mirror of
https://github.com/bevyengine/bevy
synced 2025-01-08 19:29:04 +00:00
# Objective - Closes #5262 - Fix color banding caused by quantization. ## Solution - Adds dithering to the tonemapping node from #3425. - This is inspired by Godot's default "debanding" shader: https://gist.github.com/belzecue/ - Unlike Godot: - debanding happens after tonemapping. My understanding is that this is preferred, because we are running the debanding at the last moment before quantization (`[f32, f32, f32, f32]` -> `f32`). This ensures we aren't biasing the dithering strength by applying it in a different (linear) color space. - This code instead uses and reference the origin source, Valve at GDC 2015 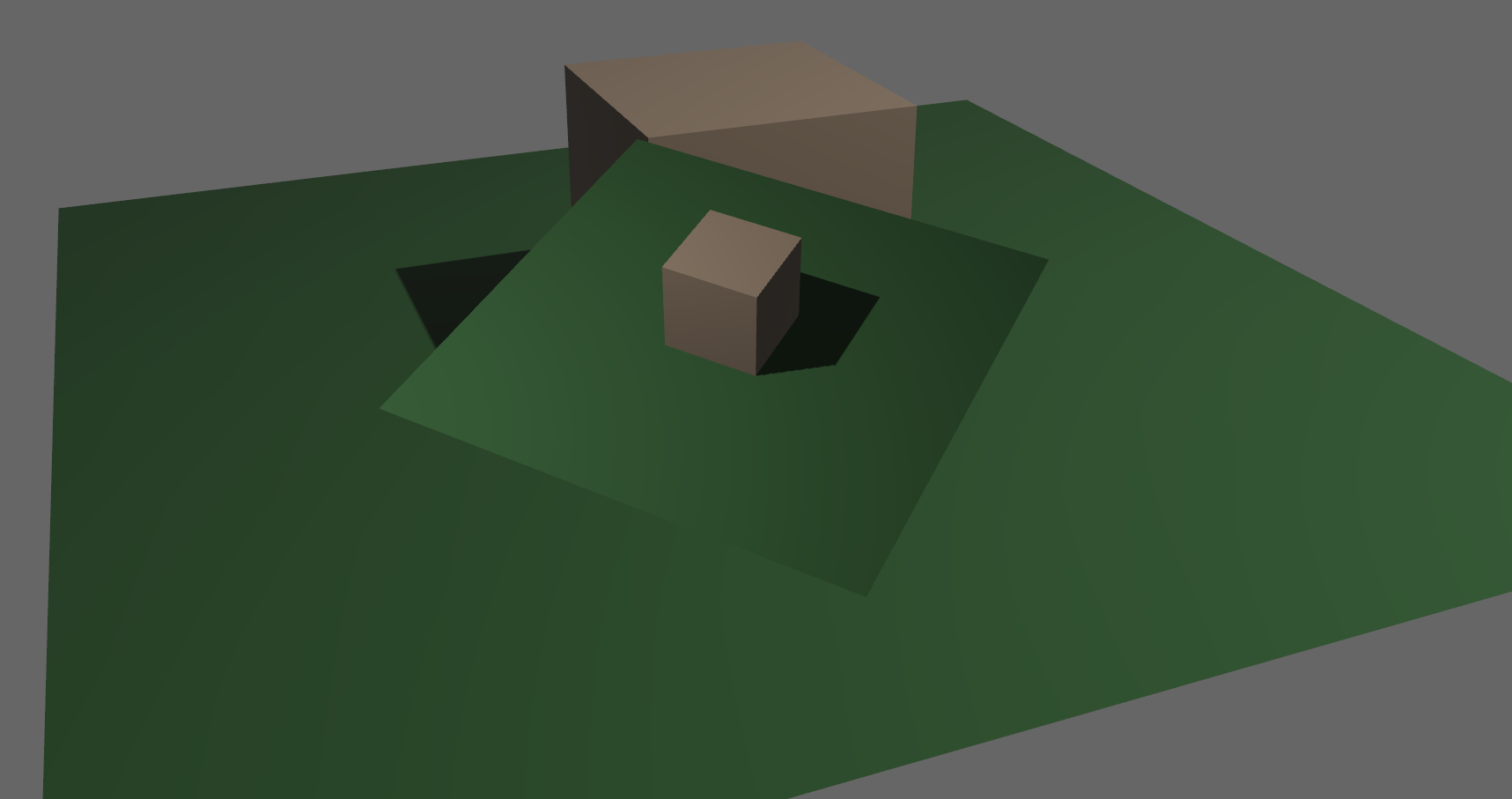 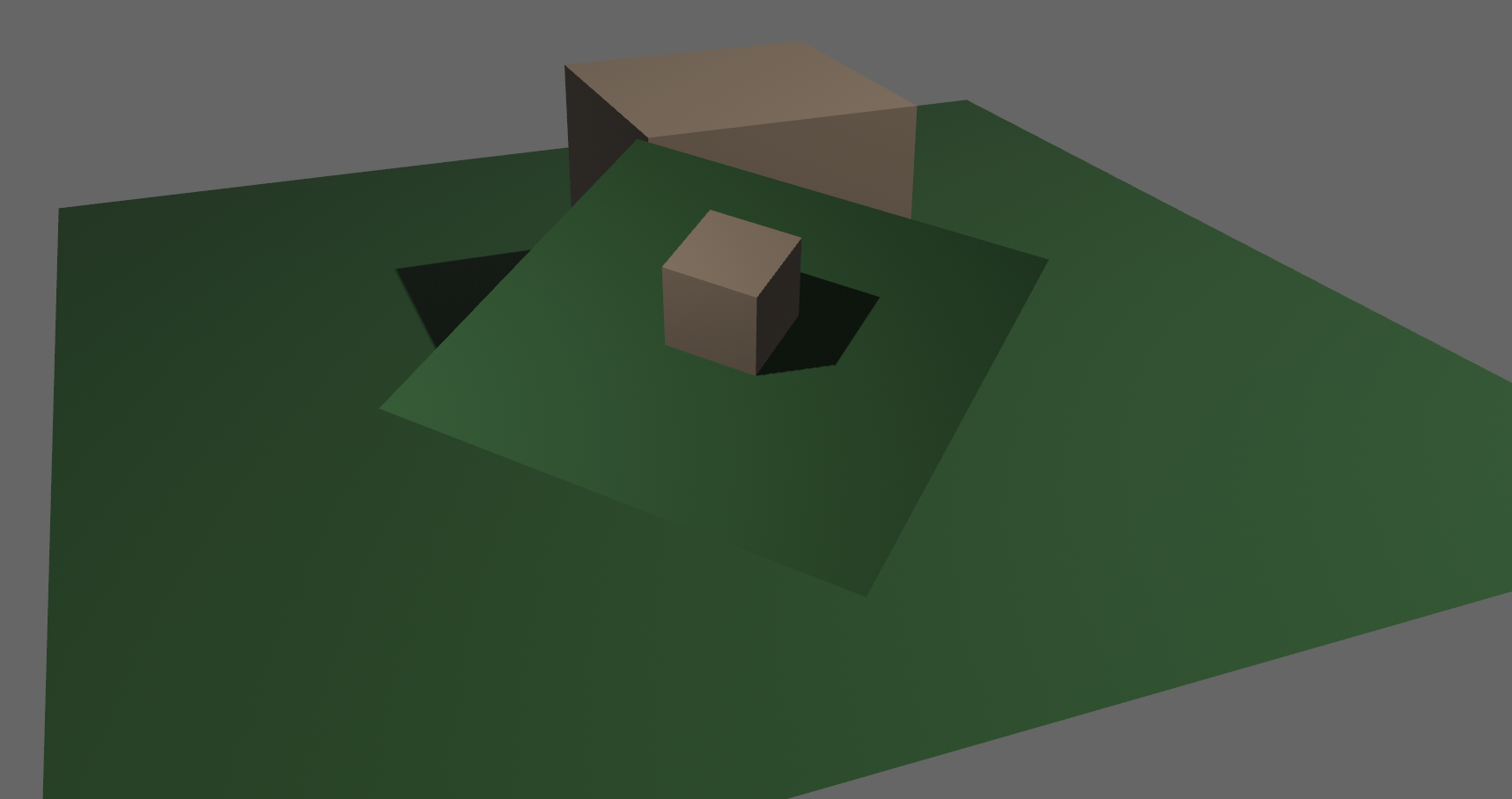 ## Additional Notes Real time rendering to standard dynamic range outputs is limited to 8 bits of depth per color channel. Internally we keep everything in full 32-bit precision (`vec4<f32>`) inside passes and 16-bit between passes until the image is ready to be displayed, at which point the GPU implicitly converts our `vec4<f32>` into a single 32bit value per pixel, with each channel (rgba) getting 8 of those 32 bits. ### The Problem 8 bits of color depth is simply not enough precision to make each step invisible - we only have 256 values per channel! Human vision can perceive steps in luma to about 14 bits of precision. When drawing a very slight gradient, the transition between steps become visible because with a gradient, neighboring pixels will all jump to the next "step" of precision at the same time. ### The Solution One solution is to simply output in HDR - more bits of color data means the transition between bands will become smaller. However, not everyone has hardware that supports 10+ bit color depth. Additionally, 10 bit color doesn't even fully solve the issue, banding will result in coherent bands on shallow gradients, but the steps will be harder to perceive. The solution in this PR adds noise to the signal before it is "quantized" or resampled from 32 to 8 bits. Done naively, it's easy to add unneeded noise to the image. To ensure dithering is correct and