2022-04-04 14:54:48 +00:00
|
|
|
use super::DescriptionMenu;
|
|
|
|
use crate::{menus::NuMenuCompleter, NuHelpCompleter};
|
2022-01-18 08:48:28 +00:00
|
|
|
use crossterm::event::{KeyCode, KeyModifiers};
|
color_config now accepts closures as color values (#7141)
# Description
Closes #6909. You can now add closures to your `color_config` themes.
Whenever a value would be printed with `table`, the closure is run with
the value piped-in. The closure must return either a {fg,bg,attr} record
or a color name (`'light_red'` etc.). This returned style is used to
colour the value.
This is entirely backwards-compatible with existing config.nu files.
Example code excerpt:
```
let my_theme = {
header: green_bold
bool: { if $in { 'light_cyan' } else { 'light_red' } }
int: purple_bold
filesize: { |e| if $e == 0b { 'gray' } else if $e < 1mb { 'purple_bold' } else { 'cyan_bold' } }
duration: purple_bold
date: { (date now) - $in | if $in > 1wk { 'cyan_bold' } else if $in > 1day { 'green_bold' } else { 'yellow_bold' } }
range: yellow_bold
string: { if $in =~ '^#\w{6}$' { $in } else { 'white' } }
nothing: white
```
Example output with this in effect:
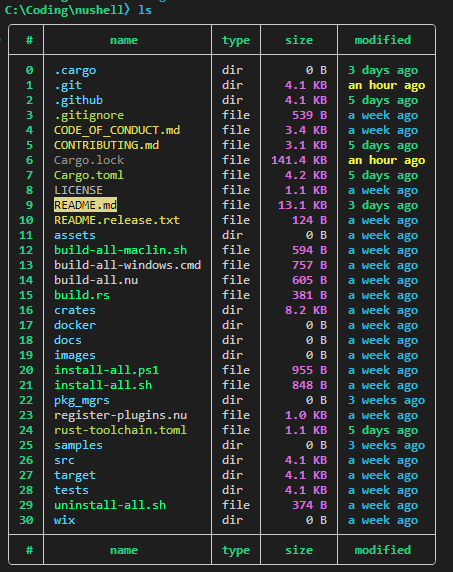
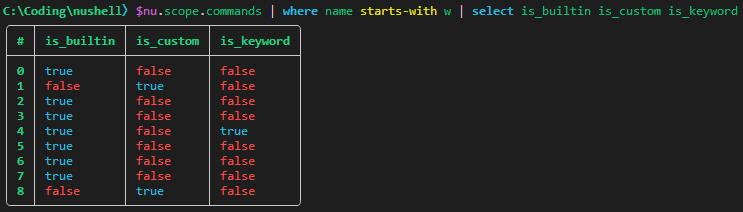
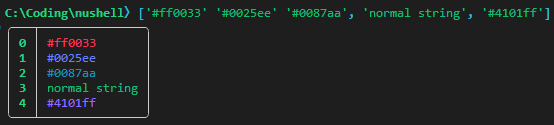
Slightly important notes:
* Some color_config names, namely "separator", "empty" and "hints", pipe
in `null` instead of a value.
* Currently, doing anything non-trivial inside a closure has an
understandably big perf hit. I currently do not actually recommend
something like `string: { if $in =~ '^#\w{6}$' { $in } else { 'white' }
}` for serious work, mainly because of the abundance of string-type data
in the world. Nevertheless, lesser-used types like "date" and "duration"
work well with this.
* I had to do some reorganisation in order to make it possible to call
`eval_block()` that late in table rendering. I invented a new struct
called "StyleComputer" which holds the engine_state and stack of the
initial `table` command (implicit or explicit).
* StyleComputer has a `compute()` method which takes a color_config name
and a nu value, and always returns the correct Style, so you don't have
to worry about A) the color_config value was set at all, B) whether it
was set to a closure or not, or C) which default style to use in those
cases.
* Currently, errors encountered during execution of the closures are
thrown in the garbage. Any other ideas are welcome. (Nonetheless, errors
result in a huge perf hit when they are encountered. I think what should
be done is to assume something terrible happened to the user's config
and invalidate the StyleComputer for that `table` run, thus causing
subsequent output to just be Style::default().)
* More thorough tests are forthcoming - ran into some difficulty using
`nu!` to take an alternative config, and for some reason `let-env config
=` statements don't seem to work inside `nu!` pipelines(???)
* The default config.nu has not been updated to make use of this yet. Do
tell if you think I should incorporate that into this.
# User-Facing Changes
See above.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace --features=extra -- -D warnings -D
clippy::unwrap_used -A clippy::needless_collect` to check that you're
using the standard code style
- `cargo test --workspace --features=extra` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2022-12-17 13:07:56 +00:00
|
|
|
use nu_color_config::{color_record_to_nustyle, lookup_ansi_color_style};
|
2022-04-04 14:54:48 +00:00
|
|
|
use nu_engine::eval_block;
|
|
|
|
use nu_parser::parse;
|
|
|
|
use nu_protocol::{
|
color_config now accepts closures as color values (#7141)
# Description
Closes #6909. You can now add closures to your `color_config` themes.
Whenever a value would be printed with `table`, the closure is run with
the value piped-in. The closure must return either a {fg,bg,attr} record
or a color name (`'light_red'` etc.). This returned style is used to
colour the value.
This is entirely backwards-compatible with existing config.nu files.
Example code excerpt:
```
let my_theme = {
header: green_bold
bool: { if $in { 'light_cyan' } else { 'light_red' } }
int: purple_bold
filesize: { |e| if $e == 0b { 'gray' } else if $e < 1mb { 'purple_bold' } else { 'cyan_bold' } }
duration: purple_bold
date: { (date now) - $in | if $in > 1wk { 'cyan_bold' } else if $in > 1day { 'green_bold' } else { 'yellow_bold' } }
range: yellow_bold
string: { if $in =~ '^#\w{6}$' { $in } else { 'white' } }
nothing: white
```
Example output with this in effect:
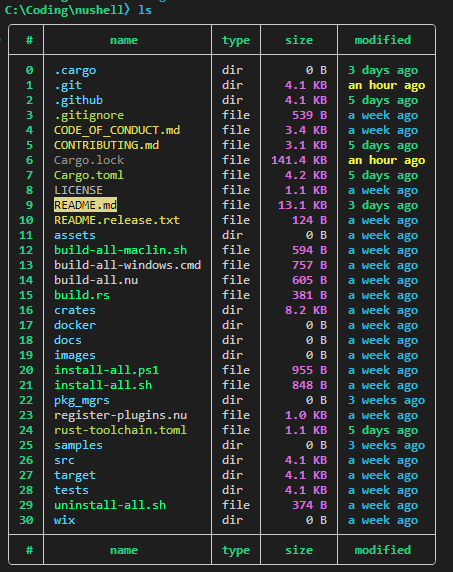
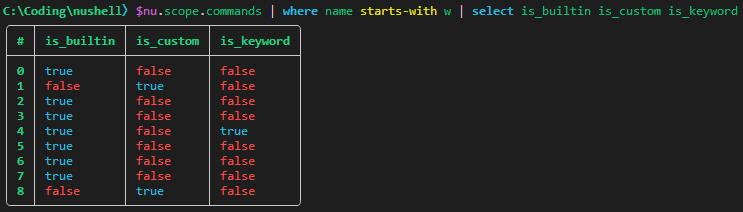
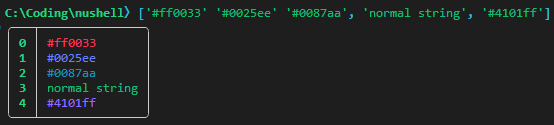
Slightly important notes:
* Some color_config names, namely "separator", "empty" and "hints", pipe
in `null` instead of a value.
* Currently, doing anything non-trivial inside a closure has an
understandably big perf hit. I currently do not actually recommend
something like `string: { if $in =~ '^#\w{6}$' { $in } else { 'white' }
}` for serious work, mainly because of the abundance of string-type data
in the world. Nevertheless, lesser-used types like "date" and "duration"
work well with this.
* I had to do some reorganisation in order to make it possible to call
`eval_block()` that late in table rendering. I invented a new struct
called "StyleComputer" which holds the engine_state and stack of the
initial `table` command (implicit or explicit).
* StyleComputer has a `compute()` method which takes a color_config name
and a nu value, and always returns the correct Style, so you don't have
to worry about A) the color_config value was set at all, B) whether it
was set to a closure or not, or C) which default style to use in those
cases.
* Currently, errors encountered during execution of the closures are
thrown in the garbage. Any other ideas are welcome. (Nonetheless, errors
result in a huge perf hit when they are encountered. I think what should
be done is to assume something terrible happened to the user's config
and invalidate the StyleComputer for that `table` run, thus causing
subsequent output to just be Style::default().)
* More thorough tests are forthcoming - ran into some difficulty using
`nu!` to take an alternative config, and for some reason `let-env config
=` statements don't seem to work inside `nu!` pipelines(???)
* The default config.nu has not been updated to make use of this yet. Do
tell if you think I should incorporate that into this.
