mirror of
https://github.com/bevyengine/bevy
synced 2025-02-19 15:38:36 +00:00
# Objective Users should be able to configure depth load operations on cameras. Currently every camera clears depth when it is rendered. But sometimes later passes need to rely on depth from previous passes. ## Solution This adds the `Camera3d::depth_load_op` field with a new `Camera3dDepthLoadOp` value. This is a custom type because Camera3d uses "reverse-z depth" and this helps us record and document that in a discoverable way. It also gives us more control over reflection + other trait impls, whereas `LoadOp` is owned by the `wgpu` crate. ```rust commands.spawn_bundle(Camera3dBundle { camera_3d: Camera3d { depth_load_op: Camera3dDepthLoadOp::Load, ..default() }, ..default() }); ``` ### two_passes example with the "second pass" camera configured to the default (clear depth to 0.0) 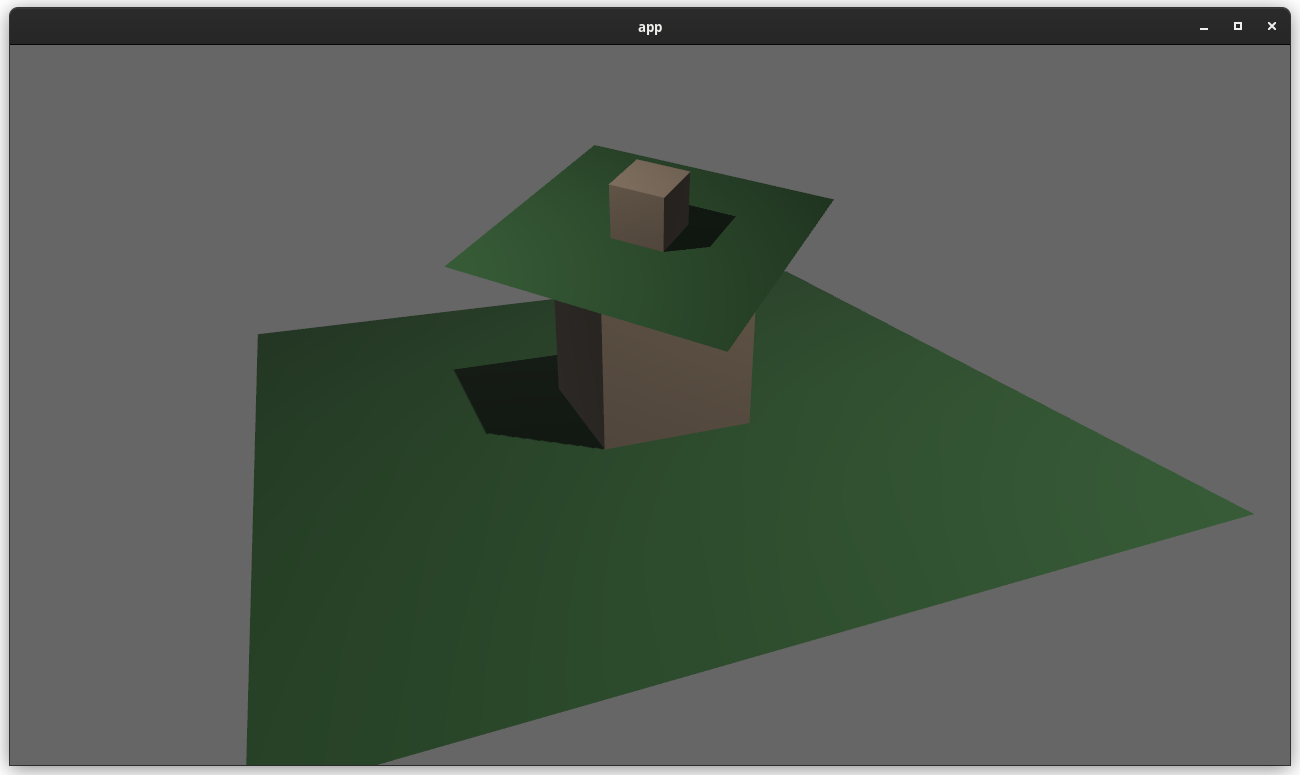 ### two_passes example with the "second pass" camera configured to "load" the depth 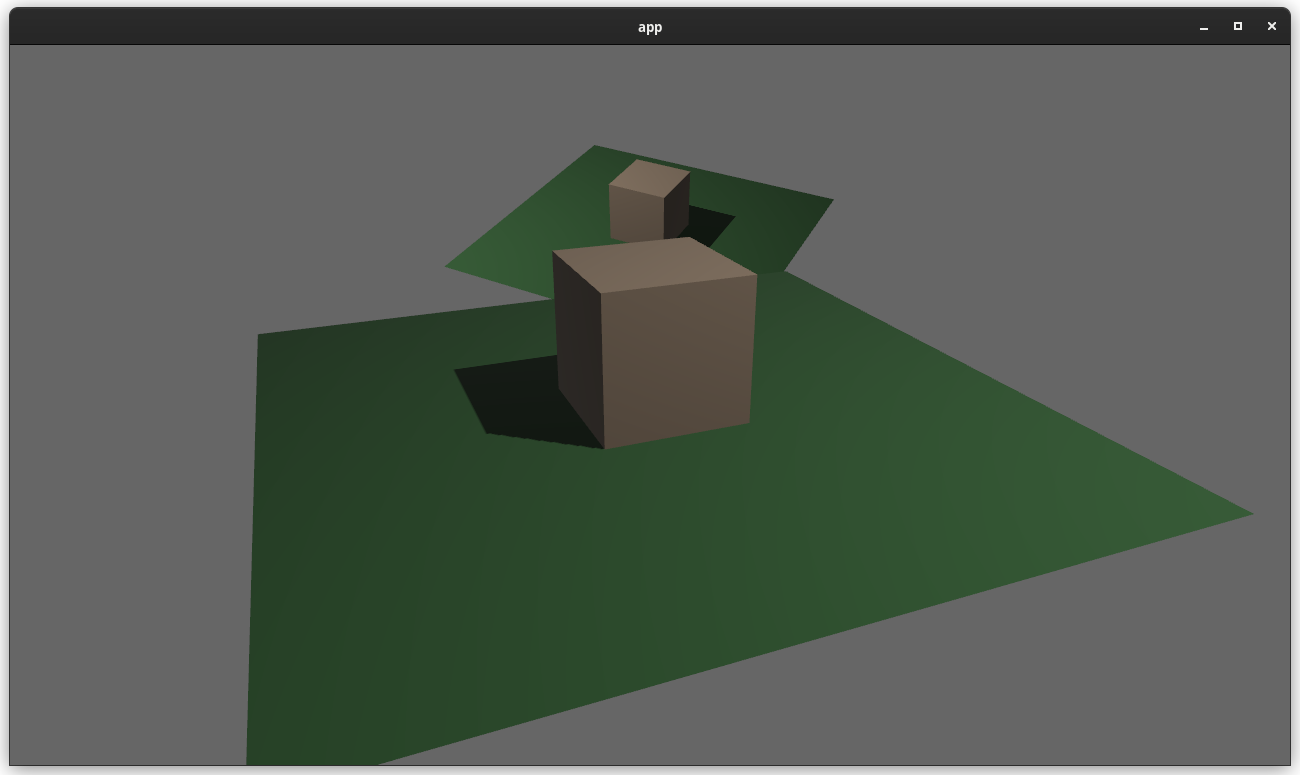 --- ## Changelog ### Added * `Camera3d` now has a `depth_load_op` field, which can configure the Camera's main 3d pass depth loading behavior.
