
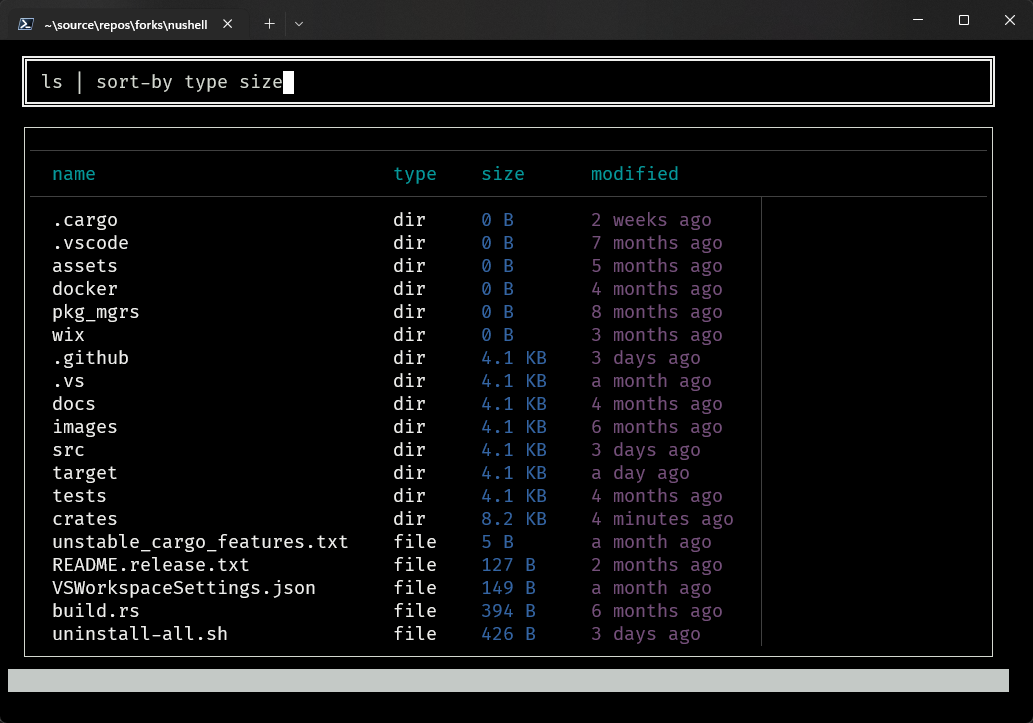
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
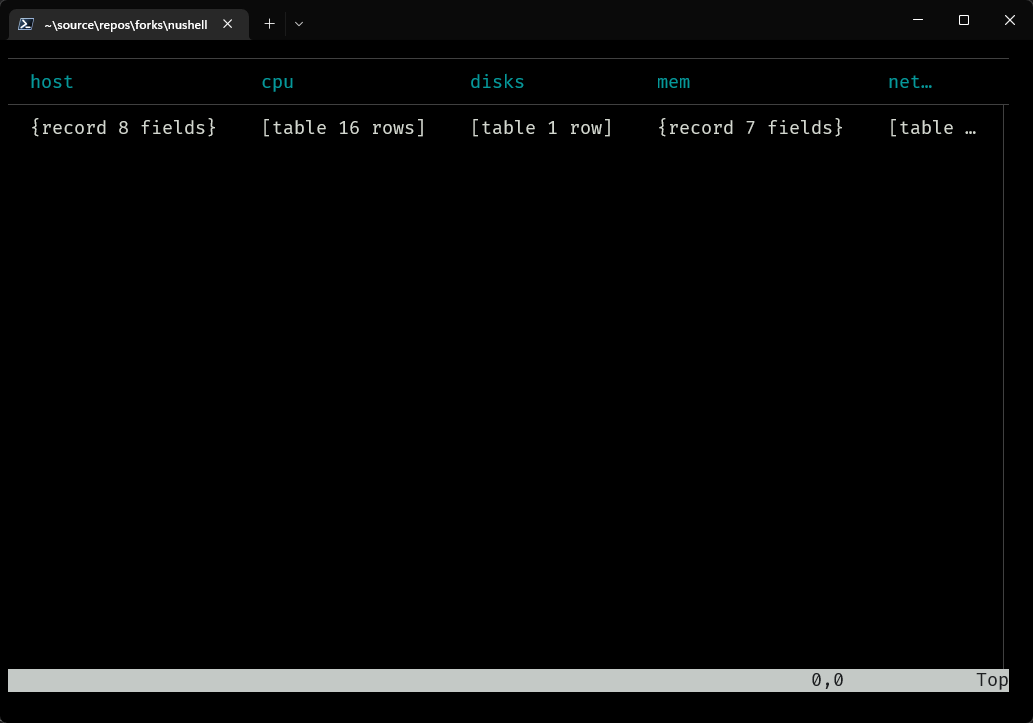
If you hit the `t` key it will now transpose the view to look like this.
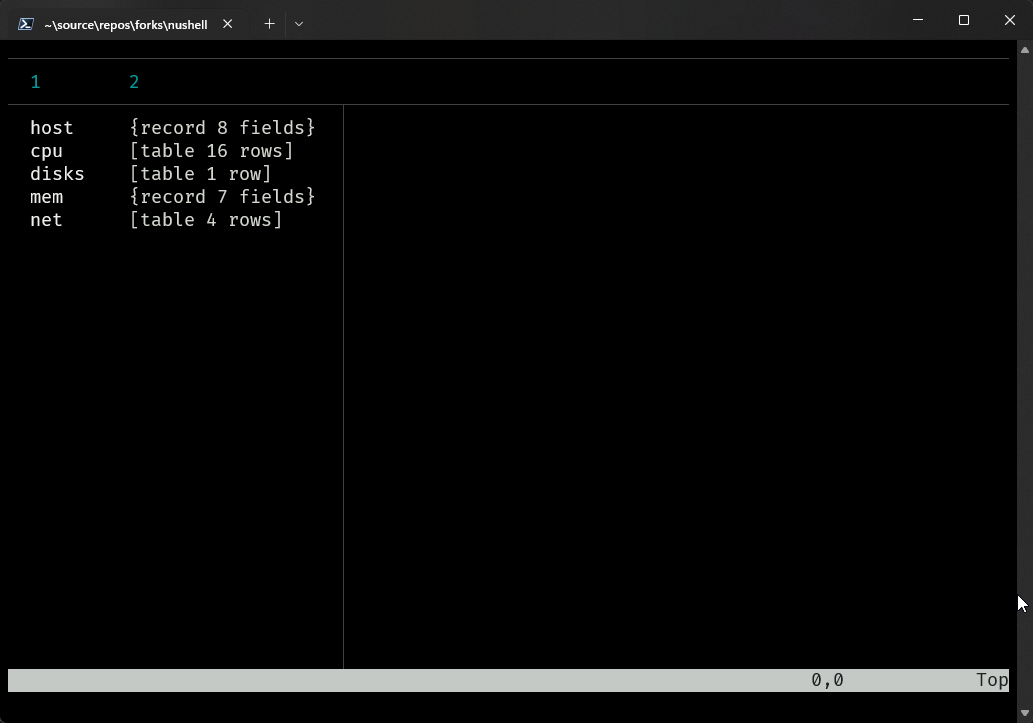
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
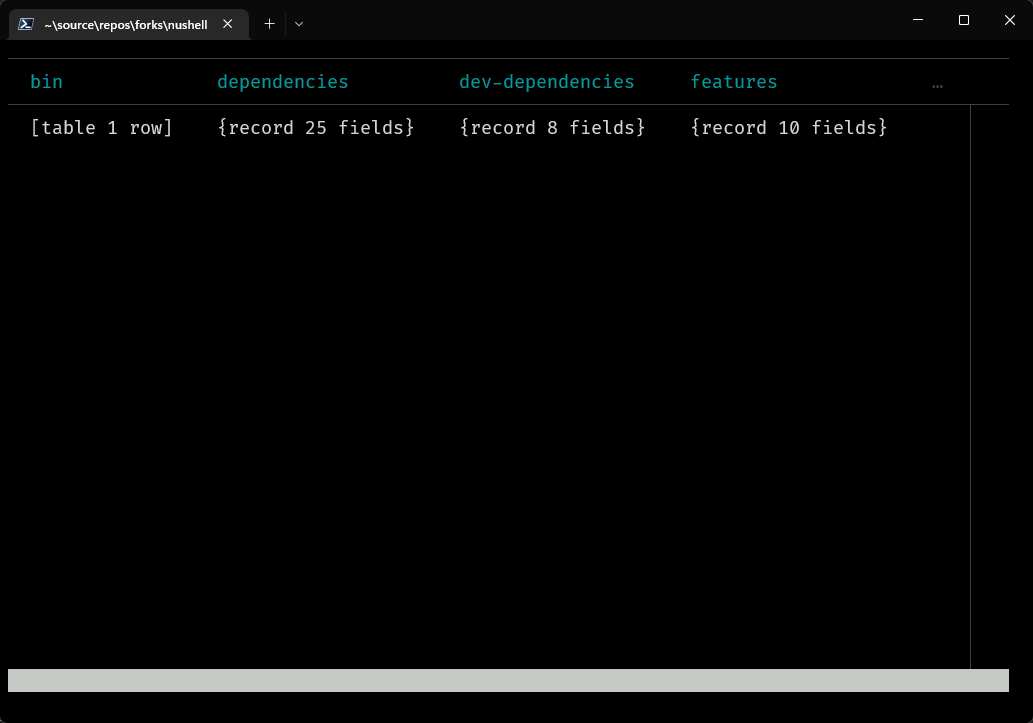
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
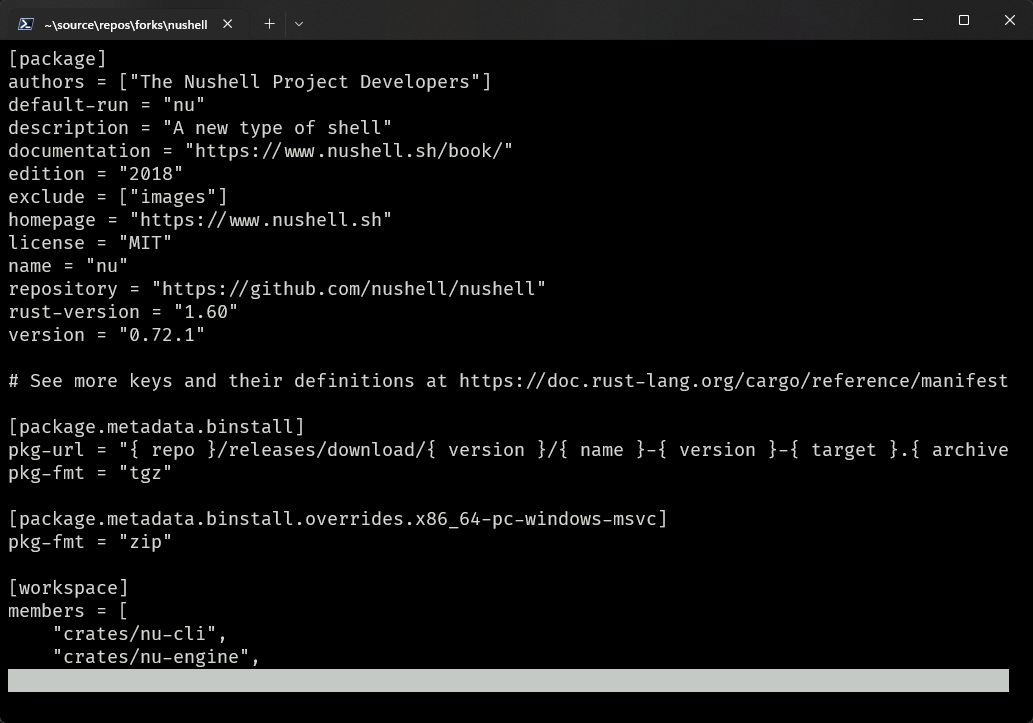
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
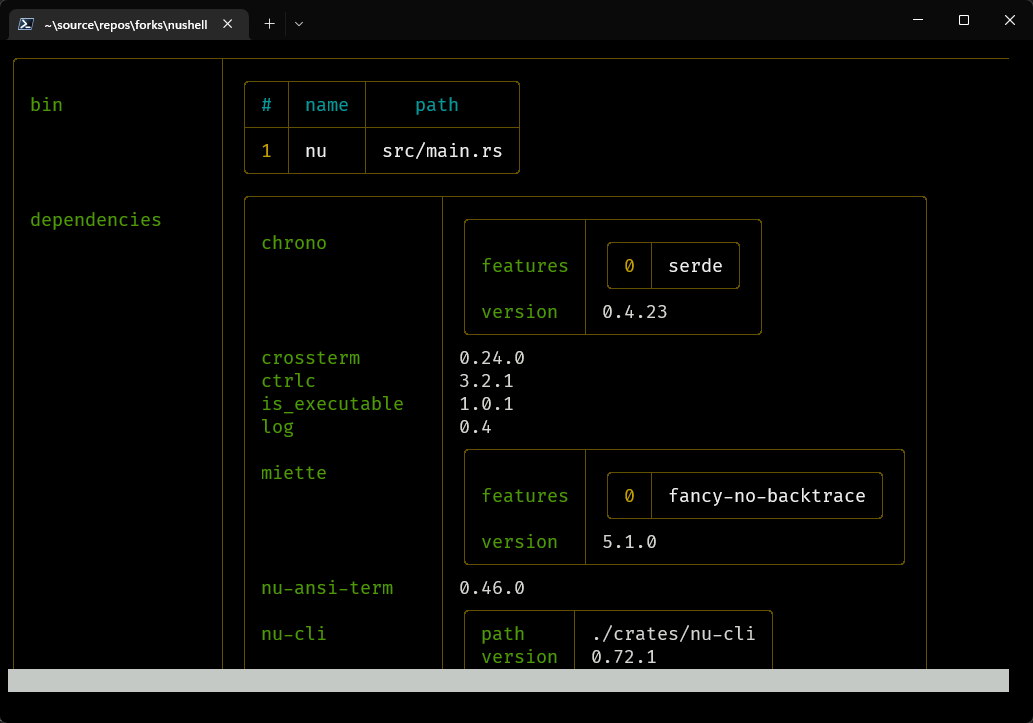
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
{
|
2022-12-16 15:47:07 +00:00
|
|
|
let mut hm = config
|
|
|
|
.get("config")
|
|
|
|
.and_then(parse_hash_map)
|
|
|
|
.unwrap_or_default();
|
|
|
|
|
|
|
|
insert_style(&mut hm, "cursor_color", CONFIG_CURSOR_COLOR);
|
|
|
|
|
|
|
|
config.insert(String::from("config"), map_into_value(hm));
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
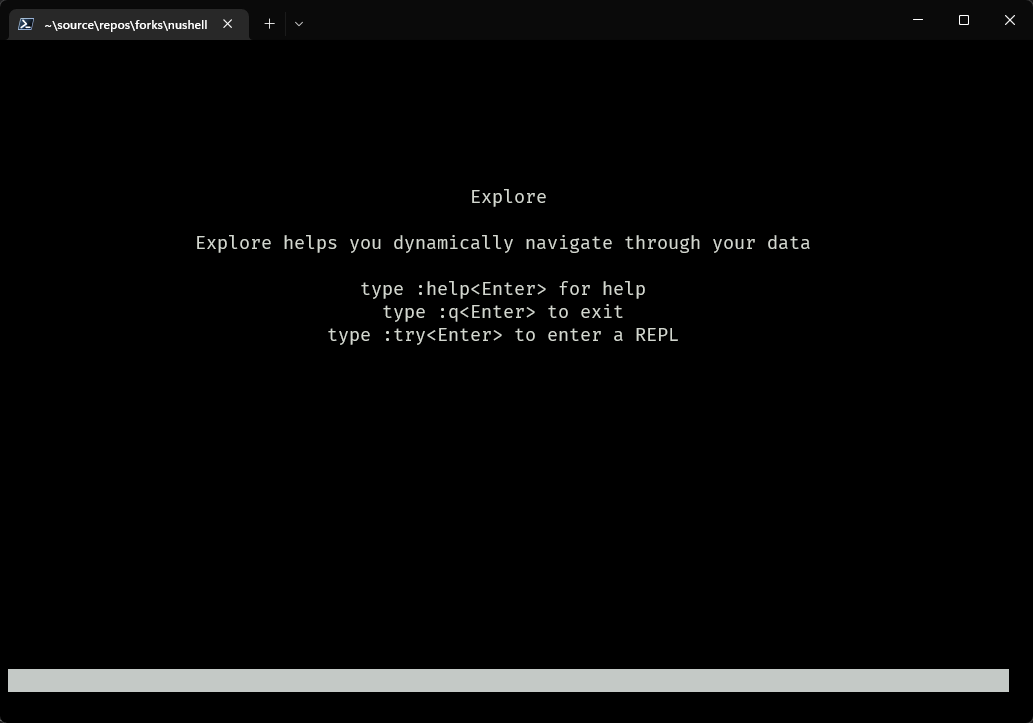
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
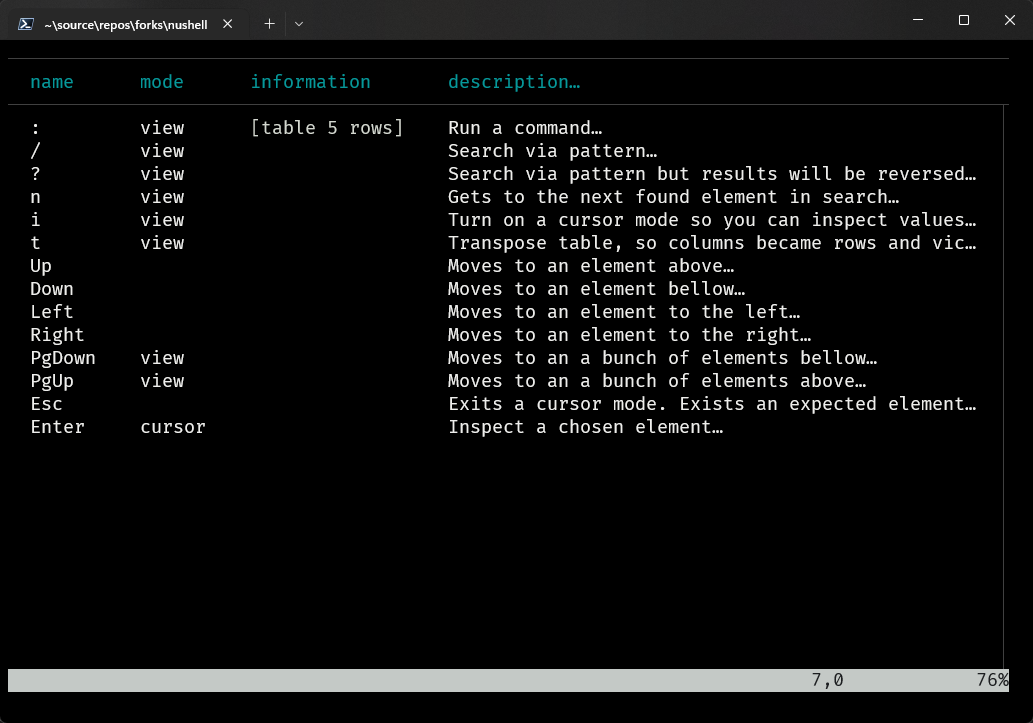
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
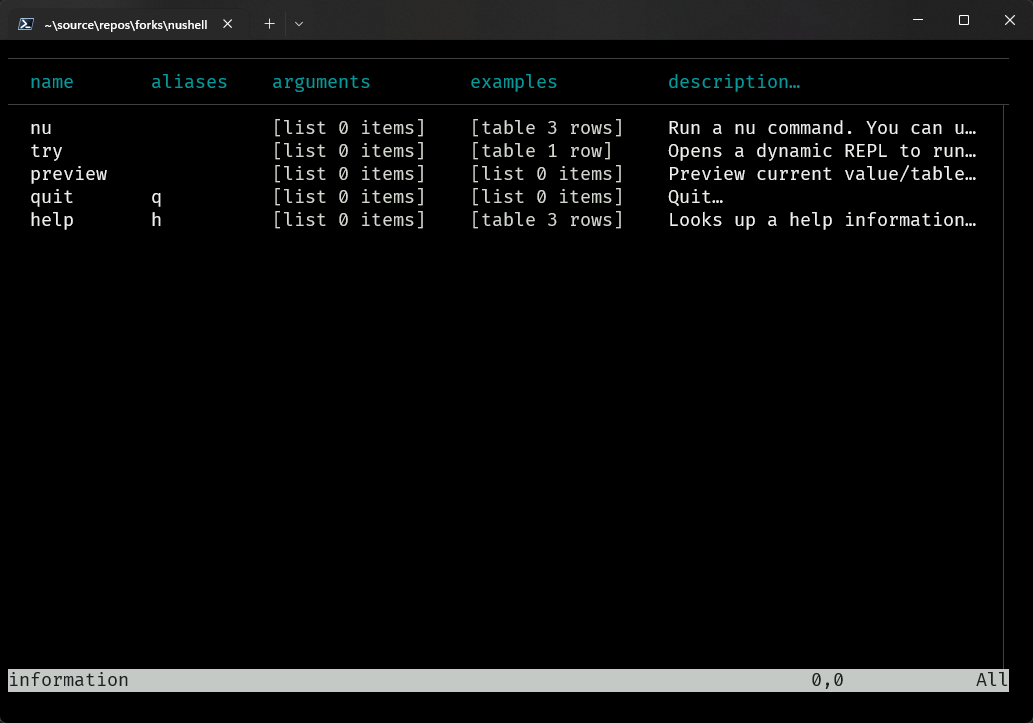
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
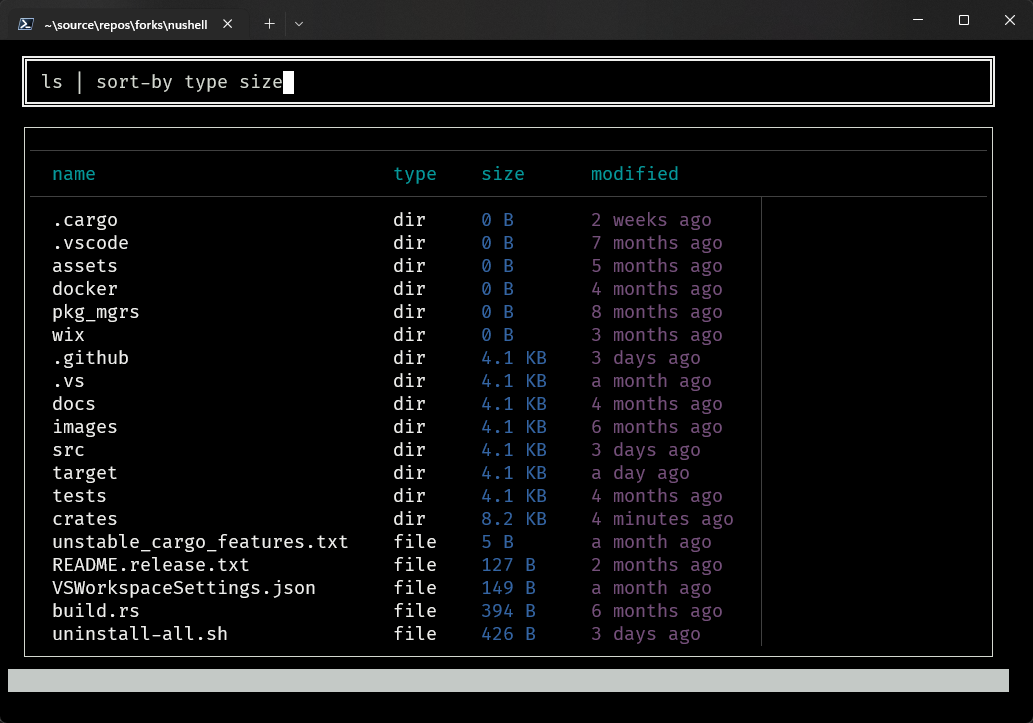
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
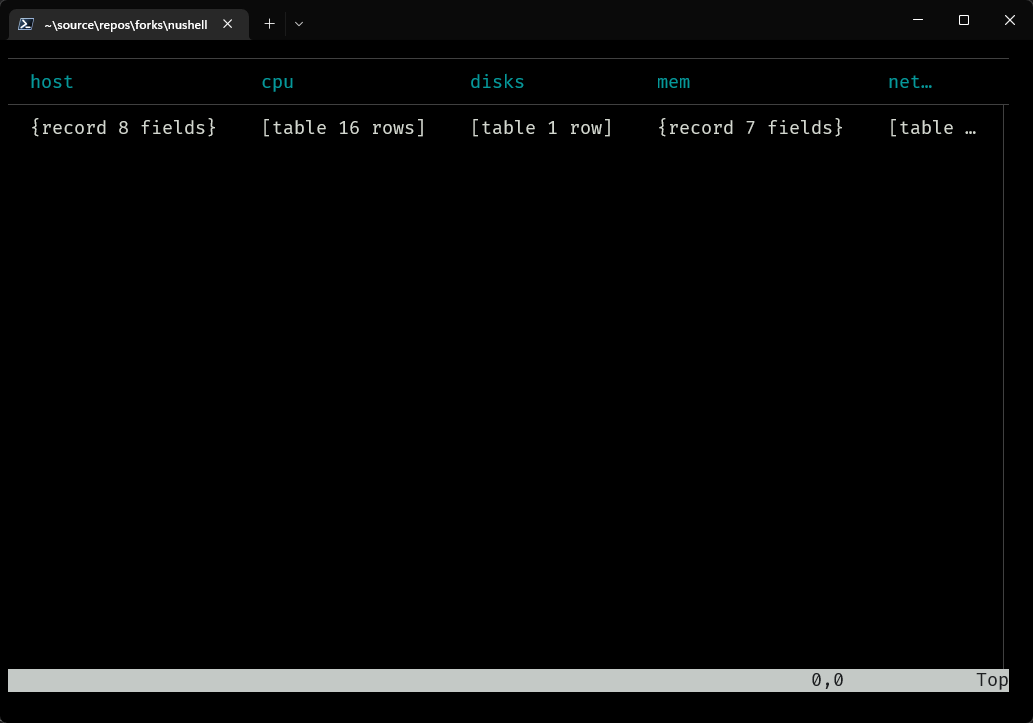
If you hit the `t` key it will now transpose the view to look like this.
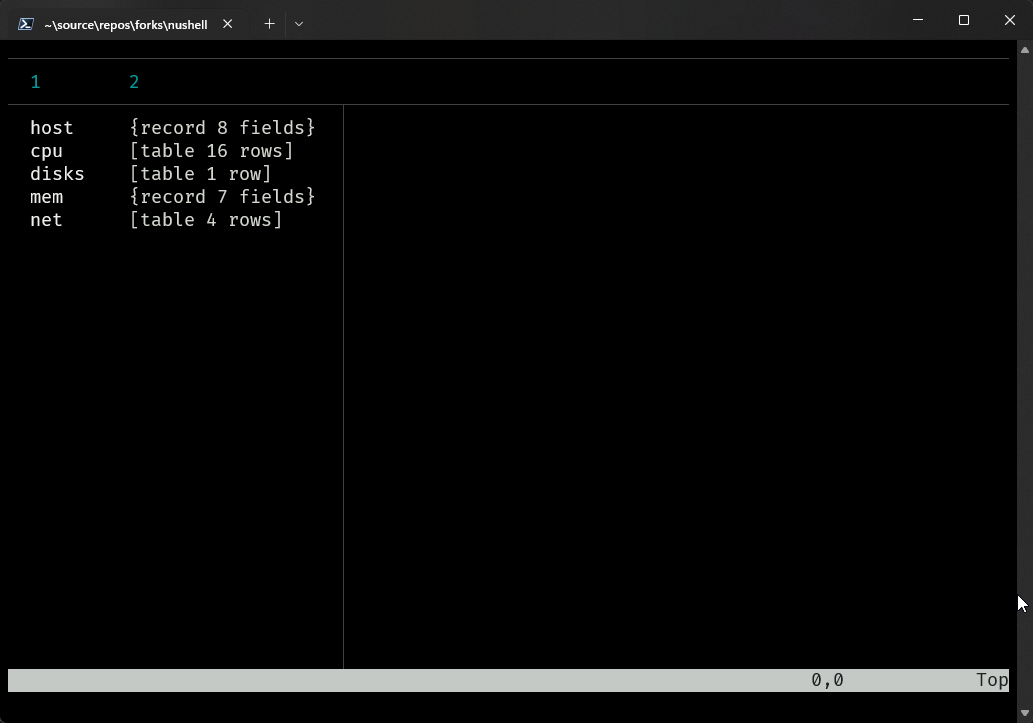