# User-Facing Changes
See above.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace --features=extra -- -D warnings -D
clippy::unwrap_used -A clippy::needless_collect` to check that you're
using the standard code style
- `cargo test --workspace --features=extra` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2022-12-17 13:07:56 +00:00
|
|
|
create_menus,
|
2022-04-04 14:54:48 +00:00
|
|
|
engine::{EngineState, Stack, StateWorkingSet},
|
2022-12-24 13:41:57 +00:00
|
|
|
extract_value, Config, ParsedKeybinding, ParsedMenu, PipelineData, ShellError, Span, Value,
|
2022-04-04 14:54:48 +00:00
|
|
|
};
|
2022-01-18 08:48:28 +00:00
|
|
|
use reedline::{
|
2022-01-18 19:32:45 +00:00
|
|
|
default_emacs_keybindings, default_vi_insert_keybindings, default_vi_normal_keybindings,
|
2022-04-04 14:54:48 +00:00
|
|
|
ColumnarMenu, EditCommand, Keybindings, ListMenu, Reedline, ReedlineEvent, ReedlineMenu,
|
2022-01-18 08:48:28 +00:00
|
|
|
};
|
2022-04-06 12:25:02 +00:00
|
|
|
use std::sync::Arc;
|
2022-01-18 08:48:28 +00:00
|
|
|
|
2022-04-04 14:54:48 +00:00
|
|
|
const DEFAULT_COMPLETION_MENU: &str = r#"
|
|
|
|
{
|
|
|
|
name: completion_menu
|
|
|
|
only_buffer_difference: false
|
|
|
|
marker: "| "
|
|
|
|
type: {
|
|
|
|
layout: columnar
|
|
|
|
columns: 4
|
2022-05-07 19:39:22 +00:00
|
|
|
col_width: 20
|
2022-04-04 14:54:48 +00:00
|
|
|
col_padding: 2
|
|
|
|
}
|
|
|
|
style: {
|
|
|
|
text: green,
|
|
|
|
selected_text: green_reverse
|
|
|
|
description_text: yellow
|
|
|
|
}
|
|
|
|
}"#;
|
|
|
|
|
|
|
|
const DEFAULT_HISTORY_MENU: &str = r#"
|
|
|
|
{
|
|
|
|
name: history_menu
|
|
|
|
only_buffer_difference: true
|
|
|
|
marker: "? "
|
|
|
|
type: {
|
|
|
|
layout: list
|
|
|
|
page_size: 10
|
|
|
|
}
|
|
|
|
style: {
|
|
|
|
text: green,
|
|
|
|
selected_text: green_reverse
|
|
|
|
description_text: yellow
|
|
|
|
}
|
|
|
|
}"#;
|
|
|
|
|
|
|
|
const DEFAULT_HELP_MENU: &str = r#"
|
|
|
|
{
|
|
|
|
name: help_menu
|
|
|
|
only_buffer_difference: true
|
|
|
|
marker: "? "
|
|
|
|
type: {
|
|
|
|
layout: description
|
|
|
|
columns: 4
|
2022-05-07 19:39:22 +00:00
|
|
|
col_width: 20
|
2022-04-04 14:54:48 +00:00
|
|
|
col_padding: 2
|
|
|
|
selection_rows: 4
|
|
|
|
description_rows: 10
|
|
|
|
}
|
|
|
|
style: {
|
|
|
|
text: green,
|
|
|
|
selected_text: green_reverse
|
|
|
|
description_text: yellow
|
|
|
|
}
|
|
|
|
}"#;
|
|
|
|
|
|
|
|
// Adds all menus to line editor
|
|
|
|
pub(crate) fn add_menus(
|
|
|
|
mut line_editor: Reedline,
|
2022-04-06 12:25:02 +00:00
|
|
|
engine_state: Arc<EngineState>,
|
2022-04-04 14:54:48 +00:00
|
|
|
stack: &Stack,
|
|
|
|
config: &Config,
|
|
|
|
) -> Result<Reedline, ShellError> {
|
|
|
|
line_editor = line_editor.clear_menus();
|
|
|
|
|
|
|
|
for menu in &config.menus {
|
2022-04-06 12:25:02 +00:00
|
|
|
line_editor = add_menu(line_editor, menu, engine_state.clone(), stack, config)?
|
2022-04-04 14:54:48 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
// Checking if the default menus have been added from the config file
|
|
|
|
let default_menus = vec![
|
|
|
|
("completion_menu", DEFAULT_COMPLETION_MENU),
|
|
|
|
("history_menu", DEFAULT_HISTORY_MENU),
|
|
|
|
("help_menu", DEFAULT_HELP_MENU),
|
|
|
|
];
|
|
|
|
|
|
|
|
for (name, definition) in default_menus {
|
|
|
|
if !config
|
|
|
|
.menus
|
|
|
|
.iter()
|
|
|
|
.any(|menu| menu.name.into_string("", config) == name)
|
|
|
|
{
|
|
|
|
let (block, _) = {
|
2022-04-06 12:25:02 +00:00
|
|
|
let mut working_set = StateWorkingSet::new(&engine_state);
|
2022-04-04 14:54:48 +00:00
|
|
|
let (output, _) = parse(
|
|
|
|
&mut working_set,
|
|
|
|
Some(name), // format!("entry #{}", entry_num)
|
|
|
|
definition.as_bytes(),
|
|
|
|
true,
|
|
|
|
&[],
|
|
|
|
);
|
|
|
|
|
|
|
|
(output, working_set.render())
|
|
|
|
};
|
|
|
|
|
|
|
|
let mut temp_stack = Stack::new();
|
2022-12-24 13:41:57 +00:00
|
|
|
let input = PipelineData::Empty;
|
2022-04-06 12:25:02 +00:00
|
|
|
let res = eval_block(&engine_state, &mut temp_stack, &block, input, false, false)?;
|
2022-04-04 14:54:48 +00:00
|
|
|
|
|
|
|
if let PipelineData::Value(value, None) = res {
|
2022-11-04 20:11:17 +00:00
|
|
|
for menu in create_menus(&value)? {
|
2022-04-06 12:25:02 +00:00
|
|
|
line_editor =
|
|
|
|
add_menu(line_editor, &menu, engine_state.clone(), stack, config)?;
|
2022-04-04 14:54:48 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
Ok(line_editor)
|
2022-01-18 08:48:28 +00:00
|
|
|
}
|
|
|
|
|
2022-04-04 14:54:48 +00:00
|
|
|
fn add_menu(
|
|
|
|
line_editor: Reedline,
|
|
|
|
menu: &ParsedMenu,
|
2022-04-06 12:25:02 +00:00
|
|
|
engine_state: Arc<EngineState>,
|
2022-04-04 14:54:48 +00:00
|
|
|
stack: &Stack,
|
|
|
|
config: &Config,
|
|
|
|
) -> Result<Reedline, ShellError> {
|
|
|
|
if let Value::Record { cols, vals, span } = &menu.menu_type {
|
|
|
|
let layout = extract_value("layout", cols, vals, span)?.into_string("", config);
|
|
|
|
|
|
|
|
match layout.as_str() {
|
|
|
|
"columnar" => add_columnar_menu(line_editor, menu, engine_state, stack, config),
|
|
|
|
"list" => add_list_menu(line_editor, menu, engine_state, stack, config),
|
|
|
|
"description" => add_description_menu(line_editor, menu, engine_state, stack, config),
|
|
|
|
_ => Err(ShellError::UnsupportedConfigValue(
|
|
|
|
"columnar, list or description".to_string(),
|
|
|
|
menu.menu_type.into_abbreviated_string(config),
|
|
|
|
menu.menu_type.span()?,
|
|
|
|
)),
|
2022-01-25 09:39:22 +00:00
|
|
|
}
|
2022-04-04 14:54:48 +00:00
|
|
|
} else {
|
|
|
|
Err(ShellError::UnsupportedConfigValue(
|
|
|
|
"only record type".to_string(),
|
|
|
|
menu.menu_type.into_abbreviated_string(config),
|
|
|
|
menu.menu_type.span()?,
|
|
|
|
))
|
|
|
|
}
|
2022-03-27 13:01:04 +00:00
|
|
|
}
|
|
|
|
|
2022-04-04 21:36:48 +00:00
|
|
|
macro_rules! add_style {
|
|
|
|
// first arm match add!(1,2), add!(2,3) etc
|
|
|
|
($name:expr, $cols: expr, $vals:expr, $span:expr, $config: expr, $menu:expr, $f:expr) => {
|
|
|
|
$menu = match extract_value($name, $cols, $vals, $span) {
|
|
|
|
Ok(text) => {
|
color_config now accepts closures as color values (#7141)
# Description
Closes #6909. You can now add closures to your `color_config` themes.
Whenever a value would be printed with `table`, the closure is run with
the value piped-in. The closure must return either a {fg,bg,attr} record
or a color name (`'light_red'` etc.). This returned style is used to
colour the value.
This is entirely backwards-compatible with existing config.nu files.
