.. | ||
frida-tutorial-1.md | ||
frida-tutorial-2.md | ||
objection-tutorial.md | ||
owaspuncrackable-1.md | ||
README.md |
Fridaチュートリアル
☁️ HackTricks Cloud ☁️ -🐦 Twitter 🐦 - 🎙️ Twitch 🎙️ - 🎥 Youtube 🎥
- サイバーセキュリティ企業で働いていますか? HackTricksで会社を宣伝したいですか?または、PEASSの最新バージョンにアクセスしたり、HackTricksをPDFでダウンロードしたいですか?SUBSCRIPTION PLANSをチェックしてください!
- The PEASS Familyを見つけてください。独占的なNFTのコレクションです。
- 公式のPEASS&HackTricksグッズを手に入れましょう。
- 💬 Discordグループまたはtelegramグループに参加するか、Twitterでフォローしてください🐦@carlospolopm。
- ハッキングのトリックを共有するには、hacktricksリポジトリとhacktricks-cloudリポジトリにPRを提出してください。
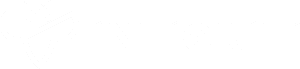
バグバウンティのヒント:ハッカーによって作成されたプレミアムなバグバウンティプラットフォームであるIntigritiにサインアップしてください!今すぐhttps://go.intigriti.com/hacktricksに参加して、最大**$100,000**のバウンティを獲得しましょう!
{% embed url="https://go.intigriti.com/hacktricks" %}
インストール
fridaツールをインストールします:
pip install frida-tools
pip install frida
frida serverをAndroidにダウンロードしてインストールします(最新リリースをダウンロード)。
ルートモードでadbを再起動し、接続し、frida-serverをアップロードし、実行権限を与えてバックグラウンドで実行するためのワンライナー:
adb root; adb connect localhost:6000; sleep 1; adb push frida-server /data/local/tmp/; adb shell "chmod 755 /data/local/tmp/frida-server"; adb shell "/data/local/tmp/frida-server &"
動作を確認する:
frida-ps -U #List packages and processes
frida-ps -U | grep -i <part_of_the_package_name> #Get all the package name
チュートリアル
チュートリアル 1
元記事: https://medium.com/infosec-adventures/introduction-to-frida-5a3f51595ca1
APK: https://github.com/t0thkr1s/frida-demo/releases
ソースコード: https://github.com/t0thkr1s/frida-demo
チュートリアル 2
元記事: https://11x256.github.io/Frida-hooking-android-part-2/ (パート 2, 3, 4)
APK とソースコード: https://github.com/11x256/frida-android-examples
チュートリアル 3
元記事: https://joshspicer.com/android-frida-1
APK: https://github.com/OWASP/owasp-mstg/blob/master/Crackmes/Android/Level_01/UnCrackable-Level1.apk
リンクをクリックして読む。
ここで素晴らしい Frida スクリプトを見つけることができます: https://codeshare.frida.re/
早い例
ここでは、Fridaの基本的で興味深い機能を使ったクイックスクリプトを見つけることができます。
コマンドラインからFridaを呼び出す
frida-ps -U
#Basic frida hooking
frida -l disableRoot.js -f owasp.mstg.uncrackable1
#Hooking before starting the app
frida -U --no-pause -l disableRoot.js -f owasp.mstg.uncrackable1
#The --no-pause and -f options allow the app to be spawned automatically,
#frozen so that the instrumentation can occur, and the automatically
#continue execution with our modified code.
基本的なPythonスクリプト
import frida
# Attach to the target process
session = frida.attach("com.example.app")
# Define the JavaScript code to be injected
js_code = """
Java.perform(function () {
// Hook the target method
var targetClass = Java.use("com.example.app.TargetClass");
targetClass.targetMethod.implementation = function () {
// Modify the behavior of the target method
console.log("Target method hooked!");
// Call the original method
this.targetMethod();
};
});
"""
# Create a script from the JavaScript code
script = session.create_script(js_code)
# Load the script into the target process
script.load()
# Detach from the target process
session.detach()
このPythonスクリプトは、Fridaを使用してターゲットプロセスにアタッチし、JavaScriptコードを注入するための基本的なスクリプトです。
import frida
# ターゲットプロセスにアタッチする
session = frida.attach("com.example.app")
# 注入するJavaScriptコードを定義する
js_code = """
Java.perform(function () {
// ターゲットメソッドをフックする
var targetClass = Java.use("com.example.app.TargetClass");
targetClass.targetMethod.implementation = function () {
// ターゲットメソッドの動作を変更する
console.log("ターゲットメソッドがフックされました!");
// オリジナルのメソッドを呼び出す
this.targetMethod();
};
});
"""
# JavaScriptコードからスクリプトを作成する
script = session.create_script(js_code)
# スクリプトをターゲットプロセスにロードする
script.load()
# ターゲットプロセスからデタッチする
session.detach()
このスクリプトでは、Fridaを使用してAndroidアプリのターゲットプロセスにアタッチし、指定したクラスのメソッドをフックして動作を変更します。フックしたメソッド内で、コンソールにメッセージを出力し、オリジナルのメソッドを呼び出します。
import frida, sys
jscode = open(sys.argv[0]).read()
process = frida.get_usb_device().attach('infosecadventures.fridademo')
script = process.create_script(jscode)
print('[ * ] Running Frida Demo application')
script.load()
sys.stdin.read()
パラメータのない関数のフック
クラス sg.vantagepoint.a.c
の関数 a()
をフックします。
Java.perform(function () {
; rootcheck1.a.overload().implementation = function() {
rootcheck1.a.overload().implementation = function() {
send("sg.vantagepoint.a.c.a()Z Root check 1 HIT! su.exists()");
return false;
};
});
Frida Tutorial: Hooking exit()
in Java
In this tutorial, we will learn how to use Frida to hook the exit()
function in Java. By hooking this function, we can intercept the program's exit and perform additional actions before the application terminates.