absolutely minimal, noise is adding *within* one step of the output color depth. When converting from the 32bit to 8bit signal, the value is rounded to the nearest 8 bit value (0 - 255). Banding occurs around the transition from one value to the next, let's say from 50-51. Dithering will never add more than +/-0.5 bits of noise, so the pixels near this transition might round to 50 instead of 51 but will never round more than one step. This means that the output image won't have excess variance: - in a gradient from 49 to 51, there will be a step between each band at 49, 50, and 51. - Done correctly, the modified image of this gradient will never have a adjacent pixels more than one step (0-255) from each other. - I.e. when scanning across the gradient you should expect to see: ``` |-band-| |-band-| |-band-| Baseline: 49 49 49 50 50 50 51 51 51 Dithered: 49 50 49 50 50 51 50 51 51 Dithered (wrong): 49 50 51 49 50 51 49 51 50 ```   You can see from above how correct dithering "fuzzes" the transition between bands to reduce distinct steps in color, without adding excess noise. ### HDR The previous section (and this PR) assumes the final output is to an 8-bit texture, however this is not always the case. When Bevy adds HDR support, the dithering code will need to take the per-channel depth into account instead of assuming it to be 0-255. Edit: I talked with Rob about this and it seems like the current solution is okay. We may need to revisit once we have actual HDR final image output. --- ## Changelog ### Added - All pipelines now support deband dithering. This is enabled by default in 3D, and can be toggled in the `Tonemapping` component in camera bundles. Banding is a graphical artifact created when the rendered image is crunched from high precision (f32 per color channel) down to the final output (u8 per channel in SDR). This results in subtle gradients becoming blocky due to the reduced color precision. Deband dithering applies a small amount of noise to the signal before it is "crunched", which breaks up the hard edges of blocks (bands) of color. Note that this does not add excess noise to the image, as the amount of noise is less than a single step of a color channel - just enough to break up the transition between color blocks in a gradient. Co-authored-by: Carter Anderson <mcanders1@gmail.com>
562 lines