Example code excerpt:
```
let my_theme = {
header: green_bold
bool: { if $in { 'light_cyan' } else { 'light_red' } }
int: purple_bold
filesize: { |e| if $e == 0b { 'gray' } else if $e < 1mb { 'purple_bold' } else { 'cyan_bold' } }
duration: purple_bold
date: { (date now) - $in | if $in > 1wk { 'cyan_bold' } else if $in > 1day { 'green_bold' } else { 'yellow_bold' } }
range: yellow_bold
string: { if $in =~ '^#\w{6}$' { $in } else { 'white' } }
nothing: white
```
Example output with this in effect:
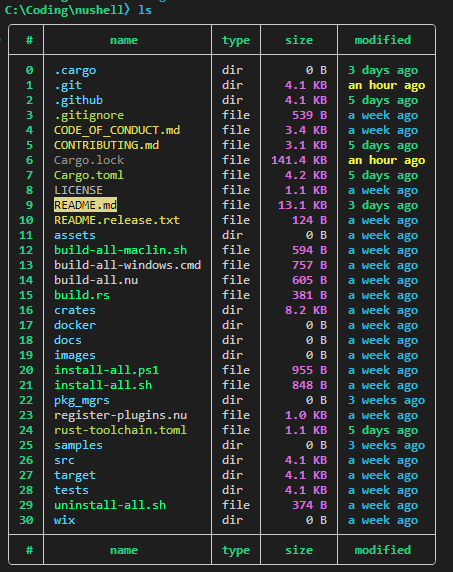
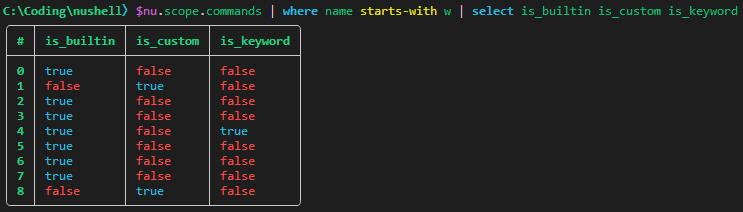
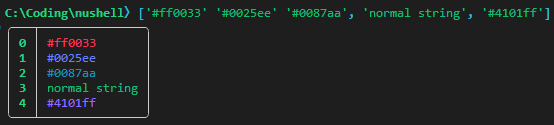
Slightly important notes:
* Some color_config names, namely "separator", "empty" and "hints", pipe
in `null` instead of a value.
* Currently, doing anything non-trivial inside a closure has an
understandably big perf hit. I currently do not actually recommend
something like `string: { if $in =~ '^#\w{6}$' { $in } else { 'white' }
}` for serious work, mainly because of the abundance of string-type data
in the world. Nevertheless, lesser-used types like "date" and "duration"
work well with this.
* I had to do some reorganisation in order to make it possible to call
`eval_block()` that late in table rendering. I invented a new struct
called "StyleComputer" which holds the engine_state and stack of the
initial `table` command (implicit or explicit).
* StyleComputer has a `compute()` method which takes a color_config name
and a nu value, and always returns the correct Style, so you don't have
to worry about A) the color_config value was set at all, B) whether it
was set to a closure or not, or C) which default style to use in those
cases.
* Currently, errors encountered during execution of the closures are
thrown in the garbage. Any other ideas are welcome. (Nonetheless, errors
result in a huge perf hit when they are encountered. I think what should
be done is to assume something terrible happened to the user's config
and invalidate the StyleComputer for that `table` run, thus causing
subsequent output to just be Style::default().)
* More thorough tests are forthcoming - ran into some difficulty using
`nu!` to take an alternative config, and for some reason `let-env config
=` statements don't seem to work inside `nu!` pipelines(???)
* The default config.nu has not been updated to make use of this yet. Do
tell if you think I should incorporate that into this.
# User-Facing Changes
See above.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace --features=extra -- -D warnings -D
clippy::unwrap_used -A clippy::needless_collect` to check that you're
using the standard code style
- `cargo test --workspace --features=extra` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2022-12-17 13:07:56 +00:00
|
|
|
let style = match text {
|
|
|
|
Value::String { val, .. } => lookup_ansi_color_style(&val),
|
|
|
|
Value::Record { .. } => color_record_to_nustyle(&text),
|
|
|
|
_ => lookup_ansi_color_style("green"),
|
2022-04-04 21:36:48 +00:00
|
|
|
};
|
|
|
|
$f($menu, style)
|
|
|
|
}
|
|
|
|
Err(_) => $menu,
|
|
|
|
};
|
|
|
|
};
|
|
|
|
}
|
|
|
|
|
2022-04-04 14:54:48 +00:00
|
|
|
// Adds a columnar menu to the editor engine
|
|
|
|
pub(crate) fn add_columnar_menu(
|
2022-03-27 13:01:04 +00:00
|
|
|
line_editor: Reedline,
|
2022-04-04 14:54:48 +00:00
|
|
|
menu: &ParsedMenu,
|
2022-04-06 12:25:02 +00:00
|
|
|
engine_state: Arc<EngineState>,
|
2022-04-04 14:54:48 +00:00
|
|
|
stack: &Stack,
|
2022-03-27 13:01:04 +00:00
|
|
|
config: &Config,
|
2022-04-04 14:54:48 +00:00
|
|
|
) -> Result<Reedline, ShellError> {
|
|
|
|
let name = menu.name.into_string("", config);
|
|
|
|
let mut columnar_menu = ColumnarMenu::default().with_name(&name);
|
|
|
|
|
|
|
|
if let Value::Record { cols, vals, span } = &menu.menu_type {
|
|
|
|
columnar_menu = match extract_value("columns", cols, vals, span) {
|
|
|
|
Ok(columns) => {
|
|
|
|
let columns = columns.as_integer()?;
|
|
|
|
columnar_menu.with_columns(columns as u16)
|
|
|
|
}
|
|
|
|
Err(_) => columnar_menu,
|
|
|
|
};
|
|
|
|
|
|
|
|
columnar_menu = match extract_value("col_width", cols, vals, span) {
|
|
|
|
Ok(col_width) => {
|
|
|
|
let col_width = col_width.as_integer()?;
|
|
|
|
columnar_menu.with_column_width(Some(col_width as usize))
|
|
|
|
}
|
|
|
|
Err(_) => columnar_menu.with_column_width(None),
|
|
|
|
};
|
|
|
|
|
|
|
|
columnar_menu = match extract_value("col_padding", cols, vals, span) {
|
|
|
|
Ok(col_padding) => {
|
|
|
|
let col_padding = col_padding.as_integer()?;
|
|
|
|
columnar_menu.with_column_padding(col_padding as usize)
|
|
|
|
}
|
|
|
|
Err(_) => columnar_menu,
|
|
|
|
};
|
|
|
|
}
|
|
|
|
|
|
|
|
if let Value::Record { cols, vals, span } = &menu.style {
|
2022-04-04 21:36:48 +00:00
|
|
|
add_style!(
|
|
|
|
"text",
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
span,
|
|
|
|
config,
|
|
|
|
columnar_menu,
|
|
|
|
ColumnarMenu::with_text_style
|
|
|
|
);
|
|
|
|
add_style!(
|
|
|
|
"selected_text",
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
span,
|
|
|
|
config,
|
|
|
|
columnar_menu,
|
|
|
|
ColumnarMenu::with_selected_text_style
|
|
|
|
);
|
|
|
|
add_style!(
|
|
|
|
"description_text",
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
span,
|
|
|
|
config,
|
|
|
|
columnar_menu,
|
|
|
|
ColumnarMenu::with_description_text_style
|
|
|
|
);
|
2022-04-04 14:54:48 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
let marker = menu.marker.into_string("", config);
|
|
|
|
columnar_menu = columnar_menu.with_marker(marker);
|
|
|
|
|
|
|
|
let only_buffer_difference = menu.only_buffer_difference.as_bool()?;
|
|
|
|
columnar_menu = columnar_menu.with_only_buffer_difference(only_buffer_difference);
|
|
|
|
|
|
|
|
match &menu.source {
|
|
|
|
Value::Nothing { .. } => {
|
|
|
|
Ok(line_editor.with_menu(ReedlineMenu::EngineCompleter(Box::new(columnar_menu))))
|
|
|
|
}
|
2022-11-10 08:21:49 +00:00
|
|
|
Value::Closure {
|
2022-04-04 14:54:48 +00:00
|
|
|
val,
|
|
|
|
captures,
|
|
|
|
span,
|
|
|
|
} => {
|
|
|
|
let menu_completer = NuMenuCompleter::new(
|
|
|
|
*val,
|
|
|
|
*span,
|
|
|
|
stack.captures_to_stack(captures),
|
2022-04-06 12:25:02 +00:00
|
|
|
engine_state,
|
2022-04-04 14:54:48 +00:00
|
|
|
only_buffer_difference,
|
|
|
|
);
|
|
|
|
Ok(line_editor.with_menu(ReedlineMenu::WithCompleter {
|
|
|
|
menu: Box::new(columnar_menu),
|
|
|
|
completer: Box::new(menu_completer),
|
|
|
|
}))
|
|
|
|
}
|
|
|
|
_ => Err(ShellError::UnsupportedConfigValue(
|
|
|
|
"block or omitted value".to_string(),
|
|
|
|
menu.source.into_abbreviated_string(config),
|
|
|
|
menu.source.span()?,
|
|
|
|
)),
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
// Adds a search menu to the line editor
|
|
|
|
pub(crate) fn add_list_menu(
|
|
|
|
line_editor: Reedline,
|
|
|
|
menu: &ParsedMenu,
|
2022-04-06 12:25:02 +00:00
|
|
|
engine_state: Arc<EngineState>,
|
2022-04-04 14:54:48 +00:00
|
|
|
stack: &Stack,
|
|
|
|
config: &Config,
|
|
|
|
) -> Result<Reedline, ShellError> {
|
|
|
|