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
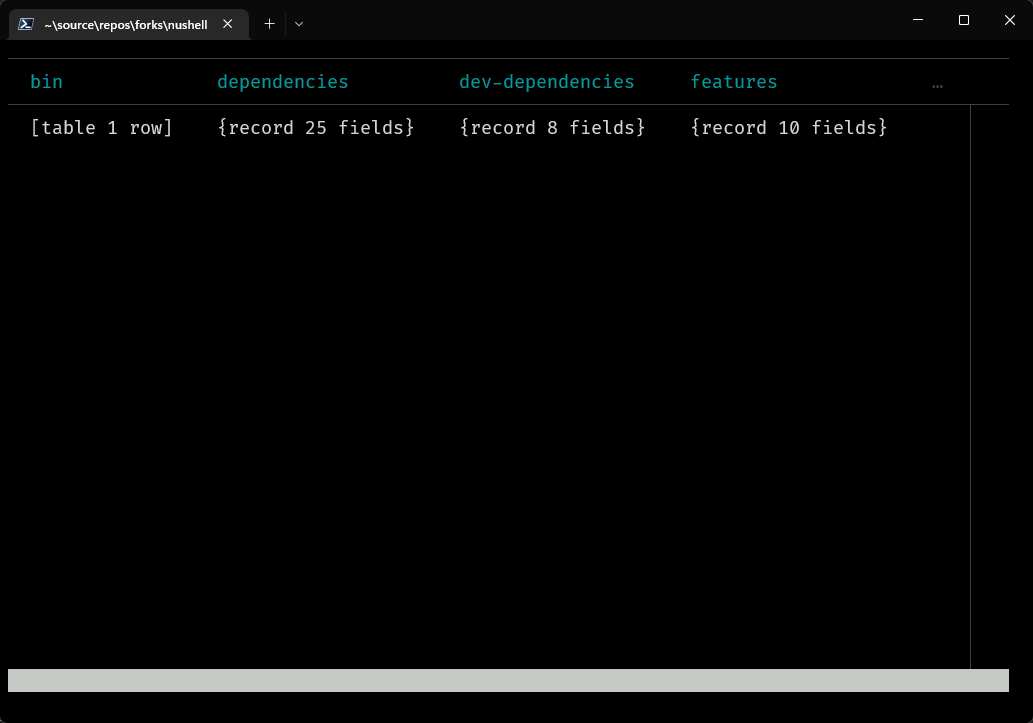
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
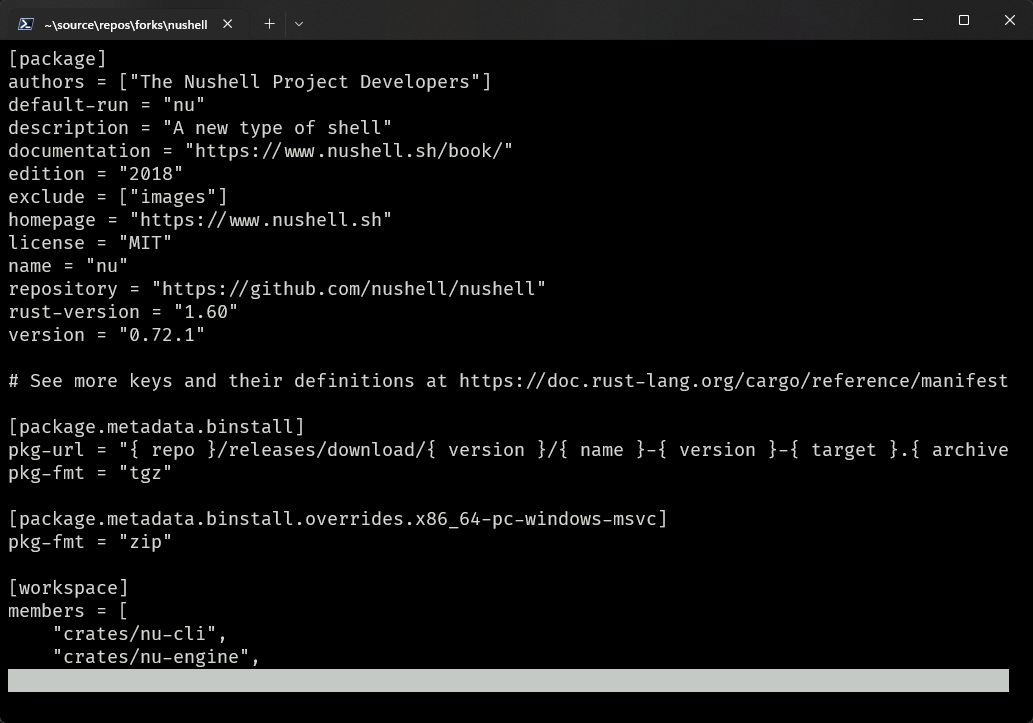
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
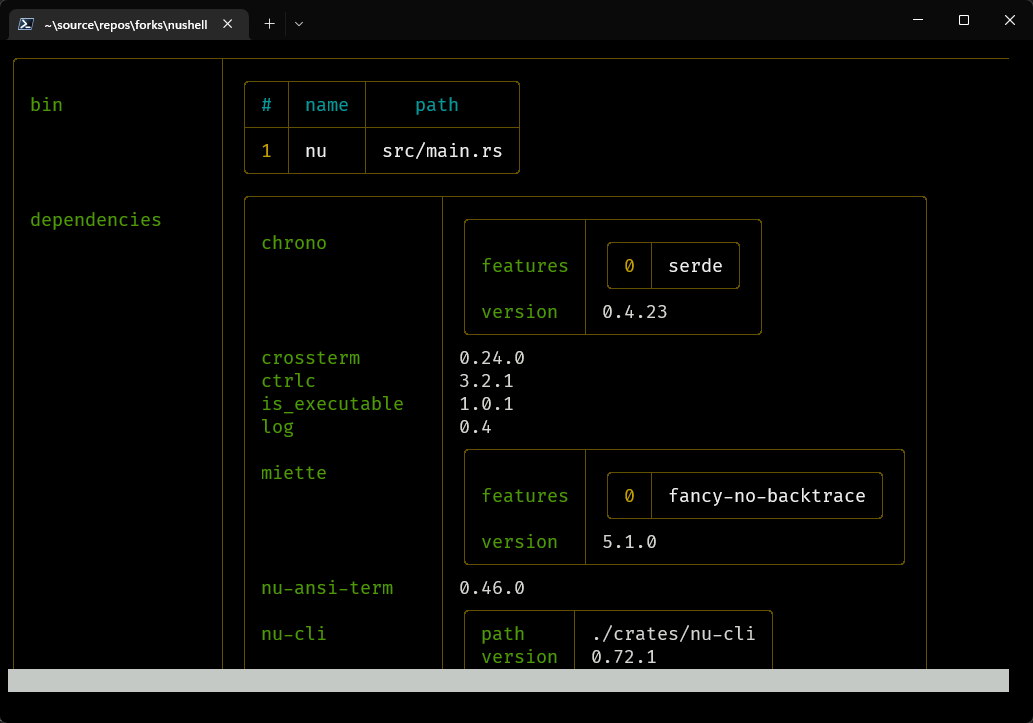
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
}
|
2022-12-16 15:47:07 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
fn parse_hash_map(value: &Value) -> Option<HashMap<String, Value>> {
|
Create `Record` type (#10103)
# Description
This PR creates a new `Record` type to reduce duplicate code and
possibly bugs as well. (This is an edited version of #9648.)
- `Record` implements `FromIterator` and `IntoIterator` and so can be
iterated over or collected into. For example, this helps with
conversions to and from (hash)maps. (Also, no more
`cols.iter().zip(vals)`!)
- `Record` has a `push(col, val)` function to help insure that the
number of columns is equal to the number of values. I caught a few
potential bugs thanks to this (e.g. in the `ls` command).
- Finally, this PR also adds a `record!` macro that helps simplify
record creation. It is used like so:
```rust
record! {
"key1" => some_value,
"key2" => Value::string("text", span),
"key3" => Value::int(optional_int.unwrap_or(0), span),
"key4" => Value::bool(config.setting, span),
}
```
Since macros hinder formatting, etc., the right hand side values should
be relatively short and sweet like the examples above.