20 KiB
Rust
562 lines
20 KiB
Rust
use bevy_app::{App, Plugin};
|
|
use bevy_asset::{AddAsset, AssetEvent, AssetServer, Assets, Handle};
|
|
use bevy_core_pipeline::{core_2d::Transparent2d, tonemapping::Tonemapping};
|
|
use bevy_derive::{Deref, DerefMut};
|
|
use bevy_ecs::{
|
|
entity::Entity,
|
|
event::EventReader,
|
|
prelude::{Bundle, World},
|
|
schedule::IntoSystemDescriptor,
|
|
system::{
|
|
lifetimeless::{Read, SQuery, SRes},
|
|
Commands, Local, Query, Res, ResMut, Resource, SystemParamItem,
|
|
},
|
|
world::FromWorld,
|
|
};
|
|
use bevy_log::error;
|
|
use bevy_reflect::TypeUuid;
|
|
use bevy_render::{
|
|
extract_component::ExtractComponentPlugin,
|
|
mesh::{Mesh, MeshVertexBufferLayout},
|
|
prelude::Image,
|
|
render_asset::{PrepareAssetLabel, RenderAssets},
|
|
render_phase::{
|
|
AddRenderCommand, DrawFunctions, EntityRenderCommand, RenderCommandResult, RenderPhase,
|
|
SetItemPipeline, TrackedRenderPass,
|
|
},
|
|
render_resource::{
|
|
AsBindGroup, AsBindGroupError, BindGroup, BindGroupLayout, OwnedBindingResource,
|
|
PipelineCache, RenderPipelineDescriptor, Shader, ShaderRef, SpecializedMeshPipeline,
|
|
SpecializedMeshPipelineError, SpecializedMeshPipelines,
|
|
},
|
|
renderer::RenderDevice,
|
|
texture::FallbackImage,
|
|
view::{ComputedVisibility, ExtractedView, Msaa, Visibility, VisibleEntities},
|
|
Extract, RenderApp, RenderStage,
|
|
};
|
|
use bevy_transform::components::{GlobalTransform, Transform};
|
|
use bevy_utils::{FloatOrd, HashMap, HashSet};
|
|
use std::hash::Hash;
|
|
use std::marker::PhantomData;
|
|
|
|
use crate::{
|
|
DrawMesh2d, Mesh2dHandle, Mesh2dPipeline, Mesh2dPipelineKey, Mesh2dUniform, SetMesh2dBindGroup,
|
|
SetMesh2dViewBindGroup,
|
|
};
|
|
|
|
/// Materials are used alongside [`Material2dPlugin`] and [`MaterialMesh2dBundle`]
|
|
/// to spawn entities that are rendered with a specific [`Material2d`] type. They serve as an easy to use high level
|
|
/// way to render [`Mesh2dHandle`] entities with custom shader logic.
