2022-12-25 00:23:13 +00:00
|
|
|
//! A simple glTF scene viewer made with Bevy.
|
|
|
|
//!
|
|
|
|
//! Just run `cargo run --release --example scene_viewer /path/to/model.gltf`,
|
|
|
|
//! replacing the path as appropriate.
|
|
|
|
//! In case of multiple scenes, you can select which to display by adapting the file path: `/path/to/model.gltf#Scene1`.
|
|
|
|
//! With no arguments it will load the `FlightHelmet` glTF model from the repository assets subdirectory.
|
|
|
|
|
|
|
|
use bevy::{
|
2023-05-16 01:26:11 +00:00
|
|
|
asset::ChangeWatcher,
|
2022-12-25 00:23:13 +00:00
|
|
|
math::Vec3A,
|
|
|
|
prelude::*,
|
|
|
|
render::primitives::{Aabb, Sphere},
|
2023-05-16 01:26:11 +00:00
|
|
|
utils::Duration,
|
2023-01-19 00:38:28 +00:00
|
|
|
window::WindowPlugin,
|
2022-12-25 00:23:13 +00:00
|
|
|
};
|
|
|
|
|
|
|
|
mod camera_controller_plugin;
|
|
|
|
mod scene_viewer_plugin;
|
|
|
|
|
|
|
|
use camera_controller_plugin::{CameraController, CameraControllerPlugin};
|
|
|
|
use scene_viewer_plugin::{SceneHandle, SceneViewerPlugin};
|
|
|
|
|
|
|
|
fn main() {
|
|
|
|
let mut app = App::new();
|
|
|
|
app.insert_resource(AmbientLight {
|
