2022-06-06 17:52:09 +00:00
|
|
|
//! Demonstrates how to use transparency with UI.
|
|
|
|
//! Shows two colored buttons with transparent text.
|
|
|
|
|
|
|
|
use bevy::prelude::*;
|
|
|
|
|
|
|
|
fn main() {
|
|
|
|
App::new()
|
2024-02-24 21:35:32 +00:00
|
|
|
.insert_resource(ClearColor(LegacyColor::BLACK))
|
2022-06-06 17:52:09 +00:00
|
|
|
.add_plugins(DefaultPlugins)
|
2023-03-18 01:45:34 +00:00
|
|
|
.add_systems(Startup, setup)
|
2022-06-06 17:52:09 +00:00
|
|
|
.run();
|
|
|
|
}
|
|
|
|
|
|
|
|
fn setup(mut commands: Commands, asset_server: Res<AssetServer>) {
|
Spawn now takes a Bundle (#6054)
# Objective
Now that we can consolidate Bundles and Components under a single insert (thanks to #2975 and #6039), almost 100% of world spawns now look like `world.spawn().insert((Some, Tuple, Here))`. Spawning an entity without any components is an extremely uncommon pattern, so it makes sense to give spawn the "first class" ergonomic api. This consolidated api should be made consistent across all spawn apis (such as World and Commands).
## Solution
All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input:
```rust
// before:
commands
.spawn()
.insert((A, B, C));
world
.spawn()
.insert((A, B, C);
// after
commands.spawn((A, B, C));
world.spawn((A, B, C));
```
All existing instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api. A new `spawn_empty` has been added, replacing the old `spawn` api.
By allowing `world.spawn(some_bundle)` to replace `world.spawn().insert(some_bundle)`, this opened the door to removing the initial entity allocation in the "empty" archetype / table done in `spawn()` (and subsequent move to the actual archetype in `.insert(some_bundle)`).
This improves spawn performance by over 10%:
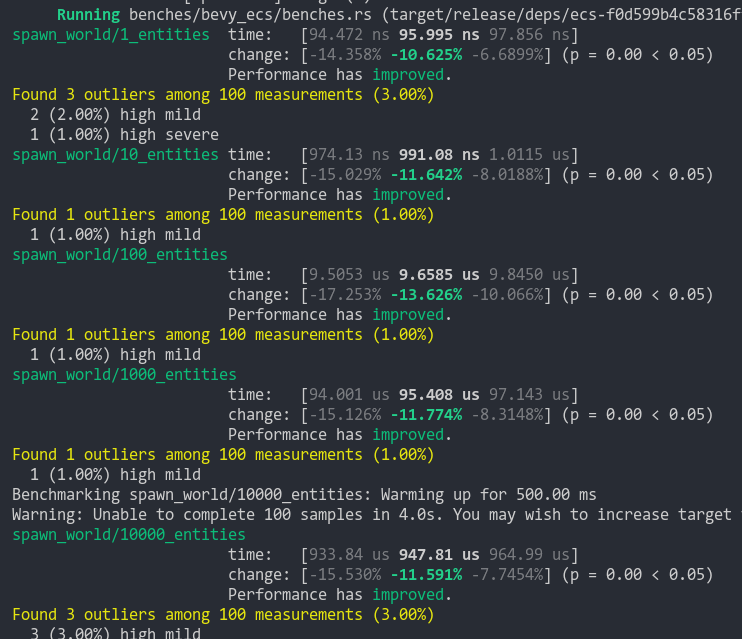
To take this measurement, I added a new `world_spawn` benchmark.
Unfortunately, optimizing `Commands::spawn` is slightly less trivial, as Commands expose the Entity id of spawned entities prior to actually spawning. Doing the optimization would (naively) require assurances that the `spawn(some_bundle)` command is applied before all other commands involving the entity (which would not necessarily be true, if memory serves). Optimizing `Commands::spawn` this way does feel possible, but it will require careful thought (and maybe some additional checks), which deserves its own PR. For now, it has the same performance characteristics of the current `Commands::spawn_bundle` on main.