|
|
///
|
|
/// Material2ds must implement [`AsBindGroup`] to define how data will be transferred to the GPU and bound in shaders.
|
|
/// [`AsBindGroup`] can be derived, which makes generating bindings straightforward. See the [`AsBindGroup`] docs for details.
|
|
///
|
|
/// Materials must also implement [`TypeUuid`] so they can be treated as an [`Asset`](bevy_asset::Asset).
|
|
///
|
|
/// # Example
|
|
///
|
|
/// Here is a simple Material2d implementation. The [`AsBindGroup`] derive has many features. To see what else is available,
|
|
/// check out the [`AsBindGroup`] documentation.
|
|
/// ```
|
|
/// # use bevy_sprite::{Material2d, MaterialMesh2dBundle};
|
|
/// # use bevy_ecs::prelude::*;
|
|
/// # use bevy_reflect::TypeUuid;
|
|
/// # use bevy_render::{render_resource::{AsBindGroup, ShaderRef}, texture::Image, color::Color};
|
|
/// # use bevy_asset::{Handle, AssetServer, Assets};
|
|
///
|
|
/// #[derive(AsBindGroup, TypeUuid, Debug, Clone)]
|
|
/// #[uuid = "f690fdae-d598-45ab-8225-97e2a3f056e0"]
|
|
/// pub struct CustomMaterial {
|
|
/// // Uniform bindings must implement `ShaderType`, which will be used to convert the value to
|
|
/// // its shader-compatible equivalent. Most core math types already implement `ShaderType`.
|
|
/// #[uniform(0)]
|
|
/// color: Color,
|
|
/// // Images can be bound as textures in shaders. If the Image's sampler is also needed, just
|
|
/// // add the sampler attribute with a different binding index.
|
|
/// #[texture(1)]
|
|
/// #[sampler(2)]
|
|
/// color_texture: Handle<Image>,
|
|
/// }
|
|
///
|
|
/// // All functions on `Material2d` have default impls. You only need to implement the
|
|
/// // functions that are relevant for your material.
|
|
/// impl Material2d for CustomMaterial {
|
|
/// fn fragment_shader() -> ShaderRef {
|
|
/// "shaders/custom_material.wgsl".into()
|
|
/// }
|
|
/// }
|
|
///
|
|
/// // Spawn an entity using `CustomMaterial`.
|
|
/// fn setup(mut commands: Commands, mut materials: ResMut<Assets<CustomMaterial>>, asset_server: Res<AssetServer>) {
|
|
/// commands.spawn(MaterialMesh2dBundle {
|
|
/// material: materials.add(CustomMaterial {
|
|
/// color: Color::RED,
|
|
/// color_texture: asset_server.load("some_image.png"),
|
|
/// }),
|
|
/// ..Default::default()
|
|
/// });
|
|
/// }
|
|
/// ```
|
|
/// In WGSL shaders, the material's binding would look like this:
|
|
///
|
|
/// ```wgsl
|
|
/// struct CustomMaterial {
|
|
/// color: vec4<f32>,
|
|
/// }
|
|
///
|
|
/// @group(1) @binding(0)
|
|
/// var<uniform> material: CustomMaterial;
|
|
/// @group(1) @binding(1)
|
|
/// var color_texture: texture_2d<f32>;
|
|
/// @group(1) @binding(2)
|
|
/// var color_sampler: sampler;
|
|
/// ```
|
|
pub trait Material2d: AsBindGroup + Send + Sync + Clone + TypeUuid + Sized + 'static {
|
|
/// Returns this material's vertex shader. If [`ShaderRef::Default`] is returned, the default mesh vertex shader
|
|
/// will be used.
|
|
fn vertex_shader() -> ShaderRef {
|
|
ShaderRef::Default
|
|
}
|
|
|
|
/// Returns this material's fragment shader. If [`ShaderRef::Default`] is returned, the default mesh fragment shader
|
|
/// will be used.
|
|
fn fragment_shader() -> ShaderRef {
|
|
ShaderRef::Default
|
|
}
|
|
|
|
/// Customizes the default [`RenderPipelineDescriptor`].