let name = menu.name.into_string("", config);
|
|
|
|
let mut list_menu = ListMenu::default().with_name(&name);
|
|
|
|
|
|
|
|
if let Value::Record { cols, vals, span } = &menu.menu_type {
|
|
|
|
list_menu = match extract_value("page_size", cols, vals, span) {
|
|
|
|
Ok(page_size) => {
|
|
|
|
let page_size = page_size.as_integer()?;
|
|
|
|
list_menu.with_page_size(page_size as usize)
|
|
|
|
}
|
|
|
|
Err(_) => list_menu,
|
|
|
|
};
|
|
|
|
}
|
|
|
|
|
|
|
|
if let Value::Record { cols, vals, span } = &menu.style {
|
2022-04-04 21:36:48 +00:00
|
|
|
add_style!(
|
|
|
|
"text",
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
span,
|
|
|
|
config,
|
|
|
|
list_menu,
|
|
|
|
ListMenu::with_text_style
|
|
|
|
);
|
|
|
|
add_style!(
|
|
|
|
"selected_text",
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
span,
|
|
|
|
config,
|
|
|
|
list_menu,
|
|
|
|
ListMenu::with_selected_text_style
|
|
|
|
);
|
|
|
|
add_style!(
|
|
|
|
"description_text",
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
span,
|
|
|
|
config,
|
|
|
|
list_menu,
|
|
|
|
ListMenu::with_description_text_style
|
|
|
|
);
|
2022-04-04 14:54:48 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
let marker = menu.marker.into_string("", config);
|
|
|
|
list_menu = list_menu.with_marker(marker);
|
|
|
|
|
|
|
|
let only_buffer_difference = menu.only_buffer_difference.as_bool()?;
|
|
|
|
list_menu = list_menu.with_only_buffer_difference(only_buffer_difference);
|
|
|
|
|
|
|
|
match &menu.source {
|
|
|
|
Value::Nothing { .. } => {
|
|
|
|
Ok(line_editor.with_menu(ReedlineMenu::HistoryMenu(Box::new(list_menu))))
|
|
|
|
}
|
2022-11-10 08:21:49 +00:00
|
|
|
Value::Closure {
|
2022-04-04 14:54:48 +00:00
|
|
|
val,
|
|
|
|
captures,
|
|
|
|
span,
|
|
|
|
} => {
|
|
|
|
let menu_completer = NuMenuCompleter::new(
|
|
|
|
*val,
|
|
|
|
*span,
|
|
|
|
stack.captures_to_stack(captures),
|
2022-04-06 12:25:02 +00:00
|
|
|
engine_state,
|
2022-04-04 14:54:48 +00:00
|
|
|
only_buffer_difference,
|
|
|
|
);
|
|
|
|
Ok(line_editor.with_menu(ReedlineMenu::WithCompleter {
|
|
|
|
menu: Box::new(list_menu),
|
|
|
|
completer: Box::new(menu_completer),
|
|
|
|
}))
|
|
|
|
}
|
|
|
|
_ => Err(ShellError::UnsupportedConfigValue(
|
|
|
|
"block or omitted value".to_string(),
|
|
|
|
menu.source.into_abbreviated_string(config),
|
|
|
|
menu.source.span()?,
|
|
|
|
)),
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
// Adds a description menu to the line editor
|
|
|
|
pub(crate) fn add_description_menu(
|
|
|
|
line_editor: Reedline,
|
|
|
|
menu: &ParsedMenu,
|
2022-04-06 12:25:02 +00:00
|
|
|
engine_state: Arc<EngineState>,
|
2022-04-04 14:54:48 +00:00
|
|
|
stack: &Stack,
|
|
|
|
config: &Config,
|
|
|
|
) -> Result<Reedline, ShellError> {
|
|
|
|
let name = menu.name.into_string("", config);
|
|
|
|
let mut description_menu = DescriptionMenu::default().with_name(&name);
|
|
|
|
|
|
|
|
if let Value::Record { cols, vals, span } = &menu.menu_type {
|
|
|
|
description_menu = match extract_value("columns", cols, vals, span) {
|
|
|
|
Ok(columns) => {
|
|
|
|
let columns = columns.as_integer()?;
|
|
|
|
description_menu.with_columns(columns as u16)
|
|
|
|
}
|
|
|
|
Err(_) => description_menu,
|
|
|
|
};
|
|
|
|
|
|
|
|
description_menu = match extract_value("col_width", cols, vals, span) {
|
|
|
|
Ok(col_width) => {
|
|
|
|
let col_width = col_width.as_integer()?;
|
|
|
|
description_menu.with_column_width(Some(col_width as usize))
|
|
|
|
}
|
|
|
|
Err(_) => description_menu.with_column_width(None),
|
|
|
|
};
|
|
|
|
|
|
|
|
description_menu = match extract_value("col_padding", cols, vals, span) {
|
|
|
|
Ok(col_padding) => {
|
|
|
|
let col_padding = col_padding.as_integer()?;
|
|
|
|
description_menu.with_column_padding(col_padding as usize)
|
|
|
|
}
|
|
|
|
Err(_) => description_menu,
|
|
|
|
};
|
|
|
|
|
|
|
|
description_menu = match extract_value("selection_rows", cols, vals, span) {
|
|
|
|
Ok(selection_rows) => {
|
|
|
|
let selection_rows = selection_rows.as_integer()?;
|
|
|
|
description_menu.with_selection_rows(selection_rows as u16)
|
|
|
|
}
|
|
|
|
Err(_) => description_menu,
|
|
|
|
};
|
|
|
|
|
|
|
|
description_menu = match extract_value("description_rows", cols, vals, span) {
|
|
|
|
Ok(description_rows) => {
|
|
|
|
let description_rows = description_rows.as_integer()?;
|
|
|
|
description_menu.with_description_rows(description_rows as usize)
|
|
|
|
}
|
|
|
|
Err(_) => description_menu,
|
|
|
|
};
|
|
|
|
}
|
|
|
|
|
|
|
|
if let Value::Record { cols, vals, span } = &menu.style {
|
2022-04-04 21:36:48 +00:00
|
|
|
add_style!(
|
|
|
|
"text",
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
span,
|
|
|
|
config,
|
|
|
|
description_menu,
|
|
|
|
DescriptionMenu::with_text_style
|
|
|
|
);
|
|
|
|
add_style!(
|
|
|
|
"selected_text",
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
span,
|
|
|
|
config,
|
|
|
|
description_menu,
|
|
|
|
DescriptionMenu::with_selected_text_style
|
|
|
|
);
|
|
|
|
add_style!(
|
|
|
|
"description_text",
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
span,
|
|
|
|
config,
|
|
|
|
description_menu,
|
|
|
|
DescriptionMenu::with_description_text_style
|
|
|
|
);
|
2022-04-04 14:54:48 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
let marker = menu.marker.into_string("", config);
|
|
|
|
description_menu = description_menu.with_marker(marker);
|
|
|
|
|
|
|
|
let only_buffer_difference = menu.only_buffer_difference.as_bool()?;
|
|
|
|
description_menu = description_menu.with_only_buffer_difference(only_buffer_difference);
|
|
|
|
|
|
|
|
match &menu.source {
|
|
|
|
Value::Nothing { .. } => {
|
2022-04-06 12:25:02 +00:00
|
|
|
let completer = Box::new(NuHelpCompleter::new(engine_state));
|
2022-04-04 14:54:48 +00:00
|
|
|
Ok(line_editor.with_menu(ReedlineMenu::WithCompleter {
|
|
|
|
menu: Box::new(description_menu),
|
|
|
|
completer,
|
|
|
|
}))
|
|
|
|
}
|
2022-11-10 08:21:49 +00:00
|
|
|
Value::Closure {
|
2022-04-04 14:54:48 +00:00
|
|
|
val,
|
|
|
|
captures,
|
|
|
|
span,
|
|
|
|
} => {
|
|
|
|
let menu_completer = NuMenuCompleter::new(
|
|
|
|
*val,
|
|
|
|
*span,
|
|
|
|
stack.captures_to_stack(captures),
|
2022-04-06 12:25:02 +00:00
|
|
|
engine_state,
|
2022-04-04 14:54:48 +00:00
|
|
|
only_buffer_difference,
|
|
|
|
);
|
|
|
|
Ok(line_editor.with_menu(ReedlineMenu::WithCompleter {
|
|
|
|
menu: Box::new(description_menu),
|
|
|
|
completer: Box::new(menu_completer),
|
|
|
|
}))
|
|
|
|
}
|
|
|
|
_ => Err(ShellError::UnsupportedConfigValue(
|
2022-11-10 08:21:49 +00:00
|
|
|
"closure or omitted value".to_string(),
|
2022-04-04 14:54:48 +00:00
|
|
|
menu.source.into_abbreviated_string(config),
|
|
|
|
menu.source.span()?,
|
|
|
|
)),
|
|
|
|
}
|
2022-01-27 07:53:23 +00:00
|
|
|
}
|
|
|
|
|
2022-02-28 13:54:40 +00:00
|
|
|
fn add_menu_keybindings(keybindings: &mut Keybindings) {
|
2022-03-27 13:01:04 +00:00
|
|
|
// Completer menu keybindings
|
|
|
|
keybindings.add_binding(
|
|
|
|
KeyModifiers::NONE,
|
|
|
|
KeyCode::Tab,
|
|
|
|