Where possible, prefer `record!` or `.collect()` on an iterator instead
of multiple `Record::push`s, since the first two automatically set the
record capacity and do less work overall.
# User-Facing Changes
Besides the changes in `nu-protocol` the only other breaking changes are
to `nu-table::{ExpandedTable::build_map, JustTable::kv_table}`.
2023-08-24 19:50:29 +00:00
|
|
|
value.as_record().ok().map(|val| {
|
|
|
|
val.iter()
|
2022-12-19 17:34:21 +00:00
|
|
|
.map(|(col, val)| (col.clone(), val.clone()))
|
|
|
|
.collect::<HashMap<_, _>>()
|
|
|
|
})
|
2022-12-16 15:47:07 +00:00
|
|
|
}
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
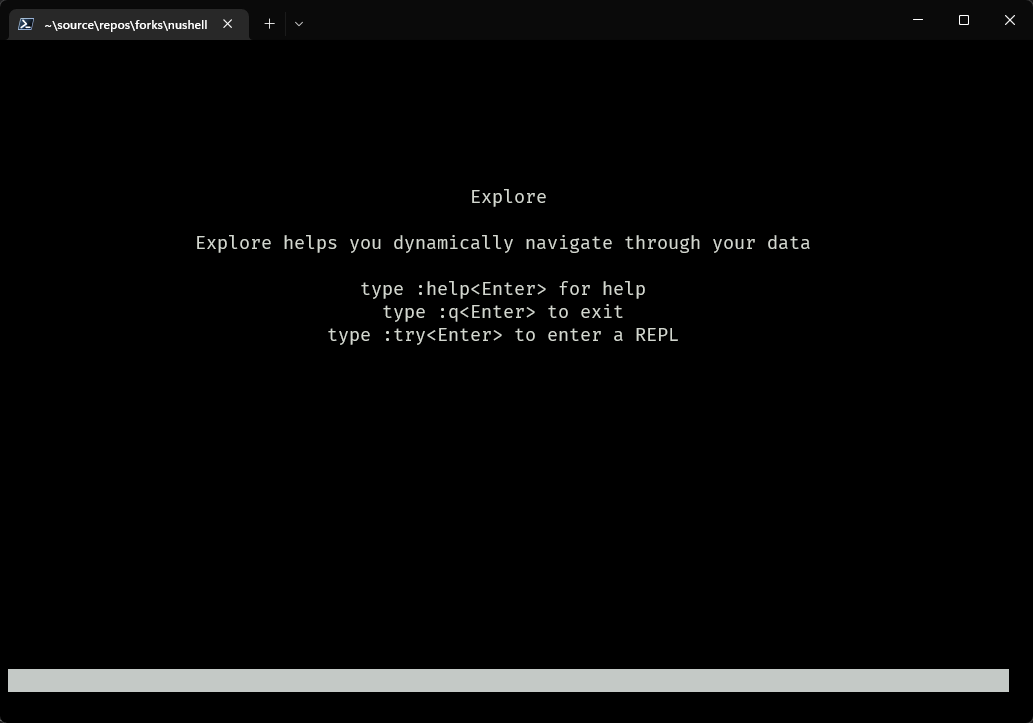
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
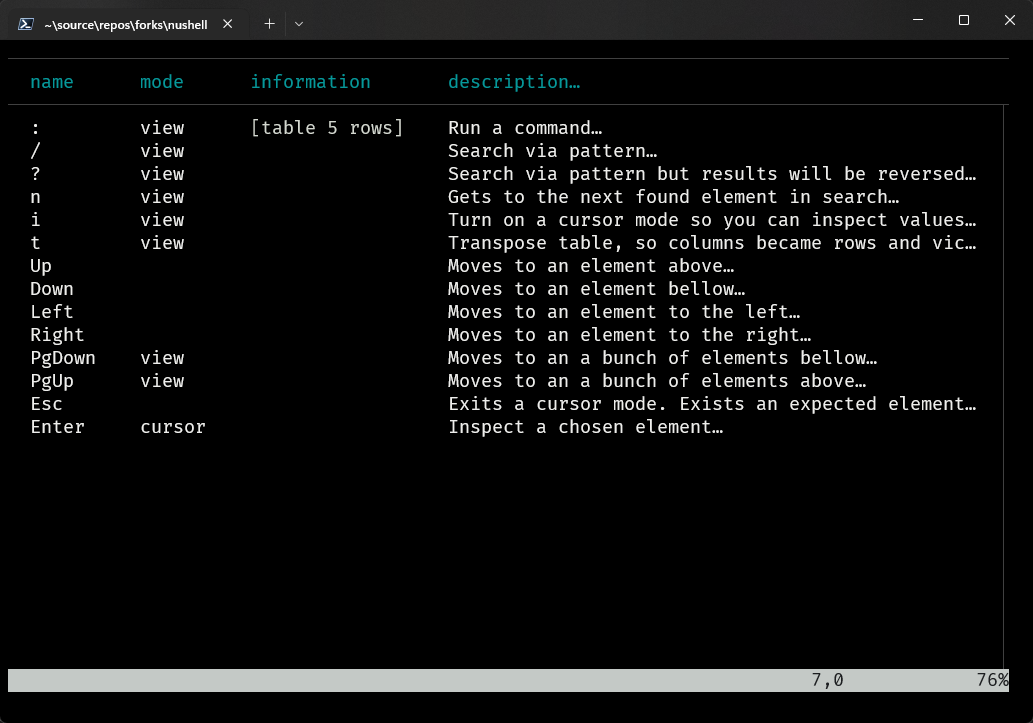
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
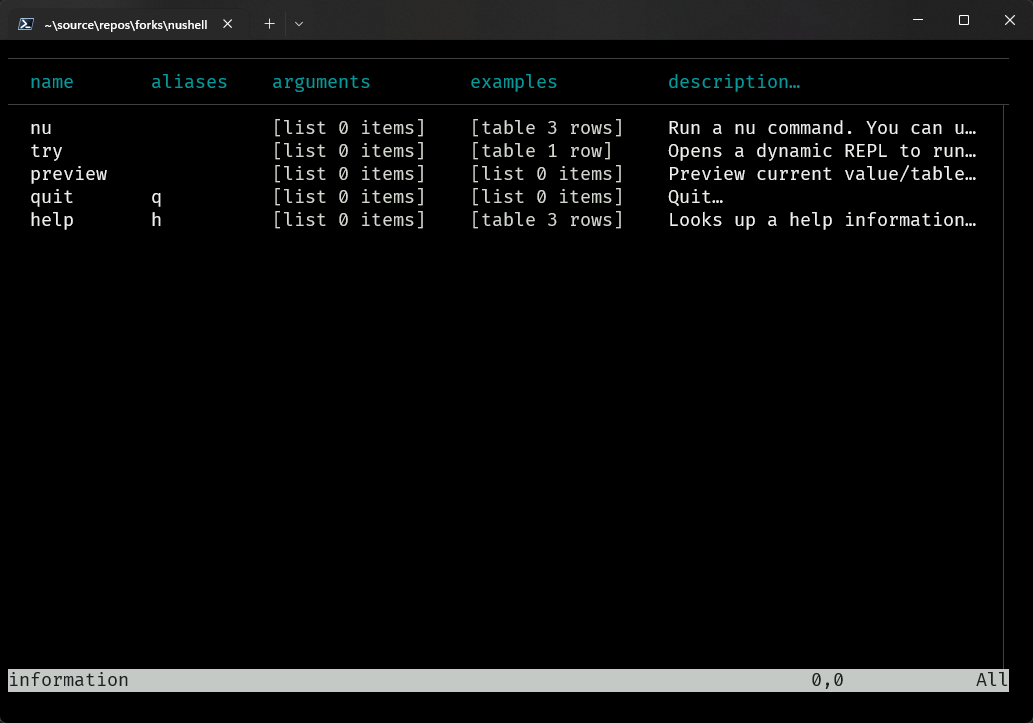
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
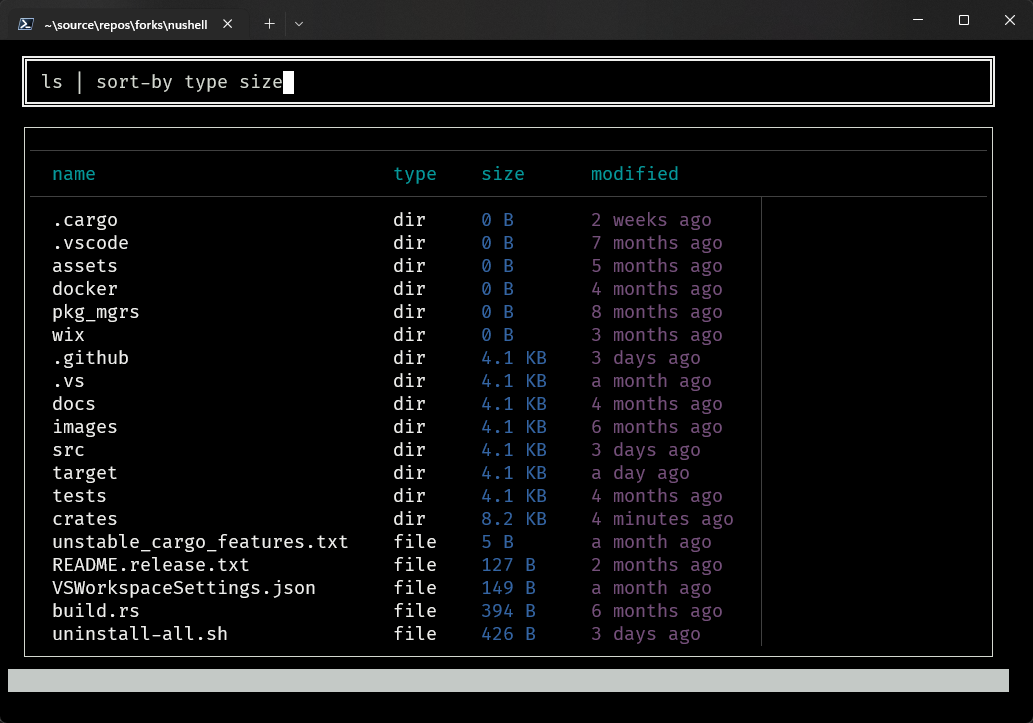
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
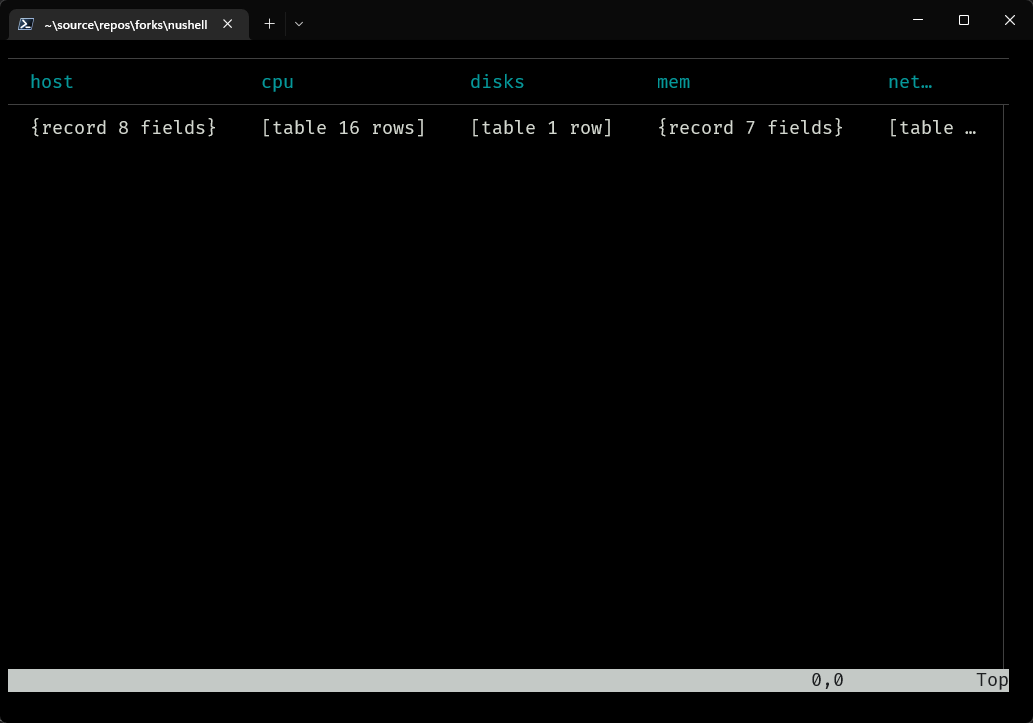
If you hit the `t` key it will now transpose the view to look like this.
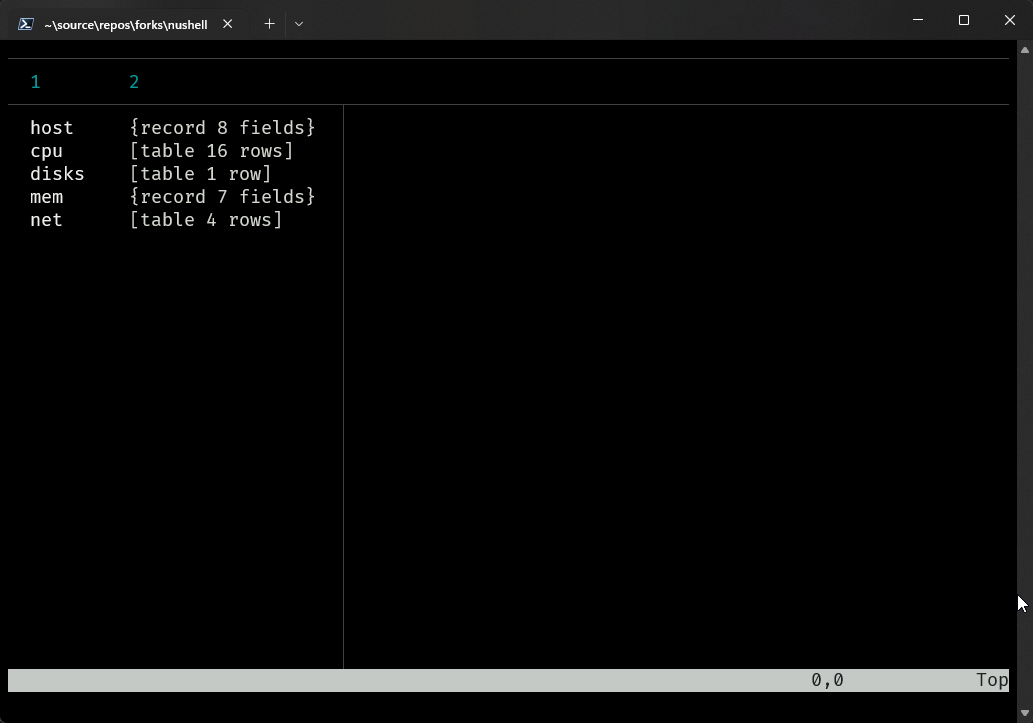
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
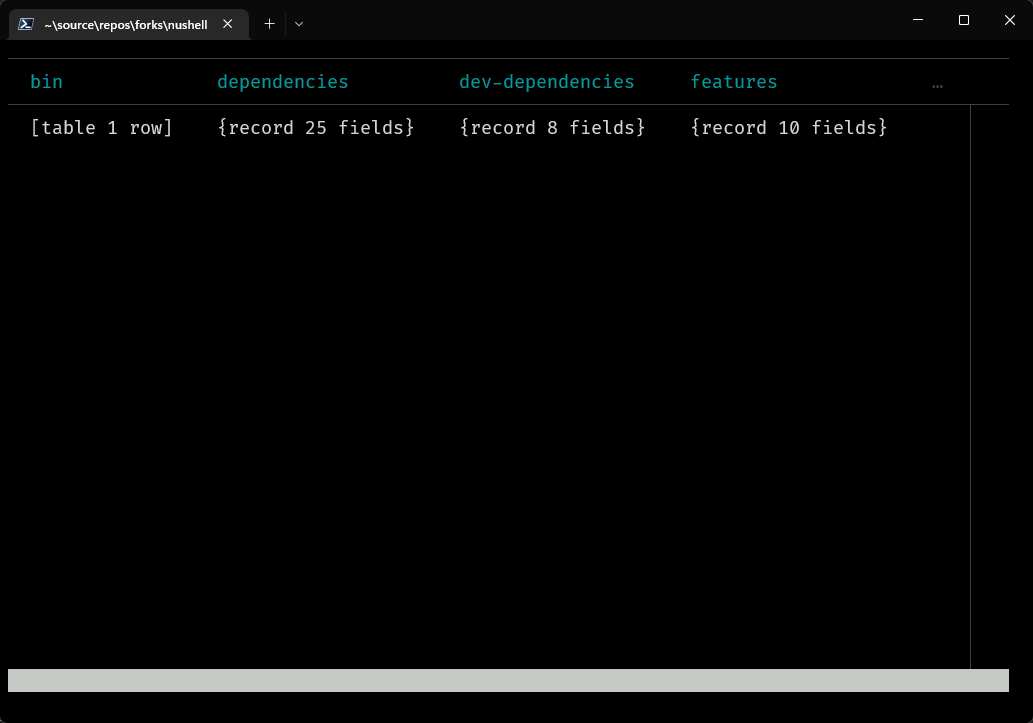