|
|
|
color: Color::WHITE,
|
|
|
|
brightness: 1.0 / 5.0f32,
|
|
|
|
})
|
|
|
|
.add_plugins(
|
|
|
|
DefaultPlugins
|
|
|
|
.set(WindowPlugin {
|
2023-01-19 00:38:28 +00:00
|
|
|
primary_window: Some(Window {
|
2022-12-25 00:23:13 +00:00
|
|
|
title: "bevy scene viewer".to_string(),
|
|
|
|
..default()
|
2023-01-19 00:38:28 +00:00
|
|
|
}),
|
2022-12-25 00:23:13 +00:00
|
|
|
..default()
|
|
|
|
})
|
|
|
|
.set(AssetPlugin {
|
|
|
|
asset_folder: std::env::var("CARGO_MANIFEST_DIR")
|
|
|
|
.unwrap_or_else(|_| ".".to_string()),
|
2023-05-16 01:26:11 +00:00
|
|
|
watch_for_changes: ChangeWatcher::with_delay(Duration::from_millis(200)),
|
2022-12-25 00:23:13 +00:00
|
|
|
}),
|
|
|
|
)
|
|
|
|
.add_plugin(CameraControllerPlugin)
|
|
|
|
.add_plugin(SceneViewerPlugin)
|
2023-03-18 01:45:34 +00:00
|
|
|
.add_systems(Startup, setup)
|
|
|
|
.add_systems(PreUpdate, setup_scene_after_load);
|
2022-12-25 00:23:13 +00:00
|
|
|
|
|
|
|
app.run();
|
|
|
|
}
|
|
|
|
|
|
|
|
fn parse_scene(scene_path: String) -> (String, usize) {
|
|
|
|
if scene_path.contains('#') {
|
|
|
|
let gltf_and_scene = scene_path.split('#').collect::<Vec<_>>();
|
|
|
|
