mirror of
https://github.com/bevyengine/bevy
synced 2024-09-20 22:42:08 +00:00
# Objective Partially addresses #4291. Speed up the sort phase for unbatched render phases. ## Solution Split out one of the optimizations in #4899 and allow implementors of `PhaseItem` to change what kind of sort is used when sorting the items in the phase. This currently includes Stable, Unstable, and Unsorted. Each of these corresponds to `Vec::sort_by_key`, `Vec::sort_unstable_by_key`, and no sorting at all. The default is `Unstable`. The last one can be used as a default if users introduce a preliminary depth prepass. ## Performance This will not impact the performance of any batched phases, as it is still using a stable sort. 2D's only phase is unchanged. All 3D phases are unbatched currently, and will benefit from this change. On `many_cubes`, where the primary phase is opaque, this change sees a speed up from 907.02us -> 477.62us, a 47.35% reduction. 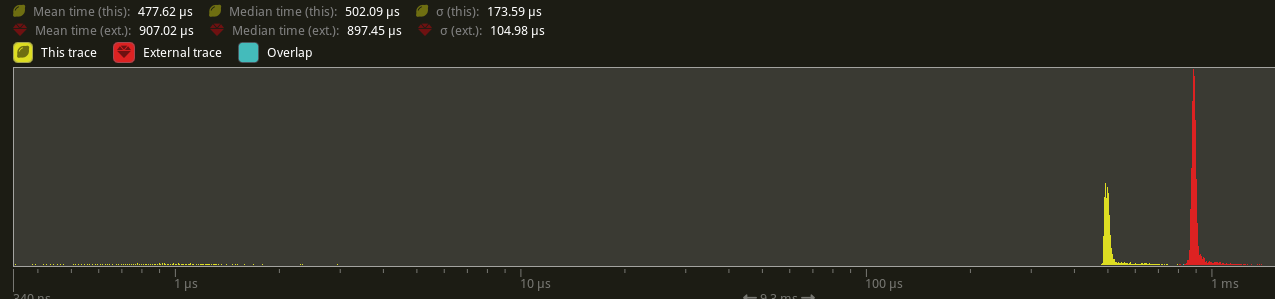 ## Future Work There were prior discussions to add support for faster radix sorts in #4291, which in theory should be a `O(n)` instead of a `O(nlog(n))` time. [`voracious`](https://crates.io/crates/voracious_radix_sort) has been proposed, but it seems to be optimize for use cases with more than 30,000 items, which may be atypical for most systems. Another optimization included in #4899 is to reduce the size of a few of the IDs commonly used in `PhaseItem` implementations to shrink the types to make swapping/sorting faster. Both `CachedPipelineId` and `DrawFunctionId` could be reduced to `u32` instead of `usize`. Ideally, this should automatically change to use stable sorts when `BatchedPhaseItem` is implemented on the same phase item type, but this requires specialization, which may not land in stable Rust for a short while. --- ## Changelog Added: `PhaseItem::sort` ## Migration Guide RenderPhases now default to a unstable sort (via `slice::sort_unstable_by_key`). This can typically improve sort phase performance, but may produce incorrect batching results when implementing `BatchedPhaseItem`. To revert to the older stable sort, manually implement `PhaseItem::sort` to implement a stable sort (i.e. via `slice::sort_by_key`). Co-authored-by: Federico Rinaldi <gisquerin@gmail.com> Co-authored-by: Robert Swain <robert.swain@gmail.com> Co-authored-by: colepoirier <colepoirier@gmail.com>
31 lines
1.1 KiB
TOML
31 lines
1.1 KiB
TOML
[package]
|
|
name = "bevy_pbr"
|
|
version = "0.8.0-dev"
|
|
edition = "2021"
|
|
description = "Adds PBR rendering to Bevy Engine"
|
|
homepage = "https://bevyengine.org"
|
|
repository = "https://github.com/bevyengine/bevy"
|
|
license = "MIT OR Apache-2.0"
|
|
keywords = ["bevy"]
|
|
|
|
[features]
|
|
webgl = []
|
|
|
|
[dependencies]
|
|
# bevy
|
|
bevy_app = { path = "../bevy_app", version = "0.8.0-dev" }
|
|
bevy_asset = { path = "../bevy_asset", version = "0.8.0-dev" }
|
|
bevy_core_pipeline = { path = "../bevy_core_pipeline", version = "0.8.0-dev" }
|
|
bevy_ecs = { path = "../bevy_ecs", version = "0.8.0-dev" }
|
|
bevy_math = { path = "../bevy_math", version = "0.8.0-dev" }
|
|
bevy_reflect = { path = "../bevy_reflect", version = "0.8.0-dev", features = ["bevy"] }
|
|
bevy_render = { path = "../bevy_render", version = "0.8.0-dev" }
|
|
bevy_transform = { path = "../bevy_transform", version = "0.8.0-dev" }
|
|
bevy_utils = { path = "../bevy_utils", version = "0.8.0-dev" }
|
|
bevy_window = { path = "../bevy_window", version = "0.8.0-dev" }
|
|
|
|
# other
|
|
bitflags = "1.2"
|
|
# direct dependency required for derive macro
|
|
bytemuck = { version = "1", features = ["derive"] }
|
|
radsort = "0.1"
|