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
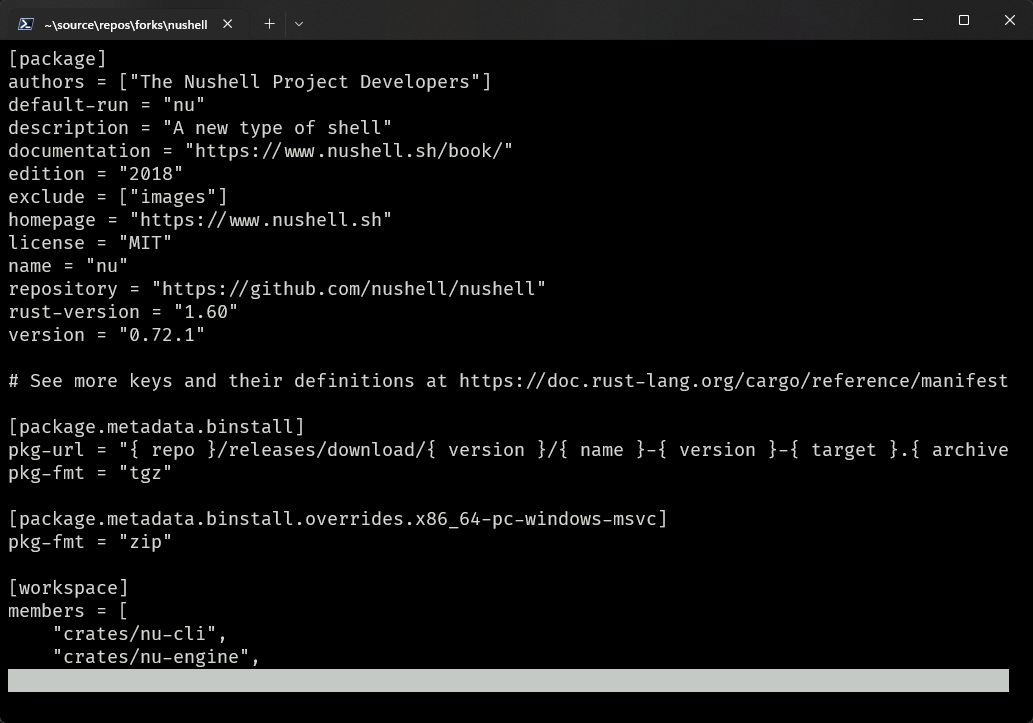
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
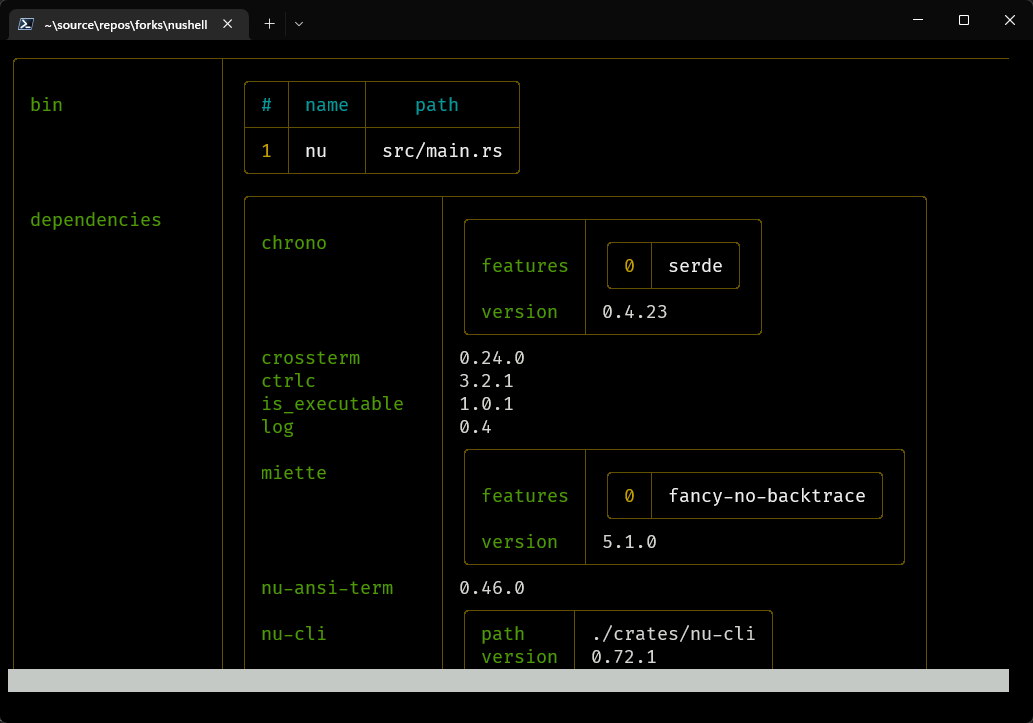
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
|
2022-12-16 15:47:07 +00:00
|
|
|
const fn color(foreground: Option<Color>, background: Option<Color>) -> Style {
|
|
|
|
Style {
|
|
|
|
background,
|
|
|
|
foreground,
|
|
|
|
is_blink: false,
|
|
|
|
is_bold: false,
|
|
|
|
is_dimmed: false,
|
|
|
|
is_hidden: false,
|
|
|
|
is_italic: false,
|
|
|
|
is_reverse: false,
|
|
|
|
is_strikethrough: false,
|
|
|
|
is_underline: false,
|
2023-07-24 11:16:18 +00:00
|
|
|
prefix_with_reset: false,
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
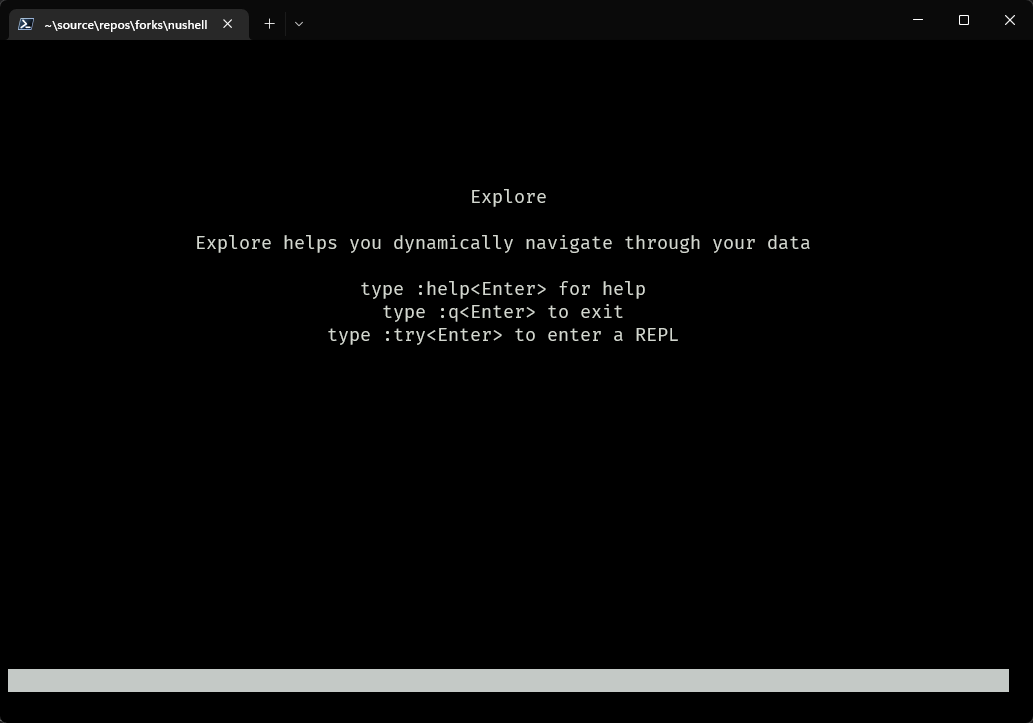
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
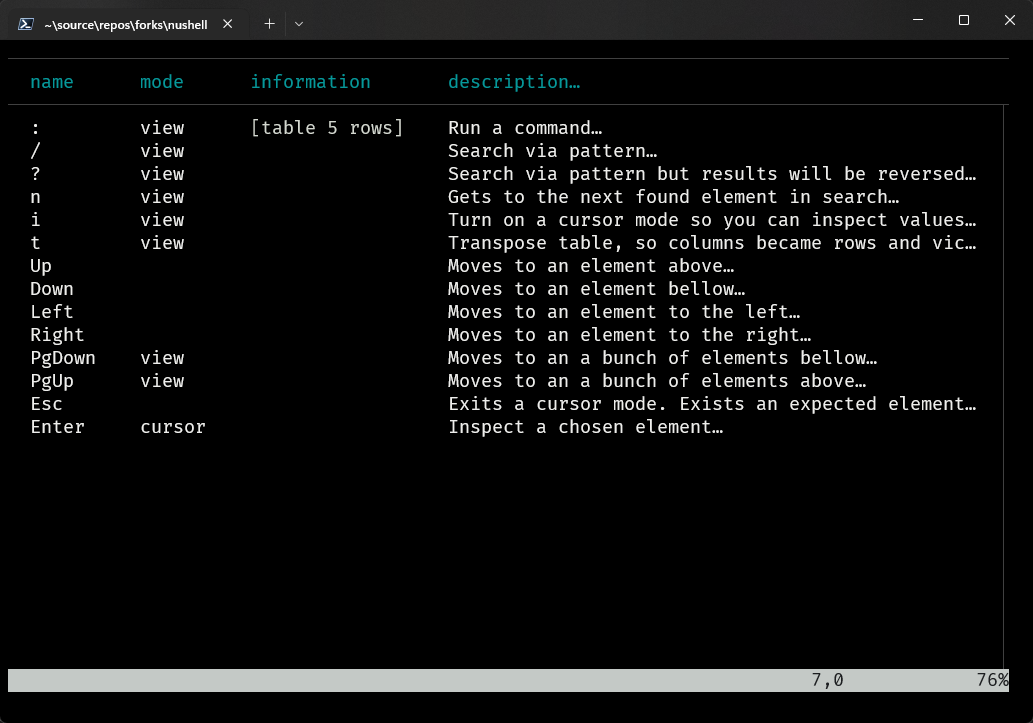
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
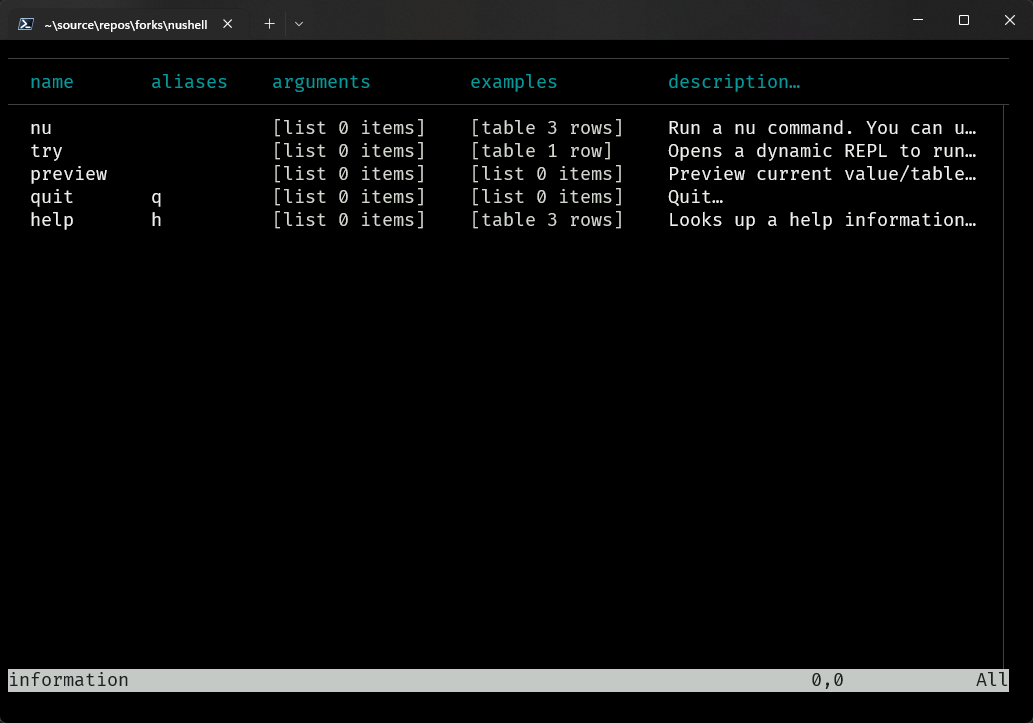
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
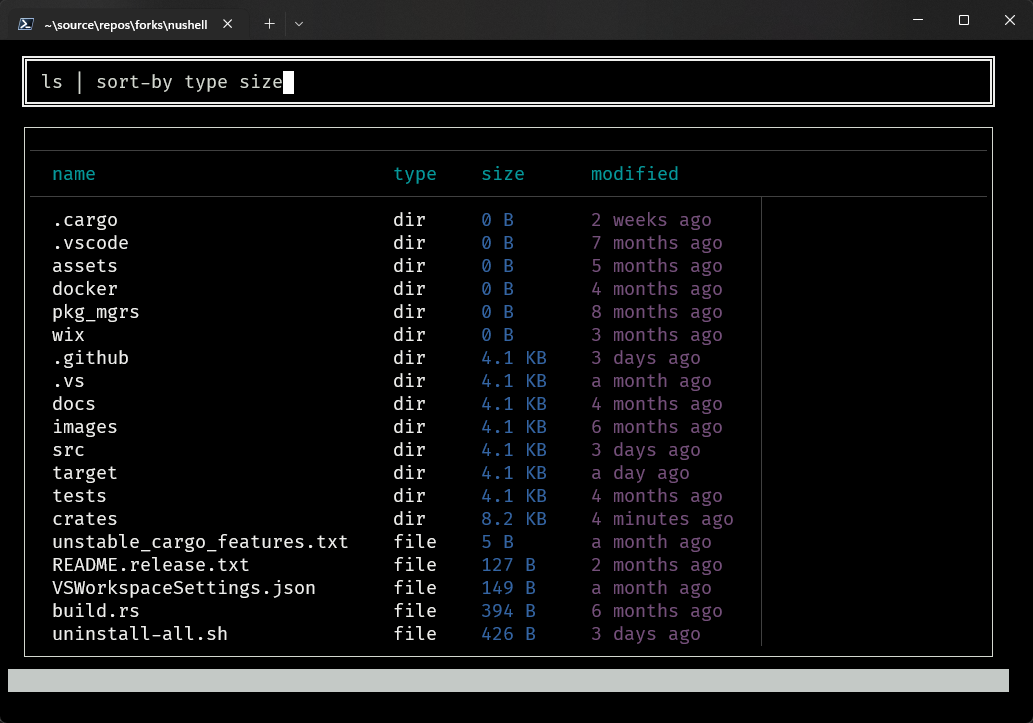
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
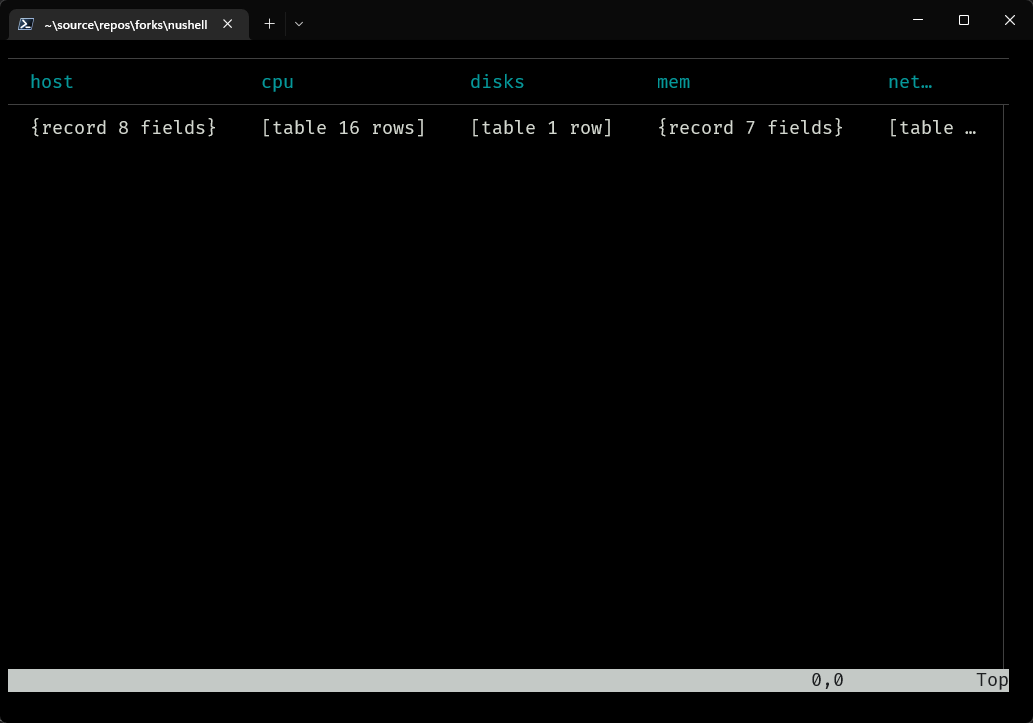
If you hit the `t` key it will now transpose the view to look like this.
