2022-07-05 18:44:54 +00:00
# B0003
As commands are executed asynchronously, it is possible to issue a command on an entity that will no longer exist at the time of the command execution.
Erroneous code example:
```rust,should_panic
use bevy::prelude::*;
fn main() {
App::new()
.add_plugins(DefaultPlugins)
2023-03-18 01:45:34 +00:00
.add_systems(Startup, setup)
.add_systems(Update, despawning)
.add_systems(Update, use_entity.after(despawning))
2022-07-05 18:44:54 +00:00
.run();
}
Make `Resource` trait opt-in, requiring `#[derive(Resource)]` V2 (#5577)
*This PR description is an edited copy of #5007, written by @alice-i-cecile.*
# Objective
Follow-up to https://github.com/bevyengine/bevy/pull/2254. The `Resource` trait currently has a blanket implementation for all types that meet its bounds.
While ergonomic, this results in several drawbacks:
* it is possible to make confusing, silent mistakes such as inserting a function pointer (Foo) rather than a value (Foo::Bar) as a resource
* it is challenging to discover if a type is intended to be used as a resource
* we cannot later add customization options (see the [RFC](https://github.com/bevyengine/rfcs/blob/main/rfcs/27-derive-component.md) for the equivalent choice for Component).
* dependencies can use the same Rust type as a resource in invisibly conflicting ways
* raw Rust types used as resources cannot preserve privacy appropriately, as anyone able to access that type can read and write to internal values
* we cannot capture a definitive list of possible resources to display to users in an editor
## Notes to reviewers
* Review this commit-by-commit; there's effectively no back-tracking and there's a lot of churn in some of these commits.
*ira: My commits are not as well organized :')*
* I've relaxed the bound on Local to Send + Sync + 'static: I don't think these concerns apply there, so this can keep things simple. Storing e.g. a u32 in a Local is fine, because there's a variable name attached explaining what it does.
* I think this is a bad place for the Resource trait to live, but I've left it in place to make reviewing easier. IMO that's best tackled with https://github.com/bevyengine/bevy/issues/4981.
## Changelog
`Resource` is no longer automatically implemented for all matching types. Instead, use the new `#[derive(Resource)]` macro.
## Migration Guide
Add `#[derive(Resource)]` to all types you are using as a resource.
If you are using a third party type as a resource, wrap it in a tuple struct to bypass orphan rules. Consider deriving `Deref` and `DerefMut` to improve ergonomics.
`ClearColor` no longer implements `Component`. Using `ClearColor` as a component in 0.8 did nothing.
Use the `ClearColorConfig` in the `Camera3d` and `Camera2d` components instead.
Co-authored-by: Alice <alice.i.cecile@gmail.com>
Co-authored-by: Alice Cecile <alice.i.cecile@gmail.com>
Co-authored-by: devil-ira <justthecooldude@gmail.com>
Co-authored-by: Carter Anderson <mcanders1@gmail.com>
2022-08-08 21:36:35 +00:00
#[derive(Resource)]
2022-07-05 18:44:54 +00:00
struct MyEntity(Entity);
#[derive(Component)]
struct Hello;
fn setup(mut commands: Commands) {
Spawn now takes a Bundle (#6054)
# Objective
Now that we can consolidate Bundles and Components under a single insert (thanks to #2975 and #6039), almost 100% of world spawns now look like `world.spawn().insert((Some, Tuple, Here))`. Spawning an entity without any components is an extremely uncommon pattern, so it makes sense to give spawn the "first class" ergonomic api. This consolidated api should be made consistent across all spawn apis (such as World and Commands).
## Solution
All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input:
```rust
// before:
commands
.spawn()
.insert((A, B, C));
world
.spawn()
.insert((A, B, C);
// after
commands.spawn((A, B, C));
world.spawn((A, B, C));
```
All existing instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api. A new `spawn_empty` has been added, replacing the old `spawn` api.
By allowing `world.spawn(some_bundle)` to replace `world.spawn().insert(some_bundle)`, this opened the door to removing the initial entity allocation in the "empty" archetype / table done in `spawn()` (and subsequent move to the actual archetype in `.insert(some_bundle)`).
This improves spawn performance by over 10%:
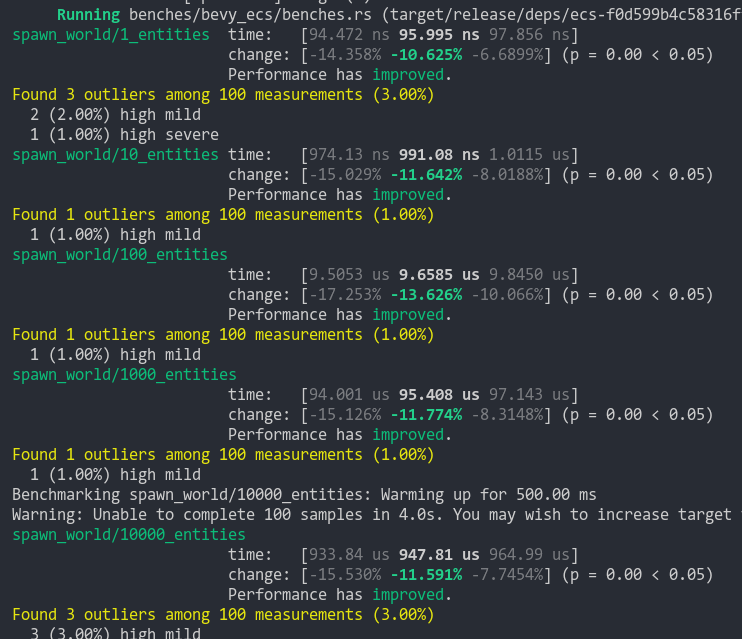
To take this measurement, I added a new `world_spawn` benchmark.