**Note that 99% of this PR is simple renames and refactors. The only code that needs careful scrutiny is the new `World::spawn()` impl, which is relatively straightforward, but it has some new unsafe code (which re-uses battle tested BundlerSpawner code path).**
---
## Changelog
- All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input
- All instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api
- World and Commands now have `spawn_empty()`, which is equivalent to the old `spawn()` behavior.
## Migration Guide
```rust
// Old (0.8):
commands
.spawn()
.insert_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
commands.spawn_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
let entity = commands.spawn().id();
// New (0.9)
let entity = commands.spawn_empty().id();
// Old (0.8)
let entity = world.spawn().id();
// New (0.9)
let entity = world.spawn_empty();
```
2022-09-23 19:55:54 +00:00
|
|
|
commands.spawn(Camera2dBundle::default());
|
2022-06-06 17:52:09 +00:00
|
|
|
|
|
|
|
let font_handle = asset_server.load("fonts/FiraSans-Bold.ttf");
|
|
|
|
|
|
|
|
commands
|
2022-11-21 14:38:35 +00:00
|
|
|
.spawn(NodeBundle {
|
2022-06-06 17:52:09 +00:00
|
|
|
style: Style {
|
Flatten UI `Style` properties that use `Size` + remove `Size` (#8548)
# Objective
- Simplify API and make authoring styles easier
See:
https://github.com/bevyengine/bevy/issues/8540#issuecomment-1536177102
## Solution
- The `size`, `min_size`, `max_size`, and `gap` properties have been
replaced by `width`, `height`, `min_width`, `min_height`, `max_width`,
`max_height`, `row_gap`, and `column_gap` properties
---
## Changelog
- Flattened `Style` properties that have a `Size` value directly into
`Style`
## Migration Guide
- The `size`, `min_size`, `max_size`, and `gap` properties have been
replaced by the `width`, `height`, `min_width`, `min_height`,
`max_width`, `max_height`, `row_gap`, and `column_gap` properties. Use
the new properties instead.
---------
Co-authored-by: ickshonpe <david.curthoys@googlemail.com>
2023-05-16 01:36:32 +00:00
|
|
|
width: Val::Percent(100.0),
|
2023-09-19 15:14:46 +00:00
|
|
|
height: Val::Percent(100.0),
|
2022-06-06 17:52:09 +00:00
|
|
|
align_items: AlignItems::Center,
|
2023-02-11 23:07:16 +00:00
|
|
|
justify_content: JustifyContent::SpaceAround,
|
2022-06-07 02:16:47 +00:00
|
|
|
..default()
|
2022-06-06 17:52:09 +00:00
|
|
|
},
|
2022-06-07 02:16:47 +00:00
|
|
|
..default()
|
2022-06-06 17:52:09 +00:00
|
|
|
})
|
|
|
|
.with_children(|parent| {
|
2022-11-21 14:38:35 +00:00
|
|
|
parent
|
|
|
|
.spawn(ButtonBundle {
|
|
|
|
style: Style {
|
Flatten UI `Style` properties that use `Size` + remove `Size` (#8548)
# Objective
- Simplify API and make authoring styles easier
See:
https://github.com/bevyengine/bevy/issues/8540#issuecomment-1536177102
## Solution
- The `size`, `min_size`, `max_size`, and `gap` properties have been
replaced by `width`, `height`, `min_width`, `min_height`, `max_width`,
`max_height`, `row_gap`, and `column_gap` properties
---
## Changelog
- Flattened `Style` properties that have a `Size` value directly into
`Style`
## Migration Guide
- The `size`, `min_size`, `max_size`, and `gap` properties have been
replaced by the `width`, `height`, `min_width`, `min_height`,
`max_width`, `max_height`, `row_gap`, and `column_gap` properties. Use
the new properties instead.
