mirror of
https://github.com/bevyengine/bevy
synced 2024-11-23 05:03:47 +00:00
# Objective Fixes #5572 ## Solution Approach is to invert the Y-axis of the UI Camera by changing the UI projection matrix to render the UI upside down. After that I'm trying to fix all issues, that pop up: - interaction expected the "old" position - images and text were displayed upside-down - baseline of text was based on the top of the glyph instead of bottom ... probably a lot more. --- Result when running examples: <details> <summary>Button example</summary> main branch: 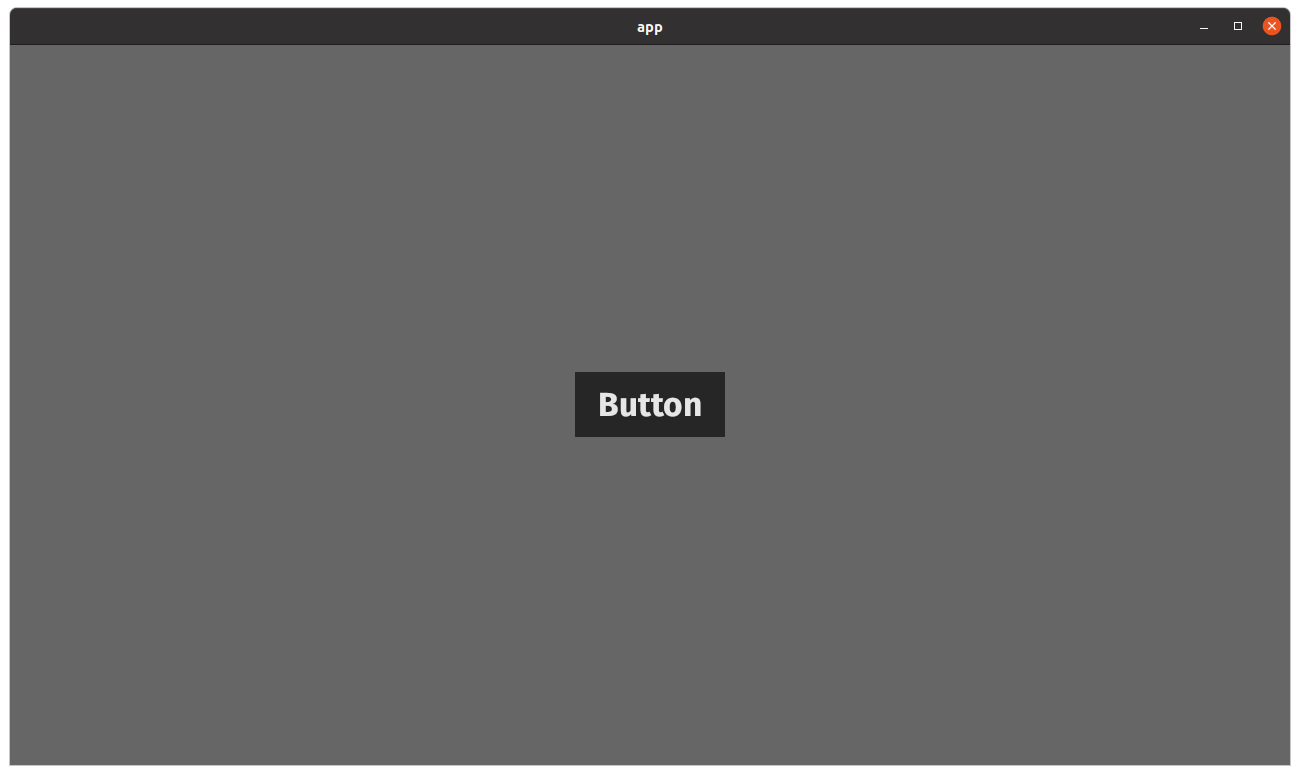 this pr: 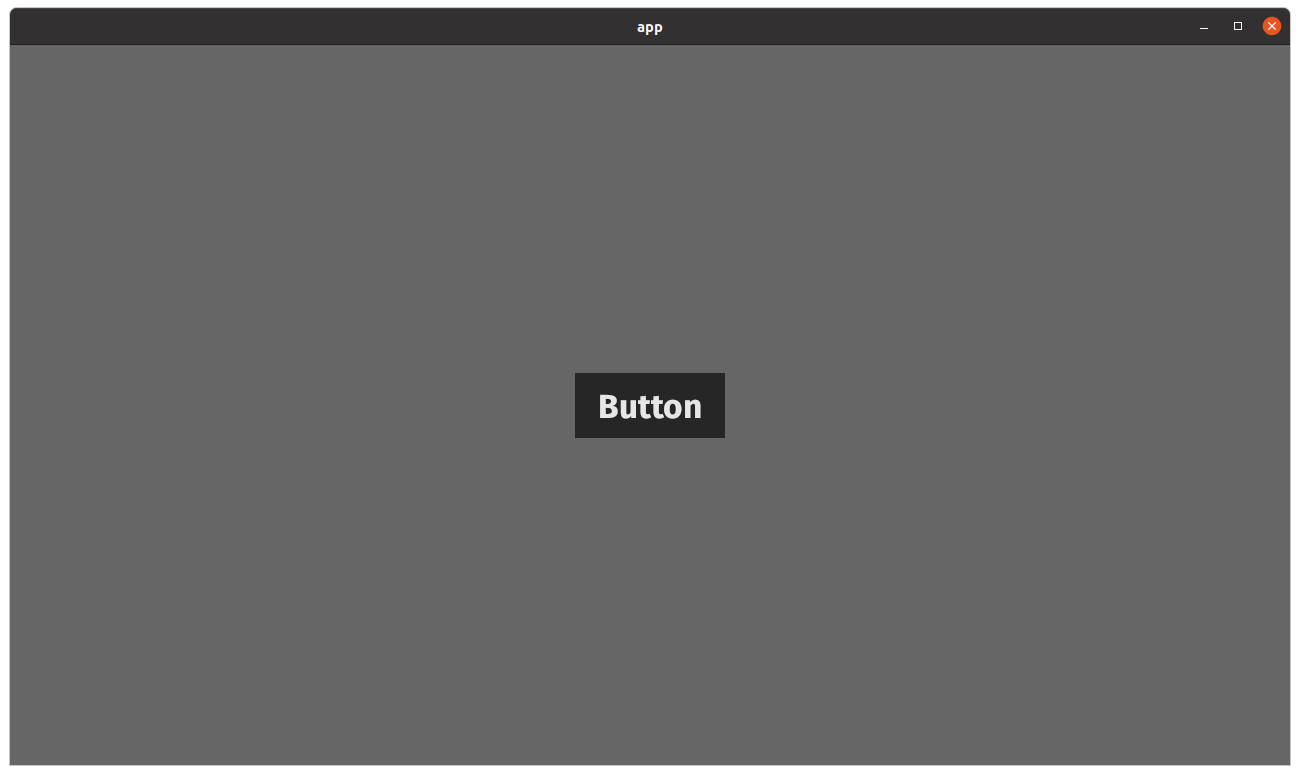 </details> <details> <summary>Text example</summary> m 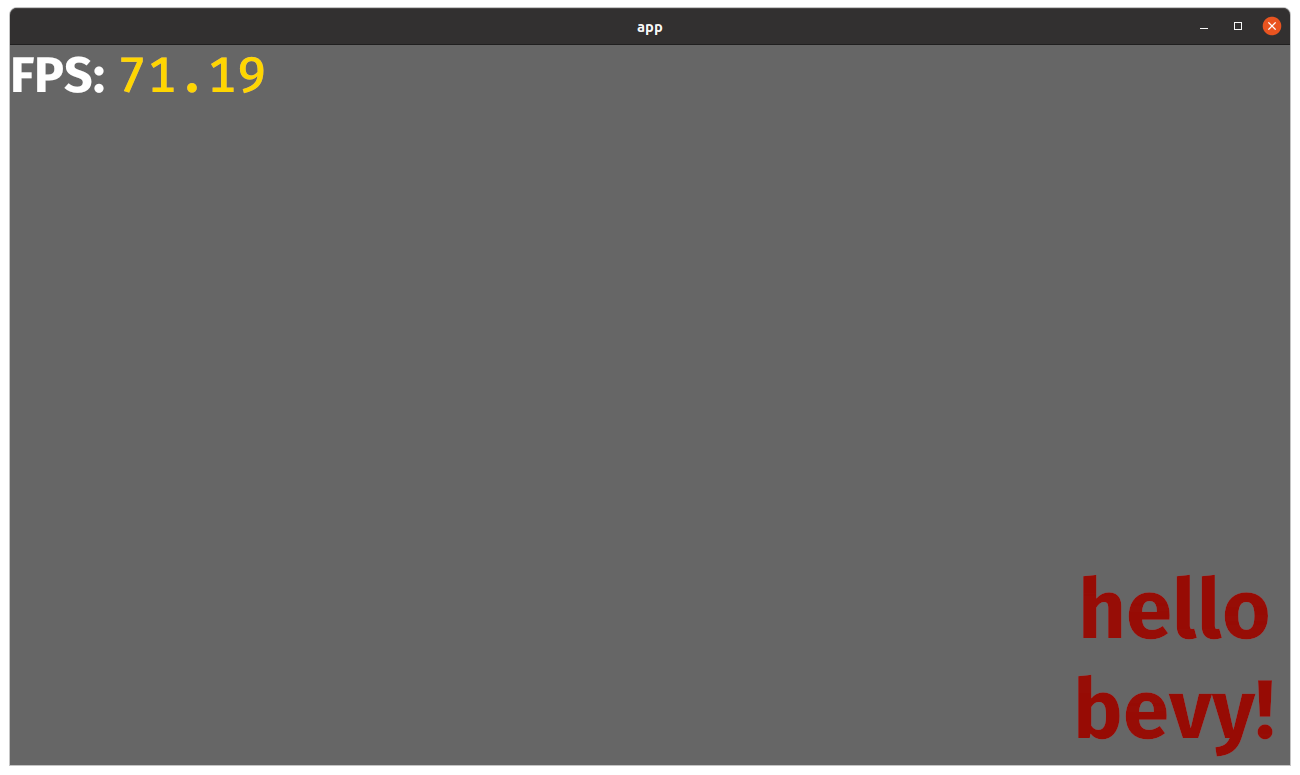 ain branch: this pr: 