mirror of
https://github.com/bevyengine/bevy
synced 2024-11-26 22:50:19 +00:00
# Objective Speed up the render phase for rendering. ## Solution - Follow up #6988 and make the internals of atomic IDs `NonZeroU32`. This niches the `Option`s of the IDs in draw state, which reduces the size and branching behavior when evaluating for equality. - Require `&RenderDevice` to get the device's `Limits` when initializing a `TrackedRenderPass` to preallocate the bind groups and vertex buffer state in `DrawState`, this removes the branch on needing to resize those `Vec`s. ## Performance This produces a similar speed up akin to that of #6885. This shows an approximate 6% speed up in `main_opaque_pass_3d` on `many_foxes` (408.79 us -> 388us). This should be orthogonal to the gains seen there. 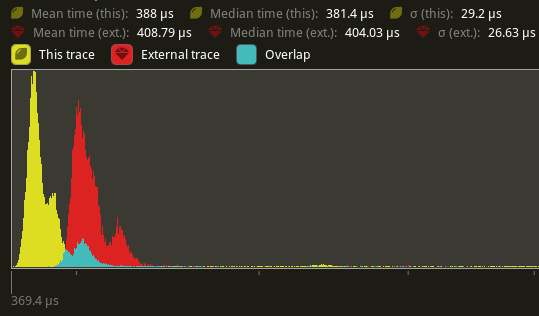 --- ## Changelog Added: `RenderContext::begin_tracked_render_pass`. Changed: `TrackedRenderPass` now requires a `&RenderDevice` on construction. Removed: `bevy_render::render_phase::DrawState`. It was not usable in any form outside of `bevy_render`. ## Migration Guide TODO
192 lines
5.3 KiB
Rust
192 lines
5.3 KiB
Rust
use super::{UiBatch, UiImageBindGroups, UiMeta};
|
|
use crate::{prelude::UiCameraConfig, DefaultCameraView};
|
|
use bevy_ecs::{
|
|
prelude::*,
|
|
system::{lifetimeless::*, SystemParamItem},
|
|
};
|
|
use bevy_render::{
|
|
render_graph::*,
|
|
render_phase::*,
|
|
render_resource::{CachedRenderPipelineId, LoadOp, Operations, RenderPassDescriptor},
|
|
renderer::*,
|
|
view::*,
|
|
};
|
|
use bevy_utils::FloatOrd;
|
|
|
|
pub struct UiPassNode {
|
|
ui_view_query: QueryState<
|
|
(
|
|
&'static RenderPhase<TransparentUi>,
|
|
&'static ViewTarget,
|
|
Option<&'static UiCameraConfig>,
|
|
),
|
|
With<ExtractedView>,
|
|
>,
|
|
default_camera_view_query: QueryState<&'static DefaultCameraView>,
|
|
}
|
|
|
|
impl UiPassNode {
|
|
pub const IN_VIEW: &'static str = "view";
|
|
|
|
pub fn new(world: &mut World) -> Self {
|
|
Self {
|
|
ui_view_query: world.query_filtered(),
|
|
default_camera_view_query: world.query(),
|
|
}
|
|
}
|
|
}
|
|
|
|
impl Node for UiPassNode {
|
|
fn input(&self) -> Vec<SlotInfo> {
|
|
vec![SlotInfo::new(UiPassNode::IN_VIEW, SlotType::Entity)]
|
|
}
|
|
|
|
fn update(&mut self, world: &mut World) {
|
|
self.ui_view_query.update_archetypes(world);
|
|
self.default_camera_view_query.update_archetypes(world);
|
|
}
|
|
|
|
fn run(
|
|
&self,
|
|
graph: &mut RenderGraphContext,
|
|
render_context: &mut RenderContext,
|
|
world: &World,
|
|
) -> Result<(), NodeRunError> {
|
|
let input_view_entity = graph.get_input_entity(Self::IN_VIEW)?;
|
|
|
|
let Ok((transparent_phase, target, camera_ui)) =
|
|
self.ui_view_query.get_manual(world, input_view_entity)
|
|
else {
|
|
return Ok(());
|
|
};
|
|
if transparent_phase.items.is_empty() {
|
|
return Ok(());
|
|
}
|
|
// Don't render UI for cameras where it is explicitly disabled
|
|
if matches!(camera_ui, Some(&UiCameraConfig { show_ui: false })) {
|
|
return Ok(());
|
|
}
|
|
|
|
// use the "default" view entity if it is defined
|
|
let view_entity = if let Ok(default_view) = self
|
|
.default_camera_view_query
|
|