ReedlineEvent::UntilFound(vec![
|
2022-03-27 20:21:40 +00:00
|
|
|
ReedlineEvent::Menu("completion_menu".to_string()),
|
2022-10-20 10:39:48 +00:00
|
|
|
ReedlineEvent::Edit(vec![EditCommand::Complete]),
|
2022-03-27 13:01:04 +00:00
|
|
|
]),
|
|
|
|
);
|
|
|
|
|
|
|
|
keybindings.add_binding(
|
|
|
|
KeyModifiers::SHIFT,
|
|
|
|
KeyCode::BackTab,
|
|
|
|
ReedlineEvent::MenuPrevious,
|
|
|
|
);
|
|
|
|
|
2022-05-13 14:26:14 +00:00
|
|
|
keybindings.add_binding(
|
|
|
|
KeyModifiers::CONTROL,
|
|
|
|
KeyCode::Char('r'),
|
|
|
|
ReedlineEvent::Menu("history_menu".to_string()),
|
|
|
|
);
|
|
|
|
|
2022-02-28 13:54:40 +00:00
|
|
|
keybindings.add_binding(
|
|
|
|
KeyModifiers::CONTROL,
|
|
|
|
KeyCode::Char('x'),
|
2022-05-13 14:26:14 +00:00
|
|
|
ReedlineEvent::MenuPageNext,
|
2022-02-28 13:54:40 +00:00
|
|
|
);
|
|
|
|
|
|
|
|
keybindings.add_binding(
|
|
|
|
KeyModifiers::CONTROL,
|
|
|
|
KeyCode::Char('z'),
|
|
|
|
ReedlineEvent::UntilFound(vec![
|
|
|
|
ReedlineEvent::MenuPagePrevious,
|
|
|
|
ReedlineEvent::Edit(vec![EditCommand::Undo]),
|
|
|
|
]),
|
|
|
|
);
|
|
|
|
|
2022-03-27 13:01:04 +00:00
|
|
|
// Help menu keybinding
|
2022-02-28 13:54:40 +00:00
|
|
|
keybindings.add_binding(
|
2022-05-19 13:24:04 +00:00
|
|
|
KeyModifiers::NONE,
|
|
|
|
KeyCode::F(1),
|
2022-03-27 13:01:04 +00:00
|
|
|
ReedlineEvent::Menu("help_menu".to_string()),
|
2022-02-28 13:54:40 +00:00
|
|
|
);
|
|
|
|
}
|
|
|
|
|
2022-01-18 19:32:45 +00:00
|
|
|
pub enum KeybindingsMode {
|
|
|
|
Emacs(Keybindings),
|
|
|
|
Vi {
|
|
|
|
insert_keybindings: Keybindings,
|
|
|
|
normal_keybindings: Keybindings,
|
|
|
|
},
|
|
|
|
}
|
|
|
|
|
|
|
|
pub(crate) fn create_keybindings(config: &Config) -> Result<KeybindingsMode, ShellError> {
|
|
|
|
let parsed_keybindings = &config.keybindings;
|
|
|
|
|
2022-03-12 11:51:08 +00:00
|
|
|
let mut emacs_keybindings = default_emacs_keybindings();
|
|
|
|
let mut insert_keybindings = default_vi_insert_keybindings();
|
|
|
|
let mut normal_keybindings = default_vi_normal_keybindings();
|
|
|
|
|
2022-04-10 20:48:55 +00:00
|
|
|
match config.edit_mode.as_str() {
|
|
|
|
"emacs" => {
|
|
|
|
add_menu_keybindings(&mut emacs_keybindings);
|
|
|
|
}
|
|
|
|
_ => {
|
|
|
|
add_menu_keybindings(&mut insert_keybindings);
|
|
|
|
add_menu_keybindings(&mut normal_keybindings);
|
|
|
|
}
|
|
|
|
}
|
2022-03-12 11:51:08 +00:00
|
|
|
for keybinding in parsed_keybindings {
|
|
|
|
add_keybinding(
|
|
|
|
&keybinding.mode,
|
|
|
|
keybinding,
|
|
|
|
config,
|
|
|
|
&mut emacs_keybindings,
|
|
|
|
&mut insert_keybindings,
|
|
|
|
&mut normal_keybindings,
|
|
|
|
)?
|
|
|
|
}
|
2022-02-28 13:54:40 +00:00
|
|
|
|
2022-03-12 11:51:08 +00:00
|
|
|
match config.edit_mode.as_str() {
|
2022-04-10 20:48:55 +00:00
|
|
|
"emacs" => Ok(KeybindingsMode::Emacs(emacs_keybindings)),
|
|
|
|
_ => Ok(KeybindingsMode::Vi {
|
|
|
|
insert_keybindings,
|
|
|
|
normal_keybindings,
|
|
|
|
}),
|
2022-01-18 08:48:28 +00:00
|
|
|
}
|
2022-01-18 19:32:45 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
fn add_keybinding(
|
2022-03-12 11:51:08 +00:00
|
|
|
mode: &Value,
|
|
|
|
keybinding: &ParsedKeybinding,
|
|
|
|
config: &Config,
|
|
|
|
emacs_keybindings: &mut Keybindings,
|
|
|
|
insert_keybindings: &mut Keybindings,
|
|
|
|
normal_keybindings: &mut Keybindings,
|
|
|
|
) -> Result<(), ShellError> {
|
|
|
|
match &mode {
|
|
|
|
Value::String { val, span } => match val.as_str() {
|
|
|
|
"emacs" => add_parsed_keybinding(emacs_keybindings, keybinding, config),
|
|
|
|
"vi_insert" => add_parsed_keybinding(insert_keybindings, keybinding, config),
|
|
|
|
"vi_normal" => add_parsed_keybinding(normal_keybindings, keybinding, config),
|
|
|
|
m => Err(ShellError::UnsupportedConfigValue(
|
|
|
|
"emacs, vi_insert or vi_normal".to_string(),
|
|
|
|
m.to_string(),
|
|
|
|
*span,
|
|
|
|
)),
|
|
|
|
},
|
|
|
|
Value::List { vals, .. } => {
|
|
|
|
for inner_mode in vals {
|
|
|
|
add_keybinding(
|
|
|
|
inner_mode,
|
|
|
|
keybinding,
|
|
|
|
config,
|
|
|
|
emacs_keybindings,
|
|
|
|
insert_keybindings,
|
|
|
|
normal_keybindings,
|
|
|
|
)?
|
|
|
|
}
|
|
|
|
|
|
|
|
Ok(())
|
|
|
|
}
|
|
|
|
v => Err(ShellError::UnsupportedConfigValue(
|
|
|
|
"string or list of strings".to_string(),
|
|
|
|
v.into_abbreviated_string(config),
|
|
|
|
v.span()?,
|
|
|
|
)),
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
fn add_parsed_keybinding(
|
2022-01-18 19:32:45 +00:00
|
|
|
keybindings: &mut Keybindings,
|
2022-01-19 13:28:08 +00:00
|
|
|
keybinding: &ParsedKeybinding,
|
|
|
|
config: &Config,
|
2022-01-18 19:32:45 +00:00
|
|
|
) -> Result<(), ShellError> {
|
2022-01-20 12:20:00 +00:00
|
|
|
let modifier = match keybinding
|
|
|
|
.modifier
|
|
|
|
.into_string("", config)
|
|
|
|
.to_lowercase()
|
|
|
|
.as_str()
|
|
|
|
{
|
|
|
|
"control" => KeyModifiers::CONTROL,
|
|
|
|
"shift" => KeyModifiers::SHIFT,
|
|
|
|
"alt" => KeyModifiers::ALT,
|
|
|
|
"none" => KeyModifiers::NONE,
|
|
|
|
"control | shift" => KeyModifiers::CONTROL | KeyModifiers::SHIFT,
|
|
|
|
"control | alt" => KeyModifiers::CONTROL | KeyModifiers::ALT,
|
|
|
|
"control | alt | shift" => KeyModifiers::CONTROL | KeyModifiers::ALT | KeyModifiers::SHIFT,
|
2022-01-18 19:32:45 +00:00
|
|
|
_ => {
|
|
|
|
return Err(ShellError::UnsupportedConfigValue(
|
|
|
|
"CONTROL, SHIFT, ALT or NONE".to_string(),
|
2022-01-28 18:14:51 +00:00
|
|
|
keybinding.modifier.into_abbreviated_string(config),
|
2022-01-19 13:28:08 +00:00
|
|
|
keybinding.modifier.span()?,
|
2022-01-18 19:32:45 +00:00
|
|
|
))
|
|
|
|
}
|
|
|
|
};
|
|
|
|
|
2022-01-20 12:20:00 +00:00
|
|
|
let keycode = match keybinding
|
|
|
|
.keycode
|
|
|
|
.into_string("", config)
|
|
|
|
.to_lowercase()
|
|
|
|
.as_str()
|
|
|
|
{
|
|
|
|
"backspace" => KeyCode::Backspace,
|
|
|
|
"enter" => KeyCode::Enter,
|
|
|
|
c if c.starts_with("char_") => {
|
2022-01-28 18:14:51 +00:00
|
|
|
let mut char_iter = c.chars().skip(5);
|
|
|
|
let pos1 = char_iter.next();
|
|
|
|
let pos2 = char_iter.next();
|
|
|
|
|
|
|
|
let char = match (pos1, pos2) {
|
|
|
|
(Some(char), None) => Ok(char),
|
|
|
|
_ => Err(ShellError::UnsupportedConfigValue(
|
|
|
|
"char_<CHAR: unicode codepoint>".to_string(),
|
2022-01-19 13:28:08 +00:00
|
|
|
c.to_string(),
|
|
|
|
keybinding.keycode.span()?,
|
2022-01-28 18:14:51 +00:00
|
|
|
)),
|
|
|
|
}?;
|
|
|
|
|
2022-01-18 19:32:45 +00:00
|
|
|
KeyCode::Char(char)
|
|
|
|
}
|
2022-09-20 11:04:35 +00:00
|
|
|
"space" => KeyCode::Char(' '),
|
2022-01-18 19:32:45 +00:00
|
|
|
"down" => KeyCode::Down,
|
|
|
|
"up" => KeyCode::Up,
|
|
|
|
"left" => KeyCode::Left,
|
|
|
|
"right" => KeyCode::Right,
|
2022-01-20 12:20:00 +00:00
|
|
|
"home" => KeyCode::Home,
|
|
|
|
"end" => KeyCode::End,
|
|
|
|
"pageup" => KeyCode::PageUp,
|
|
|
|
"pagedown" => KeyCode::PageDown,
|
|
|
|
"tab" => KeyCode::Tab,
|
|
|
|
"backtab" => KeyCode::BackTab,
|
|
|
|
"delete" => KeyCode::Delete,
|
|
|
|
"insert" => KeyCode::Insert,
|
2022-01-28 18:14:51 +00:00
|
|
|
c if c.starts_with('f') => {
|
|
|
|
let fn_num: u8 = c[1..]