|
|
#[allow(unused_variables)]
|
|
#[inline]
|
|
fn specialize(
|
|
descriptor: &mut RenderPipelineDescriptor,
|
|
layout: &MeshVertexBufferLayout,
|
|
key: Material2dKey<Self>,
|
|
) -> Result<(), SpecializedMeshPipelineError> {
|
|
Ok(())
|
|
}
|
|
}
|
|
|
|
/// Adds the necessary ECS resources and render logic to enable rendering entities using the given [`Material2d`]
|
|
/// asset type (which includes [`Material2d`] types).
|
|
pub struct Material2dPlugin<M: Material2d>(PhantomData<M>);
|
|
|
|
impl<M: Material2d> Default for Material2dPlugin<M> {
|
|
fn default() -> Self {
|
|
Self(Default::default())
|
|
}
|
|
}
|
|
|
|
impl<M: Material2d> Plugin for Material2dPlugin<M>
|
|
where
|
|
M::Data: PartialEq + Eq + Hash + Clone,
|
|
{
|
|
fn build(&self, app: &mut App) {
|
|
app.add_asset::<M>()
|
|
.add_plugin(ExtractComponentPlugin::<Handle<M>>::extract_visible());
|
|
if let Ok(render_app) = app.get_sub_app_mut(RenderApp) {
|
|
render_app
|
|
.add_render_command::<Transparent2d, DrawMaterial2d<M>>()
|
|
.init_resource::<Material2dPipeline<M>>()
|
|
.init_resource::<ExtractedMaterials2d<M>>()
|
|
.init_resource::<RenderMaterials2d<M>>()
|
|
.init_resource::<SpecializedMeshPipelines<Material2dPipeline<M>>>()
|
|
.add_system_to_stage(RenderStage::Extract, extract_materials_2d::<M>)
|
|
.add_system_to_stage(
|
|
RenderStage::Prepare,
|
|
prepare_materials_2d::<M>.after(PrepareAssetLabel::PreAssetPrepare),
|
|
)
|
|
.add_system_to_stage(RenderStage::Queue, queue_material2d_meshes::<M>);
|
|
}
|
|
}
|
|
}
|
|
|
|
/// Render pipeline data for a given [`Material2d`]
|
|
#[derive(Resource)]
|
|
pub struct Material2dPipeline<M: Material2d> {
|
|
pub mesh2d_pipeline: Mesh2dPipeline,
|
|
pub material2d_layout: BindGroupLayout,
|
|
pub vertex_shader: Option<Handle<Shader>>,
|
|
pub fragment_shader: Option<Handle<Shader>>,
|
|
marker: PhantomData<M>,
|
|
}
|
|
|
|
pub struct Material2dKey<M: Material2d> {
|
|
pub mesh_key: Mesh2dPipelineKey,
|
|
pub bind_group_data: M::Data,
|
|
}
|
|
|
|
impl<M: Material2d> Eq for Material2dKey<M> where M::Data: PartialEq {}
|
|
|
|
impl<M: Material2d> PartialEq for Material2dKey<M>
|
|
where
|
|
M::Data: PartialEq,
|
|
{
|
|
fn eq(&self, other: &Self) -> bool {
|
|
self.mesh_key == other.mesh_key && self.bind_group_data == other.bind_group_data
|
|
}
|
|
}
|
|
|
|
impl<M: Material2d> Clone for Material2dKey<M>
|
|
where
|
|
M::Data: Clone,
|
|
{
|
|
fn clone(&self) -> Self {
|
|
Self {
|
|
mesh_key: self.mesh_key,
|
|
bind_group_data: self.bind_group_data.clone(),
|
|
}
|
|
}
|
|
}
|
|
|
|
impl<M: Material2d> Hash for Material2dKey<M>
|
|
where
|
|
M::Data: Hash,
|
|
{
|
|
fn hash<H: std::hash::Hasher>(&self, state: &mut H) {
|
|
self.mesh_key.hash(state);
|
|
self.bind_group_data.hash(state);
|
|
}
|
|
}
|
|
|
|
impl<M: Material2d> SpecializedMeshPipeline for Material2dPipeline<M>
|
|
where
|
|
M::Data: PartialEq + Eq + Hash + Clone,
|
|
{
|
|
type Key = Material2dKey<M>;
|
|
|
|
fn specialize(
|
|
&self,
|
|
key: Self::Key,
|
|
layout: &MeshVertexBufferLayout,
|
|
) -> Result<RenderPipelineDescriptor, SpecializedMeshPipelineError> {
|
|
let mut descriptor = self.mesh2d_pipeline.specialize(key.mesh_key, layout)?;
|
|
if let Some(vertex_shader) = &self.vertex_shader {
|
|
descriptor.vertex.shader = vertex_shader.clone();
|
|
}
|
|
|
|
if let Some(fragment_shader) = &self.fragment_shader {
|
|