if let Some((last, path)) = gltf_and_scene.split_last() {
|
|
|
|
if let Some(index) = last
|
|
|
|
.strip_prefix("Scene")
|
|
|
|
.and_then(|index| index.parse::<usize>().ok())
|
|
|
|
{
|
|
|
|
return (path.join("#"), index);
|
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|
|
|
|
(scene_path, 0)
|
|
|
|
}
|
|
|
|
|
|
|
|
fn setup(mut commands: Commands, asset_server: Res<AssetServer>) {
|
|
|
|
let scene_path = std::env::args()
|
|
|
|
.nth(1)
|
|
|
|
.unwrap_or_else(|| "assets/models/FlightHelmet/FlightHelmet.gltf".to_string());
|
|
|
|
info!("Loading {}", scene_path);
|
|
|
|
let (file_path, scene_index) = parse_scene(scene_path);
|
|
|
|
|
|
|
|
commands.insert_resource(SceneHandle::new(asset_server.load(file_path), scene_index));
|
|
|
|
}
|
|
|
|
|
|
|
|
fn setup_scene_after_load(
|
|
|
|
mut commands: Commands,
|
|
|
|
mut setup: Local<bool>,
|
|
|
|
mut scene_handle: ResMut<SceneHandle>,
|
EnvironmentMapLight, BRDF Improvements (#7051)
(Before)
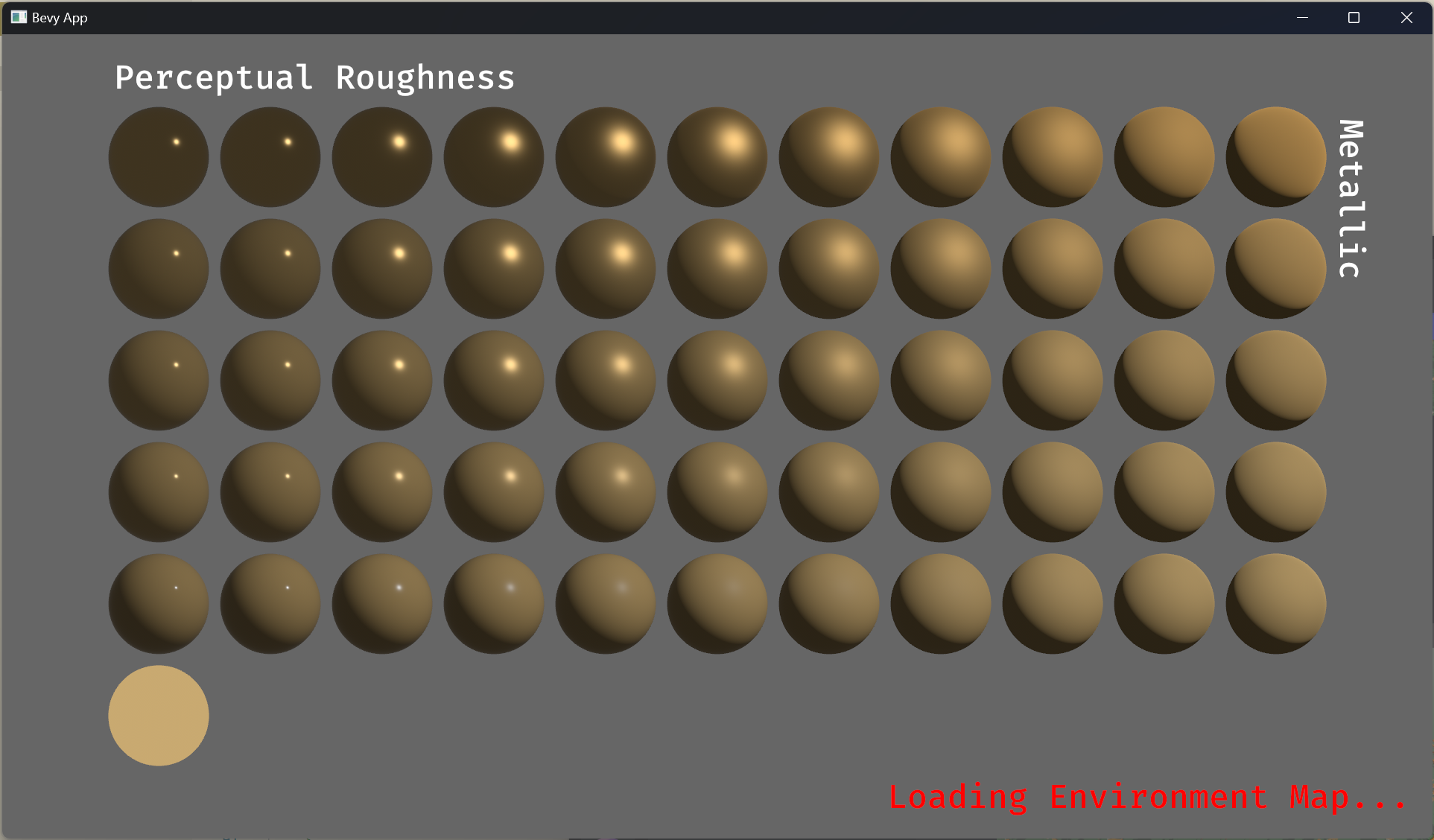
(After)
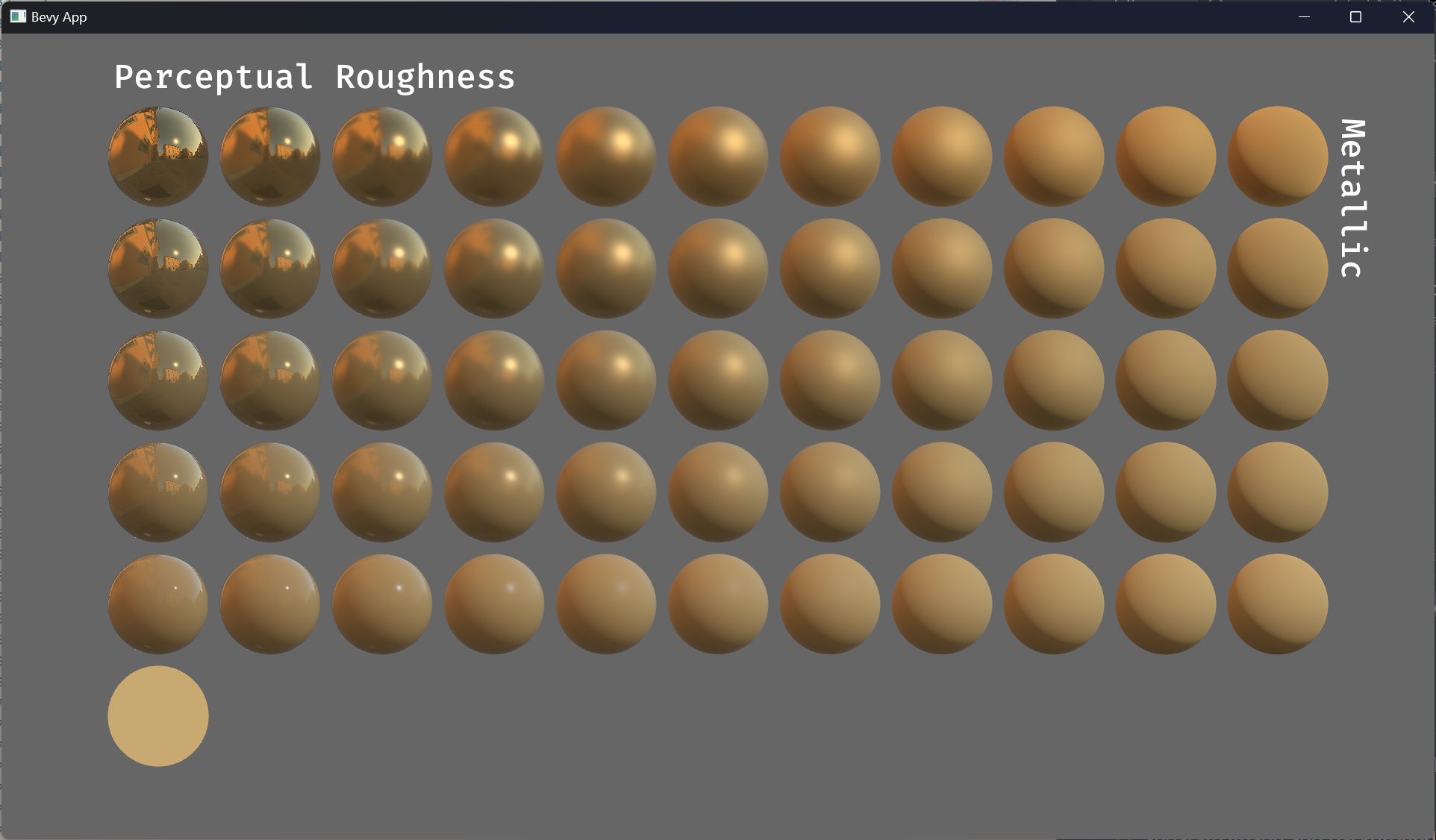
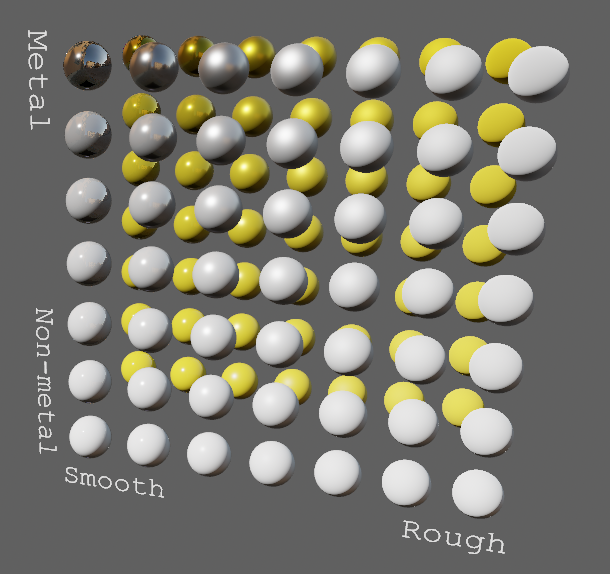
# Objective
- Improve lighting; especially reflections.