109 lines
3.5 KiB
Rust
109 lines
3.5 KiB
Rust
//! Renders two cameras to the same window to accomplish "split screen".
|
|
|
|
use bevy::{
|
|
core_pipeline::clear_color::ClearColorConfig,
|
|
prelude::*,
|
|
render::camera::Viewport,
|
|
window::{WindowId, WindowResized},
|
|
};
|
|
|
|
fn main() {
|
|
App::new()
|
|
.add_plugins(DefaultPlugins)
|
|
.add_startup_system(setup)
|
|
.add_system(set_camera_viewports)
|
|
.run();
|
|
}
|
|
|
|
/// set up a simple 3D scene
|
|
fn setup(
|
|
mut commands: Commands,
|
|
asset_server: Res<AssetServer>,
|
|
mut meshes: ResMut<Assets<Mesh>>,
|
|
mut materials: ResMut<Assets<StandardMaterial>>,
|
|
) {
|
|
// plane
|
|
commands.spawn_bundle(PbrBundle {
|
|
mesh: meshes.add(Mesh::from(shape::Plane { size: 100.0 })),
|
|
material: materials.add(Color::rgb(0.3, 0.5, 0.3).into()),
|
|
..default()
|
|
});
|
|
|
|
commands.spawn_scene(asset_server.load("models/animated/Fox.glb#Scene0"));
|
|
|
|
// Light
|
|
commands.spawn_bundle(DirectionalLightBundle {
|
|
transform: Transform::from_rotation(Quat::from_euler(
|
|
EulerRot::ZYX,
|
|
0.0,
|
|
1.0,
|
|
-std::f32::consts::FRAC_PI_4,
|
|
)),
|
|
directional_light: DirectionalLight {
|
|
shadows_enabled: true,
|
|
..default()
|
|
},
|
|
..default()
|
|
});
|
|
|
|
// Left Camera
|
|
commands
|
|
.spawn_bundle(Camera3dBundle {
|
|
transform: Transform::from_xyz(0.0, 200.0, -100.0).looking_at(Vec3::ZERO, Vec3::Y),
|
|
..default()
|
|
})
|
|
.insert(LeftCamera);
|
|
|
|
// Right Camera
|
|
commands
|
|
.spawn_bundle(Camera3dBundle {
|
|
transform: Transform::from_xyz(100.0, 100., 150.0).looking_at(Vec3::ZERO, Vec3::Y),
|
|
camera: Camera {
|
|
// Renders the right camera after the left camera, which has a default priority of 0
|
|
priority: 1,
|
|
..default()
|
|
},
|
|
camera_3d: Camera3d {
|
|
// dont clear on the second camera because the first camera already cleared the window
|
|
clear_color: ClearColorConfig::None,
|
|
..default()
|
|
},
|
|
..default()
|
|
})
|
|
.insert(RightCamera);
|
|
}
|
|
|
|
#[derive(Component)]
|
|
struct LeftCamera;
|
|
|
|
#[derive(Component)]
|
|
struct RightCamera;
|
|
|
|
fn set_camera_viewports(
|
|
windows: Res<Windows>,
|
|
mut resize_events: EventReader<WindowResized>,
|
|
mut left_camera: Query<&mut Camera, (With<LeftCamera>, Without<RightCamera>)>,
|
|
mut right_camera: Query<&mut Camera, With<RightCamera>>,
|
|
) {
|
|
// We need to dynamically resize the camera's viewports whenever the window size changes
|
|
// so then each camera always takes up half the screen.
|
|
// A resize_event is sent when the window is first created, allowing us to reuse this system for initial setup.
|
|
for resize_event in resize_events.iter() {
|
|
if resize_event.id == WindowId::primary() {
|
|
let window = windows.primary();
|
|
let mut left_camera = left_camera.single_mut();
|
|
left_camera.viewport = Some(Viewport {
|
|
physical_position: UVec2::new(0, 0),
|
|
physical_size: UVec2::new(window.physical_width() / 2, window.physical_height()),
|
|
..default()
|
|
});
|
|
|
|
let mut right_camera = right_camera.single_mut();
|
|
right_camera.viewport = Some(Viewport {
|
|
physical_position: UVec2::new(window.physical_width() / 2, 0),
|
|
physical_size: UVec2::new(window.physical_width() / 2, window.physical_height()),
|
|
..default()
|
|
});
|
|
}
|
|
}
|
|
}
|