|
|
|
|
.parse()
|
|
|
|
.ok()
|
|
|
|
.filter(|num| matches!(num, 1..=12))
|
|
|
|
.ok_or(ShellError::UnsupportedConfigValue(
|
|
|
|
"(f1|f2|...|f12)".to_string(),
|
|
|
|
format!("unknown function key: {}", c),
|
|
|
|
keybinding.keycode.span()?,
|
|
|
|
))?;
|
|
|
|
KeyCode::F(fn_num)
|
|
|
|
}
|
2022-01-20 12:20:00 +00:00
|
|
|
"null" => KeyCode::Null,
|
|
|
|
"esc" | "escape" => KeyCode::Esc,
|
2022-01-18 19:32:45 +00:00
|
|
|
_ => {
|
|
|
|
return Err(ShellError::UnsupportedConfigValue(
|
|
|
|
"crossterm KeyCode".to_string(),
|
2022-01-28 18:14:51 +00:00
|
|
|
keybinding.keycode.into_abbreviated_string(config),
|
2022-01-19 13:28:08 +00:00
|
|
|
keybinding.keycode.span()?,
|
2022-01-18 19:32:45 +00:00
|
|
|
))
|
|
|
|
}
|
|
|
|
};
|
2022-04-10 21:54:09 +00:00
|
|
|
if let Some(event) = parse_event(&keybinding.event, config)? {
|
|
|
|
keybindings.add_binding(modifier, keycode, event);
|
|
|
|
} else {
|
|
|
|
keybindings.remove_binding(modifier, keycode);
|
|
|
|
}
|
2022-01-18 08:48:28 +00:00
|
|
|
|
2022-01-18 19:32:45 +00:00
|
|
|
Ok(())
|
2022-01-18 08:48:28 +00:00
|
|
|
}
|
2022-01-19 13:28:08 +00:00
|
|
|
|
2022-02-19 01:00:23 +00:00
|
|
|
enum EventType<'config> {
|
|
|
|
Send(&'config Value),
|
|
|
|
Edit(&'config Value),
|
|
|
|
Until(&'config Value),
|
|
|
|
}
|
|
|
|
|
|
|
|
impl<'config> EventType<'config> {
|
|
|
|
fn try_from_columns(
|
|
|
|
cols: &'config [String],
|
|
|
|
vals: &'config [Value],
|
|
|
|
span: &'config Span,
|
|
|
|
) -> Result<Self, ShellError> {
|
|
|
|
extract_value("send", cols, vals, span)
|
|
|
|
.map(Self::Send)
|
|
|
|
.or_else(|_| extract_value("edit", cols, vals, span).map(Self::Edit))
|
|
|
|
.or_else(|_| extract_value("until", cols, vals, span).map(Self::Until))
|
|
|
|
.map_err(|_| ShellError::MissingConfigValue("send, edit or until".to_string(), *span))
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-04-10 21:54:09 +00:00
|
|
|
fn parse_event(value: &Value, config: &Config) -> Result<Option<ReedlineEvent>, ShellError> {
|
2022-01-19 13:28:08 +00:00
|
|
|
match value {
|
|
|
|
Value::Record { cols, vals, span } => {
|
2022-02-19 01:00:23 +00:00
|
|
|
match EventType::try_from_columns(cols, vals, span)? {
|
|
|
|
EventType::Send(value) => event_from_record(
|
|
|
|
value.into_string("", config).to_lowercase().as_str(),
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
config,
|
|
|
|
span,
|
2022-04-10 21:54:09 +00:00
|
|
|
)
|
|
|
|
.map(Some),
|
2022-02-19 01:00:23 +00:00
|
|
|
EventType::Edit(value) => {
|
2022-03-13 20:05:13 +00:00
|
|
|
let edit = edit_from_record(
|
|
|
|
value.into_string("", config).to_lowercase().as_str(),
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
config,
|
|
|
|
span,
|
|
|
|
)?;
|
2022-04-10 21:54:09 +00:00
|
|
|
Ok(Some(ReedlineEvent::Edit(vec![edit])))
|
2022-02-19 01:00:23 +00:00
|
|
|
}
|
|
|
|
EventType::Until(value) => match value {
|
|
|
|
Value::List { vals, .. } => {
|
|
|
|
let events = vals
|
|
|
|
.iter()
|
2022-04-10 21:54:09 +00:00
|
|
|
.map(|value| match parse_event(value, config) {
|
|
|
|
Ok(inner) => match inner {
|
|
|
|
None => Err(ShellError::UnsupportedConfigValue(
|
|
|
|
"List containing valid events".to_string(),
|
|
|
|
"Nothing value (null)".to_string(),
|
|
|
|
value.span()?,
|
|
|
|
)),
|
|
|
|
Some(event) => Ok(event),
|
|
|
|
},
|
|
|
|
Err(e) => Err(e),
|
|
|
|
})
|
2022-02-19 01:00:23 +00:00
|
|
|
.collect::<Result<Vec<ReedlineEvent>, ShellError>>()?;
|
|
|
|
|
2022-04-10 21:54:09 +00:00
|
|
|
Ok(Some(ReedlineEvent::UntilFound(events)))
|
2022-01-19 13:28:08 +00:00
|
|
|
}
|
2022-02-19 01:00:23 +00:00
|
|
|
v => Err(ShellError::UnsupportedConfigValue(
|
|
|
|
"list of events".to_string(),
|
|
|
|
v.into_abbreviated_string(config),
|
|
|
|
v.span()?,
|
|
|
|
)),
|
2022-01-19 13:28:08 +00:00
|
|
|
},
|
2022-02-19 01:00:23 +00:00
|
|
|
}
|
2022-01-19 13:28:08 +00:00
|
|
|
}
|
|
|
|
Value::List { vals, .. } => {
|
|
|
|
let events = vals
|
2022-02-19 01:00:23 +00:00
|
|
|
.iter()
|
2022-04-10 21:54:09 +00:00
|
|
|
.map(|value| match parse_event(value, config) {
|
|
|
|
Ok(inner) => match inner {
|
|
|
|
None => Err(ShellError::UnsupportedConfigValue(
|
|
|
|
"List containing valid events".to_string(),
|
|
|
|
"Nothing value (null)".to_string(),
|
|
|
|
value.span()?,
|
|
|
|
)),
|
|
|
|
Some(event) => Ok(event),
|
|
|
|
},
|
|
|
|
Err(e) => Err(e),
|
|
|
|
})
|
2022-01-19 13:28:08 +00:00
|
|
|
.collect::<Result<Vec<ReedlineEvent>, ShellError>>()?;
|
|
|
|
|
2022-04-10 21:54:09 +00:00
|
|
|
Ok(Some(ReedlineEvent::Multiple(events)))
|
2022-01-19 13:28:08 +00:00
|
|
|
}
|
2022-04-10 21:54:09 +00:00
|
|
|
Value::Nothing { .. } => Ok(None),
|
2022-01-19 13:28:08 +00:00
|
|
|
v => Err(ShellError::UnsupportedConfigValue(
|
2022-04-10 21:54:09 +00:00
|
|
|
"record or list of records, null to unbind key".to_string(),
|
2022-01-28 18:14:51 +00:00
|
|
|
v.into_abbreviated_string(config),
|
2022-01-19 13:28:08 +00:00
|
|
|
v.span()?,
|
|
|
|
)),
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2022-02-19 01:00:23 +00:00
|
|
|
fn event_from_record(
|
|
|
|
name: &str,
|
|
|
|
cols: &[String],
|
|
|
|
vals: &[Value],
|
|
|
|
config: &Config,
|
|
|
|
span: &Span,
|
|
|
|
) -> Result<ReedlineEvent, ShellError> {
|
2022-03-13 20:05:13 +00:00
|
|
|
let event = match name {
|
|
|
|
"none" => ReedlineEvent::None,
|
|
|
|
"clearscreen" => ReedlineEvent::ClearScreen,
|
2022-04-20 09:10:33 +00:00
|
|
|
"clearscrollback" => ReedlineEvent::ClearScrollback,
|
2022-03-13 20:05:13 +00:00
|
|
|
"historyhintcomplete" => ReedlineEvent::HistoryHintComplete,
|
|
|
|
"historyhintwordcomplete" => ReedlineEvent::HistoryHintWordComplete,
|
|
|
|
"ctrld" => ReedlineEvent::CtrlD,
|
|
|
|
"ctrlc" => ReedlineEvent::CtrlC,
|
|
|
|
"enter" => ReedlineEvent::Enter,
|
2022-10-16 21:51:15 +00:00
|
|
|
"submit" => ReedlineEvent::Submit,
|
|
|
|
"submitornewline" => ReedlineEvent::SubmitOrNewline,
|
2022-03-13 20:05:13 +00:00
|
|
|
"esc" | "escape" => ReedlineEvent::Esc,
|
|
|
|
"up" => ReedlineEvent::Up,
|
|
|
|
"down" => ReedlineEvent::Down,
|
|
|
|
"right" => ReedlineEvent::Right,
|
|
|
|
"left" => ReedlineEvent::Left,
|
|
|
|
"searchhistory" => ReedlineEvent::SearchHistory,
|
|
|
|
"nexthistory" => ReedlineEvent::NextHistory,
|
|
|
|
"previoushistory" => ReedlineEvent::PreviousHistory,
|
|
|
|
"repaint" => ReedlineEvent::Repaint,
|
|
|
|
"menudown" => ReedlineEvent::MenuDown,
|
|
|
|
"menuup" => ReedlineEvent::MenuUp,
|
|
|
|