Prerequisites
Before we begin, make sure you have the following:
- A rooted Android device or an emulator
- Frida installed on your machine
- Basic knowledge of JavaScript and Java
Steps
-
Start by creating a new JavaScript file, for example
hook_exit.js
, and open it in a text editor. -
Import the necessary Frida modules:
const { Java } = require('frida');
- Attach to the target process using Frida:
Java.perform(function () {
// Code goes here
});
- Find the class and method that contains the
exit()
function. You can use theJava.use()
function to obtain a reference to the class:
const System = Java.use('java.lang.System');
- Hook the
exit()
method using theSystem.exit.implementation
property:
System.exit.implementation = function (code) {
// Code goes here
this.exit(code);
};
- Inside the hook, you can add your custom logic. For example, you can log the exit code before the application terminates:
System.exit.implementation = function (code) {
console.log('Exit code:', code);
this.exit(code);
};
-
Save the JavaScript file.
-
Launch the target application on your Android device or emulator.
-
Use Frida to inject the JavaScript code into the target process:
frida -U -l hook_exit.js -f <package_name>
Replace <package_name>
with the package name of the target application.
- You should see the exit code being logged in the console whenever the
exit()
function is called.
Conclusion
By hooking the exit()
function in Java using Frida, we can intercept the program's exit and perform additional actions. This technique can be useful for debugging or analyzing the behavior of an application during its termination process.
var sysexit = Java.use("java.lang.System");
sysexit.exit.overload("int").implementation = function(var_0) {
send("java.lang.System.exit(I)V // We avoid exiting the application :)");
};
Frida Tutorial: メインアクティビティの .onStart()
と .onCreate()
をフックする
このチュートリアルでは、Fridaを使用してAndroidアプリのメインアクティビティの .onStart()
と .onCreate()
メソッドをフックする方法を学びます。
前提条件
- Fridaがインストールされていること
- ターゲットとなるAndroidデバイスが接続されていること
ステップ1: Fridaスクリプトの作成
まず、Fridaスクリプトを作成します。以下のコードを使用して、メインアクティビティの .onStart()
と .onCreate()
メソッドをフックするスクリプトを作成します。
Java.perform(function() {
var MainActivity = Java.use('com.example.MainActivity');
MainActivity.onStart.implementation = function() {
console.log('MainActivity.onStart() メソッドが呼び出されました');
this.onStart();
};
MainActivity.onCreate.implementation = function() {
console.log('MainActivity.onCreate() メソッドが呼び出されました');
this.onCreate();
};
});
ステップ2: Fridaスクリプトの実行
次に、作成したFridaスクリプトを実行します。以下のコマンドを使用して、スクリプトを実行します。
frida -U -l script.js -f com.example.app
-U
フラグは、USB接続されたデバイスを使用することを指定します。-l
フラグは、実行するFridaスクリプトのパスを指定します。-f
フラグは、ターゲットとなるアプリのパッケージ名を指定します。
ステップ3: フックされたメソッドの確認
Fridaスクリプトが正常に実行されると、メインアクティビティの .onStart()
と .onCreate()
メソッドがフックされ、ログにメッセージが表示されます。
これで、Androidアプリのメインアクティビティの .onStart()
と .onCreate()
メソッドをフックすることができました。
var mainactivity = Java.use("sg.vantagepoint.uncrackable1.MainActivity");
mainactivity.onStart.overload().implementation = function() {
send("MainActivity.onStart() HIT!!!");
var ret = this.onStart.overload().call(this);
};
mainactivity.onCreate.overload("android.os.Bundle").implementation = function(var_0) {
send("MainActivity.onCreate() HIT!!!");
var ret = this.onCreate.overload("android.os.Bundle").call(this,var_0);
};
Frida Tutorial: フック Android の .onCreate()
このチュートリアルでは、Frida を使用して Android アプリの .onCreate()
メソッドをフックする方法について説明します。
