mirror of
https://github.com/bevyengine/bevy
synced 2024-11-10 15:14:50 +00:00
# Objective Adopt code from [bevy_polyline](https://github.com/ForesightMiningSoftwareCorporation/bevy_polyline) for gizmo line-rendering. This adds configurable width and perspective rendering for the lines. Many thanks to @mtsr for the initial work on bevy_polyline. Thanks to @aevyrie for maintaining it, @nicopap for adding the depth_bias feature and the other [contributors](https://github.com/ForesightMiningSoftwareCorporation/bevy_polyline/graphs/contributors) for squashing bugs and keeping bevy_polyline up-to-date. #### Before 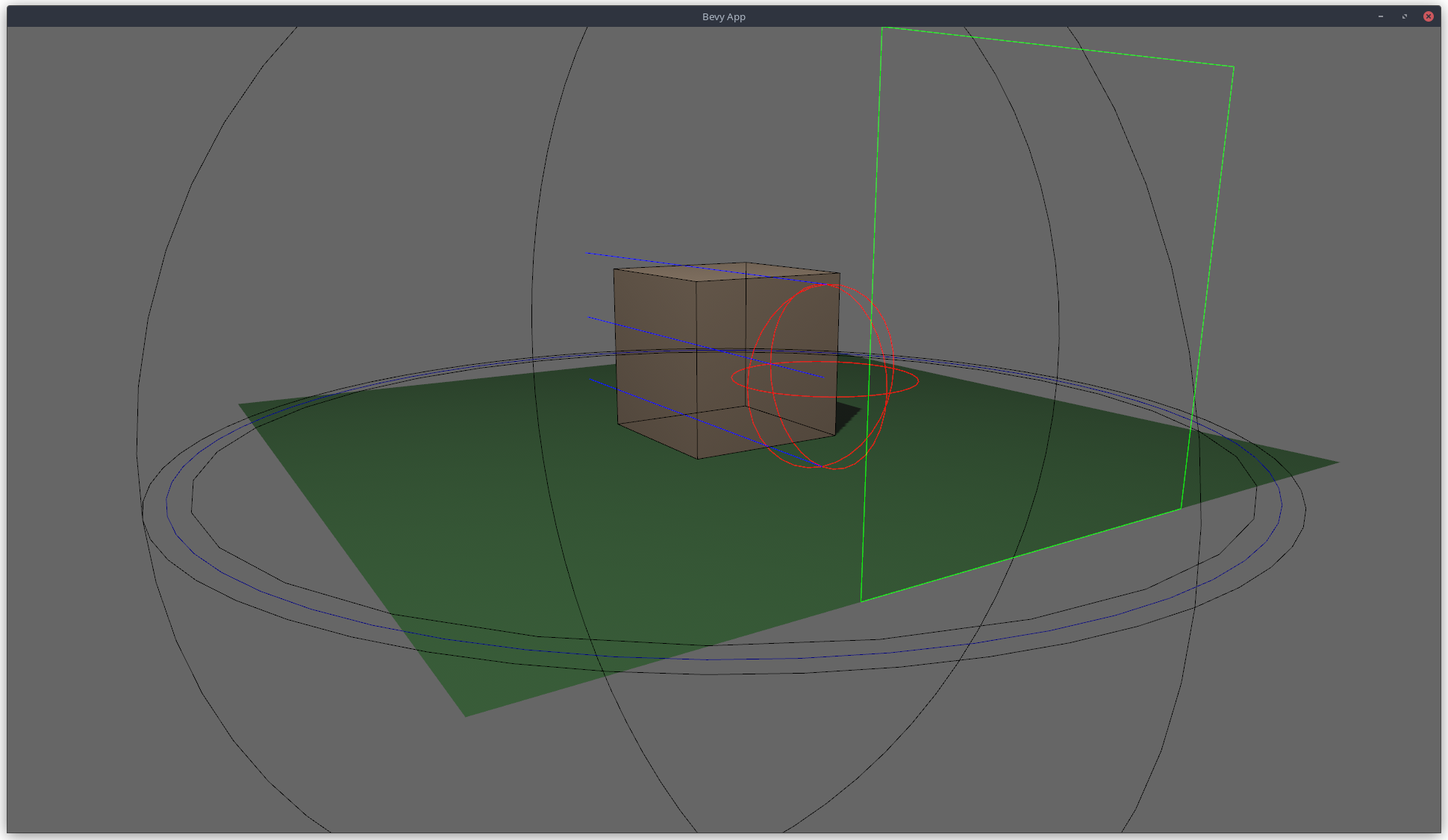 #### After - with line perspective 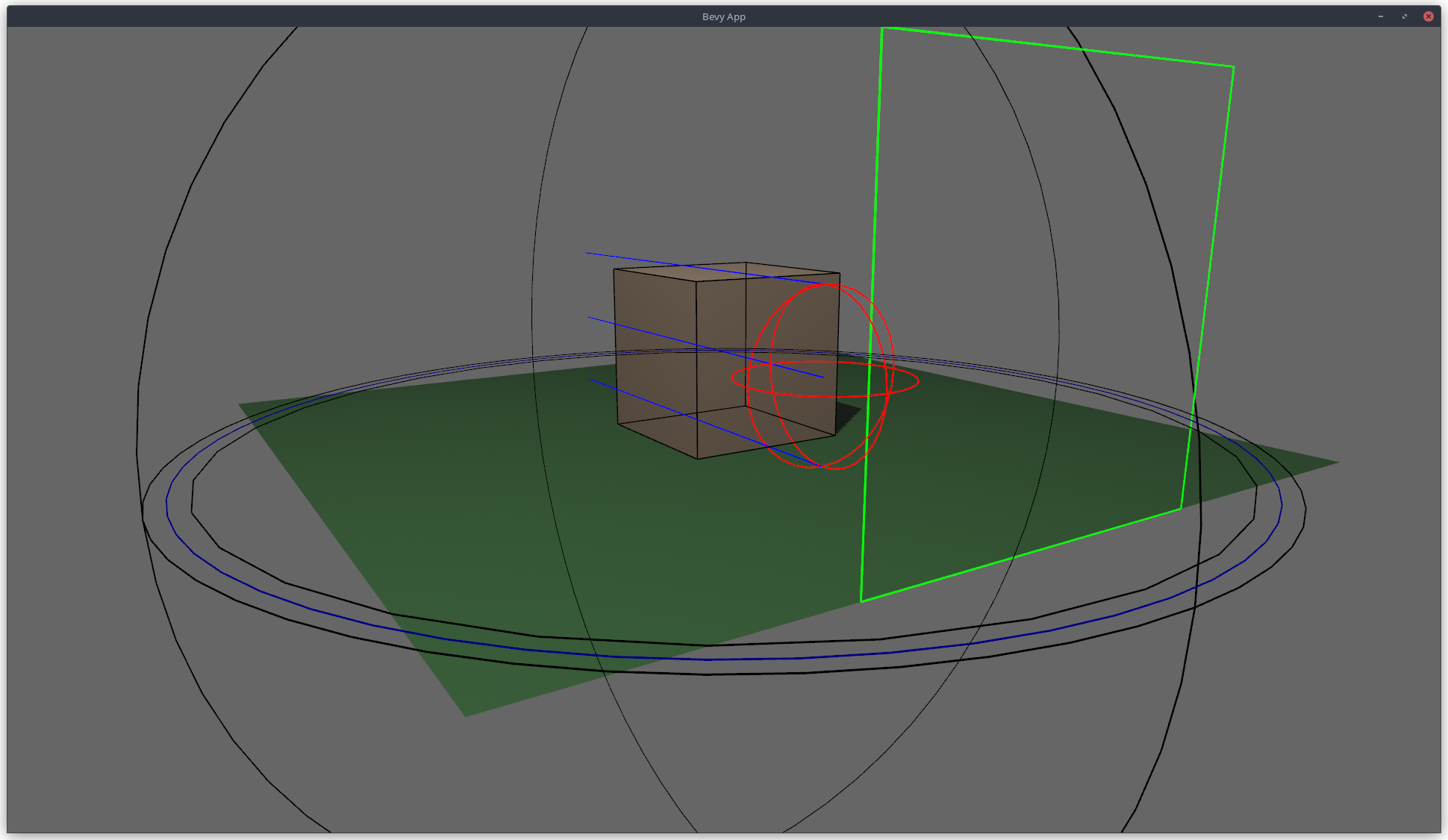 Line perspective is not on by default because with perspective there is no default line width that works for every scene. <details><summary>After - without line perspective</summary> <p> 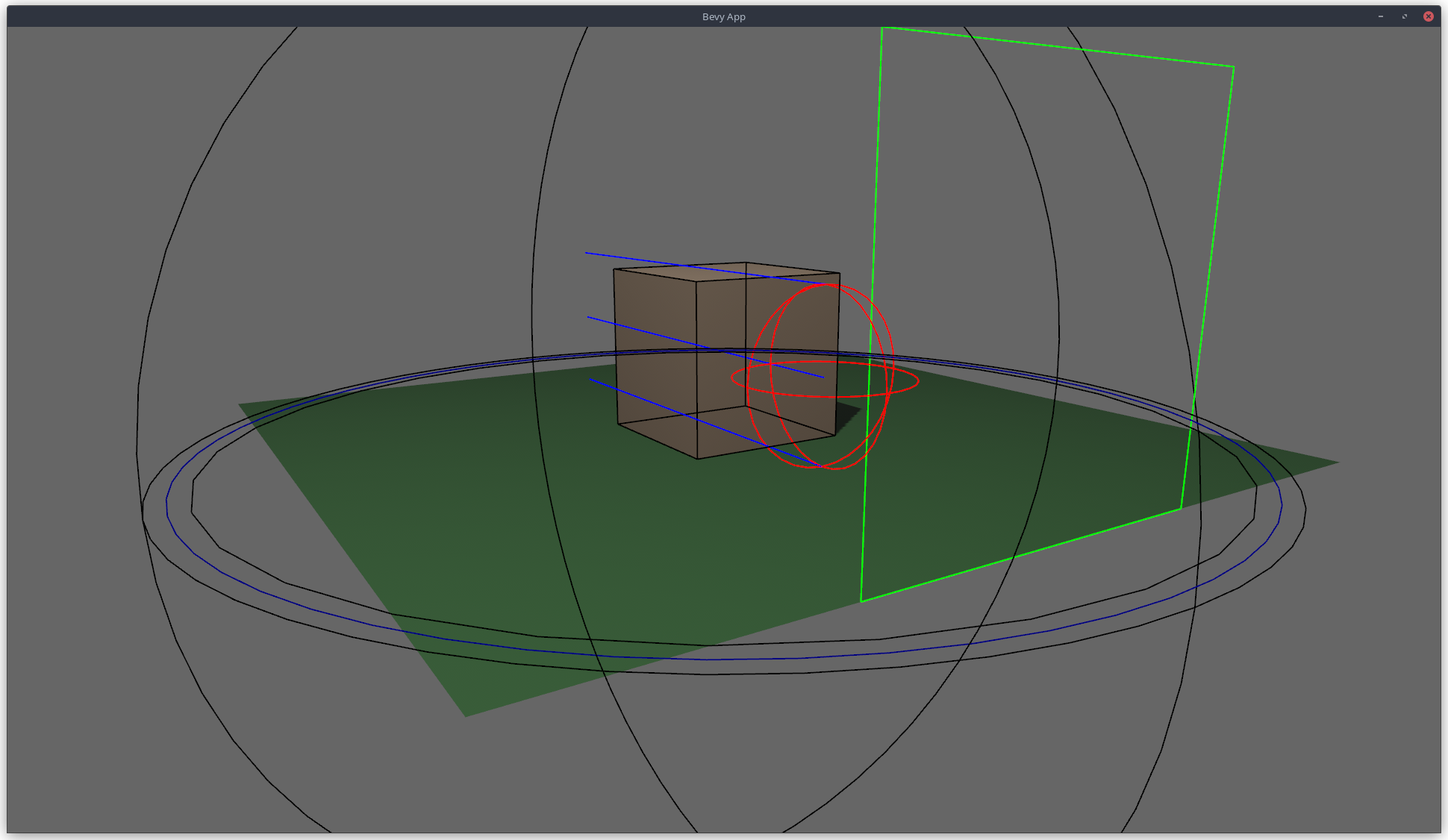 </p> </details> Somewhat unexpectedly, the performance is improved with this PR. At 200,000 lines in many_gizmos I get ~110 FPS on main and ~200 FPS with this PR. I'm guessing this is a CPU side difference as I would expect the rendering technique to be more expensive on the GPU to some extent, but I am not entirely sure. --------- Co-authored-by: Jonas Matser <github@jonasmatser.nl> Co-authored-by: Aevyrie <aevyrie@gmail.com> Co-authored-by: Nicola Papale <nico@nicopap.ch> Co-authored-by: Nicola Papale <nicopap@users.noreply.github.com>
124 lines
3.8 KiB
Rust
124 lines
3.8 KiB
Rust
//! This example demonstrates Bevy's immediate mode drawing API intended for visual debugging.