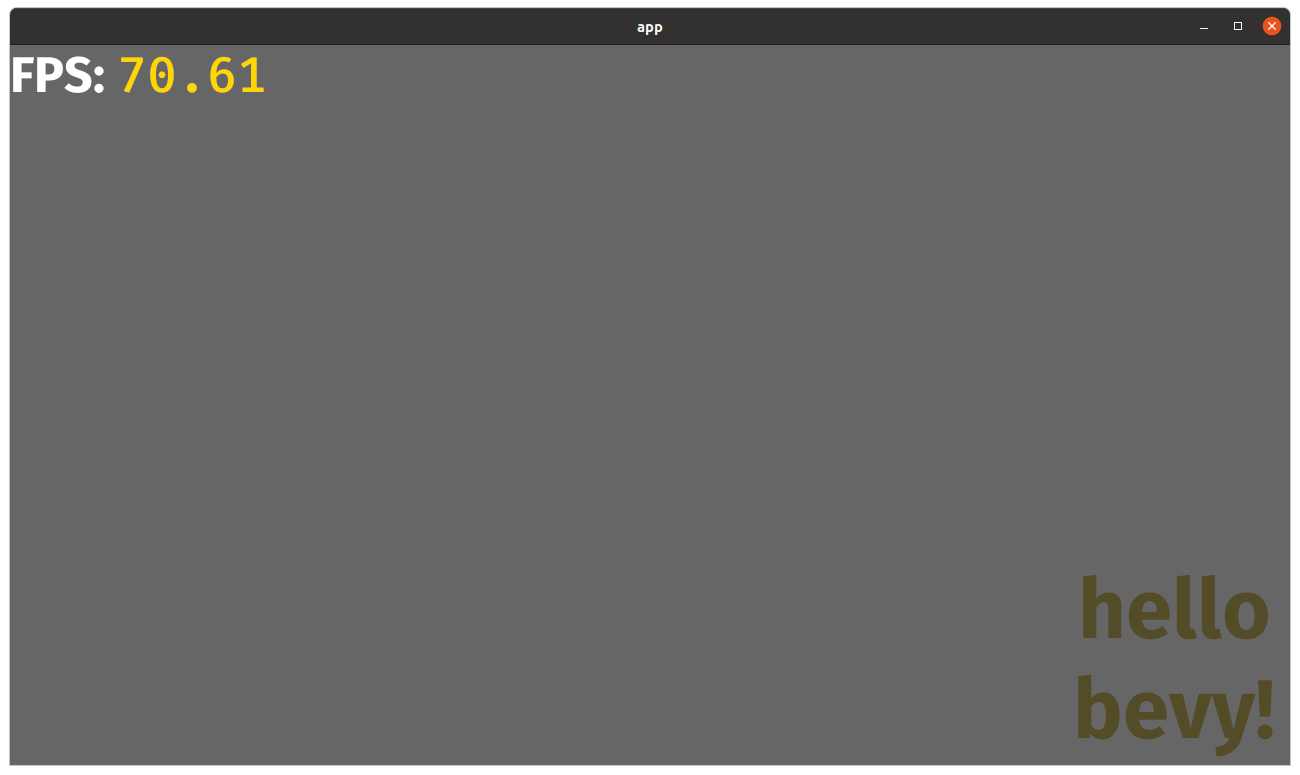 </details> <details> <summary>Text debug example</summary> main branch: 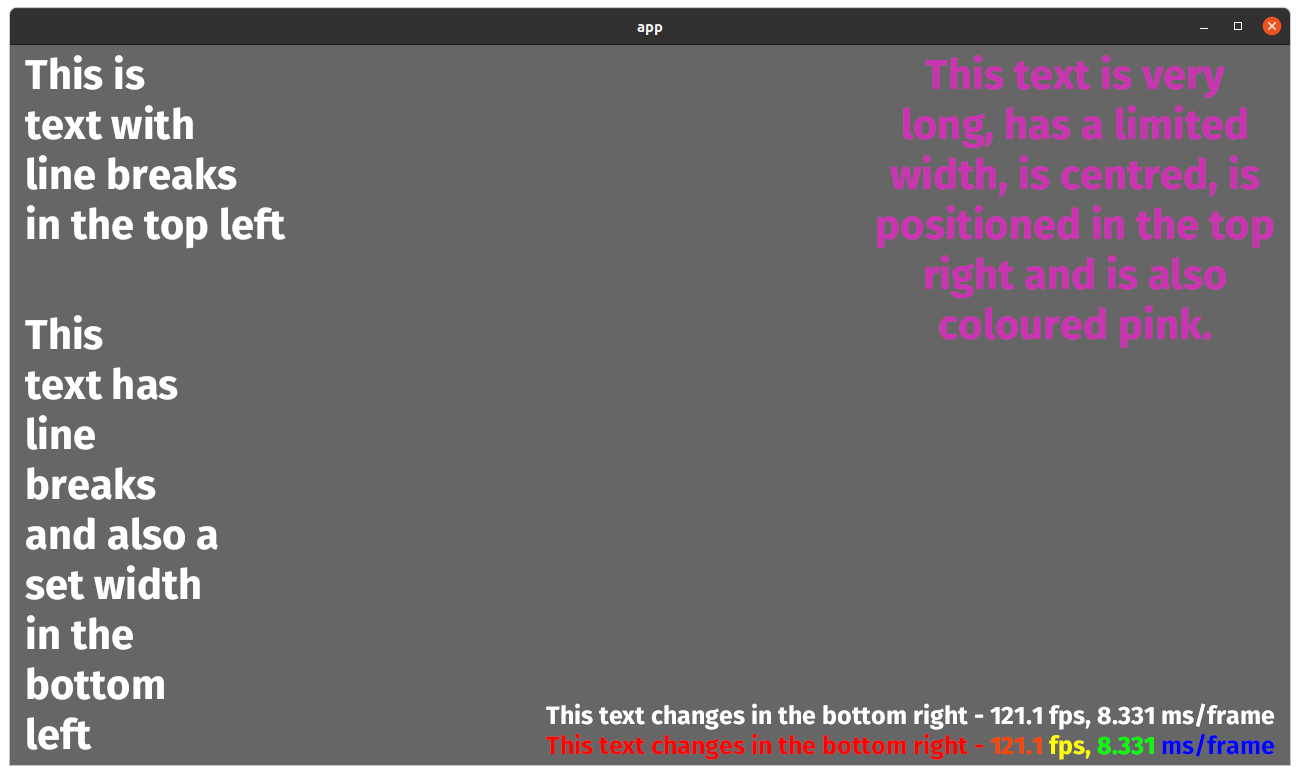 this pr: 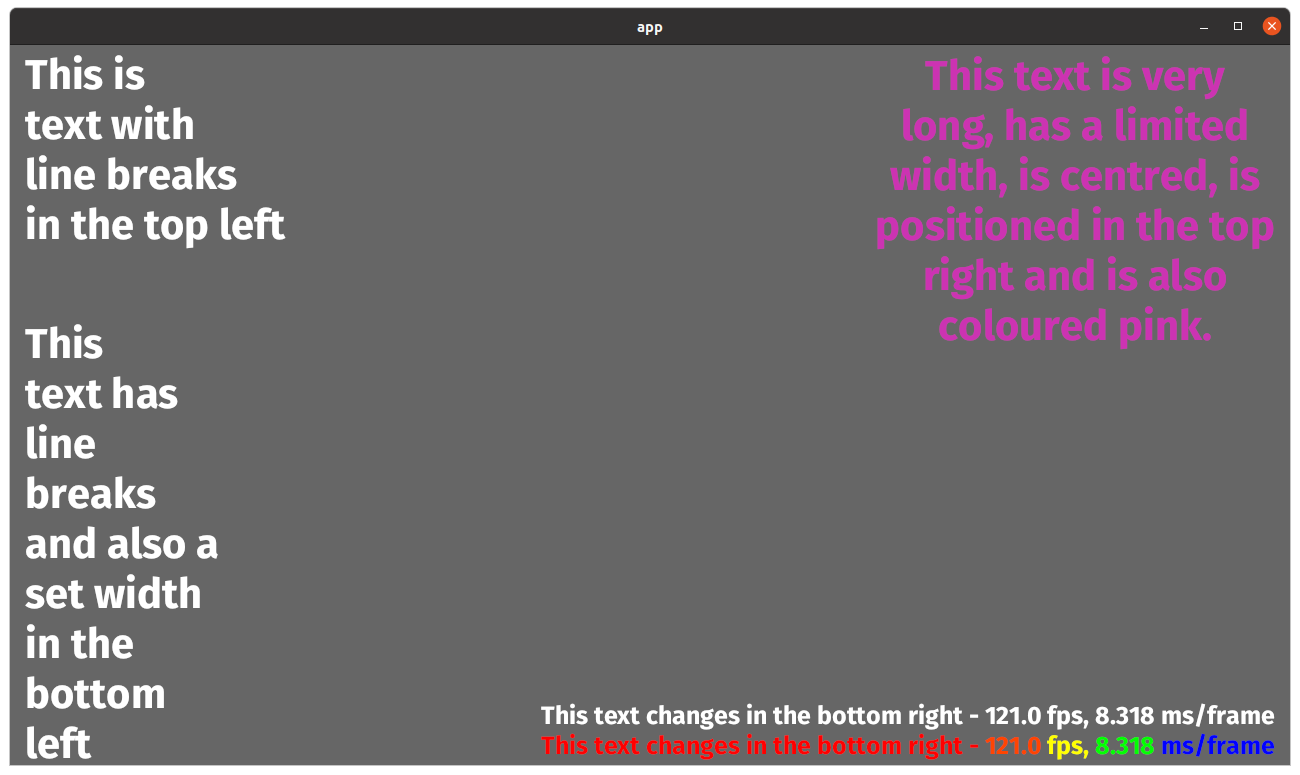 </details> <details> <summary>Transparency UI example</summary> main branch: 