.get_manual(world, input_view_entity)
|
|
{
|
|
default_view.0
|
|
} else {
|
|
input_view_entity
|
|
};
|
|
let mut render_pass = render_context.begin_tracked_render_pass(RenderPassDescriptor {
|
|
label: Some("ui_pass"),
|
|
color_attachments: &[Some(target.get_unsampled_color_attachment(Operations {
|
|
load: LoadOp::Load,
|
|
store: true,
|
|
}))],
|
|
depth_stencil_attachment: None,
|
|
});
|
|
|
|
transparent_phase.render(&mut render_pass, world, view_entity);
|
|
|
|
Ok(())
|
|
}
|
|
}
|
|
|
|
pub struct TransparentUi {
|
|
pub sort_key: FloatOrd,
|
|
pub entity: Entity,
|
|
pub pipeline: CachedRenderPipelineId,
|
|
pub draw_function: DrawFunctionId,
|
|
}
|
|
|
|
impl PhaseItem for TransparentUi {
|
|
type SortKey = FloatOrd;
|
|
|
|
#[inline]
|
|
fn entity(&self) -> Entity {
|
|
self.entity
|
|
}
|
|
|
|
#[inline]
|
|
fn sort_key(&self) -> Self::SortKey {
|
|
self.sort_key
|
|
}
|
|
|
|
#[inline]
|
|
fn draw_function(&self) -> DrawFunctionId {
|
|
self.draw_function
|
|
}
|
|
}
|
|
|
|
impl CachedRenderPipelinePhaseItem for TransparentUi {
|
|
#[inline]
|
|
fn cached_pipeline(&self) -> CachedRenderPipelineId {
|
|
self.pipeline
|
|
}
|
|
}
|
|
|
|
pub type DrawUi = (
|
|
SetItemPipeline,
|
|
SetUiViewBindGroup<0>,
|
|
SetUiTextureBindGroup<1>,
|
|
DrawUiNode,
|
|
);
|
|
|
|
pub struct SetUiViewBindGroup<const I: usize>;
|
|
impl<P: PhaseItem, const I: usize> RenderCommand<P> for SetUiViewBindGroup<I> {
|
|
type Param = SRes<UiMeta>;
|
|
type ViewWorldQuery = Read<ViewUniformOffset>;
|
|
type ItemWorldQuery = ();
|
|
|
|
fn render<'w>(
|
|
_item: &P,
|
|
view_uniform: &'w ViewUniformOffset,
|
|
_entity: (),
|
|
ui_meta: SystemParamItem<'w, '_, Self::Param>,
|
|
pass: &mut TrackedRenderPass<'w>,
|
|
) -> RenderCommandResult {
|
|
pass.set_bind_group(
|
|
I,
|
|
ui_meta.into_inner().view_bind_group.as_ref().unwrap(),
|
|
&[view_uniform.offset],
|
|
);
|
|
RenderCommandResult::Success
|
|
}
|
|
}
|
|
pub struct SetUiTextureBindGroup<const I: usize>;
|
|
impl<P: PhaseItem, const I: usize> RenderCommand<P> for SetUiTextureBindGroup<I> {
|
|
type Param = SRes<UiImageBindGroups>;
|
|
type ViewWorldQuery = ();
|
|
type ItemWorldQuery = Read<UiBatch>;
|
|
|
|
#[inline]
|
|
fn render<'w>(
|
|
_item: &P,
|
|
_view: (),
|
|
batch: &'w UiBatch,
|
|
image_bind_groups: SystemParamItem<'w, '_, Self::Param>,
|
|
pass: &mut TrackedRenderPass<'w>,
|
|
) -> RenderCommandResult {
|
|
let image_bind_groups = image_bind_groups.into_inner();
|
|
pass.set_bind_group(I, image_bind_groups.values.get(&batch.image).unwrap(), &[]);
|
|
RenderCommandResult::Success
|
|
}
|
|
}
|
|
pub struct DrawUiNode;
|
|
impl<P: PhaseItem> RenderCommand<P> for DrawUiNode {
|
|
type Param = SRes<UiMeta>;
|
|
type ViewWorldQuery = ();
|
|
type ItemWorldQuery = Read<UiBatch>;
|
|
|
|
#[inline]
|
|
fn render<'w>(
|
|
_item: &P,
|
|
_view: (),
|
|
batch: &'w UiBatch,
|
|
ui_meta: SystemParamItem<'w, '_, Self::Param>,
|
|
pass: &mut TrackedRenderPass<'w>,
|
|
) -> RenderCommandResult {
|
|
pass.set_vertex_buffer(0, ui_meta.into_inner().vertices.buffer().unwrap().slice(..));
|
|
pass.draw(batch.range.clone(), 0..1);
|
|
RenderCommandResult::Success
|
|
}
|
|
}
|