- Closes https://github.com/bevyengine/bevy/issues/4581.
## Solution
- Implement environment maps, providing better ambient light.
- Add microfacet multibounce approximation for specular highlights from Filament.
- Occlusion is no longer incorrectly applied to direct lighting. It now only applies to diffuse indirect light. Unsure if it's also supposed to apply to specular indirect light - the glTF specification just says "indirect light". In the case of ambient occlusion, for instance, that's usually only calculated as diffuse though. For now, I'm choosing to apply this just to indirect diffuse light, and not specular.
- Modified the PBR example to use an environment map, and have labels.
- Added `FallbackImageCubemap`.
## Implementation
- IBL technique references can be found in environment_map.wgsl.
- It's more accurate to use a LUT for the scale/bias. Filament has a good reference on generating this LUT. For now, I just used an analytic approximation.
- For now, environment maps must first be prefiltered outside of bevy using a 3rd party tool. See the `EnvironmentMap` documentation.
- Eventually, we should have our own prefiltering code, so that we can have dynamically changing environment maps, as well as let users drop in an HDR image and use asset preprocessing to create the needed textures using only bevy.
---
## Changelog
- Added an `EnvironmentMapLight` camera component that adds additional ambient light to a scene.
- StandardMaterials will now appear brighter and more saturated at high roughness, due to internal material changes. This is more physically correct.
- Fixed StandardMaterial occlusion being incorrectly applied to direct lighting.
- Added `FallbackImageCubemap`.
Co-authored-by: IceSentry <c.giguere42@gmail.com>
Co-authored-by: James Liu <contact@jamessliu.com>
Co-authored-by: Rob Parrett <robparrett@gmail.com>
2023-02-09 16:46:32 +00:00
|
|
|
asset_server: Res<AssetServer>,
|
2022-12-25 00:23:13 +00:00
|
|
|
meshes: Query<(&GlobalTransform, Option<&Aabb>), With<Handle<Mesh>>>,
|
|
|
|
) {
|
|
|
|
if scene_handle.is_loaded && !*setup {
|
|
|
|
*setup = true;
|
|
|
|
// Find an approximate bounding box of the scene from its meshes
|
|
|
|
if meshes.iter().any(|(_, maybe_aabb)| maybe_aabb.is_none()) {
|
|
|
|
return;
|
|
|
|
}
|
|
|
|
|
|
|
|
let mut min = Vec3A::splat(f32::MAX);
|
|
|
|
let mut max = Vec3A::splat(f32::MIN);
|
|
|
|
for (transform, maybe_aabb) in &meshes {
|
|
|
|
let aabb = maybe_aabb.unwrap();
|
|
|
|
// If the Aabb had not been rotated, applying the non-uniform scale would produce the
|
|
|
|
// correct bounds. However, it could very well be rotated and so we first convert to
|
|
|
|
// a Sphere, and then back to an Aabb to find the conservative min and max points.