|
|
|
|
use std::f32::consts::PI;
|
|
|
|
use bevy::prelude::*;
|
|
|
|
fn main() {
|
|
App::new()
|
|
.add_plugins(DefaultPlugins)
|
|
.add_systems(Startup, setup)
|
|
.add_systems(Update, (system, rotate_camera, update_config))
|
|
.run();
|
|
}
|
|
|
|
fn setup(
|
|
mut commands: Commands,
|
|
mut meshes: ResMut<Assets<Mesh>>,
|
|
mut materials: ResMut<Assets<StandardMaterial>>,
|
|
) {
|
|
commands.spawn(Camera3dBundle {
|
|
transform: Transform::from_xyz(0., 1.5, 6.).looking_at(Vec3::ZERO, Vec3::Y),
|
|
..default()
|
|
});
|
|
// plane
|
|
commands.spawn(PbrBundle {
|
|
mesh: meshes.add(Mesh::from(shape::Plane::from_size(5.0))),
|
|
material: materials.add(Color::rgb(0.3, 0.5, 0.3).into()),
|
|
..default()
|
|
});
|
|
// cube
|
|
commands.spawn(PbrBundle {
|
|
mesh: meshes.add(Mesh::from(shape::Cube { size: 1.0 })),
|
|
material: materials.add(Color::rgb(0.8, 0.7, 0.6).into()),
|
|
transform: Transform::from_xyz(0.0, 0.5, 0.0),
|
|
..default()
|
|
});
|
|
// light
|
|
commands.spawn(PointLightBundle {
|
|
point_light: PointLight {
|
|
intensity: 1500.0,
|
|
shadows_enabled: true,
|
|
..default()
|
|
},
|
|
transform: Transform::from_xyz(4.0, 8.0, 4.0),
|
|
..default()
|
|
});
|
|
|
|
// example instructions
|
|
commands.spawn(
|
|
TextBundle::from_section(
|
|
"Press 'D' to toggle drawing gizmos on top of everything else in the scene\n\
|
|
Press 'P' to toggle perspective for line gizmos\n\
|
|
Hold 'Left' or 'Right' to change the line width",
|
|
TextStyle {
|
|
font_size: 20.,
|
|
..default()
|
|
},
|
|
)
|
|
.with_style(Style {
|
|
position_type: PositionType::Absolute,
|
|
top: Val::Px(12.0),
|
|
left: Val::Px(12.0),
|
|
..default()
|
|
}),
|
|
);
|
|
}
|
|
|
|
fn system(mut gizmos: Gizmos, time: Res<Time>) {
|
|
gizmos.cuboid(
|
|
Transform::from_translation(Vec3::Y * 0.5).with_scale(Vec3::splat(1.)),
|
|
Color::BLACK,
|
|
);
|
|
gizmos.rect(
|
|
Vec3::new(time.elapsed_seconds().cos() * 2.5, 1., 0.),
|
|
Quat::from_rotation_y(PI / 2.),
|
|
Vec2::splat(2.),
|
|
Color::GREEN,
|
|
);
|
|
|
|
gizmos.sphere(Vec3::new(1., 0.5, 0.), Quat::IDENTITY, 0.5, Color::RED);
|
|
|
|
for y in [0., 0.5, 1.] {
|
|
gizmos.ray(
|
|
Vec3::new(1., y, 0.),
|
|
Vec3::new(-3., (time.elapsed_seconds() * 3.).sin(), 0.),
|
|
Color::BLUE,
|
|
);
|
|
}
|
|
|
|
// Circles have 32 line-segments by default.
|
|
gizmos.circle(Vec3::ZERO, Vec3::Y, 3., Color::BLACK);
|
|
// You may want to increase this for larger circles or spheres.
|
|
gizmos
|
|
.circle(Vec3::ZERO, Vec3::Y, 3.1, Color::NAVY)
|
|
.segments(64);
|
|
gizmos
|
|
.sphere(Vec3::ZERO, Quat::IDENTITY, 3.2, Color::BLACK)
|
|
.circle_segments(64);
|
|
}
|
|
|
|
fn rotate_camera(mut query: Query<&mut Transform, With<Camera>>, time: Res<Time>) {
|
|
let mut transform = query.single_mut();
|
|
|
|
transform.rotate_around(Vec3::ZERO, Quat::from_rotation_y(time.delta_seconds() / 2.));
|
|
}
|
|
|
|
fn update_config(mut config: ResMut<GizmoConfig>, keyboard: Res<Input<KeyCode>>, time: Res<Time>) {
|
|
if keyboard.just_pressed(KeyCode::D) {
|
|
config.depth_bias = if config.depth_bias == 0. { -1. } else { 0. };
|
|
}
|
|
if keyboard.just_pressed(KeyCode::P) {
|
|
// Toggle line_perspective
|
|
config.line_perspective ^= true;
|
|
// Increase the line width when line_perspective is on
|
|
config.line_width *= if config.line_perspective { 5. } else { 1. / 5. };
|
|
}
|
|
|
|
if keyboard.pressed(KeyCode::Right) {
|
|
config.line_width += 5. * time.delta_seconds();
|
|
}
|
|
if keyboard.pressed(KeyCode::Left) {
|
|
config.line_width -= 5. * time.delta_seconds();
|
|
}
|
|
}
|