Unfortunately, optimizing `Commands::spawn` is slightly less trivial, as Commands expose the Entity id of spawned entities prior to actually spawning. Doing the optimization would (naively) require assurances that the `spawn(some_bundle)` command is applied before all other commands involving the entity (which would not necessarily be true, if memory serves). Optimizing `Commands::spawn` this way does feel possible, but it will require careful thought (and maybe some additional checks), which deserves its own PR. For now, it has the same performance characteristics of the current `Commands::spawn_bundle` on main.
**Note that 99% of this PR is simple renames and refactors. The only code that needs careful scrutiny is the new `World::spawn()` impl, which is relatively straightforward, but it has some new unsafe code (which re-uses battle tested BundlerSpawner code path).**
---
## Changelog
- All `spawn` apis (`World::spawn`, `Commands:;spawn`, `ChildBuilder::spawn`, and `WorldChildBuilder::spawn`) now accept a bundle as input
- All instances of `spawn_bundle` have been deprecated in favor of the new `spawn` api
- World and Commands now have `spawn_empty()`, which is equivalent to the old `spawn()` behavior.
## Migration Guide
```rust
// Old (0.8):
commands
.spawn()
.insert_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
commands.spawn_bundle((A, B, C));
// New (0.9)
commands.spawn((A, B, C));
// Old (0.8):
let entity = commands.spawn().id();
// New (0.9)
let entity = commands.spawn_empty().id();
// Old (0.8)
let entity = world.spawn().id();
// New (0.9)
let entity = world.spawn_empty();
```
2022-09-23 19:55:54 +00:00
let entity = commands.spawn_empty().id();
2022-07-05 18:44:54 +00:00
commands.insert_resource(MyEntity(entity));
}
fn despawning(mut commands: Commands, entity: Option< Res < MyEntity > >) {
if let Some(my_entity) = entity {
commands.entity(my_entity.0).despawn();
commands.remove_resource::< MyEntity > ();
}
}
fn use_entity(mut commands: Commands, entity: Option< Res < MyEntity > >) {
if let Some(my_entity) = entity {
commands.entity(my_entity.0).insert(Hello);
}
}
```
This will panic, as system `use_entity` is executed after system `despawning` . Without the system ordering specified here, the ordering would be random and this code would panic half the time.
The default panic message is telling you the entity id (`0v0`) and the command that failed (adding a component `Hello` ):
```text
thread 'main' panicked at 'error[B0003]: Could not add a component (of type `use_entity_after_despawn::Hello` ) to entity 0v0 because it doesn't exist in this World.', /bevy/crates/bevy_ecs/src/system/commands/mod.rs:752:13
```
But you don't know which system tried to add a component, and which system despawned the entity.
2023-01-27 12:12:53 +00:00
To get the system that created the command that panics, you can enable the `trace` feature of Bevy. This will add a panic handler that will print more information:
2022-07-05 18:44:54 +00:00
```text
0: bevy_ecs::schedule::stage::system_commands
with name="use_entity_after_despawn::use_entity"
at crates/bevy_ecs/src/schedule/stage.rs:880
1: bevy_ecs::schedule::stage
with name=Update
at crates/bevy_ecs/src/schedule/mod.rs:337
2: bevy_app::app::frame
at crates/bevy_app/src/app.rs:113
3: bevy_app::app::bevy_app
at crates/bevy_app/src/app.rs:126
thread 'main' panicked at 'error[B0003]: Could not add a component (of type `use_entity_after_despawn::Hello` ) to entity 0v0 because it doesn't exist in this World.', /bevy/crates/bevy_ecs/src/system/commands/mod.rs:752:13
```
From the first two lines, you now know that it panics while executing a command from the system `use_entity` .
To get the system that created the despawn command, you can enable DEBUG logs for crate `bevy_ecs` , for example by setting the environment variable `RUST_LOG=bevy_ecs=debug` . This will log:
```text
DEBUG stage{name=Update}:system_commands{name="use_entity_after_despawn::despawning"}: bevy_ecs::world: Despawning entity 0v0
thread 'main' panicked at 'error[B0003]: Could not add a component (of type `use_entity_after_despawn::Hello` ) to entity 0v0 because it doesn't exist in this World.', /bevy/crates/bevy_ecs/src/system/commands/mod.rs:752:13
```
From the first line, you know the entity `0v0` was despawned when executing a command from system `despawning` . In a real case, you could have many log lines, you will need to search for the exact entity from the panic message.
2023-01-27 12:12:53 +00:00
Combining those two, you should get enough information to understand why this panic is happening and how to fix it:
2022-07-05 18:44:54 +00:00
```text
DEBUG stage{name=Update}:system_commands{name="use_entity_after_despawn::despawning"}: bevy_ecs::world: Despawning entity 0v0
0: bevy_ecs::schedule::stage::system_commands
with name="use_entity_after_despawn::use_entity"
at crates/bevy_ecs/src/schedule/stage.rs:880
1: bevy_ecs::schedule::stage
with name=Update
at crates/bevy_ecs/src/schedule/mod.rs:337
thread 'main' panicked at 'error[B0003]: Could not add a component (of type `use_entity_after_despawn::Hello` ) to entity 0v0 because it doesn't exist in this World.', /bevy/crates/bevy_ecs/src/system/commands/mod.rs:752:13
```