mirror of
https://github.com/bevyengine/bevy
synced 2024-12-25 20:43:07 +00:00
Adopted #8266, so copy-pasting the description from there: # Objective Support the KHR_texture_transform extension for the glTF loader. - Fixes #6335 - Fixes #11869 - Implements part of #11350 - Implements the GLTF part of #399 ## Solution As is, this only supports a single transform. Looking at Godot's source, they support one transform with an optional second one for detail, AO, and emission. glTF specifies one per texture. The public domain materials I looked at seem to share the same transform. So maybe having just one is acceptable for now. I tried to include a warning if multiple different transforms exist for the same material. Note the gltf crate doesn't expose the texture transform for the normal and occlusion textures, which it should, so I just ignored those for now. (note by @janhohenheim: this is still the case) Via `cargo run --release --example scene_viewer ~/src/clone/glTF-Sample-Models/2.0/TextureTransformTest/glTF/TextureTransformTest.gltf`: 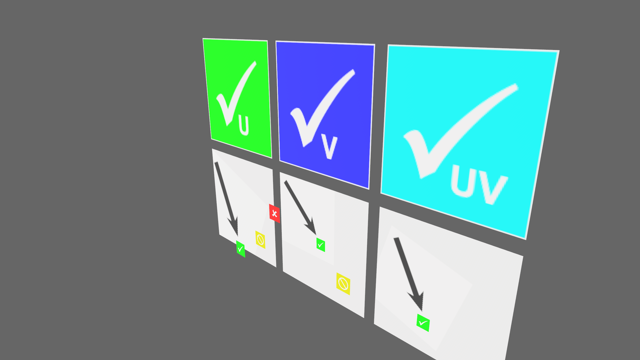 ## Changelog Support for the [KHR_texture_transform](https://github.com/KhronosGroup/glTF/tree/main/extensions/2.0/Khronos/KHR_texture_transform) extension added. Texture UVs that were scaled, rotated, or offset in a GLTF are now properly handled. --------- Co-authored-by: Al McElrath <hello@yrns.org> Co-authored-by: Kanabenki <lucien.menassol@gmail.com>
63 lines
1.8 KiB
WebGPU Shading Language
63 lines
1.8 KiB
WebGPU Shading Language
#ifdef TONEMAP_IN_SHADER
|
|
#import bevy_core_pipeline::tonemapping
|
|
#endif
|
|
|
|
#import bevy_render::{
|
|
maths::affine3_to_square,
|
|
view::View,
|
|
}
|
|
|
|
@group(0) @binding(0) var<uniform> view: View;
|
|
|
|
struct VertexInput {
|
|
@builtin(vertex_index) index: u32,
|
|
// NOTE: Instance-rate vertex buffer members prefixed with i_
|
|
// NOTE: i_model_transpose_colN are the 3 columns of a 3x4 matrix that is the transpose of the
|
|
// affine 4x3 model matrix.
|
|
@location(0) i_model_transpose_col0: vec4<f32>,
|
|
@location(1) i_model_transpose_col1: vec4<f32>,
|
|
@location(2) i_model_transpose_col2: vec4<f32>,
|
|
@location(3) i_color: vec4<f32>,
|
|
@location(4) i_uv_offset_scale: vec4<f32>,
|
|
}
|
|
|
|
struct VertexOutput {
|
|
@builtin(position) clip_position: vec4<f32>,
|
|
@location(0) uv: vec2<f32>,
|
|
@location(1) @interpolate(flat) color: vec4<f32>,
|
|
};
|
|
|
|
@vertex
|
|
fn vertex(in: VertexInput) -> VertexOutput {
|
|
var out: VertexOutput;
|
|
|
|
let vertex_position = vec3<f32>(
|
|
f32(in.index & 0x1u),
|
|
f32((in.index & 0x2u) >> 1u),
|
|
0.0
|
|
);
|
|
|
|
out.clip_position = view.view_proj * affine3_to_square(mat3x4<f32>(
|
|
in.i_model_transpose_col0,
|
|
in.i_model_transpose_col1,
|
|
in.i_model_transpose_col2,
|
|
)) * vec4<f32>(vertex_position, 1.0);
|
|
out.uv = vec2<f32>(vertex_position.xy) * in.i_uv_offset_scale.zw + in.i_uv_offset_scale.xy;
|
|
out.color = in.i_color;
|
|
|
|
return out;
|
|
}
|
|
|
|
@group(1) @binding(0) var sprite_texture: texture_2d<f32>;
|
|
@group(1) @binding(1) var sprite_sampler: sampler;
|
|
|
|
@fragment
|
|
fn fragment(in: VertexOutput) -> @location(0) vec4<f32> {
|
|
var color = in.color * textureSample(sprite_texture, sprite_sampler, in.uv);
|
|
|
|
#ifdef TONEMAP_IN_SHADER
|
|
color = tonemapping::tone_mapping(color, view.color_grading);
|
|
#endif
|
|
|
|
return color;
|
|
}
|