"menuleft" => ReedlineEvent::MenuLeft,
|
|
|
|
"menuright" => ReedlineEvent::MenuRight,
|
|
|
|
"menunext" => ReedlineEvent::MenuNext,
|
|
|
|
"menuprevious" => ReedlineEvent::MenuPrevious,
|
|
|
|
"menupagenext" => ReedlineEvent::MenuPageNext,
|
|
|
|
"menupageprevious" => ReedlineEvent::MenuPagePrevious,
|
2022-05-03 19:52:53 +00:00
|
|
|
"openeditor" => ReedlineEvent::OpenEditor,
|
2022-02-19 01:00:23 +00:00
|
|
|
"menu" => {
|
|
|
|
let menu = extract_value("name", cols, vals, span)?;
|
2022-03-13 20:05:13 +00:00
|
|
|
ReedlineEvent::Menu(menu.into_string("", config))
|
2022-02-19 01:00:23 +00:00
|
|
|
}
|
2022-03-12 11:51:08 +00:00
|
|
|
"executehostcommand" => {
|
|
|
|
let cmd = extract_value("cmd", cols, vals, span)?;
|
2022-03-13 20:05:13 +00:00
|
|
|
ReedlineEvent::ExecuteHostCommand(cmd.into_string("", config))
|
|
|
|
}
|
|
|
|
v => {
|
|
|
|
return Err(ShellError::UnsupportedConfigValue(
|
|
|
|
"Reedline event".to_string(),
|
|
|
|
v.to_string(),
|
|
|
|
*span,
|
2022-03-12 11:51:08 +00:00
|
|
|
))
|
2022-02-28 13:54:40 +00:00
|
|
|
}
|
2022-03-13 20:05:13 +00:00
|
|
|
};
|
|
|
|
|
|
|
|
Ok(event)
|
2022-02-19 01:00:23 +00:00
|
|
|
}
|
|
|
|
|
2022-03-13 20:05:13 +00:00
|
|
|
fn edit_from_record(
|
|
|
|
name: &str,
|
|
|
|
cols: &[String],
|
|
|
|
vals: &[Value],
|
|
|
|
config: &Config,
|
|
|
|
span: &Span,
|
|
|
|
) -> Result<EditCommand, ShellError> {
|
|
|
|
let edit = match name {
|
|
|
|
"movetostart" => EditCommand::MoveToStart,
|
|
|
|
"movetolinestart" => EditCommand::MoveToLineStart,
|
|
|
|
"movetoend" => EditCommand::MoveToEnd,
|
|
|
|
"movetolineend" => EditCommand::MoveToLineEnd,
|
|
|
|
"moveleft" => EditCommand::MoveLeft,
|
|
|
|
"moveright" => EditCommand::MoveRight,
|
|
|
|
"movewordleft" => EditCommand::MoveWordLeft,
|
2022-06-21 17:22:11 +00:00
|
|
|
"movebigwordleft" => EditCommand::MoveBigWordLeft,
|
2022-03-13 20:05:13 +00:00
|
|
|
"movewordright" => EditCommand::MoveWordRight,
|
2022-06-21 17:22:11 +00:00
|
|
|
"movewordrightend" => EditCommand::MoveWordRightEnd,
|
|
|
|
"movebigwordrightend" => EditCommand::MoveBigWordRightEnd,
|
|
|
|
"movewordrightstart" => EditCommand::MoveWordRightStart,
|
|
|
|
"movebigwordrightstart" => EditCommand::MoveBigWordRightStart,
|
|
|
|
"movetoposition" => {
|
|
|
|
let value = extract_value("value", cols, vals, span)?;
|
|
|
|
EditCommand::MoveToPosition(value.as_integer()? as usize)
|
|
|
|
}
|
2022-03-13 20:05:13 +00:00
|
|
|
"insertchar" => {
|
|
|
|
let value = extract_value("value", cols, vals, span)?;
|
|
|
|
let char = extract_char(value, config)?;
|
|
|
|
EditCommand::InsertChar(char)
|
|
|
|
}
|
|
|
|
"insertstring" => {
|
|
|
|
let value = extract_value("value", cols, vals, span)?;
|
|
|
|
EditCommand::InsertString(value.into_string("", config))
|
|
|
|
}
|
2022-04-20 09:10:33 +00:00
|
|
|
"insertnewline" => EditCommand::InsertNewline,
|
2022-03-13 20:05:13 +00:00
|
|
|
"backspace" => EditCommand::Backspace,
|
|
|
|
"delete" => EditCommand::Delete,
|
2022-06-21 17:22:11 +00:00
|
|
|
"cutchar" => EditCommand::CutChar,
|
2022-03-13 20:05:13 +00:00
|
|
|
"backspaceword" => EditCommand::BackspaceWord,
|
|
|
|
"deleteword" => EditCommand::DeleteWord,
|
|
|
|
"clear" => EditCommand::Clear,
|
|
|
|
"cleartolineend" => EditCommand::ClearToLineEnd,
|
|
|
|
"cutcurrentline" => EditCommand::CutCurrentLine,
|
|
|
|
"cutfromstart" => EditCommand::CutFromStart,
|
|
|
|
"cutfromlinestart" => EditCommand::CutFromLineStart,
|
|
|
|
"cuttoend" => EditCommand::CutToEnd,
|
|
|
|
"cuttolineend" => EditCommand::CutToLineEnd,
|
|
|
|
"cutwordleft" => EditCommand::CutWordLeft,
|
2022-06-21 17:22:11 +00:00
|
|
|
"cutbigwordleft" => EditCommand::CutBigWordLeft,
|
2022-03-13 20:05:13 +00:00
|
|
|
"cutwordright" => EditCommand::CutWordRight,
|
2022-06-21 17:22:11 +00:00
|
|
|
"cutbigwordright" => EditCommand::CutBigWordRight,
|
|
|
|
"cutwordrighttonext" => EditCommand::CutWordRightToNext,
|
|
|
|
"cutbigwordrighttonext" => EditCommand::CutBigWordRightToNext,
|
2022-03-13 20:05:13 +00:00
|
|
|
"pastecutbufferbefore" => EditCommand::PasteCutBufferBefore,
|
|
|
|
"pastecutbufferafter" => EditCommand::PasteCutBufferAfter,
|
|
|
|
"uppercaseword" => EditCommand::UppercaseWord,
|
|
|
|
"lowercaseword" => EditCommand::LowercaseWord,
|
|
|
|
"capitalizechar" => EditCommand::CapitalizeChar,
|
|
|
|
"swapwords" => EditCommand::SwapWords,
|
|
|
|
"swapgraphemes" => EditCommand::SwapGraphemes,
|
|
|
|
"undo" => EditCommand::Undo,
|
|
|
|
"redo" => EditCommand::Redo,
|
|
|
|
"cutrightuntil" => {
|
|
|
|
let value = extract_value("value", cols, vals, span)?;
|
|
|
|
let char = extract_char(value, config)?;
|
|
|
|
EditCommand::CutRightUntil(char)
|
|
|
|
}
|
|
|
|
"cutrightbefore" => {
|
|
|
|
let value = extract_value("value", cols, vals, span)?;
|
|
|
|
let char = extract_char(value, config)?;
|
|
|
|
EditCommand::CutRightBefore(char)
|
|
|
|
}
|
|
|
|
"moverightuntil" => {
|
|
|
|
let value = extract_value("value", cols, vals, span)?;
|
|
|
|
let char = extract_char(value, config)?;
|
|
|
|
EditCommand::MoveRightUntil(char)
|
|
|
|
}
|
|
|
|
"moverightbefore" => {
|
|
|
|
let value = extract_value("value", cols, vals, span)?;
|
|
|
|
let char = extract_char(value, config)?;
|
|
|
|
EditCommand::MoveRightBefore(char)
|
|
|
|
}
|
|
|
|
"cutleftuntil" => {
|
|
|
|
let value = extract_value("value", cols, vals, span)?;
|
|
|
|
let char = extract_char(value, config)?;
|
|
|
|
EditCommand::CutLeftUntil(char)
|
|
|
|
}
|
|
|
|
"cutleftbefore" => {
|
|
|
|
let value = extract_value("value", cols, vals, span)?;
|
|
|
|
let char = extract_char(value, config)?;
|
|
|
|
EditCommand::CutLeftBefore(char)
|
|
|
|
}
|
|
|
|
"moveleftuntil" => {
|
|
|
|
let value = extract_value("value", cols, vals, span)?;
|
|
|
|
let char = extract_char(value, config)?;
|
|
|
|
EditCommand::MoveLeftUntil(char)
|
|
|
|
}
|
|
|
|
"moveleftbefore" => {
|
|
|
|
let value = extract_value("value", cols, vals, span)?;
|
|
|
|
let char = extract_char(value, config)?;
|
|
|
|
EditCommand::MoveLeftBefore(char)
|
2022-01-19 13:28:08 +00:00
|
|
|
}
|
2022-10-22 16:32:07 +00:00
|
|
|
"complete" => EditCommand::Complete,
|
2022-01-19 13:28:08 +00:00
|
|
|
e => {
|
|
|
|
return Err(ShellError::UnsupportedConfigValue(
|
2022-03-13 20:05:13 +00:00
|
|
|
"reedline EditCommand".to_string(),
|
|
|
|
e.to_string(),
|
|
|
|
*span,
|
2022-01-19 13:28:08 +00:00
|
|
|
))
|
|
|
|
}
|
|
|
|
};
|
|
|
|
|
|
|
|
Ok(edit)
|
|
|
|
}
