mirror of
https://github.com/bevyengine/bevy
synced 2024-11-10 07:04:33 +00:00
Spherical area lights example (#3498)
I was putting together the Bevy 0.6 release blog post and wanted a simple area light radius example, so here we are :) 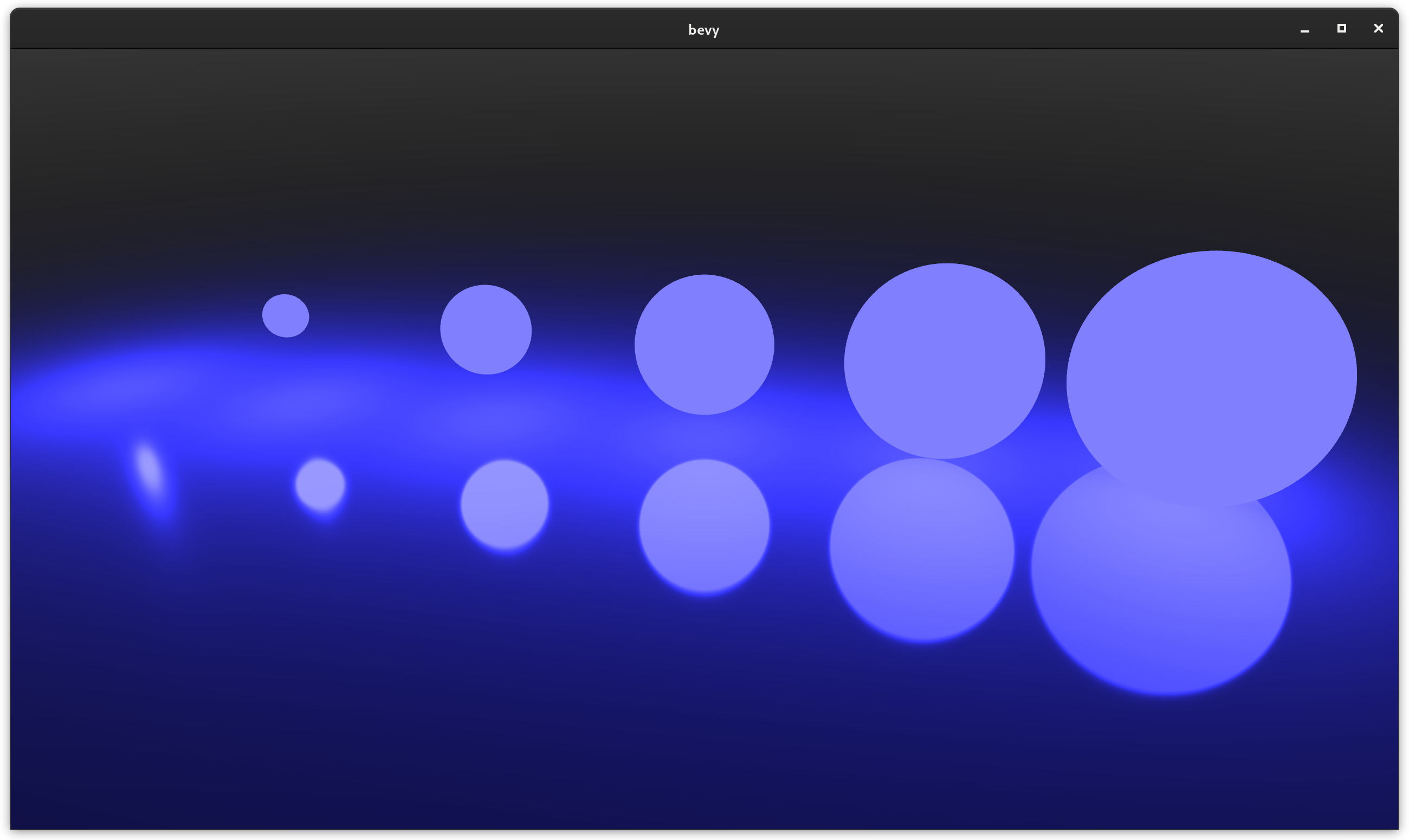
This commit is contained in:
parent
c641a905dc
commit
1d0d8a3397
3 changed files with 78 additions and 0 deletions
|
@ -181,6 +181,10 @@ path = "examples/3d/shadow_biases.rs"
|
|||
name = "shadow_caster_receiver"
|
||||
path = "examples/3d/shadow_caster_receiver.rs"
|
||||
|
||||
[[example]]
|
||||
name = "spherical_area_lights"
|
||||
path = "examples/3d/spherical_area_lights.rs"
|
||||
|
||||
[[example]]
|
||||
name = "texture"
|
||||
path = "examples/3d/texture.rs"
|
||||
|
|
73
examples/3d/spherical_area_lights.rs
Normal file
73
examples/3d/spherical_area_lights.rs
Normal file
|
@ -0,0 +1,73 @@
|
|||
use bevy::prelude::*;
|
||||
|
||||
fn main() {
|
||||
App::new()
|
||||
.insert_resource(ClearColor(Color::BLACK))
|
||||
.add_plugins(DefaultPlugins)
|
||||
.add_startup_system(setup)
|
||||
.run();
|
||||
}
|
||||
|
||||
fn setup(
|
||||
mut commands: Commands,
|
||||
mut meshes: ResMut<Assets<Mesh>>,
|
||||
mut materials: ResMut<Assets<StandardMaterial>>,
|
||||
) {
|
||||
// camera
|
||||
commands.spawn_bundle(PerspectiveCameraBundle {
|
||||
transform: Transform::from_xyz(1.0, 2.5, 5.0).looking_at(Vec3::ZERO, Vec3::Y),
|
||||
..Default::default()
|
||||
});
|
||||
|
||||
// plane
|
||||
commands.spawn_bundle(PbrBundle {
|
||||
mesh: meshes.add(Mesh::from(shape::Plane { size: 100.0 })),
|
||||
material: materials.add(StandardMaterial {
|
||||
base_color: Color::rgb(0.2, 0.2, 0.2),
|
||||
perceptual_roughness: 0.08,
|
||||
..Default::default()
|
||||
}),
|
||||
..Default::default()
|
||||
});
|
||||
|
||||
const COUNT: usize = 6;
|
||||
let position_range = -4.0..4.0;
|
||||
let radius_range = 0.0..0.8;
|
||||
let pos_len = position_range.end - position_range.start;
|
||||
let radius_len = radius_range.end - radius_range.start;
|
||||
let mesh = meshes.add(Mesh::from(shape::UVSphere {