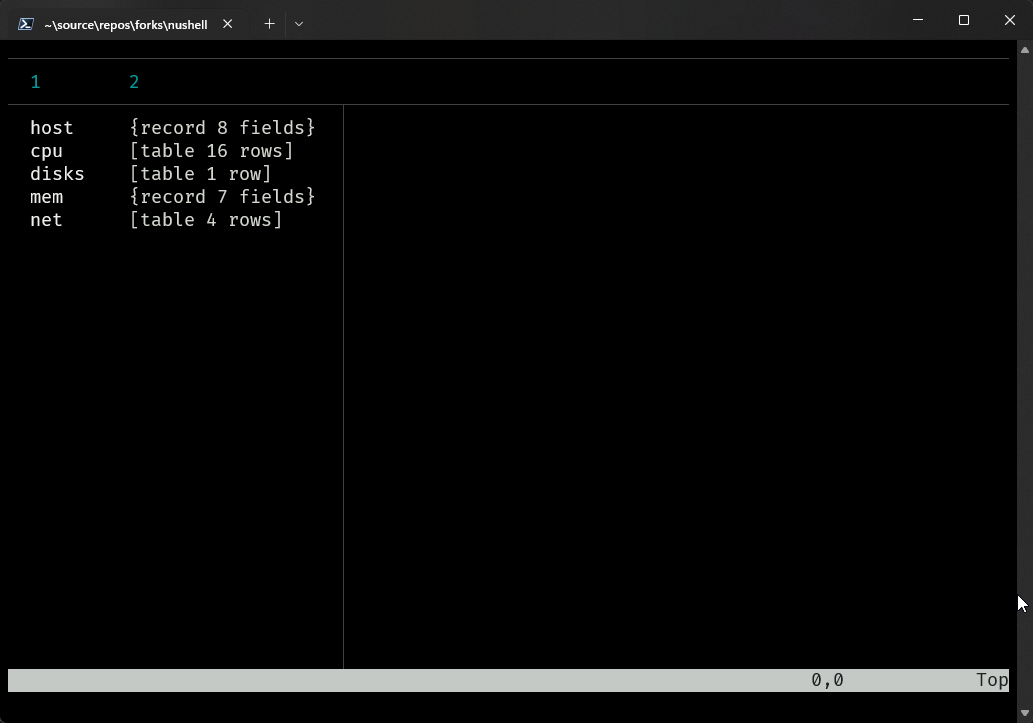
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
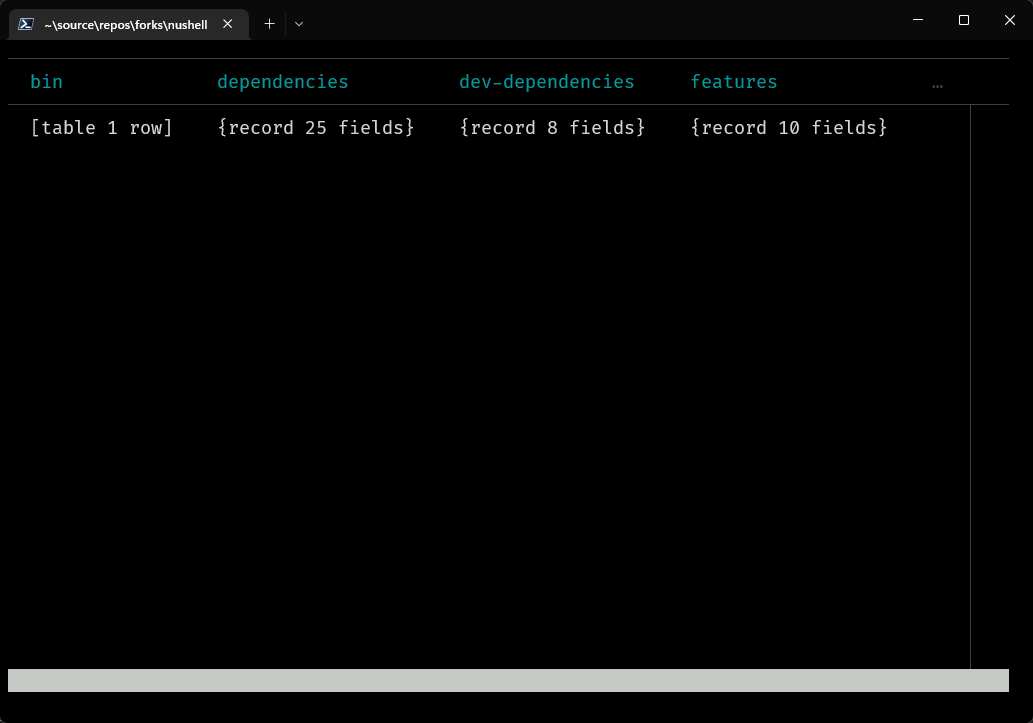
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
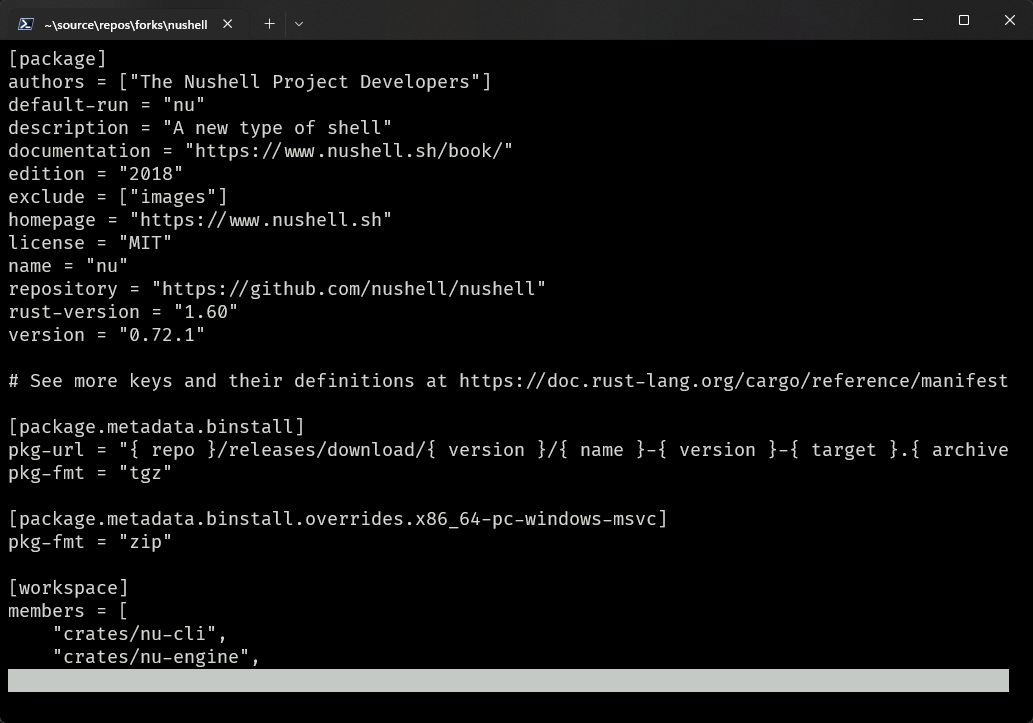
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
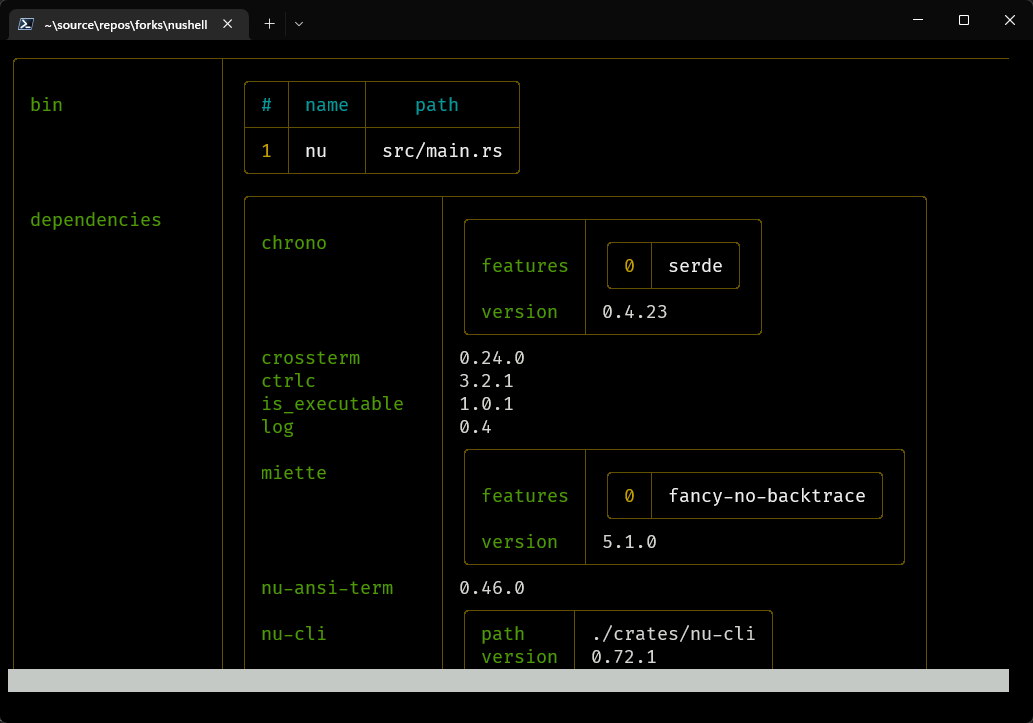
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
}
|
2022-12-16 15:47:07 +00:00
|
|
|
}
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
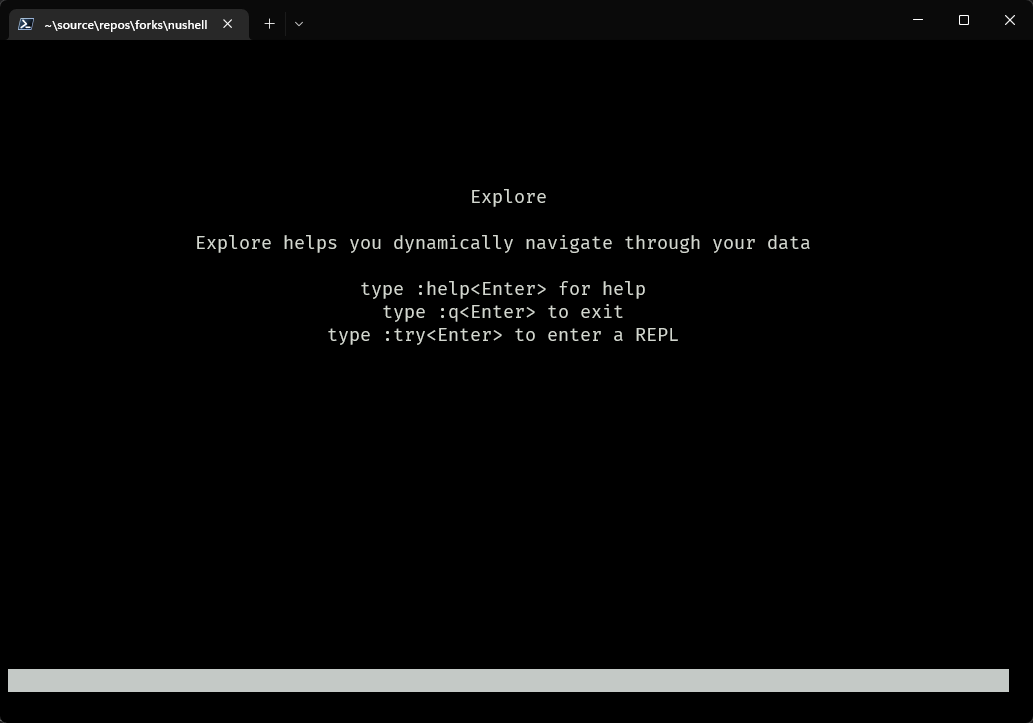
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
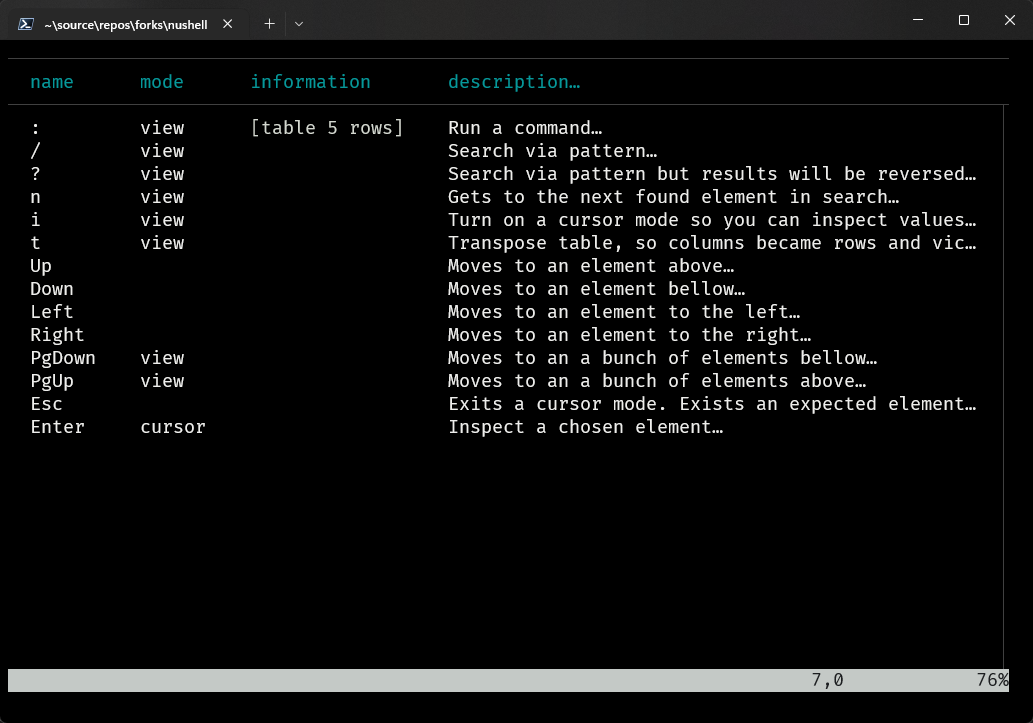
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
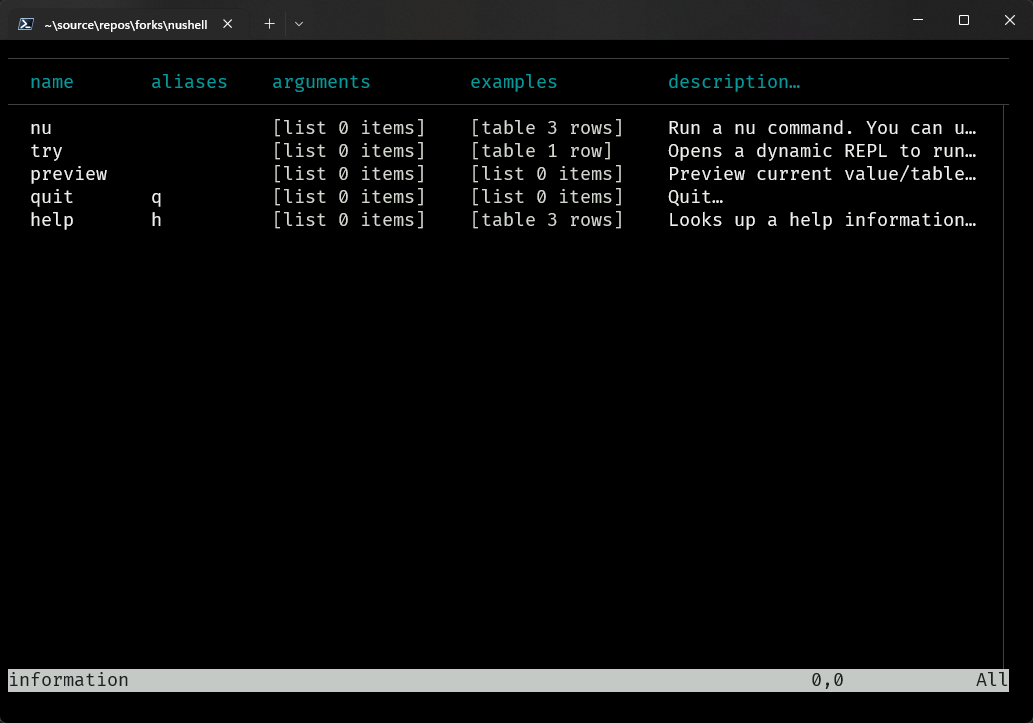
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
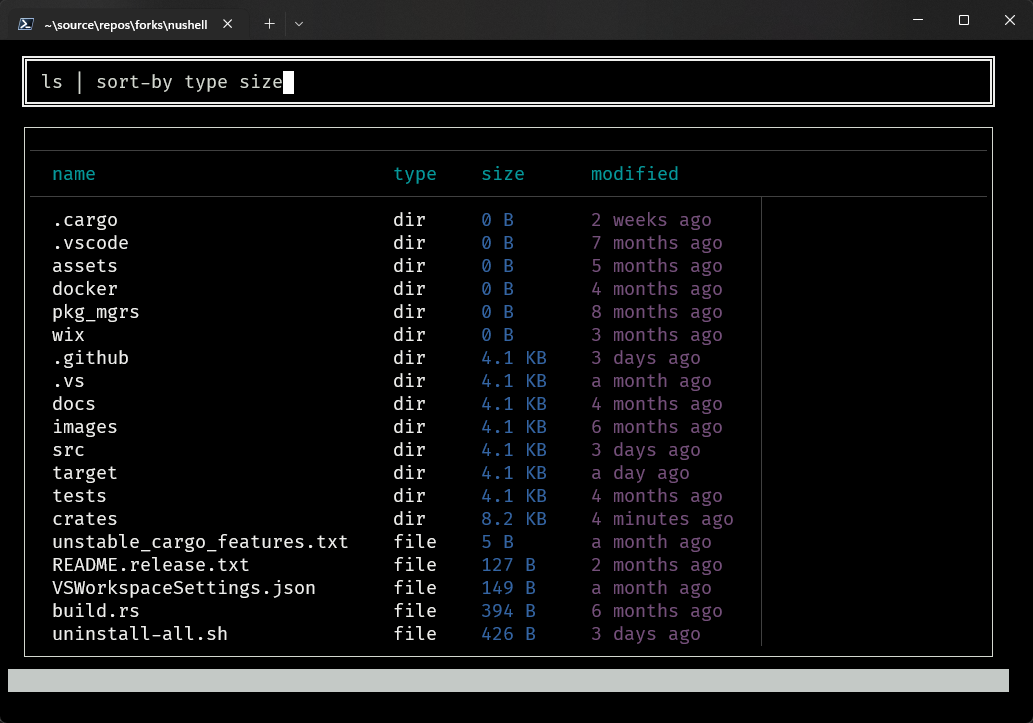
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
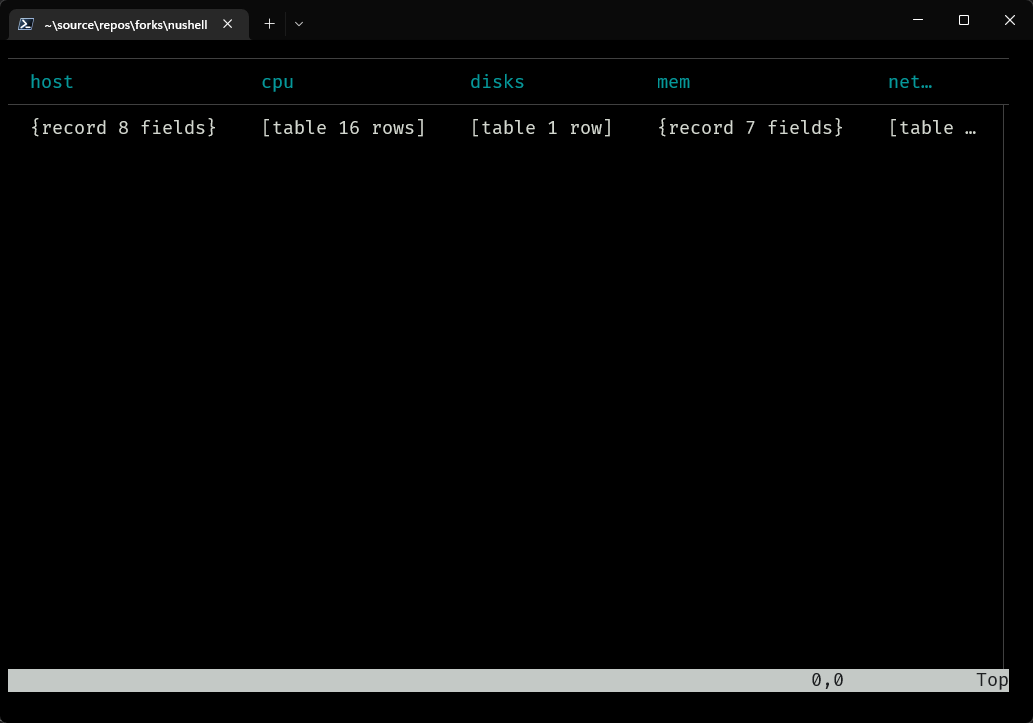
If you hit the `t` key it will now transpose the view to look like this.