descriptor.fragment.as_mut().unwrap().shader = fragment_shader.clone();
|
|
}
|
|
descriptor.layout = Some(vec![
|
|
self.mesh2d_pipeline.view_layout.clone(),
|
|
self.material2d_layout.clone(),
|
|
self.mesh2d_pipeline.mesh_layout.clone(),
|
|
]);
|
|
|
|
M::specialize(&mut descriptor, layout, key)?;
|
|
Ok(descriptor)
|
|
}
|
|
}
|
|
|
|
impl<M: Material2d> FromWorld for Material2dPipeline<M> {
|
|
fn from_world(world: &mut World) -> Self {
|
|
let asset_server = world.resource::<AssetServer>();
|
|
let render_device = world.resource::<RenderDevice>();
|
|
let material2d_layout = M::bind_group_layout(render_device);
|
|
|
|
Material2dPipeline {
|
|
mesh2d_pipeline: world.resource::<Mesh2dPipeline>().clone(),
|
|
material2d_layout,
|
|
vertex_shader: match M::vertex_shader() {
|
|
ShaderRef::Default => None,
|
|
ShaderRef::Handle(handle) => Some(handle),
|
|
ShaderRef::Path(path) => Some(asset_server.load(path)),
|
|
},
|
|
fragment_shader: match M::fragment_shader() {
|
|
ShaderRef::Default => None,
|
|
ShaderRef::Handle(handle) => Some(handle),
|
|
ShaderRef::Path(path) => Some(asset_server.load(path)),
|
|
},
|
|
marker: PhantomData,
|
|
}
|
|
}
|
|
}
|
|
|
|
type DrawMaterial2d<M> = (
|
|
SetItemPipeline,
|
|
SetMesh2dViewBindGroup<0>,
|
|
SetMaterial2dBindGroup<M, 1>,
|
|
SetMesh2dBindGroup<2>,
|
|
DrawMesh2d,
|
|
);
|
|
|
|
pub struct SetMaterial2dBindGroup<M: Material2d, const I: usize>(PhantomData<M>);
|
|
impl<M: Material2d, const I: usize> EntityRenderCommand for SetMaterial2dBindGroup<M, I> {
|
|
type Param = (SRes<RenderMaterials2d<M>>, SQuery<Read<Handle<M>>>);
|
|
fn render<'w>(
|
|
_view: Entity,
|
|
item: Entity,
|
|
(materials, query): SystemParamItem<'w, '_, Self::Param>,
|
|
pass: &mut TrackedRenderPass<'w>,
|
|
) -> RenderCommandResult {
|
|
let material2d_handle = query.get(item).unwrap();
|
|
let material2d = materials.into_inner().get(material2d_handle).unwrap();
|
|
pass.set_bind_group(I, &material2d.bind_group, &[]);
|
|
RenderCommandResult::Success
|
|
}
|
|
}
|
|
|
|
#[allow(clippy::too_many_arguments)]
|
|
pub fn queue_material2d_meshes<M: Material2d>(
|
|
transparent_draw_functions: Res<DrawFunctions<Transparent2d>>,
|
|
material2d_pipeline: Res<Material2dPipeline<M>>,
|
|
mut pipelines: ResMut<SpecializedMeshPipelines<Material2dPipeline<M>>>,
|
|
mut pipeline_cache: ResMut<PipelineCache>,
|
|
msaa: Res<Msaa>,
|
|
render_meshes: Res<RenderAssets<Mesh>>,
|
|
render_materials: Res<RenderMaterials2d<M>>,
|
|
material2d_meshes: Query<(&Handle<M>, &Mesh2dHandle, &Mesh2dUniform)>,
|
|
mut views: Query<(
|
|
&ExtractedView,
|
|
&VisibleEntities,
|
|
Option<&Tonemapping>,
|
|
&mut RenderPhase<Transparent2d>,
|
|
)>,
|
|
) where
|
|
M::Data: PartialEq + Eq + Hash + Clone,
|
|
{
|
|
if material2d_meshes.is_empty() {
|
|
return;
|
|
}
|
|
|
|
for (view, visible_entities, tonemapping, mut transparent_phase) in &mut views {
|
|
let draw_transparent_pbr = transparent_draw_functions
|
|
.read()
|
|
.get_id::<DrawMaterial2d<M>>()
|
|
.unwrap();
|
|
|
|
let mut view_key = Mesh2dPipelineKey::from_msaa_samples(msaa.samples)
|
|
| Mesh2dPipelineKey::from_hdr(view.hdr);
|
|
|
|
if let Some(Tonemapping::Enabled { deband_dither }) = tonemapping {
|
|
if !view.hdr {
|
|
view_key |= Mesh2dPipelineKey::TONEMAP_IN_SHADER;
|
|
|
|
if *deband_dither {
|
|
view_key |= Mesh2dPipelineKey::DEBAND_DITHER;
|
|
}
|
|
}
|
|
}
|
|
|
|
for visible_entity in &visible_entities.entities {