|
||||
sectors: 128,
|
||||
stacks: 64,
|
||||
..Default::default()
|
||||
}));
|
||||
|
||||
for i in 0..COUNT {
|
||||
let percent = i as f32 / COUNT as f32;
|
||||
let radius = radius_range.start + percent * radius_len;
|
||||
|
||||
// sphere light
|
||||
commands
|
||||
.spawn_bundle(PbrBundle {
|
||||
mesh: mesh.clone(),
|
||||
material: materials.add(StandardMaterial {
|
||||
base_color: Color::rgb(0.5, 0.5, 1.0),
|
||||
unlit: true,
|
||||
..Default::default()
|
||||
}),
|
||||
transform: Transform::from_xyz(position_range.start + percent * pos_len, 0.6, 0.0)
|
||||
.with_scale(Vec3::splat(radius)),
|
||||
..Default::default()
|
||||
})
|
||||
.with_children(|children| {
|
||||
children.spawn_bundle(PointLightBundle {
|
||||
point_light: PointLight {
|
||||
intensity: 1500.0,
|
||||
radius,
|
||||
color: Color::rgb(0.2, 0.2, 1.0),
|
||||
..Default::default()
|
||||
},
|
||||
..Default::default()
|
||||
});
|
||||
});
|
||||
}
|
||||
}
|
|
@ -105,6 +105,7 @@ Example | File | Description
|
|||
`pbr` | [`3d/pbr.rs`](./3d/pbr.rs) | Demonstrates use of Physically Based Rendering (PBR) properties
|
||||
`shadow_caster_receiver` | [`3d/shadow_caster_receiver.rs`](./3d/shadow_caster_receiver.rs) | Demonstrates how to prevent meshes from casting/receiving shadows in a 3d scene
|
||||
`shadow_biases` | [`3d/shadow_biases.rs`](./3d/shadow_biases.rs) | Demonstrates how shadow biases affect shadows in a 3d scene
|
||||
`spherical_area_lights` | [`3d/spherical_area_lights.rs`](./3d/spherical_area_lights.rs) | Demonstrates how point light radius values affect light behavior.
|
||||
`texture` | [`3d/texture.rs`](./3d/texture.rs) | Shows configuration of texture materials
|
||||
`update_gltf_scene` | [`3d/update_gltf_scene.rs`](./3d/update_gltf_scene.rs) | Update a scene from a gltf file, either by spawning the scene as a child of another entity, or by accessing the entities of the scene
|
||||
`wireframe` | [`3d/wireframe.rs`](./3d/wireframe.rs) | Showcases wireframe rendering
|
||||
|
|
Loading…
Reference in a new issue