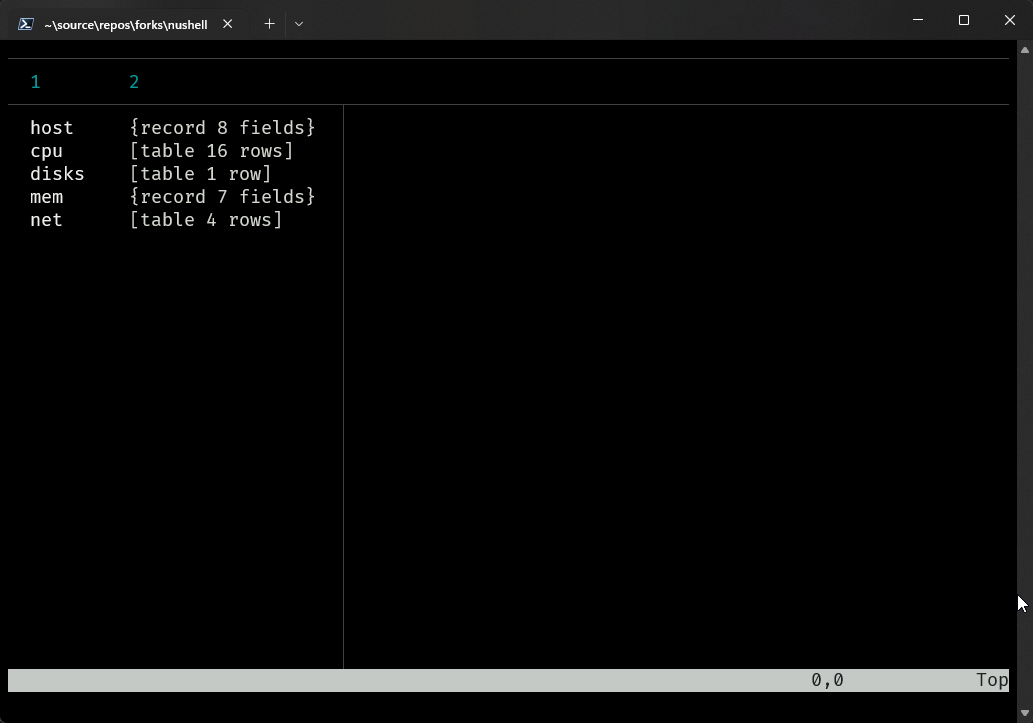
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
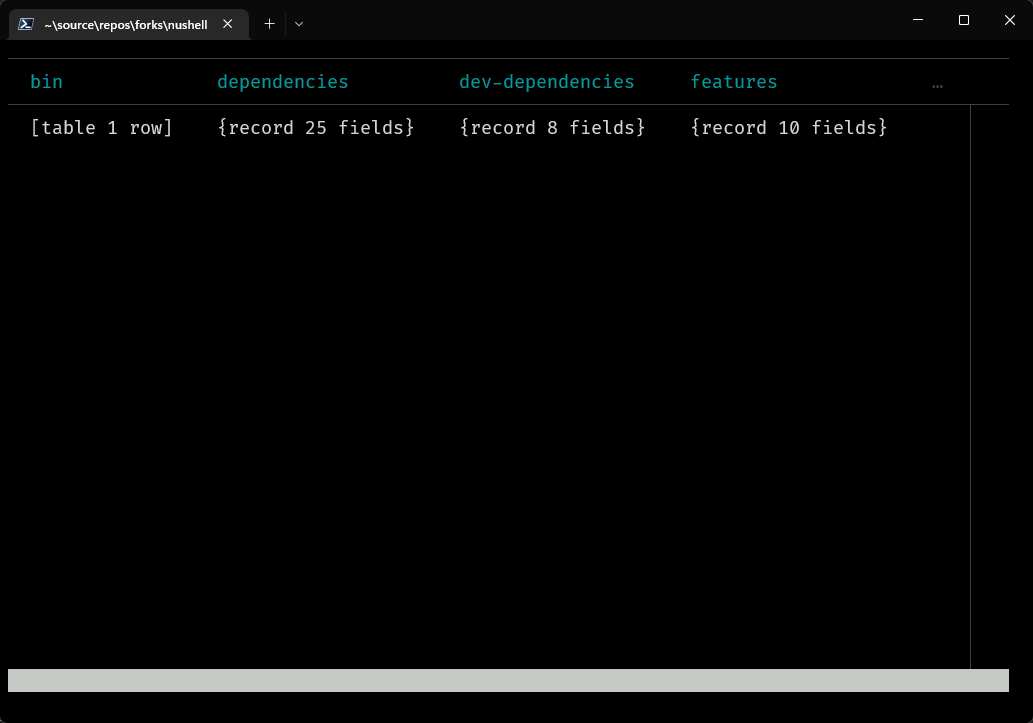
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
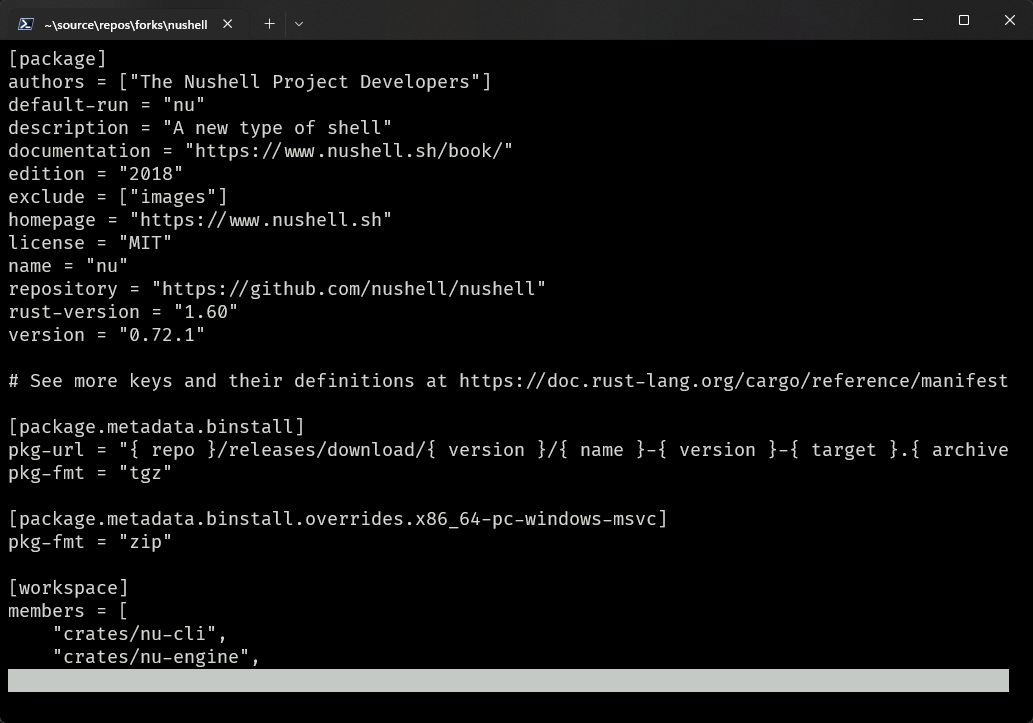
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
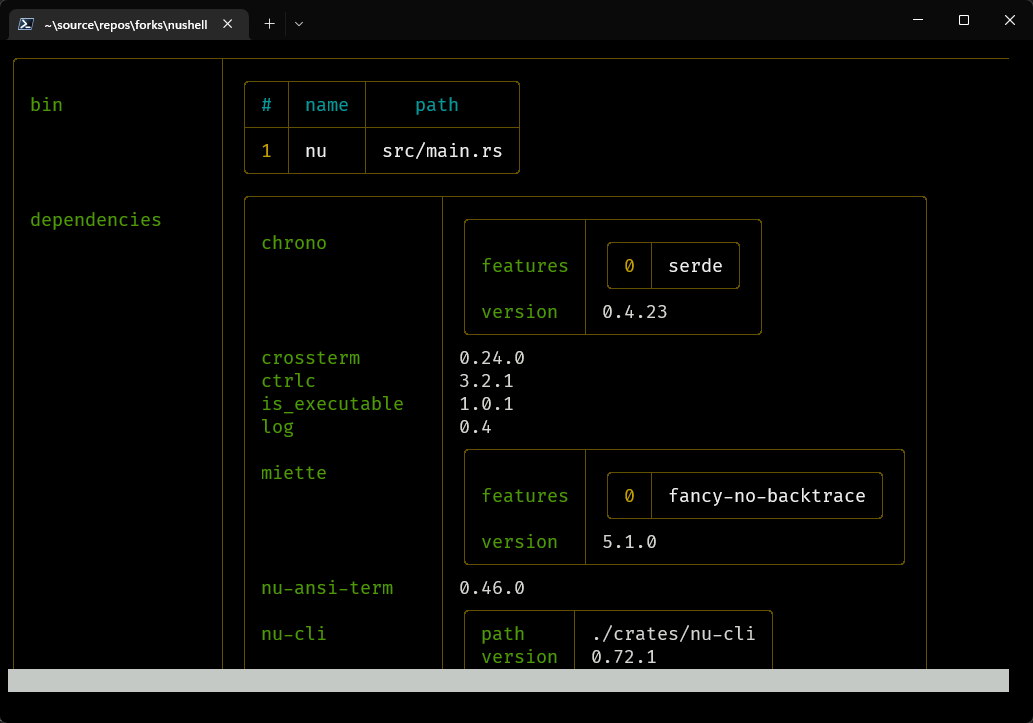
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
|
2022-12-16 15:47:07 +00:00
|
|
|
fn insert_style(map: &mut HashMap<String, Value>, key: &str, value: Style) {
|
|
|
|
if map.contains_key(key) {
|
|
|
|
return;
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
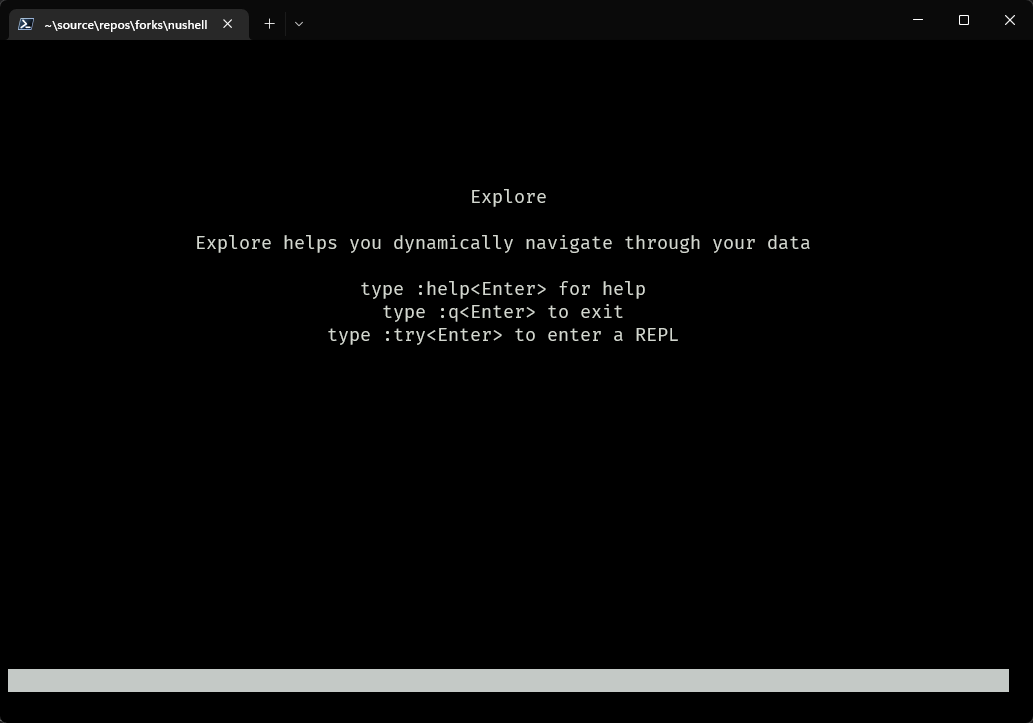
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
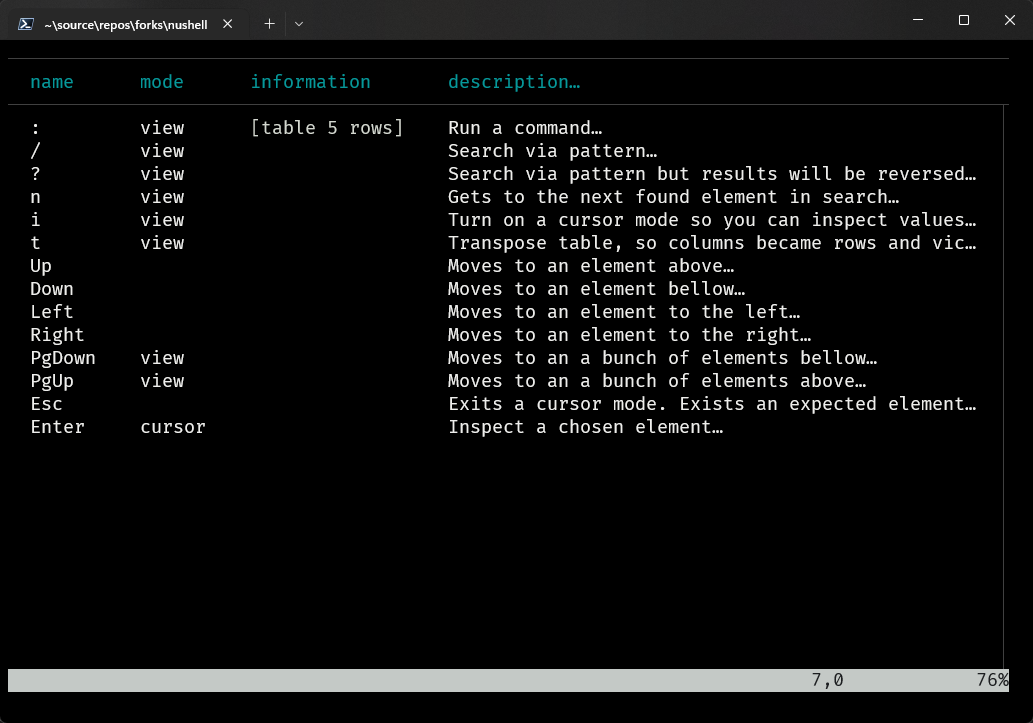
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
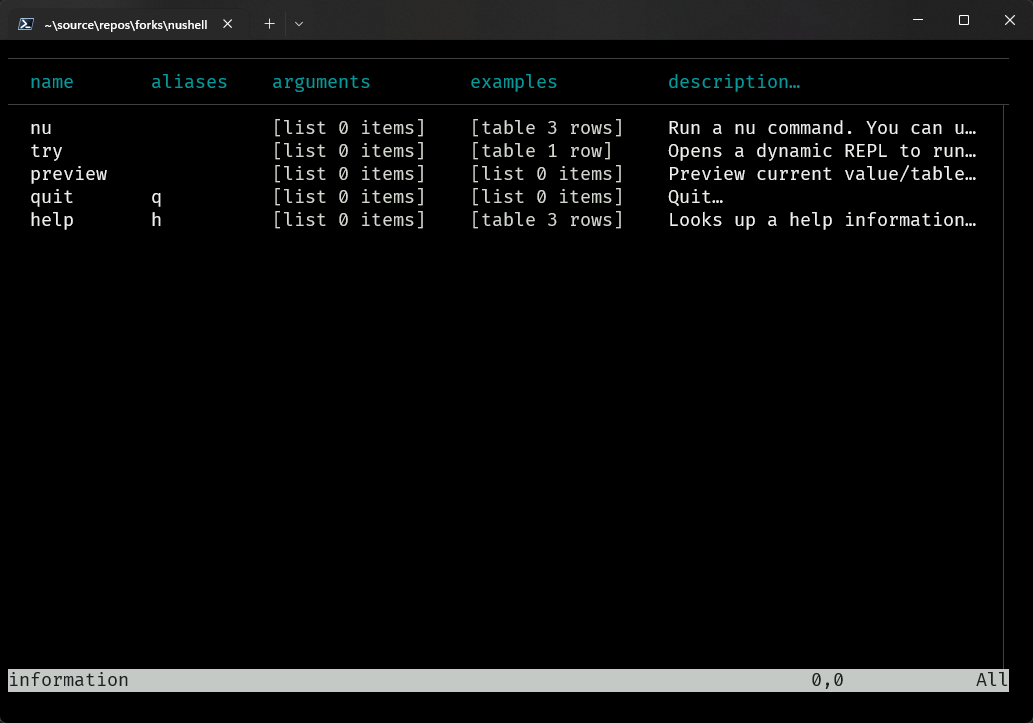
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
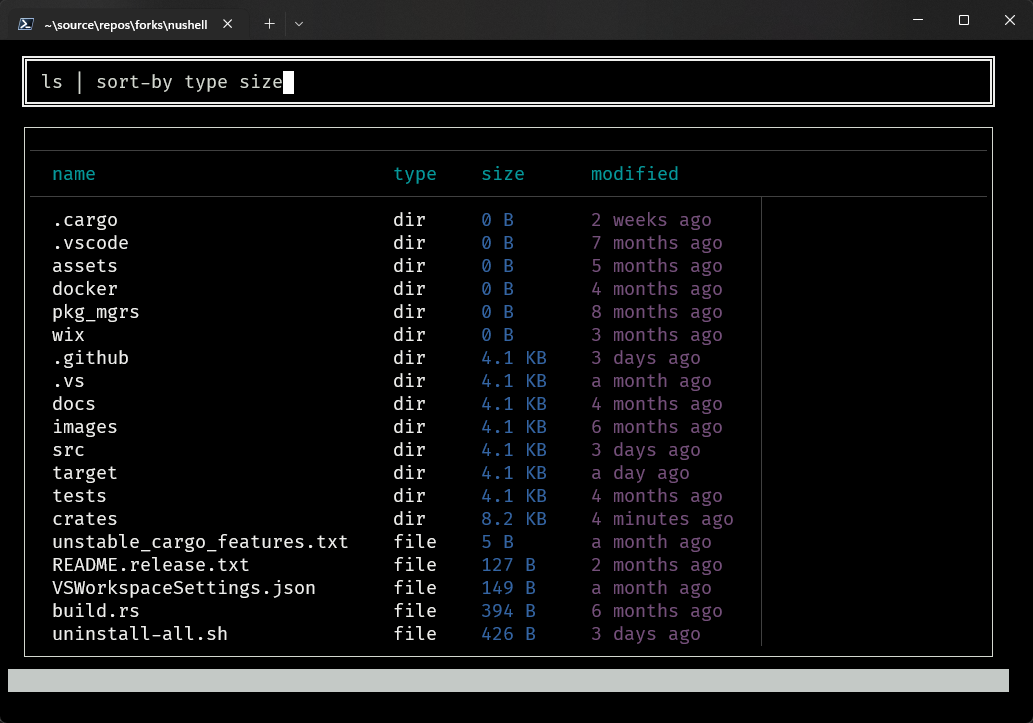
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
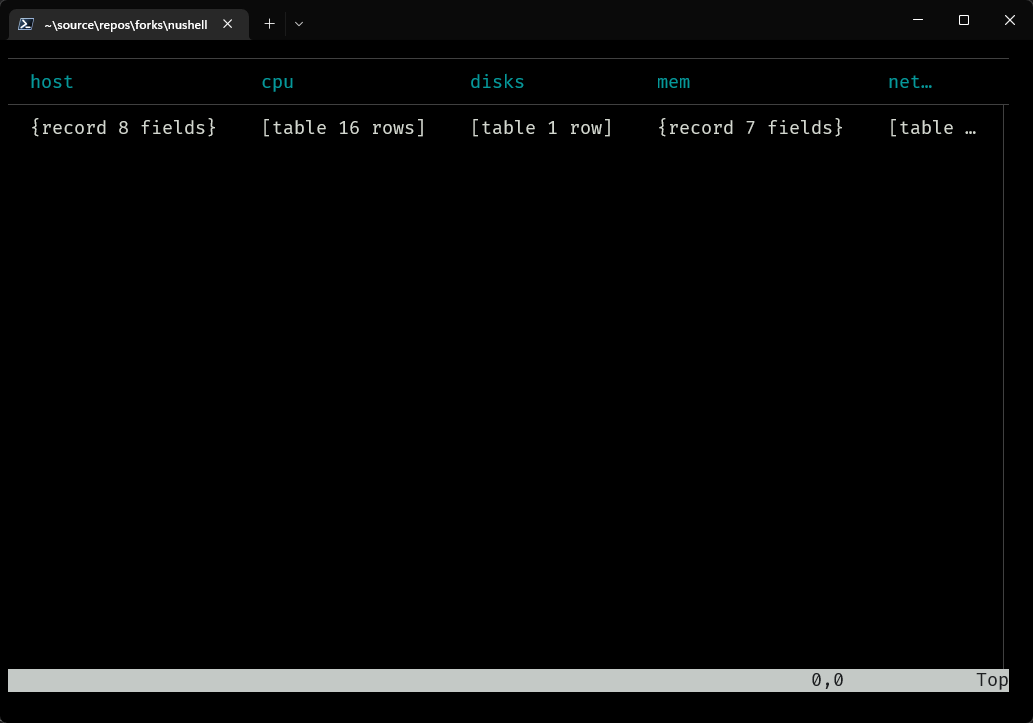
If you hit the `t` key it will now transpose the view to look like this.
