mirror of
https://github.com/bevyengine/bevy
synced 2024-11-10 07:04:33 +00:00
Added offset
parameter to TextureAtlas::from_grid_with_padding
(#4836)
# Objective Increase compatibility with a fairly common format of padded spritesheets, in which half the padding value occurs before the first sprite box begins. The original behaviour falls out when `Vec2::ZERO` is used for `offset`. See below unity screenshot for an example of a spritesheet with padding 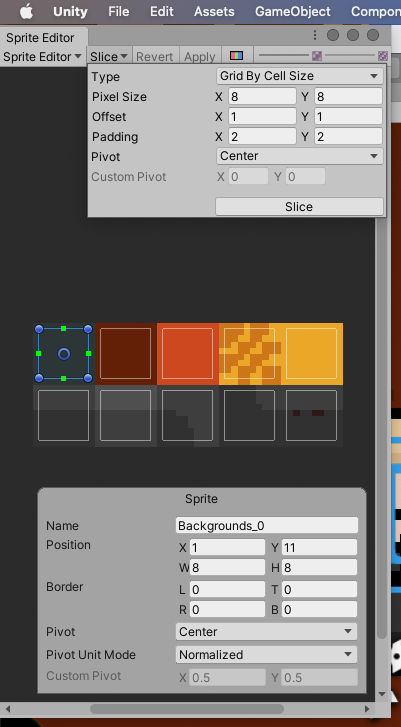 ## Solution Tiny change to `crates/bevy_sprite/src/texture_atlas.rs` ## Migration Guide Calls to `TextureAtlas::from_grid_with_padding` should be modified to include a new parameter, which can be set to `Vec2::ZERO` to retain old behaviour. ```rust from_grid_with_padding(texture, tile_size, columns, rows, padding) | V from_grid_with_padding(texture, tile_size, columns, rows, padding, Vec2::ZERO) ``` Co-authored-by: FraserLee <30442265+FraserLee@users.noreply.github.com>
This commit is contained in:
parent
fb813a3a64
commit
575ffa7c0c
1 changed files with 8 additions and 6 deletions
|
@ -68,25 +68,27 @@ impl TextureAtlas {
|
|||
}
|
||||
|
||||
/// Generate a `TextureAtlas` by splitting a texture into a grid where each
|
||||
/// cell of the grid of `tile_size` is one of the textures in the atlas
|
||||
/// `tile_size` by `tile_size` grid-cell is one of the textures in the atlas
|
||||
pub fn from_grid(
|
||||
texture: Handle<Image>,
|
||||
tile_size: Vec2,
|
||||
columns: usize,
|
||||
rows: usize,
|
||||
) -> TextureAtlas {
|
||||
Self::from_grid_with_padding(texture, tile_size, columns, rows, Vec2::new(0f32, 0f32))
|
||||
Self::from_grid_with_padding(texture, tile_size, columns, rows, Vec2::ZERO, Vec2::ZERO)
|
||||
}
|
||||
|
||||
/// Generate a `TextureAtlas` by splitting a texture into a grid where each
|
||||
/// cell of the grid of `tile_size` is one of the textures in the atlas and is separated by
|
||||
/// some `padding` in the texture
|
||||
/// `tile_size` by `tile_size` grid-cell is one of the textures in the
|
||||
/// atlas. Grid cells are separated by some `padding`, and the grid starts
|
||||
/// at `offset` pixels from the top left corner.
|
||||
pub fn from_grid_with_padding(
|
||||
texture: Handle<Image>,
|
||||
tile_size: Vec2,
|
||||
columns: usize,
|
||||
rows: usize,
|
||||
padding: Vec2,
|
||||
offset: Vec2,
|
||||
) -> TextureAtlas {
|
||||
let mut sprites = Vec::new();
|
||||
let mut x_padding = 0.0;
|
||||
|
@ -102,8 +104,8 @@ impl TextureAtlas {
|
|||
}
|
||||
|
||||
let rect_min = Vec2::new(
|
||||
(tile_size.x + x_padding) * x as f32,
|
||||
(tile_size.y + y_padding) * y as f32,
|
||||
(tile_size.x + x_padding) * x as f32 + offset.x,
|
||||
(tile_size.y + y_padding) * y as f32 + offset.y,
|
||||
);
|
||||
|
||||
sprites.push(Rect {
|
||||
|
|
Loading…
Reference in a new issue