1. Frida のセットアップ
まず、Frida をセットアップする必要があります。Frida をインストールするには、以下のコマンドを実行します。
$ pip install frida-tools
2. デバイスへの接続
Frida を使用するためには、デバイスに接続する必要があります。以下のコマンドを使用して、デバイスに接続します。
$ adb devices
3. フックの実装
Frida を使用して .onCreate()
メソッドをフックするために、以下のスクリプトを作成します。
Java.perform(function() {
var MainActivity = Java.use('com.example.MainActivity');
MainActivity.onCreate.implementation = function() {
console.log('onCreate() メソッドがフックされました!');
// ここにフック後の処理を追加します
// 元のメソッドを呼び出します
this.onCreate();
};
});
4. フックの実行
作成したスクリプトを実行するために、以下のコマンドを使用します。
$ frida -U -f com.example.app -l script.js --no-pause
com.example.app
の部分をターゲットアプリのパッケージ名に置き換えてください。script.js
の部分を作成したスクリプトのファイル名に置き換えてください。
5. 結果の確認
スクリプトが正常に実行されると、.onCreate()
メソッドがフックされたことを示すメッセージが表示されます。フック後の処理を追加することで、必要な操作を実行することができます。
以上で、Android アプリの .onCreate()
メソッドをフックする方法が完了です。
var activity = Java.use("android.app.Activity");
activity.onCreate.overload("android.os.Bundle").implementation = function(var_0) {
send("Activity HIT!!!");
var ret = this.onCreate.overload("android.os.Bundle").call(this,var_0);
};
パラメータを持つ関数のフックと値の取得
復号化関数のフック。入力を表示し、元の関数を呼び出して入力を復号化し、最後に平文データを表示します。
function getString(data){
var ret = "";
for (var i=0; i < data.length; i++){
ret += data[i].toString();
}
return ret
}
var aes_decrypt = Java.use("sg.vantagepoint.a.a");
aes_decrypt.a.overload("[B","[B").implementation = function(var_0,var_1) {
send("sg.vantagepoint.a.a.a([B[B)[B doFinal(enc) // AES/ECB/PKCS7Padding");
send("Key : " + getString(var_0));
send("Encrypted : " + getString(var_1));
var ret = this.a.overload("[B","[B").call(this,var_0,var_1);
send("Decrypted : " + ret);
var flag = "";
for (var i=0; i < ret.length; i++){
flag += String.fromCharCode(ret[i]);
}
send("Decrypted flag: " + flag);
return ret; //[B
};
ファンクションのフックと入力を使用した呼び出し
関数をフックして、文字列を受け取り、他の文字列で呼び出します(こちらから)。
var string_class = Java.use("java.lang.String"); // get a JS wrapper for java's String class
my_class.fun.overload("java.lang.String").implementation = function(x){ //hooking the new function
var my_string = string_class.$new("My TeSt String#####"); //creating a new String by using `new` operator
console.log("Original arg: " +x );
var ret = this.fun(my_string); // calling the original function with the new String, and putting its return value in ret variable
console.log("Return value: "+ret);
return ret;
};
クラスの既存オブジェクトの取得
作成済みのオブジェクトの属性を抽出したい場合は、これを使用できます。
この例では、クラスmy_activityのオブジェクトを取得し、オブジェクトのプライベート属性を表示する関数.secret()を呼び出す方法を示します。
Java.choose("com.example.a11x256.frida_test.my_activity" , {
onMatch : function(instance){ //This function will be called for every instance found by frida
console.log("Found instance: "+instance);
console.log("Result of secret func: " + instance.secret());
},
onComplete:function(){}
});
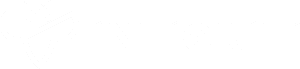
バグバウンティのヒント: Intigritiにサインアップしてください。これはハッカーによって作成されたプレミアムなバグバウンティプラットフォームです!今すぐhttps://go.intigriti.com/hacktricksに参加して、最大**$100,000**の報酬を獲得しましょう!
{% embed url="https://go.intigriti.com/hacktricks" %}
☁️ HackTricks Cloud ☁️ -🐦 Twitter 🐦 - 🎙️ Twitch 🎙️ - 🎥 Youtube 🎥
- サイバーセキュリティ企業で働いていますか? HackTricksで会社を宣伝したいですか?または、最新バージョンのPEASSを入手したり、HackTricksをPDFでダウンロードしたいですか?SUBSCRIPTION PLANSをチェックしてください!
- The PEASS Familyを見つけてください。これは私たちの独占的なNFTのコレクションです。
- 公式のPEASS&HackTricksグッズを手に入れましょう。
- 💬 Discordグループまたはtelegramグループに参加するか、Twitterで私をフォローしてください🐦@carlospolopm。
- ハッキングのトリックを共有するには、hacktricksリポジトリとhacktricks-cloudリポジトリにPRを提出してください。