|
|
if let Ok((material2d_handle, mesh2d_handle, mesh2d_uniform)) =
|
|
material2d_meshes.get(*visible_entity)
|
|
{
|
|
if let Some(material2d) = render_materials.get(material2d_handle) {
|
|
if let Some(mesh) = render_meshes.get(&mesh2d_handle.0) {
|
|
let mesh_key = view_key
|
|
| Mesh2dPipelineKey::from_primitive_topology(mesh.primitive_topology);
|
|
|
|
let pipeline_id = pipelines.specialize(
|
|
&mut pipeline_cache,
|
|
&material2d_pipeline,
|
|
Material2dKey {
|
|
mesh_key,
|
|
bind_group_data: material2d.key.clone(),
|
|
},
|
|
&mesh.layout,
|
|
);
|
|
|
|
let pipeline_id = match pipeline_id {
|
|
Ok(id) => id,
|
|
Err(err) => {
|
|
error!("{}", err);
|
|
continue;
|
|
}
|
|
};
|
|
|
|
let mesh_z = mesh2d_uniform.transform.w_axis.z;
|
|
transparent_phase.add(Transparent2d {
|
|
entity: *visible_entity,
|
|
draw_function: draw_transparent_pbr,
|
|
pipeline: pipeline_id,
|
|
// NOTE: Back-to-front ordering for transparent with ascending sort means far should have the
|
|
// lowest sort key and getting closer should increase. As we have
|
|
// -z in front of the camera, the largest distance is -far with values increasing toward the
|
|
// camera. As such we can just use mesh_z as the distance
|
|
sort_key: FloatOrd(mesh_z),
|
|
// This material is not batched
|
|
batch_range: None,
|
|
});
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
/// Data prepared for a [`Material2d`] instance.
|
|
pub struct PreparedMaterial2d<T: Material2d> {
|
|
pub bindings: Vec<OwnedBindingResource>,
|
|
pub bind_group: BindGroup,
|
|
pub key: T::Data,
|
|
}
|
|
|
|
#[derive(Resource)]
|
|
struct ExtractedMaterials2d<M: Material2d> {
|
|
extracted: Vec<(Handle<M>, M)>,
|
|
removed: Vec<Handle<M>>,
|
|
}
|
|
|
|
impl<M: Material2d> Default for ExtractedMaterials2d<M> {
|
|
fn default() -> Self {
|
|
Self {
|
|
extracted: Default::default(),
|
|
removed: Default::default(),
|
|
}
|
|
}
|
|
}
|
|
|
|
/// Stores all prepared representations of [`Material2d`] assets for as long as they exist.
|
|
#[derive(Resource, Deref, DerefMut)]
|
|
pub struct RenderMaterials2d<T: Material2d>(HashMap<Handle<T>, PreparedMaterial2d<T>>);
|
|
|
|
impl<T: Material2d> Default for RenderMaterials2d<T> {
|
|
fn default() -> Self {
|
|
Self(Default::default())
|
|
}
|
|
}
|
|
|
|
/// This system extracts all created or modified assets of the corresponding [`Material2d`] type
|
|
/// into the "render world".
|
|
fn extract_materials_2d<M: Material2d>(
|
|
mut commands: Commands,
|
|
mut events: Extract<EventReader<AssetEvent<M>>>,
|
|
assets: Extract<Res<Assets<M>>>,
|
|
) {
|
|
let mut changed_assets = HashSet::default();
|
|
let mut removed = Vec::new();
|
|
for event in events.iter() {
|
|
match event {
|
|
AssetEvent::Created { handle } | AssetEvent::Modified { handle } => {
|
|
changed_assets.insert(handle.clone_weak());
|
|
}
|
|
AssetEvent::Removed { handle } => {
|
|
changed_assets.remove(handle);
|
|
removed.push(handle.clone_weak());
|
|
}
|
|
}
|
|
}
|
|
|
|
let mut extracted_assets = Vec::new();
|
|
for handle in changed_assets.drain() {
|
|
if let Some(asset) = assets.get(&handle) {
|
|
extracted_assets.push((handle, asset.clone()));
|
|
}
|
|
}
|
|
|
|
commands.insert_resource(ExtractedMaterials2d {
|
|
extracted: extracted_assets,
|
|
removed,
|
|
});
|
|
}
|
|
|
|
/// All [`Material2d`] values of a given type that should be prepared next frame.