|
|
|
|
|
2022-03-13 20:05:13 +00:00
|
|
|
fn extract_char(value: &Value, config: &Config) -> Result<char, ShellError> {
|
|
|
|
let span = value.span()?;
|
2022-01-19 13:28:08 +00:00
|
|
|
value
|
|
|
|
.into_string("", config)
|
|
|
|
.chars()
|
|
|
|
.next()
|
2022-03-13 20:05:13 +00:00
|
|
|
.ok_or_else(|| ShellError::MissingConfigValue("char to insert".to_string(), span))
|
2022-01-19 13:28:08 +00:00
|
|
|
}
|
2022-02-19 01:00:23 +00:00
|
|
|
|
|
|
|
#[cfg(test)]
|
|
|
|
mod test {
|
|
|
|
use super::*;
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn test_send_event() {
|
|
|
|
let cols = vec!["send".to_string()];
|
2022-12-24 09:25:38 +00:00
|
|
|
let vals = vec![Value::test_string("Enter")];
|
2022-02-19 01:00:23 +00:00
|
|
|
|
|
|
|
let span = Span::test_data();
|
|
|
|
let b = EventType::try_from_columns(&cols, &vals, &span).unwrap();
|
|
|
|
assert!(matches!(b, EventType::Send(_)));
|
|
|
|
|
|
|
|
let event = Value::Record {
|
|
|
|
vals,
|
|
|
|
cols,
|
|
|
|
span: Span::test_data(),
|
|
|
|
};
|
|
|
|
let config = Config::default();
|
|
|
|
|
|
|
|
let parsed_event = parse_event(&event, &config).unwrap();
|
2022-04-10 21:54:09 +00:00
|
|
|
assert_eq!(parsed_event, Some(ReedlineEvent::Enter));
|
2022-02-19 01:00:23 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn test_edit_event() {
|
|
|
|
let cols = vec!["edit".to_string()];
|
2022-12-24 09:25:38 +00:00
|
|
|
let vals = vec![Value::test_string("Clear")];
|
2022-02-19 01:00:23 +00:00
|
|
|
|
|
|
|
let span = Span::test_data();
|
|
|
|
let b = EventType::try_from_columns(&cols, &vals, &span).unwrap();
|
|
|
|
assert!(matches!(b, EventType::Edit(_)));
|
|
|
|
|
|
|
|
let event = Value::Record {
|
|
|
|
vals,
|
|
|
|
cols,
|
|
|
|
span: Span::test_data(),
|
|
|
|
};
|
|
|
|
let config = Config::default();
|
|
|
|
|
|
|
|
let parsed_event = parse_event(&event, &config).unwrap();
|
2022-04-10 21:54:09 +00:00
|
|
|
assert_eq!(
|
|
|
|
parsed_event,
|
|
|
|
Some(ReedlineEvent::Edit(vec![EditCommand::Clear]))
|
|
|
|
);
|
2022-02-19 01:00:23 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn test_send_menu() {
|
|
|
|
let cols = vec!["send".to_string(), "name".to_string()];
|
|
|
|
let vals = vec![
|
2022-12-24 09:25:38 +00:00
|
|
|
Value::test_string("Menu"),
|
|
|
|
Value::test_string("history_menu"),
|
2022-02-19 01:00:23 +00:00
|
|
|
];
|
|
|
|
|
|
|
|
let span = Span::test_data();
|
|
|
|
let b = EventType::try_from_columns(&cols, &vals, &span).unwrap();
|
|
|
|
assert!(matches!(b, EventType::Send(_)));
|
|
|
|
|
|
|
|
let event = Value::Record {
|
|
|
|
vals,
|
|
|
|
cols,
|
|
|
|
span: Span::test_data(),
|
|
|
|
};
|
|
|
|
let config = Config::default();
|
|
|
|
|
|
|
|
let parsed_event = parse_event(&event, &config).unwrap();
|
|
|
|
assert_eq!(
|
|
|
|
parsed_event,
|
2022-04-10 21:54:09 +00:00
|
|
|
Some(ReedlineEvent::Menu("history_menu".to_string()))
|
2022-02-19 01:00:23 +00:00
|
|
|
);
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn test_until_event() {
|
|
|
|
// Menu event
|
|
|
|
let cols = vec!["send".to_string(), "name".to_string()];
|
|
|
|
let vals = vec![
|
2022-12-24 09:25:38 +00:00
|
|
|
Value::test_string("Menu"),
|
|
|
|
Value::test_string("history_menu"),
|
2022-02-19 01:00:23 +00:00
|
|
|
];
|
|
|
|
|
|
|
|
let menu_event = Value::Record {
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
span: Span::test_data(),
|
|
|
|
};
|
|
|
|
|
|
|
|
// Enter event
|
|
|
|
let cols = vec!["send".to_string()];
|
2022-12-24 09:25:38 +00:00
|
|
|
let vals = vec![Value::test_string("Enter")];
|
2022-02-19 01:00:23 +00:00
|
|
|
|
|
|
|
let enter_event = Value::Record {
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
span: Span::test_data(),
|
|
|
|
};
|
|
|
|
|
|
|
|
// Until event
|
|
|
|
let cols = vec!["until".to_string()];
|
|
|
|
let vals = vec![Value::List {
|
|
|
|
vals: vec![menu_event, enter_event],
|
|
|
|
span: Span::test_data(),
|
|
|
|
}];
|
|
|
|
|
|
|
|
let span = Span::test_data();
|
|
|
|
let b = EventType::try_from_columns(&cols, &vals, &span).unwrap();
|
|
|
|
assert!(matches!(b, EventType::Until(_)));
|
|
|
|
|
|
|
|
let event = Value::Record {
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
span: Span::test_data(),
|
|
|
|
};
|
|
|
|
let config = Config::default();
|
|
|
|
|
|
|
|
let parsed_event = parse_event(&event, &config).unwrap();
|
|
|
|
assert_eq!(
|
|
|
|
parsed_event,
|
2022-04-10 21:54:09 +00:00
|
|
|
Some(ReedlineEvent::UntilFound(vec![
|
2022-02-19 01:00:23 +00:00
|
|
|
ReedlineEvent::Menu("history_menu".to_string()),
|
|
|
|
ReedlineEvent::Enter,
|
2022-04-10 21:54:09 +00:00
|
|
|
]))
|
2022-02-19 01:00:23 +00:00
|
|
|
);
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn test_multiple_event() {
|
|
|
|
// Menu event
|
|
|
|
let cols = vec!["send".to_string(), "name".to_string()];
|
|
|
|
let vals = vec![
|
2022-12-24 09:25:38 +00:00
|
|
|
Value::test_string("Menu"),
|
|
|
|
Value::test_string("history_menu"),
|
2022-02-19 01:00:23 +00:00
|
|
|
];
|
|
|
|
|
|
|
|
let menu_event = Value::Record {
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
span: Span::test_data(),
|
|
|
|
};
|
|
|
|
|
|
|
|
// Enter event
|
|
|
|
let cols = vec!["send".to_string()];
|
2022-12-24 09:25:38 +00:00
|
|
|
let vals = vec![Value::test_string("Enter")];
|
2022-02-19 01:00:23 +00:00
|
|
|
|
|
|
|
let enter_event = Value::Record {
|
|
|
|
cols,
|
|
|
|
vals,
|
|
|
|
span: Span::test_data(),
|
|
|
|
};
|
|
|
|
|
|
|
|
// Multiple event
|
|
|
|
let event = Value::List {
|
|
|
|
vals: vec![menu_event, enter_event],
|
|
|
|
span: Span::test_data(),
|
|
|
|
};
|
|
|
|
|
|
|
|
let config = Config::default();
|
|
|
|
let parsed_event = parse_event(&event, &config).unwrap();
|
|
|
|
assert_eq!(
|
|
|
|
parsed_event,
|
2022-04-10 21:54:09 +00:00
|
|
|
Some(ReedlineEvent::Multiple(vec![
|
2022-02-19 01:00:23 +00:00
|
|
|
ReedlineEvent::Menu("history_menu".to_string()),
|
|
|
|
ReedlineEvent::Enter,
|
2022-04-10 21:54:09 +00:00
|
|
|
]))
|
2022-02-19 01:00:23 +00:00
|
|
|
);
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn test_error() {
|
|
|
|
let cols = vec!["not_exist".to_string()];
|
2022-12-24 09:25:38 +00:00
|
|
|
let vals = vec![Value::test_string("Enter")];
|
2022-02-19 01:00:23 +00:00
|
|
|
|
|
|
|
let span = Span::test_data();
|
|
|
|
let b = EventType::try_from_columns(&cols, &vals, &span);
|
|
|
|
assert!(matches!(b, Err(ShellError::MissingConfigValue(_, _))));
|
|
|
|
}
|
|
|
|
}
|