|
|
|
|
let sphere = Sphere {
|
|
|
|
center: Vec3A::from(transform.transform_point(Vec3::from(aabb.center))),
|
|
|
|
radius: transform.radius_vec3a(aabb.half_extents),
|
|
|
|
};
|
|
|
|
let aabb = Aabb::from(sphere);
|
|
|
|
min = min.min(aabb.min());
|
|
|
|
max = max.max(aabb.max());
|
|
|
|
}
|
|
|
|
|
|
|
|
let size = (max - min).length();
|
|
|
|
let aabb = Aabb::from_min_max(Vec3::from(min), Vec3::from(max));
|
|
|
|
|
|
|
|
info!("Spawning a controllable 3D perspective camera");
|
|
|
|
let mut projection = PerspectiveProjection::default();
|
|
|
|
projection.far = projection.far.max(size * 10.0);
|
|
|
|
|
|
|
|
let camera_controller = CameraController::default();
|
|
|
|
|
|
|
|
// Display the controls of the scene viewer
|
|
|
|
info!("{}", camera_controller);
|
|
|
|
info!("{}", *scene_handle);
|
|
|
|
|
|
|
|
commands.spawn((
|
|
|
|
Camera3dBundle {
|
|
|
|
projection: projection.into(),
|
|
|
|
transform: Transform::from_translation(
|
|
|
|
Vec3::from(aabb.center) + size * Vec3::new(0.5, 0.25, 0.5),
|
|
|
|
)
|
|
|
|
.looking_at(Vec3::from(aabb.center), Vec3::Y),
|
|
|
|
camera: Camera {
|
|
|
|
is_active: false,
|
|
|
|
..default()
|
|
|
|
},
|
|
|
|
..default()
|
|
|
|
},
|
EnvironmentMapLight, BRDF Improvements (#7051)
(Before)
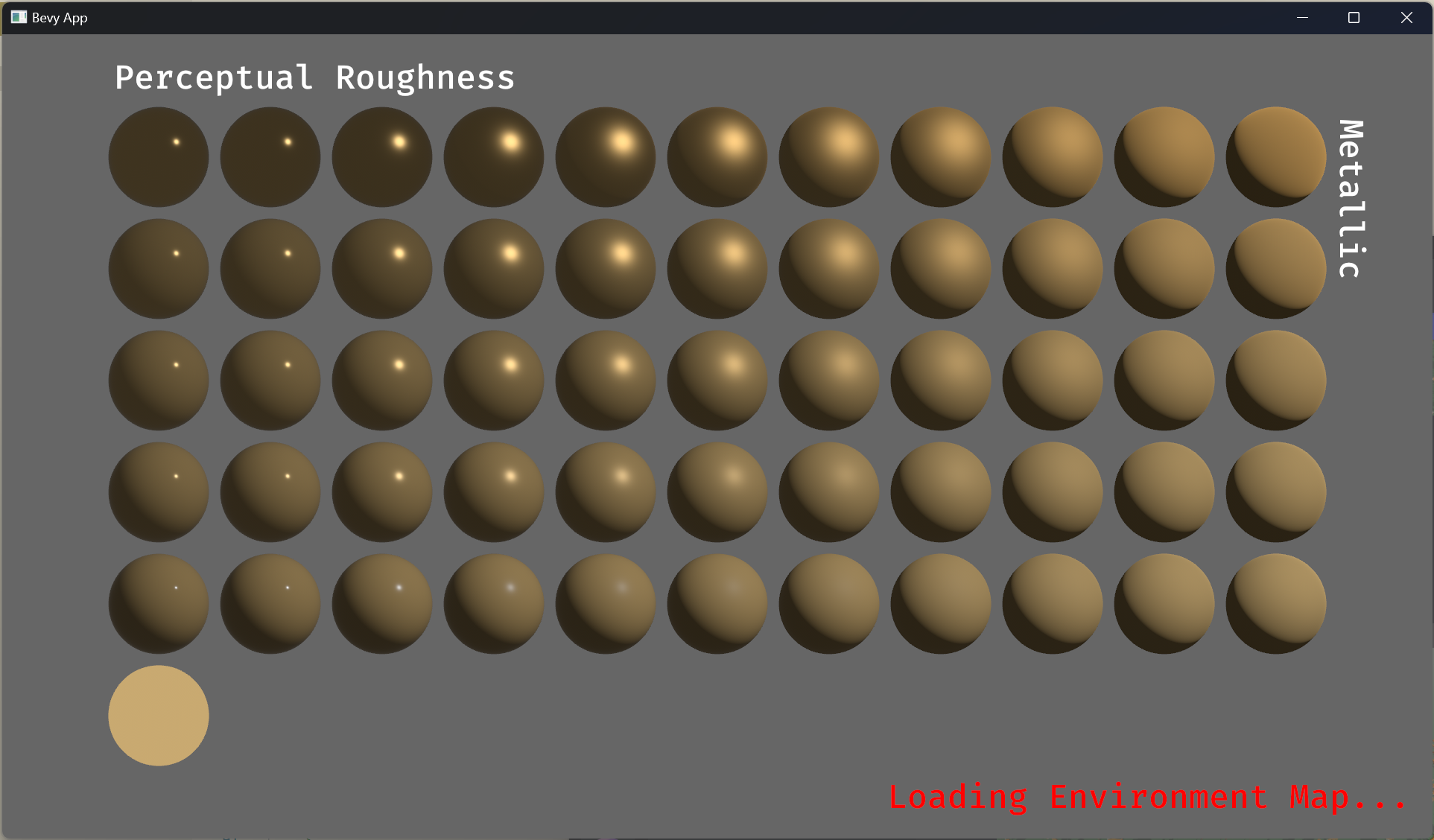
(After)
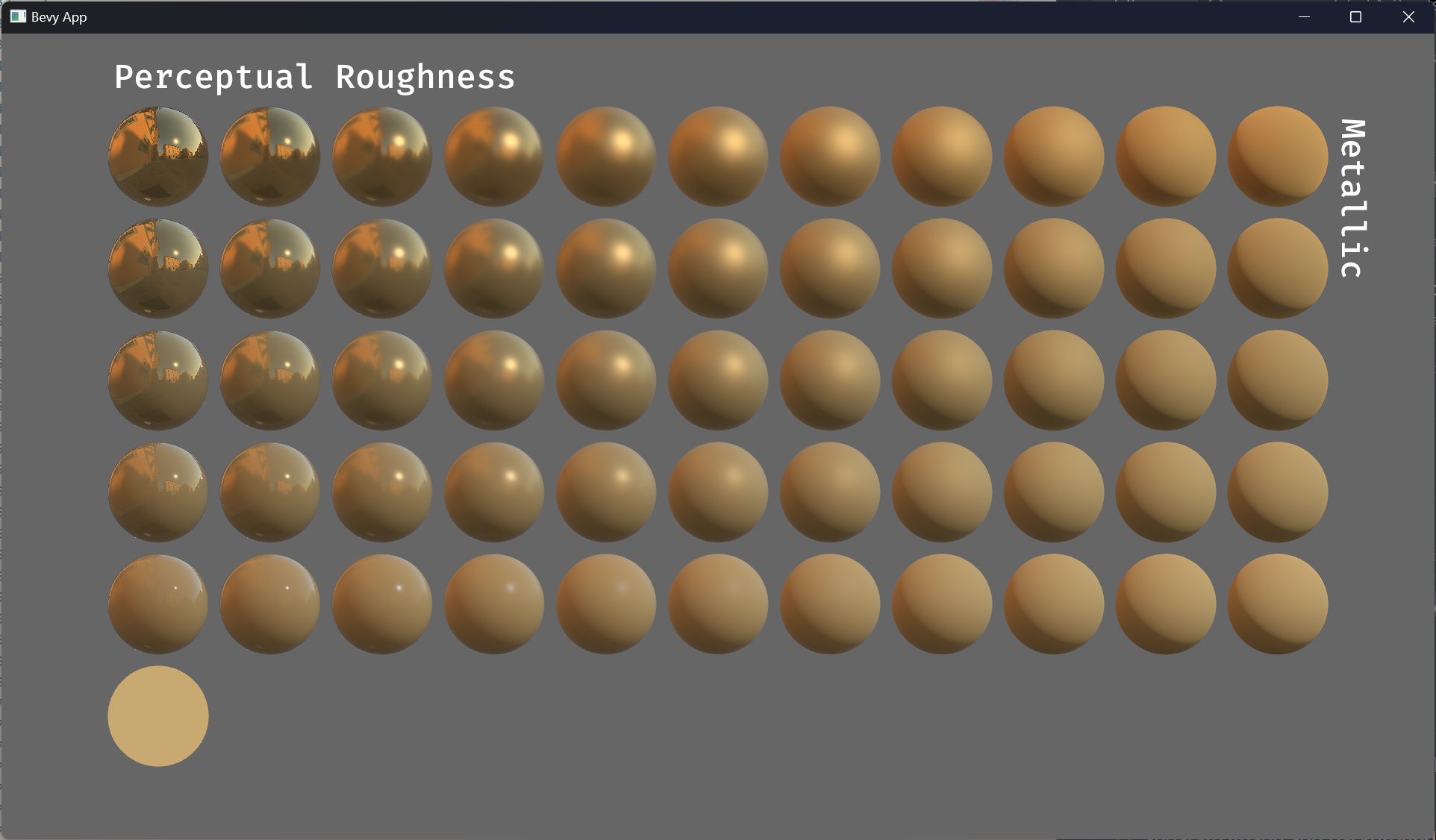