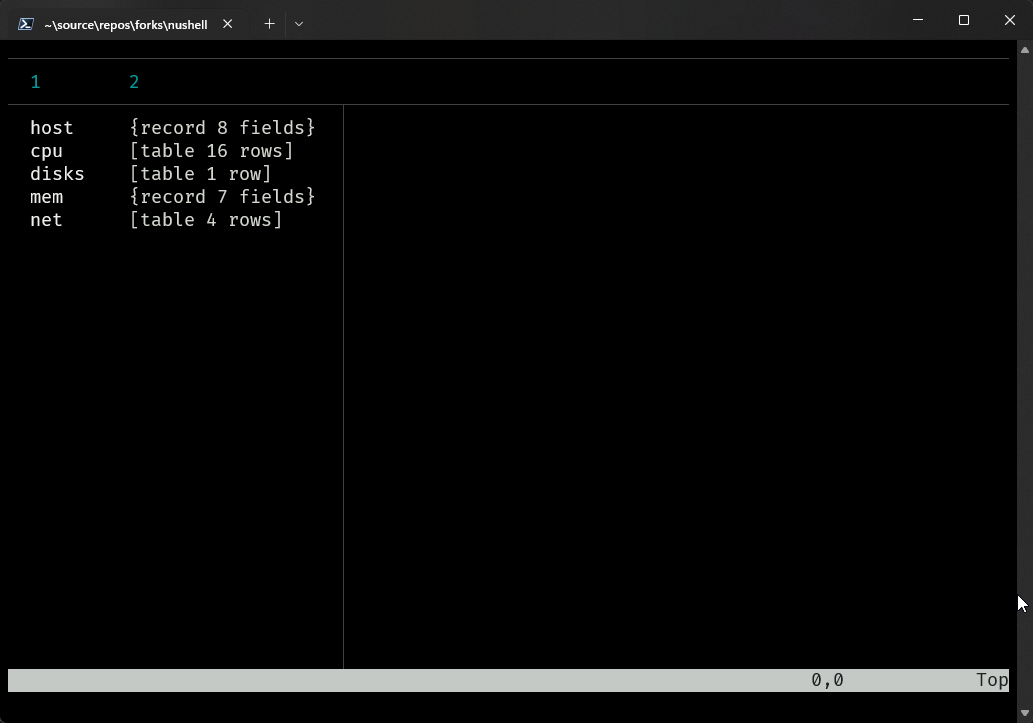
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
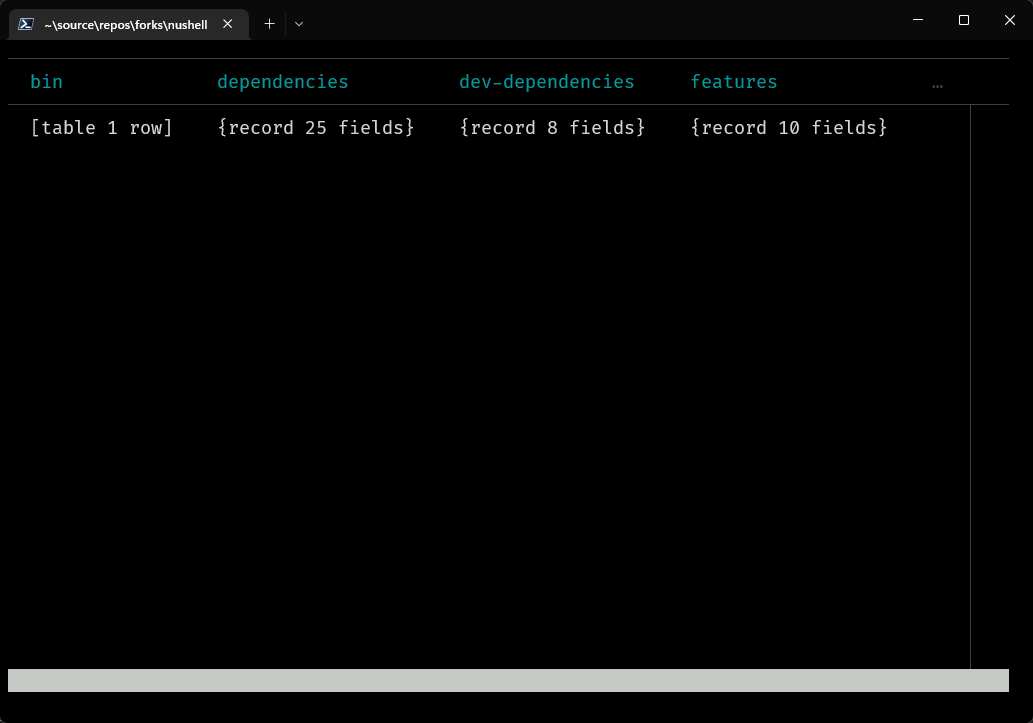
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
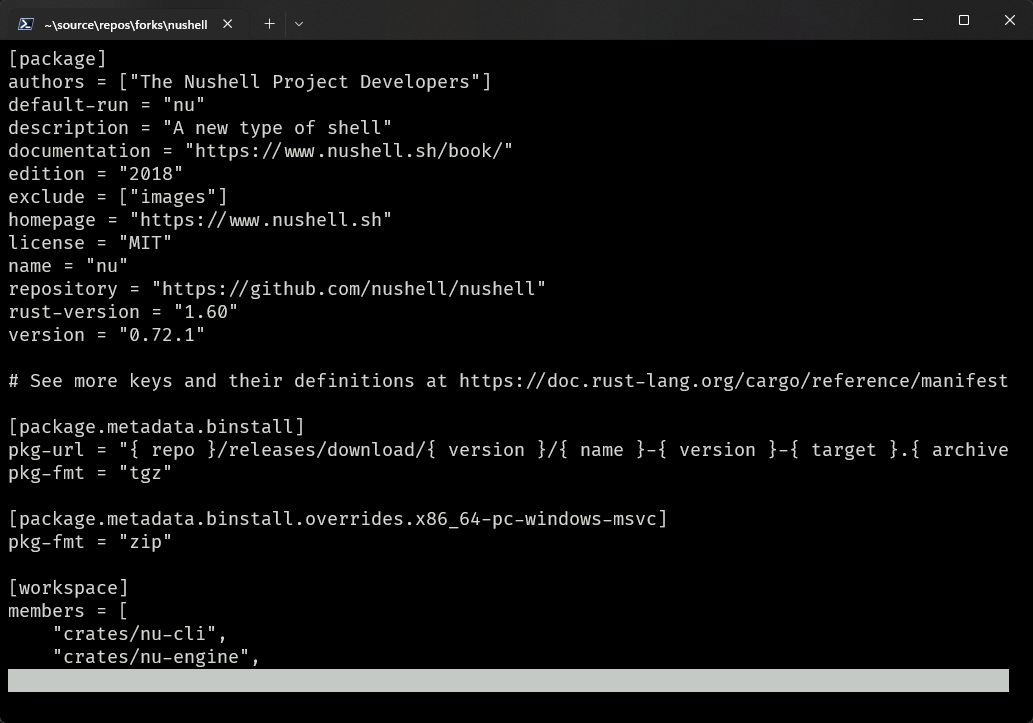
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
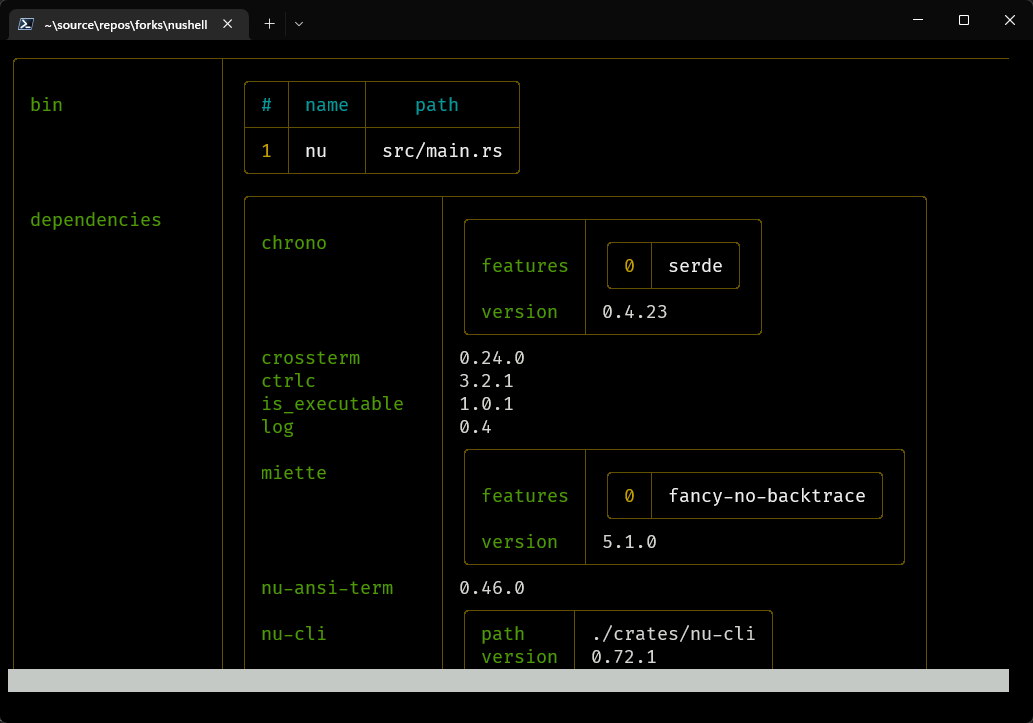
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
}
|
|
|
|
|
2022-12-16 15:47:07 +00:00
|
|
|
if value == Style::default() {
|
|
|
|
return;
|
|
|
|
}
|
|
|
|
|
|
|
|
let value = nu_color_config::NuStyle::from(value);
|
2022-12-18 14:43:15 +00:00
|
|
|
if let Ok(val) = nu_json::to_string_raw(&value) {
|
2022-12-16 15:47:07 +00:00
|
|
|
map.insert(String::from(key), Value::string(val, Span::unknown()));
|
|
|
|
}
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
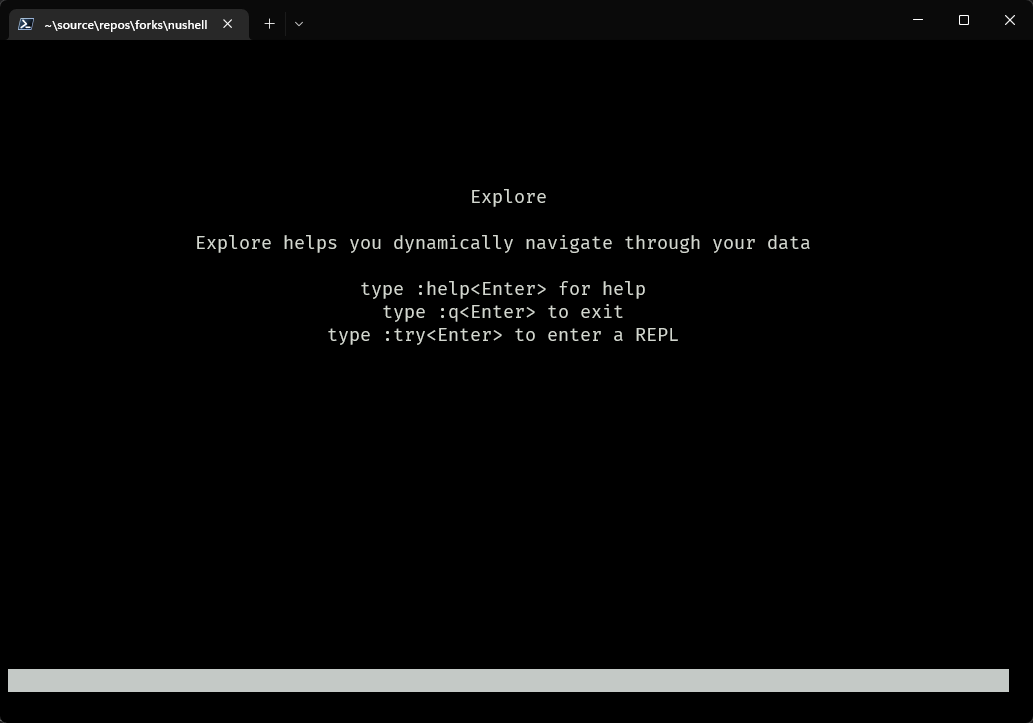
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
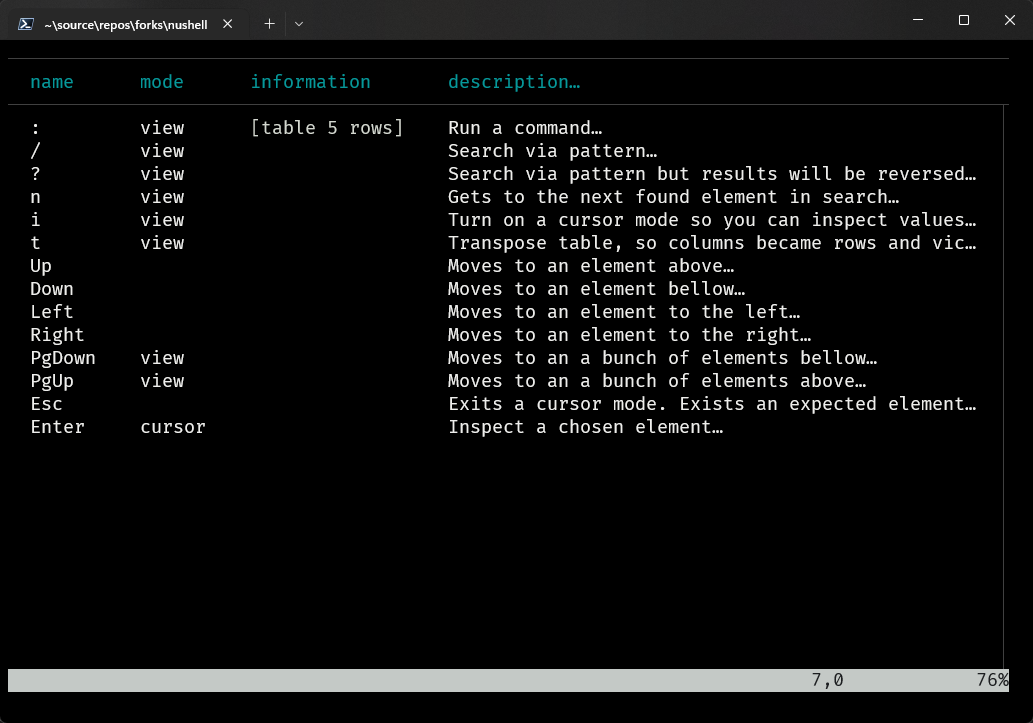
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
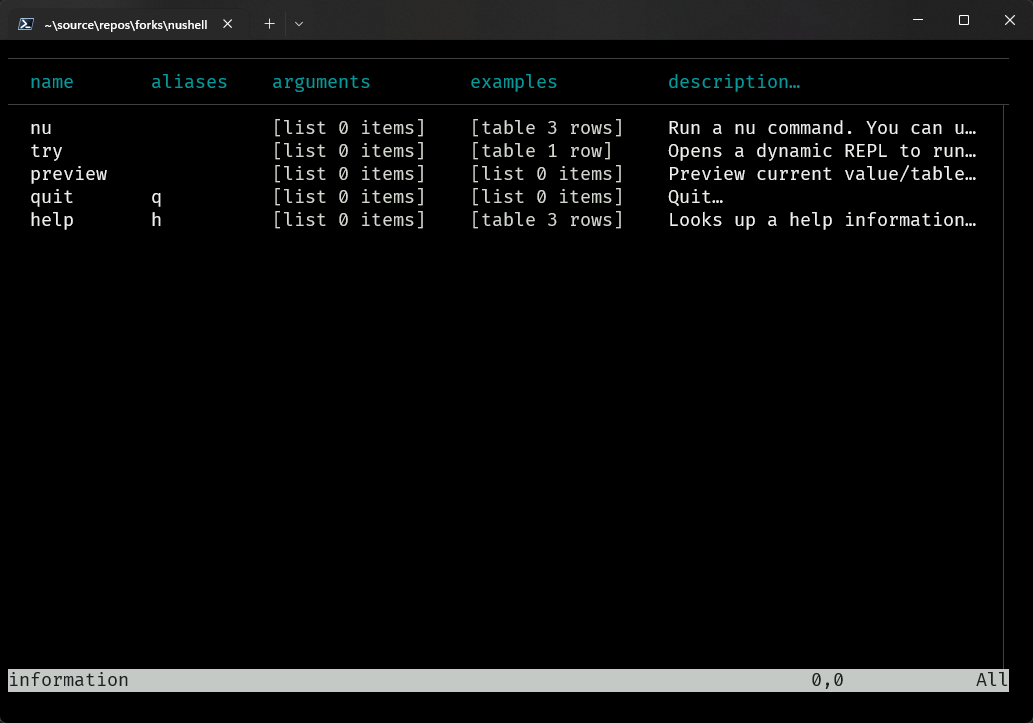
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
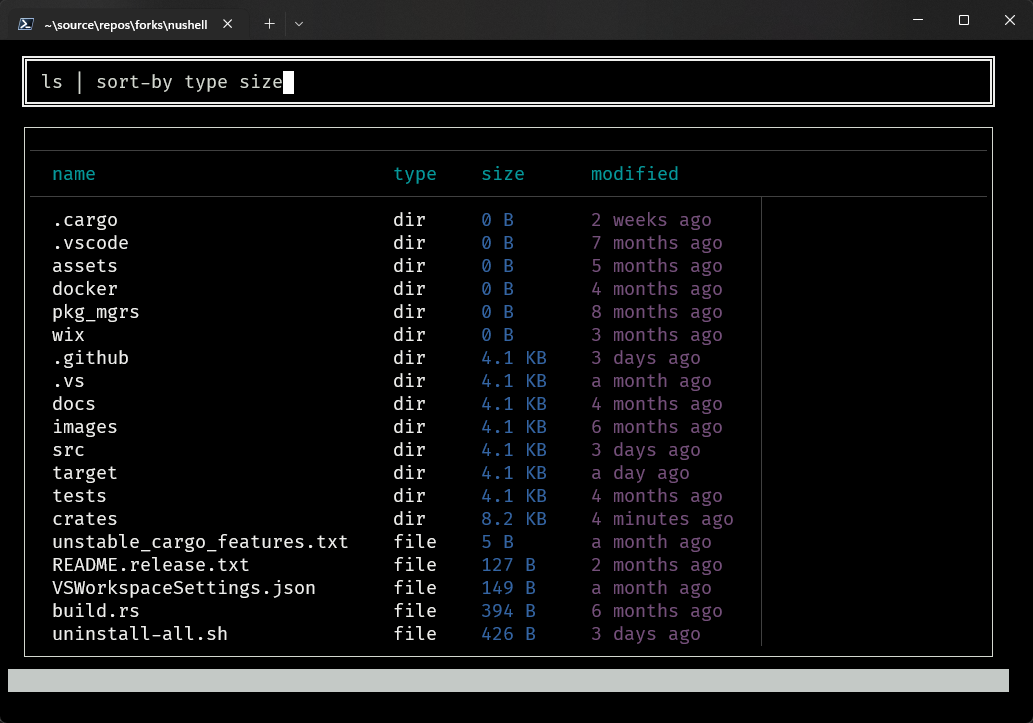
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
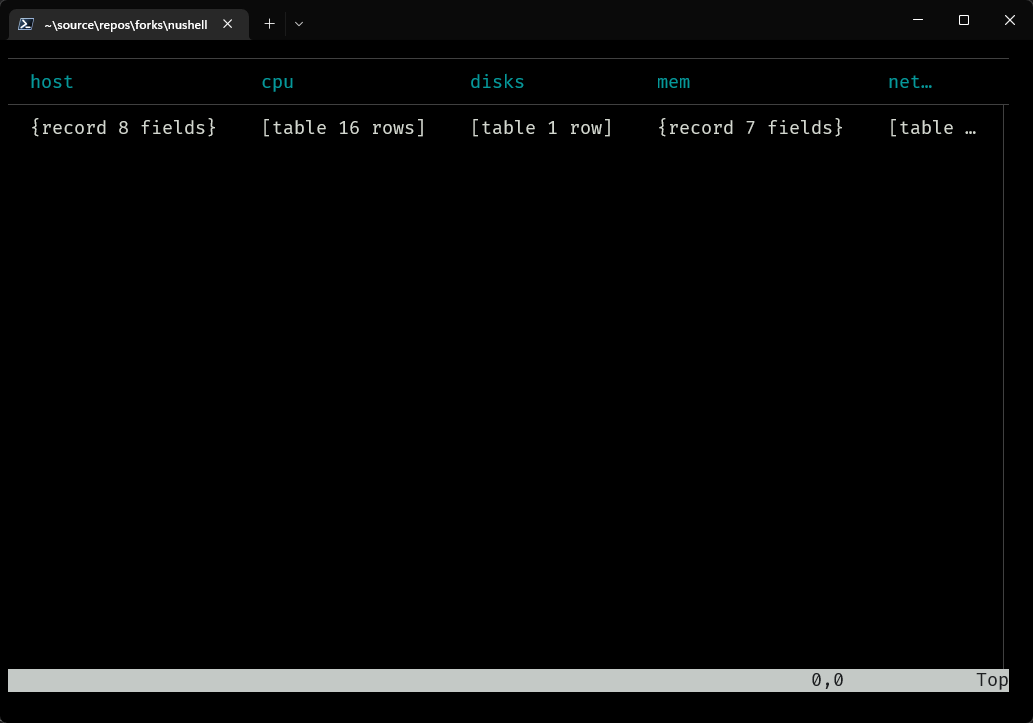
If you hit the `t` key it will now transpose the view to look like this.
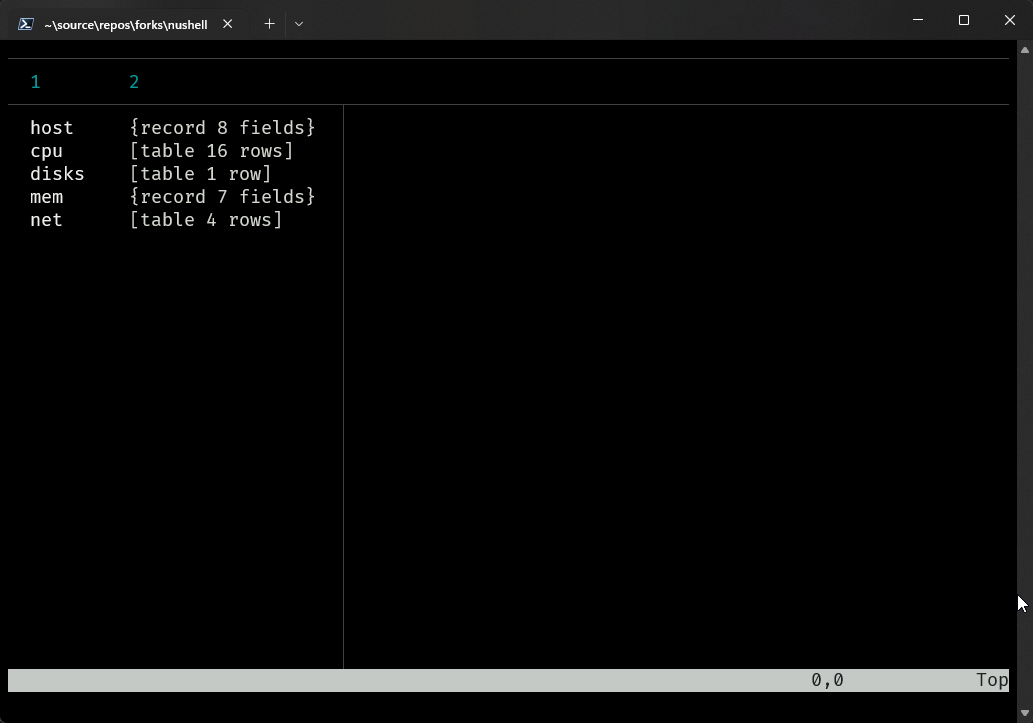
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
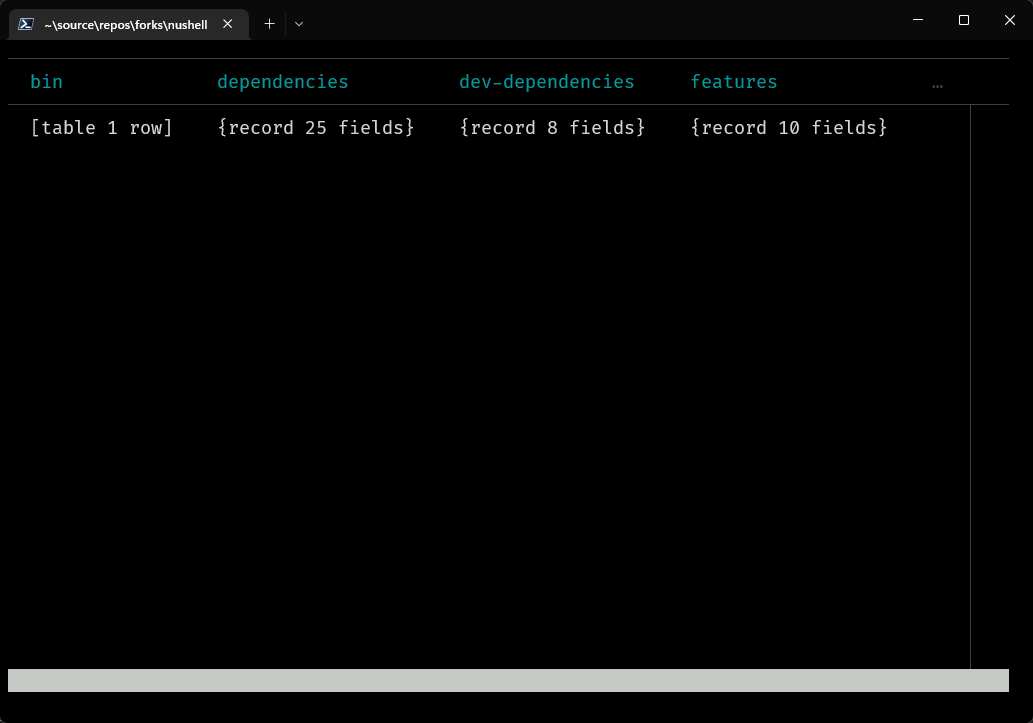
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
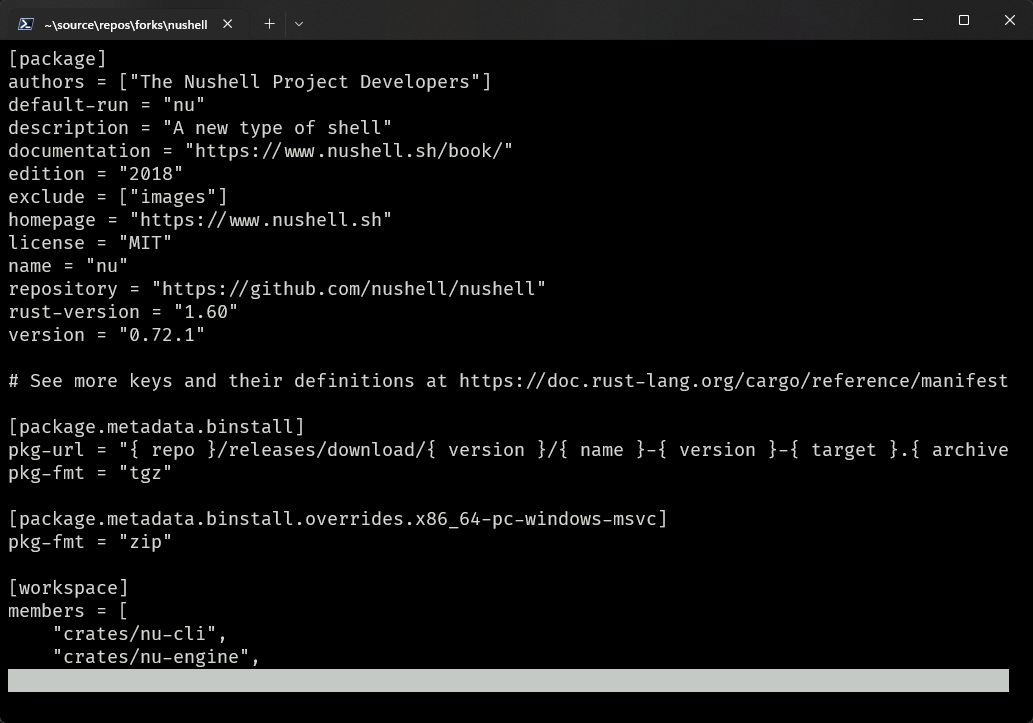
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
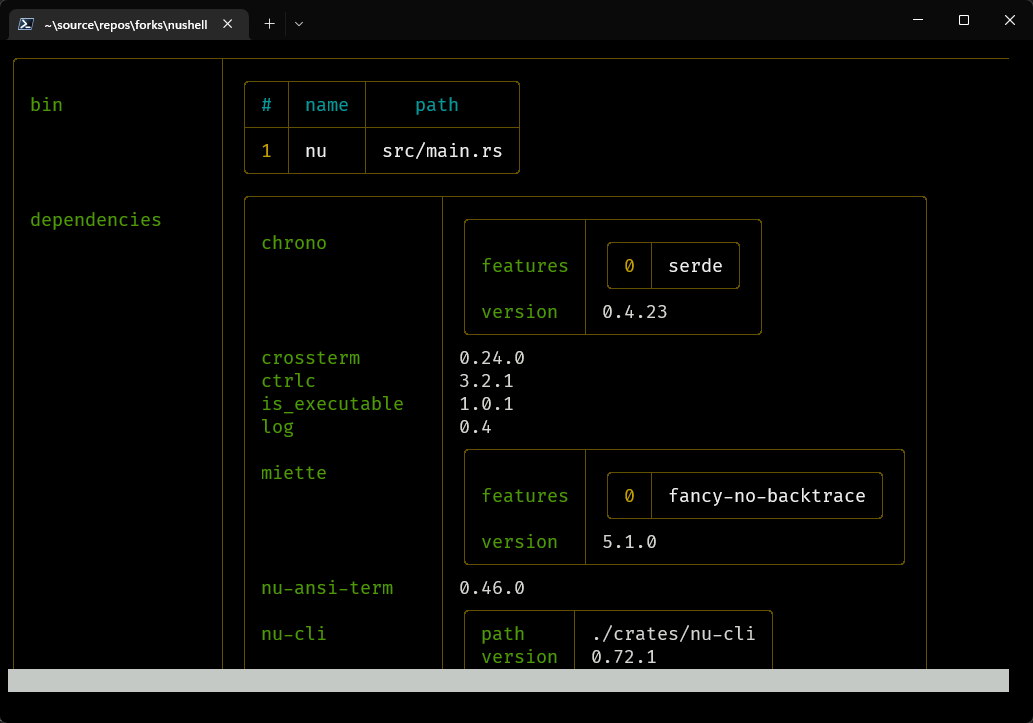
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 15:32:10 +00:00
|
|
|
}
|
|
|
|
|