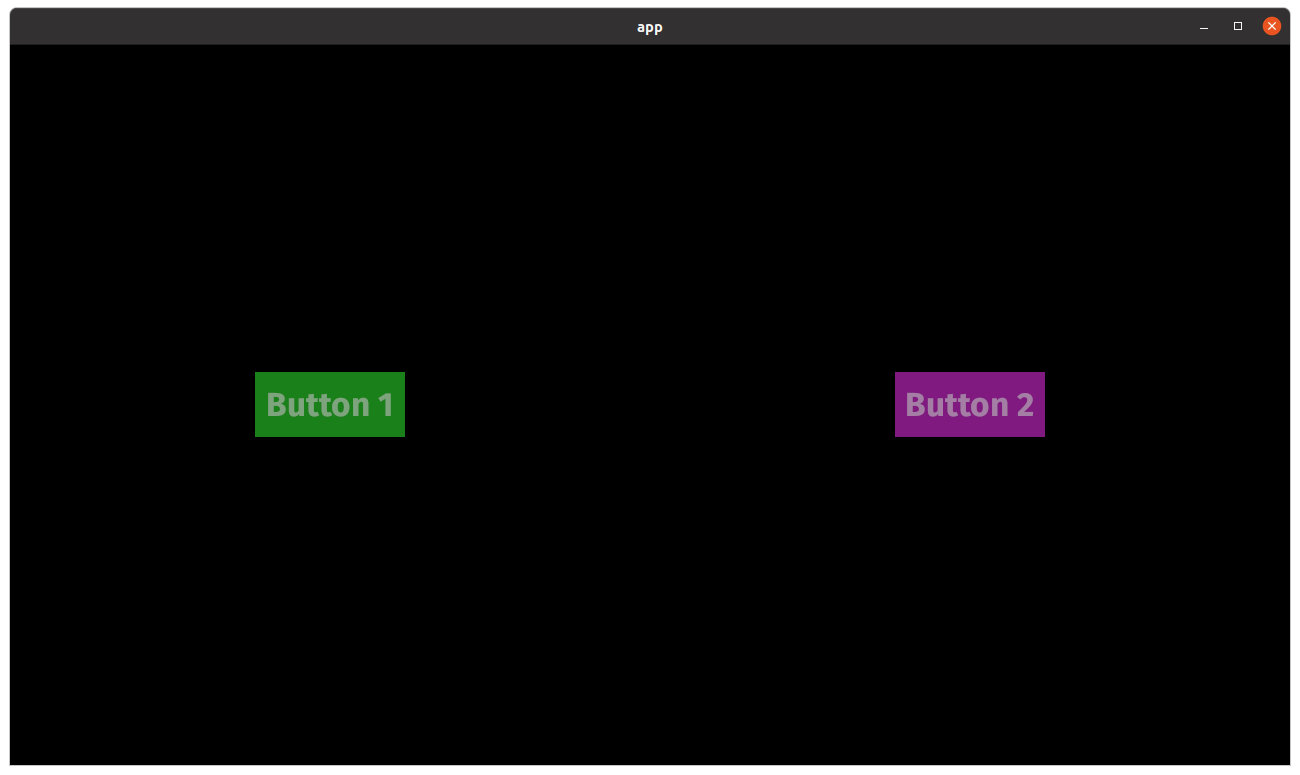 this pr: 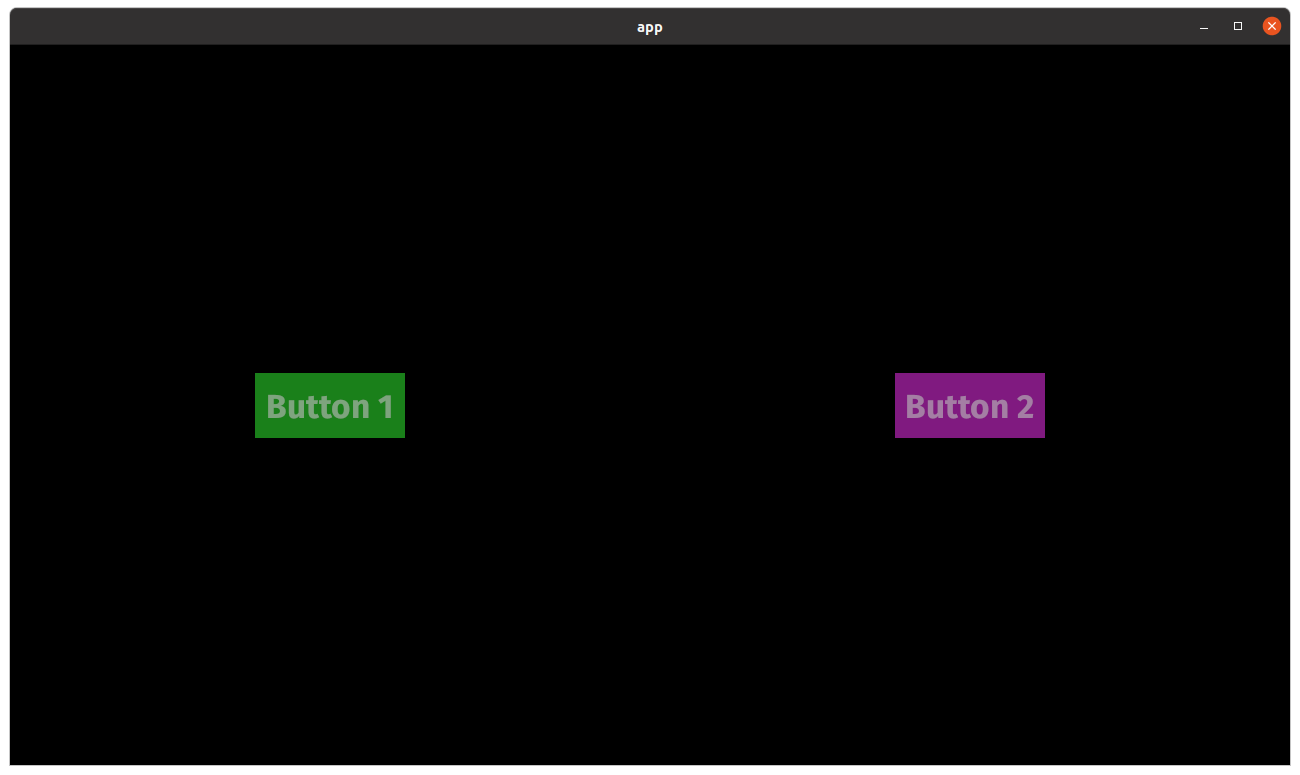 </details> <details> <summary>UI example</summary> **ui example** main branch: 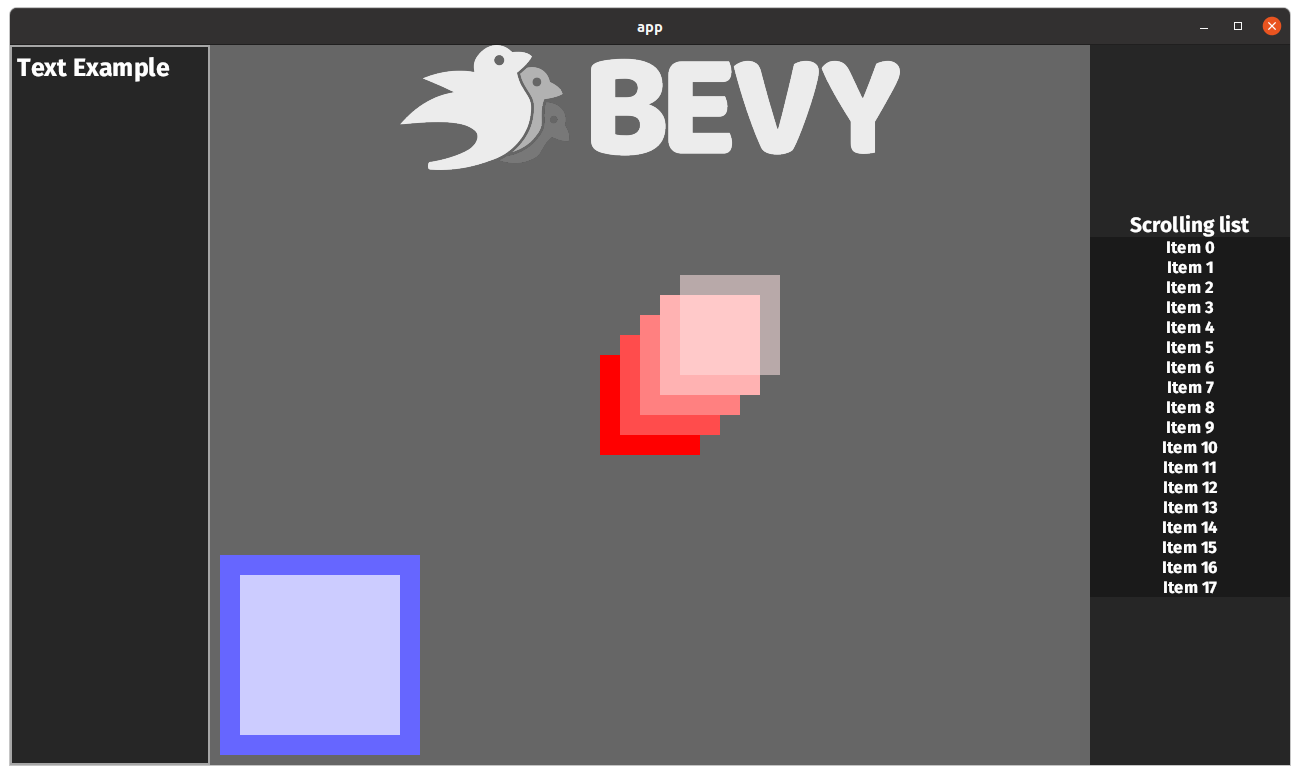 this pr: 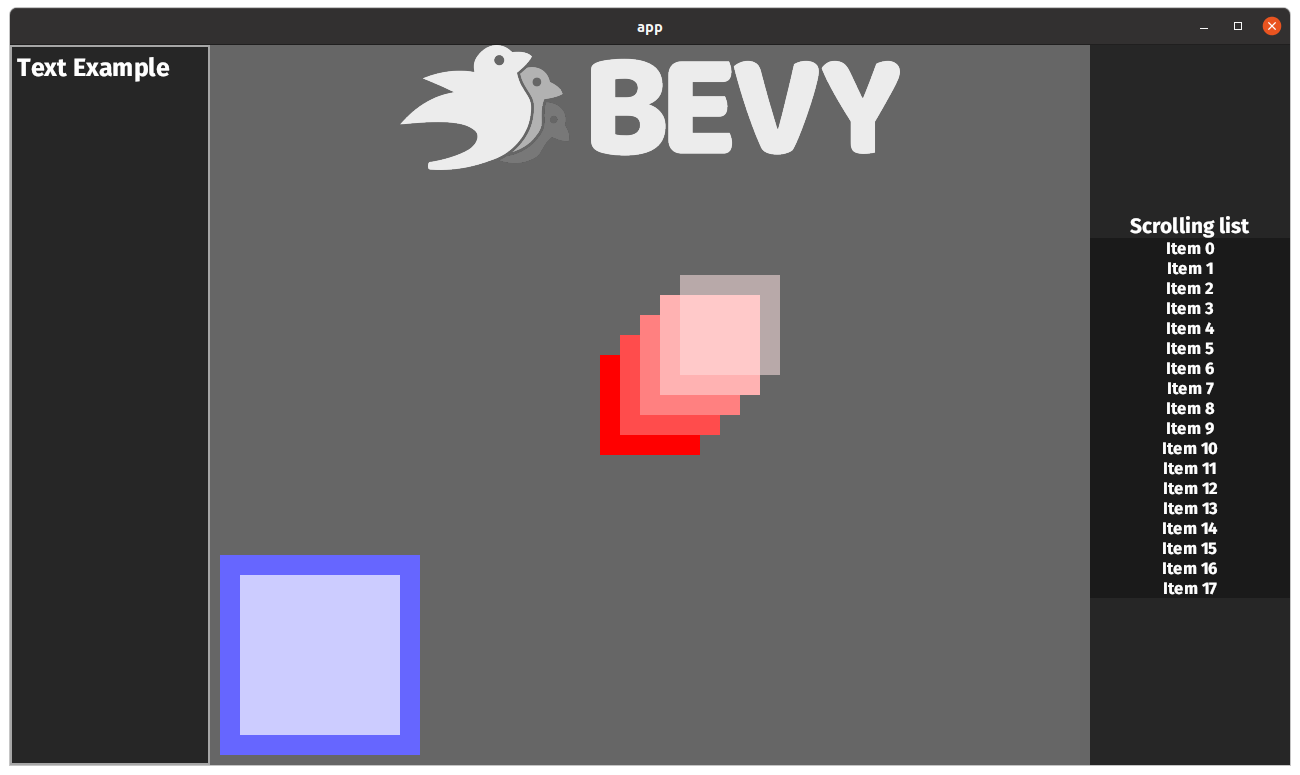 </details> ## Changelog UI coordinate system and cursor position was changed from bottom left origin, y+ up to top left origin, y+ down. ## Migration Guide All flex layout should be inverted (ColumnReverse => Column, FlexStart => FlexEnd, WrapReverse => Wrap) System where dealing with cursor position should be changed to account for cursor position being based on the top left instead of bottom left
102 lines
3.4 KiB
Rust
102 lines
3.4 KiB
Rust
//! This example illustrates how to create UI text and update it in a system.