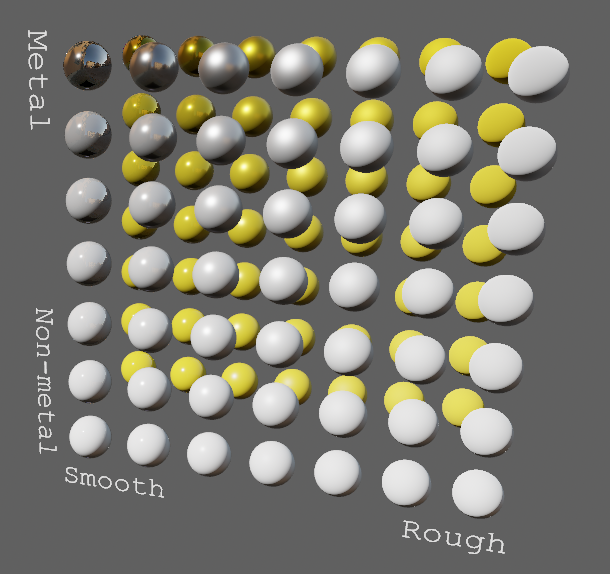
# Objective
- Improve lighting; especially reflections.
- Closes https://github.com/bevyengine/bevy/issues/4581.
## Solution
- Implement environment maps, providing better ambient light.
- Add microfacet multibounce approximation for specular highlights from Filament.
- Occlusion is no longer incorrectly applied to direct lighting. It now only applies to diffuse indirect light. Unsure if it's also supposed to apply to specular indirect light - the glTF specification just says "indirect light". In the case of ambient occlusion, for instance, that's usually only calculated as diffuse though. For now, I'm choosing to apply this just to indirect diffuse light, and not specular.
- Modified the PBR example to use an environment map, and have labels.
- Added `FallbackImageCubemap`.
## Implementation
- IBL technique references can be found in environment_map.wgsl.
- It's more accurate to use a LUT for the scale/bias. Filament has a good reference on generating this LUT. For now, I just used an analytic approximation.
- For now, environment maps must first be prefiltered outside of bevy using a 3rd party tool. See the `EnvironmentMap` documentation.
- Eventually, we should have our own prefiltering code, so that we can have dynamically changing environment maps, as well as let users drop in an HDR image and use asset preprocessing to create the needed textures using only bevy.