---------
Co-authored-by: ickshonpe <david.curthoys@googlemail.com>
2023-05-16 01:36:32 +00:00
|
|
|
width: Val::Px(150.0),
|
|
|
|
height: Val::Px(65.0),
|
2022-11-21 14:38:35 +00:00
|
|
|
justify_content: JustifyContent::Center,
|
|
|
|
align_items: AlignItems::Center,
|
|
|
|
..default()
|
|
|
|
},
|
2024-02-24 21:35:32 +00:00
|
|
|
background_color: LegacyColor::rgb(0.1, 0.5, 0.1).into(),
|
2022-11-21 14:38:35 +00:00
|
|
|
..default()
|
|
|
|
})
|
|
|
|
.with_children(|parent| {
|
|
|
|
parent.spawn(TextBundle::from_section(
|
|
|
|
"Button 1",
|
|
|
|
TextStyle {
|
|
|
|
font: font_handle.clone(),
|
|
|
|
font_size: 40.0,
|
|
|
|
// Alpha channel of the color controls transparency.
|
2024-02-24 21:35:32 +00:00
|
|
|
color: LegacyColor::rgba(1.0, 1.0, 1.0, 0.2),
|
2022-11-21 14:38:35 +00:00
|
|
|
},
|
|
|
|
));
|
|
|
|
});
|
2022-06-06 17:52:09 +00:00
|
|
|
|
2022-11-21 14:38:35 +00:00
|
|
|
// Button with a different color,
|
|
|
|
// to demonstrate the text looks different due to its transparency.
|
|
|
|
parent
|
|
|
|
.spawn(ButtonBundle {
|
|
|
|
style: Style {
|
Flatten UI `Style` properties that use `Size` + remove `Size` (#8548)
# Objective
- Simplify API and make authoring styles easier
See:
https://github.com/bevyengine/bevy/issues/8540#issuecomment-1536177102
## Solution
- The `size`, `min_size`, `max_size`, and `gap` properties have been
replaced by `width`, `height`, `min_width`, `min_height`, `max_width`,
`max_height`, `row_gap`, and `column_gap` properties
---
## Changelog
- Flattened `Style` properties that have a `Size` value directly into
`Style`
## Migration Guide
- The `size`, `min_size`, `max_size`, and `gap` properties have been
replaced by the `width`, `height`, `min_width`, `min_height`,
`max_width`, `max_height`, `row_gap`, and `column_gap` properties. Use
the new properties instead.
---------
Co-authored-by: ickshonpe <david.curthoys@googlemail.com>
2023-05-16 01:36:32 +00:00
|
|
|
width: Val::Px(150.0),
|
|
|
|
height: Val::Px(65.0),
|
2022-11-21 14:38:35 +00:00
|
|
|
justify_content: JustifyContent::Center,
|
|
|
|
align_items: AlignItems::Center,
|
|
|
|
..default()
|
|
|
|
},
|
2024-02-24 21:35:32 +00:00
|
|
|
background_color: LegacyColor::rgb(0.5, 0.1, 0.5).into(),
|
2022-11-21 14:38:35 +00:00
|
|
|
..default()
|
|
|
|
})
|
|
|
|
.with_children(|parent| {
|
|
|
|
parent.spawn(TextBundle::from_section(
|
|
|
|
"Button 2",
|
|
|
|
TextStyle {
|
|
|
|
font: font_handle.clone(),
|
|
|
|
font_size: 40.0,
|
|
|
|
// Alpha channel of the color controls transparency.
|
2024-02-24 21:35:32 +00:00
|
|
|
color: LegacyColor::rgba(1.0, 1.0, 1.0, 0.2),
|
2022-11-21 14:38:35 +00:00
|
|
|
},
|
|
|
|
));
|
|
|
|
});
|
2022-06-06 17:52:09 +00:00
|
|
|
});
|
|
|
|
}
|