|
|
//!
|
|
//! It displays the current FPS in the top left corner, as well as text that changes color
|
|
//! in the bottom right. For text within a scene, please see the text2d example.
|
|
|
|
use bevy::{
|
|
diagnostic::{Diagnostics, FrameTimeDiagnosticsPlugin},
|
|
prelude::*,
|
|
};
|
|
|
|
fn main() {
|
|
App::new()
|
|
.add_plugins(DefaultPlugins)
|
|
.add_plugin(FrameTimeDiagnosticsPlugin::default())
|
|
.add_startup_system(setup)
|
|
.add_system(text_update_system)
|
|
.add_system(text_color_system)
|
|
.run();
|
|
}
|
|
|
|
// A unit struct to help identify the FPS UI component, since there may be many Text components
|
|
#[derive(Component)]
|
|
struct FpsText;
|
|
|
|
// A unit struct to help identify the color-changing Text component
|
|
#[derive(Component)]
|
|
struct ColorText;
|
|
|
|
fn setup(mut commands: Commands, asset_server: Res<AssetServer>) {
|
|
// UI camera
|
|
commands.spawn(Camera2dBundle::default());
|
|
// Text with one section
|
|
commands.spawn((
|
|
// Create a TextBundle that has a Text with a single section.
|
|
TextBundle::from_section(
|
|
// Accepts a `String` or any type that converts into a `String`, such as `&str`
|
|
"hello\nbevy!",
|
|
TextStyle {
|
|
font: asset_server.load("fonts/FiraSans-Bold.ttf"),
|
|
font_size: 100.0,
|
|
color: Color::WHITE,
|
|
},
|
|
) // Set the alignment of the Text
|
|
.with_text_alignment(TextAlignment::TOP_CENTER)
|
|
// Set the style of the TextBundle itself.
|
|
.with_style(Style {
|
|
position_type: PositionType::Absolute,
|
|
position: UiRect {
|
|
bottom: Val::Px(5.0),
|
|
right: Val::Px(15.0),
|
|
..default()
|
|
},
|
|
..default()
|
|
}),
|
|
ColorText,
|
|
));
|
|
// Text with multiple sections
|
|
commands.spawn((
|
|
// Create a TextBundle that has a Text with a list of sections.
|
|
TextBundle::from_sections([
|
|
TextSection::new(
|
|
"FPS: ",
|
|
TextStyle {
|
|
font: asset_server.load("fonts/FiraSans-Bold.ttf"),
|
|
font_size: 60.0,
|
|
color: Color::WHITE,
|
|
},
|
|
),
|
|
TextSection::from_style(TextStyle {
|
|
font: asset_server.load("fonts/FiraMono-Medium.ttf"),
|
|
font_size: 60.0,
|
|
color: Color::GOLD,
|
|
}),
|
|
]),
|
|
FpsText,
|
|
));
|
|
}
|
|
|
|
fn text_color_system(time: Res<Time>, mut query: Query<&mut Text, With<ColorText>>) {
|
|
for mut text in &mut query {
|
|
let seconds = time.seconds_since_startup() as f32;
|
|
|
|
// Update the color of the first and only section.
|
|
text.sections[0].style.color = Color::Rgba {
|
|
red: (1.25 * seconds).sin() / 2.0 + 0.5,
|
|
green: (0.75 * seconds).sin() / 2.0 + 0.5,
|
|
blue: (0.50 * seconds).sin() / 2.0 + 0.5,
|
|
alpha: 1.0,
|
|
};
|
|
}
|
|
}
|
|
|
|
fn text_update_system(diagnostics: Res<Diagnostics>, mut query: Query<&mut Text, With<FpsText>>) {
|
|
for mut text in &mut query {
|
|
if let Some(fps) = diagnostics.get(FrameTimeDiagnosticsPlugin::FPS) {
|
|
if let Some(average) = fps.average() {
|
|
// Update the value of the second section
|
|
text.sections[1].value = format!("{average:.2}");
|
|
}
|
|
}
|
|
}
|
|
}
|