---
## Changelog
- Added an `EnvironmentMapLight` camera component that adds additional ambient light to a scene.
- StandardMaterials will now appear brighter and more saturated at high roughness, due to internal material changes. This is more physically correct.
- Fixed StandardMaterial occlusion being incorrectly applied to direct lighting.
- Added `FallbackImageCubemap`.
Co-authored-by: IceSentry <c.giguere42@gmail.com>
Co-authored-by: James Liu <contact@jamessliu.com>
Co-authored-by: Rob Parrett <robparrett@gmail.com>
2023-02-09 16:46:32 +00:00
|
|
|
EnvironmentMapLight {
|
|
|
|
diffuse_map: asset_server
|
|
|
|
.load("assets/environment_maps/pisa_diffuse_rgb9e5_zstd.ktx2"),
|
|
|
|
specular_map: asset_server
|
|
|
|
.load("assets/environment_maps/pisa_specular_rgb9e5_zstd.ktx2"),
|
|
|
|
},
|
2022-12-25 00:23:13 +00:00
|
|
|
camera_controller,
|
|
|
|
));
|
|
|
|
|
|
|
|
// Spawn a default light if the scene does not have one
|
|
|
|
if !scene_handle.has_light {
|
|
|
|
info!("Spawning a directional light");
|
|
|
|
commands.spawn(DirectionalLightBundle {
|
|
|
|
directional_light: DirectionalLight {
|
|
|
|
shadows_enabled: false,
|
|
|
|
..default()
|
|
|
|
},
|
|
|
|
..default()
|
|
|
|
});
|
|
|
|
|
|
|
|
scene_handle.has_light = true;
|
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|