2022-12-16 15:47:07 +00:00
|
|
|
fn insert_bool(map: &mut HashMap<String, Value>, key: &str, value: bool) {
|
|
|
|
if map.contains_key(key) {
|
|
|
|
return;
|
|
|
|
}
|
|
|
|
|
2023-07-21 13:20:33 +00:00
|
|
|
map.insert(String::from(key), Value::bool(value, Span::unknown()));
|
2022-12-16 15:47:07 +00:00
|
|
|
}
|
|
|
|
|
2022-12-18 14:43:15 +00:00
|
|
|
fn include_nu_config(config: &mut HashMap<String, Value>, style_computer: &StyleComputer) {
|
|
|
|
let line_color = lookup_color(style_computer, "separator");
|
|
|
|
if line_color != nu_ansi_term::Style::default() {
|
|
|
|
{
|
|
|
|
let mut map = config
|
|
|
|
.get("table")
|
|
|
|
.and_then(parse_hash_map)
|
|
|
|
.unwrap_or_default();
|
|
|
|
insert_style(&mut map, "split_line", line_color);
|
|
|
|
config.insert(String::from("table"), map_into_value(map));
|
|
|
|
}
|
|
|
|
|
|
|
|
{
|
|
|
|
let mut map = config
|
|
|
|
.get("try")
|
|
|
|
.and_then(parse_hash_map)
|
|
|
|
.unwrap_or_default();
|
|
|
|
insert_style(&mut map, "border_color", line_color);
|
|
|
|
config.insert(String::from("try"), map_into_value(map));
|
|
|
|
}
|
|
|
|
|
|
|
|
{
|
|
|
|
let mut map = config
|
|
|
|
.get("config")
|
|
|
|
.and_then(parse_hash_map)
|
|
|
|
.unwrap_or_default();
|
2022-12-19 17:34:21 +00:00
|
|
|
|
2022-12-18 14:43:15 +00:00
|
|
|
insert_style(&mut map, "border_color", line_color);
|
2022-12-19 17:34:21 +00:00
|
|
|
|
2022-12-18 14:43:15 +00:00
|
|
|
config.insert(String::from("config"), map_into_value(map));
|
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
fn lookup_color(style_computer: &StyleComputer, key: &str) -> nu_ansi_term::Style {
|
|
|
|
style_computer.compute(key, &Value::nothing(Span::unknown()))
|
|
|
|
}
|