|
|
pub struct PrepareNextFrameMaterials<M: Material2d> {
|
|
assets: Vec<(Handle<M>, M)>,
|
|
}
|
|
|
|
impl<M: Material2d> Default for PrepareNextFrameMaterials<M> {
|
|
fn default() -> Self {
|
|
Self {
|
|
assets: Default::default(),
|
|
}
|
|
}
|
|
}
|
|
|
|
/// This system prepares all assets of the corresponding [`Material2d`] type
|
|
/// which where extracted this frame for the GPU.
|
|
fn prepare_materials_2d<M: Material2d>(
|
|
mut prepare_next_frame: Local<PrepareNextFrameMaterials<M>>,
|
|
mut extracted_assets: ResMut<ExtractedMaterials2d<M>>,
|
|
mut render_materials: ResMut<RenderMaterials2d<M>>,
|
|
render_device: Res<RenderDevice>,
|
|
images: Res<RenderAssets<Image>>,
|
|
fallback_image: Res<FallbackImage>,
|
|
pipeline: Res<Material2dPipeline<M>>,
|
|
) {
|
|
let queued_assets = std::mem::take(&mut prepare_next_frame.assets);
|
|
for (handle, material) in queued_assets {
|
|
match prepare_material2d(
|
|
&material,
|
|
&render_device,
|
|
&images,
|
|
&fallback_image,
|
|
&pipeline,
|
|
) {
|
|
Ok(prepared_asset) => {
|
|
render_materials.insert(handle, prepared_asset);
|
|
}
|
|
Err(AsBindGroupError::RetryNextUpdate) => {
|
|
prepare_next_frame.assets.push((handle, material));
|
|
}
|
|
}
|
|
}
|
|
|
|
for removed in std::mem::take(&mut extracted_assets.removed) {
|
|
render_materials.remove(&removed);
|
|
}
|
|
|
|
for (handle, material) in std::mem::take(&mut extracted_assets.extracted) {
|
|
match prepare_material2d(
|
|
&material,
|
|
&render_device,
|
|
&images,
|
|
&fallback_image,
|
|
&pipeline,
|
|
) {
|
|
Ok(prepared_asset) => {
|
|
render_materials.insert(handle, prepared_asset);
|
|
}
|
|
Err(AsBindGroupError::RetryNextUpdate) => {
|
|
prepare_next_frame.assets.push((handle, material));
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
fn prepare_material2d<M: Material2d>(
|
|
material: &M,
|
|
render_device: &RenderDevice,
|
|
images: &RenderAssets<Image>,
|
|
fallback_image: &FallbackImage,
|
|
pipeline: &Material2dPipeline<M>,
|
|
) -> Result<PreparedMaterial2d<M>, AsBindGroupError> {
|
|
let prepared = material.as_bind_group(
|
|
&pipeline.material2d_layout,
|
|
render_device,
|
|
images,
|
|
fallback_image,
|
|
)?;
|
|
Ok(PreparedMaterial2d {
|
|
bindings: prepared.bindings,
|
|
bind_group: prepared.bind_group,
|
|
key: prepared.data,
|
|
})
|
|
}
|
|
|
|
/// A component bundle for entities with a [`Mesh2dHandle`] and a [`Material2d`].
|
|
#[derive(Bundle, Clone)]
|
|
pub struct MaterialMesh2dBundle<M: Material2d> {
|
|
pub mesh: Mesh2dHandle,
|
|
pub material: Handle<M>,
|
|
pub transform: Transform,
|
|
pub global_transform: GlobalTransform,
|
|
/// User indication of whether an entity is visible
|
|
pub visibility: Visibility,
|
|
/// Algorithmically-computed indication of whether an entity is visible and should be extracted for rendering
|
|
pub computed_visibility: ComputedVisibility,
|
|
}
|
|
|
|
impl<M: Material2d> Default for MaterialMesh2dBundle<M> {
|
|
fn default() -> Self {
|
|
Self {
|
|
mesh: Default::default(),
|
|
material: Default::default(),
|
|
transform: Default::default(),
|
|
global_transform: Default::default(),
|
|
visibility: Default::default(),
|
|
computed_visibility: Default::default(),
|
|
}
|
|
}
|
|
}
|